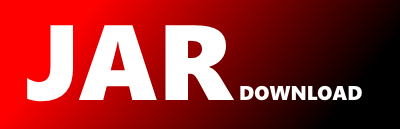
com.google.api.services.vision.v1.model.EntityAnnotation Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-07-26 at 19:10:38 UTC
* Modify at your own risk.
*/
package com.google.api.services.vision.v1.model;
/**
* Set of detected entity features.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Cloud Vision API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class EntityAnnotation extends com.google.api.client.json.GenericJson {
/**
* Image region to which this entity belongs. Not filled currently for `LABEL_DETECTION` features.
* For `TEXT_DETECTION` (OCR), `boundingPoly`s are produced for the entire text detected in an
* image region, followed by `boundingPoly`s for each word within the detected text.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BoundingPoly boundingPoly;
/**
* The accuracy of the entity detection in an image. For example, for an image containing 'Eiffel
* Tower,' this field represents the confidence that there is a tower in the query image. Range
* [0, 1].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float confidence;
/**
* Entity textual description, expressed in its locale language.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The language code for the locale in which the entity textual description (next field) is
* expressed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String locale;
/**
* The location information for the detected entity. Multiple LocationInfo elements can be present
* since one location may indicate the location of the scene in the query image, and another the
* location of the place where the query image was taken. Location information is usually present
* for landmarks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List locations;
/**
* Opaque entity ID. Some IDs might be available in Knowledge Graph(KG). For more details on KG
* please see: https://developers.google.com/knowledge-graph/
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mid;
/**
* Some entities can have additional optional Property fields. For example a different kind of
* score or string that qualifies the entity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List properties;
/**
* Overall score of the result. Range [0, 1].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float score;
/**
* The relevancy of the ICA (Image Content Annotation) label to the image. For example, the
* relevancy of 'tower' to an image containing 'Eiffel Tower' is likely higher than an image
* containing a distant towering building, though the confidence that there is a tower may be the
* same. Range [0, 1].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float topicality;
/**
* Image region to which this entity belongs. Not filled currently for `LABEL_DETECTION` features.
* For `TEXT_DETECTION` (OCR), `boundingPoly`s are produced for the entire text detected in an
* image region, followed by `boundingPoly`s for each word within the detected text.
* @return value or {@code null} for none
*/
public BoundingPoly getBoundingPoly() {
return boundingPoly;
}
/**
* Image region to which this entity belongs. Not filled currently for `LABEL_DETECTION` features.
* For `TEXT_DETECTION` (OCR), `boundingPoly`s are produced for the entire text detected in an
* image region, followed by `boundingPoly`s for each word within the detected text.
* @param boundingPoly boundingPoly or {@code null} for none
*/
public EntityAnnotation setBoundingPoly(BoundingPoly boundingPoly) {
this.boundingPoly = boundingPoly;
return this;
}
/**
* The accuracy of the entity detection in an image. For example, for an image containing 'Eiffel
* Tower,' this field represents the confidence that there is a tower in the query image. Range
* [0, 1].
* @return value or {@code null} for none
*/
public java.lang.Float getConfidence() {
return confidence;
}
/**
* The accuracy of the entity detection in an image. For example, for an image containing 'Eiffel
* Tower,' this field represents the confidence that there is a tower in the query image. Range
* [0, 1].
* @param confidence confidence or {@code null} for none
*/
public EntityAnnotation setConfidence(java.lang.Float confidence) {
this.confidence = confidence;
return this;
}
/**
* Entity textual description, expressed in its locale language.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Entity textual description, expressed in its locale language.
* @param description description or {@code null} for none
*/
public EntityAnnotation setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The language code for the locale in which the entity textual description (next field) is
* expressed.
* @return value or {@code null} for none
*/
public java.lang.String getLocale() {
return locale;
}
/**
* The language code for the locale in which the entity textual description (next field) is
* expressed.
* @param locale locale or {@code null} for none
*/
public EntityAnnotation setLocale(java.lang.String locale) {
this.locale = locale;
return this;
}
/**
* The location information for the detected entity. Multiple LocationInfo elements can be present
* since one location may indicate the location of the scene in the query image, and another the
* location of the place where the query image was taken. Location information is usually present
* for landmarks.
* @return value or {@code null} for none
*/
public java.util.List getLocations() {
return locations;
}
/**
* The location information for the detected entity. Multiple LocationInfo elements can be present
* since one location may indicate the location of the scene in the query image, and another the
* location of the place where the query image was taken. Location information is usually present
* for landmarks.
* @param locations locations or {@code null} for none
*/
public EntityAnnotation setLocations(java.util.List locations) {
this.locations = locations;
return this;
}
/**
* Opaque entity ID. Some IDs might be available in Knowledge Graph(KG). For more details on KG
* please see: https://developers.google.com/knowledge-graph/
* @return value or {@code null} for none
*/
public java.lang.String getMid() {
return mid;
}
/**
* Opaque entity ID. Some IDs might be available in Knowledge Graph(KG). For more details on KG
* please see: https://developers.google.com/knowledge-graph/
* @param mid mid or {@code null} for none
*/
public EntityAnnotation setMid(java.lang.String mid) {
this.mid = mid;
return this;
}
/**
* Some entities can have additional optional Property fields. For example a different kind of
* score or string that qualifies the entity.
* @return value or {@code null} for none
*/
public java.util.List getProperties() {
return properties;
}
/**
* Some entities can have additional optional Property fields. For example a different kind of
* score or string that qualifies the entity.
* @param properties properties or {@code null} for none
*/
public EntityAnnotation setProperties(java.util.List properties) {
this.properties = properties;
return this;
}
/**
* Overall score of the result. Range [0, 1].
* @return value or {@code null} for none
*/
public java.lang.Float getScore() {
return score;
}
/**
* Overall score of the result. Range [0, 1].
* @param score score or {@code null} for none
*/
public EntityAnnotation setScore(java.lang.Float score) {
this.score = score;
return this;
}
/**
* The relevancy of the ICA (Image Content Annotation) label to the image. For example, the
* relevancy of 'tower' to an image containing 'Eiffel Tower' is likely higher than an image
* containing a distant towering building, though the confidence that there is a tower may be the
* same. Range [0, 1].
* @return value or {@code null} for none
*/
public java.lang.Float getTopicality() {
return topicality;
}
/**
* The relevancy of the ICA (Image Content Annotation) label to the image. For example, the
* relevancy of 'tower' to an image containing 'Eiffel Tower' is likely higher than an image
* containing a distant towering building, though the confidence that there is a tower may be the
* same. Range [0, 1].
* @param topicality topicality or {@code null} for none
*/
public EntityAnnotation setTopicality(java.lang.Float topicality) {
this.topicality = topicality;
return this;
}
@Override
public EntityAnnotation set(String fieldName, Object value) {
return (EntityAnnotation) super.set(fieldName, value);
}
@Override
public EntityAnnotation clone() {
return (EntityAnnotation) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy