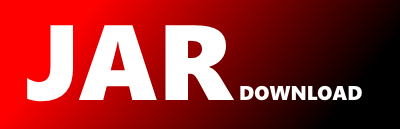
com.google.api.services.tpu.v2.model.QueuedResourceState Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.tpu.v2.model;
/**
* QueuedResourceState defines the details of the QueuedResource request.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud TPU API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class QueuedResourceState extends com.google.api.client.json.GenericJson {
/**
* Output only. Further data for the accepted state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AcceptedData acceptedData;
/**
* Output only. Further data for the active state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ActiveData activeData;
/**
* Output only. Further data for the creating state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CreatingData creatingData;
/**
* Output only. Further data for the deleting state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeletingData deletingData;
/**
* Output only. Further data for the failed state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FailedData failedData;
/**
* Output only. Further data for the provisioning state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProvisioningData provisioningData;
/**
* Output only. State of the QueuedResource request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Output only. The initiator of the QueuedResources's current state. Used to indicate whether the
* SUSPENDING/SUSPENDED state was initiated by the user or the service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String stateInitiator;
/**
* Output only. Further data for the suspended state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SuspendedData suspendedData;
/**
* Output only. Further data for the suspending state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SuspendingData suspendingData;
/**
* Output only. Further data for the accepted state.
* @return value or {@code null} for none
*/
public AcceptedData getAcceptedData() {
return acceptedData;
}
/**
* Output only. Further data for the accepted state.
* @param acceptedData acceptedData or {@code null} for none
*/
public QueuedResourceState setAcceptedData(AcceptedData acceptedData) {
this.acceptedData = acceptedData;
return this;
}
/**
* Output only. Further data for the active state.
* @return value or {@code null} for none
*/
public ActiveData getActiveData() {
return activeData;
}
/**
* Output only. Further data for the active state.
* @param activeData activeData or {@code null} for none
*/
public QueuedResourceState setActiveData(ActiveData activeData) {
this.activeData = activeData;
return this;
}
/**
* Output only. Further data for the creating state.
* @return value or {@code null} for none
*/
public CreatingData getCreatingData() {
return creatingData;
}
/**
* Output only. Further data for the creating state.
* @param creatingData creatingData or {@code null} for none
*/
public QueuedResourceState setCreatingData(CreatingData creatingData) {
this.creatingData = creatingData;
return this;
}
/**
* Output only. Further data for the deleting state.
* @return value or {@code null} for none
*/
public DeletingData getDeletingData() {
return deletingData;
}
/**
* Output only. Further data for the deleting state.
* @param deletingData deletingData or {@code null} for none
*/
public QueuedResourceState setDeletingData(DeletingData deletingData) {
this.deletingData = deletingData;
return this;
}
/**
* Output only. Further data for the failed state.
* @return value or {@code null} for none
*/
public FailedData getFailedData() {
return failedData;
}
/**
* Output only. Further data for the failed state.
* @param failedData failedData or {@code null} for none
*/
public QueuedResourceState setFailedData(FailedData failedData) {
this.failedData = failedData;
return this;
}
/**
* Output only. Further data for the provisioning state.
* @return value or {@code null} for none
*/
public ProvisioningData getProvisioningData() {
return provisioningData;
}
/**
* Output only. Further data for the provisioning state.
* @param provisioningData provisioningData or {@code null} for none
*/
public QueuedResourceState setProvisioningData(ProvisioningData provisioningData) {
this.provisioningData = provisioningData;
return this;
}
/**
* Output only. State of the QueuedResource request.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. State of the QueuedResource request.
* @param state state or {@code null} for none
*/
public QueuedResourceState setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* Output only. The initiator of the QueuedResources's current state. Used to indicate whether the
* SUSPENDING/SUSPENDED state was initiated by the user or the service.
* @return value or {@code null} for none
*/
public java.lang.String getStateInitiator() {
return stateInitiator;
}
/**
* Output only. The initiator of the QueuedResources's current state. Used to indicate whether the
* SUSPENDING/SUSPENDED state was initiated by the user or the service.
* @param stateInitiator stateInitiator or {@code null} for none
*/
public QueuedResourceState setStateInitiator(java.lang.String stateInitiator) {
this.stateInitiator = stateInitiator;
return this;
}
/**
* Output only. Further data for the suspended state.
* @return value or {@code null} for none
*/
public SuspendedData getSuspendedData() {
return suspendedData;
}
/**
* Output only. Further data for the suspended state.
* @param suspendedData suspendedData or {@code null} for none
*/
public QueuedResourceState setSuspendedData(SuspendedData suspendedData) {
this.suspendedData = suspendedData;
return this;
}
/**
* Output only. Further data for the suspending state.
* @return value or {@code null} for none
*/
public SuspendingData getSuspendingData() {
return suspendingData;
}
/**
* Output only. Further data for the suspending state.
* @param suspendingData suspendingData or {@code null} for none
*/
public QueuedResourceState setSuspendingData(SuspendingData suspendingData) {
this.suspendingData = suspendingData;
return this;
}
@Override
public QueuedResourceState set(String fieldName, Object value) {
return (QueuedResourceState) super.set(fieldName, value);
}
@Override
public QueuedResourceState clone() {
return (QueuedResourceState) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy