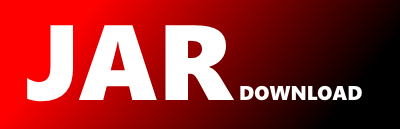
com.google.api.services.toolresults.model.Duration Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-06-07 at 03:00:34 UTC
* Modify at your own risk.
*/
package com.google.api.services.toolresults.model;
/**
* A Duration represents a signed, fixed-length span of time represented as a count of seconds and
* fractions of seconds at nanosecond resolution. It is independent of any calendar and concepts
* like "day" or "month". It is related to Timestamp in that the difference between two Timestamp
* values is a Duration and it can be added or subtracted from a Timestamp. Range is approximately
* +-10,000 years.
*
* # Examples
*
* Example 1: Compute Duration from two Timestamps in pseudo code.
*
* Timestamp start = ...; Timestamp end = ...; Duration duration = ...;
*
* duration.seconds = end.seconds - start.seconds; duration.nanos = end.nanos - start.nanos;
*
* if (duration.seconds 0) { duration.seconds += 1; duration.nanos -= 1000000000; } else if
* (durations.seconds > 0 && duration.nanos < 0) { duration.seconds -= 1; duration.nanos +=
* 1000000000; }
*
* Example 2: Compute Timestamp from Timestamp + Duration in pseudo code.
*
* Timestamp start = ...; Duration duration = ...; Timestamp end = ...;
*
* end.seconds = start.seconds + duration.seconds; end.nanos = start.nanos + duration.nanos;
*
* if (end.nanos = 1000000000) { end.seconds += 1; end.nanos -= 1000000000; }
*
* Example 3: Compute Duration from datetime.timedelta in Python.
*
* td = datetime.timedelta(days=3, minutes=10) duration = Duration() duration.FromTimedelta(td)
*
* # JSON Mapping
*
* In JSON format, the Duration type is encoded as a string rather than an object, where the string
* ends in the suffix "s" (indicating seconds) and is preceded by the number of seconds, with
* nanoseconds expressed as fractional seconds. For example, 3 seconds with 0 nanoseconds should be
* encoded in JSON format as "3s", while 3 seconds and 1 nanosecond should be expressed in JSON
* format as "3.000000001s", and 3 seconds and 1 microsecond should be expressed in JSON format as
* "3.000001s".
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Tool Results API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Duration extends com.google.api.client.json.GenericJson {
/**
* Signed fractions of a second at nanosecond resolution of the span of time. Durations less than
* one second are represented with a 0 `seconds` field and a positive or negative `nanos` field.
* For durations of one second or more, a non-zero value for the `nanos` field must be of the same
* sign as the `seconds` field. Must be from -999,999,999 to +999,999,999 inclusive.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer nanos;
/**
* Signed seconds of the span of time. Must be from -315,576,000,000 to +315,576,000,000
* inclusive. Note: these bounds are computed from: 60 sec/min * 60 min/hr * 24 hr/day * 365.25
* days/year * 10000 years
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long seconds;
/**
* Signed fractions of a second at nanosecond resolution of the span of time. Durations less than
* one second are represented with a 0 `seconds` field and a positive or negative `nanos` field.
* For durations of one second or more, a non-zero value for the `nanos` field must be of the same
* sign as the `seconds` field. Must be from -999,999,999 to +999,999,999 inclusive.
* @return value or {@code null} for none
*/
public java.lang.Integer getNanos() {
return nanos;
}
/**
* Signed fractions of a second at nanosecond resolution of the span of time. Durations less than
* one second are represented with a 0 `seconds` field and a positive or negative `nanos` field.
* For durations of one second or more, a non-zero value for the `nanos` field must be of the same
* sign as the `seconds` field. Must be from -999,999,999 to +999,999,999 inclusive.
* @param nanos nanos or {@code null} for none
*/
public Duration setNanos(java.lang.Integer nanos) {
this.nanos = nanos;
return this;
}
/**
* Signed seconds of the span of time. Must be from -315,576,000,000 to +315,576,000,000
* inclusive. Note: these bounds are computed from: 60 sec/min * 60 min/hr * 24 hr/day * 365.25
* days/year * 10000 years
* @return value or {@code null} for none
*/
public java.lang.Long getSeconds() {
return seconds;
}
/**
* Signed seconds of the span of time. Must be from -315,576,000,000 to +315,576,000,000
* inclusive. Note: these bounds are computed from: 60 sec/min * 60 min/hr * 24 hr/day * 365.25
* days/year * 10000 years
* @param seconds seconds or {@code null} for none
*/
public Duration setSeconds(java.lang.Long seconds) {
this.seconds = seconds;
return this;
}
@Override
public Duration set(String fieldName, Object value) {
return (Duration) super.set(fieldName, value);
}
@Override
public Duration clone() {
return (Duration) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy