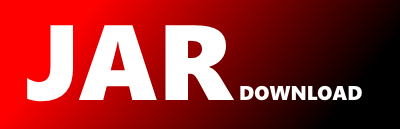
target.apidocs.com.google.api.services.youtube.model.SuperChatEventSnippet.html Maven / Gradle / Ivy
SuperChatEventSnippet (YouTube Data API v3 v3-rev20210410-1.31.0)
com.google.api.services.youtube.model
Class SuperChatEventSnippet
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.youtube.model.SuperChatEventSnippet
-
public final class SuperChatEventSnippet
extends GenericJson
Model definition for SuperChatEventSnippet.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the YouTube Data API v3. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
SuperChatEventSnippet()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
SuperChatEventSnippet
clone()
BigInteger
getAmountMicros()
The purchase amount, in micros of the purchase currency.
String
getChannelId()
Channel id where the event occurred.
String
getCommentText()
The text contents of the comment left by the user.
DateTime
getCreatedAt()
The date and time when the event occurred.
String
getCurrency()
The currency in which the purchase was made.
String
getDisplayString()
A rendered string that displays the purchase amount and currency (e.g., "$1.00").
Boolean
getIsSuperStickerEvent()
True if this event is a Super Sticker event.
Long
getMessageType()
The tier for the paid message, which is based on the amount of money spent to purchase the
message.
SuperStickerMetadata
getSuperStickerMetadata()
If this event is a Super Sticker event, this field will contain metadata about the Super
Sticker.
ChannelProfileDetails
getSupporterDetails()
Details about the supporter.
SuperChatEventSnippet
set(String fieldName,
Object value)
SuperChatEventSnippet
setAmountMicros(BigInteger amountMicros)
The purchase amount, in micros of the purchase currency.
SuperChatEventSnippet
setChannelId(String channelId)
Channel id where the event occurred.
SuperChatEventSnippet
setCommentText(String commentText)
The text contents of the comment left by the user.
SuperChatEventSnippet
setCreatedAt(DateTime createdAt)
The date and time when the event occurred.
SuperChatEventSnippet
setCurrency(String currency)
The currency in which the purchase was made.
SuperChatEventSnippet
setDisplayString(String displayString)
A rendered string that displays the purchase amount and currency (e.g., "$1.00").
SuperChatEventSnippet
setIsSuperStickerEvent(Boolean isSuperStickerEvent)
True if this event is a Super Sticker event.
SuperChatEventSnippet
setMessageType(Long messageType)
The tier for the paid message, which is based on the amount of money spent to purchase the
message.
SuperChatEventSnippet
setSuperStickerMetadata(SuperStickerMetadata superStickerMetadata)
If this event is a Super Sticker event, this field will contain metadata about the Super
Sticker.
SuperChatEventSnippet
setSupporterDetails(ChannelProfileDetails supporterDetails)
Details about the supporter.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAmountMicros
public BigInteger getAmountMicros()
The purchase amount, in micros of the purchase currency. e.g., 1 is represented as 1000000.
- Returns:
- value or
null
for none
-
setAmountMicros
public SuperChatEventSnippet setAmountMicros(BigInteger amountMicros)
The purchase amount, in micros of the purchase currency. e.g., 1 is represented as 1000000.
- Parameters:
amountMicros
- amountMicros or null
for none
-
getChannelId
public String getChannelId()
Channel id where the event occurred.
- Returns:
- value or
null
for none
-
setChannelId
public SuperChatEventSnippet setChannelId(String channelId)
Channel id where the event occurred.
- Parameters:
channelId
- channelId or null
for none
-
getCommentText
public String getCommentText()
The text contents of the comment left by the user.
- Returns:
- value or
null
for none
-
setCommentText
public SuperChatEventSnippet setCommentText(String commentText)
The text contents of the comment left by the user.
- Parameters:
commentText
- commentText or null
for none
-
getCreatedAt
public DateTime getCreatedAt()
The date and time when the event occurred.
- Returns:
- value or
null
for none
-
setCreatedAt
public SuperChatEventSnippet setCreatedAt(DateTime createdAt)
The date and time when the event occurred.
- Parameters:
createdAt
- createdAt or null
for none
-
getCurrency
public String getCurrency()
The currency in which the purchase was made. ISO 4217.
- Returns:
- value or
null
for none
-
setCurrency
public SuperChatEventSnippet setCurrency(String currency)
The currency in which the purchase was made. ISO 4217.
- Parameters:
currency
- currency or null
for none
-
getDisplayString
public String getDisplayString()
A rendered string that displays the purchase amount and currency (e.g., "$1.00"). The string is
rendered for the given language.
- Returns:
- value or
null
for none
-
setDisplayString
public SuperChatEventSnippet setDisplayString(String displayString)
A rendered string that displays the purchase amount and currency (e.g., "$1.00"). The string is
rendered for the given language.
- Parameters:
displayString
- displayString or null
for none
-
getIsSuperStickerEvent
public Boolean getIsSuperStickerEvent()
True if this event is a Super Sticker event.
- Returns:
- value or
null
for none
-
setIsSuperStickerEvent
public SuperChatEventSnippet setIsSuperStickerEvent(Boolean isSuperStickerEvent)
True if this event is a Super Sticker event.
- Parameters:
isSuperStickerEvent
- isSuperStickerEvent or null
for none
-
getMessageType
public Long getMessageType()
The tier for the paid message, which is based on the amount of money spent to purchase the
message.
- Returns:
- value or
null
for none
-
setMessageType
public SuperChatEventSnippet setMessageType(Long messageType)
The tier for the paid message, which is based on the amount of money spent to purchase the
message.
- Parameters:
messageType
- messageType or null
for none
-
getSuperStickerMetadata
public SuperStickerMetadata getSuperStickerMetadata()
If this event is a Super Sticker event, this field will contain metadata about the Super
Sticker.
- Returns:
- value or
null
for none
-
setSuperStickerMetadata
public SuperChatEventSnippet setSuperStickerMetadata(SuperStickerMetadata superStickerMetadata)
If this event is a Super Sticker event, this field will contain metadata about the Super
Sticker.
- Parameters:
superStickerMetadata
- superStickerMetadata or null
for none
-
getSupporterDetails
public ChannelProfileDetails getSupporterDetails()
Details about the supporter.
- Returns:
- value or
null
for none
-
setSupporterDetails
public SuperChatEventSnippet setSupporterDetails(ChannelProfileDetails supporterDetails)
Details about the supporter.
- Parameters:
supporterDetails
- supporterDetails or null
for none
-
set
public SuperChatEventSnippet set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public SuperChatEventSnippet clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2021 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy