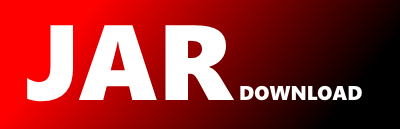
com.google.api.services.tracing.v2.model.Span Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2017-02-15 17:18:02 UTC)
* on 2017-05-17 at 20:21:11 UTC
* Modify at your own risk.
*/
package com.google.api.services.tracing.v2.model;
/**
* A span represents a single operation within a trace. Spans can be nested to form a trace tree.
* Often, a trace contains a root span that describes the end-to-end latency and, optionally, one or
* more subspans for its sub-operations. (A trace could alternatively contain multiple root spans,
* or none at all.) Spans do not need to be contiguous. There may be gaps and/or overlaps between
* spans in a trace.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Tracing API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Span extends com.google.api.client.json.GenericJson {
/**
* A set of attributes on the span. A maximum of 32 attributes are allowed per Span.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Attributes attributes;
/**
* Description of the operation in the span. It is sanitized and displayed in the Stackdriver
* Trace tool in the {% dynamic print site_values.console_name %}. The display_name may be a
* method name or some other per-call site name. For the same executable and the same call point,
* a best practice is to use a consistent operation name, which makes it easier to correlate
* cross-trace spans. The maximum length for the display_name is 128 bytes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TruncatableString displayName;
/**
* End time of the span. On the client side, this is the local machine clock time at which the
* span execution was ended; on the server side, this is the time at which the server application
* handler stopped running.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String endTime;
/**
* A maximum of 128 links are allowed per Span.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Links links;
/**
* The resource name of Span in the format `projects/PROJECT_ID/traces/TRACE_ID/spans/SPAN_ID`.
* `TRACE_ID` is a unique identifier for a trace within a project and is a base16-encoded, case-
* insensitive string and is required to be 32 char long. `SPAN_ID` is a unique identifier for a
* span within a trace. It is a base 16-encoded, case-insensitive string of a 8-bytes array and is
* required to be 16 char long.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* ID of parent span which is a base 16-encoded, case-insensitive string of a 8-bytes array and is
* required to be 16 char long. If this is a root span, the value must be empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String parentSpanId;
/**
* Unique identifier for a span within a trace. It is a base 16-encoded, case-insensitive string
* of a 8-bytes array and is required.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String spanId;
/**
* Stack trace captured at the start of the span.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private StackTrace stackTrace;
/**
* Start time of the span. On the client side, this is the local machine clock time at which the
* span execution was started; on the server side, this is the time at which the server
* application handler started running.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String startTime;
/**
* An optional final status for this span.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Status status;
/**
* A maximum of 32 annotations and 128 network events are allowed per Span.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TimeEvents timeEvents;
/**
* A set of attributes on the span. A maximum of 32 attributes are allowed per Span.
* @return value or {@code null} for none
*/
public Attributes getAttributes() {
return attributes;
}
/**
* A set of attributes on the span. A maximum of 32 attributes are allowed per Span.
* @param attributes attributes or {@code null} for none
*/
public Span setAttributes(Attributes attributes) {
this.attributes = attributes;
return this;
}
/**
* Description of the operation in the span. It is sanitized and displayed in the Stackdriver
* Trace tool in the {% dynamic print site_values.console_name %}. The display_name may be a
* method name or some other per-call site name. For the same executable and the same call point,
* a best practice is to use a consistent operation name, which makes it easier to correlate
* cross-trace spans. The maximum length for the display_name is 128 bytes.
* @return value or {@code null} for none
*/
public TruncatableString getDisplayName() {
return displayName;
}
/**
* Description of the operation in the span. It is sanitized and displayed in the Stackdriver
* Trace tool in the {% dynamic print site_values.console_name %}. The display_name may be a
* method name or some other per-call site name. For the same executable and the same call point,
* a best practice is to use a consistent operation name, which makes it easier to correlate
* cross-trace spans. The maximum length for the display_name is 128 bytes.
* @param displayName displayName or {@code null} for none
*/
public Span setDisplayName(TruncatableString displayName) {
this.displayName = displayName;
return this;
}
/**
* End time of the span. On the client side, this is the local machine clock time at which the
* span execution was ended; on the server side, this is the time at which the server application
* handler stopped running.
* @return value or {@code null} for none
*/
public String getEndTime() {
return endTime;
}
/**
* End time of the span. On the client side, this is the local machine clock time at which the
* span execution was ended; on the server side, this is the time at which the server application
* handler stopped running.
* @param endTime endTime or {@code null} for none
*/
public Span setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
/**
* A maximum of 128 links are allowed per Span.
* @return value or {@code null} for none
*/
public Links getLinks() {
return links;
}
/**
* A maximum of 128 links are allowed per Span.
* @param links links or {@code null} for none
*/
public Span setLinks(Links links) {
this.links = links;
return this;
}
/**
* The resource name of Span in the format `projects/PROJECT_ID/traces/TRACE_ID/spans/SPAN_ID`.
* `TRACE_ID` is a unique identifier for a trace within a project and is a base16-encoded, case-
* insensitive string and is required to be 32 char long. `SPAN_ID` is a unique identifier for a
* span within a trace. It is a base 16-encoded, case-insensitive string of a 8-bytes array and is
* required to be 16 char long.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of Span in the format `projects/PROJECT_ID/traces/TRACE_ID/spans/SPAN_ID`.
* `TRACE_ID` is a unique identifier for a trace within a project and is a base16-encoded, case-
* insensitive string and is required to be 32 char long. `SPAN_ID` is a unique identifier for a
* span within a trace. It is a base 16-encoded, case-insensitive string of a 8-bytes array and is
* required to be 16 char long.
* @param name name or {@code null} for none
*/
public Span setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* ID of parent span which is a base 16-encoded, case-insensitive string of a 8-bytes array and is
* required to be 16 char long. If this is a root span, the value must be empty.
* @return value or {@code null} for none
*/
public java.lang.String getParentSpanId() {
return parentSpanId;
}
/**
* ID of parent span which is a base 16-encoded, case-insensitive string of a 8-bytes array and is
* required to be 16 char long. If this is a root span, the value must be empty.
* @param parentSpanId parentSpanId or {@code null} for none
*/
public Span setParentSpanId(java.lang.String parentSpanId) {
this.parentSpanId = parentSpanId;
return this;
}
/**
* Unique identifier for a span within a trace. It is a base 16-encoded, case-insensitive string
* of a 8-bytes array and is required.
* @return value or {@code null} for none
*/
public java.lang.String getSpanId() {
return spanId;
}
/**
* Unique identifier for a span within a trace. It is a base 16-encoded, case-insensitive string
* of a 8-bytes array and is required.
* @param spanId spanId or {@code null} for none
*/
public Span setSpanId(java.lang.String spanId) {
this.spanId = spanId;
return this;
}
/**
* Stack trace captured at the start of the span.
* @return value or {@code null} for none
*/
public StackTrace getStackTrace() {
return stackTrace;
}
/**
* Stack trace captured at the start of the span.
* @param stackTrace stackTrace or {@code null} for none
*/
public Span setStackTrace(StackTrace stackTrace) {
this.stackTrace = stackTrace;
return this;
}
/**
* Start time of the span. On the client side, this is the local machine clock time at which the
* span execution was started; on the server side, this is the time at which the server
* application handler started running.
* @return value or {@code null} for none
*/
public String getStartTime() {
return startTime;
}
/**
* Start time of the span. On the client side, this is the local machine clock time at which the
* span execution was started; on the server side, this is the time at which the server
* application handler started running.
* @param startTime startTime or {@code null} for none
*/
public Span setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
/**
* An optional final status for this span.
* @return value or {@code null} for none
*/
public Status getStatus() {
return status;
}
/**
* An optional final status for this span.
* @param status status or {@code null} for none
*/
public Span setStatus(Status status) {
this.status = status;
return this;
}
/**
* A maximum of 32 annotations and 128 network events are allowed per Span.
* @return value or {@code null} for none
*/
public TimeEvents getTimeEvents() {
return timeEvents;
}
/**
* A maximum of 32 annotations and 128 network events are allowed per Span.
* @param timeEvents timeEvents or {@code null} for none
*/
public Span setTimeEvents(TimeEvents timeEvents) {
this.timeEvents = timeEvents;
return this;
}
@Override
public Span set(String fieldName, Object value) {
return (Span) super.set(fieldName, value);
}
@Override
public Span clone() {
return (Span) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy