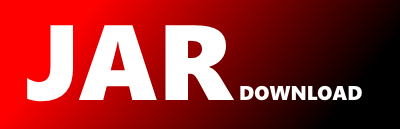
target.apidocs.com.google.api.services.youtube.model.ChannelSnippet.html Maven / Gradle / Ivy
ChannelSnippet (YouTube Data API v3 v3-rev20240909-2.0.0)
com.google.api.services.youtube.model
Class ChannelSnippet
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.youtube.model.ChannelSnippet
-
public final class ChannelSnippet
extends com.google.api.client.json.GenericJson
Basic details about a channel, including title, description and thumbnails.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the YouTube Data API v3. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
ChannelSnippet()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
ChannelSnippet
clone()
String
getCountry()
The country of the channel.
String
getCustomUrl()
The custom url of the channel.
String
getDefaultLanguage()
The language of the channel's default title and description.
String
getDescription()
The description of the channel.
ChannelLocalization
getLocalized()
Localized title and description, read-only.
com.google.api.client.util.DateTime
getPublishedAt()
The date and time that the channel was created.
ThumbnailDetails
getThumbnails()
A map of thumbnail images associated with the channel.
String
getTitle()
The channel's title.
ChannelSnippet
set(String fieldName,
Object value)
ChannelSnippet
setCountry(String country)
The country of the channel.
ChannelSnippet
setCustomUrl(String customUrl)
The custom url of the channel.
ChannelSnippet
setDefaultLanguage(String defaultLanguage)
The language of the channel's default title and description.
ChannelSnippet
setDescription(String description)
The description of the channel.
ChannelSnippet
setLocalized(ChannelLocalization localized)
Localized title and description, read-only.
ChannelSnippet
setPublishedAt(com.google.api.client.util.DateTime publishedAt)
The date and time that the channel was created.
ChannelSnippet
setThumbnails(ThumbnailDetails thumbnails)
A map of thumbnail images associated with the channel.
ChannelSnippet
setTitle(String title)
The channel's title.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getCountry
public String getCountry()
The country of the channel.
- Returns:
- value or
null
for none
-
setCountry
public ChannelSnippet setCountry(String country)
The country of the channel.
- Parameters:
country
- country or null
for none
-
getCustomUrl
public String getCustomUrl()
The custom url of the channel.
- Returns:
- value or
null
for none
-
setCustomUrl
public ChannelSnippet setCustomUrl(String customUrl)
The custom url of the channel.
- Parameters:
customUrl
- customUrl or null
for none
-
getDefaultLanguage
public String getDefaultLanguage()
The language of the channel's default title and description.
- Returns:
- value or
null
for none
-
setDefaultLanguage
public ChannelSnippet setDefaultLanguage(String defaultLanguage)
The language of the channel's default title and description.
- Parameters:
defaultLanguage
- defaultLanguage or null
for none
-
getDescription
public String getDescription()
The description of the channel.
- Returns:
- value or
null
for none
-
setDescription
public ChannelSnippet setDescription(String description)
The description of the channel.
- Parameters:
description
- description or null
for none
-
getLocalized
public ChannelLocalization getLocalized()
Localized title and description, read-only.
- Returns:
- value or
null
for none
-
setLocalized
public ChannelSnippet setLocalized(ChannelLocalization localized)
Localized title and description, read-only.
- Parameters:
localized
- localized or null
for none
-
getPublishedAt
public com.google.api.client.util.DateTime getPublishedAt()
The date and time that the channel was created.
- Returns:
- value or
null
for none
-
setPublishedAt
public ChannelSnippet setPublishedAt(com.google.api.client.util.DateTime publishedAt)
The date and time that the channel was created.
- Parameters:
publishedAt
- publishedAt or null
for none
-
getThumbnails
public ThumbnailDetails getThumbnails()
A map of thumbnail images associated with the channel. For each object in the map, the key is
the name of the thumbnail image, and the value is an object that contains other information
about the thumbnail. When displaying thumbnails in your application, make sure that your code
uses the image URLs exactly as they are returned in API responses. For example, your
application should not use the http domain instead of the https domain in a URL returned in an
API response. Beginning in July 2018, channel thumbnail URLs will only be available in the
https domain, which is how the URLs appear in API responses. After that time, you might see
broken images in your application if it tries to load YouTube images from the http domain.
Thumbnail images might be empty for newly created channels and might take up to one day to
populate.
- Returns:
- value or
null
for none
-
setThumbnails
public ChannelSnippet setThumbnails(ThumbnailDetails thumbnails)
A map of thumbnail images associated with the channel. For each object in the map, the key is
the name of the thumbnail image, and the value is an object that contains other information
about the thumbnail. When displaying thumbnails in your application, make sure that your code
uses the image URLs exactly as they are returned in API responses. For example, your
application should not use the http domain instead of the https domain in a URL returned in an
API response. Beginning in July 2018, channel thumbnail URLs will only be available in the
https domain, which is how the URLs appear in API responses. After that time, you might see
broken images in your application if it tries to load YouTube images from the http domain.
Thumbnail images might be empty for newly created channels and might take up to one day to
populate.
- Parameters:
thumbnails
- thumbnails or null
for none
-
getTitle
public String getTitle()
The channel's title.
- Returns:
- value or
null
for none
-
setTitle
public ChannelSnippet setTitle(String title)
The channel's title.
- Parameters:
title
- title or null
for none
-
set
public ChannelSnippet set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public ChannelSnippet clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy