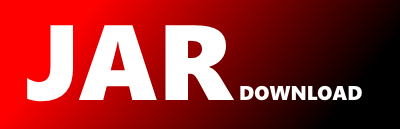
target.apidocs.com.google.api.services.youtube.model.Video.html Maven / Gradle / Ivy
Video (YouTube Data API v3 v3-rev20210410-1.31.0)
com.google.api.services.youtube.model
Class Video
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.youtube.model.Video
-
public final class Video
extends GenericJson
A *video* resource represents a YouTube video.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the YouTube Data API v3. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Video()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Video
clone()
VideoAgeGating
getAgeGating()
Age restriction details related to a video.
VideoContentDetails
getContentDetails()
The contentDetails object contains information about the video content, including the length of
the video and its aspect ratio.
String
getEtag()
Etag of this resource.
VideoFileDetails
getFileDetails()
The fileDetails object encapsulates information about the video file that was uploaded to
YouTube, including the file's resolution, duration, audio and video codecs, stream bitrates,
and more.
String
getId()
The ID that YouTube uses to uniquely identify the video.
String
getKind()
Identifies what kind of resource this is.
VideoLiveStreamingDetails
getLiveStreamingDetails()
The liveStreamingDetails object contains metadata about a live video broadcast.
Map<String,VideoLocalization>
getLocalizations()
The localizations object contains localized versions of the basic details about the video, such
as its title and description.
VideoMonetizationDetails
getMonetizationDetails()
The monetizationDetails object encapsulates information about the monetization status of the
video.
VideoPlayer
getPlayer()
The player object contains information that you would use to play the video in an embedded
player.
VideoProcessingDetails
getProcessingDetails()
The processingDetails object encapsulates information about YouTube's progress in processing
the uploaded video file.
VideoProjectDetails
getProjectDetails()
The projectDetails object contains information about the project specific video metadata.
VideoRecordingDetails
getRecordingDetails()
The recordingDetails object encapsulates information about the location, date and address where
the video was recorded.
VideoSnippet
getSnippet()
The snippet object contains basic details about the video, such as its title, description, and
category.
VideoStatistics
getStatistics()
The statistics object contains statistics about the video.
VideoStatus
getStatus()
The status object contains information about the video's uploading, processing, and privacy
statuses.
VideoSuggestions
getSuggestions()
The suggestions object encapsulates suggestions that identify opportunities to improve the
video quality or the metadata for the uploaded video.
VideoTopicDetails
getTopicDetails()
The topicDetails object encapsulates information about Freebase topics associated with the
video.
Video
set(String fieldName,
Object value)
Video
setAgeGating(VideoAgeGating ageGating)
Age restriction details related to a video.
Video
setContentDetails(VideoContentDetails contentDetails)
The contentDetails object contains information about the video content, including the length of
the video and its aspect ratio.
Video
setEtag(String etag)
Etag of this resource.
Video
setFileDetails(VideoFileDetails fileDetails)
The fileDetails object encapsulates information about the video file that was uploaded to
YouTube, including the file's resolution, duration, audio and video codecs, stream bitrates,
and more.
Video
setId(String id)
The ID that YouTube uses to uniquely identify the video.
Video
setKind(String kind)
Identifies what kind of resource this is.
Video
setLiveStreamingDetails(VideoLiveStreamingDetails liveStreamingDetails)
The liveStreamingDetails object contains metadata about a live video broadcast.
Video
setLocalizations(Map<String,VideoLocalization> localizations)
The localizations object contains localized versions of the basic details about the video, such
as its title and description.
Video
setMonetizationDetails(VideoMonetizationDetails monetizationDetails)
The monetizationDetails object encapsulates information about the monetization status of the
video.
Video
setPlayer(VideoPlayer player)
The player object contains information that you would use to play the video in an embedded
player.
Video
setProcessingDetails(VideoProcessingDetails processingDetails)
The processingDetails object encapsulates information about YouTube's progress in processing
the uploaded video file.
Video
setProjectDetails(VideoProjectDetails projectDetails)
The projectDetails object contains information about the project specific video metadata.
Video
setRecordingDetails(VideoRecordingDetails recordingDetails)
The recordingDetails object encapsulates information about the location, date and address where
the video was recorded.
Video
setSnippet(VideoSnippet snippet)
The snippet object contains basic details about the video, such as its title, description, and
category.
Video
setStatistics(VideoStatistics statistics)
The statistics object contains statistics about the video.
Video
setStatus(VideoStatus status)
The status object contains information about the video's uploading, processing, and privacy
statuses.
Video
setSuggestions(VideoSuggestions suggestions)
The suggestions object encapsulates suggestions that identify opportunities to improve the
video quality or the metadata for the uploaded video.
Video
setTopicDetails(VideoTopicDetails topicDetails)
The topicDetails object encapsulates information about Freebase topics associated with the
video.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAgeGating
public VideoAgeGating getAgeGating()
Age restriction details related to a video. This data can only be retrieved by the video owner.
- Returns:
- value or
null
for none
-
setAgeGating
public Video setAgeGating(VideoAgeGating ageGating)
Age restriction details related to a video. This data can only be retrieved by the video owner.
- Parameters:
ageGating
- ageGating or null
for none
-
getContentDetails
public VideoContentDetails getContentDetails()
The contentDetails object contains information about the video content, including the length of
the video and its aspect ratio.
- Returns:
- value or
null
for none
-
setContentDetails
public Video setContentDetails(VideoContentDetails contentDetails)
The contentDetails object contains information about the video content, including the length of
the video and its aspect ratio.
- Parameters:
contentDetails
- contentDetails or null
for none
-
getEtag
public String getEtag()
Etag of this resource.
- Returns:
- value or
null
for none
-
setEtag
public Video setEtag(String etag)
Etag of this resource.
- Parameters:
etag
- etag or null
for none
-
getFileDetails
public VideoFileDetails getFileDetails()
The fileDetails object encapsulates information about the video file that was uploaded to
YouTube, including the file's resolution, duration, audio and video codecs, stream bitrates,
and more. This data can only be retrieved by the video owner.
- Returns:
- value or
null
for none
-
setFileDetails
public Video setFileDetails(VideoFileDetails fileDetails)
The fileDetails object encapsulates information about the video file that was uploaded to
YouTube, including the file's resolution, duration, audio and video codecs, stream bitrates,
and more. This data can only be retrieved by the video owner.
- Parameters:
fileDetails
- fileDetails or null
for none
-
getId
public String getId()
The ID that YouTube uses to uniquely identify the video.
- Returns:
- value or
null
for none
-
setId
public Video setId(String id)
The ID that YouTube uses to uniquely identify the video.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
Identifies what kind of resource this is. Value: the fixed string "youtube#video".
- Returns:
- value or
null
for none
-
setKind
public Video setKind(String kind)
Identifies what kind of resource this is. Value: the fixed string "youtube#video".
- Parameters:
kind
- kind or null
for none
-
getLiveStreamingDetails
public VideoLiveStreamingDetails getLiveStreamingDetails()
The liveStreamingDetails object contains metadata about a live video broadcast. The object will
only be present in a video resource if the video is an upcoming, live, or completed live
broadcast.
- Returns:
- value or
null
for none
-
setLiveStreamingDetails
public Video setLiveStreamingDetails(VideoLiveStreamingDetails liveStreamingDetails)
The liveStreamingDetails object contains metadata about a live video broadcast. The object will
only be present in a video resource if the video is an upcoming, live, or completed live
broadcast.
- Parameters:
liveStreamingDetails
- liveStreamingDetails or null
for none
-
getLocalizations
public Map<String,VideoLocalization> getLocalizations()
The localizations object contains localized versions of the basic details about the video, such
as its title and description.
- Returns:
- value or
null
for none
-
setLocalizations
public Video setLocalizations(Map<String,VideoLocalization> localizations)
The localizations object contains localized versions of the basic details about the video, such
as its title and description.
- Parameters:
localizations
- localizations or null
for none
-
getMonetizationDetails
public VideoMonetizationDetails getMonetizationDetails()
The monetizationDetails object encapsulates information about the monetization status of the
video.
- Returns:
- value or
null
for none
-
setMonetizationDetails
public Video setMonetizationDetails(VideoMonetizationDetails monetizationDetails)
The monetizationDetails object encapsulates information about the monetization status of the
video.
- Parameters:
monetizationDetails
- monetizationDetails or null
for none
-
getPlayer
public VideoPlayer getPlayer()
The player object contains information that you would use to play the video in an embedded
player.
- Returns:
- value or
null
for none
-
setPlayer
public Video setPlayer(VideoPlayer player)
The player object contains information that you would use to play the video in an embedded
player.
- Parameters:
player
- player or null
for none
-
getProcessingDetails
public VideoProcessingDetails getProcessingDetails()
The processingDetails object encapsulates information about YouTube's progress in processing
the uploaded video file. The properties in the object identify the current processing status
and an estimate of the time remaining until YouTube finishes processing the video. This part
also indicates whether different types of data or content, such as file details or thumbnail
images, are available for the video. The processingProgress object is designed to be polled so
that the video uploaded can track the progress that YouTube has made in processing the uploaded
video file. This data can only be retrieved by the video owner.
- Returns:
- value or
null
for none
-
setProcessingDetails
public Video setProcessingDetails(VideoProcessingDetails processingDetails)
The processingDetails object encapsulates information about YouTube's progress in processing
the uploaded video file. The properties in the object identify the current processing status
and an estimate of the time remaining until YouTube finishes processing the video. This part
also indicates whether different types of data or content, such as file details or thumbnail
images, are available for the video. The processingProgress object is designed to be polled so
that the video uploaded can track the progress that YouTube has made in processing the uploaded
video file. This data can only be retrieved by the video owner.
- Parameters:
processingDetails
- processingDetails or null
for none
-
getProjectDetails
public VideoProjectDetails getProjectDetails()
The projectDetails object contains information about the project specific video metadata.
b/157517979: This part was never populated after it was added. However, it sees non-zero
traffic because there is generated client code in the wild that refers to it [1]. We keep this
field and do NOT remove it because otherwise V3 would return an error when this part gets
requested [2]. [1] https://developers.google.com/resources/api-libraries/documentation/youtube/
v3/csharp/latest/classGoogle_1_1Apis_1_1YouTube_1_1v3_1_1Data_1_1VideoProjectDetails.html [2]
http://google3/video/youtube/src/python/servers/data_api/common.py?l=1565-1569=344141677
- Returns:
- value or
null
for none
-
setProjectDetails
public Video setProjectDetails(VideoProjectDetails projectDetails)
The projectDetails object contains information about the project specific video metadata.
b/157517979: This part was never populated after it was added. However, it sees non-zero
traffic because there is generated client code in the wild that refers to it [1]. We keep this
field and do NOT remove it because otherwise V3 would return an error when this part gets
requested [2]. [1] https://developers.google.com/resources/api-libraries/documentation/youtube/
v3/csharp/latest/classGoogle_1_1Apis_1_1YouTube_1_1v3_1_1Data_1_1VideoProjectDetails.html [2]
http://google3/video/youtube/src/python/servers/data_api/common.py?l=1565-1569=344141677
- Parameters:
projectDetails
- projectDetails or null
for none
-
getRecordingDetails
public VideoRecordingDetails getRecordingDetails()
The recordingDetails object encapsulates information about the location, date and address where
the video was recorded.
- Returns:
- value or
null
for none
-
setRecordingDetails
public Video setRecordingDetails(VideoRecordingDetails recordingDetails)
The recordingDetails object encapsulates information about the location, date and address where
the video was recorded.
- Parameters:
recordingDetails
- recordingDetails or null
for none
-
getSnippet
public VideoSnippet getSnippet()
The snippet object contains basic details about the video, such as its title, description, and
category.
- Returns:
- value or
null
for none
-
setSnippet
public Video setSnippet(VideoSnippet snippet)
The snippet object contains basic details about the video, such as its title, description, and
category.
- Parameters:
snippet
- snippet or null
for none
-
getStatistics
public VideoStatistics getStatistics()
The statistics object contains statistics about the video.
- Returns:
- value or
null
for none
-
setStatistics
public Video setStatistics(VideoStatistics statistics)
The statistics object contains statistics about the video.
- Parameters:
statistics
- statistics or null
for none
-
getStatus
public VideoStatus getStatus()
The status object contains information about the video's uploading, processing, and privacy
statuses.
- Returns:
- value or
null
for none
-
setStatus
public Video setStatus(VideoStatus status)
The status object contains information about the video's uploading, processing, and privacy
statuses.
- Parameters:
status
- status or null
for none
-
getSuggestions
public VideoSuggestions getSuggestions()
The suggestions object encapsulates suggestions that identify opportunities to improve the
video quality or the metadata for the uploaded video. This data can only be retrieved by the
video owner.
- Returns:
- value or
null
for none
-
setSuggestions
public Video setSuggestions(VideoSuggestions suggestions)
The suggestions object encapsulates suggestions that identify opportunities to improve the
video quality or the metadata for the uploaded video. This data can only be retrieved by the
video owner.
- Parameters:
suggestions
- suggestions or null
for none
-
getTopicDetails
public VideoTopicDetails getTopicDetails()
The topicDetails object encapsulates information about Freebase topics associated with the
video.
- Returns:
- value or
null
for none
-
setTopicDetails
public Video setTopicDetails(VideoTopicDetails topicDetails)
The topicDetails object encapsulates information about Freebase topics associated with the
video.
- Parameters:
topicDetails
- topicDetails or null
for none
-
set
public Video set(String fieldName,
Object value)
- Overrides:
set
in class GenericJson
-
clone
public Video clone()
- Overrides:
clone
in class GenericJson
Copyright © 2011–2021 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy