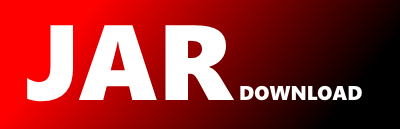
com.google.api.services.tpu.v2.model.Node Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.tpu.v2.model;
/**
* A TPU instance.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud TPU API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Node extends com.google.api.client.json.GenericJson {
/**
* The AccleratorConfig for the TPU Node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AcceleratorConfig acceleratorConfig;
/**
* Optional. The type of hardware accelerators associated with this node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String acceleratorType;
/**
* Output only. The API version that created this Node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String apiVersion;
/**
* The CIDR block that the TPU node will use when selecting an IP address. This CIDR block must be
* a /29 block; the Compute Engine networks API forbids a smaller block, and using a larger block
* would be wasteful (a node can only consume one IP address). Errors will occur if the CIDR block
* has already been used for a currently existing TPU node, the CIDR block conflicts with any
* subnetworks in the user's provided network, or the provided network is peered with another
* network that is using that CIDR block.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cidrBlock;
/**
* Output only. The time when the node was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* The additional data disks for the Node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List dataDisks;
static {
// hack to force ProGuard to consider AttachedDisk used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AttachedDisk.class);
}
/**
* The user-supplied description of the TPU. Maximum of 512 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The health status of the TPU node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String health;
/**
* Output only. If this field is populated, it contains a description of why the TPU Node is
* unhealthy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String healthDescription;
/**
* Output only. The unique identifier for the TPU Node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long id;
/**
* Resource labels to represent user-provided metadata.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Custom metadata to apply to the TPU Node. Can set startup-script and shutdown-script
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map metadata;
/**
* Output only. Whether the Node belongs to a Multislice group.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean multisliceNode;
/**
* Output only. Immutable. The name of the TPU.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Network configurations for the TPU node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NetworkConfig networkConfig;
/**
* Output only. The network endpoints where TPU workers can be accessed and sent work. It is
* recommended that runtime clients of the node reach out to the 0th entry in this map first.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List networkEndpoints;
static {
// hack to force ProGuard to consider NetworkEndpoint used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(NetworkEndpoint.class);
}
/**
* Output only. The qualified name of the QueuedResource that requested this Node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String queuedResource;
/**
* Required. The runtime version running in the Node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String runtimeVersion;
/**
* The scheduling options for this node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SchedulingConfig schedulingConfig;
/**
* The Google Cloud Platform Service Account to be used by the TPU node VMs. If None is specified,
* the default compute service account will be used.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ServiceAccount serviceAccount;
/**
* Shielded Instance options.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ShieldedInstanceConfig shieldedInstanceConfig;
/**
* Output only. The current state for the TPU Node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Output only. The Symptoms that have occurred to the TPU Node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List symptoms;
/**
* Tags to apply to the TPU Node. Tags are used to identify valid sources or targets for network
* firewalls.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tags;
/**
* The AccleratorConfig for the TPU Node.
* @return value or {@code null} for none
*/
public AcceleratorConfig getAcceleratorConfig() {
return acceleratorConfig;
}
/**
* The AccleratorConfig for the TPU Node.
* @param acceleratorConfig acceleratorConfig or {@code null} for none
*/
public Node setAcceleratorConfig(AcceleratorConfig acceleratorConfig) {
this.acceleratorConfig = acceleratorConfig;
return this;
}
/**
* Optional. The type of hardware accelerators associated with this node.
* @return value or {@code null} for none
*/
public java.lang.String getAcceleratorType() {
return acceleratorType;
}
/**
* Optional. The type of hardware accelerators associated with this node.
* @param acceleratorType acceleratorType or {@code null} for none
*/
public Node setAcceleratorType(java.lang.String acceleratorType) {
this.acceleratorType = acceleratorType;
return this;
}
/**
* Output only. The API version that created this Node.
* @return value or {@code null} for none
*/
public java.lang.String getApiVersion() {
return apiVersion;
}
/**
* Output only. The API version that created this Node.
* @param apiVersion apiVersion or {@code null} for none
*/
public Node setApiVersion(java.lang.String apiVersion) {
this.apiVersion = apiVersion;
return this;
}
/**
* The CIDR block that the TPU node will use when selecting an IP address. This CIDR block must be
* a /29 block; the Compute Engine networks API forbids a smaller block, and using a larger block
* would be wasteful (a node can only consume one IP address). Errors will occur if the CIDR block
* has already been used for a currently existing TPU node, the CIDR block conflicts with any
* subnetworks in the user's provided network, or the provided network is peered with another
* network that is using that CIDR block.
* @return value or {@code null} for none
*/
public java.lang.String getCidrBlock() {
return cidrBlock;
}
/**
* The CIDR block that the TPU node will use when selecting an IP address. This CIDR block must be
* a /29 block; the Compute Engine networks API forbids a smaller block, and using a larger block
* would be wasteful (a node can only consume one IP address). Errors will occur if the CIDR block
* has already been used for a currently existing TPU node, the CIDR block conflicts with any
* subnetworks in the user's provided network, or the provided network is peered with another
* network that is using that CIDR block.
* @param cidrBlock cidrBlock or {@code null} for none
*/
public Node setCidrBlock(java.lang.String cidrBlock) {
this.cidrBlock = cidrBlock;
return this;
}
/**
* Output only. The time when the node was created.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The time when the node was created.
* @param createTime createTime or {@code null} for none
*/
public Node setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* The additional data disks for the Node.
* @return value or {@code null} for none
*/
public java.util.List getDataDisks() {
return dataDisks;
}
/**
* The additional data disks for the Node.
* @param dataDisks dataDisks or {@code null} for none
*/
public Node setDataDisks(java.util.List dataDisks) {
this.dataDisks = dataDisks;
return this;
}
/**
* The user-supplied description of the TPU. Maximum of 512 characters.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* The user-supplied description of the TPU. Maximum of 512 characters.
* @param description description or {@code null} for none
*/
public Node setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The health status of the TPU node.
* @return value or {@code null} for none
*/
public java.lang.String getHealth() {
return health;
}
/**
* The health status of the TPU node.
* @param health health or {@code null} for none
*/
public Node setHealth(java.lang.String health) {
this.health = health;
return this;
}
/**
* Output only. If this field is populated, it contains a description of why the TPU Node is
* unhealthy.
* @return value or {@code null} for none
*/
public java.lang.String getHealthDescription() {
return healthDescription;
}
/**
* Output only. If this field is populated, it contains a description of why the TPU Node is
* unhealthy.
* @param healthDescription healthDescription or {@code null} for none
*/
public Node setHealthDescription(java.lang.String healthDescription) {
this.healthDescription = healthDescription;
return this;
}
/**
* Output only. The unique identifier for the TPU Node.
* @return value or {@code null} for none
*/
public java.lang.Long getId() {
return id;
}
/**
* Output only. The unique identifier for the TPU Node.
* @param id id or {@code null} for none
*/
public Node setId(java.lang.Long id) {
this.id = id;
return this;
}
/**
* Resource labels to represent user-provided metadata.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Resource labels to represent user-provided metadata.
* @param labels labels or {@code null} for none
*/
public Node setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Custom metadata to apply to the TPU Node. Can set startup-script and shutdown-script
* @return value or {@code null} for none
*/
public java.util.Map getMetadata() {
return metadata;
}
/**
* Custom metadata to apply to the TPU Node. Can set startup-script and shutdown-script
* @param metadata metadata or {@code null} for none
*/
public Node setMetadata(java.util.Map metadata) {
this.metadata = metadata;
return this;
}
/**
* Output only. Whether the Node belongs to a Multislice group.
* @return value or {@code null} for none
*/
public java.lang.Boolean getMultisliceNode() {
return multisliceNode;
}
/**
* Output only. Whether the Node belongs to a Multislice group.
* @param multisliceNode multisliceNode or {@code null} for none
*/
public Node setMultisliceNode(java.lang.Boolean multisliceNode) {
this.multisliceNode = multisliceNode;
return this;
}
/**
* Output only. Immutable. The name of the TPU.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Immutable. The name of the TPU.
* @param name name or {@code null} for none
*/
public Node setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Network configurations for the TPU node.
* @return value or {@code null} for none
*/
public NetworkConfig getNetworkConfig() {
return networkConfig;
}
/**
* Network configurations for the TPU node.
* @param networkConfig networkConfig or {@code null} for none
*/
public Node setNetworkConfig(NetworkConfig networkConfig) {
this.networkConfig = networkConfig;
return this;
}
/**
* Output only. The network endpoints where TPU workers can be accessed and sent work. It is
* recommended that runtime clients of the node reach out to the 0th entry in this map first.
* @return value or {@code null} for none
*/
public java.util.List getNetworkEndpoints() {
return networkEndpoints;
}
/**
* Output only. The network endpoints where TPU workers can be accessed and sent work. It is
* recommended that runtime clients of the node reach out to the 0th entry in this map first.
* @param networkEndpoints networkEndpoints or {@code null} for none
*/
public Node setNetworkEndpoints(java.util.List networkEndpoints) {
this.networkEndpoints = networkEndpoints;
return this;
}
/**
* Output only. The qualified name of the QueuedResource that requested this Node.
* @return value or {@code null} for none
*/
public java.lang.String getQueuedResource() {
return queuedResource;
}
/**
* Output only. The qualified name of the QueuedResource that requested this Node.
* @param queuedResource queuedResource or {@code null} for none
*/
public Node setQueuedResource(java.lang.String queuedResource) {
this.queuedResource = queuedResource;
return this;
}
/**
* Required. The runtime version running in the Node.
* @return value or {@code null} for none
*/
public java.lang.String getRuntimeVersion() {
return runtimeVersion;
}
/**
* Required. The runtime version running in the Node.
* @param runtimeVersion runtimeVersion or {@code null} for none
*/
public Node setRuntimeVersion(java.lang.String runtimeVersion) {
this.runtimeVersion = runtimeVersion;
return this;
}
/**
* The scheduling options for this node.
* @return value or {@code null} for none
*/
public SchedulingConfig getSchedulingConfig() {
return schedulingConfig;
}
/**
* The scheduling options for this node.
* @param schedulingConfig schedulingConfig or {@code null} for none
*/
public Node setSchedulingConfig(SchedulingConfig schedulingConfig) {
this.schedulingConfig = schedulingConfig;
return this;
}
/**
* The Google Cloud Platform Service Account to be used by the TPU node VMs. If None is specified,
* the default compute service account will be used.
* @return value or {@code null} for none
*/
public ServiceAccount getServiceAccount() {
return serviceAccount;
}
/**
* The Google Cloud Platform Service Account to be used by the TPU node VMs. If None is specified,
* the default compute service account will be used.
* @param serviceAccount serviceAccount or {@code null} for none
*/
public Node setServiceAccount(ServiceAccount serviceAccount) {
this.serviceAccount = serviceAccount;
return this;
}
/**
* Shielded Instance options.
* @return value or {@code null} for none
*/
public ShieldedInstanceConfig getShieldedInstanceConfig() {
return shieldedInstanceConfig;
}
/**
* Shielded Instance options.
* @param shieldedInstanceConfig shieldedInstanceConfig or {@code null} for none
*/
public Node setShieldedInstanceConfig(ShieldedInstanceConfig shieldedInstanceConfig) {
this.shieldedInstanceConfig = shieldedInstanceConfig;
return this;
}
/**
* Output only. The current state for the TPU Node.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. The current state for the TPU Node.
* @param state state or {@code null} for none
*/
public Node setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* Output only. The Symptoms that have occurred to the TPU Node.
* @return value or {@code null} for none
*/
public java.util.List getSymptoms() {
return symptoms;
}
/**
* Output only. The Symptoms that have occurred to the TPU Node.
* @param symptoms symptoms or {@code null} for none
*/
public Node setSymptoms(java.util.List symptoms) {
this.symptoms = symptoms;
return this;
}
/**
* Tags to apply to the TPU Node. Tags are used to identify valid sources or targets for network
* firewalls.
* @return value or {@code null} for none
*/
public java.util.List getTags() {
return tags;
}
/**
* Tags to apply to the TPU Node. Tags are used to identify valid sources or targets for network
* firewalls.
* @param tags tags or {@code null} for none
*/
public Node setTags(java.util.List tags) {
this.tags = tags;
return this;
}
@Override
public Node set(String fieldName, Object value) {
return (Node) super.set(fieldName, value);
}
@Override
public Node clone() {
return (Node) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy