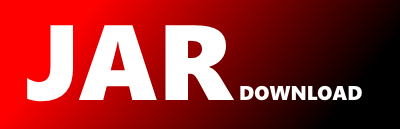
target.apidocs.com.google.api.services.youtube.model.VideoStatus.html Maven / Gradle / Ivy
VideoStatus (YouTube Data API v3 v3-rev20230319-2.0.0)
com.google.api.services.youtube.model
Class VideoStatus
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.youtube.model.VideoStatus
-
public final class VideoStatus
extends com.google.api.client.json.GenericJson
Basic details about a video category, such as its localized title. Next Id: 18
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the YouTube Data API v3. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
VideoStatus()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
VideoStatus
clone()
Boolean
getEmbeddable()
This value indicates if the video can be embedded on another website.
String
getFailureReason()
This value explains why a video failed to upload.
String
getLicense()
The video's license.
Boolean
getMadeForKids()
String
getPrivacyStatus()
The video's privacy status.
Boolean
getPublicStatsViewable()
This value indicates if the extended video statistics on the watch page can be viewed by
everyone.
com.google.api.client.util.DateTime
getPublishAt()
The date and time when the video is scheduled to publish.
String
getRejectionReason()
This value explains why YouTube rejected an uploaded video.
Boolean
getSelfDeclaredMadeForKids()
String
getUploadStatus()
The status of the uploaded video.
VideoStatus
set(String fieldName,
Object value)
VideoStatus
setEmbeddable(Boolean embeddable)
This value indicates if the video can be embedded on another website.
VideoStatus
setFailureReason(String failureReason)
This value explains why a video failed to upload.
VideoStatus
setLicense(String license)
The video's license.
VideoStatus
setMadeForKids(Boolean madeForKids)
VideoStatus
setPrivacyStatus(String privacyStatus)
The video's privacy status.
VideoStatus
setPublicStatsViewable(Boolean publicStatsViewable)
This value indicates if the extended video statistics on the watch page can be viewed by
everyone.
VideoStatus
setPublishAt(com.google.api.client.util.DateTime publishAt)
The date and time when the video is scheduled to publish.
VideoStatus
setRejectionReason(String rejectionReason)
This value explains why YouTube rejected an uploaded video.
VideoStatus
setSelfDeclaredMadeForKids(Boolean selfDeclaredMadeForKids)
VideoStatus
setUploadStatus(String uploadStatus)
The status of the uploaded video.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getEmbeddable
public Boolean getEmbeddable()
This value indicates if the video can be embedded on another website. @mutable
youtube.videos.insert youtube.videos.update
- Returns:
- value or
null
for none
-
setEmbeddable
public VideoStatus setEmbeddable(Boolean embeddable)
This value indicates if the video can be embedded on another website. @mutable
youtube.videos.insert youtube.videos.update
- Parameters:
embeddable
- embeddable or null
for none
-
getFailureReason
public String getFailureReason()
This value explains why a video failed to upload. This property is only present if the
uploadStatus property indicates that the upload failed.
- Returns:
- value or
null
for none
-
setFailureReason
public VideoStatus setFailureReason(String failureReason)
This value explains why a video failed to upload. This property is only present if the
uploadStatus property indicates that the upload failed.
- Parameters:
failureReason
- failureReason or null
for none
-
getLicense
public String getLicense()
The video's license. @mutable youtube.videos.insert youtube.videos.update
- Returns:
- value or
null
for none
-
setLicense
public VideoStatus setLicense(String license)
The video's license. @mutable youtube.videos.insert youtube.videos.update
- Parameters:
license
- license or null
for none
-
getMadeForKids
public Boolean getMadeForKids()
- Returns:
- value or
null
for none
-
setMadeForKids
public VideoStatus setMadeForKids(Boolean madeForKids)
- Parameters:
madeForKids
- madeForKids or null
for none
-
getPrivacyStatus
public String getPrivacyStatus()
The video's privacy status.
- Returns:
- value or
null
for none
-
setPrivacyStatus
public VideoStatus setPrivacyStatus(String privacyStatus)
The video's privacy status.
- Parameters:
privacyStatus
- privacyStatus or null
for none
-
getPublicStatsViewable
public Boolean getPublicStatsViewable()
This value indicates if the extended video statistics on the watch page can be viewed by
everyone. Note that the view count, likes, etc will still be visible if this is disabled.
- Returns:
- value or
null
for none
-
setPublicStatsViewable
public VideoStatus setPublicStatsViewable(Boolean publicStatsViewable)
This value indicates if the extended video statistics on the watch page can be viewed by
everyone. Note that the view count, likes, etc will still be visible if this is disabled.
- Parameters:
publicStatsViewable
- publicStatsViewable or null
for none
-
getPublishAt
public com.google.api.client.util.DateTime getPublishAt()
The date and time when the video is scheduled to publish. It can be set only if the privacy
status of the video is private..
- Returns:
- value or
null
for none
-
setPublishAt
public VideoStatus setPublishAt(com.google.api.client.util.DateTime publishAt)
The date and time when the video is scheduled to publish. It can be set only if the privacy
status of the video is private..
- Parameters:
publishAt
- publishAt or null
for none
-
getRejectionReason
public String getRejectionReason()
This value explains why YouTube rejected an uploaded video. This property is only present if
the uploadStatus property indicates that the upload was rejected.
- Returns:
- value or
null
for none
-
setRejectionReason
public VideoStatus setRejectionReason(String rejectionReason)
This value explains why YouTube rejected an uploaded video. This property is only present if
the uploadStatus property indicates that the upload was rejected.
- Parameters:
rejectionReason
- rejectionReason or null
for none
-
getSelfDeclaredMadeForKids
public Boolean getSelfDeclaredMadeForKids()
- Returns:
- value or
null
for none
-
setSelfDeclaredMadeForKids
public VideoStatus setSelfDeclaredMadeForKids(Boolean selfDeclaredMadeForKids)
- Parameters:
selfDeclaredMadeForKids
- selfDeclaredMadeForKids or null
for none
-
getUploadStatus
public String getUploadStatus()
The status of the uploaded video.
- Returns:
- value or
null
for none
-
setUploadStatus
public VideoStatus setUploadStatus(String uploadStatus)
The status of the uploaded video.
- Parameters:
uploadStatus
- uploadStatus or null
for none
-
set
public VideoStatus set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public VideoStatus clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2023 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy