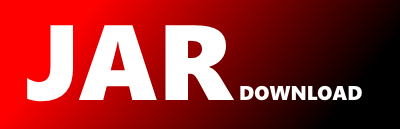
target.apidocs.com.google.api.services.youtube.model.LiveBroadcastContentDetails.html Maven / Gradle / Ivy
LiveBroadcastContentDetails (YouTube Data API v3 v3-rev20210410-1.31.0)
com.google.api.services.youtube.model
Class LiveBroadcastContentDetails
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.youtube.model.LiveBroadcastContentDetails
-
public final class LiveBroadcastContentDetails
extends GenericJson
Detailed settings of a broadcast.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the YouTube Data API v3. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
LiveBroadcastContentDetails()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
LiveBroadcastContentDetails
clone()
byte[]
decodeMesh()
The mesh for projecting the video if projection is mesh.
LiveBroadcastContentDetails
encodeMesh(byte[] mesh)
The mesh for projecting the video if projection is mesh.
String
getBoundStreamId()
This value uniquely identifies the live stream bound to the broadcast.
DateTime
getBoundStreamLastUpdateTimeMs()
The date and time that the live stream referenced by boundStreamId was last updated.
String
getClosedCaptionsType()
Boolean
getEnableAutoStart()
This setting indicates whether auto start is enabled for this broadcast.
Boolean
getEnableAutoStop()
This setting indicates whether auto stop is enabled for this broadcast.
Boolean
getEnableClosedCaptions()
This setting indicates whether HTTP POST closed captioning is enabled for this broadcast.
Boolean
getEnableContentEncryption()
This setting indicates whether YouTube should enable content encryption for the broadcast.
Boolean
getEnableDvr()
This setting determines whether viewers can access DVR controls while watching the video.
Boolean
getEnableEmbed()
This setting indicates whether the broadcast video can be played in an embedded player.
Boolean
getEnableLowLatency()
Indicates whether this broadcast has low latency enabled.
String
getLatencyPreference()
If both this and enable_low_latency are set, they must match.
String
getMesh()
The mesh for projecting the video if projection is mesh.
MonitorStreamInfo
getMonitorStream()
The monitorStream object contains information about the monitor stream, which the broadcaster
can use to review the event content before the broadcast stream is shown publicly.
String
getProjection()
The projection format of this broadcast.
Boolean
getRecordFromStart()
Automatically start recording after the event goes live.
Boolean
getStartWithSlate()
This setting indicates whether the broadcast should automatically begin with an in-stream slate
when you update the broadcast's status to live.
String
getStereoLayout()
The 3D stereo layout of this broadcast.
LiveBroadcastContentDetails
set(String fieldName,
Object value)
LiveBroadcastContentDetails
setBoundStreamId(String boundStreamId)
This value uniquely identifies the live stream bound to the broadcast.
LiveBroadcastContentDetails
setBoundStreamLastUpdateTimeMs(DateTime boundStreamLastUpdateTimeMs)
The date and time that the live stream referenced by boundStreamId was last updated.
LiveBroadcastContentDetails
setClosedCaptionsType(String closedCaptionsType)
LiveBroadcastContentDetails
setEnableAutoStart(Boolean enableAutoStart)
This setting indicates whether auto start is enabled for this broadcast.
LiveBroadcastContentDetails
setEnableAutoStop(Boolean enableAutoStop)
This setting indicates whether auto stop is enabled for this broadcast.
LiveBroadcastContentDetails
setEnableClosedCaptions(Boolean enableClosedCaptions)
This setting indicates whether HTTP POST closed captioning is enabled for this broadcast.
LiveBroadcastContentDetails
setEnableContentEncryption(Boolean enableContentEncryption)
This setting indicates whether YouTube should enable content encryption for the broadcast.
LiveBroadcastContentDetails
setEnableDvr(Boolean enableDvr)
This setting determines whether viewers can access DVR controls while watching the video.
LiveBroadcastContentDetails
setEnableEmbed(Boolean enableEmbed)
This setting indicates whether the broadcast video can be played in an embedded player.
LiveBroadcastContentDetails
setEnableLowLatency(Boolean enableLowLatency)
Indicates whether this broadcast has low latency enabled.
LiveBroadcastContentDetails
setLatencyPreference(String latencyPreference)
If both this and enable_low_latency are set, they must match.
LiveBroadcastContentDetails
setMesh(String mesh)
The mesh for projecting the video if projection is mesh.
LiveBroadcastContentDetails
setMonitorStream(MonitorStreamInfo monitorStream)
The monitorStream object contains information about the monitor stream, which the broadcaster
can use to review the event content before the broadcast stream is shown publicly.
LiveBroadcastContentDetails
setProjection(String projection)
The projection format of this broadcast.
LiveBroadcastContentDetails
setRecordFromStart(Boolean recordFromStart)
Automatically start recording after the event goes live.
LiveBroadcastContentDetails
setStartWithSlate(Boolean startWithSlate)
This setting indicates whether the broadcast should automatically begin with an in-stream slate
when you update the broadcast's status to live.
LiveBroadcastContentDetails
setStereoLayout(String stereoLayout)
The 3D stereo layout of this broadcast.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getBoundStreamId
public String getBoundStreamId()
This value uniquely identifies the live stream bound to the broadcast.
- Returns:
- value or
null
for none
-
setBoundStreamId
public LiveBroadcastContentDetails setBoundStreamId(String boundStreamId)
This value uniquely identifies the live stream bound to the broadcast.
- Parameters:
boundStreamId
- boundStreamId or null
for none
-
getBoundStreamLastUpdateTimeMs
public DateTime getBoundStreamLastUpdateTimeMs()
The date and time that the live stream referenced by boundStreamId was last updated.
- Returns:
- value or
null
for none
-
setBoundStreamLastUpdateTimeMs
public LiveBroadcastContentDetails setBoundStreamLastUpdateTimeMs(DateTime boundStreamLastUpdateTimeMs)
The date and time that the live stream referenced by boundStreamId was last updated.
- Parameters:
boundStreamLastUpdateTimeMs
- boundStreamLastUpdateTimeMs or null
for none
-
getClosedCaptionsType
public String getClosedCaptionsType()
- Returns:
- value or
null
for none
-
setClosedCaptionsType
public LiveBroadcastContentDetails setClosedCaptionsType(String closedCaptionsType)
- Parameters:
closedCaptionsType
- closedCaptionsType or null
for none
-
getEnableAutoStart
public Boolean getEnableAutoStart()
This setting indicates whether auto start is enabled for this broadcast. The default value for
this property is false. This setting can only be used by Events.
- Returns:
- value or
null
for none
-
setEnableAutoStart
public LiveBroadcastContentDetails setEnableAutoStart(Boolean enableAutoStart)
This setting indicates whether auto start is enabled for this broadcast. The default value for
this property is false. This setting can only be used by Events.
- Parameters:
enableAutoStart
- enableAutoStart or null
for none
-
getEnableAutoStop
public Boolean getEnableAutoStop()
This setting indicates whether auto stop is enabled for this broadcast. The default value for
this property is false. This setting can only be used by Events.
- Returns:
- value or
null
for none
-
setEnableAutoStop
public LiveBroadcastContentDetails setEnableAutoStop(Boolean enableAutoStop)
This setting indicates whether auto stop is enabled for this broadcast. The default value for
this property is false. This setting can only be used by Events.
- Parameters:
enableAutoStop
- enableAutoStop or null
for none
-
getEnableClosedCaptions
public Boolean getEnableClosedCaptions()
This setting indicates whether HTTP POST closed captioning is enabled for this broadcast. The
ingestion URL of the closed captions is returned through the liveStreams API. This is mutually
exclusive with using the closed_captions_type property, and is equivalent to setting
closed_captions_type to CLOSED_CAPTIONS_HTTP_POST.
- Returns:
- value or
null
for none
-
setEnableClosedCaptions
public LiveBroadcastContentDetails setEnableClosedCaptions(Boolean enableClosedCaptions)
This setting indicates whether HTTP POST closed captioning is enabled for this broadcast. The
ingestion URL of the closed captions is returned through the liveStreams API. This is mutually
exclusive with using the closed_captions_type property, and is equivalent to setting
closed_captions_type to CLOSED_CAPTIONS_HTTP_POST.
- Parameters:
enableClosedCaptions
- enableClosedCaptions or null
for none
-
getEnableContentEncryption
public Boolean getEnableContentEncryption()
This setting indicates whether YouTube should enable content encryption for the broadcast.
- Returns:
- value or
null
for none
-
setEnableContentEncryption
public LiveBroadcastContentDetails setEnableContentEncryption(Boolean enableContentEncryption)
This setting indicates whether YouTube should enable content encryption for the broadcast.
- Parameters:
enableContentEncryption
- enableContentEncryption or null
for none
-
getEnableDvr
public Boolean getEnableDvr()
This setting determines whether viewers can access DVR controls while watching the video. DVR
controls enable the viewer to control the video playback experience by pausing, rewinding, or
fast forwarding content. The default value for this property is true. *Important:* You must set
the value to true and also set the enableArchive property's value to true if you want to make
playback available immediately after the broadcast ends.
- Returns:
- value or
null
for none
-
setEnableDvr
public LiveBroadcastContentDetails setEnableDvr(Boolean enableDvr)
This setting determines whether viewers can access DVR controls while watching the video. DVR
controls enable the viewer to control the video playback experience by pausing, rewinding, or
fast forwarding content. The default value for this property is true. *Important:* You must set
the value to true and also set the enableArchive property's value to true if you want to make
playback available immediately after the broadcast ends.
- Parameters:
enableDvr
- enableDvr or null
for none
-
getEnableEmbed
public Boolean getEnableEmbed()
This setting indicates whether the broadcast video can be played in an embedded player. If you
choose to archive the video (using the enableArchive property), this setting will also apply to
the archived video.
- Returns:
- value or
null
for none
-
setEnableEmbed
public LiveBroadcastContentDetails setEnableEmbed(Boolean enableEmbed)
This setting indicates whether the broadcast video can be played in an embedded player. If you
choose to archive the video (using the enableArchive property), this setting will also apply to
the archived video.
- Parameters:
enableEmbed
- enableEmbed or null
for none
-
getEnableLowLatency
public Boolean getEnableLowLatency()
Indicates whether this broadcast has low latency enabled.
- Returns:
- value or
null
for none
-
setEnableLowLatency
public LiveBroadcastContentDetails setEnableLowLatency(Boolean enableLowLatency)
Indicates whether this broadcast has low latency enabled.
- Parameters:
enableLowLatency
- enableLowLatency or null
for none
-
getLatencyPreference
public String getLatencyPreference()
If both this and enable_low_latency are set, they must match. LATENCY_NORMAL should match
enable_low_latency=false LATENCY_LOW should match enable_low_latency=true LATENCY_ULTRA_LOW
should have enable_low_latency omitted.
- Returns:
- value or
null
for none
-
setLatencyPreference
public LiveBroadcastContentDetails setLatencyPreference(String latencyPreference)
If both this and enable_low_latency are set, they must match. LATENCY_NORMAL should match
enable_low_latency=false LATENCY_LOW should match enable_low_latency=true LATENCY_ULTRA_LOW
should have enable_low_latency omitted.
- Parameters:
latencyPreference
- latencyPreference or null
for none
-
getMesh
public String getMesh()
The mesh for projecting the video if projection is mesh. The mesh value must be a UTF-8 string
containing the base-64 encoding of 3D mesh data that follows the Spherical Video V2 RFC
specification for an mshp box, excluding the box size and type but including the following four
reserved zero bytes for the version and flags.
- Returns:
- value or
null
for none
- See Also:
decodeMesh()
-
decodeMesh
public byte[] decodeMesh()
The mesh for projecting the video if projection is mesh. The mesh value must be a UTF-8 string
containing the base-64 encoding of 3D mesh data that follows the Spherical Video V2 RFC
specification for an mshp box, excluding the box size and type but including the following four
reserved zero bytes for the version and flags.
- Returns:
- Base64 decoded value or
null
for none
- Since:
- 1.14
- See Also:
getMesh()
-
setMesh
public LiveBroadcastContentDetails setMesh(String mesh)
The mesh for projecting the video if projection is mesh. The mesh value must be a UTF-8 string
containing the base-64 encoding of 3D mesh data that follows the Spherical Video V2 RFC
specification for an mshp box, excluding the box size and type but including the following four
reserved zero bytes for the version and flags.
- Parameters:
mesh
- mesh or null
for none
- See Also:
#encodeMesh()
-
encodeMesh
public LiveBroadcastContentDetails encodeMesh(byte[] mesh)
The mesh for projecting the video if projection is mesh. The mesh value must be a UTF-8 string
containing the base-64 encoding of 3D mesh data that follows the Spherical Video V2 RFC
specification for an mshp box, excluding the box size and type but including the following four
reserved zero bytes for the version and flags.
- Since:
- 1.14
- See Also:
The value is encoded Base64 or {@code null} for none.
-
getMonitorStream
public MonitorStreamInfo getMonitorStream()
The monitorStream object contains information about the monitor stream, which the broadcaster
can use to review the event content before the broadcast stream is shown publicly.
- Returns:
- value or
null
for none
-
setMonitorStream
public LiveBroadcastContentDetails setMonitorStream(MonitorStreamInfo monitorStream)
The monitorStream object contains information about the monitor stream, which the broadcaster
can use to review the event content before the broadcast stream is shown publicly.
- Parameters:
monitorStream
- monitorStream or null
for none
-
getProjection
public String getProjection()
The projection format of this broadcast. This defaults to rectangular.
- Returns:
- value or
null
for none
-
setProjection
public LiveBroadcastContentDetails setProjection(String projection)
The projection format of this broadcast. This defaults to rectangular.
- Parameters:
projection
- projection or null
for none
-
getRecordFromStart
public Boolean getRecordFromStart()
Automatically start recording after the event goes live. The default value for this property is
true. *Important:* You must also set the enableDvr property's value to true if you want the
playback to be available immediately after the broadcast ends. If you set this property's value
to true but do not also set the enableDvr property to true, there may be a delay of around one
day before the archived video will be available for playback.
- Returns:
- value or
null
for none
-
setRecordFromStart
public LiveBroadcastContentDetails setRecordFromStart(Boolean recordFromStart)
Automatically start recording after the event goes live. The default value for this property is
true. *Important:* You must also set the enableDvr property's value to true if you want the
playback to be available immediately after the broadcast ends. If you set this property's value
to true but do not also set the enableDvr property to true, there may be a delay of around one
day before the archived video will be available for playback.
- Parameters:
recordFromStart
- recordFromStart or null
for none
-
getStartWithSlate
public Boolean getStartWithSlate()
This setting indicates whether the broadcast should automatically begin with an in-stream slate
when you update the broadcast's status to live. After updating the status, you then need to
send a liveCuepoints.insert request that sets the cuepoint's eventState to end to remove the
in-stream slate and make your broadcast stream visible to viewers.
- Returns:
- value or
null
for none
-
setStartWithSlate
public LiveBroadcastContentDetails setStartWithSlate(Boolean startWithSlate)
This setting indicates whether the broadcast should automatically begin with an in-stream slate
when you update the broadcast's status to live. After updating the status, you then need to
send a liveCuepoints.insert request that sets the cuepoint's eventState to end to remove the
in-stream slate and make your broadcast stream visible to viewers.
- Parameters:
startWithSlate
- startWithSlate or null
for none
-
getStereoLayout
public String getStereoLayout()
The 3D stereo layout of this broadcast. This defaults to mono.
- Returns:
- value or
null
for none
-
setStereoLayout
public LiveBroadcastContentDetails setStereoLayout(String stereoLayout)
The 3D stereo layout of this broadcast. This defaults to mono.
- Parameters:
stereoLayout
- stereoLayout or null
for none
-
set
public LiveBroadcastContentDetails set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public LiveBroadcastContentDetails clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2021 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy