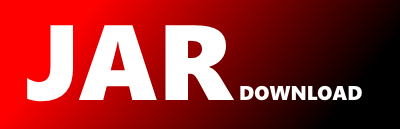
com.google.api.services.vmwareengine.v1.VMwareEngine Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.vmwareengine.v1;
/**
* Service definition for VMwareEngine (v1).
*
*
* The Google VMware Engine API lets you programmatically manage VMware environments.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link VMwareEngineRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class VMwareEngine extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the VMware Engine API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://vmwareengine.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://vmwareengine.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public VMwareEngine(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
VMwareEngine(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.Projects.List request = vmwareengine.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.Locations.List request = vmwareengine.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets all the principals having bind permission on the intranet VPC associated with the consumer
* project granted by the Grant API. DnsBindPermission is a global resource and location can only be
* global.
*
* Create a request for the method "locations.getDnsBindPermission".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link GetDnsBindPermission#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the resource which stores the users/service accounts having the permission to
* bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a
* global resource. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/dnsBindPermission`
* @return the request
*/
public GetDnsBindPermission getDnsBindPermission(java.lang.String name) throws java.io.IOException {
GetDnsBindPermission result = new GetDnsBindPermission(name);
initialize(result);
return result;
}
public class GetDnsBindPermission extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
/**
* Gets all the principals having bind permission on the intranet VPC associated with the consumer
* project granted by the Grant API. DnsBindPermission is a global resource and location can only
* be global.
*
* Create a request for the method "locations.getDnsBindPermission".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link GetDnsBindPermission#execute()} method to invoke the
* remote operation. {@link GetDnsBindPermission#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. The name of the resource which stores the users/service accounts having the permission to
* bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a
* global resource. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/dnsBindPermission`
* @since 1.13
*/
protected GetDnsBindPermission(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.DnsBindPermission.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetDnsBindPermission set$Xgafv(java.lang.String $Xgafv) {
return (GetDnsBindPermission) super.set$Xgafv($Xgafv);
}
@Override
public GetDnsBindPermission setAccessToken(java.lang.String accessToken) {
return (GetDnsBindPermission) super.setAccessToken(accessToken);
}
@Override
public GetDnsBindPermission setAlt(java.lang.String alt) {
return (GetDnsBindPermission) super.setAlt(alt);
}
@Override
public GetDnsBindPermission setCallback(java.lang.String callback) {
return (GetDnsBindPermission) super.setCallback(callback);
}
@Override
public GetDnsBindPermission setFields(java.lang.String fields) {
return (GetDnsBindPermission) super.setFields(fields);
}
@Override
public GetDnsBindPermission setKey(java.lang.String key) {
return (GetDnsBindPermission) super.setKey(key);
}
@Override
public GetDnsBindPermission setOauthToken(java.lang.String oauthToken) {
return (GetDnsBindPermission) super.setOauthToken(oauthToken);
}
@Override
public GetDnsBindPermission setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetDnsBindPermission) super.setPrettyPrint(prettyPrint);
}
@Override
public GetDnsBindPermission setQuotaUser(java.lang.String quotaUser) {
return (GetDnsBindPermission) super.setQuotaUser(quotaUser);
}
@Override
public GetDnsBindPermission setUploadType(java.lang.String uploadType) {
return (GetDnsBindPermission) super.setUploadType(uploadType);
}
@Override
public GetDnsBindPermission setUploadProtocol(java.lang.String uploadProtocol) {
return (GetDnsBindPermission) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource which stores the users/service accounts having the
* permission to bind to the corresponding intranet VPC of the consumer project.
* DnsBindPermission is a global resource. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/global/dnsBindPermission`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the resource which stores the users/service accounts having the permission to
bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a global
resource. Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/global/dnsBindPermission`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource which stores the users/service accounts having the
* permission to bind to the corresponding intranet VPC of the consumer project.
* DnsBindPermission is a global resource. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/global/dnsBindPermission`
*/
public GetDnsBindPermission setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
}
this.name = name;
return this;
}
@Override
public GetDnsBindPermission set(String parameterName, Object value) {
return (GetDnsBindPermission) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
`"displayName=tokyo"`, and is documented in more detail in [AIP-160](https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the `next_page_token` field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the DnsBindPermission collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.DnsBindPermission.List request = vmwareengine.dnsBindPermission().list(parameters ...)}
*
*
* @return the resource collection
*/
public DnsBindPermission dnsBindPermission() {
return new DnsBindPermission();
}
/**
* The "dnsBindPermission" collection of methods.
*/
public class DnsBindPermission {
/**
* Grants the bind permission to the customer provided principal(user / service account) to bind
* their DNS zone with the intranet VPC associated with the project. DnsBindPermission is a global
* resource and location can only be global.
*
* Create a request for the method "dnsBindPermission.grant".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Grant#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the resource which stores the users/service accounts having the permission to
* bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a
* global resource. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/dnsBindPermission`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.GrantDnsBindPermissionRequest}
* @return the request
*/
public Grant grant(java.lang.String name, com.google.api.services.vmwareengine.v1.model.GrantDnsBindPermissionRequest content) throws java.io.IOException {
Grant result = new Grant(name, content);
initialize(result);
return result;
}
public class Grant extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}:grant";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
/**
* Grants the bind permission to the customer provided principal(user / service account) to bind
* their DNS zone with the intranet VPC associated with the project. DnsBindPermission is a global
* resource and location can only be global.
*
* Create a request for the method "dnsBindPermission.grant".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Grant#execute()} method to invoke the remote operation.
* {@link
* Grant#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the resource which stores the users/service accounts having the permission to
* bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a
* global resource. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/dnsBindPermission`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.GrantDnsBindPermissionRequest}
* @since 1.13
*/
protected Grant(java.lang.String name, com.google.api.services.vmwareengine.v1.model.GrantDnsBindPermissionRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
}
}
@Override
public Grant set$Xgafv(java.lang.String $Xgafv) {
return (Grant) super.set$Xgafv($Xgafv);
}
@Override
public Grant setAccessToken(java.lang.String accessToken) {
return (Grant) super.setAccessToken(accessToken);
}
@Override
public Grant setAlt(java.lang.String alt) {
return (Grant) super.setAlt(alt);
}
@Override
public Grant setCallback(java.lang.String callback) {
return (Grant) super.setCallback(callback);
}
@Override
public Grant setFields(java.lang.String fields) {
return (Grant) super.setFields(fields);
}
@Override
public Grant setKey(java.lang.String key) {
return (Grant) super.setKey(key);
}
@Override
public Grant setOauthToken(java.lang.String oauthToken) {
return (Grant) super.setOauthToken(oauthToken);
}
@Override
public Grant setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Grant) super.setPrettyPrint(prettyPrint);
}
@Override
public Grant setQuotaUser(java.lang.String quotaUser) {
return (Grant) super.setQuotaUser(quotaUser);
}
@Override
public Grant setUploadType(java.lang.String uploadType) {
return (Grant) super.setUploadType(uploadType);
}
@Override
public Grant setUploadProtocol(java.lang.String uploadProtocol) {
return (Grant) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource which stores the users/service accounts having the
* permission to bind to the corresponding intranet VPC of the consumer project.
* DnsBindPermission is a global resource. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/global/dnsBindPermission`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the resource which stores the users/service accounts having the permission to
bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a global
resource. Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/global/dnsBindPermission`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource which stores the users/service accounts having the
* permission to bind to the corresponding intranet VPC of the consumer project.
* DnsBindPermission is a global resource. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/global/dnsBindPermission`
*/
public Grant setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
}
this.name = name;
return this;
}
@Override
public Grant set(String parameterName, Object value) {
return (Grant) super.set(parameterName, value);
}
}
/**
* Revokes the bind permission from the customer provided principal(user / service account) on the
* intranet VPC associated with the consumer project. DnsBindPermission is a global resource and
* location can only be global.
*
* Create a request for the method "dnsBindPermission.revoke".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Revoke#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the resource which stores the users/service accounts having the permission to
* bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a
* global resource. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/dnsBindPermission`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.RevokeDnsBindPermissionRequest}
* @return the request
*/
public Revoke revoke(java.lang.String name, com.google.api.services.vmwareengine.v1.model.RevokeDnsBindPermissionRequest content) throws java.io.IOException {
Revoke result = new Revoke(name, content);
initialize(result);
return result;
}
public class Revoke extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}:revoke";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
/**
* Revokes the bind permission from the customer provided principal(user / service account) on the
* intranet VPC associated with the consumer project. DnsBindPermission is a global resource and
* location can only be global.
*
* Create a request for the method "dnsBindPermission.revoke".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Revoke#execute()} method to invoke the remote operation.
* {@link
* Revoke#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the resource which stores the users/service accounts having the permission to
* bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a
* global resource. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/dnsBindPermission`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.RevokeDnsBindPermissionRequest}
* @since 1.13
*/
protected Revoke(java.lang.String name, com.google.api.services.vmwareengine.v1.model.RevokeDnsBindPermissionRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
}
}
@Override
public Revoke set$Xgafv(java.lang.String $Xgafv) {
return (Revoke) super.set$Xgafv($Xgafv);
}
@Override
public Revoke setAccessToken(java.lang.String accessToken) {
return (Revoke) super.setAccessToken(accessToken);
}
@Override
public Revoke setAlt(java.lang.String alt) {
return (Revoke) super.setAlt(alt);
}
@Override
public Revoke setCallback(java.lang.String callback) {
return (Revoke) super.setCallback(callback);
}
@Override
public Revoke setFields(java.lang.String fields) {
return (Revoke) super.setFields(fields);
}
@Override
public Revoke setKey(java.lang.String key) {
return (Revoke) super.setKey(key);
}
@Override
public Revoke setOauthToken(java.lang.String oauthToken) {
return (Revoke) super.setOauthToken(oauthToken);
}
@Override
public Revoke setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Revoke) super.setPrettyPrint(prettyPrint);
}
@Override
public Revoke setQuotaUser(java.lang.String quotaUser) {
return (Revoke) super.setQuotaUser(quotaUser);
}
@Override
public Revoke setUploadType(java.lang.String uploadType) {
return (Revoke) super.setUploadType(uploadType);
}
@Override
public Revoke setUploadProtocol(java.lang.String uploadProtocol) {
return (Revoke) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the resource which stores the users/service accounts having the
* permission to bind to the corresponding intranet VPC of the consumer project.
* DnsBindPermission is a global resource. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/global/dnsBindPermission`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the resource which stores the users/service accounts having the permission to
bind to the corresponding intranet VPC of the consumer project. DnsBindPermission is a global
resource. Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/global/dnsBindPermission`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the resource which stores the users/service accounts having the
* permission to bind to the corresponding intranet VPC of the consumer project.
* DnsBindPermission is a global resource. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/global/dnsBindPermission`
*/
public Revoke setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/dnsBindPermission$");
}
this.name = name;
return this;
}
@Override
public Revoke set(String parameterName, Object value) {
return (Revoke) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the NetworkPeerings collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.NetworkPeerings.List request = vmwareengine.networkPeerings().list(parameters ...)}
*
*
* @return the resource collection
*/
public NetworkPeerings networkPeerings() {
return new NetworkPeerings();
}
/**
* The "networkPeerings" collection of methods.
*/
public class NetworkPeerings {
/**
* Creates a new network peering between the peer network and VMware Engine network provided in a
* `NetworkPeering` resource. NetworkPeering is a global resource and location can only be global.
*
* Create a request for the method "networkPeerings.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location to create the new network peering in. This value is
* always `global`, because `NetworkPeering` is a global resource. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.NetworkPeering}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.NetworkPeering content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/networkPeerings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new network peering between the peer network and VMware Engine network provided in a
* `NetworkPeering` resource. NetworkPeering is a global resource and location can only be global.
*
* Create a request for the method "networkPeerings.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location to create the new network peering in. This value is
* always `global`, because `NetworkPeering` is a global resource. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.NetworkPeering}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.NetworkPeering content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location to create the new network peering in. This
* value is always `global`, because `NetworkPeering` is a global resource. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location to create the new network peering in. This value is
always `global`, because `NetworkPeering` is a global resource. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/global`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location to create the new network peering in. This
* value is always `global`, because `NetworkPeering` is a global resource. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the new `NetworkPeering`. This identifier
* must be unique among `NetworkPeering` resources within the parent and becomes the final
* token in the name URI. The identifier must meet the following requirements: * Only
* contains 1-63 alphanumeric characters and hyphens * Begins with an alphabetical
* character * Ends with a non-hyphen character * Not formatted as a UUID * Complies with
* [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String networkPeeringId;
/** Required. The user-provided identifier of the new `NetworkPeering`. This identifier must be unique
among `NetworkPeering` resources within the parent and becomes the final token in the name URI. The
identifier must meet the following requirements: * Only contains 1-63 alphanumeric characters and
hyphens * Begins with an alphabetical character * Ends with a non-hyphen character * Not formatted
as a UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public java.lang.String getNetworkPeeringId() {
return networkPeeringId;
}
/**
* Required. The user-provided identifier of the new `NetworkPeering`. This identifier
* must be unique among `NetworkPeering` resources within the parent and becomes the final
* token in the name URI. The identifier must meet the following requirements: * Only
* contains 1-63 alphanumeric characters and hyphens * Begins with an alphabetical
* character * Ends with a non-hyphen character * Not formatted as a UUID * Complies with
* [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public Create setNetworkPeeringId(java.lang.String networkPeeringId) {
this.networkPeeringId = networkPeeringId;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `NetworkPeering` resource. When a network peering is deleted for a VMware Engine
* network, the peer network becomes inaccessible to that VMware Engine network. NetworkPeering is a
* global resource and location can only be global.
*
* Create a request for the method "networkPeerings.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the network peering to be deleted. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global/networkPeerings/my-peering`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
/**
* Deletes a `NetworkPeering` resource. When a network peering is deleted for a VMware Engine
* network, the peer network becomes inaccessible to that VMware Engine network. NetworkPeering is
* a global resource and location can only be global.
*
* Create a request for the method "networkPeerings.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the network peering to be deleted. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global/networkPeerings/my-peering`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the network peering to be deleted. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the network peering to be deleted. Resource names are schemeless
URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
example: `projects/my-project/locations/global/networkPeerings/my-peering`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the network peering to be deleted. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `NetworkPeering` resource by its resource name. The resource contains details of the
* network peering, such as peered networks, import and export custom route configurations, and
* peering state. NetworkPeering is a global resource and location can only be global.
*
* Create a request for the method "networkPeerings.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the network peering to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global/networkPeerings/my-peering`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
/**
* Retrieves a `NetworkPeering` resource by its resource name. The resource contains details of
* the network peering, such as peered networks, import and export custom route configurations,
* and peering state. NetworkPeering is a global resource and location can only be global.
*
* Create a request for the method "networkPeerings.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the network peering to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global/networkPeerings/my-peering`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.NetworkPeering.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the network peering to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the network peering to retrieve. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/global/networkPeerings/my-peering`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the network peering to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists `NetworkPeering` resources in a given project. NetworkPeering is a global resource and
* location can only be global.
*
* Create a request for the method "networkPeerings.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location (global) to query for network peerings. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/networkPeerings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists `NetworkPeering` resources in a given project. NetworkPeering is a global resource and
* location can only be global.
*
* Create a request for the method "networkPeerings.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location (global) to query for network peerings. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListNetworkPeeringsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location (global) to query for network peerings.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location (global) to query for network peerings. Resource names
are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/global`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location (global) to query for network peerings.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* network peerings, you can exclude the ones named `example-peering` by specifying `name
* != "example-peering"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-peering") (createTime
* > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name
* = "example-peering-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-peering-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of network peerings, you can exclude the ones named
`example-peering` by specifying `name != "example-peering"`. To filter on multiple expressions,
provide each separate expression within parentheses. For example: ``` (name = "example-peering")
(createTime > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name = "example-
peering-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-peering-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* network peerings, you can exclude the ones named `example-peering` by specifying `name
* != "example-peering"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-peering") (createTime
* > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name
* = "example-peering-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-peering-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of network peerings to return in one page. The maximum value is
* coerced to 1000. The default value of this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of network peerings to return in one page. The maximum value is coerced to 1000.
The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of network peerings to return in one page. The maximum value is
* coerced to 1000. The default value of this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListNetworkPeerings` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListNetworkPeerings` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListNetworkPeerings` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListNetworkPeerings` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListNetworkPeerings` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListNetworkPeerings` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies a `NetworkPeering` resource. Only the `description` field can be updated. Only fields
* specified in `updateMask` are applied. NetworkPeering is a global resource and location can only
* be global.
*
* Create a request for the method "networkPeerings.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of the network peering. NetworkPeering is a global resource and
* location can only be global. Resource names are scheme-less URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/global/networkPeerings/my-peering`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.NetworkPeering}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.NetworkPeering content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
/**
* Modifies a `NetworkPeering` resource. Only the `description` field can be updated. Only fields
* specified in `updateMask` are applied. NetworkPeering is a global resource and location can
* only be global.
*
* Create a request for the method "networkPeerings.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of the network peering. NetworkPeering is a global resource and
* location can only be global. Resource names are scheme-less URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/global/networkPeerings/my-peering`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.NetworkPeering}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.NetworkPeering content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of the network peering. NetworkPeering is a global
* resource and location can only be global. Resource names are scheme-less URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global/networkPeerings/my-peering`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of the network peering. NetworkPeering is a global resource and
location can only be global. Resource names are scheme-less URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/global/networkPeerings/my-peering`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of the network peering. NetworkPeering is a global
* resource and location can only be global. Resource names are scheme-less URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global/networkPeerings/my-peering`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `NetworkPeering` resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `NetworkPeering`
resource by the update. The fields specified in the `update_mask` are relative to the resource, not
the full request. A field will be overwritten if it is in the mask. If the user does not provide a
mask then all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `NetworkPeering` resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the PeeringRoutes collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.PeeringRoutes.List request = vmwareengine.peeringRoutes().list(parameters ...)}
*
*
* @return the resource collection
*/
public PeeringRoutes peeringRoutes() {
return new PeeringRoutes();
}
/**
* The "peeringRoutes" collection of methods.
*/
public class PeeringRoutes {
/**
* Lists the network peering routes exchanged over a peering connection. NetworkPeering is a global
* resource and location can only be global.
*
* Create a request for the method "peeringRoutes.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the network peering to retrieve peering routes from. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/peeringRoutes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
/**
* Lists the network peering routes exchanged over a peering connection. NetworkPeering is a
* global resource and location can only be global.
*
* Create a request for the method "peeringRoutes.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the network peering to retrieve peering routes from. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListPeeringRoutesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the network peering to retrieve peering routes from.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the network peering to retrieve peering routes from. Resource names
are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/global/networkPeerings/my-peering`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the network peering to retrieve peering routes from.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPeerings/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. Currently, only
* filtering on the `direction` field is supported. To return routes imported from the
* peer network, provide "direction=INCOMING". To return routes exported from the VMware
* Engine network, provide "direction=OUTGOING". Other filter expressions return an
* error.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. Currently, only filtering on
the `direction` field is supported. To return routes imported from the peer network, provide
"direction=INCOMING". To return routes exported from the VMware Engine network, provide
"direction=OUTGOING". Other filter expressions return an error.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. Currently, only
* filtering on the `direction` field is supported. To return routes imported from the
* peer network, provide "direction=INCOMING". To return routes exported from the VMware
* Engine network, provide "direction=OUTGOING". Other filter expressions return an
* error.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of peering routes to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of
* this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of peering routes to return in one page. The service may return fewer than this
value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of peering routes to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of
* this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListPeeringRoutes` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPeeringRoutes` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListPeeringRoutes` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListPeeringRoutes` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListPeeringRoutes` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPeeringRoutes` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the NetworkPolicies collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.NetworkPolicies.List request = vmwareengine.networkPolicies().list(parameters ...)}
*
*
* @return the resource collection
*/
public NetworkPolicies networkPolicies() {
return new NetworkPolicies();
}
/**
* The "networkPolicies" collection of methods.
*/
public class NetworkPolicies {
/**
* Creates a new network policy in a given VMware Engine network of a project and location (region).
* A new network policy cannot be created if another network policy already exists in the same
* scope.
*
* Create a request for the method "networkPolicies.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location (region) to create the new network policy in. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.NetworkPolicy}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.NetworkPolicy content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/networkPolicies";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new network policy in a given VMware Engine network of a project and location
* (region). A new network policy cannot be created if another network policy already exists in
* the same scope.
*
* Create a request for the method "networkPolicies.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location (region) to create the new network policy in. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.NetworkPolicy}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.NetworkPolicy content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location (region) to create the new network policy
* in. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location (region) to create the new network policy in. Resource
names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location (region) to create the new network policy
* in. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the network policy to be created. This
* identifier must be unique within parent `projects/{my-project}/locations/{us-
* central1}/networkPolicies` and becomes the final token in the name URI. The identifier
* must meet the following requirements: * Only contains 1-63 alphanumeric characters and
* hyphens * Begins with an alphabetical character * Ends with a non-hyphen character *
* Not formatted as a UUID * Complies with [RFC
* 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String networkPolicyId;
/** Required. The user-provided identifier of the network policy to be created. This identifier must be
unique within parent `projects/{my-project}/locations/{us-central1}/networkPolicies` and becomes
the final token in the name URI. The identifier must meet the following requirements: * Only
contains 1-63 alphanumeric characters and hyphens * Begins with an alphabetical character * Ends
with a non-hyphen character * Not formatted as a UUID * Complies with [RFC
1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public java.lang.String getNetworkPolicyId() {
return networkPolicyId;
}
/**
* Required. The user-provided identifier of the network policy to be created. This
* identifier must be unique within parent `projects/{my-project}/locations/{us-
* central1}/networkPolicies` and becomes the final token in the name URI. The identifier
* must meet the following requirements: * Only contains 1-63 alphanumeric characters and
* hyphens * Begins with an alphabetical character * Ends with a non-hyphen character *
* Not formatted as a UUID * Complies with [RFC
* 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public Create setNetworkPolicyId(java.lang.String networkPolicyId) {
this.networkPolicyId = networkPolicyId;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `NetworkPolicy` resource. A network policy cannot be deleted when
* `NetworkService.state` is set to `RECONCILING` for either its external IP or internet access
* service.
*
* Create a request for the method "networkPolicies.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the network policy to delete. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
/**
* Deletes a `NetworkPolicy` resource. A network policy cannot be deleted when
* `NetworkService.state` is set to `RECONCILING` for either its external IP or internet access
* service.
*
* Create a request for the method "networkPolicies.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the network policy to delete. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the network policy to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-network-policy`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the network policy to delete. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the network policy to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-network-policy`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Lists external IP addresses assigned to VMware workload VMs within the scope of the given network
* policy.
*
* Create a request for the method "networkPolicies.fetchExternalAddresses".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link FetchExternalAddresses#execute()} method to invoke the remote
* operation.
*
* @param networkPolicy Required. The resource name of the network policy to query for assigned external IP addresses.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
* @return the request
*/
public FetchExternalAddresses fetchExternalAddresses(java.lang.String networkPolicy) throws java.io.IOException {
FetchExternalAddresses result = new FetchExternalAddresses(networkPolicy);
initialize(result);
return result;
}
public class FetchExternalAddresses extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+networkPolicy}:fetchExternalAddresses";
private final java.util.regex.Pattern NETWORK_POLICY_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
/**
* Lists external IP addresses assigned to VMware workload VMs within the scope of the given
* network policy.
*
* Create a request for the method "networkPolicies.fetchExternalAddresses".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link FetchExternalAddresses#execute()} method to invoke the
* remote operation. {@link FetchExternalAddresses#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param networkPolicy Required. The resource name of the network policy to query for assigned external IP addresses.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
* @since 1.13
*/
protected FetchExternalAddresses(java.lang.String networkPolicy) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.FetchNetworkPolicyExternalAddressesResponse.class);
this.networkPolicy = com.google.api.client.util.Preconditions.checkNotNull(networkPolicy, "Required parameter networkPolicy must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NETWORK_POLICY_PATTERN.matcher(networkPolicy).matches(),
"Parameter networkPolicy must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public FetchExternalAddresses set$Xgafv(java.lang.String $Xgafv) {
return (FetchExternalAddresses) super.set$Xgafv($Xgafv);
}
@Override
public FetchExternalAddresses setAccessToken(java.lang.String accessToken) {
return (FetchExternalAddresses) super.setAccessToken(accessToken);
}
@Override
public FetchExternalAddresses setAlt(java.lang.String alt) {
return (FetchExternalAddresses) super.setAlt(alt);
}
@Override
public FetchExternalAddresses setCallback(java.lang.String callback) {
return (FetchExternalAddresses) super.setCallback(callback);
}
@Override
public FetchExternalAddresses setFields(java.lang.String fields) {
return (FetchExternalAddresses) super.setFields(fields);
}
@Override
public FetchExternalAddresses setKey(java.lang.String key) {
return (FetchExternalAddresses) super.setKey(key);
}
@Override
public FetchExternalAddresses setOauthToken(java.lang.String oauthToken) {
return (FetchExternalAddresses) super.setOauthToken(oauthToken);
}
@Override
public FetchExternalAddresses setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FetchExternalAddresses) super.setPrettyPrint(prettyPrint);
}
@Override
public FetchExternalAddresses setQuotaUser(java.lang.String quotaUser) {
return (FetchExternalAddresses) super.setQuotaUser(quotaUser);
}
@Override
public FetchExternalAddresses setUploadType(java.lang.String uploadType) {
return (FetchExternalAddresses) super.setUploadType(uploadType);
}
@Override
public FetchExternalAddresses setUploadProtocol(java.lang.String uploadProtocol) {
return (FetchExternalAddresses) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the network policy to query for assigned external IP
* addresses. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
*/
@com.google.api.client.util.Key
private java.lang.String networkPolicy;
/** Required. The resource name of the network policy to query for assigned external IP addresses.
Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1/networkPolicies/my-policy`
*/
public java.lang.String getNetworkPolicy() {
return networkPolicy;
}
/**
* Required. The resource name of the network policy to query for assigned external IP
* addresses. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
*/
public FetchExternalAddresses setNetworkPolicy(java.lang.String networkPolicy) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NETWORK_POLICY_PATTERN.matcher(networkPolicy).matches(),
"Parameter networkPolicy must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
this.networkPolicy = networkPolicy;
return this;
}
/**
* The maximum number of external IP addresses to return in one page. The service may
* return fewer than this value. The maximum value is coerced to 1000. The default value
* of this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of external IP addresses to return in one page. The service may return fewer
than this value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of external IP addresses to return in one page. The service may
* return fewer than this value. The maximum value is coerced to 1000. The default value
* of this field is 500.
*/
public FetchExternalAddresses setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `FetchNetworkPolicyExternalAddresses` call.
* Provide this to retrieve the subsequent page. When paginating, all parameters provided
* to `FetchNetworkPolicyExternalAddresses`, except for `page_size` and `page_token`, must
* match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `FetchNetworkPolicyExternalAddresses` call. Provide this to
retrieve the subsequent page. When paginating, all parameters provided to
`FetchNetworkPolicyExternalAddresses`, except for `page_size` and `page_token`, must match the call
that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `FetchNetworkPolicyExternalAddresses` call.
* Provide this to retrieve the subsequent page. When paginating, all parameters provided
* to `FetchNetworkPolicyExternalAddresses`, except for `page_size` and `page_token`, must
* match the call that provided the page token.
*/
public FetchExternalAddresses setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public FetchExternalAddresses set(String parameterName, Object value) {
return (FetchExternalAddresses) super.set(parameterName, value);
}
}
/**
* Retrieves a `NetworkPolicy` resource by its resource name.
*
* Create a request for the method "networkPolicies.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the network policy to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
/**
* Retrieves a `NetworkPolicy` resource by its resource name.
*
* Create a request for the method "networkPolicies.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the network policy to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.NetworkPolicy.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the network policy to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-network-policy`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the network policy to retrieve. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the network policy to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-network-policy`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists `NetworkPolicy` resources in a specified project and location.
*
* Create a request for the method "networkPolicies.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location (region) to query for network policies. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/networkPolicies";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists `NetworkPolicy` resources in a specified project and location.
*
* Create a request for the method "networkPolicies.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location (region) to query for network policies. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListNetworkPoliciesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location (region) to query for network policies.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location (region) to query for network policies. Resource names
are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location (region) to query for network policies.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* network policies, you can exclude the ones named `example-policy` by specifying `name
* != "example-policy"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-policy") (createTime >
* "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name
* = "example-policy-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-
* policy-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of network policies, you can exclude the ones named
`example-policy` by specifying `name != "example-policy"`. To filter on multiple expressions,
provide each separate expression within parentheses. For example: ``` (name = "example-policy")
(createTime > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name = "example-
policy-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-policy-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* network policies, you can exclude the ones named `example-policy` by specifying `name
* != "example-policy"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-policy") (createTime >
* "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name
* = "example-policy-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-
* policy-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of network policies to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of network policies to return in one page. The service may return fewer than
this value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of network policies to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListNetworkPolicies` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListNetworkPolicies` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListNetworkPolicies` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListNetworkPolicies` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListNetworkPolicies` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListNetworkPolicies` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies a `NetworkPolicy` resource. Only the following fields can be updated: `internet_access`,
* `external_ip`, `edge_services_cidr`. Only fields specified in `updateMask` are applied. When
* updating a network policy, the external IP network service can only be disabled if there are no
* external IP addresses present in the scope of the policy. Also, a `NetworkService` cannot be
* updated when `NetworkService.state` is set to `RECONCILING`. During operation processing, the
* resource is temporarily in the `ACTIVE` state before the operation fully completes. For that
* period of time, you can't update the resource. Use the operation status to determine when the
* processing fully completes.
*
* Create a request for the method "networkPolicies.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this network policy. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.NetworkPolicy}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.NetworkPolicy content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
/**
* Modifies a `NetworkPolicy` resource. Only the following fields can be updated:
* `internet_access`, `external_ip`, `edge_services_cidr`. Only fields specified in `updateMask`
* are applied. When updating a network policy, the external IP network service can only be
* disabled if there are no external IP addresses present in the scope of the policy. Also, a
* `NetworkService` cannot be updated when `NetworkService.state` is set to `RECONCILING`. During
* operation processing, the resource is temporarily in the `ACTIVE` state before the operation
* fully completes. For that period of time, you can't update the resource. Use the operation
* status to determine when the processing fully completes.
*
* Create a request for the method "networkPolicies.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of this network policy. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.NetworkPolicy}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.NetworkPolicy content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this network policy. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-network-policy`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this network policy. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1/networkPolicies/my-network-policy`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this network policy. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-network-policy`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `NetworkPolicy` resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `NetworkPolicy`
resource by the update. The fields specified in the `update_mask` are relative to the resource, not
the full request. A field will be overwritten if it is in the mask. If the user does not provide a
mask then all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `NetworkPolicy` resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ExternalAccessRules collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.ExternalAccessRules.List request = vmwareengine.externalAccessRules().list(parameters ...)}
*
*
* @return the resource collection
*/
public ExternalAccessRules externalAccessRules() {
return new ExternalAccessRules();
}
/**
* The "externalAccessRules" collection of methods.
*/
public class ExternalAccessRules {
/**
* Creates a new external access rule in a given network policy.
*
* Create a request for the method "externalAccessRules.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the network policy to create a new external access firewall rule in.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ExternalAccessRule}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.ExternalAccessRule content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/externalAccessRules";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
/**
* Creates a new external access rule in a given network policy.
*
* Create a request for the method "externalAccessRules.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the network policy to create a new external access firewall rule in.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ExternalAccessRule}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.ExternalAccessRule content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the network policy to create a new external access
* firewall rule in. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the network policy to create a new external access firewall rule in.
Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1/networkPolicies/my-policy`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the network policy to create a new external access
* firewall rule in. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the `ExternalAccessRule` to be created.
* This identifier must be unique among `ExternalAccessRule` resources within the parent
* and becomes the final token in the name URI. The identifier must meet the following
* requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
* an alphabetical character * Ends with a non-hyphen character * Not formatted as a
* UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
* (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String externalAccessRuleId;
/** Required. The user-provided identifier of the `ExternalAccessRule` to be created. This identifier
must be unique among `ExternalAccessRule` resources within the parent and becomes the final token
in the name URI. The identifier must meet the following requirements: * Only contains 1-63
alphanumeric characters and hyphens * Begins with an alphabetical character * Ends with a non-
hyphen character * Not formatted as a UUID * Complies with [RFC
1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public java.lang.String getExternalAccessRuleId() {
return externalAccessRuleId;
}
/**
* Required. The user-provided identifier of the `ExternalAccessRule` to be created.
* This identifier must be unique among `ExternalAccessRule` resources within the parent
* and becomes the final token in the name URI. The identifier must meet the following
* requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
* an alphabetical character * Ends with a non-hyphen character * Not formatted as a
* UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
* (section 3.5)
*/
public Create setExternalAccessRuleId(java.lang.String externalAccessRuleId) {
this.externalAccessRuleId = externalAccessRuleId;
return this;
}
/**
* A request ID to identify requests. Specify a unique request ID so that if you must
* retry your request, the server will know to ignore the request if it has already been
* completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation
* where you make an initial request and the request times out. If you make the request
* again with the same request ID, the server can check if the original operation with
* the same request ID was received, and if so, will ignore the second request. This
* prevents clients from accidentally creating duplicate commitments. The request ID
* must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A request ID to identify requests. Specify a unique request ID so that if you must retry your
request, the server will know to ignore the request if it has already been completed. The server
guarantees that a request doesn't result in creation of duplicate commitments for at least 60
minutes. For example, consider a situation where you make an initial request and the request times
out. If you make the request again with the same request ID, the server can check if the original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A request ID to identify requests. Specify a unique request ID so that if you must
* retry your request, the server will know to ignore the request if it has already been
* completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation
* where you make an initial request and the request times out. If you make the request
* again with the same request ID, the server can check if the original operation with
* the same request ID was received, and if so, will ignore the second request. This
* prevents clients from accidentally creating duplicate commitments. The request ID
* must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single external access rule.
*
* Create a request for the method "externalAccessRules.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the external access firewall rule to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
/**
* Deletes a single external access rule.
*
* Create a request for the method "externalAccessRules.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the external access firewall rule to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the external access firewall rule to delete. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the external access firewall rule to delete. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1/networkPolicies/my-
policy/externalAccessRules/my-rule`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the external access firewall rule to delete. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if the
original operation with the same request ID was received, and if so, will ignore the second
request. This prevents clients from accidentally creating duplicate commitments. The request ID
must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single external access rule.
*
* Create a request for the method "externalAccessRules.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the external access firewall rule to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
/**
* Gets details of a single external access rule.
*
* Create a request for the method "externalAccessRules.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the external access firewall rule to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ExternalAccessRule.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the external access firewall rule to retrieve.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the external access firewall rule to retrieve. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1/networkPolicies/my-
policy/externalAccessRules/my-rule`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the external access firewall rule to retrieve.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists `ExternalAccessRule` resources in the specified network policy.
*
* Create a request for the method "externalAccessRules.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the network policy to query for external access firewall rules.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/externalAccessRules";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
/**
* Lists `ExternalAccessRule` resources in the specified network policy.
*
* Create a request for the method "externalAccessRules.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the network policy to query for external access firewall rules.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListExternalAccessRulesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the network policy to query for external access
* firewall rules. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the network policy to query for external access firewall rules.
Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1/networkPolicies/my-policy`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the network policy to query for external access
* firewall rules. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to
* use for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* external access rules, you can exclude the ones named `example-rule` by specifying
* `name != "example-rule"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-rule") (createTime >
* "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ```
* (name = "example-rule-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-rule-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of external access rules, you can exclude the ones named
`example-rule` by specifying `name != "example-rule"`. To filter on multiple expressions, provide
each separate expression within parentheses. For example: ``` (name = "example-rule") (createTime >
"2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression. However, you can
include `AND` and `OR` expressions explicitly. For example: ``` (name = "example-rule-1") AND
(createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-rule-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to
* use for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* external access rules, you can exclude the ones named `example-rule` by specifying
* `name != "example-rule"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-rule") (createTime >
* "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ```
* (name = "example-rule-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-rule-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of external access rules to return in one page. The service may
* return fewer than this value. The maximum value is coerced to 1000. The default value
* of this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of external access rules to return in one page. The service may return fewer
than this value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of external access rules to return in one page. The service may
* return fewer than this value. The maximum value is coerced to 1000. The default value
* of this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListExternalAccessRulesRequest` call. Provide
* this to retrieve the subsequent page. When paginating, all other parameters provided
* to `ListExternalAccessRulesRequest` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListExternalAccessRulesRequest` call. Provide this to
retrieve the subsequent page. When paginating, all other parameters provided to
`ListExternalAccessRulesRequest` must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListExternalAccessRulesRequest` call. Provide
* this to retrieve the subsequent page. When paginating, all other parameters provided
* to `ListExternalAccessRulesRequest` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the parameters of a single external access rule. Only fields specified in `update_mask`
* are applied.
*
* Create a request for the method "externalAccessRules.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this external access rule. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/networkPolicies/my-
* policy/externalAccessRules/my-rule`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ExternalAccessRule}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.ExternalAccessRule content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
/**
* Updates the parameters of a single external access rule. Only fields specified in `update_mask`
* are applied.
*
* Create a request for the method "externalAccessRules.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of this external access rule. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/networkPolicies/my-
* policy/externalAccessRules/my-rule`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ExternalAccessRule}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.ExternalAccessRule content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this external access rule. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this external access rule. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this external access rule. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/networkPolicies/my-policy/externalAccessRules/my-rule`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/networkPolicies/[^/]+/externalAccessRules/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if the
original operation with the same request ID was received, and if so, will ignore the second
request. This prevents clients from accidentally creating duplicate commitments. The request ID
must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `ExternalAccessRule` resource by the update. The fields specified in the
* `update_mask` are relative to the resource, not the full request. A field will be
* overwritten if it is in the mask. If the user does not provide a mask then all fields
* will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `ExternalAccessRule`
resource by the update. The fields specified in the `update_mask` are relative to the resource, not
the full request. A field will be overwritten if it is in the mask. If the user does not provide a
mask then all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `ExternalAccessRule` resource by the update. The fields specified in the
* `update_mask` are relative to the resource, not the full request. A field will be
* overwritten if it is in the mask. If the user does not provide a mask then all fields
* will be overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the NodeTypes collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.NodeTypes.List request = vmwareengine.nodeTypes().list(parameters ...)}
*
*
* @return the resource collection
*/
public NodeTypes nodeTypes() {
return new NodeTypes();
}
/**
* The "nodeTypes" collection of methods.
*/
public class NodeTypes {
/**
* Gets details of a single `NodeType`.
*
* Create a request for the method "nodeTypes.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the node type to retrieve. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-proj/locations/us-central1-a/nodeTypes/standard-72`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/nodeTypes/[^/]+$");
/**
* Gets details of a single `NodeType`.
*
* Create a request for the method "nodeTypes.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the node type to retrieve. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-proj/locations/us-central1-a/nodeTypes/standard-72`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.NodeType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/nodeTypes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the node type to retrieve. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* proj/locations/us-central1-a/nodeTypes/standard-72`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the node type to retrieve. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-proj/locations/us-central1-a/nodeTypes/standard-72`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the node type to retrieve. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* proj/locations/us-central1-a/nodeTypes/standard-72`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/nodeTypes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists node types
*
* Create a request for the method "nodeTypes.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location to be queried for node types. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/nodeTypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists node types
*
* Create a request for the method "nodeTypes.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location to be queried for node types. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListNodeTypesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location to be queried for node types. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location to be queried for node types. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location to be queried for node types. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* node types, you can exclude the ones named `standard-72` by specifying `name !=
* "standard-72"`. To filter on multiple expressions, provide each separate expression
* within parentheses. For example: ``` (name = "standard-72") (virtual_cpu_count > 2) ```
* By default, each expression is an `AND` expression. However, you can include `AND` and
* `OR` expressions explicitly. For example: ``` (name = "standard-96") AND
* (virtual_cpu_count > 2) OR (name = "standard-72") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of node types, you can exclude the ones named
`standard-72` by specifying `name != "standard-72"`. To filter on multiple expressions, provide
each separate expression within parentheses. For example: ``` (name = "standard-72")
(virtual_cpu_count > 2) ``` By default, each expression is an `AND` expression. However, you can
include `AND` and `OR` expressions explicitly. For example: ``` (name = "standard-96") AND
(virtual_cpu_count > 2) OR (name = "standard-72") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* node types, you can exclude the ones named `standard-72` by specifying `name !=
* "standard-72"`. To filter on multiple expressions, provide each separate expression
* within parentheses. For example: ``` (name = "standard-72") (virtual_cpu_count > 2) ```
* By default, each expression is an `AND` expression. However, you can include `AND` and
* `OR` expressions explicitly. For example: ``` (name = "standard-96") AND
* (virtual_cpu_count > 2) OR (name = "standard-72") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of node types to return in one page. The service may return fewer
* than this value. The maximum value is coerced to 1000. The default value of this field
* is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of node types to return in one page. The service may return fewer than this
value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of node types to return in one page. The service may return fewer
* than this value. The maximum value is coerced to 1000. The default value of this field
* is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListNodeTypes` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListNodeTypes`
* must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListNodeTypes` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListNodeTypes` must match the
call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListNodeTypes` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListNodeTypes`
* must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.Operations.List request = vmwareengine.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the PrivateClouds collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.PrivateClouds.List request = vmwareengine.privateClouds().list(parameters ...)}
*
*
* @return the resource collection
*/
public PrivateClouds privateClouds() {
return new PrivateClouds();
}
/**
* The "privateClouds" collection of methods.
*/
public class PrivateClouds {
/**
* Creates a new `PrivateCloud` resource in a given project and location. Private clouds of type
* `STANDARD` and `TIME_LIMITED` are zonal resources, `STRETCHED` private clouds are regional.
* Creating a private cloud also creates a [management cluster](https://cloud.google.com/vmware-
* engine/docs/concepts-vmware-components) for that private cloud.
*
* Create a request for the method "privateClouds.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location to create the new private cloud in. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.PrivateCloud}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.PrivateCloud content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/privateClouds";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new `PrivateCloud` resource in a given project and location. Private clouds of type
* `STANDARD` and `TIME_LIMITED` are zonal resources, `STRETCHED` private clouds are regional.
* Creating a private cloud also creates a [management cluster](https://cloud.google.com/vmware-
* engine/docs/concepts-vmware-components) for that private cloud.
*
* Create a request for the method "privateClouds.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location to create the new private cloud in. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.PrivateCloud}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.PrivateCloud content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location to create the new private cloud in.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location to create the new private cloud in. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location to create the new private cloud in.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the private cloud to be created. This
* identifier must be unique among each `PrivateCloud` within the parent and becomes the
* final token in the name URI. The identifier must meet the following requirements: *
* Only contains 1-63 alphanumeric characters and hyphens * Begins with an alphabetical
* character * Ends with a non-hyphen character * Not formatted as a UUID * Complies with
* [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String privateCloudId;
/** Required. The user-provided identifier of the private cloud to be created. This identifier must be
unique among each `PrivateCloud` within the parent and becomes the final token in the name URI. The
identifier must meet the following requirements: * Only contains 1-63 alphanumeric characters and
hyphens * Begins with an alphabetical character * Ends with a non-hyphen character * Not formatted
as a UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public java.lang.String getPrivateCloudId() {
return privateCloudId;
}
/**
* Required. The user-provided identifier of the private cloud to be created. This
* identifier must be unique among each `PrivateCloud` within the parent and becomes the
* final token in the name URI. The identifier must meet the following requirements: *
* Only contains 1-63 alphanumeric characters and hyphens * Begins with an alphabetical
* character * Ends with a non-hyphen character * Not formatted as a UUID * Complies with
* [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public Create setPrivateCloudId(java.lang.String privateCloudId) {
this.privateCloudId = privateCloudId;
return this;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is not
* supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. The request ID must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is not
* supported (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Optional. True if you want the request to be validated and not executed; false
* otherwise.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Optional. True if you want the request to be validated and not executed; false otherwise.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Optional. True if you want the request to be validated and not executed; false
* otherwise.
*/
public Create setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Schedules a `PrivateCloud` resource for deletion. A `PrivateCloud` resource scheduled for
* deletion has `PrivateCloud.state` set to `DELETED` and `expireTime` set to the time when deletion
* is final and can no longer be reversed. The delete operation is marked as done as soon as the
* `PrivateCloud` is successfully scheduled for deletion (this also applies when `delayHours` is set
* to zero), and the operation is not kept in pending state until `PrivateCloud` is purged.
* `PrivateCloud` can be restored using `UndeletePrivateCloud` method before the `expireTime`
* elapses. When `expireTime` is reached, deletion is final and all private cloud resources are
* irreversibly removed and billing stops. During the final removal process, `PrivateCloud.state` is
* set to `PURGING`. `PrivateCloud` can be polled using standard `GET` method for the whole period
* of deletion and purging. It will not be returned only when it is completely purged.
*
* Create a request for the method "privateClouds.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the private cloud to delete. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Schedules a `PrivateCloud` resource for deletion. A `PrivateCloud` resource scheduled for
* deletion has `PrivateCloud.state` set to `DELETED` and `expireTime` set to the time when
* deletion is final and can no longer be reversed. The delete operation is marked as done as soon
* as the `PrivateCloud` is successfully scheduled for deletion (this also applies when
* `delayHours` is set to zero), and the operation is not kept in pending state until
* `PrivateCloud` is purged. `PrivateCloud` can be restored using `UndeletePrivateCloud` method
* before the `expireTime` elapses. When `expireTime` is reached, deletion is final and all
* private cloud resources are irreversibly removed and billing stops. During the final removal
* process, `PrivateCloud.state` is set to `PURGING`. `PrivateCloud` can be polled using standard
* `GET` method for the whole period of deletion and purging. It will not be returned only when it
* is completely purged.
*
* Create a request for the method "privateClouds.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the private cloud to delete. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the private cloud to delete. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the private cloud to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Time delay of the deletion specified in hours. The default value is `3`.
* Specifying a non-zero value for this field changes the value of `PrivateCloud.state` to
* `DELETED` and sets `expire_time` to the planned deletion time. Deletion can be
* cancelled before `expire_time` elapses using VmwareEngine.UndeletePrivateCloud.
* Specifying a value of `0` for this field instead begins the deletion process and ceases
* billing immediately. During the final deletion process, the value of
* `PrivateCloud.state` becomes `PURGING`.
*/
@com.google.api.client.util.Key
private java.lang.Integer delayHours;
/** Optional. Time delay of the deletion specified in hours. The default value is `3`. Specifying a
non-zero value for this field changes the value of `PrivateCloud.state` to `DELETED` and sets
`expire_time` to the planned deletion time. Deletion can be cancelled before `expire_time` elapses
using VmwareEngine.UndeletePrivateCloud. Specifying a value of `0` for this field instead begins
the deletion process and ceases billing immediately. During the final deletion process, the value
of `PrivateCloud.state` becomes `PURGING`.
*/
public java.lang.Integer getDelayHours() {
return delayHours;
}
/**
* Optional. Time delay of the deletion specified in hours. The default value is `3`.
* Specifying a non-zero value for this field changes the value of `PrivateCloud.state` to
* `DELETED` and sets `expire_time` to the planned deletion time. Deletion can be
* cancelled before `expire_time` elapses using VmwareEngine.UndeletePrivateCloud.
* Specifying a value of `0` for this field instead begins the deletion process and ceases
* billing immediately. During the final deletion process, the value of
* `PrivateCloud.state` becomes `PURGING`.
*/
public Delete setDelayHours(java.lang.Integer delayHours) {
this.delayHours = delayHours;
return this;
}
/**
* Optional. If set to true, cascade delete is enabled and all children of this private
* cloud resource are also deleted. When this flag is set to false, the private cloud will
* not be deleted if there are any children other than the management cluster. The
* management cluster is always deleted.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Optional. If set to true, cascade delete is enabled and all children of this private cloud resource
are also deleted. When this flag is set to false, the private cloud will not be deleted if there
are any children other than the management cluster. The management cluster is always deleted.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Optional. If set to true, cascade delete is enabled and all children of this private
* cloud resource are also deleted. When this flag is set to false, the private cloud will
* not be deleted if there are any children other than the management cluster. The
* management cluster is always deleted.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is not
* supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. The request ID must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is not
* supported (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `PrivateCloud` resource by its resource name.
*
* Create a request for the method "privateClouds.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the private cloud to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Retrieves a `PrivateCloud` resource by its resource name.
*
* Create a request for the method "privateClouds.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the private cloud to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.PrivateCloud.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the private cloud to retrieve. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the private cloud to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets details of the `DnsForwarding` config.
*
* Create a request for the method "privateClouds.getDnsForwarding".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link GetDnsForwarding#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of a `DnsForwarding` to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/dnsForwarding`
* @return the request
*/
public GetDnsForwarding getDnsForwarding(java.lang.String name) throws java.io.IOException {
GetDnsForwarding result = new GetDnsForwarding(name);
initialize(result);
return result;
}
public class GetDnsForwarding extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/dnsForwarding$");
/**
* Gets details of the `DnsForwarding` config.
*
* Create a request for the method "privateClouds.getDnsForwarding".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link GetDnsForwarding#execute()} method to invoke the remote
* operation. {@link GetDnsForwarding#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The resource name of a `DnsForwarding` to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/dnsForwarding`
* @since 1.13
*/
protected GetDnsForwarding(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.DnsForwarding.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/dnsForwarding$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetDnsForwarding set$Xgafv(java.lang.String $Xgafv) {
return (GetDnsForwarding) super.set$Xgafv($Xgafv);
}
@Override
public GetDnsForwarding setAccessToken(java.lang.String accessToken) {
return (GetDnsForwarding) super.setAccessToken(accessToken);
}
@Override
public GetDnsForwarding setAlt(java.lang.String alt) {
return (GetDnsForwarding) super.setAlt(alt);
}
@Override
public GetDnsForwarding setCallback(java.lang.String callback) {
return (GetDnsForwarding) super.setCallback(callback);
}
@Override
public GetDnsForwarding setFields(java.lang.String fields) {
return (GetDnsForwarding) super.setFields(fields);
}
@Override
public GetDnsForwarding setKey(java.lang.String key) {
return (GetDnsForwarding) super.setKey(key);
}
@Override
public GetDnsForwarding setOauthToken(java.lang.String oauthToken) {
return (GetDnsForwarding) super.setOauthToken(oauthToken);
}
@Override
public GetDnsForwarding setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetDnsForwarding) super.setPrettyPrint(prettyPrint);
}
@Override
public GetDnsForwarding setQuotaUser(java.lang.String quotaUser) {
return (GetDnsForwarding) super.setQuotaUser(quotaUser);
}
@Override
public GetDnsForwarding setUploadType(java.lang.String uploadType) {
return (GetDnsForwarding) super.setUploadType(uploadType);
}
@Override
public GetDnsForwarding setUploadProtocol(java.lang.String uploadProtocol) {
return (GetDnsForwarding) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of a `DnsForwarding` to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/dnsForwarding`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of a `DnsForwarding` to retrieve. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud/dnsForwarding`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of a `DnsForwarding` to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/dnsForwarding`
*/
public GetDnsForwarding setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/dnsForwarding$");
}
this.name = name;
return this;
}
@Override
public GetDnsForwarding set(String parameterName, Object value) {
return (GetDnsForwarding) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "privateClouds.getIamPolicy".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "privateClouds.getIamPolicy".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists `PrivateCloud` resources in a given project and location.
*
* Create a request for the method "privateClouds.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to be queried for clusters. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/privateClouds";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists `PrivateCloud` resources in a given project and location.
*
* Create a request for the method "privateClouds.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to be queried for clusters. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListPrivateCloudsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to be queried for clusters. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to be queried for clusters. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to be queried for clusters. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* private clouds, you can exclude the ones named `example-pc` by specifying `name !=
* "example-pc"`. You can also filter nested fields. For example, you could specify
* `networkConfig.managementCidr = "192.168.0.0/24"` to include private clouds only if
* they have a matching address in their network configuration. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (name = "example-pc") (createTime > "2021-04-12T08:15:10.40Z") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (name = "private-cloud-1") AND (createTime >
* "2021-04-12T08:15:10.40Z") OR (name = "private-cloud-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of private clouds, you can exclude the ones named
`example-pc` by specifying `name != "example-pc"`. You can also filter nested fields. For example,
you could specify `networkConfig.managementCidr = "192.168.0.0/24"` to include private clouds only
if they have a matching address in their network configuration. To filter on multiple expressions,
provide each separate expression within parentheses. For example: ``` (name = "example-pc")
(createTime > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name = "private-
cloud-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "private-cloud-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* private clouds, you can exclude the ones named `example-pc` by specifying `name !=
* "example-pc"`. You can also filter nested fields. For example, you could specify
* `networkConfig.managementCidr = "192.168.0.0/24"` to include private clouds only if
* they have a matching address in their network configuration. To filter on multiple
* expressions, provide each separate expression within parentheses. For example: ```
* (name = "example-pc") (createTime > "2021-04-12T08:15:10.40Z") ``` By default, each
* expression is an `AND` expression. However, you can include `AND` and `OR` expressions
* explicitly. For example: ``` (name = "private-cloud-1") AND (createTime >
* "2021-04-12T08:15:10.40Z") OR (name = "private-cloud-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of private clouds to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of private clouds to return in one page. The service may return fewer than this
value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of private clouds to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListPrivateClouds` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPrivateClouds` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListPrivateClouds` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListPrivateClouds` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListPrivateClouds` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPrivateClouds` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies a `PrivateCloud` resource. Only the following fields can be updated: `description`. Only
* fields specified in `updateMask` are applied. During operation processing, the resource is
* temporarily in the `ACTIVE` state before the operation fully completes. For that period of time,
* you can't update the resource. Use the operation status to determine when the processing fully
* completes.
*
* Create a request for the method "privateClouds.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this private cloud. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.PrivateCloud}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.PrivateCloud content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Modifies a `PrivateCloud` resource. Only the following fields can be updated: `description`.
* Only fields specified in `updateMask` are applied. During operation processing, the resource is
* temporarily in the `ACTIVE` state before the operation fully completes. For that period of
* time, you can't update the resource. Use the operation status to determine when the processing
* fully completes.
*
* Create a request for the method "privateClouds.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of this private cloud. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.PrivateCloud}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.PrivateCloud content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this private cloud. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this private cloud. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this private cloud. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is not
* supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. The request ID must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is not
* supported (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `PrivateCloud` resource by the update. The fields specified in `updateMask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `PrivateCloud` resource
by the update. The fields specified in `updateMask` are relative to the resource, not the full
request. A field will be overwritten if it is in the mask. If the user does not provide a mask then
all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `PrivateCloud` resource by the update. The fields specified in `updateMask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Resets credentials of the NSX appliance.
*
* Create a request for the method "privateClouds.resetNsxCredentials".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link ResetNsxCredentials#execute()} method to invoke the remote operation.
*
* @param privateCloud Required. The resource name of the private cloud to reset credentials for. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ResetNsxCredentialsRequest}
* @return the request
*/
public ResetNsxCredentials resetNsxCredentials(java.lang.String privateCloud, com.google.api.services.vmwareengine.v1.model.ResetNsxCredentialsRequest content) throws java.io.IOException {
ResetNsxCredentials result = new ResetNsxCredentials(privateCloud, content);
initialize(result);
return result;
}
public class ResetNsxCredentials extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+privateCloud}:resetNsxCredentials";
private final java.util.regex.Pattern PRIVATE_CLOUD_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Resets credentials of the NSX appliance.
*
* Create a request for the method "privateClouds.resetNsxCredentials".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link ResetNsxCredentials#execute()} method to invoke the remote
* operation. {@link ResetNsxCredentials#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param privateCloud Required. The resource name of the private cloud to reset credentials for. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ResetNsxCredentialsRequest}
* @since 1.13
*/
protected ResetNsxCredentials(java.lang.String privateCloud, com.google.api.services.vmwareengine.v1.model.ResetNsxCredentialsRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.privateCloud = com.google.api.client.util.Preconditions.checkNotNull(privateCloud, "Required parameter privateCloud must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRIVATE_CLOUD_PATTERN.matcher(privateCloud).matches(),
"Parameter privateCloud must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public ResetNsxCredentials set$Xgafv(java.lang.String $Xgafv) {
return (ResetNsxCredentials) super.set$Xgafv($Xgafv);
}
@Override
public ResetNsxCredentials setAccessToken(java.lang.String accessToken) {
return (ResetNsxCredentials) super.setAccessToken(accessToken);
}
@Override
public ResetNsxCredentials setAlt(java.lang.String alt) {
return (ResetNsxCredentials) super.setAlt(alt);
}
@Override
public ResetNsxCredentials setCallback(java.lang.String callback) {
return (ResetNsxCredentials) super.setCallback(callback);
}
@Override
public ResetNsxCredentials setFields(java.lang.String fields) {
return (ResetNsxCredentials) super.setFields(fields);
}
@Override
public ResetNsxCredentials setKey(java.lang.String key) {
return (ResetNsxCredentials) super.setKey(key);
}
@Override
public ResetNsxCredentials setOauthToken(java.lang.String oauthToken) {
return (ResetNsxCredentials) super.setOauthToken(oauthToken);
}
@Override
public ResetNsxCredentials setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ResetNsxCredentials) super.setPrettyPrint(prettyPrint);
}
@Override
public ResetNsxCredentials setQuotaUser(java.lang.String quotaUser) {
return (ResetNsxCredentials) super.setQuotaUser(quotaUser);
}
@Override
public ResetNsxCredentials setUploadType(java.lang.String uploadType) {
return (ResetNsxCredentials) super.setUploadType(uploadType);
}
@Override
public ResetNsxCredentials setUploadProtocol(java.lang.String uploadProtocol) {
return (ResetNsxCredentials) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to reset credentials for. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String privateCloud;
/** Required. The resource name of the private cloud to reset credentials for. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getPrivateCloud() {
return privateCloud;
}
/**
* Required. The resource name of the private cloud to reset credentials for. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public ResetNsxCredentials setPrivateCloud(java.lang.String privateCloud) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRIVATE_CLOUD_PATTERN.matcher(privateCloud).matches(),
"Parameter privateCloud must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.privateCloud = privateCloud;
return this;
}
@Override
public ResetNsxCredentials set(String parameterName, Object value) {
return (ResetNsxCredentials) super.set(parameterName, value);
}
}
/**
* Resets credentials of the Vcenter appliance.
*
* Create a request for the method "privateClouds.resetVcenterCredentials".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link ResetVcenterCredentials#execute()} method to invoke the remote
* operation.
*
* @param privateCloud Required. The resource name of the private cloud to reset credentials for. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ResetVcenterCredentialsRequest}
* @return the request
*/
public ResetVcenterCredentials resetVcenterCredentials(java.lang.String privateCloud, com.google.api.services.vmwareengine.v1.model.ResetVcenterCredentialsRequest content) throws java.io.IOException {
ResetVcenterCredentials result = new ResetVcenterCredentials(privateCloud, content);
initialize(result);
return result;
}
public class ResetVcenterCredentials extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+privateCloud}:resetVcenterCredentials";
private final java.util.regex.Pattern PRIVATE_CLOUD_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Resets credentials of the Vcenter appliance.
*
* Create a request for the method "privateClouds.resetVcenterCredentials".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link ResetVcenterCredentials#execute()} method to invoke the
* remote operation. {@link ResetVcenterCredentials#initialize(com.google.api.client.googleapi
* s.services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param privateCloud Required. The resource name of the private cloud to reset credentials for. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ResetVcenterCredentialsRequest}
* @since 1.13
*/
protected ResetVcenterCredentials(java.lang.String privateCloud, com.google.api.services.vmwareengine.v1.model.ResetVcenterCredentialsRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.privateCloud = com.google.api.client.util.Preconditions.checkNotNull(privateCloud, "Required parameter privateCloud must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRIVATE_CLOUD_PATTERN.matcher(privateCloud).matches(),
"Parameter privateCloud must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public ResetVcenterCredentials set$Xgafv(java.lang.String $Xgafv) {
return (ResetVcenterCredentials) super.set$Xgafv($Xgafv);
}
@Override
public ResetVcenterCredentials setAccessToken(java.lang.String accessToken) {
return (ResetVcenterCredentials) super.setAccessToken(accessToken);
}
@Override
public ResetVcenterCredentials setAlt(java.lang.String alt) {
return (ResetVcenterCredentials) super.setAlt(alt);
}
@Override
public ResetVcenterCredentials setCallback(java.lang.String callback) {
return (ResetVcenterCredentials) super.setCallback(callback);
}
@Override
public ResetVcenterCredentials setFields(java.lang.String fields) {
return (ResetVcenterCredentials) super.setFields(fields);
}
@Override
public ResetVcenterCredentials setKey(java.lang.String key) {
return (ResetVcenterCredentials) super.setKey(key);
}
@Override
public ResetVcenterCredentials setOauthToken(java.lang.String oauthToken) {
return (ResetVcenterCredentials) super.setOauthToken(oauthToken);
}
@Override
public ResetVcenterCredentials setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ResetVcenterCredentials) super.setPrettyPrint(prettyPrint);
}
@Override
public ResetVcenterCredentials setQuotaUser(java.lang.String quotaUser) {
return (ResetVcenterCredentials) super.setQuotaUser(quotaUser);
}
@Override
public ResetVcenterCredentials setUploadType(java.lang.String uploadType) {
return (ResetVcenterCredentials) super.setUploadType(uploadType);
}
@Override
public ResetVcenterCredentials setUploadProtocol(java.lang.String uploadProtocol) {
return (ResetVcenterCredentials) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to reset credentials for. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String privateCloud;
/** Required. The resource name of the private cloud to reset credentials for. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getPrivateCloud() {
return privateCloud;
}
/**
* Required. The resource name of the private cloud to reset credentials for. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public ResetVcenterCredentials setPrivateCloud(java.lang.String privateCloud) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRIVATE_CLOUD_PATTERN.matcher(privateCloud).matches(),
"Parameter privateCloud must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.privateCloud = privateCloud;
return this;
}
@Override
public ResetVcenterCredentials set(String parameterName, Object value) {
return (ResetVcenterCredentials) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "privateClouds.setIamPolicy".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "privateClouds.setIamPolicy".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Gets details of credentials for NSX appliance.
*
* Create a request for the method "privateClouds.showNsxCredentials".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link ShowNsxCredentials#execute()} method to invoke the remote operation.
*
* @param privateCloud Required. The resource name of the private cloud to be queried for credentials. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public ShowNsxCredentials showNsxCredentials(java.lang.String privateCloud) throws java.io.IOException {
ShowNsxCredentials result = new ShowNsxCredentials(privateCloud);
initialize(result);
return result;
}
public class ShowNsxCredentials extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+privateCloud}:showNsxCredentials";
private final java.util.regex.Pattern PRIVATE_CLOUD_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Gets details of credentials for NSX appliance.
*
* Create a request for the method "privateClouds.showNsxCredentials".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link ShowNsxCredentials#execute()} method to invoke the remote
* operation. {@link ShowNsxCredentials#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param privateCloud Required. The resource name of the private cloud to be queried for credentials. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected ShowNsxCredentials(java.lang.String privateCloud) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Credentials.class);
this.privateCloud = com.google.api.client.util.Preconditions.checkNotNull(privateCloud, "Required parameter privateCloud must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRIVATE_CLOUD_PATTERN.matcher(privateCloud).matches(),
"Parameter privateCloud must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ShowNsxCredentials set$Xgafv(java.lang.String $Xgafv) {
return (ShowNsxCredentials) super.set$Xgafv($Xgafv);
}
@Override
public ShowNsxCredentials setAccessToken(java.lang.String accessToken) {
return (ShowNsxCredentials) super.setAccessToken(accessToken);
}
@Override
public ShowNsxCredentials setAlt(java.lang.String alt) {
return (ShowNsxCredentials) super.setAlt(alt);
}
@Override
public ShowNsxCredentials setCallback(java.lang.String callback) {
return (ShowNsxCredentials) super.setCallback(callback);
}
@Override
public ShowNsxCredentials setFields(java.lang.String fields) {
return (ShowNsxCredentials) super.setFields(fields);
}
@Override
public ShowNsxCredentials setKey(java.lang.String key) {
return (ShowNsxCredentials) super.setKey(key);
}
@Override
public ShowNsxCredentials setOauthToken(java.lang.String oauthToken) {
return (ShowNsxCredentials) super.setOauthToken(oauthToken);
}
@Override
public ShowNsxCredentials setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ShowNsxCredentials) super.setPrettyPrint(prettyPrint);
}
@Override
public ShowNsxCredentials setQuotaUser(java.lang.String quotaUser) {
return (ShowNsxCredentials) super.setQuotaUser(quotaUser);
}
@Override
public ShowNsxCredentials setUploadType(java.lang.String uploadType) {
return (ShowNsxCredentials) super.setUploadType(uploadType);
}
@Override
public ShowNsxCredentials setUploadProtocol(java.lang.String uploadProtocol) {
return (ShowNsxCredentials) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to be queried for credentials.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String privateCloud;
/** Required. The resource name of the private cloud to be queried for credentials. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getPrivateCloud() {
return privateCloud;
}
/**
* Required. The resource name of the private cloud to be queried for credentials.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public ShowNsxCredentials setPrivateCloud(java.lang.String privateCloud) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRIVATE_CLOUD_PATTERN.matcher(privateCloud).matches(),
"Parameter privateCloud must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.privateCloud = privateCloud;
return this;
}
@Override
public ShowNsxCredentials set(String parameterName, Object value) {
return (ShowNsxCredentials) super.set(parameterName, value);
}
}
/**
* Gets details of credentials for Vcenter appliance.
*
* Create a request for the method "privateClouds.showVcenterCredentials".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link ShowVcenterCredentials#execute()} method to invoke the remote
* operation.
*
* @param privateCloud Required. The resource name of the private cloud to be queried for credentials. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public ShowVcenterCredentials showVcenterCredentials(java.lang.String privateCloud) throws java.io.IOException {
ShowVcenterCredentials result = new ShowVcenterCredentials(privateCloud);
initialize(result);
return result;
}
public class ShowVcenterCredentials extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+privateCloud}:showVcenterCredentials";
private final java.util.regex.Pattern PRIVATE_CLOUD_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Gets details of credentials for Vcenter appliance.
*
* Create a request for the method "privateClouds.showVcenterCredentials".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link ShowVcenterCredentials#execute()} method to invoke the
* remote operation. {@link ShowVcenterCredentials#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param privateCloud Required. The resource name of the private cloud to be queried for credentials. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected ShowVcenterCredentials(java.lang.String privateCloud) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Credentials.class);
this.privateCloud = com.google.api.client.util.Preconditions.checkNotNull(privateCloud, "Required parameter privateCloud must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRIVATE_CLOUD_PATTERN.matcher(privateCloud).matches(),
"Parameter privateCloud must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ShowVcenterCredentials set$Xgafv(java.lang.String $Xgafv) {
return (ShowVcenterCredentials) super.set$Xgafv($Xgafv);
}
@Override
public ShowVcenterCredentials setAccessToken(java.lang.String accessToken) {
return (ShowVcenterCredentials) super.setAccessToken(accessToken);
}
@Override
public ShowVcenterCredentials setAlt(java.lang.String alt) {
return (ShowVcenterCredentials) super.setAlt(alt);
}
@Override
public ShowVcenterCredentials setCallback(java.lang.String callback) {
return (ShowVcenterCredentials) super.setCallback(callback);
}
@Override
public ShowVcenterCredentials setFields(java.lang.String fields) {
return (ShowVcenterCredentials) super.setFields(fields);
}
@Override
public ShowVcenterCredentials setKey(java.lang.String key) {
return (ShowVcenterCredentials) super.setKey(key);
}
@Override
public ShowVcenterCredentials setOauthToken(java.lang.String oauthToken) {
return (ShowVcenterCredentials) super.setOauthToken(oauthToken);
}
@Override
public ShowVcenterCredentials setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ShowVcenterCredentials) super.setPrettyPrint(prettyPrint);
}
@Override
public ShowVcenterCredentials setQuotaUser(java.lang.String quotaUser) {
return (ShowVcenterCredentials) super.setQuotaUser(quotaUser);
}
@Override
public ShowVcenterCredentials setUploadType(java.lang.String uploadType) {
return (ShowVcenterCredentials) super.setUploadType(uploadType);
}
@Override
public ShowVcenterCredentials setUploadProtocol(java.lang.String uploadProtocol) {
return (ShowVcenterCredentials) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to be queried for credentials.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String privateCloud;
/** Required. The resource name of the private cloud to be queried for credentials. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getPrivateCloud() {
return privateCloud;
}
/**
* Required. The resource name of the private cloud to be queried for credentials.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public ShowVcenterCredentials setPrivateCloud(java.lang.String privateCloud) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PRIVATE_CLOUD_PATTERN.matcher(privateCloud).matches(),
"Parameter privateCloud must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.privateCloud = privateCloud;
return this;
}
/**
* Optional. The username of the user to be queried for credentials. The default value of
* this field is [email protected]. The provided value must be one of the following:
* [email protected], [email protected], [email protected], solution-
* [email protected], [email protected], [email protected],
* [email protected].
*/
@com.google.api.client.util.Key
private java.lang.String username;
/** Optional. The username of the user to be queried for credentials. The default value of this field
is [email protected]. The provided value must be one of the following: [email protected],
[email protected], [email protected], [email protected], solution-
[email protected], [email protected], [email protected].
*/
public java.lang.String getUsername() {
return username;
}
/**
* Optional. The username of the user to be queried for credentials. The default value of
* this field is [email protected]. The provided value must be one of the following:
* [email protected], [email protected], [email protected], solution-
* [email protected], [email protected], [email protected],
* [email protected].
*/
public ShowVcenterCredentials setUsername(java.lang.String username) {
this.username = username;
return this;
}
@Override
public ShowVcenterCredentials set(String parameterName, Object value) {
return (ShowVcenterCredentials) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "privateClouds.testIamPermissions".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "privateClouds.testIamPermissions".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Restores a private cloud that was previously scheduled for deletion by `DeletePrivateCloud`. A
* `PrivateCloud` resource scheduled for deletion has `PrivateCloud.state` set to `DELETED` and
* `PrivateCloud.expireTime` set to the time when deletion can no longer be reversed.
*
* Create a request for the method "privateClouds.undelete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the private cloud scheduled for deletion. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.UndeletePrivateCloudRequest}
* @return the request
*/
public Undelete undelete(java.lang.String name, com.google.api.services.vmwareengine.v1.model.UndeletePrivateCloudRequest content) throws java.io.IOException {
Undelete result = new Undelete(name, content);
initialize(result);
return result;
}
public class Undelete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}:undelete";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Restores a private cloud that was previously scheduled for deletion by `DeletePrivateCloud`. A
* `PrivateCloud` resource scheduled for deletion has `PrivateCloud.state` set to `DELETED` and
* `PrivateCloud.expireTime` set to the time when deletion can no longer be reversed.
*
* Create a request for the method "privateClouds.undelete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
* {@link
* Undelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the private cloud scheduled for deletion. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.UndeletePrivateCloudRequest}
* @since 1.13
*/
protected Undelete(java.lang.String name, com.google.api.services.vmwareengine.v1.model.UndeletePrivateCloudRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public Undelete set$Xgafv(java.lang.String $Xgafv) {
return (Undelete) super.set$Xgafv($Xgafv);
}
@Override
public Undelete setAccessToken(java.lang.String accessToken) {
return (Undelete) super.setAccessToken(accessToken);
}
@Override
public Undelete setAlt(java.lang.String alt) {
return (Undelete) super.setAlt(alt);
}
@Override
public Undelete setCallback(java.lang.String callback) {
return (Undelete) super.setCallback(callback);
}
@Override
public Undelete setFields(java.lang.String fields) {
return (Undelete) super.setFields(fields);
}
@Override
public Undelete setKey(java.lang.String key) {
return (Undelete) super.setKey(key);
}
@Override
public Undelete setOauthToken(java.lang.String oauthToken) {
return (Undelete) super.setOauthToken(oauthToken);
}
@Override
public Undelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Undelete) super.setPrettyPrint(prettyPrint);
}
@Override
public Undelete setQuotaUser(java.lang.String quotaUser) {
return (Undelete) super.setQuotaUser(quotaUser);
}
@Override
public Undelete setUploadType(java.lang.String uploadType) {
return (Undelete) super.setUploadType(uploadType);
}
@Override
public Undelete setUploadProtocol(java.lang.String uploadProtocol) {
return (Undelete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud scheduled for deletion. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the private cloud scheduled for deletion. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the private cloud scheduled for deletion. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public Undelete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Undelete set(String parameterName, Object value) {
return (Undelete) super.set(parameterName, value);
}
}
/**
* Updates the parameters of the `DnsForwarding` config, like associated domains. Only fields
* specified in `update_mask` are applied.
*
* Create a request for the method "privateClouds.updateDnsForwarding".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link UpdateDnsForwarding#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this DNS profile. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/dnsForwarding`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.DnsForwarding}
* @return the request
*/
public UpdateDnsForwarding updateDnsForwarding(java.lang.String name, com.google.api.services.vmwareengine.v1.model.DnsForwarding content) throws java.io.IOException {
UpdateDnsForwarding result = new UpdateDnsForwarding(name, content);
initialize(result);
return result;
}
public class UpdateDnsForwarding extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/dnsForwarding$");
/**
* Updates the parameters of the `DnsForwarding` config, like associated domains. Only fields
* specified in `update_mask` are applied.
*
* Create a request for the method "privateClouds.updateDnsForwarding".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link UpdateDnsForwarding#execute()} method to invoke the remote
* operation. {@link UpdateDnsForwarding#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Output only. The resource name of this DNS profile. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/dnsForwarding`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.DnsForwarding}
* @since 1.13
*/
protected UpdateDnsForwarding(java.lang.String name, com.google.api.services.vmwareengine.v1.model.DnsForwarding content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/dnsForwarding$");
}
}
@Override
public UpdateDnsForwarding set$Xgafv(java.lang.String $Xgafv) {
return (UpdateDnsForwarding) super.set$Xgafv($Xgafv);
}
@Override
public UpdateDnsForwarding setAccessToken(java.lang.String accessToken) {
return (UpdateDnsForwarding) super.setAccessToken(accessToken);
}
@Override
public UpdateDnsForwarding setAlt(java.lang.String alt) {
return (UpdateDnsForwarding) super.setAlt(alt);
}
@Override
public UpdateDnsForwarding setCallback(java.lang.String callback) {
return (UpdateDnsForwarding) super.setCallback(callback);
}
@Override
public UpdateDnsForwarding setFields(java.lang.String fields) {
return (UpdateDnsForwarding) super.setFields(fields);
}
@Override
public UpdateDnsForwarding setKey(java.lang.String key) {
return (UpdateDnsForwarding) super.setKey(key);
}
@Override
public UpdateDnsForwarding setOauthToken(java.lang.String oauthToken) {
return (UpdateDnsForwarding) super.setOauthToken(oauthToken);
}
@Override
public UpdateDnsForwarding setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateDnsForwarding) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateDnsForwarding setQuotaUser(java.lang.String quotaUser) {
return (UpdateDnsForwarding) super.setQuotaUser(quotaUser);
}
@Override
public UpdateDnsForwarding setUploadType(java.lang.String uploadType) {
return (UpdateDnsForwarding) super.setUploadType(uploadType);
}
@Override
public UpdateDnsForwarding setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateDnsForwarding) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this DNS profile. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/dnsForwarding`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this DNS profile. Resource names are schemeless URIs that follow
the conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/us-central1-a/privateClouds/my-cloud/dnsForwarding`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this DNS profile. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/dnsForwarding`
*/
public UpdateDnsForwarding setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/dnsForwarding$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public UpdateDnsForwarding setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `DnsForwarding` resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `DnsForwarding`
resource by the update. The fields specified in the `update_mask` are relative to the resource, not
the full request. A field will be overwritten if it is in the mask. If the user does not provide a
mask then all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `DnsForwarding` resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten.
*/
public UpdateDnsForwarding setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateDnsForwarding set(String parameterName, Object value) {
return (UpdateDnsForwarding) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Clusters collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.Clusters.List request = vmwareengine.clusters().list(parameters ...)}
*
*
* @return the resource collection
*/
public Clusters clusters() {
return new Clusters();
}
/**
* The "clusters" collection of methods.
*/
public class Clusters {
/**
* Creates a new cluster in a given private cloud. Creating a new cluster provides additional nodes
* for use in the parent private cloud and requires sufficient [node quota](https://cloud.google.com
* /vmware-engine/quotas).
*
* Create a request for the method "clusters.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to create a new cluster in. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.Cluster}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.Cluster content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/clusters";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Creates a new cluster in a given private cloud. Creating a new cluster provides additional
* nodes for use in the parent private cloud and requires sufficient [node
* quota](https://cloud.google.com/vmware-engine/quotas).
*
* Create a request for the method "clusters.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to create a new cluster in. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.Cluster}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.Cluster content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to create a new cluster in. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to create a new cluster in. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to create a new cluster in. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the new `Cluster`. This identifier must be
* unique among clusters within the parent and becomes the final token in the name URI.
* The identifier must meet the following requirements: * Only contains 1-63
* alphanumeric characters and hyphens * Begins with an alphabetical character * Ends
* with a non-hyphen character * Not formatted as a UUID * Complies with [RFC
* 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String clusterId;
/** Required. The user-provided identifier of the new `Cluster`. This identifier must be unique among
clusters within the parent and becomes the final token in the name URI. The identifier must meet
the following requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
an alphabetical character * Ends with a non-hyphen character * Not formatted as a UUID * Complies
with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public java.lang.String getClusterId() {
return clusterId;
}
/**
* Required. The user-provided identifier of the new `Cluster`. This identifier must be
* unique among clusters within the parent and becomes the final token in the name URI.
* The identifier must meet the following requirements: * Only contains 1-63
* alphanumeric characters and hyphens * Begins with an alphabetical character * Ends
* with a non-hyphen character * Not formatted as a UUID * Complies with [RFC
* 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public Create setClusterId(java.lang.String clusterId) {
this.clusterId = clusterId;
return this;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is
* not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. The request ID must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is
* not supported (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Optional. True if you want the request to be validated and not executed; false
* otherwise.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Optional. True if you want the request to be validated and not executed; false otherwise.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Optional. True if you want the request to be validated and not executed; false
* otherwise.
*/
public Create setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `Cluster` resource. To avoid unintended data loss, migrate or gracefully shut down any
* workloads running on the cluster before deletion. You cannot delete the management cluster of a
* private cloud using this method.
*
* Create a request for the method "clusters.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the cluster to delete. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
/**
* Deletes a `Cluster` resource. To avoid unintended data loss, migrate or gracefully shut down
* any workloads running on the cluster before deletion. You cannot delete the management cluster
* of a private cloud using this method.
*
* Create a request for the method "clusters.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the cluster to delete. Resource names are schemeless URIs that follow
* the conventions in https://cloud.google.com/apis/design/resource_names. For example:
* `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the cluster to delete. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the cluster to delete. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the cluster to delete. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is
* not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. The request ID must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is
* not supported (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `Cluster` resource by its resource name.
*
* Create a request for the method "clusters.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The cluster resource name to retrieve. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
/**
* Retrieves a `Cluster` resource by its resource name.
*
* Create a request for the method "clusters.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The cluster resource name to retrieve. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Cluster.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The cluster resource name to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/clusters/my-cluster`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The cluster resource name to retrieve. Resource names are schemeless URIs that follow the
conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The cluster resource name to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/clusters/my-cluster`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "clusters.getIamPolicy".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "clusters.getIamPolicy".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists `Cluster` resources in a given private cloud.
*
* Create a request for the method "clusters.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to query for clusters. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/clusters";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Lists `Cluster` resources in a given private cloud.
*
* Create a request for the method "clusters.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to query for clusters. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListClustersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to query for clusters. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to query for clusters. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to query for clusters. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* To filter on multiple expressions, provide each separate expression within
* parentheses. For example: ``` (name = "example-cluster") (nodeCount = "3") ``` By
* default, each expression is an `AND` expression. However, you can include `AND` and
* `OR` expressions explicitly. For example: ``` (name = "example-cluster-1") AND
* (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-cluster-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** To filter on multiple expressions, provide each separate expression within parentheses. For
example: ``` (name = "example-cluster") (nodeCount = "3") ``` By default, each expression is an
`AND` expression. However, you can include `AND` and `OR` expressions explicitly. For example: ```
(name = "example-cluster-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-
cluster-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* To filter on multiple expressions, provide each separate expression within
* parentheses. For example: ``` (name = "example-cluster") (nodeCount = "3") ``` By
* default, each expression is an `AND` expression. However, you can include `AND` and
* `OR` expressions explicitly. For example: ``` (name = "example-cluster-1") AND
* (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-cluster-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of clusters to return in one page. The service may return fewer
* than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of clusters to return in one page. The service may return fewer than this value.
The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of clusters to return in one page. The service may return fewer
* than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListClusters` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListClusters`
* must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListClusters` call. Provide this to retrieve the subsequent
page. When paginating, all other parameters provided to `ListClusters` must match the call that
provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListClusters` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListClusters`
* must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies a `Cluster` resource. Only fields specified in `updateMask` are applied. During
* operation processing, the resource is temporarily in the `ACTIVE` state before the operation
* fully completes. For that period of time, you can't update the resource. Use the operation status
* to determine when the processing fully completes.
*
* Create a request for the method "clusters.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this cluster. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.Cluster}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.Cluster content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
/**
* Modifies a `Cluster` resource. Only fields specified in `updateMask` are applied. During
* operation processing, the resource is temporarily in the `ACTIVE` state before the operation
* fully completes. For that period of time, you can't update the resource. Use the operation
* status to determine when the processing fully completes.
*
* Create a request for the method "clusters.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of this cluster. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.Cluster}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.Cluster content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this cluster. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/clusters/my-cluster`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this cluster. Resource names are schemeless URIs that follow the
conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this cluster. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/clusters/my-cluster`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is
* not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. The request ID must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. The request ID must be a valid UUID with the exception that zero UUID is
* not supported (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the `Cluster`
* resource by the update. The fields specified in the `updateMask` are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask. If
* the user does not provide a mask then all fields will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `Cluster` resource by
the update. The fields specified in the `updateMask` are relative to the resource, not the full
request. A field will be overwritten if it is in the mask. If the user does not provide a mask then
all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the `Cluster`
* resource by the update. The fields specified in the `updateMask` are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask. If
* the user does not provide a mask then all fields will be overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
/**
* Optional. True if you want the request to be validated and not executed; false
* otherwise.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Optional. True if you want the request to be validated and not executed; false otherwise.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Optional. True if you want the request to be validated and not executed; false
* otherwise.
*/
public Patch setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "clusters.setIamPolicy".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "clusters.setIamPolicy".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "clusters.testIamPermissions".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "clusters.testIamPermissions".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Nodes collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.Nodes.List request = vmwareengine.nodes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Nodes nodes() {
return new Nodes();
}
/**
* The "nodes" collection of methods.
*/
public class Nodes {
/**
* Gets details of a single node.
*
* Create a request for the method "nodes.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the node to retrieve. For example: `projects/{project}/locations/{loc
* ation}/privateClouds/{private_cloud}/clusters/{cluster}/nodes/{node}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+/nodes/[^/]+$");
/**
* Gets details of a single node.
*
* Create a request for the method "nodes.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the node to retrieve. For example: `projects/{project}/locations/{loc
* ation}/privateClouds/{private_cloud}/clusters/{cluster}/nodes/{node}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Node.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+/nodes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the node to retrieve. For example: `projects/{projec
* t}/locations/{location}/privateClouds/{private_cloud}/clusters/{cluster}/nodes/{nod
* e}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the node to retrieve. For example: `projects/{project}/locations/{lo
cation}/privateClouds/{private_cloud}/clusters/{cluster}/nodes/{node}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the node to retrieve. For example: `projects/{projec
* t}/locations/{location}/privateClouds/{private_cloud}/clusters/{cluster}/nodes/{nod
* e}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+/nodes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists nodes in a given cluster.
*
* Create a request for the method "nodes.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the cluster to be queried for nodes. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/clusters
* /my-cluster`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/nodes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
/**
* Lists nodes in a given cluster.
*
* Create a request for the method "nodes.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the cluster to be queried for nodes. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/clusters
* /my-cluster`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListNodesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the cluster to be queried for nodes. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the cluster to be queried for nodes. Resource names are schemeless
URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the cluster to be queried for nodes. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/clusters/my-cluster`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/clusters/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of nodes to return in one page. The service may return fewer
* than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of nodes to return in one page. The service may return fewer than this value.
The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of nodes to return in one page. The service may return fewer
* than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListNodes` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListNodes`
* must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListNodes` call. Provide this to retrieve the subsequent
page. When paginating, all other parameters provided to `ListNodes` must match the call that
provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListNodes` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListNodes`
* must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the ExternalAddresses collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.ExternalAddresses.List request = vmwareengine.externalAddresses().list(parameters ...)}
*
*
* @return the resource collection
*/
public ExternalAddresses externalAddresses() {
return new ExternalAddresses();
}
/**
* The "externalAddresses" collection of methods.
*/
public class ExternalAddresses {
/**
* Creates a new `ExternalAddress` resource in a given private cloud. The network policy that
* corresponds to the private cloud must have the external IP address network service enabled
* (`NetworkPolicy.external_ip`).
*
* Create a request for the method "externalAddresses.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to create a new external IP address in. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ExternalAddress}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.ExternalAddress content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/externalAddresses";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Creates a new `ExternalAddress` resource in a given private cloud. The network policy that
* corresponds to the private cloud must have the external IP address network service enabled
* (`NetworkPolicy.external_ip`).
*
* Create a request for the method "externalAddresses.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to create a new external IP address in. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ExternalAddress}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.ExternalAddress content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to create a new external IP address
* in. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to create a new external IP address in. Resource
names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to create a new external IP address
* in. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the `ExternalAddress` to be created. This
* identifier must be unique among `ExternalAddress` resources within the parent and
* becomes the final token in the name URI. The identifier must meet the following
* requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
* an alphabetical character * Ends with a non-hyphen character * Not formatted as a
* UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
* (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String externalAddressId;
/** Required. The user-provided identifier of the `ExternalAddress` to be created. This identifier must
be unique among `ExternalAddress` resources within the parent and becomes the final token in the
name URI. The identifier must meet the following requirements: * Only contains 1-63 alphanumeric
characters and hyphens * Begins with an alphabetical character * Ends with a non-hyphen character *
Not formatted as a UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
(section 3.5)
*/
public java.lang.String getExternalAddressId() {
return externalAddressId;
}
/**
* Required. The user-provided identifier of the `ExternalAddress` to be created. This
* identifier must be unique among `ExternalAddress` resources within the parent and
* becomes the final token in the name URI. The identifier must meet the following
* requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
* an alphabetical character * Ends with a non-hyphen character * Not formatted as a
* UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
* (section 3.5)
*/
public Create setExternalAddressId(java.lang.String externalAddressId) {
this.externalAddressId = externalAddressId;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if the
original operation with the same request ID was received, and if so, will ignore the second
request. This prevents clients from accidentally creating duplicate commitments. The request ID
must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single external IP address. When you delete an external IP address, connectivity
* between the external IP address and the corresponding internal IP address is lost.
*
* Create a request for the method "externalAddresses.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the external IP address to delete. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/externalAddresses/my-ip`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
/**
* Deletes a single external IP address. When you delete an external IP address, connectivity
* between the external IP address and the corresponding internal IP address is lost.
*
* Create a request for the method "externalAddresses.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the external IP address to delete. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/externalAddresses/my-ip`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the external IP address to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-ip`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the external IP address to delete. Resource names are schemeless
URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-
ip`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the external IP address to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-ip`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if the
original operation with the same request ID was received, and if so, will ignore the second
request. This prevents clients from accidentally creating duplicate commitments. The request ID
must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single external IP address.
*
* Create a request for the method "externalAddresses.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the external IP address to retrieve. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/externalAddresses/my-ip`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
/**
* Gets details of a single external IP address.
*
* Create a request for the method "externalAddresses.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the external IP address to retrieve. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/externalAddresses/my-ip`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ExternalAddress.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the external IP address to retrieve. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-ip`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the external IP address to retrieve. Resource names are schemeless
URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-
ip`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the external IP address to retrieve. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-ip`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists external IP addresses assigned to VMware workload VMs in a given private cloud.
*
* Create a request for the method "externalAddresses.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to be queried for external IP addresses. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/externalAddresses";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Lists external IP addresses assigned to VMware workload VMs in a given private cloud.
*
* Create a request for the method "externalAddresses.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to be queried for external IP addresses. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListExternalAddressesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to be queried for external IP
* addresses. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to be queried for external IP addresses. Resource
names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to be queried for external IP
* addresses. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to
* use for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* IP addresses, you can exclude the ones named `example-ip` by specifying `name !=
* "example-ip"`. To filter on multiple expressions, provide each separate expression
* within parentheses. For example: ``` (name = "example-ip") (createTime >
* "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ```
* (name = "example-ip-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-ip-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of IP addresses, you can exclude the ones named `example-
ip` by specifying `name != "example-ip"`. To filter on multiple expressions, provide each separate
expression within parentheses. For example: ``` (name = "example-ip") (createTime >
"2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression. However, you can
include `AND` and `OR` expressions explicitly. For example: ``` (name = "example-ip-1") AND
(createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-ip-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to
* use for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* IP addresses, you can exclude the ones named `example-ip` by specifying `name !=
* "example-ip"`. To filter on multiple expressions, provide each separate expression
* within parentheses. For example: ``` (name = "example-ip") (createTime >
* "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ```
* (name = "example-ip-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-ip-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of external IP addresses to return in one page. The service may
* return fewer than this value. The maximum value is coerced to 1000. The default value
* of this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of external IP addresses to return in one page. The service may return fewer
than this value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of external IP addresses to return in one page. The service may
* return fewer than this value. The maximum value is coerced to 1000. The default value
* of this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListExternalAddresses` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListExternalAddresses` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListExternalAddresses` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListExternalAddresses` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListExternalAddresses` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListExternalAddresses` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the parameters of a single external IP address. Only fields specified in `update_mask`
* are applied. During operation processing, the resource is temporarily in the `ACTIVE` state
* before the operation fully completes. For that period of time, you can't update the resource. Use
* the operation status to determine when the processing fully completes.
*
* Create a request for the method "externalAddresses.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this external IP address. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/externalAddresses/my-address`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ExternalAddress}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.ExternalAddress content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
/**
* Updates the parameters of a single external IP address. Only fields specified in `update_mask`
* are applied. During operation processing, the resource is temporarily in the `ACTIVE` state
* before the operation fully completes. For that period of time, you can't update the resource.
* Use the operation status to determine when the processing fully completes.
*
* Create a request for the method "externalAddresses.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of this external IP address. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/externalAddresses/my-address`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ExternalAddress}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.ExternalAddress content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this external IP address. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-address`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this external IP address. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-address`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this external IP address. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/externalAddresses/my-address`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/externalAddresses/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if the
original operation with the same request ID was received, and if so, will ignore the second
request. This prevents clients from accidentally creating duplicate commitments. The request ID
must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `ExternalAddress` resource by the update. The fields specified in the `update_mask`
* are relative to the resource, not the full request. A field will be overwritten if it
* is in the mask. If the user does not provide a mask then all fields will be
* overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `ExternalAddress`
resource by the update. The fields specified in the `update_mask` are relative to the resource, not
the full request. A field will be overwritten if it is in the mask. If the user does not provide a
mask then all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `ExternalAddress` resource by the update. The fields specified in the `update_mask`
* are relative to the resource, not the full request. A field will be overwritten if it
* is in the mask. If the user does not provide a mask then all fields will be
* overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the HcxActivationKeys collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.HcxActivationKeys.List request = vmwareengine.hcxActivationKeys().list(parameters ...)}
*
*
* @return the resource collection
*/
public HcxActivationKeys hcxActivationKeys() {
return new HcxActivationKeys();
}
/**
* The "hcxActivationKeys" collection of methods.
*/
public class HcxActivationKeys {
/**
* Creates a new HCX activation key in a given private cloud.
*
* Create a request for the method "hcxActivationKeys.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to create the key for. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.HcxActivationKey}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.HcxActivationKey content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/hcxActivationKeys";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Creates a new HCX activation key in a given private cloud.
*
* Create a request for the method "hcxActivationKeys.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to create the key for. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.HcxActivationKey}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.HcxActivationKey content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to create the key for. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to create the key for. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to create the key for. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the `HcxActivationKey` to be created. This
* identifier must be unique among `HcxActivationKey` resources within the parent and
* becomes the final token in the name URI. The identifier must meet the following
* requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
* an alphabetical character * Ends with a non-hyphen character * Not formatted as a
* UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
* (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String hcxActivationKeyId;
/** Required. The user-provided identifier of the `HcxActivationKey` to be created. This identifier
must be unique among `HcxActivationKey` resources within the parent and becomes the final token in
the name URI. The identifier must meet the following requirements: * Only contains 1-63
alphanumeric characters and hyphens * Begins with an alphabetical character * Ends with a non-
hyphen character * Not formatted as a UUID * Complies with [RFC
1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public java.lang.String getHcxActivationKeyId() {
return hcxActivationKeyId;
}
/**
* Required. The user-provided identifier of the `HcxActivationKey` to be created. This
* identifier must be unique among `HcxActivationKey` resources within the parent and
* becomes the final token in the name URI. The identifier must meet the following
* requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
* an alphabetical character * Ends with a non-hyphen character * Not formatted as a
* UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
* (section 3.5)
*/
public Create setHcxActivationKeyId(java.lang.String hcxActivationKeyId) {
this.hcxActivationKeyId = hcxActivationKeyId;
return this;
}
/**
* A request ID to identify requests. Specify a unique request ID so that if you must
* retry your request, the server will know to ignore the request if it has already been
* completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation
* where you make an initial request and the request times out. If you make the request
* again with the same request ID, the server can check if original operation with the
* same request ID was received, and if so, will ignore the second request. This
* prevents clients from accidentally creating duplicate commitments. The request ID
* must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** A request ID to identify requests. Specify a unique request ID so that if you must retry your
request, the server will know to ignore the request if it has already been completed. The server
guarantees that a request doesn't result in creation of duplicate commitments for at least 60
minutes. For example, consider a situation where you make an initial request and the request times
out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* A request ID to identify requests. Specify a unique request ID so that if you must
* retry your request, the server will know to ignore the request if it has already been
* completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation
* where you make an initial request and the request times out. If you make the request
* again with the same request ID, the server can check if original operation with the
* same request ID was received, and if so, will ignore the second request. This
* prevents clients from accidentally creating duplicate commitments. The request ID
* must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Retrieves a `HcxActivationKey` resource by its resource name.
*
* Create a request for the method "hcxActivationKeys.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the HCX activation key to retrieve. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1/privateClouds/my-
* cloud/hcxActivationKeys/my-key`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
/**
* Retrieves a `HcxActivationKey` resource by its resource name.
*
* Create a request for the method "hcxActivationKeys.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the HCX activation key to retrieve. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1/privateClouds/my-
* cloud/hcxActivationKeys/my-key`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.HcxActivationKey.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the HCX activation key to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud/hcxActivationKeys/my-key`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the HCX activation key to retrieve. Resource names are schemeless
URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
example: `projects/my-project/locations/us-central1/privateClouds/my-cloud/hcxActivationKeys/my-
key`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the HCX activation key to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud/hcxActivationKeys/my-key`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "hcxActivationKeys.getIamPolicy".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "hcxActivationKeys.getIamPolicy".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected.
* Requests for policies with any conditional role bindings must specify version 3.
* Policies with no conditional role bindings may specify any valid value or leave the
* field unset. The policy in the response might use the policy version that you
* specified, or it might use a lower policy version. For example, if you specify
* version 3, but the policy has no conditional role bindings, the response uses version
* 1. To learn which resources support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists `HcxActivationKey` resources in a given private cloud.
*
* Create a request for the method "hcxActivationKeys.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to be queried for HCX activation keys. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/hcxActivationKeys";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Lists `HcxActivationKey` resources in a given private cloud.
*
* Create a request for the method "hcxActivationKeys.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to be queried for HCX activation keys. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListHcxActivationKeysResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to be queried for HCX activation
* keys. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to be queried for HCX activation keys. Resource
names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to be queried for HCX activation
* keys. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateClouds/my-cloud`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of HCX activation keys to return in one page. The service may
* return fewer than this value. The maximum value is coerced to 1000. The default value
* of this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of HCX activation keys to return in one page. The service may return fewer than
this value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of HCX activation keys to return in one page. The service may
* return fewer than this value. The maximum value is coerced to 1000. The default value
* of this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListHcxActivationKeys` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListHcxActivationKeys` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListHcxActivationKeys` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListHcxActivationKeys` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListHcxActivationKeys` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListHcxActivationKeys` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "hcxActivationKeys.setIamPolicy".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "hcxActivationKeys.setIamPolicy".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.SetIamPolicyRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "hcxActivationKeys.testIamPermissions".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "hcxActivationKeys.testIamPermissions".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/hcxActivationKeys/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the LoggingServers collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.LoggingServers.List request = vmwareengine.loggingServers().list(parameters ...)}
*
*
* @return the resource collection
*/
public LoggingServers loggingServers() {
return new LoggingServers();
}
/**
* The "loggingServers" collection of methods.
*/
public class LoggingServers {
/**
* Create a new logging server for a given private cloud.
*
* Create a request for the method "loggingServers.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to create a new Logging Server in. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.LoggingServer}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.LoggingServer content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/loggingServers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Create a new logging server for a given private cloud.
*
* Create a request for the method "loggingServers.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to create a new Logging Server in. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.LoggingServer}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.LoggingServer content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to create a new Logging Server in.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to create a new Logging Server in. Resource names
are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to create a new Logging Server in.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the `LoggingServer` to be created. This
* identifier must be unique among `LoggingServer` resources within the parent and
* becomes the final token in the name URI. The identifier must meet the following
* requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
* an alphabetical character * Ends with a non-hyphen character * Not formatted as a
* UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
* (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String loggingServerId;
/** Required. The user-provided identifier of the `LoggingServer` to be created. This identifier must
be unique among `LoggingServer` resources within the parent and becomes the final token in the name
URI. The identifier must meet the following requirements: * Only contains 1-63 alphanumeric
characters and hyphens * Begins with an alphabetical character * Ends with a non-hyphen character *
Not formatted as a UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
(section 3.5)
*/
public java.lang.String getLoggingServerId() {
return loggingServerId;
}
/**
* Required. The user-provided identifier of the `LoggingServer` to be created. This
* identifier must be unique among `LoggingServer` resources within the parent and
* becomes the final token in the name URI. The identifier must meet the following
* requirements: * Only contains 1-63 alphanumeric characters and hyphens * Begins with
* an alphabetical character * Ends with a non-hyphen character * Not formatted as a
* UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
* (section 3.5)
*/
public Create setLoggingServerId(java.lang.String loggingServerId) {
this.loggingServerId = loggingServerId;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single logging server.
*
* Create a request for the method "loggingServers.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the logging server to delete. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/loggingServers/my-logging-server`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
/**
* Deletes a single logging server.
*
* Create a request for the method "loggingServers.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the logging server to delete. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/loggingServers/my-logging-server`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the logging server to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
* server`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the logging server to delete. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
server`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the logging server to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
* server`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a logging server.
*
* Create a request for the method "loggingServers.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the Logging Server to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/loggingServers/my-logging-server`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
/**
* Gets details of a logging server.
*
* Create a request for the method "loggingServers.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the Logging Server to retrieve. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/loggingServers/my-logging-server`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.LoggingServer.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Logging Server to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
* server`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the Logging Server to retrieve. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
server`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the Logging Server to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
* server`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists logging servers configured for a given private cloud.
*
* Create a request for the method "loggingServers.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to be queried for logging servers. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/loggingServers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Lists logging servers configured for a given private cloud.
*
* Create a request for the method "loggingServers.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to be queried for logging servers. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListLoggingServersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to be queried for logging servers.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to be queried for logging servers. Resource names
are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to be queried for logging servers.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to
* use for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* logging servers, you can exclude the ones named `example-server` by specifying `name
* != "example-server"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-server") (createTime
* > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ```
* (name = "example-server-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-server-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of logging servers, you can exclude the ones named
`example-server` by specifying `name != "example-server"`. To filter on multiple expressions,
provide each separate expression within parentheses. For example: ``` (name = "example-server")
(createTime > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name = "example-
server-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-server-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to
* use for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* logging servers, you can exclude the ones named `example-server` by specifying `name
* != "example-server"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-server") (createTime
* > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ```
* (name = "example-server-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-server-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of logging servers to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of
* this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of logging servers to return in one page. The service may return fewer than this
value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of logging servers to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of
* this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListLoggingServersRequest` call. Provide this
* to retrieve the subsequent page. When paginating, all other parameters provided to
* `ListLoggingServersRequest` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListLoggingServersRequest` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListLoggingServersRequest`
must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListLoggingServersRequest` call. Provide this
* to retrieve the subsequent page. When paginating, all other parameters provided to
* `ListLoggingServersRequest` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the parameters of a single logging server. Only fields specified in `update_mask` are
* applied.
*
* Create a request for the method "loggingServers.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this logging server. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/loggingServers/my-logging-server`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.LoggingServer}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.LoggingServer content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
/**
* Updates the parameters of a single logging server. Only fields specified in `update_mask` are
* applied.
*
* Create a request for the method "loggingServers.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of this logging server. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/loggingServers/my-logging-server`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.LoggingServer}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.LoggingServer content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this logging server. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
* server`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this logging server. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
server`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this logging server. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/loggingServers/my-logging-
* server`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/loggingServers/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `LoggingServer` resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is
* in the mask. If the user does not provide a mask then all fields will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `LoggingServer`
resource by the update. The fields specified in the `update_mask` are relative to the resource, not
the full request. A field will be overwritten if it is in the mask. If the user does not provide a
mask then all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `LoggingServer` resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is
* in the mask. If the user does not provide a mask then all fields will be overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ManagementDnsZoneBindings collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.ManagementDnsZoneBindings.List request = vmwareengine.managementDnsZoneBindings().list(parameters ...)}
*
*
* @return the resource collection
*/
public ManagementDnsZoneBindings managementDnsZoneBindings() {
return new ManagementDnsZoneBindings();
}
/**
* The "managementDnsZoneBindings" collection of methods.
*/
public class ManagementDnsZoneBindings {
/**
* Creates a new `ManagementDnsZoneBinding` resource in a private cloud. This RPC creates the DNS
* binding and the resource that represents the DNS binding of the consumer VPC network to the
* management DNS zone. A management DNS zone is the Cloud DNS cross-project binding zone that
* VMware Engine creates for each private cloud. It contains FQDNs and corresponding IP addresses
* for the private cloud's ESXi hosts and management VM appliances like vCenter and NSX Manager.
*
* Create a request for the method "managementDnsZoneBindings.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to create a new management DNS zone binding for.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/managementDnsZoneBindings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Creates a new `ManagementDnsZoneBinding` resource in a private cloud. This RPC creates the DNS
* binding and the resource that represents the DNS binding of the consumer VPC network to the
* management DNS zone. A management DNS zone is the Cloud DNS cross-project binding zone that
* VMware Engine creates for each private cloud. It contains FQDNs and corresponding IP addresses
* for the private cloud's ESXi hosts and management VM appliances like vCenter and NSX Manager.
*
* Create a request for the method "managementDnsZoneBindings.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to create a new management DNS zone binding for.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to create a new management DNS zone
* binding for. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to create a new management DNS zone binding for.
Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to create a new management DNS zone
* binding for. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the `ManagementDnsZoneBinding` resource to
* be created. This identifier must be unique among `ManagementDnsZoneBinding` resources
* within the parent and becomes the final token in the name URI. The identifier must
* meet the following requirements: * Only contains 1-63 alphanumeric characters and
* hyphens * Begins with an alphabetical character * Ends with a non-hyphen character *
* Not formatted as a UUID * Complies with [RFC
* 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String managementDnsZoneBindingId;
/** Required. The user-provided identifier of the `ManagementDnsZoneBinding` resource to be created.
This identifier must be unique among `ManagementDnsZoneBinding` resources within the parent and
becomes the final token in the name URI. The identifier must meet the following requirements: *
Only contains 1-63 alphanumeric characters and hyphens * Begins with an alphabetical character *
Ends with a non-hyphen character * Not formatted as a UUID * Complies with [RFC
1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public java.lang.String getManagementDnsZoneBindingId() {
return managementDnsZoneBindingId;
}
/**
* Required. The user-provided identifier of the `ManagementDnsZoneBinding` resource to
* be created. This identifier must be unique among `ManagementDnsZoneBinding` resources
* within the parent and becomes the final token in the name URI. The identifier must
* meet the following requirements: * Only contains 1-63 alphanumeric characters and
* hyphens * Begins with an alphabetical character * Ends with a non-hyphen character *
* Not formatted as a UUID * Complies with [RFC
* 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public Create setManagementDnsZoneBindingId(java.lang.String managementDnsZoneBindingId) {
this.managementDnsZoneBindingId = managementDnsZoneBindingId;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if the
original operation with the same request ID was received, and if so, will ignore the second
request. This prevents clients from accidentally creating duplicate commitments. The request ID
must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `ManagementDnsZoneBinding` resource. When a management DNS zone binding is deleted, the
* corresponding consumer VPC network is no longer bound to the management DNS zone.
*
* Create a request for the method "managementDnsZoneBindings.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the management DNS zone binding to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
/**
* Deletes a `ManagementDnsZoneBinding` resource. When a management DNS zone binding is deleted,
* the corresponding consumer VPC network is no longer bound to the management DNS zone.
*
* Create a request for the method "managementDnsZoneBindings.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the management DNS zone binding to delete. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the management DNS zone binding to delete. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the management DNS zone binding to delete. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
cloud/managementDnsZoneBindings/my-management-dns-zone-binding`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the management DNS zone binding to delete. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if the
original operation with the same request ID was received, and if so, will ignore the second
request. This prevents clients from accidentally creating duplicate commitments. The request ID
must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a 'ManagementDnsZoneBinding' resource by its resource name.
*
* Create a request for the method "managementDnsZoneBindings.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the management DNS zone binding to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
/**
* Retrieves a 'ManagementDnsZoneBinding' resource by its resource name.
*
* Create a request for the method "managementDnsZoneBindings.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the management DNS zone binding to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the management DNS zone binding to retrieve. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the management DNS zone binding to retrieve. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
cloud/managementDnsZoneBindings/my-management-dns-zone-binding`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the management DNS zone binding to retrieve. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists Consumer VPCs bound to Management DNS Zone of a given private cloud.
*
* Create a request for the method "managementDnsZoneBindings.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to be queried for management DNS zone bindings.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/managementDnsZoneBindings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Lists Consumer VPCs bound to Management DNS Zone of a given private cloud.
*
* Create a request for the method "managementDnsZoneBindings.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to be queried for management DNS zone bindings.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListManagementDnsZoneBindingsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to be queried for management DNS
* zone bindings. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to be queried for management DNS zone bindings.
Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to be queried for management DNS
* zone bindings. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to
* use for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* Management DNS Zone Bindings, you can exclude the ones named `example-management-dns-
* zone-binding` by specifying `name != "example-management-dns-zone-binding"`. To
* filter on multiple expressions, provide each separate expression within parentheses.
* For example: ``` (name = "example-management-dns-zone-binding") (createTime >
* "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ```
* (name = "example-management-dns-zone-binding-1") AND (createTime >
* "2021-04-12T08:15:10.40Z") OR (name = "example-management-dns-zone-binding-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of Management DNS Zone Bindings, you can exclude the ones
named `example-management-dns-zone-binding` by specifying `name != "example-management-dns-zone-
binding"`. To filter on multiple expressions, provide each separate expression within parentheses.
For example: ``` (name = "example-management-dns-zone-binding") (createTime >
"2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression. However, you can
include `AND` and `OR` expressions explicitly. For example: ``` (name = "example-management-dns-
zone-binding-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-management-dns-
zone-binding-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to
* use for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* Management DNS Zone Bindings, you can exclude the ones named `example-management-dns-
* zone-binding` by specifying `name != "example-management-dns-zone-binding"`. To
* filter on multiple expressions, provide each separate expression within parentheses.
* For example: ``` (name = "example-management-dns-zone-binding") (createTime >
* "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ```
* (name = "example-management-dns-zone-binding-1") AND (createTime >
* "2021-04-12T08:15:10.40Z") OR (name = "example-management-dns-zone-binding-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of management DNS zone bindings to return in one page. The service
* may return fewer than this value. The maximum value is coerced to 1000. The default
* value of this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of management DNS zone bindings to return in one page. The service may return
fewer than this value. The maximum value is coerced to 1000. The default value of this field is
500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of management DNS zone bindings to return in one page. The service
* may return fewer than this value. The maximum value is coerced to 1000. The default
* value of this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListManagementDnsZoneBindings` call. Provide
* this to retrieve the subsequent page. When paginating, all other parameters provided
* to `ListManagementDnsZoneBindings` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListManagementDnsZoneBindings` call. Provide this to
retrieve the subsequent page. When paginating, all other parameters provided to
`ListManagementDnsZoneBindings` must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListManagementDnsZoneBindings` call. Provide
* this to retrieve the subsequent page. When paginating, all other parameters provided
* to `ListManagementDnsZoneBindings` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a `ManagementDnsZoneBinding` resource. Only fields specified in `update_mask` are
* applied.
*
* Create a request for the method "managementDnsZoneBindings.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this binding. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
/**
* Updates a `ManagementDnsZoneBinding` resource. Only fields specified in `update_mask` are
* applied.
*
* Create a request for the method "managementDnsZoneBindings.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of this binding. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.ManagementDnsZoneBinding content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this binding. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/managementDnsZoneBindings/my-management-dns-zone-binding`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this binding. Resource names are schemeless URIs that follow the
conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-management-dns-
zone-binding`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this binding. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/managementDnsZoneBindings/my-management-dns-zone-binding`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if the
original operation with the same request ID was received, and if so, will ignore the second
request. This prevents clients from accidentally creating duplicate commitments. The request ID
must be a valid UUID with the exception that zero UUID is not supported
(00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if
* you must retry your request, the server will know to ignore the request if it has
* already been completed. The server guarantees that a request doesn't result in
* creation of duplicate commitments for at least 60 minutes. For example, consider a
* situation where you make an initial request and the request times out. If you make
* the request again with the same request ID, the server can check if the original
* operation with the same request ID was received, and if so, will ignore the second
* request. This prevents clients from accidentally creating duplicate commitments. The
* request ID must be a valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `ManagementDnsZoneBinding` resource by the update. The fields specified in the
* `update_mask` are relative to the resource, not the full request. A field will be
* overwritten if it is in the mask. If the user does not provide a mask then all fields
* will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the
`ManagementDnsZoneBinding` resource by the update. The fields specified in the `update_mask` are
relative to the resource, not the full request. A field will be overwritten if it is in the mask.
If the user does not provide a mask then all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `ManagementDnsZoneBinding` resource by the update. The fields specified in the
* `update_mask` are relative to the resource, not the full request. A field will be
* overwritten if it is in the mask. If the user does not provide a mask then all fields
* will be overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Retries to create a `ManagementDnsZoneBinding` resource that is in failed state.
*
* Create a request for the method "managementDnsZoneBindings.repair".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Repair#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the management DNS zone binding to repair. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.RepairManagementDnsZoneBindingRequest}
* @return the request
*/
public Repair repair(java.lang.String name, com.google.api.services.vmwareengine.v1.model.RepairManagementDnsZoneBindingRequest content) throws java.io.IOException {
Repair result = new Repair(name, content);
initialize(result);
return result;
}
public class Repair extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}:repair";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
/**
* Retries to create a `ManagementDnsZoneBinding` resource that is in failed state.
*
* Create a request for the method "managementDnsZoneBindings.repair".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Repair#execute()} method to invoke the remote operation.
* {@link
* Repair#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the management DNS zone binding to repair. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.RepairManagementDnsZoneBindingRequest}
* @since 1.13
*/
protected Repair(java.lang.String name, com.google.api.services.vmwareengine.v1.model.RepairManagementDnsZoneBindingRequest content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
}
}
@Override
public Repair set$Xgafv(java.lang.String $Xgafv) {
return (Repair) super.set$Xgafv($Xgafv);
}
@Override
public Repair setAccessToken(java.lang.String accessToken) {
return (Repair) super.setAccessToken(accessToken);
}
@Override
public Repair setAlt(java.lang.String alt) {
return (Repair) super.setAlt(alt);
}
@Override
public Repair setCallback(java.lang.String callback) {
return (Repair) super.setCallback(callback);
}
@Override
public Repair setFields(java.lang.String fields) {
return (Repair) super.setFields(fields);
}
@Override
public Repair setKey(java.lang.String key) {
return (Repair) super.setKey(key);
}
@Override
public Repair setOauthToken(java.lang.String oauthToken) {
return (Repair) super.setOauthToken(oauthToken);
}
@Override
public Repair setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Repair) super.setPrettyPrint(prettyPrint);
}
@Override
public Repair setQuotaUser(java.lang.String quotaUser) {
return (Repair) super.setQuotaUser(quotaUser);
}
@Override
public Repair setUploadType(java.lang.String uploadType) {
return (Repair) super.setUploadType(uploadType);
}
@Override
public Repair setUploadProtocol(java.lang.String uploadProtocol) {
return (Repair) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the management DNS zone binding to repair. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the management DNS zone binding to repair. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
cloud/managementDnsZoneBindings/my-management-dns-zone-binding`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the management DNS zone binding to repair. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/managementDnsZoneBindings/my-
* management-dns-zone-binding`
*/
public Repair setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/managementDnsZoneBindings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Repair set(String parameterName, Object value) {
return (Repair) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Subnets collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.Subnets.List request = vmwareengine.subnets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Subnets subnets() {
return new Subnets();
}
/**
* The "subnets" collection of methods.
*/
public class Subnets {
/**
* Gets details of a single subnet.
*
* Create a request for the method "subnets.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the subnet to retrieve. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/subnets/my-
* subnet`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/subnets/[^/]+$");
/**
* Gets details of a single subnet.
*
* Create a request for the method "subnets.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the subnet to retrieve. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud/subnets/my-
* subnet`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Subnet.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/subnets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the subnet to retrieve. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/subnets/my-subnet`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the subnet to retrieve. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1-a/privateClouds/my-cloud/subnets/my-subnet`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the subnet to retrieve. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud/subnets/my-subnet`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/subnets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists subnets in a given private cloud.
*
* Create a request for the method "subnets.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private cloud to be queried for subnets. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/subnets";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
/**
* Lists subnets in a given private cloud.
*
* Create a request for the method "subnets.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private cloud to be queried for subnets. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListSubnetsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private cloud to be queried for subnets. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private cloud to be queried for subnets. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1-a/privateClouds/my-cloud`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private cloud to be queried for subnets. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1-a/privateClouds/my-cloud`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of subnets to return in one page. The service may return fewer
* than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of subnets to return in one page. The service may return fewer than this value.
The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of subnets to return in one page. The service may return fewer
* than this value. The maximum value is coerced to 1000. The default value of this
* field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListSubnetsRequest` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSubnetsRequest` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListSubnetsRequest` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListSubnetsRequest` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListSubnetsRequest` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSubnetsRequest` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the parameters of a single subnet. Only fields specified in `update_mask` are applied.
* *Note*: This API is synchronous and always returns a successful `google.longrunning.Operation`
* (LRO). The returned LRO will only have `done` and `response` fields.
*
* Create a request for the method "subnets.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of this subnet. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1-a/privateClouds/my-cloud/subnets/my-subnet`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.Subnet}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.Subnet content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/subnets/[^/]+$");
/**
* Updates the parameters of a single subnet. Only fields specified in `update_mask` are applied.
* *Note*: This API is synchronous and always returns a successful `google.longrunning.Operation`
* (LRO). The returned LRO will only have `done` and `response` fields.
*
* Create a request for the method "subnets.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of this subnet. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1-a/privateClouds/my-cloud/subnets/my-subnet`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.Subnet}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.Subnet content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/subnets/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of this subnet. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/subnets/my-subnet`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of this subnet. Resource names are schemeless URIs that follow the
conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/us-central1-a/privateClouds/my-cloud/subnets/my-subnet`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of this subnet. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1-a/privateClouds/my-
* cloud/subnets/my-subnet`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateClouds/[^/]+/subnets/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the `Subnet`
* resource by the update. The fields specified in the `update_mask` are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask. If
* the user does not provide a mask then all fields will be overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `Subnet` resource by
the update. The fields specified in the `update_mask` are relative to the resource, not the full
request. A field will be overwritten if it is in the mask. If the user does not provide a mask then
all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the `Subnet`
* resource by the update. The fields specified in the `update_mask` are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask. If
* the user does not provide a mask then all fields will be overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the PrivateConnections collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.PrivateConnections.List request = vmwareengine.privateConnections().list(parameters ...)}
*
*
* @return the resource collection
*/
public PrivateConnections privateConnections() {
return new PrivateConnections();
}
/**
* The "privateConnections" collection of methods.
*/
public class PrivateConnections {
/**
* Creates a new private connection that can be used for accessing private Clouds.
*
* Create a request for the method "privateConnections.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location to create the new private connection in. Private
* connection is a regional resource. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.PrivateConnection}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.PrivateConnection content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/privateConnections";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new private connection that can be used for accessing private Clouds.
*
* Create a request for the method "privateConnections.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location to create the new private connection in. Private
* connection is a regional resource. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/us-central1`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.PrivateConnection}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.PrivateConnection content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location to create the new private connection in.
* Private connection is a regional resource. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location to create the new private connection in. Private
connection is a regional resource. Resource names are schemeless URIs that follow the conventions
in https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-central1`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location to create the new private connection in.
* Private connection is a regional resource. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The user-provided identifier of the new private connection. This identifier
* must be unique among private connection resources within the parent and becomes the
* final token in the name URI. The identifier must meet the following requirements: *
* Only contains 1-63 alphanumeric characters and hyphens * Begins with an alphabetical
* character * Ends with a non-hyphen character * Not formatted as a UUID * Complies with
* [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String privateConnectionId;
/** Required. The user-provided identifier of the new private connection. This identifier must be
unique among private connection resources within the parent and becomes the final token in the name
URI. The identifier must meet the following requirements: * Only contains 1-63 alphanumeric
characters and hyphens * Begins with an alphabetical character * Ends with a non-hyphen character *
Not formatted as a UUID * Complies with [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034)
(section 3.5)
*/
public java.lang.String getPrivateConnectionId() {
return privateConnectionId;
}
/**
* Required. The user-provided identifier of the new private connection. This identifier
* must be unique among private connection resources within the parent and becomes the
* final token in the name URI. The identifier must meet the following requirements: *
* Only contains 1-63 alphanumeric characters and hyphens * Begins with an alphabetical
* character * Ends with a non-hyphen character * Not formatted as a UUID * Complies with
* [RFC 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public Create setPrivateConnectionId(java.lang.String privateConnectionId) {
this.privateConnectionId = privateConnectionId;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `PrivateConnection` resource. When a private connection is deleted for a VMware Engine
* network, the connected network becomes inaccessible to that VMware Engine network.
*
* Create a request for the method "privateConnections.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the private connection to be deleted. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1/privateConnections/my-connection`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Deletes a `PrivateConnection` resource. When a private connection is deleted for a VMware
* Engine network, the connected network becomes inaccessible to that VMware Engine network.
*
* Create a request for the method "privateConnections.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the private connection to be deleted. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1/privateConnections/my-connection`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private connection to be deleted. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateConnections/my-connection`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the private connection to be deleted. Resource names are schemeless
URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
example: `projects/my-project/locations/us-central1/privateConnections/my-connection`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the private connection to be deleted. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateConnections/my-connection`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `PrivateConnection` resource by its resource name. The resource contains details of
* the private connection, such as connected network, routing mode and state.
*
* Create a request for the method "privateConnections.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the private connection to retrieve. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1/privateConnections/my-connection`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Retrieves a `PrivateConnection` resource by its resource name. The resource contains details of
* the private connection, such as connected network, routing mode and state.
*
* Create a request for the method "privateConnections.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the private connection to retrieve. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/us-central1/privateConnections/my-connection`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.PrivateConnection.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private connection to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateConnections/my-connection`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the private connection to retrieve. Resource names are schemeless
URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
example: `projects/my-project/locations/us-central1/privateConnections/my-connection`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the private connection to retrieve. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateConnections/my-connection`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists `PrivateConnection` resources in a given project and location.
*
* Create a request for the method "privateConnections.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location to query for private connections. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/privateConnections";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists `PrivateConnection` resources in a given project and location.
*
* Create a request for the method "privateConnections.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location to query for private connections. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListPrivateConnectionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location to query for private connections. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location to query for private connections. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/us-central1`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location to query for private connections. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* private connections, you can exclude the ones named `example-connection` by specifying
* `name != "example-connection"`. To filter on multiple expressions, provide each
* separate expression within parentheses. For example: ``` (name = "example-connection")
* (createTime > "2022-09-22T08:15:10.40Z") ``` By default, each expression is an `AND`
* expression. However, you can include `AND` and `OR` expressions explicitly. For
* example: ``` (name = "example-connection-1") AND (createTime >
* "2021-04-12T08:15:10.40Z") OR (name = "example-connection-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of private connections, you can exclude the ones named
`example-connection` by specifying `name != "example-connection"`. To filter on multiple
expressions, provide each separate expression within parentheses. For example: ``` (name =
"example-connection") (createTime > "2022-09-22T08:15:10.40Z") ``` By default, each expression is
an `AND` expression. However, you can include `AND` and `OR` expressions explicitly. For example:
``` (name = "example-connection-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
"example-connection-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* private connections, you can exclude the ones named `example-connection` by specifying
* `name != "example-connection"`. To filter on multiple expressions, provide each
* separate expression within parentheses. For example: ``` (name = "example-connection")
* (createTime > "2022-09-22T08:15:10.40Z") ``` By default, each expression is an `AND`
* expression. However, you can include `AND` and `OR` expressions explicitly. For
* example: ``` (name = "example-connection-1") AND (createTime >
* "2021-04-12T08:15:10.40Z") OR (name = "example-connection-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of private connections to return in one page. The maximum value is
* coerced to 1000. The default value of this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of private connections to return in one page. The maximum value is coerced to
1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of private connections to return in one page. The maximum value is
* coerced to 1000. The default value of this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListPrivateConnections` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPrivateConnections` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListPrivateConnections` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListPrivateConnections` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListPrivateConnections` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListPrivateConnections` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies a `PrivateConnection` resource. Only `description` and `routing_mode` fields can be
* updated. Only fields specified in `updateMask` are applied.
*
* Create a request for the method "privateConnections.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of the private connection. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/privateConnections/my-connection`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.PrivateConnection}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.PrivateConnection content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Modifies a `PrivateConnection` resource. Only `description` and `routing_mode` fields can be
* updated. Only fields specified in `updateMask` are applied.
*
* Create a request for the method "privateConnections.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of the private connection. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/us-central1/privateConnections/my-connection`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.PrivateConnection}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.PrivateConnection content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of the private connection. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateConnections/my-connection`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of the private connection. Resource names are schemeless URIs that
follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/us-central1/privateConnections/my-connection`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of the private connection. Resource names are schemeless
* URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-central1/privateConnections/my-connection`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `PrivateConnection` resource by the update. The fields specified in the `update_mask`
* are relative to the resource, not the full request. A field will be overwritten if it
* is in the mask. If the user does not provide a mask then all fields will be
* overwritten.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the `PrivateConnection`
resource by the update. The fields specified in the `update_mask` are relative to the resource, not
the full request. A field will be overwritten if it is in the mask. If the user does not provide a
mask then all fields will be overwritten.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* `PrivateConnection` resource by the update. The fields specified in the `update_mask`
* are relative to the resource, not the full request. A field will be overwritten if it
* is in the mask. If the user does not provide a mask then all fields will be
* overwritten.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the PeeringRoutes collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.PeeringRoutes.List request = vmwareengine.peeringRoutes().list(parameters ...)}
*
*
* @return the resource collection
*/
public PeeringRoutes peeringRoutes() {
return new PeeringRoutes();
}
/**
* The "peeringRoutes" collection of methods.
*/
public class PeeringRoutes {
/**
* Lists the private connection routes exchanged over a peering connection.
*
* Create a request for the method "peeringRoutes.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the private connection to retrieve peering routes from. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-west1/privateConnections/my-connection`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/peeringRoutes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
/**
* Lists the private connection routes exchanged over a peering connection.
*
* Create a request for the method "peeringRoutes.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the private connection to retrieve peering routes from. Resource
* names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-west1/privateConnections/my-connection`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListPrivateConnectionPeeringRoutesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the private connection to retrieve peering routes
* from. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-west1/privateConnections/my-connection`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the private connection to retrieve peering routes from. Resource
names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-project/locations
/us-west1/privateConnections/my-connection`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the private connection to retrieve peering routes
* from. Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/us-west1/privateConnections/my-connection`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/privateConnections/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of peering routes to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of
* this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of peering routes to return in one page. The service may return fewer than this
value. The maximum value is coerced to 1000. The default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of peering routes to return in one page. The service may return
* fewer than this value. The maximum value is coerced to 1000. The default value of
* this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListPrivateConnectionPeeringRoutes` call.
* Provide this to retrieve the subsequent page. When paginating, all other parameters
* provided to `ListPrivateConnectionPeeringRoutes` must match the call that provided
* the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListPrivateConnectionPeeringRoutes` call. Provide this to
retrieve the subsequent page. When paginating, all other parameters provided to
`ListPrivateConnectionPeeringRoutes` must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListPrivateConnectionPeeringRoutes` call.
* Provide this to retrieve the subsequent page. When paginating, all other parameters
* provided to `ListPrivateConnectionPeeringRoutes` must match the call that provided
* the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the VmwareEngineNetworks collection.
*
* The typical use is:
*
* {@code VMwareEngine vmwareengine = new VMwareEngine(...);}
* {@code VMwareEngine.VmwareEngineNetworks.List request = vmwareengine.vmwareEngineNetworks().list(parameters ...)}
*
*
* @return the resource collection
*/
public VmwareEngineNetworks vmwareEngineNetworks() {
return new VmwareEngineNetworks();
}
/**
* The "vmwareEngineNetworks" collection of methods.
*/
public class VmwareEngineNetworks {
/**
* Creates a new VMware Engine network that can be used by a private cloud.
*
* Create a request for the method "vmwareEngineNetworks.create".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location to create the new VMware Engine network in. A VMware
* Engine network of type `LEGACY` is a regional resource, and a VMware Engine network of
* type `STANDARD` is a global resource. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/global`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/vmwareEngineNetworks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new VMware Engine network that can be used by a private cloud.
*
* Create a request for the method "vmwareEngineNetworks.create".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location to create the new VMware Engine network in. A VMware
* Engine network of type `LEGACY` is a regional resource, and a VMware Engine network of
* type `STANDARD` is a global resource. Resource names are schemeless URIs that follow the
* conventions in https://cloud.google.com/apis/design/resource_names. For example: `projects
* /my-project/locations/global`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork content) {
super(VMwareEngine.this, "POST", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location to create the new VMware Engine network in.
* A VMware Engine network of type `LEGACY` is a regional resource, and a VMware Engine
* network of type `STANDARD` is a global resource. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location to create the new VMware Engine network in. A VMware
Engine network of type `LEGACY` is a regional resource, and a VMware Engine network of type
`STANDARD` is a global resource. Resource names are schemeless URIs that follow the conventions in
https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
project/locations/global`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location to create the new VMware Engine network in.
* A VMware Engine network of type `LEGACY` is a regional resource, and a VMware Engine
* network of type `STANDARD` is a global resource. Resource names are schemeless URIs
* that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. The user-provided identifier of the new VMware Engine network. This
* identifier must be unique among VMware Engine network resources within the parent and
* becomes the final token in the name URI. The identifier must meet the following
* requirements: * For networks of type LEGACY, adheres to the format: `{region-
* id}-default`. Replace `{region-id}` with the region where you want to create the VMware
* Engine network. For example, "us-central1-default". * Only contains 1-63 alphanumeric
* characters and hyphens * Begins with an alphabetical character * Ends with a non-hyphen
* character * Not formatted as a UUID * Complies with [RFC
* 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
@com.google.api.client.util.Key
private java.lang.String vmwareEngineNetworkId;
/** Required. The user-provided identifier of the new VMware Engine network. This identifier must be
unique among VMware Engine network resources within the parent and becomes the final token in the
name URI. The identifier must meet the following requirements: * For networks of type LEGACY,
adheres to the format: `{region-id}-default`. Replace `{region-id}` with the region where you want
to create the VMware Engine network. For example, "us-central1-default". * Only contains 1-63
alphanumeric characters and hyphens * Begins with an alphabetical character * Ends with a non-
hyphen character * Not formatted as a UUID * Complies with [RFC
1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public java.lang.String getVmwareEngineNetworkId() {
return vmwareEngineNetworkId;
}
/**
* Required. The user-provided identifier of the new VMware Engine network. This
* identifier must be unique among VMware Engine network resources within the parent and
* becomes the final token in the name URI. The identifier must meet the following
* requirements: * For networks of type LEGACY, adheres to the format: `{region-
* id}-default`. Replace `{region-id}` with the region where you want to create the VMware
* Engine network. For example, "us-central1-default". * Only contains 1-63 alphanumeric
* characters and hyphens * Begins with an alphabetical character * Ends with a non-hyphen
* character * Not formatted as a UUID * Complies with [RFC
* 1034](https://datatracker.ietf.org/doc/html/rfc1034) (section 3.5)
*/
public Create setVmwareEngineNetworkId(java.lang.String vmwareEngineNetworkId) {
this.vmwareEngineNetworkId = vmwareEngineNetworkId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a `VmwareEngineNetwork` resource. You can only delete a VMware Engine network after all
* resources that refer to it are deleted. For example, a private cloud, a network peering, and a
* network policy can all refer to the same VMware Engine network.
*
* Create a request for the method "vmwareEngineNetworks.delete".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the VMware Engine network to be deleted. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/vmwareEngineNetworks/my-network`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
/**
* Deletes a `VmwareEngineNetwork` resource. You can only delete a VMware Engine network after all
* resources that refer to it are deleted. For example, a private cloud, a network peering, and a
* network policy can all refer to the same VMware Engine network.
*
* Create a request for the method "vmwareEngineNetworks.delete".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the VMware Engine network to be deleted. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/vmwareEngineNetworks/my-network`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(VMwareEngine.this, "DELETE", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the VMware Engine network to be deleted. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/vmwareEngineNetworks/my-network`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the VMware Engine network to be deleted. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/global/vmwareEngineNetworks/my-network`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the VMware Engine network to be deleted. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/vmwareEngineNetworks/my-network`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. Checksum used to ensure that the user-provided value is up to date before the
* server processes the request. The server compares provided checksum with the current
* checksum of the resource. If the user-provided value is out of date, this request
* returns an `ABORTED` error.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** Optional. Checksum used to ensure that the user-provided value is up to date before the server
processes the request. The server compares provided checksum with the current checksum of the
resource. If the user-provided value is out of date, this request returns an `ABORTED` error.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Optional. Checksum used to ensure that the user-provided value is up to date before the
* server processes the request. The server compares provided checksum with the current
* checksum of the resource. If the user-provided value is out of date, this request
* returns an `ABORTED` error.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves a `VmwareEngineNetwork` resource by its resource name. The resource contains details of
* the VMware Engine network, such as its VMware Engine network type, peered networks in a service
* project, and state (for example, `CREATING`, `ACTIVE`, `DELETING`).
*
* Create a request for the method "vmwareEngineNetworks.get".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the VMware Engine network to retrieve. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/global/vmwareEngineNetworks/my-network`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
/**
* Retrieves a `VmwareEngineNetwork` resource by its resource name. The resource contains details
* of the VMware Engine network, such as its VMware Engine network type, peered networks in a
* service project, and state (for example, `CREATING`, `ACTIVE`, `DELETING`).
*
* Create a request for the method "vmwareEngineNetworks.get".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the VMware Engine network to retrieve. Resource names are schemeless
* URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
* For example: `projects/my-project/locations/global/vmwareEngineNetworks/my-network`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the VMware Engine network to retrieve. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/vmwareEngineNetworks/my-network`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the VMware Engine network to retrieve. Resource names are schemeless
URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names. For
example: `projects/my-project/locations/global/vmwareEngineNetworks/my-network`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the VMware Engine network to retrieve. Resource names
* are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/vmwareEngineNetworks/my-network`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists `VmwareEngineNetwork` resources in a given project and location.
*
* Create a request for the method "vmwareEngineNetworks.list".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name of the location to query for VMware Engine networks. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+parent}/vmwareEngineNetworks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists `VmwareEngineNetwork` resources in a given project and location.
*
* Create a request for the method "vmwareEngineNetworks.list".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name of the location to query for VMware Engine networks. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(VMwareEngine.this, "GET", REST_PATH, null, com.google.api.services.vmwareengine.v1.model.ListVmwareEngineNetworksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the location to query for VMware Engine networks.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name of the location to query for VMware Engine networks. Resource names are
schemeless URIs that follow the conventions in https://cloud.google.com/apis/design/resource_names.
For example: `projects/my-project/locations/global`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name of the location to query for VMware Engine networks.
* Resource names are schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* network peerings, you can exclude the ones named `example-network` by specifying `name
* != "example-network"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-network") (createTime
* > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name
* = "example-network-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-network-2") ```
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter expression that matches resources returned in the response. The expression must specify
the field name, a comparison operator, and the value that you want to use for filtering. The value
must be a string, a number, or a boolean. The comparison operator must be `=`, `!=`, `>`, or `<`.
For example, if you are filtering a list of network peerings, you can exclude the ones named
`example-network` by specifying `name != "example-network"`. To filter on multiple expressions,
provide each separate expression within parentheses. For example: ``` (name = "example-network")
(createTime > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name = "example-
network-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name = "example-network-2") ```
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter expression that matches resources returned in the response. The expression
* must specify the field name, a comparison operator, and the value that you want to use
* for filtering. The value must be a string, a number, or a boolean. The comparison
* operator must be `=`, `!=`, `>`, or `<`. For example, if you are filtering a list of
* network peerings, you can exclude the ones named `example-network` by specifying `name
* != "example-network"`. To filter on multiple expressions, provide each separate
* expression within parentheses. For example: ``` (name = "example-network") (createTime
* > "2021-04-12T08:15:10.40Z") ``` By default, each expression is an `AND` expression.
* However, you can include `AND` and `OR` expressions explicitly. For example: ``` (name
* = "example-network-1") AND (createTime > "2021-04-12T08:15:10.40Z") OR (name =
* "example-network-2") ```
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Sorts list results by a certain order. By default, returned results are ordered by `name` in
ascending order. You can also sort results in descending order based on the `name` value using
`orderBy="name desc"`. Currently, only ordering by `name` is supported.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/**
* Sorts list results by a certain order. By default, returned results are ordered by
* `name` in ascending order. You can also sort results in descending order based on the
* `name` value using `orderBy="name desc"`. Currently, only ordering by `name` is
* supported.
*/
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The maximum number of results to return in one page. The maximum value is coerced to
* 1000. The default value of this field is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return in one page. The maximum value is coerced to 1000. The
default value of this field is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return in one page. The maximum value is coerced to
* 1000. The default value of this field is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListVmwareEngineNetworks` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListVmwareEngineNetworks` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListVmwareEngineNetworks` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListVmwareEngineNetworks`
must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListVmwareEngineNetworks` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListVmwareEngineNetworks` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies a VMware Engine network resource. Only the following fields can be updated:
* `description`. Only fields specified in `updateMask` are applied.
*
* Create a request for the method "vmwareEngineNetworks.patch".
*
* This request holds the parameters needed by the vmwareengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The resource name of the VMware Engine network. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global/vmwareEngineNetworks/my-network`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends VMwareEngineRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
/**
* Modifies a VMware Engine network resource. Only the following fields can be updated:
* `description`. Only fields specified in `updateMask` are applied.
*
* Create a request for the method "vmwareEngineNetworks.patch".
*
* This request holds the parameters needed by the the vmwareengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The resource name of the VMware Engine network. Resource names are schemeless URIs that
* follow the conventions in https://cloud.google.com/apis/design/resource_names. For
* example: `projects/my-project/locations/global/vmwareEngineNetworks/my-network`
* @param content the {@link com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.vmwareengine.v1.model.VmwareEngineNetwork content) {
super(VMwareEngine.this, "PATCH", REST_PATH, content, com.google.api.services.vmwareengine.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The resource name of the VMware Engine network. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/vmwareEngineNetworks/my-network`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The resource name of the VMware Engine network. Resource names are schemeless URIs
that follow the conventions in https://cloud.google.com/apis/design/resource_names. For example:
`projects/my-project/locations/global/vmwareEngineNetworks/my-network`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of the VMware Engine network. Resource names are
* schemeless URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/vmwareEngineNetworks/my-network`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/vmwareEngineNetworks/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. A request ID to identify requests. Specify a unique request ID so that if you must retry
your request, the server will know to ignore the request if it has already been completed. The
server guarantees that a request doesn't result in creation of duplicate commitments for at least
60 minutes. For example, consider a situation where you make an initial request and the request
times out. If you make the request again with the same request ID, the server can check if original
operation with the same request ID was received, and if so, will ignore the second request. This
prevents clients from accidentally creating duplicate commitments. The request ID must be a valid
UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. A request ID to identify requests. Specify a unique request ID so that if you
* must retry your request, the server will know to ignore the request if it has already
* been completed. The server guarantees that a request doesn't result in creation of
* duplicate commitments for at least 60 minutes. For example, consider a situation where
* you make an initial request and the request times out. If you make the request again
* with the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the VMware
* Engine network resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten. Only
* the following fields can be updated: `description`.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the VMware Engine network
resource by the update. The fields specified in the `update_mask` are relative to the resource, not
the full request. A field will be overwritten if it is in the mask. If the user does not provide a
mask then all fields will be overwritten. Only the following fields can be updated: `description`.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the VMware
* Engine network resource by the update. The fields specified in the `update_mask` are
* relative to the resource, not the full request. A field will be overwritten if it is in
* the mask. If the user does not provide a mask then all fields will be overwritten. Only
* the following fields can be updated: `description`.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link VMwareEngine}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link VMwareEngine}. */
@Override
public VMwareEngine build() {
return new VMwareEngine(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link VMwareEngineRequestInitializer}.
*
* @since 1.12
*/
public Builder setVMwareEngineRequestInitializer(
VMwareEngineRequestInitializer vmwareengineRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(vmwareengineRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}