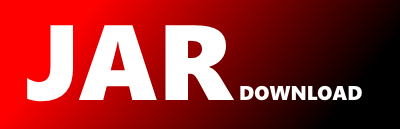
com.google.api.services.testing.model.AndroidModel Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-10-08 17:45:39 UTC)
* on 2019-04-09 at 00:25:22 UTC
* Modify at your own risk.
*/
package com.google.api.services.testing.model;
/**
* A description of an Android device tests may be run on.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Testing API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AndroidModel extends com.google.api.client.json.GenericJson {
/**
* The company that this device is branded with. Example: "Google", "Samsung".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String brand;
/**
* The name of the industrial design. This corresponds to android.os.Build.DEVICE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String codename;
/**
* Whether this device is virtual or physical.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String form;
/**
* Whether this device is a phone, tablet, wearable, etc.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String formFactor;
/**
* The unique opaque id for this model. Use this for invoking the TestExecutionService.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* True if and only if tests with this model are recorded by stitching together screenshots. See
* use_low_spec_video_recording in device config.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean lowFpsVideoRecording;
/**
* The manufacturer of this device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String manufacturer;
/**
* The human-readable marketing name for this device model. Examples: "Nexus 5", "Galaxy S5".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Screen density in DPI. This corresponds to ro.sf.lcd_density
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer screenDensity;
/**
* Screen size in the horizontal (X) dimension measured in pixels.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer screenX;
/**
* Screen size in the vertical (Y) dimension measured in pixels.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer screenY;
/**
* The list of supported ABIs for this device. This corresponds to either
* android.os.Build.SUPPORTED_ABIS (for API level 21 and above) or
* android.os.Build.CPU_ABI/CPU_ABI2. The most preferred ABI is the first element in the list.
*
* Elements are optionally prefixed by "version_id:" (where version_id is the id of an
* AndroidVersion), denoting an ABI that is supported only on a particular version.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List supportedAbis;
/**
* The set of Android versions this device supports.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List supportedVersionIds;
/**
* Tags for this dimension. Examples: "default", "preview", "deprecated".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tags;
/**
* The company that this device is branded with. Example: "Google", "Samsung".
* @return value or {@code null} for none
*/
public java.lang.String getBrand() {
return brand;
}
/**
* The company that this device is branded with. Example: "Google", "Samsung".
* @param brand brand or {@code null} for none
*/
public AndroidModel setBrand(java.lang.String brand) {
this.brand = brand;
return this;
}
/**
* The name of the industrial design. This corresponds to android.os.Build.DEVICE.
* @return value or {@code null} for none
*/
public java.lang.String getCodename() {
return codename;
}
/**
* The name of the industrial design. This corresponds to android.os.Build.DEVICE.
* @param codename codename or {@code null} for none
*/
public AndroidModel setCodename(java.lang.String codename) {
this.codename = codename;
return this;
}
/**
* Whether this device is virtual or physical.
* @return value or {@code null} for none
*/
public java.lang.String getForm() {
return form;
}
/**
* Whether this device is virtual or physical.
* @param form form or {@code null} for none
*/
public AndroidModel setForm(java.lang.String form) {
this.form = form;
return this;
}
/**
* Whether this device is a phone, tablet, wearable, etc.
* @return value or {@code null} for none
*/
public java.lang.String getFormFactor() {
return formFactor;
}
/**
* Whether this device is a phone, tablet, wearable, etc.
* @param formFactor formFactor or {@code null} for none
*/
public AndroidModel setFormFactor(java.lang.String formFactor) {
this.formFactor = formFactor;
return this;
}
/**
* The unique opaque id for this model. Use this for invoking the TestExecutionService.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The unique opaque id for this model. Use this for invoking the TestExecutionService.
* @param id id or {@code null} for none
*/
public AndroidModel setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* True if and only if tests with this model are recorded by stitching together screenshots. See
* use_low_spec_video_recording in device config.
* @return value or {@code null} for none
*/
public java.lang.Boolean getLowFpsVideoRecording() {
return lowFpsVideoRecording;
}
/**
* True if and only if tests with this model are recorded by stitching together screenshots. See
* use_low_spec_video_recording in device config.
* @param lowFpsVideoRecording lowFpsVideoRecording or {@code null} for none
*/
public AndroidModel setLowFpsVideoRecording(java.lang.Boolean lowFpsVideoRecording) {
this.lowFpsVideoRecording = lowFpsVideoRecording;
return this;
}
/**
* The manufacturer of this device.
* @return value or {@code null} for none
*/
public java.lang.String getManufacturer() {
return manufacturer;
}
/**
* The manufacturer of this device.
* @param manufacturer manufacturer or {@code null} for none
*/
public AndroidModel setManufacturer(java.lang.String manufacturer) {
this.manufacturer = manufacturer;
return this;
}
/**
* The human-readable marketing name for this device model. Examples: "Nexus 5", "Galaxy S5".
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The human-readable marketing name for this device model. Examples: "Nexus 5", "Galaxy S5".
* @param name name or {@code null} for none
*/
public AndroidModel setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Screen density in DPI. This corresponds to ro.sf.lcd_density
* @return value or {@code null} for none
*/
public java.lang.Integer getScreenDensity() {
return screenDensity;
}
/**
* Screen density in DPI. This corresponds to ro.sf.lcd_density
* @param screenDensity screenDensity or {@code null} for none
*/
public AndroidModel setScreenDensity(java.lang.Integer screenDensity) {
this.screenDensity = screenDensity;
return this;
}
/**
* Screen size in the horizontal (X) dimension measured in pixels.
* @return value or {@code null} for none
*/
public java.lang.Integer getScreenX() {
return screenX;
}
/**
* Screen size in the horizontal (X) dimension measured in pixels.
* @param screenX screenX or {@code null} for none
*/
public AndroidModel setScreenX(java.lang.Integer screenX) {
this.screenX = screenX;
return this;
}
/**
* Screen size in the vertical (Y) dimension measured in pixels.
* @return value or {@code null} for none
*/
public java.lang.Integer getScreenY() {
return screenY;
}
/**
* Screen size in the vertical (Y) dimension measured in pixels.
* @param screenY screenY or {@code null} for none
*/
public AndroidModel setScreenY(java.lang.Integer screenY) {
this.screenY = screenY;
return this;
}
/**
* The list of supported ABIs for this device. This corresponds to either
* android.os.Build.SUPPORTED_ABIS (for API level 21 and above) or
* android.os.Build.CPU_ABI/CPU_ABI2. The most preferred ABI is the first element in the list.
*
* Elements are optionally prefixed by "version_id:" (where version_id is the id of an
* AndroidVersion), denoting an ABI that is supported only on a particular version.
* @return value or {@code null} for none
*/
public java.util.List getSupportedAbis() {
return supportedAbis;
}
/**
* The list of supported ABIs for this device. This corresponds to either
* android.os.Build.SUPPORTED_ABIS (for API level 21 and above) or
* android.os.Build.CPU_ABI/CPU_ABI2. The most preferred ABI is the first element in the list.
*
* Elements are optionally prefixed by "version_id:" (where version_id is the id of an
* AndroidVersion), denoting an ABI that is supported only on a particular version.
* @param supportedAbis supportedAbis or {@code null} for none
*/
public AndroidModel setSupportedAbis(java.util.List supportedAbis) {
this.supportedAbis = supportedAbis;
return this;
}
/**
* The set of Android versions this device supports.
* @return value or {@code null} for none
*/
public java.util.List getSupportedVersionIds() {
return supportedVersionIds;
}
/**
* The set of Android versions this device supports.
* @param supportedVersionIds supportedVersionIds or {@code null} for none
*/
public AndroidModel setSupportedVersionIds(java.util.List supportedVersionIds) {
this.supportedVersionIds = supportedVersionIds;
return this;
}
/**
* Tags for this dimension. Examples: "default", "preview", "deprecated".
* @return value or {@code null} for none
*/
public java.util.List getTags() {
return tags;
}
/**
* Tags for this dimension. Examples: "default", "preview", "deprecated".
* @param tags tags or {@code null} for none
*/
public AndroidModel setTags(java.util.List tags) {
this.tags = tags;
return this;
}
@Override
public AndroidModel set(String fieldName, Object value) {
return (AndroidModel) super.set(fieldName, value);
}
@Override
public AndroidModel clone() {
return (AndroidModel) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy