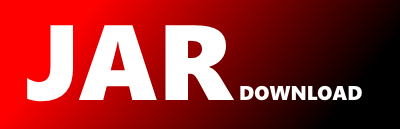
com.google.api.services.translate.v3.model.BatchTranslateDocumentRequest Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.translate.v3.model;
/**
* The BatchTranslateDocument request.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Translation API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class BatchTranslateDocumentRequest extends com.google.api.client.json.GenericJson {
/**
* Optional. This flag is to support user customized attribution. If not provided, the default is
* `Machine Translated by Google`. Customized attribution should follow rules in
* https://cloud.google.com/translate/attribution#attribution_and_logos
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customizedAttribution;
/**
* Optional. If true, enable auto rotation correction in DVS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableRotationCorrection;
/**
* Optional. If true, use the text removal server to remove the shadow text on background image
* for native pdf translation. Shadow removal feature can only be enabled when
* is_translate_native_pdf_only: false && pdf_native_only: false
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableShadowRemovalNativePdf;
/**
* Optional. The file format conversion map that is applied to all input files. The map key is the
* original mime_type. The map value is the target mime_type of translated documents. Supported
* file format conversion includes: - `application/pdf` to `application/vnd.openxmlformats-
* officedocument.wordprocessingml.document` If nothing specified, output files will be in the
* same format as the original file.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map formatConversions;
/**
* Optional. Glossaries to be applied. It's keyed by target language code.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map glossaries;
/**
* Required. Input configurations. The total number of files matched should be <= 100. The total
* content size to translate should be <= 100M Unicode codepoints. The files must use UTF-8
* encoding.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List inputConfigs;
static {
// hack to force ProGuard to consider BatchDocumentInputConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(BatchDocumentInputConfig.class);
}
/**
* Optional. The models to use for translation. Map's key is target language code. Map's value is
* the model name. Value can be a built-in general model, or an AutoML Translation model. The
* value format depends on model type: - AutoML Translation models: `projects/{project-number-or-
* id}/locations/{location-id}/models/{model-id}` - General (built-in) models: `projects/{project-
* number-or-id}/locations/{location-id}/models/general/nmt`, If the map is empty or a specific
* model is not requested for a language pair, then default google model (nmt) is used.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map models;
/**
* Required. Output configuration. If 2 input configs match to the same file (that is, same input
* path), we don't generate output for duplicate inputs.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BatchDocumentOutputConfig outputConfig;
/**
* Required. The ISO-639 language code of the input document if known, for example, "en-US" or
* "sr-Latn". Supported language codes are listed in [Language
* Support](https://cloud.google.com/translate/docs/languages).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sourceLanguageCode;
/**
* Required. The ISO-639 language code to use for translation of the input document. Specify up to
* 10 language codes here.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List targetLanguageCodes;
/**
* Optional. This flag is to support user customized attribution. If not provided, the default is
* `Machine Translated by Google`. Customized attribution should follow rules in
* https://cloud.google.com/translate/attribution#attribution_and_logos
* @return value or {@code null} for none
*/
public java.lang.String getCustomizedAttribution() {
return customizedAttribution;
}
/**
* Optional. This flag is to support user customized attribution. If not provided, the default is
* `Machine Translated by Google`. Customized attribution should follow rules in
* https://cloud.google.com/translate/attribution#attribution_and_logos
* @param customizedAttribution customizedAttribution or {@code null} for none
*/
public BatchTranslateDocumentRequest setCustomizedAttribution(java.lang.String customizedAttribution) {
this.customizedAttribution = customizedAttribution;
return this;
}
/**
* Optional. If true, enable auto rotation correction in DVS.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnableRotationCorrection() {
return enableRotationCorrection;
}
/**
* Optional. If true, enable auto rotation correction in DVS.
* @param enableRotationCorrection enableRotationCorrection or {@code null} for none
*/
public BatchTranslateDocumentRequest setEnableRotationCorrection(java.lang.Boolean enableRotationCorrection) {
this.enableRotationCorrection = enableRotationCorrection;
return this;
}
/**
* Optional. If true, use the text removal server to remove the shadow text on background image
* for native pdf translation. Shadow removal feature can only be enabled when
* is_translate_native_pdf_only: false && pdf_native_only: false
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnableShadowRemovalNativePdf() {
return enableShadowRemovalNativePdf;
}
/**
* Optional. If true, use the text removal server to remove the shadow text on background image
* for native pdf translation. Shadow removal feature can only be enabled when
* is_translate_native_pdf_only: false && pdf_native_only: false
* @param enableShadowRemovalNativePdf enableShadowRemovalNativePdf or {@code null} for none
*/
public BatchTranslateDocumentRequest setEnableShadowRemovalNativePdf(java.lang.Boolean enableShadowRemovalNativePdf) {
this.enableShadowRemovalNativePdf = enableShadowRemovalNativePdf;
return this;
}
/**
* Optional. The file format conversion map that is applied to all input files. The map key is the
* original mime_type. The map value is the target mime_type of translated documents. Supported
* file format conversion includes: - `application/pdf` to `application/vnd.openxmlformats-
* officedocument.wordprocessingml.document` If nothing specified, output files will be in the
* same format as the original file.
* @return value or {@code null} for none
*/
public java.util.Map getFormatConversions() {
return formatConversions;
}
/**
* Optional. The file format conversion map that is applied to all input files. The map key is the
* original mime_type. The map value is the target mime_type of translated documents. Supported
* file format conversion includes: - `application/pdf` to `application/vnd.openxmlformats-
* officedocument.wordprocessingml.document` If nothing specified, output files will be in the
* same format as the original file.
* @param formatConversions formatConversions or {@code null} for none
*/
public BatchTranslateDocumentRequest setFormatConversions(java.util.Map formatConversions) {
this.formatConversions = formatConversions;
return this;
}
/**
* Optional. Glossaries to be applied. It's keyed by target language code.
* @return value or {@code null} for none
*/
public java.util.Map getGlossaries() {
return glossaries;
}
/**
* Optional. Glossaries to be applied. It's keyed by target language code.
* @param glossaries glossaries or {@code null} for none
*/
public BatchTranslateDocumentRequest setGlossaries(java.util.Map glossaries) {
this.glossaries = glossaries;
return this;
}
/**
* Required. Input configurations. The total number of files matched should be <= 100. The total
* content size to translate should be <= 100M Unicode codepoints. The files must use UTF-8
* encoding.
* @return value or {@code null} for none
*/
public java.util.List getInputConfigs() {
return inputConfigs;
}
/**
* Required. Input configurations. The total number of files matched should be <= 100. The total
* content size to translate should be <= 100M Unicode codepoints. The files must use UTF-8
* encoding.
* @param inputConfigs inputConfigs or {@code null} for none
*/
public BatchTranslateDocumentRequest setInputConfigs(java.util.List inputConfigs) {
this.inputConfigs = inputConfigs;
return this;
}
/**
* Optional. The models to use for translation. Map's key is target language code. Map's value is
* the model name. Value can be a built-in general model, or an AutoML Translation model. The
* value format depends on model type: - AutoML Translation models: `projects/{project-number-or-
* id}/locations/{location-id}/models/{model-id}` - General (built-in) models: `projects/{project-
* number-or-id}/locations/{location-id}/models/general/nmt`, If the map is empty or a specific
* model is not requested for a language pair, then default google model (nmt) is used.
* @return value or {@code null} for none
*/
public java.util.Map getModels() {
return models;
}
/**
* Optional. The models to use for translation. Map's key is target language code. Map's value is
* the model name. Value can be a built-in general model, or an AutoML Translation model. The
* value format depends on model type: - AutoML Translation models: `projects/{project-number-or-
* id}/locations/{location-id}/models/{model-id}` - General (built-in) models: `projects/{project-
* number-or-id}/locations/{location-id}/models/general/nmt`, If the map is empty or a specific
* model is not requested for a language pair, then default google model (nmt) is used.
* @param models models or {@code null} for none
*/
public BatchTranslateDocumentRequest setModels(java.util.Map models) {
this.models = models;
return this;
}
/**
* Required. Output configuration. If 2 input configs match to the same file (that is, same input
* path), we don't generate output for duplicate inputs.
* @return value or {@code null} for none
*/
public BatchDocumentOutputConfig getOutputConfig() {
return outputConfig;
}
/**
* Required. Output configuration. If 2 input configs match to the same file (that is, same input
* path), we don't generate output for duplicate inputs.
* @param outputConfig outputConfig or {@code null} for none
*/
public BatchTranslateDocumentRequest setOutputConfig(BatchDocumentOutputConfig outputConfig) {
this.outputConfig = outputConfig;
return this;
}
/**
* Required. The ISO-639 language code of the input document if known, for example, "en-US" or
* "sr-Latn". Supported language codes are listed in [Language
* Support](https://cloud.google.com/translate/docs/languages).
* @return value or {@code null} for none
*/
public java.lang.String getSourceLanguageCode() {
return sourceLanguageCode;
}
/**
* Required. The ISO-639 language code of the input document if known, for example, "en-US" or
* "sr-Latn". Supported language codes are listed in [Language
* Support](https://cloud.google.com/translate/docs/languages).
* @param sourceLanguageCode sourceLanguageCode or {@code null} for none
*/
public BatchTranslateDocumentRequest setSourceLanguageCode(java.lang.String sourceLanguageCode) {
this.sourceLanguageCode = sourceLanguageCode;
return this;
}
/**
* Required. The ISO-639 language code to use for translation of the input document. Specify up to
* 10 language codes here.
* @return value or {@code null} for none
*/
public java.util.List getTargetLanguageCodes() {
return targetLanguageCodes;
}
/**
* Required. The ISO-639 language code to use for translation of the input document. Specify up to
* 10 language codes here.
* @param targetLanguageCodes targetLanguageCodes or {@code null} for none
*/
public BatchTranslateDocumentRequest setTargetLanguageCodes(java.util.List targetLanguageCodes) {
this.targetLanguageCodes = targetLanguageCodes;
return this;
}
@Override
public BatchTranslateDocumentRequest set(String fieldName, Object value) {
return (BatchTranslateDocumentRequest) super.set(fieldName, value);
}
@Override
public BatchTranslateDocumentRequest clone() {
return (BatchTranslateDocumentRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy