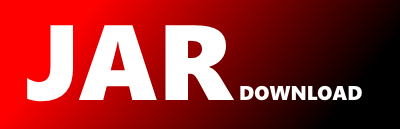
com.google.api.services.toolresults.ToolResults Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-06-07 at 03:00:34 UTC
* Modify at your own risk.
*/
package com.google.api.services.toolresults;
/**
* Service definition for ToolResults (v1beta3).
*
*
* Reads and publishes results from Firebase Test Lab.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link ToolResultsRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class ToolResults extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Cloud Tool Results API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "toolresults/v1beta3/projects/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public ToolResults(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
ToolResults(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code ToolResults toolresults = new ToolResults(...);}
* {@code ToolResults.Projects.List request = toolresults.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* Gets the Tool Results settings for a project.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read from project
*
* Create a request for the method "projects.getSettings".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link GetSettings#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @return the request
*/
public GetSettings getSettings(java.lang.String projectId) throws java.io.IOException {
GetSettings result = new GetSettings(projectId);
initialize(result);
return result;
}
public class GetSettings extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/settings";
/**
* Gets the Tool Results settings for a project.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read from project
*
* Create a request for the method "projects.getSettings".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link GetSettings#execute()} method to invoke the remote
* operation. {@link
* GetSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @since 1.13
*/
protected GetSettings(java.lang.String projectId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.ProjectSettings.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetSettings setAlt(java.lang.String alt) {
return (GetSettings) super.setAlt(alt);
}
@Override
public GetSettings setFields(java.lang.String fields) {
return (GetSettings) super.setFields(fields);
}
@Override
public GetSettings setKey(java.lang.String key) {
return (GetSettings) super.setKey(key);
}
@Override
public GetSettings setOauthToken(java.lang.String oauthToken) {
return (GetSettings) super.setOauthToken(oauthToken);
}
@Override
public GetSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSettings setQuotaUser(java.lang.String quotaUser) {
return (GetSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetSettings setUserIp(java.lang.String userIp) {
return (GetSettings) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public GetSettings setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public GetSettings set(String parameterName, Object value) {
return (GetSettings) super.set(parameterName, value);
}
}
/**
* Creates resources for settings which have not yet been set.
*
* Currently, this creates a single resource: a Google Cloud Storage bucket, to be used as the
* default bucket for this project. The bucket is created in an FTL-own storage project. Except for
* in rare cases, calling this method in parallel from multiple clients will only create a single
* bucket. In order to avoid unnecessary storage charges, the bucket is configured to automatically
* delete objects older than 90 days.
*
* The bucket is created with the following permissions: - Owner access for owners of central
* storage project (FTL-owned) - Writer access for owners/editors of customer project - Reader
* access for viewers of customer project The default ACL on objects created in the bucket is: -
* Owner access for owners of central storage project - Reader access for owners/editors/viewers of
* customer project See Google Cloud Storage documentation for more details.
*
* If there is already a default bucket set and the project can access the bucket, this call does
* nothing. However, if the project doesn't have the permission to access the bucket or the bucket
* is deleted, a new bucket will be created.
*
* May return any canonical error codes, including the following:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - Any error code raised
* by Google Cloud Storage
*
* Create a request for the method "projects.initializeSettings".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link InitializeSettings#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @return the request
*/
public InitializeSettings initializeSettings(java.lang.String projectId) throws java.io.IOException {
InitializeSettings result = new InitializeSettings(projectId);
initialize(result);
return result;
}
public class InitializeSettings extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}:initializeSettings";
/**
* Creates resources for settings which have not yet been set.
*
* Currently, this creates a single resource: a Google Cloud Storage bucket, to be used as the
* default bucket for this project. The bucket is created in an FTL-own storage project. Except
* for in rare cases, calling this method in parallel from multiple clients will only create a
* single bucket. In order to avoid unnecessary storage charges, the bucket is configured to
* automatically delete objects older than 90 days.
*
* The bucket is created with the following permissions: - Owner access for owners of central
* storage project (FTL-owned) - Writer access for owners/editors of customer project - Reader
* access for viewers of customer project The default ACL on objects created in the bucket is: -
* Owner access for owners of central storage project - Reader access for owners/editors/viewers
* of customer project See Google Cloud Storage documentation for more details.
*
* If there is already a default bucket set and the project can access the bucket, this call does
* nothing. However, if the project doesn't have the permission to access the bucket or the bucket
* is deleted, a new bucket will be created.
*
* May return any canonical error codes, including the following:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - Any error code raised
* by Google Cloud Storage
*
* Create a request for the method "projects.initializeSettings".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link InitializeSettings#execute()} method to invoke the remote
* operation. {@link InitializeSettings#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param projectId A Project id.
Required.
* @since 1.13
*/
protected InitializeSettings(java.lang.String projectId) {
super(ToolResults.this, "POST", REST_PATH, null, com.google.api.services.toolresults.model.ProjectSettings.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public InitializeSettings setAlt(java.lang.String alt) {
return (InitializeSettings) super.setAlt(alt);
}
@Override
public InitializeSettings setFields(java.lang.String fields) {
return (InitializeSettings) super.setFields(fields);
}
@Override
public InitializeSettings setKey(java.lang.String key) {
return (InitializeSettings) super.setKey(key);
}
@Override
public InitializeSettings setOauthToken(java.lang.String oauthToken) {
return (InitializeSettings) super.setOauthToken(oauthToken);
}
@Override
public InitializeSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (InitializeSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public InitializeSettings setQuotaUser(java.lang.String quotaUser) {
return (InitializeSettings) super.setQuotaUser(quotaUser);
}
@Override
public InitializeSettings setUserIp(java.lang.String userIp) {
return (InitializeSettings) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public InitializeSettings setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public InitializeSettings set(String parameterName, Object value) {
return (InitializeSettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Histories collection.
*
* The typical use is:
*
* {@code ToolResults toolresults = new ToolResults(...);}
* {@code ToolResults.Histories.List request = toolresults.histories().list(parameters ...)}
*
*
* @return the resource collection
*/
public Histories histories() {
return new Histories();
}
/**
* The "histories" collection of methods.
*/
public class Histories {
/**
* Creates a History.
*
* The returned History will have the id set.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - NOT_FOUND - if the containing project does not exist
*
* Create a request for the method "histories.create".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param content the {@link com.google.api.services.toolresults.model.History}
* @return the request
*/
public Create create(java.lang.String projectId, com.google.api.services.toolresults.model.History content) throws java.io.IOException {
Create result = new Create(projectId, content);
initialize(result);
return result;
}
public class Create extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories";
/**
* Creates a History.
*
* The returned History will have the id set.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - NOT_FOUND - if the containing project does not exist
*
* Create a request for the method "histories.create".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param content the {@link com.google.api.services.toolresults.model.History}
* @since 1.13
*/
protected Create(java.lang.String projectId, com.google.api.services.toolresults.model.History content) {
super(ToolResults.this, "POST", REST_PATH, content, com.google.api.services.toolresults.model.History.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique request ID for server to detect duplicated requests. For example, a UUID.
Optional, but strongly recommended.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a History.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the History does not exist
*
* Create a request for the method "histories.get".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @return the request
*/
public Get get(java.lang.String projectId, java.lang.String historyId) throws java.io.IOException {
Get result = new Get(projectId, historyId);
initialize(result);
return result;
}
public class Get extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}";
/**
* Gets a History.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the History does not exist
*
* Create a request for the method "histories.get".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @since 1.13
*/
protected Get(java.lang.String projectId, java.lang.String historyId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.History.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public Get setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public Get setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists Histories for a given Project.
*
* The histories are sorted by modification time in descending order. The history_id key will be
* used to order the history with the same modification time.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the History does not exist
*
* Create a request for the method "histories.list".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @return the request
*/
public List list(java.lang.String projectId) throws java.io.IOException {
List result = new List(projectId);
initialize(result);
return result;
}
public class List extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories";
/**
* Lists Histories for a given Project.
*
* The histories are sorted by modification time in descending order. The history_id key will be
* used to order the history with the same modification time.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the History does not exist
*
* Create a request for the method "histories.list".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @since 1.13
*/
protected List(java.lang.String projectId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.ListHistoriesResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* If set, only return histories with the given name.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.String filterByName;
/** If set, only return histories with the given name.
Optional.
*/
public java.lang.String getFilterByName() {
return filterByName;
}
/**
* If set, only return histories with the given name.
*
* Optional.
*/
public List setFilterByName(java.lang.String filterByName) {
this.filterByName = filterByName;
return this;
}
/**
* The maximum number of Histories to fetch.
*
* Default value: 20. The server will use this default if the field is not set or has a
* value of 0. Any value greater than 100 will be treated as 100.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of Histories to fetch.
Default value: 20. The server will use this default if the field is not set or has a value of 0.
Any value greater than 100 will be treated as 100.
Optional.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of Histories to fetch.
*
* Default value: 20. The server will use this default if the field is not set or has a
* value of 0. Any value greater than 100 will be treated as 100.
*
* Optional.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A continuation token to resume the query at the next item.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token to resume the query at the next item.
Optional.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A continuation token to resume the query at the next item.
*
* Optional.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Executions collection.
*
* The typical use is:
*
* {@code ToolResults toolresults = new ToolResults(...);}
* {@code ToolResults.Executions.List request = toolresults.executions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Executions executions() {
return new Executions();
}
/**
* The "executions" collection of methods.
*/
public class Executions {
/**
* Creates an Execution.
*
* The returned Execution will have the id set.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - NOT_FOUND - if the containing History does not exist
*
* Create a request for the method "executions.create".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param content the {@link com.google.api.services.toolresults.model.Execution}
* @return the request
*/
public Create create(java.lang.String projectId, java.lang.String historyId, com.google.api.services.toolresults.model.Execution content) throws java.io.IOException {
Create result = new Create(projectId, historyId, content);
initialize(result);
return result;
}
public class Create extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions";
/**
* Creates an Execution.
*
* The returned Execution will have the id set.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - NOT_FOUND - if the containing History does not exist
*
* Create a request for the method "executions.create".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param content the {@link com.google.api.services.toolresults.model.Execution}
* @since 1.13
*/
protected Create(java.lang.String projectId, java.lang.String historyId, com.google.api.services.toolresults.model.Execution content) {
super(ToolResults.this, "POST", REST_PATH, content, com.google.api.services.toolresults.model.Execution.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public Create setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique request ID for server to detect duplicated requests. For example, a UUID.
Optional, but strongly recommended.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets an Execution.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - NOT_FOUND - if the Execution does not exist
*
* Create a request for the method "executions.get".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId An Execution id.
Required.
* @return the request
*/
public Get get(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId) throws java.io.IOException {
Get result = new Get(projectId, historyId, executionId);
initialize(result);
return result;
}
public class Get extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}";
/**
* Gets an Execution.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - NOT_FOUND - if the Execution does not exist
*
* Create a request for the method "executions.get".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId An Execution id.
Required.
* @since 1.13
*/
protected Get(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.Execution.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public Get setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public Get setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* An Execution id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String executionId;
/** An Execution id.
Required.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/**
* An Execution id.
*
* Required.
*/
public Get setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists Histories for a given Project.
*
* The executions are sorted by creation_time in descending order. The execution_id key will be used
* to order the executions with the same creation_time.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the containing History does not exist
*
* Create a request for the method "executions.list".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @return the request
*/
public List list(java.lang.String projectId, java.lang.String historyId) throws java.io.IOException {
List result = new List(projectId, historyId);
initialize(result);
return result;
}
public class List extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions";
/**
* Lists Histories for a given Project.
*
* The executions are sorted by creation_time in descending order. The execution_id key will be
* used to order the executions with the same creation_time.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the containing History does not exist
*
* Create a request for the method "executions.list".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @since 1.13
*/
protected List(java.lang.String projectId, java.lang.String historyId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.ListExecutionsResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public List setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* The maximum number of Executions to fetch.
*
* Default value: 25. The server will use this default if the field is not set or has a
* value of 0.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of Executions to fetch.
Default value: 25. The server will use this default if the field is not set or has a value of 0.
Optional.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of Executions to fetch.
*
* Default value: 25. The server will use this default if the field is not set or has a
* value of 0.
*
* Optional.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A continuation token to resume the query at the next item.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token to resume the query at the next item.
Optional.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A continuation token to resume the query at the next item.
*
* Optional.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing Execution with the supplied partial entity.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - FAILED_PRECONDITION - if the requested state transition is illegal -
* NOT_FOUND - if the containing History does not exist
*
* Create a request for the method "executions.patch".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param projectId A Project id. Required.
* @param historyId Required.
* @param executionId Required.
* @param content the {@link com.google.api.services.toolresults.model.Execution}
* @return the request
*/
public Patch patch(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, com.google.api.services.toolresults.model.Execution content) throws java.io.IOException {
Patch result = new Patch(projectId, historyId, executionId, content);
initialize(result);
return result;
}
public class Patch extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}";
/**
* Updates an existing Execution with the supplied partial entity.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - FAILED_PRECONDITION - if the requested state transition is illegal -
* NOT_FOUND - if the containing History does not exist
*
* Create a request for the method "executions.patch".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id. Required.
* @param historyId Required.
* @param executionId Required.
* @param content the {@link com.google.api.services.toolresults.model.Execution}
* @since 1.13
*/
protected Patch(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, com.google.api.services.toolresults.model.Execution content) {
super(ToolResults.this, "PATCH", REST_PATH, content, com.google.api.services.toolresults.model.Execution.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/** A Project id. Required. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id. Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** A Project id. Required. */
public Patch setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Required. */
@com.google.api.client.util.Key
private java.lang.String historyId;
/** Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/** Required. */
public Patch setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/** Required. */
@com.google.api.client.util.Key
private java.lang.String executionId;
/** Required.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/** Required. */
public Patch setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique request ID for server to detect duplicated requests. For example, a UUID.
Optional, but strongly recommended.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Steps collection.
*
* The typical use is:
*
* {@code ToolResults toolresults = new ToolResults(...);}
* {@code ToolResults.Steps.List request = toolresults.steps().list(parameters ...)}
*
*
* @return the resource collection
*/
public Steps steps() {
return new Steps();
}
/**
* The "steps" collection of methods.
*/
public class Steps {
/**
* Creates a Step.
*
* The returned Step will have the id set.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - FAILED_PRECONDITION - if the step is too large (more than 10Mib) -
* NOT_FOUND - if the containing Execution does not exist
*
* Create a request for the method "steps.create".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @param content the {@link com.google.api.services.toolresults.model.Step}
* @return the request
*/
public Create create(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, com.google.api.services.toolresults.model.Step content) throws java.io.IOException {
Create result = new Create(projectId, historyId, executionId, content);
initialize(result);
return result;
}
public class Create extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps";
/**
* Creates a Step.
*
* The returned Step will have the id set.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write to project - INVALID_ARGUMENT - if
* the request is malformed - FAILED_PRECONDITION - if the step is too large (more than 10Mib) -
* NOT_FOUND - if the containing Execution does not exist
*
* Create a request for the method "steps.create".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @param content the {@link com.google.api.services.toolresults.model.Step}
* @since 1.13
*/
protected Create(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, com.google.api.services.toolresults.model.Step content) {
super(ToolResults.this, "POST", REST_PATH, content, com.google.api.services.toolresults.model.Step.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public Create setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* A Execution id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A Execution id.
Required.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/**
* A Execution id.
*
* Required.
*/
public Create setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique request ID for server to detect duplicated requests. For example, a UUID.
Optional, but strongly recommended.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a Step.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the Step does not exist
*
* Create a request for the method "steps.get".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @param stepId A Step id.
Required.
* @return the request
*/
public Get get(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId) throws java.io.IOException {
Get result = new Get(projectId, historyId, executionId, stepId);
initialize(result);
return result;
}
public class Get extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}";
/**
* Gets a Step.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the Step does not exist
*
* Create a request for the method "steps.get".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @param stepId A Step id.
Required.
* @since 1.13
*/
protected Get(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.Step.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public Get setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public Get setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* A Execution id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A Execution id.
Required.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/**
* A Execution id.
*
* Required.
*/
public Get setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/**
* A Step id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A Step id.
Required.
*/
public java.lang.String getStepId() {
return stepId;
}
/**
* A Step id.
*
* Required.
*/
public Get setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves a PerfMetricsSummary.
*
* May return any of the following error code(s): - NOT_FOUND - The specified PerfMetricsSummary
* does not exist
*
* Create a request for the method "steps.getPerfMetricsSummary".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link GetPerfMetricsSummary#execute()} method to invoke the remote
* operation.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @return the request
*/
public GetPerfMetricsSummary getPerfMetricsSummary(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId) throws java.io.IOException {
GetPerfMetricsSummary result = new GetPerfMetricsSummary(projectId, historyId, executionId, stepId);
initialize(result);
return result;
}
public class GetPerfMetricsSummary extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}/perfMetricsSummary";
/**
* Retrieves a PerfMetricsSummary.
*
* May return any of the following error code(s): - NOT_FOUND - The specified PerfMetricsSummary
* does not exist
*
* Create a request for the method "steps.getPerfMetricsSummary".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link GetPerfMetricsSummary#execute()} method to invoke the
* remote operation. {@link GetPerfMetricsSummary#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @since 1.13
*/
protected GetPerfMetricsSummary(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.PerfMetricsSummary.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetPerfMetricsSummary setAlt(java.lang.String alt) {
return (GetPerfMetricsSummary) super.setAlt(alt);
}
@Override
public GetPerfMetricsSummary setFields(java.lang.String fields) {
return (GetPerfMetricsSummary) super.setFields(fields);
}
@Override
public GetPerfMetricsSummary setKey(java.lang.String key) {
return (GetPerfMetricsSummary) super.setKey(key);
}
@Override
public GetPerfMetricsSummary setOauthToken(java.lang.String oauthToken) {
return (GetPerfMetricsSummary) super.setOauthToken(oauthToken);
}
@Override
public GetPerfMetricsSummary setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetPerfMetricsSummary) super.setPrettyPrint(prettyPrint);
}
@Override
public GetPerfMetricsSummary setQuotaUser(java.lang.String quotaUser) {
return (GetPerfMetricsSummary) super.setQuotaUser(quotaUser);
}
@Override
public GetPerfMetricsSummary setUserIp(java.lang.String userIp) {
return (GetPerfMetricsSummary) super.setUserIp(userIp);
}
/** The cloud project */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The cloud project
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The cloud project */
public GetPerfMetricsSummary setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** A tool results history ID. */
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A tool results history ID.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/** A tool results history ID. */
public GetPerfMetricsSummary setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/** A tool results execution ID. */
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A tool results execution ID.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/** A tool results execution ID. */
public GetPerfMetricsSummary setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/** A tool results step ID. */
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A tool results step ID.
*/
public java.lang.String getStepId() {
return stepId;
}
/** A tool results step ID. */
public GetPerfMetricsSummary setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
@Override
public GetPerfMetricsSummary set(String parameterName, Object value) {
return (GetPerfMetricsSummary) super.set(parameterName, value);
}
}
/**
* Lists Steps for a given Execution.
*
* The steps are sorted by creation_time in descending order. The step_id key will be used to order
* the steps with the same creation_time.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - FAILED_PRECONDITION - if an argument in the request happens to be invalid;
* e.g. if an attempt is made to list the children of a nonexistent Step - NOT_FOUND - if the
* containing Execution does not exist
*
* Create a request for the method "steps.list".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @return the request
*/
public List list(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId) throws java.io.IOException {
List result = new List(projectId, historyId, executionId);
initialize(result);
return result;
}
public class List extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps";
/**
* Lists Steps for a given Execution.
*
* The steps are sorted by creation_time in descending order. The step_id key will be used to
* order the steps with the same creation_time.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to read project - INVALID_ARGUMENT - if the
* request is malformed - FAILED_PRECONDITION - if an argument in the request happens to be
* invalid; e.g. if an attempt is made to list the children of a nonexistent Step - NOT_FOUND - if
* the containing Execution does not exist
*
* Create a request for the method "steps.list".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @since 1.13
*/
protected List(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.ListStepsResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public List setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* A Execution id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A Execution id.
Required.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/**
* A Execution id.
*
* Required.
*/
public List setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/**
* The maximum number of Steps to fetch.
*
* Default value: 25. The server will use this default if the field is not set or has a
* value of 0.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of Steps to fetch.
Default value: 25. The server will use this default if the field is not set or has a value of 0.
Optional.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of Steps to fetch.
*
* Default value: 25. The server will use this default if the field is not set or has a
* value of 0.
*
* Optional.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A continuation token to resume the query at the next item.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token to resume the query at the next item.
Optional.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A continuation token to resume the query at the next item.
*
* Optional.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing Step with the supplied partial entity.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write project - INVALID_ARGUMENT - if the
* request is malformed - FAILED_PRECONDITION - if the requested state transition is illegal (e.g
* try to upload a duplicate xml file), if the updated step is too large (more than 10Mib) -
* NOT_FOUND - if the containing Execution does not exist
*
* Create a request for the method "steps.patch".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @param stepId A Step id.
Required.
* @param content the {@link com.google.api.services.toolresults.model.Step}
* @return the request
*/
public Patch patch(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, com.google.api.services.toolresults.model.Step content) throws java.io.IOException {
Patch result = new Patch(projectId, historyId, executionId, stepId, content);
initialize(result);
return result;
}
public class Patch extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}";
/**
* Updates an existing Step with the supplied partial entity.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write project - INVALID_ARGUMENT - if
* the request is malformed - FAILED_PRECONDITION - if the requested state transition is illegal
* (e.g try to upload a duplicate xml file), if the updated step is too large (more than 10Mib) -
* NOT_FOUND - if the containing Execution does not exist
*
* Create a request for the method "steps.patch".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @param stepId A Step id.
Required.
* @param content the {@link com.google.api.services.toolresults.model.Step}
* @since 1.13
*/
protected Patch(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, com.google.api.services.toolresults.model.Step content) {
super(ToolResults.this, "PATCH", REST_PATH, content, com.google.api.services.toolresults.model.Step.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public Patch setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public Patch setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* A Execution id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A Execution id.
Required.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/**
* A Execution id.
*
* Required.
*/
public Patch setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/**
* A Step id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A Step id.
Required.
*/
public java.lang.String getStepId() {
return stepId;
}
/**
* A Step id.
*
* Required.
*/
public Patch setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A unique request ID for server to detect duplicated requests. For example, a UUID.
Optional, but strongly recommended.
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A unique request ID for server to detect duplicated requests. For example, a UUID.
*
* Optional, but strongly recommended.
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Publish xml files to an existing Step.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write project - INVALID_ARGUMENT - if the
* request is malformed - FAILED_PRECONDITION - if the requested state transition is illegal, e.g
* try to upload a duplicate xml file or a file too large. - NOT_FOUND - if the containing Execution
* does not exist
*
* Create a request for the method "steps.publishXunitXmlFiles".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link PublishXunitXmlFiles#execute()} method to invoke the remote
* operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @param stepId A Step id. Note: This step must include a TestExecutionStep.
Required.
* @param content the {@link com.google.api.services.toolresults.model.PublishXunitXmlFilesRequest}
* @return the request
*/
public PublishXunitXmlFiles publishXunitXmlFiles(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, com.google.api.services.toolresults.model.PublishXunitXmlFilesRequest content) throws java.io.IOException {
PublishXunitXmlFiles result = new PublishXunitXmlFiles(projectId, historyId, executionId, stepId, content);
initialize(result);
return result;
}
public class PublishXunitXmlFiles extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}:publishXunitXmlFiles";
/**
* Publish xml files to an existing Step.
*
* May return any of the following canonical error codes:
*
* - PERMISSION_DENIED - if the user is not authorized to write project - INVALID_ARGUMENT - if
* the request is malformed - FAILED_PRECONDITION - if the requested state transition is illegal,
* e.g try to upload a duplicate xml file or a file too large. - NOT_FOUND - if the containing
* Execution does not exist
*
* Create a request for the method "steps.publishXunitXmlFiles".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link PublishXunitXmlFiles#execute()} method to invoke the
* remote operation. {@link PublishXunitXmlFiles#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId A Execution id.
Required.
* @param stepId A Step id. Note: This step must include a TestExecutionStep.
Required.
* @param content the {@link com.google.api.services.toolresults.model.PublishXunitXmlFilesRequest}
* @since 1.13
*/
protected PublishXunitXmlFiles(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, com.google.api.services.toolresults.model.PublishXunitXmlFilesRequest content) {
super(ToolResults.this, "POST", REST_PATH, content, com.google.api.services.toolresults.model.Step.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
}
@Override
public PublishXunitXmlFiles setAlt(java.lang.String alt) {
return (PublishXunitXmlFiles) super.setAlt(alt);
}
@Override
public PublishXunitXmlFiles setFields(java.lang.String fields) {
return (PublishXunitXmlFiles) super.setFields(fields);
}
@Override
public PublishXunitXmlFiles setKey(java.lang.String key) {
return (PublishXunitXmlFiles) super.setKey(key);
}
@Override
public PublishXunitXmlFiles setOauthToken(java.lang.String oauthToken) {
return (PublishXunitXmlFiles) super.setOauthToken(oauthToken);
}
@Override
public PublishXunitXmlFiles setPrettyPrint(java.lang.Boolean prettyPrint) {
return (PublishXunitXmlFiles) super.setPrettyPrint(prettyPrint);
}
@Override
public PublishXunitXmlFiles setQuotaUser(java.lang.String quotaUser) {
return (PublishXunitXmlFiles) super.setQuotaUser(quotaUser);
}
@Override
public PublishXunitXmlFiles setUserIp(java.lang.String userIp) {
return (PublishXunitXmlFiles) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public PublishXunitXmlFiles setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public PublishXunitXmlFiles setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* A Execution id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A Execution id.
Required.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/**
* A Execution id.
*
* Required.
*/
public PublishXunitXmlFiles setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/**
* A Step id. Note: This step must include a TestExecutionStep.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A Step id. Note: This step must include a TestExecutionStep.
Required.
*/
public java.lang.String getStepId() {
return stepId;
}
/**
* A Step id. Note: This step must include a TestExecutionStep.
*
* Required.
*/
public PublishXunitXmlFiles setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
@Override
public PublishXunitXmlFiles set(String parameterName, Object value) {
return (PublishXunitXmlFiles) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the PerfMetricsSummary collection.
*
* The typical use is:
*
* {@code ToolResults toolresults = new ToolResults(...);}
* {@code ToolResults.PerfMetricsSummary.List request = toolresults.perfMetricsSummary().list(parameters ...)}
*
*
* @return the resource collection
*/
public PerfMetricsSummary perfMetricsSummary() {
return new PerfMetricsSummary();
}
/**
* The "perfMetricsSummary" collection of methods.
*/
public class PerfMetricsSummary {
/**
* Creates a PerfMetricsSummary resource.
*
* May return any of the following error code(s): - ALREADY_EXISTS - A PerfMetricSummary already
* exists for the given Step - NOT_FOUND - The containing Step does not exist
*
* Create a request for the method "perfMetricsSummary.create".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param content the {@link com.google.api.services.toolresults.model.PerfMetricsSummary}
* @return the request
*/
public Create create(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, com.google.api.services.toolresults.model.PerfMetricsSummary content) throws java.io.IOException {
Create result = new Create(projectId, historyId, executionId, stepId, content);
initialize(result);
return result;
}
public class Create extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}/perfMetricsSummary";
/**
* Creates a PerfMetricsSummary resource.
*
* May return any of the following error code(s): - ALREADY_EXISTS - A PerfMetricSummary already
* exists for the given Step - NOT_FOUND - The containing Step does not exist
*
* Create a request for the method "perfMetricsSummary.create".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param content the {@link com.google.api.services.toolresults.model.PerfMetricsSummary}
* @since 1.13
*/
protected Create(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, com.google.api.services.toolresults.model.PerfMetricsSummary content) {
super(ToolResults.this, "POST", REST_PATH, content, com.google.api.services.toolresults.model.PerfMetricsSummary.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/** The cloud project */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The cloud project
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The cloud project */
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** A tool results history ID. */
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A tool results history ID.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/** A tool results history ID. */
public Create setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/** A tool results execution ID. */
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A tool results execution ID.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/** A tool results execution ID. */
public Create setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/** A tool results step ID. */
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A tool results step ID.
*/
public java.lang.String getStepId() {
return stepId;
}
/** A tool results step ID. */
public Create setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the PerfSampleSeries collection.
*
* The typical use is:
*
* {@code ToolResults toolresults = new ToolResults(...);}
* {@code ToolResults.PerfSampleSeries.List request = toolresults.perfSampleSeries().list(parameters ...)}
*
*
* @return the resource collection
*/
public PerfSampleSeries perfSampleSeries() {
return new PerfSampleSeries();
}
/**
* The "perfSampleSeries" collection of methods.
*/
public class PerfSampleSeries {
/**
* Creates a PerfSampleSeries.
*
* May return any of the following error code(s): - ALREADY_EXISTS - PerfMetricSummary already
* exists for the given Step - NOT_FOUND - The containing Step does not exist
*
* Create a request for the method "perfSampleSeries.create".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param content the {@link com.google.api.services.toolresults.model.PerfSampleSeries}
* @return the request
*/
public Create create(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, com.google.api.services.toolresults.model.PerfSampleSeries content) throws java.io.IOException {
Create result = new Create(projectId, historyId, executionId, stepId, content);
initialize(result);
return result;
}
public class Create extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}/perfSampleSeries";
/**
* Creates a PerfSampleSeries.
*
* May return any of the following error code(s): - ALREADY_EXISTS - PerfMetricSummary already
* exists for the given Step - NOT_FOUND - The containing Step does not exist
*
* Create a request for the method "perfSampleSeries.create".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param content the {@link com.google.api.services.toolresults.model.PerfSampleSeries}
* @since 1.13
*/
protected Create(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, com.google.api.services.toolresults.model.PerfSampleSeries content) {
super(ToolResults.this, "POST", REST_PATH, content, com.google.api.services.toolresults.model.PerfSampleSeries.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/** The cloud project */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The cloud project
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The cloud project */
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** A tool results history ID. */
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A tool results history ID.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/** A tool results history ID. */
public Create setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/** A tool results execution ID. */
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A tool results execution ID.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/** A tool results execution ID. */
public Create setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/** A tool results step ID. */
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A tool results step ID.
*/
public java.lang.String getStepId() {
return stepId;
}
/** A tool results step ID. */
public Create setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a PerfSampleSeries.
*
* May return any of the following error code(s): - NOT_FOUND - The specified PerfSampleSeries does
* not exist
*
* Create a request for the method "perfSampleSeries.get".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param sampleSeriesId A sample series id
* @return the request
*/
public Get get(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, java.lang.String sampleSeriesId) throws java.io.IOException {
Get result = new Get(projectId, historyId, executionId, stepId, sampleSeriesId);
initialize(result);
return result;
}
public class Get extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}/perfSampleSeries/{sampleSeriesId}";
/**
* Gets a PerfSampleSeries.
*
* May return any of the following error code(s): - NOT_FOUND - The specified PerfSampleSeries
* does not exist
*
* Create a request for the method "perfSampleSeries.get".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param sampleSeriesId A sample series id
* @since 1.13
*/
protected Get(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, java.lang.String sampleSeriesId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.PerfSampleSeries.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
this.sampleSeriesId = com.google.api.client.util.Preconditions.checkNotNull(sampleSeriesId, "Required parameter sampleSeriesId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The cloud project */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The cloud project
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The cloud project */
public Get setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** A tool results history ID. */
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A tool results history ID.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/** A tool results history ID. */
public Get setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/** A tool results execution ID. */
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A tool results execution ID.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/** A tool results execution ID. */
public Get setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/** A tool results step ID. */
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A tool results step ID.
*/
public java.lang.String getStepId() {
return stepId;
}
/** A tool results step ID. */
public Get setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
/** A sample series id */
@com.google.api.client.util.Key
private java.lang.String sampleSeriesId;
/** A sample series id
*/
public java.lang.String getSampleSeriesId() {
return sampleSeriesId;
}
/** A sample series id */
public Get setSampleSeriesId(java.lang.String sampleSeriesId) {
this.sampleSeriesId = sampleSeriesId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists PerfSampleSeries for a given Step.
*
* The request provides an optional filter which specifies one or more PerfMetricsType to include in
* the result; if none returns all. The resulting PerfSampleSeries are sorted by ids.
*
* May return any of the following canonical error codes: - NOT_FOUND - The containing Step does not
* exist
*
* Create a request for the method "perfSampleSeries.list".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @return the request
*/
public List list(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId) throws java.io.IOException {
List result = new List(projectId, historyId, executionId, stepId);
initialize(result);
return result;
}
public class List extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}/perfSampleSeries";
/**
* Lists PerfSampleSeries for a given Step.
*
* The request provides an optional filter which specifies one or more PerfMetricsType to include
* in the result; if none returns all. The resulting PerfSampleSeries are sorted by ids.
*
* May return any of the following canonical error codes: - NOT_FOUND - The containing Step does
* not exist
*
* Create a request for the method "perfSampleSeries.list".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @since 1.13
*/
protected List(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.ListPerfSampleSeriesResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The cloud project */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The cloud project
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The cloud project */
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** A tool results history ID. */
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A tool results history ID.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/** A tool results history ID. */
public List setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/** A tool results execution ID. */
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A tool results execution ID.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/** A tool results execution ID. */
public List setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/** A tool results step ID. */
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A tool results step ID.
*/
public java.lang.String getStepId() {
return stepId;
}
/** A tool results step ID. */
public List setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
/** Specify one or more PerfMetricType values such as CPU to filter the result */
@com.google.api.client.util.Key
private java.util.List filter;
/** Specify one or more PerfMetricType values such as CPU to filter the result
*/
public java.util.List getFilter() {
return filter;
}
/** Specify one or more PerfMetricType values such as CPU to filter the result */
public List setFilter(java.util.List filter) {
this.filter = filter;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Samples collection.
*
* The typical use is:
*
* {@code ToolResults toolresults = new ToolResults(...);}
* {@code ToolResults.Samples.List request = toolresults.samples().list(parameters ...)}
*
*
* @return the resource collection
*/
public Samples samples() {
return new Samples();
}
/**
* The "samples" collection of methods.
*/
public class Samples {
/**
* Creates a batch of PerfSamples - a client can submit multiple batches of Perf Samples through
* repeated calls to this method in order to split up a large request payload - duplicates and
* existing timestamp entries will be ignored. - the batch operation may partially succeed - the set
* of elements successfully inserted is returned in the response (omits items which already existed
* in the database).
*
* May return any of the following canonical error codes: - NOT_FOUND - The containing
* PerfSampleSeries does not exist
*
* Create a request for the method "samples.batchCreate".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link BatchCreate#execute()} method to invoke the remote operation.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param sampleSeriesId A sample series id
* @param content the {@link com.google.api.services.toolresults.model.BatchCreatePerfSamplesRequest}
* @return the request
*/
public BatchCreate batchCreate(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, java.lang.String sampleSeriesId, com.google.api.services.toolresults.model.BatchCreatePerfSamplesRequest content) throws java.io.IOException {
BatchCreate result = new BatchCreate(projectId, historyId, executionId, stepId, sampleSeriesId, content);
initialize(result);
return result;
}
public class BatchCreate extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}/perfSampleSeries/{sampleSeriesId}/samples:batchCreate";
/**
* Creates a batch of PerfSamples - a client can submit multiple batches of Perf Samples through
* repeated calls to this method in order to split up a large request payload - duplicates and
* existing timestamp entries will be ignored. - the batch operation may partially succeed - the
* set of elements successfully inserted is returned in the response (omits items which already
* existed in the database).
*
* May return any of the following canonical error codes: - NOT_FOUND - The containing
* PerfSampleSeries does not exist
*
* Create a request for the method "samples.batchCreate".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link BatchCreate#execute()} method to invoke the remote
* operation. {@link
* BatchCreate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param sampleSeriesId A sample series id
* @param content the {@link com.google.api.services.toolresults.model.BatchCreatePerfSamplesRequest}
* @since 1.13
*/
protected BatchCreate(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, java.lang.String sampleSeriesId, com.google.api.services.toolresults.model.BatchCreatePerfSamplesRequest content) {
super(ToolResults.this, "POST", REST_PATH, content, com.google.api.services.toolresults.model.BatchCreatePerfSamplesResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
this.sampleSeriesId = com.google.api.client.util.Preconditions.checkNotNull(sampleSeriesId, "Required parameter sampleSeriesId must be specified.");
}
@Override
public BatchCreate setAlt(java.lang.String alt) {
return (BatchCreate) super.setAlt(alt);
}
@Override
public BatchCreate setFields(java.lang.String fields) {
return (BatchCreate) super.setFields(fields);
}
@Override
public BatchCreate setKey(java.lang.String key) {
return (BatchCreate) super.setKey(key);
}
@Override
public BatchCreate setOauthToken(java.lang.String oauthToken) {
return (BatchCreate) super.setOauthToken(oauthToken);
}
@Override
public BatchCreate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchCreate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchCreate setQuotaUser(java.lang.String quotaUser) {
return (BatchCreate) super.setQuotaUser(quotaUser);
}
@Override
public BatchCreate setUserIp(java.lang.String userIp) {
return (BatchCreate) super.setUserIp(userIp);
}
/** The cloud project */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The cloud project
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The cloud project */
public BatchCreate setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** A tool results history ID. */
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A tool results history ID.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/** A tool results history ID. */
public BatchCreate setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/** A tool results execution ID. */
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A tool results execution ID.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/** A tool results execution ID. */
public BatchCreate setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/** A tool results step ID. */
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A tool results step ID.
*/
public java.lang.String getStepId() {
return stepId;
}
/** A tool results step ID. */
public BatchCreate setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
/** A sample series id */
@com.google.api.client.util.Key
private java.lang.String sampleSeriesId;
/** A sample series id
*/
public java.lang.String getSampleSeriesId() {
return sampleSeriesId;
}
/** A sample series id */
public BatchCreate setSampleSeriesId(java.lang.String sampleSeriesId) {
this.sampleSeriesId = sampleSeriesId;
return this;
}
@Override
public BatchCreate set(String parameterName, Object value) {
return (BatchCreate) super.set(parameterName, value);
}
}
/**
* Lists the Performance Samples of a given Sample Series - The list results are sorted by
* timestamps ascending - The default page size is 500 samples; and maximum size allowed 5000 - The
* response token indicates the last returned PerfSample timestamp - When the results size exceeds
* the page size, submit a subsequent request including the page token to return the rest of the
* samples up to the page limit
*
* May return any of the following canonical error codes: - OUT_OF_RANGE - The specified request
* page_token is out of valid range - NOT_FOUND - The containing PerfSampleSeries does not exist
*
* Create a request for the method "samples.list".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param sampleSeriesId A sample series id
* @return the request
*/
public List list(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, java.lang.String sampleSeriesId) throws java.io.IOException {
List result = new List(projectId, historyId, executionId, stepId, sampleSeriesId);
initialize(result);
return result;
}
public class List extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}/perfSampleSeries/{sampleSeriesId}/samples";
/**
* Lists the Performance Samples of a given Sample Series - The list results are sorted by
* timestamps ascending - The default page size is 500 samples; and maximum size allowed 5000 -
* The response token indicates the last returned PerfSample timestamp - When the results size
* exceeds the page size, submit a subsequent request including the page token to return the rest
* of the samples up to the page limit
*
* May return any of the following canonical error codes: - OUT_OF_RANGE - The specified request
* page_token is out of valid range - NOT_FOUND - The containing PerfSampleSeries does not exist
*
* Create a request for the method "samples.list".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId The cloud project
* @param historyId A tool results history ID.
* @param executionId A tool results execution ID.
* @param stepId A tool results step ID.
* @param sampleSeriesId A sample series id
* @since 1.13
*/
protected List(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId, java.lang.String sampleSeriesId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.ListPerfSamplesResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
this.sampleSeriesId = com.google.api.client.util.Preconditions.checkNotNull(sampleSeriesId, "Required parameter sampleSeriesId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The cloud project */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The cloud project
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The cloud project */
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** A tool results history ID. */
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A tool results history ID.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/** A tool results history ID. */
public List setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/** A tool results execution ID. */
@com.google.api.client.util.Key
private java.lang.String executionId;
/** A tool results execution ID.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/** A tool results execution ID. */
public List setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/** A tool results step ID. */
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A tool results step ID.
*/
public java.lang.String getStepId() {
return stepId;
}
/** A tool results step ID. */
public List setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
/** A sample series id */
@com.google.api.client.util.Key
private java.lang.String sampleSeriesId;
/** A sample series id
*/
public java.lang.String getSampleSeriesId() {
return sampleSeriesId;
}
/** A sample series id */
public List setSampleSeriesId(java.lang.String sampleSeriesId) {
this.sampleSeriesId = sampleSeriesId;
return this;
}
/**
* The default page size is 500 samples, and the maximum size is 5000. If the
* page_size is greater than 5000, the effective page size will be 5000
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The default page size is 500 samples, and the maximum size is 5000. If the page_size is greater
than 5000, the effective page size will be 5000
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The default page size is 500 samples, and the maximum size is 5000. If the
* page_size is greater than 5000, the effective page size will be 5000
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional, the next_page_token returned in the previous response */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional, the next_page_token returned in the previous response
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional, the next_page_token returned in the previous response */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Thumbnails collection.
*
* The typical use is:
*
* {@code ToolResults toolresults = new ToolResults(...);}
* {@code ToolResults.Thumbnails.List request = toolresults.thumbnails().list(parameters ...)}
*
*
* @return the resource collection
*/
public Thumbnails thumbnails() {
return new Thumbnails();
}
/**
* The "thumbnails" collection of methods.
*/
public class Thumbnails {
/**
* Lists thumbnails of images attached to a step.
*
* May return any of the following canonical error codes: - PERMISSION_DENIED - if the user is not
* authorized to read from the project, or from any of the images - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the step does not exist, or if any of the images do not
* exist
*
* Create a request for the method "thumbnails.list".
*
* This request holds the parameters needed by the toolresults server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId An Execution id.
Required.
* @param stepId A Step id.
Required.
* @return the request
*/
public List list(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId) throws java.io.IOException {
List result = new List(projectId, historyId, executionId, stepId);
initialize(result);
return result;
}
public class List extends ToolResultsRequest {
private static final String REST_PATH = "{projectId}/histories/{historyId}/executions/{executionId}/steps/{stepId}/thumbnails";
/**
* Lists thumbnails of images attached to a step.
*
* May return any of the following canonical error codes: - PERMISSION_DENIED - if the user is not
* authorized to read from the project, or from any of the images - INVALID_ARGUMENT - if the
* request is malformed - NOT_FOUND - if the step does not exist, or if any of the images do not
* exist
*
* Create a request for the method "thumbnails.list".
*
* This request holds the parameters needed by the the toolresults server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param projectId A Project id.
Required.
* @param historyId A History id.
Required.
* @param executionId An Execution id.
Required.
* @param stepId A Step id.
Required.
* @since 1.13
*/
protected List(java.lang.String projectId, java.lang.String historyId, java.lang.String executionId, java.lang.String stepId) {
super(ToolResults.this, "GET", REST_PATH, null, com.google.api.services.toolresults.model.ListStepThumbnailsResponse.class);
this.projectId = com.google.api.client.util.Preconditions.checkNotNull(projectId, "Required parameter projectId must be specified.");
this.historyId = com.google.api.client.util.Preconditions.checkNotNull(historyId, "Required parameter historyId must be specified.");
this.executionId = com.google.api.client.util.Preconditions.checkNotNull(executionId, "Required parameter executionId must be specified.");
this.stepId = com.google.api.client.util.Preconditions.checkNotNull(stepId, "Required parameter stepId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* A Project id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String projectId;
/** A Project id.
Required.
*/
public java.lang.String getProjectId() {
return projectId;
}
/**
* A Project id.
*
* Required.
*/
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* A History id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String historyId;
/** A History id.
Required.
*/
public java.lang.String getHistoryId() {
return historyId;
}
/**
* A History id.
*
* Required.
*/
public List setHistoryId(java.lang.String historyId) {
this.historyId = historyId;
return this;
}
/**
* An Execution id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String executionId;
/** An Execution id.
Required.
*/
public java.lang.String getExecutionId() {
return executionId;
}
/**
* An Execution id.
*
* Required.
*/
public List setExecutionId(java.lang.String executionId) {
this.executionId = executionId;
return this;
}
/**
* A Step id.
*
* Required.
*/
@com.google.api.client.util.Key
private java.lang.String stepId;
/** A Step id.
Required.
*/
public java.lang.String getStepId() {
return stepId;
}
/**
* A Step id.
*
* Required.
*/
public List setStepId(java.lang.String stepId) {
this.stepId = stepId;
return this;
}
/**
* The maximum number of thumbnails to fetch.
*
* Default value: 50. The server will use this default if the field is not set or has
* a value of 0.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of thumbnails to fetch.
Default value: 50. The server will use this default if the field is not set or has a value of 0.
Optional.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of thumbnails to fetch.
*
* Default value: 50. The server will use this default if the field is not set or has
* a value of 0.
*
* Optional.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A continuation token to resume the query at the next item.
*
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A continuation token to resume the query at the next item.
Optional.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A continuation token to resume the query at the next item.
*
* Optional.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
}
}
/**
* Builder for {@link ToolResults}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link ToolResults}. */
@Override
public ToolResults build() {
return new ToolResults(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link ToolResultsRequestInitializer}.
*
* @since 1.12
*/
public Builder setToolResultsRequestInitializer(
ToolResultsRequestInitializer toolresultsRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(toolresultsRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}