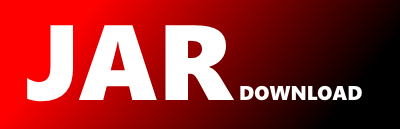
com.google.api.services.texttospeech.v1.model.AudioConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.texttospeech.v1.model;
/**
* Description of audio data to be synthesized.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Text-to-Speech API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AudioConfig extends com.google.api.client.json.GenericJson {
/**
* Required. The format of the audio byte stream.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String audioEncoding;
/**
* Optional. Input only. An identifier which selects 'audio effects' profiles that are applied on
* (post synthesized) text to speech. Effects are applied on top of each other in the order they
* are given. See [audio profiles](https://cloud.google.com/text-to-speech/docs/audio-profiles)
* for current supported profile ids.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List effectsProfileId;
/**
* Optional. Input only. Speaking pitch, in the range [-20.0, 20.0]. 20 means increase 20
* semitones from the original pitch. -20 means decrease 20 semitones from the original pitch.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double pitch;
/**
* Optional. The synthesis sample rate (in hertz) for this audio. When this is specified in
* SynthesizeSpeechRequest, if this is different from the voice's natural sample rate, then the
* synthesizer will honor this request by converting to the desired sample rate (which might
* result in worse audio quality), unless the specified sample rate is not supported for the
* encoding chosen, in which case it will fail the request and return
* google.rpc.Code.INVALID_ARGUMENT.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer sampleRateHertz;
/**
* Optional. Input only. Speaking rate/speed, in the range [0.25, 4.0]. 1.0 is the normal native
* speed supported by the specific voice. 2.0 is twice as fast, and 0.5 is half as fast. If
* unset(0.0), defaults to the native 1.0 speed. Any other values < 0.25 or > 4.0 will return an
* error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double speakingRate;
/**
* Optional. Input only. Volume gain (in dB) of the normal native volume supported by the specific
* voice, in the range [-96.0, 16.0]. If unset, or set to a value of 0.0 (dB), will play at normal
* native signal amplitude. A value of -6.0 (dB) will play at approximately half the amplitude of
* the normal native signal amplitude. A value of +6.0 (dB) will play at approximately twice the
* amplitude of the normal native signal amplitude. Strongly recommend not to exceed +10 (dB) as
* there's usually no effective increase in loudness for any value greater than that.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double volumeGainDb;
/**
* Required. The format of the audio byte stream.
* @return value or {@code null} for none
*/
public java.lang.String getAudioEncoding() {
return audioEncoding;
}
/**
* Required. The format of the audio byte stream.
* @param audioEncoding audioEncoding or {@code null} for none
*/
public AudioConfig setAudioEncoding(java.lang.String audioEncoding) {
this.audioEncoding = audioEncoding;
return this;
}
/**
* Optional. Input only. An identifier which selects 'audio effects' profiles that are applied on
* (post synthesized) text to speech. Effects are applied on top of each other in the order they
* are given. See [audio profiles](https://cloud.google.com/text-to-speech/docs/audio-profiles)
* for current supported profile ids.
* @return value or {@code null} for none
*/
public java.util.List getEffectsProfileId() {
return effectsProfileId;
}
/**
* Optional. Input only. An identifier which selects 'audio effects' profiles that are applied on
* (post synthesized) text to speech. Effects are applied on top of each other in the order they
* are given. See [audio profiles](https://cloud.google.com/text-to-speech/docs/audio-profiles)
* for current supported profile ids.
* @param effectsProfileId effectsProfileId or {@code null} for none
*/
public AudioConfig setEffectsProfileId(java.util.List effectsProfileId) {
this.effectsProfileId = effectsProfileId;
return this;
}
/**
* Optional. Input only. Speaking pitch, in the range [-20.0, 20.0]. 20 means increase 20
* semitones from the original pitch. -20 means decrease 20 semitones from the original pitch.
* @return value or {@code null} for none
*/
public java.lang.Double getPitch() {
return pitch;
}
/**
* Optional. Input only. Speaking pitch, in the range [-20.0, 20.0]. 20 means increase 20
* semitones from the original pitch. -20 means decrease 20 semitones from the original pitch.
* @param pitch pitch or {@code null} for none
*/
public AudioConfig setPitch(java.lang.Double pitch) {
this.pitch = pitch;
return this;
}
/**
* Optional. The synthesis sample rate (in hertz) for this audio. When this is specified in
* SynthesizeSpeechRequest, if this is different from the voice's natural sample rate, then the
* synthesizer will honor this request by converting to the desired sample rate (which might
* result in worse audio quality), unless the specified sample rate is not supported for the
* encoding chosen, in which case it will fail the request and return
* google.rpc.Code.INVALID_ARGUMENT.
* @return value or {@code null} for none
*/
public java.lang.Integer getSampleRateHertz() {
return sampleRateHertz;
}
/**
* Optional. The synthesis sample rate (in hertz) for this audio. When this is specified in
* SynthesizeSpeechRequest, if this is different from the voice's natural sample rate, then the
* synthesizer will honor this request by converting to the desired sample rate (which might
* result in worse audio quality), unless the specified sample rate is not supported for the
* encoding chosen, in which case it will fail the request and return
* google.rpc.Code.INVALID_ARGUMENT.
* @param sampleRateHertz sampleRateHertz or {@code null} for none
*/
public AudioConfig setSampleRateHertz(java.lang.Integer sampleRateHertz) {
this.sampleRateHertz = sampleRateHertz;
return this;
}
/**
* Optional. Input only. Speaking rate/speed, in the range [0.25, 4.0]. 1.0 is the normal native
* speed supported by the specific voice. 2.0 is twice as fast, and 0.5 is half as fast. If
* unset(0.0), defaults to the native 1.0 speed. Any other values < 0.25 or > 4.0 will return an
* error.
* @return value or {@code null} for none
*/
public java.lang.Double getSpeakingRate() {
return speakingRate;
}
/**
* Optional. Input only. Speaking rate/speed, in the range [0.25, 4.0]. 1.0 is the normal native
* speed supported by the specific voice. 2.0 is twice as fast, and 0.5 is half as fast. If
* unset(0.0), defaults to the native 1.0 speed. Any other values < 0.25 or > 4.0 will return an
* error.
* @param speakingRate speakingRate or {@code null} for none
*/
public AudioConfig setSpeakingRate(java.lang.Double speakingRate) {
this.speakingRate = speakingRate;
return this;
}
/**
* Optional. Input only. Volume gain (in dB) of the normal native volume supported by the specific
* voice, in the range [-96.0, 16.0]. If unset, or set to a value of 0.0 (dB), will play at normal
* native signal amplitude. A value of -6.0 (dB) will play at approximately half the amplitude of
* the normal native signal amplitude. A value of +6.0 (dB) will play at approximately twice the
* amplitude of the normal native signal amplitude. Strongly recommend not to exceed +10 (dB) as
* there's usually no effective increase in loudness for any value greater than that.
* @return value or {@code null} for none
*/
public java.lang.Double getVolumeGainDb() {
return volumeGainDb;
}
/**
* Optional. Input only. Volume gain (in dB) of the normal native volume supported by the specific
* voice, in the range [-96.0, 16.0]. If unset, or set to a value of 0.0 (dB), will play at normal
* native signal amplitude. A value of -6.0 (dB) will play at approximately half the amplitude of
* the normal native signal amplitude. A value of +6.0 (dB) will play at approximately twice the
* amplitude of the normal native signal amplitude. Strongly recommend not to exceed +10 (dB) as
* there's usually no effective increase in loudness for any value greater than that.
* @param volumeGainDb volumeGainDb or {@code null} for none
*/
public AudioConfig setVolumeGainDb(java.lang.Double volumeGainDb) {
this.volumeGainDb = volumeGainDb;
return this;
}
@Override
public AudioConfig set(String fieldName, Object value) {
return (AudioConfig) super.set(fieldName, value);
}
@Override
public AudioConfig clone() {
return (AudioConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy