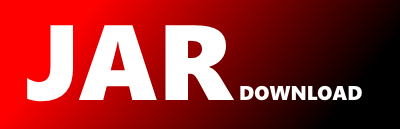
com.google.api.services.tracing.v2.model.StackFrame Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-05-10 at 21:58:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.tracing.v2.model;
/**
* Represents a single stack frame in a stack trace.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Tracing API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class StackFrame extends com.google.api.client.json.GenericJson {
/**
* Column number is important in JavaScript (anonymous functions). May not be available in some
* languages.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long columnNumber;
/**
* The filename of the file containing this frame (up to 256 characters).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TruncatableString fileName;
/**
* The fully-qualified name that uniquely identifies this function or method (up to 1024
* characters).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TruncatableString functionName;
/**
* Line number of the frame.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long lineNumber;
/**
* Binary module the code is loaded from.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Module loadModule;
/**
* Used when the function name is [mangled](http://www.avabodh.com/cxxin/namemangling.html). May
* be fully-qualified (up to 1024 characters).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TruncatableString originalFunctionName;
/**
* The version of the deployed source code (up to 128 characters).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TruncatableString sourceVersion;
/**
* Column number is important in JavaScript (anonymous functions). May not be available in some
* languages.
* @return value or {@code null} for none
*/
public java.lang.Long getColumnNumber() {
return columnNumber;
}
/**
* Column number is important in JavaScript (anonymous functions). May not be available in some
* languages.
* @param columnNumber columnNumber or {@code null} for none
*/
public StackFrame setColumnNumber(java.lang.Long columnNumber) {
this.columnNumber = columnNumber;
return this;
}
/**
* The filename of the file containing this frame (up to 256 characters).
* @return value or {@code null} for none
*/
public TruncatableString getFileName() {
return fileName;
}
/**
* The filename of the file containing this frame (up to 256 characters).
* @param fileName fileName or {@code null} for none
*/
public StackFrame setFileName(TruncatableString fileName) {
this.fileName = fileName;
return this;
}
/**
* The fully-qualified name that uniquely identifies this function or method (up to 1024
* characters).
* @return value or {@code null} for none
*/
public TruncatableString getFunctionName() {
return functionName;
}
/**
* The fully-qualified name that uniquely identifies this function or method (up to 1024
* characters).
* @param functionName functionName or {@code null} for none
*/
public StackFrame setFunctionName(TruncatableString functionName) {
this.functionName = functionName;
return this;
}
/**
* Line number of the frame.
* @return value or {@code null} for none
*/
public java.lang.Long getLineNumber() {
return lineNumber;
}
/**
* Line number of the frame.
* @param lineNumber lineNumber or {@code null} for none
*/
public StackFrame setLineNumber(java.lang.Long lineNumber) {
this.lineNumber = lineNumber;
return this;
}
/**
* Binary module the code is loaded from.
* @return value or {@code null} for none
*/
public Module getLoadModule() {
return loadModule;
}
/**
* Binary module the code is loaded from.
* @param loadModule loadModule or {@code null} for none
*/
public StackFrame setLoadModule(Module loadModule) {
this.loadModule = loadModule;
return this;
}
/**
* Used when the function name is [mangled](http://www.avabodh.com/cxxin/namemangling.html). May
* be fully-qualified (up to 1024 characters).
* @return value or {@code null} for none
*/
public TruncatableString getOriginalFunctionName() {
return originalFunctionName;
}
/**
* Used when the function name is [mangled](http://www.avabodh.com/cxxin/namemangling.html). May
* be fully-qualified (up to 1024 characters).
* @param originalFunctionName originalFunctionName or {@code null} for none
*/
public StackFrame setOriginalFunctionName(TruncatableString originalFunctionName) {
this.originalFunctionName = originalFunctionName;
return this;
}
/**
* The version of the deployed source code (up to 128 characters).
* @return value or {@code null} for none
*/
public TruncatableString getSourceVersion() {
return sourceVersion;
}
/**
* The version of the deployed source code (up to 128 characters).
* @param sourceVersion sourceVersion or {@code null} for none
*/
public StackFrame setSourceVersion(TruncatableString sourceVersion) {
this.sourceVersion = sourceVersion;
return this;
}
@Override
public StackFrame set(String fieldName, Object value) {
return (StackFrame) super.set(fieldName, value);
}
@Override
public StackFrame clone() {
return (StackFrame) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy