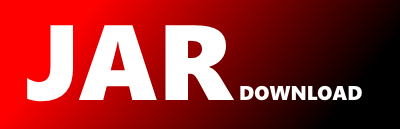
target.apidocs.com.google.api.services.youtube.model.VideoProcessingDetails.html Maven / Gradle / Ivy
VideoProcessingDetails (YouTube Data API v3 v3-rev20240909-2.0.0)
com.google.api.services.youtube.model
Class VideoProcessingDetails
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.youtube.model.VideoProcessingDetails
-
public final class VideoProcessingDetails
extends com.google.api.client.json.GenericJson
Describes processing status and progress and availability of some other Video resource parts.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the YouTube Data API v3. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
VideoProcessingDetails()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
VideoProcessingDetails
clone()
String
getEditorSuggestionsAvailability()
This value indicates whether video editing suggestions, which might improve video quality or
the playback experience, are available for the video.
String
getFileDetailsAvailability()
This value indicates whether file details are available for the uploaded video.
String
getProcessingFailureReason()
The reason that YouTube failed to process the video.
String
getProcessingIssuesAvailability()
This value indicates whether the video processing engine has generated suggestions that might
improve YouTube's ability to process the the video, warnings that explain video processing
problems, or errors that cause video processing problems.
VideoProcessingDetailsProcessingProgress
getProcessingProgress()
The processingProgress object contains information about the progress YouTube has made in
processing the video.
String
getProcessingStatus()
The video's processing status.
String
getTagSuggestionsAvailability()
This value indicates whether keyword (tag) suggestions are available for the video.
String
getThumbnailsAvailability()
This value indicates whether thumbnail images have been generated for the video.
VideoProcessingDetails
set(String fieldName,
Object value)
VideoProcessingDetails
setEditorSuggestionsAvailability(String editorSuggestionsAvailability)
This value indicates whether video editing suggestions, which might improve video quality or
the playback experience, are available for the video.
VideoProcessingDetails
setFileDetailsAvailability(String fileDetailsAvailability)
This value indicates whether file details are available for the uploaded video.
VideoProcessingDetails
setProcessingFailureReason(String processingFailureReason)
The reason that YouTube failed to process the video.
VideoProcessingDetails
setProcessingIssuesAvailability(String processingIssuesAvailability)
This value indicates whether the video processing engine has generated suggestions that might
improve YouTube's ability to process the the video, warnings that explain video processing
problems, or errors that cause video processing problems.
VideoProcessingDetails
setProcessingProgress(VideoProcessingDetailsProcessingProgress processingProgress)
The processingProgress object contains information about the progress YouTube has made in
processing the video.
VideoProcessingDetails
setProcessingStatus(String processingStatus)
The video's processing status.
VideoProcessingDetails
setTagSuggestionsAvailability(String tagSuggestionsAvailability)
This value indicates whether keyword (tag) suggestions are available for the video.
VideoProcessingDetails
setThumbnailsAvailability(String thumbnailsAvailability)
This value indicates whether thumbnail images have been generated for the video.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getEditorSuggestionsAvailability
public String getEditorSuggestionsAvailability()
This value indicates whether video editing suggestions, which might improve video quality or
the playback experience, are available for the video. You can retrieve these suggestions by
requesting the suggestions part in your videos.list() request.
- Returns:
- value or
null
for none
-
setEditorSuggestionsAvailability
public VideoProcessingDetails setEditorSuggestionsAvailability(String editorSuggestionsAvailability)
This value indicates whether video editing suggestions, which might improve video quality or
the playback experience, are available for the video. You can retrieve these suggestions by
requesting the suggestions part in your videos.list() request.
- Parameters:
editorSuggestionsAvailability
- editorSuggestionsAvailability or null
for none
-
getFileDetailsAvailability
public String getFileDetailsAvailability()
This value indicates whether file details are available for the uploaded video. You can
retrieve a video's file details by requesting the fileDetails part in your videos.list()
request.
- Returns:
- value or
null
for none
-
setFileDetailsAvailability
public VideoProcessingDetails setFileDetailsAvailability(String fileDetailsAvailability)
This value indicates whether file details are available for the uploaded video. You can
retrieve a video's file details by requesting the fileDetails part in your videos.list()
request.
- Parameters:
fileDetailsAvailability
- fileDetailsAvailability or null
for none
-
getProcessingFailureReason
public String getProcessingFailureReason()
The reason that YouTube failed to process the video. This property will only have a value if
the processingStatus property's value is failed.
- Returns:
- value or
null
for none
-
setProcessingFailureReason
public VideoProcessingDetails setProcessingFailureReason(String processingFailureReason)
The reason that YouTube failed to process the video. This property will only have a value if
the processingStatus property's value is failed.
- Parameters:
processingFailureReason
- processingFailureReason or null
for none
-
getProcessingIssuesAvailability
public String getProcessingIssuesAvailability()
This value indicates whether the video processing engine has generated suggestions that might
improve YouTube's ability to process the the video, warnings that explain video processing
problems, or errors that cause video processing problems. You can retrieve these suggestions by
requesting the suggestions part in your videos.list() request.
- Returns:
- value or
null
for none
-
setProcessingIssuesAvailability
public VideoProcessingDetails setProcessingIssuesAvailability(String processingIssuesAvailability)
This value indicates whether the video processing engine has generated suggestions that might
improve YouTube's ability to process the the video, warnings that explain video processing
problems, or errors that cause video processing problems. You can retrieve these suggestions by
requesting the suggestions part in your videos.list() request.
- Parameters:
processingIssuesAvailability
- processingIssuesAvailability or null
for none
-
getProcessingProgress
public VideoProcessingDetailsProcessingProgress getProcessingProgress()
The processingProgress object contains information about the progress YouTube has made in
processing the video. The values are really only relevant if the video's processing status is
processing.
- Returns:
- value or
null
for none
-
setProcessingProgress
public VideoProcessingDetails setProcessingProgress(VideoProcessingDetailsProcessingProgress processingProgress)
The processingProgress object contains information about the progress YouTube has made in
processing the video. The values are really only relevant if the video's processing status is
processing.
- Parameters:
processingProgress
- processingProgress or null
for none
-
getProcessingStatus
public String getProcessingStatus()
The video's processing status. This value indicates whether YouTube was able to process the
video or if the video is still being processed.
- Returns:
- value or
null
for none
-
setProcessingStatus
public VideoProcessingDetails setProcessingStatus(String processingStatus)
The video's processing status. This value indicates whether YouTube was able to process the
video or if the video is still being processed.
- Parameters:
processingStatus
- processingStatus or null
for none
-
getTagSuggestionsAvailability
public String getTagSuggestionsAvailability()
This value indicates whether keyword (tag) suggestions are available for the video. Tags can be
added to a video's metadata to make it easier for other users to find the video. You can
retrieve these suggestions by requesting the suggestions part in your videos.list() request.
- Returns:
- value or
null
for none
-
setTagSuggestionsAvailability
public VideoProcessingDetails setTagSuggestionsAvailability(String tagSuggestionsAvailability)
This value indicates whether keyword (tag) suggestions are available for the video. Tags can be
added to a video's metadata to make it easier for other users to find the video. You can
retrieve these suggestions by requesting the suggestions part in your videos.list() request.
- Parameters:
tagSuggestionsAvailability
- tagSuggestionsAvailability or null
for none
-
getThumbnailsAvailability
public String getThumbnailsAvailability()
This value indicates whether thumbnail images have been generated for the video.
- Returns:
- value or
null
for none
-
setThumbnailsAvailability
public VideoProcessingDetails setThumbnailsAvailability(String thumbnailsAvailability)
This value indicates whether thumbnail images have been generated for the video.
- Parameters:
thumbnailsAvailability
- thumbnailsAvailability or null
for none
-
set
public VideoProcessingDetails set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public VideoProcessingDetails clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy