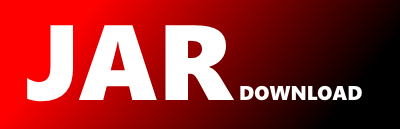
target.apidocs.com.google.api.services.youtube.model.CommentSnippet.html Maven / Gradle / Ivy
CommentSnippet (YouTube Data API v3 v3-rev20240417-2.0.0)
com.google.api.services.youtube.model
Class CommentSnippet
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.youtube.model.CommentSnippet
-
public final class CommentSnippet
extends com.google.api.client.json.GenericJson
Basic details about a comment, such as its author and text.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the YouTube Data API v3. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
CommentSnippet()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
CommentSnippet
clone()
CommentSnippetAuthorChannelId
getAuthorChannelId()
String
getAuthorChannelUrl()
Link to the author's YouTube channel, if any.
String
getAuthorDisplayName()
The name of the user who posted the comment.
String
getAuthorProfileImageUrl()
The URL for the avatar of the user who posted the comment.
Boolean
getCanRate()
Whether the current viewer can rate this comment.
String
getChannelId()
The id of the corresponding YouTube channel.
Long
getLikeCount()
The total number of likes this comment has received.
String
getModerationStatus()
The comment's moderation status.
String
getParentId()
The unique id of the parent comment, only set for replies.
com.google.api.client.util.DateTime
getPublishedAt()
The date and time when the comment was originally published.
String
getTextDisplay()
The comment's text.
String
getTextOriginal()
The comment's original raw text as initially posted or last updated.
com.google.api.client.util.DateTime
getUpdatedAt()
The date and time when the comment was last updated.
String
getVideoId()
The ID of the video the comment refers to, if any.
String
getViewerRating()
The rating the viewer has given to this comment.
CommentSnippet
set(String fieldName,
Object value)
CommentSnippet
setAuthorChannelId(CommentSnippetAuthorChannelId authorChannelId)
CommentSnippet
setAuthorChannelUrl(String authorChannelUrl)
Link to the author's YouTube channel, if any.
CommentSnippet
setAuthorDisplayName(String authorDisplayName)
The name of the user who posted the comment.
CommentSnippet
setAuthorProfileImageUrl(String authorProfileImageUrl)
The URL for the avatar of the user who posted the comment.
CommentSnippet
setCanRate(Boolean canRate)
Whether the current viewer can rate this comment.
CommentSnippet
setChannelId(String channelId)
The id of the corresponding YouTube channel.
CommentSnippet
setLikeCount(Long likeCount)
The total number of likes this comment has received.
CommentSnippet
setModerationStatus(String moderationStatus)
The comment's moderation status.
CommentSnippet
setParentId(String parentId)
The unique id of the parent comment, only set for replies.
CommentSnippet
setPublishedAt(com.google.api.client.util.DateTime publishedAt)
The date and time when the comment was originally published.
CommentSnippet
setTextDisplay(String textDisplay)
The comment's text.
CommentSnippet
setTextOriginal(String textOriginal)
The comment's original raw text as initially posted or last updated.
CommentSnippet
setUpdatedAt(com.google.api.client.util.DateTime updatedAt)
The date and time when the comment was last updated.
CommentSnippet
setVideoId(String videoId)
The ID of the video the comment refers to, if any.
CommentSnippet
setViewerRating(String viewerRating)
The rating the viewer has given to this comment.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAuthorChannelId
public CommentSnippetAuthorChannelId getAuthorChannelId()
- Returns:
- value or
null
for none
-
setAuthorChannelId
public CommentSnippet setAuthorChannelId(CommentSnippetAuthorChannelId authorChannelId)
- Parameters:
authorChannelId
- authorChannelId or null
for none
-
getAuthorChannelUrl
public String getAuthorChannelUrl()
Link to the author's YouTube channel, if any.
- Returns:
- value or
null
for none
-
setAuthorChannelUrl
public CommentSnippet setAuthorChannelUrl(String authorChannelUrl)
Link to the author's YouTube channel, if any.
- Parameters:
authorChannelUrl
- authorChannelUrl or null
for none
-
getAuthorDisplayName
public String getAuthorDisplayName()
The name of the user who posted the comment.
- Returns:
- value or
null
for none
-
setAuthorDisplayName
public CommentSnippet setAuthorDisplayName(String authorDisplayName)
The name of the user who posted the comment.
- Parameters:
authorDisplayName
- authorDisplayName or null
for none
-
getAuthorProfileImageUrl
public String getAuthorProfileImageUrl()
The URL for the avatar of the user who posted the comment.
- Returns:
- value or
null
for none
-
setAuthorProfileImageUrl
public CommentSnippet setAuthorProfileImageUrl(String authorProfileImageUrl)
The URL for the avatar of the user who posted the comment.
- Parameters:
authorProfileImageUrl
- authorProfileImageUrl or null
for none
-
getCanRate
public Boolean getCanRate()
Whether the current viewer can rate this comment.
- Returns:
- value or
null
for none
-
setCanRate
public CommentSnippet setCanRate(Boolean canRate)
Whether the current viewer can rate this comment.
- Parameters:
canRate
- canRate or null
for none
-
getChannelId
public String getChannelId()
The id of the corresponding YouTube channel. In case of a channel comment this is the channel
the comment refers to. In case of a video comment it's the video's channel.
- Returns:
- value or
null
for none
-
setChannelId
public CommentSnippet setChannelId(String channelId)
The id of the corresponding YouTube channel. In case of a channel comment this is the channel
the comment refers to. In case of a video comment it's the video's channel.
- Parameters:
channelId
- channelId or null
for none
-
getLikeCount
public Long getLikeCount()
The total number of likes this comment has received.
- Returns:
- value or
null
for none
-
setLikeCount
public CommentSnippet setLikeCount(Long likeCount)
The total number of likes this comment has received.
- Parameters:
likeCount
- likeCount or null
for none
-
getModerationStatus
public String getModerationStatus()
The comment's moderation status. Will not be set if the comments were requested through the id
filter.
- Returns:
- value or
null
for none
-
setModerationStatus
public CommentSnippet setModerationStatus(String moderationStatus)
The comment's moderation status. Will not be set if the comments were requested through the id
filter.
- Parameters:
moderationStatus
- moderationStatus or null
for none
-
getParentId
public String getParentId()
The unique id of the parent comment, only set for replies.
- Returns:
- value or
null
for none
-
setParentId
public CommentSnippet setParentId(String parentId)
The unique id of the parent comment, only set for replies.
- Parameters:
parentId
- parentId or null
for none
-
getPublishedAt
public com.google.api.client.util.DateTime getPublishedAt()
The date and time when the comment was originally published.
- Returns:
- value or
null
for none
-
setPublishedAt
public CommentSnippet setPublishedAt(com.google.api.client.util.DateTime publishedAt)
The date and time when the comment was originally published.
- Parameters:
publishedAt
- publishedAt or null
for none
-
getTextDisplay
public String getTextDisplay()
The comment's text. The format is either plain text or HTML dependent on what has been
requested. Even the plain text representation may differ from the text originally posted in
that it may replace video links with video titles etc.
- Returns:
- value or
null
for none
-
setTextDisplay
public CommentSnippet setTextDisplay(String textDisplay)
The comment's text. The format is either plain text or HTML dependent on what has been
requested. Even the plain text representation may differ from the text originally posted in
that it may replace video links with video titles etc.
- Parameters:
textDisplay
- textDisplay or null
for none
-
getTextOriginal
public String getTextOriginal()
The comment's original raw text as initially posted or last updated. The original text will
only be returned if it is accessible to the viewer, which is only guaranteed if the viewer is
the comment's author.
- Returns:
- value or
null
for none
-
setTextOriginal
public CommentSnippet setTextOriginal(String textOriginal)
The comment's original raw text as initially posted or last updated. The original text will
only be returned if it is accessible to the viewer, which is only guaranteed if the viewer is
the comment's author.
- Parameters:
textOriginal
- textOriginal or null
for none
-
getUpdatedAt
public com.google.api.client.util.DateTime getUpdatedAt()
The date and time when the comment was last updated.
- Returns:
- value or
null
for none
-
setUpdatedAt
public CommentSnippet setUpdatedAt(com.google.api.client.util.DateTime updatedAt)
The date and time when the comment was last updated.
- Parameters:
updatedAt
- updatedAt or null
for none
-
getVideoId
public String getVideoId()
The ID of the video the comment refers to, if any.
- Returns:
- value or
null
for none
-
setVideoId
public CommentSnippet setVideoId(String videoId)
The ID of the video the comment refers to, if any.
- Parameters:
videoId
- videoId or null
for none
-
getViewerRating
public String getViewerRating()
The rating the viewer has given to this comment. For the time being this will never return
RATE_TYPE_DISLIKE and instead return RATE_TYPE_NONE. This may change in the future.
- Returns:
- value or
null
for none
-
setViewerRating
public CommentSnippet setViewerRating(String viewerRating)
The rating the viewer has given to this comment. For the time being this will never return
RATE_TYPE_DISLIKE and instead return RATE_TYPE_NONE. This may change in the future.
- Parameters:
viewerRating
- viewerRating or null
for none
-
set
public CommentSnippet set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public CommentSnippet clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy