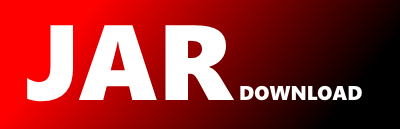
com.google.api.services.vmwareengine.v1.model.NetworkPeering Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.vmwareengine.v1.model;
/**
* Details of a network peering.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the VMware Engine API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class NetworkPeering extends com.google.api.client.json.GenericJson {
/**
* Output only. Creation time of this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Optional. User-provided description for this network peering.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Optional. True if full mesh connectivity is created and managed automatically between peered
* networks; false otherwise. Currently this field is always true because Google Compute Engine
* automatically creates and manages subnetwork routes between two VPC networks when peering state
* is 'ACTIVE'.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean exchangeSubnetRoutes;
/**
* Optional. True if custom routes are exported to the peered network; false otherwise. The
* default value is true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean exportCustomRoutes;
/**
* Optional. True if all subnet routes with a public IP address range are exported; false
* otherwise. The default value is true. IPv4 special-use ranges
* (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always exported to peers and are not
* controlled by this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean exportCustomRoutesWithPublicIp;
/**
* Optional. True if custom routes are imported from the peered network; false otherwise. The
* default value is true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean importCustomRoutes;
/**
* Optional. True if all subnet routes with public IP address range are imported; false otherwise.
* The default value is true. IPv4 special-use ranges
* (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always imported to peers and are not
* controlled by this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean importCustomRoutesWithPublicIp;
/**
* Output only. The resource name of the network peering. NetworkPeering is a global resource and
* location can only be global. Resource names are scheme-less URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Optional. Maximum transmission unit (MTU) in bytes. The default value is `1500`. If a value of
* `0` is provided for this field, VMware Engine uses the default value instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer peerMtu;
/**
* Required. The relative resource name of the network to peer with a standard VMware Engine
* network. The provided network can be a consumer VPC network or another standard VMware Engine
* network. If the `peer_network_type` is VMWARE_ENGINE_NETWORK, specify the name in the form:
* `projects/{project}/locations/global/vmwareEngineNetworks/{vmware_engine_network_id}`.
* Otherwise specify the name in the form: `projects/{project}/global/networks/{network_id}`,
* where `{project}` can either be a project number or a project ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String peerNetwork;
/**
* Required. The type of the network to peer with the VMware Engine network.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String peerNetworkType;
/**
* Output only. State of the network peering. This field has a value of 'ACTIVE' when there's a
* matching configuration in the peer network. New values may be added to this enum when
* appropriate.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Output only. Output Only. Details about the current state of the network peering.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String stateDetails;
/**
* Output only. System-generated unique identifier for the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uid;
/**
* Output only. Last update time of this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Required. The relative resource name of the VMware Engine network. Specify the name in the
* following form:
* `projects/{project}/locations/{location}/vmwareEngineNetworks/{vmware_engine_network_id}` where
* `{project}` can either be a project number or a project ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String vmwareEngineNetwork;
/**
* Output only. Creation time of this resource.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. Creation time of this resource.
* @param createTime createTime or {@code null} for none
*/
public NetworkPeering setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Optional. User-provided description for this network peering.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Optional. User-provided description for this network peering.
* @param description description or {@code null} for none
*/
public NetworkPeering setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Optional. True if full mesh connectivity is created and managed automatically between peered
* networks; false otherwise. Currently this field is always true because Google Compute Engine
* automatically creates and manages subnetwork routes between two VPC networks when peering state
* is 'ACTIVE'.
* @return value or {@code null} for none
*/
public java.lang.Boolean getExchangeSubnetRoutes() {
return exchangeSubnetRoutes;
}
/**
* Optional. True if full mesh connectivity is created and managed automatically between peered
* networks; false otherwise. Currently this field is always true because Google Compute Engine
* automatically creates and manages subnetwork routes between two VPC networks when peering state
* is 'ACTIVE'.
* @param exchangeSubnetRoutes exchangeSubnetRoutes or {@code null} for none
*/
public NetworkPeering setExchangeSubnetRoutes(java.lang.Boolean exchangeSubnetRoutes) {
this.exchangeSubnetRoutes = exchangeSubnetRoutes;
return this;
}
/**
* Optional. True if custom routes are exported to the peered network; false otherwise. The
* default value is true.
* @return value or {@code null} for none
*/
public java.lang.Boolean getExportCustomRoutes() {
return exportCustomRoutes;
}
/**
* Optional. True if custom routes are exported to the peered network; false otherwise. The
* default value is true.
* @param exportCustomRoutes exportCustomRoutes or {@code null} for none
*/
public NetworkPeering setExportCustomRoutes(java.lang.Boolean exportCustomRoutes) {
this.exportCustomRoutes = exportCustomRoutes;
return this;
}
/**
* Optional. True if all subnet routes with a public IP address range are exported; false
* otherwise. The default value is true. IPv4 special-use ranges
* (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always exported to peers and are not
* controlled by this field.
* @return value or {@code null} for none
*/
public java.lang.Boolean getExportCustomRoutesWithPublicIp() {
return exportCustomRoutesWithPublicIp;
}
/**
* Optional. True if all subnet routes with a public IP address range are exported; false
* otherwise. The default value is true. IPv4 special-use ranges
* (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always exported to peers and are not
* controlled by this field.
* @param exportCustomRoutesWithPublicIp exportCustomRoutesWithPublicIp or {@code null} for none
*/
public NetworkPeering setExportCustomRoutesWithPublicIp(java.lang.Boolean exportCustomRoutesWithPublicIp) {
this.exportCustomRoutesWithPublicIp = exportCustomRoutesWithPublicIp;
return this;
}
/**
* Optional. True if custom routes are imported from the peered network; false otherwise. The
* default value is true.
* @return value or {@code null} for none
*/
public java.lang.Boolean getImportCustomRoutes() {
return importCustomRoutes;
}
/**
* Optional. True if custom routes are imported from the peered network; false otherwise. The
* default value is true.
* @param importCustomRoutes importCustomRoutes or {@code null} for none
*/
public NetworkPeering setImportCustomRoutes(java.lang.Boolean importCustomRoutes) {
this.importCustomRoutes = importCustomRoutes;
return this;
}
/**
* Optional. True if all subnet routes with public IP address range are imported; false otherwise.
* The default value is true. IPv4 special-use ranges
* (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always imported to peers and are not
* controlled by this field.
* @return value or {@code null} for none
*/
public java.lang.Boolean getImportCustomRoutesWithPublicIp() {
return importCustomRoutesWithPublicIp;
}
/**
* Optional. True if all subnet routes with public IP address range are imported; false otherwise.
* The default value is true. IPv4 special-use ranges
* (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always imported to peers and are not
* controlled by this field.
* @param importCustomRoutesWithPublicIp importCustomRoutesWithPublicIp or {@code null} for none
*/
public NetworkPeering setImportCustomRoutesWithPublicIp(java.lang.Boolean importCustomRoutesWithPublicIp) {
this.importCustomRoutesWithPublicIp = importCustomRoutesWithPublicIp;
return this;
}
/**
* Output only. The resource name of the network peering. NetworkPeering is a global resource and
* location can only be global. Resource names are scheme-less URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of the network peering. NetworkPeering is a global resource and
* location can only be global. Resource names are scheme-less URIs that follow the conventions in
* https://cloud.google.com/apis/design/resource_names. For example: `projects/my-
* project/locations/global/networkPeerings/my-peering`
* @param name name or {@code null} for none
*/
public NetworkPeering setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Optional. Maximum transmission unit (MTU) in bytes. The default value is `1500`. If a value of
* `0` is provided for this field, VMware Engine uses the default value instead.
* @return value or {@code null} for none
*/
public java.lang.Integer getPeerMtu() {
return peerMtu;
}
/**
* Optional. Maximum transmission unit (MTU) in bytes. The default value is `1500`. If a value of
* `0` is provided for this field, VMware Engine uses the default value instead.
* @param peerMtu peerMtu or {@code null} for none
*/
public NetworkPeering setPeerMtu(java.lang.Integer peerMtu) {
this.peerMtu = peerMtu;
return this;
}
/**
* Required. The relative resource name of the network to peer with a standard VMware Engine
* network. The provided network can be a consumer VPC network or another standard VMware Engine
* network. If the `peer_network_type` is VMWARE_ENGINE_NETWORK, specify the name in the form:
* `projects/{project}/locations/global/vmwareEngineNetworks/{vmware_engine_network_id}`.
* Otherwise specify the name in the form: `projects/{project}/global/networks/{network_id}`,
* where `{project}` can either be a project number or a project ID.
* @return value or {@code null} for none
*/
public java.lang.String getPeerNetwork() {
return peerNetwork;
}
/**
* Required. The relative resource name of the network to peer with a standard VMware Engine
* network. The provided network can be a consumer VPC network or another standard VMware Engine
* network. If the `peer_network_type` is VMWARE_ENGINE_NETWORK, specify the name in the form:
* `projects/{project}/locations/global/vmwareEngineNetworks/{vmware_engine_network_id}`.
* Otherwise specify the name in the form: `projects/{project}/global/networks/{network_id}`,
* where `{project}` can either be a project number or a project ID.
* @param peerNetwork peerNetwork or {@code null} for none
*/
public NetworkPeering setPeerNetwork(java.lang.String peerNetwork) {
this.peerNetwork = peerNetwork;
return this;
}
/**
* Required. The type of the network to peer with the VMware Engine network.
* @return value or {@code null} for none
*/
public java.lang.String getPeerNetworkType() {
return peerNetworkType;
}
/**
* Required. The type of the network to peer with the VMware Engine network.
* @param peerNetworkType peerNetworkType or {@code null} for none
*/
public NetworkPeering setPeerNetworkType(java.lang.String peerNetworkType) {
this.peerNetworkType = peerNetworkType;
return this;
}
/**
* Output only. State of the network peering. This field has a value of 'ACTIVE' when there's a
* matching configuration in the peer network. New values may be added to this enum when
* appropriate.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. State of the network peering. This field has a value of 'ACTIVE' when there's a
* matching configuration in the peer network. New values may be added to this enum when
* appropriate.
* @param state state or {@code null} for none
*/
public NetworkPeering setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* Output only. Output Only. Details about the current state of the network peering.
* @return value or {@code null} for none
*/
public java.lang.String getStateDetails() {
return stateDetails;
}
/**
* Output only. Output Only. Details about the current state of the network peering.
* @param stateDetails stateDetails or {@code null} for none
*/
public NetworkPeering setStateDetails(java.lang.String stateDetails) {
this.stateDetails = stateDetails;
return this;
}
/**
* Output only. System-generated unique identifier for the resource.
* @return value or {@code null} for none
*/
public java.lang.String getUid() {
return uid;
}
/**
* Output only. System-generated unique identifier for the resource.
* @param uid uid or {@code null} for none
*/
public NetworkPeering setUid(java.lang.String uid) {
this.uid = uid;
return this;
}
/**
* Output only. Last update time of this resource.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. Last update time of this resource.
* @param updateTime updateTime or {@code null} for none
*/
public NetworkPeering setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* Required. The relative resource name of the VMware Engine network. Specify the name in the
* following form:
* `projects/{project}/locations/{location}/vmwareEngineNetworks/{vmware_engine_network_id}` where
* `{project}` can either be a project number or a project ID.
* @return value or {@code null} for none
*/
public java.lang.String getVmwareEngineNetwork() {
return vmwareEngineNetwork;
}
/**
* Required. The relative resource name of the VMware Engine network. Specify the name in the
* following form:
* `projects/{project}/locations/{location}/vmwareEngineNetworks/{vmware_engine_network_id}` where
* `{project}` can either be a project number or a project ID.
* @param vmwareEngineNetwork vmwareEngineNetwork or {@code null} for none
*/
public NetworkPeering setVmwareEngineNetwork(java.lang.String vmwareEngineNetwork) {
this.vmwareEngineNetwork = vmwareEngineNetwork;
return this;
}
@Override
public NetworkPeering set(String fieldName, Object value) {
return (NetworkPeering) super.set(fieldName, value);
}
@Override
public NetworkPeering clone() {
return (NetworkPeering) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy