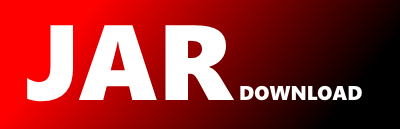
target.apidocs.com.google.api.services.youtube.model.CaptionSnippet.html Maven / Gradle / Ivy
CaptionSnippet (YouTube Data API v3 v3-rev20240916-2.0.0)
com.google.api.services.youtube.model
Class CaptionSnippet
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.youtube.model.CaptionSnippet
-
public final class CaptionSnippet
extends com.google.api.client.json.GenericJson
Basic details about a caption track, such as its language and name.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the YouTube Data API v3. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
CaptionSnippet()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
CaptionSnippet
clone()
String
getAudioTrackType()
The type of audio track associated with the caption track.
String
getFailureReason()
The reason that YouTube failed to process the caption track.
Boolean
getIsAutoSynced()
Indicates whether YouTube synchronized the caption track to the audio track in the video.
Boolean
getIsCC()
Indicates whether the track contains closed captions for the deaf and hard of hearing.
Boolean
getIsDraft()
Indicates whether the caption track is a draft.
Boolean
getIsEasyReader()
Indicates whether caption track is formatted for "easy reader," meaning it is at a third-grade
level for language learners.
Boolean
getIsLarge()
Indicates whether the caption track uses large text for the vision-impaired.
String
getLanguage()
The language of the caption track.
com.google.api.client.util.DateTime
getLastUpdated()
The date and time when the caption track was last updated.
String
getName()
The name of the caption track.
String
getStatus()
The caption track's status.
String
getTrackKind()
The caption track's type.
String
getVideoId()
The ID that YouTube uses to uniquely identify the video associated with the caption track.
CaptionSnippet
set(String fieldName,
Object value)
CaptionSnippet
setAudioTrackType(String audioTrackType)
The type of audio track associated with the caption track.
CaptionSnippet
setFailureReason(String failureReason)
The reason that YouTube failed to process the caption track.
CaptionSnippet
setIsAutoSynced(Boolean isAutoSynced)
Indicates whether YouTube synchronized the caption track to the audio track in the video.
CaptionSnippet
setIsCC(Boolean isCC)
Indicates whether the track contains closed captions for the deaf and hard of hearing.
CaptionSnippet
setIsDraft(Boolean isDraft)
Indicates whether the caption track is a draft.
CaptionSnippet
setIsEasyReader(Boolean isEasyReader)
Indicates whether caption track is formatted for "easy reader," meaning it is at a third-grade
level for language learners.
CaptionSnippet
setIsLarge(Boolean isLarge)
Indicates whether the caption track uses large text for the vision-impaired.
CaptionSnippet
setLanguage(String language)
The language of the caption track.
CaptionSnippet
setLastUpdated(com.google.api.client.util.DateTime lastUpdated)
The date and time when the caption track was last updated.
CaptionSnippet
setName(String name)
The name of the caption track.
CaptionSnippet
setStatus(String status)
The caption track's status.
CaptionSnippet
setTrackKind(String trackKind)
The caption track's type.
CaptionSnippet
setVideoId(String videoId)
The ID that YouTube uses to uniquely identify the video associated with the caption track.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAudioTrackType
public String getAudioTrackType()
The type of audio track associated with the caption track.
- Returns:
- value or
null
for none
-
setAudioTrackType
public CaptionSnippet setAudioTrackType(String audioTrackType)
The type of audio track associated with the caption track.
- Parameters:
audioTrackType
- audioTrackType or null
for none
-
getFailureReason
public String getFailureReason()
The reason that YouTube failed to process the caption track. This property is only present if
the state property's value is failed.
- Returns:
- value or
null
for none
-
setFailureReason
public CaptionSnippet setFailureReason(String failureReason)
The reason that YouTube failed to process the caption track. This property is only present if
the state property's value is failed.
- Parameters:
failureReason
- failureReason or null
for none
-
getIsAutoSynced
public Boolean getIsAutoSynced()
Indicates whether YouTube synchronized the caption track to the audio track in the video. The
value will be true if a sync was explicitly requested when the caption track was uploaded. For
example, when calling the captions.insert or captions.update methods, you can set the sync
parameter to true to instruct YouTube to sync the uploaded track to the video. If the value is
false, YouTube uses the time codes in the uploaded caption track to determine when to display
captions.
- Returns:
- value or
null
for none
-
setIsAutoSynced
public CaptionSnippet setIsAutoSynced(Boolean isAutoSynced)
Indicates whether YouTube synchronized the caption track to the audio track in the video. The
value will be true if a sync was explicitly requested when the caption track was uploaded. For
example, when calling the captions.insert or captions.update methods, you can set the sync
parameter to true to instruct YouTube to sync the uploaded track to the video. If the value is
false, YouTube uses the time codes in the uploaded caption track to determine when to display
captions.
- Parameters:
isAutoSynced
- isAutoSynced or null
for none
-
getIsCC
public Boolean getIsCC()
Indicates whether the track contains closed captions for the deaf and hard of hearing. The
default value is false.
- Returns:
- value or
null
for none
-
setIsCC
public CaptionSnippet setIsCC(Boolean isCC)
Indicates whether the track contains closed captions for the deaf and hard of hearing. The
default value is false.
- Parameters:
isCC
- isCC or null
for none
-
getIsDraft
public Boolean getIsDraft()
Indicates whether the caption track is a draft. If the value is true, then the track is not
publicly visible. The default value is false. @mutable youtube.captions.insert
youtube.captions.update
- Returns:
- value or
null
for none
-
setIsDraft
public CaptionSnippet setIsDraft(Boolean isDraft)
Indicates whether the caption track is a draft. If the value is true, then the track is not
publicly visible. The default value is false. @mutable youtube.captions.insert
youtube.captions.update
- Parameters:
isDraft
- isDraft or null
for none
-
getIsEasyReader
public Boolean getIsEasyReader()
Indicates whether caption track is formatted for "easy reader," meaning it is at a third-grade
level for language learners. The default value is false.
- Returns:
- value or
null
for none
-
setIsEasyReader
public CaptionSnippet setIsEasyReader(Boolean isEasyReader)
Indicates whether caption track is formatted for "easy reader," meaning it is at a third-grade
level for language learners. The default value is false.
- Parameters:
isEasyReader
- isEasyReader or null
for none
-
getIsLarge
public Boolean getIsLarge()
Indicates whether the caption track uses large text for the vision-impaired. The default value
is false.
- Returns:
- value or
null
for none
-
setIsLarge
public CaptionSnippet setIsLarge(Boolean isLarge)
Indicates whether the caption track uses large text for the vision-impaired. The default value
is false.
- Parameters:
isLarge
- isLarge or null
for none
-
getLanguage
public String getLanguage()
The language of the caption track. The property value is a BCP-47 language tag.
- Returns:
- value or
null
for none
-
setLanguage
public CaptionSnippet setLanguage(String language)
The language of the caption track. The property value is a BCP-47 language tag.
- Parameters:
language
- language or null
for none
-
getLastUpdated
public com.google.api.client.util.DateTime getLastUpdated()
The date and time when the caption track was last updated.
- Returns:
- value or
null
for none
-
setLastUpdated
public CaptionSnippet setLastUpdated(com.google.api.client.util.DateTime lastUpdated)
The date and time when the caption track was last updated.
- Parameters:
lastUpdated
- lastUpdated or null
for none
-
getName
public String getName()
The name of the caption track. The name is intended to be visible to the user as an option
during playback.
- Returns:
- value or
null
for none
-
setName
public CaptionSnippet setName(String name)
The name of the caption track. The name is intended to be visible to the user as an option
during playback.
- Parameters:
name
- name or null
for none
-
getStatus
public String getStatus()
The caption track's status.
- Returns:
- value or
null
for none
-
setStatus
public CaptionSnippet setStatus(String status)
The caption track's status.
- Parameters:
status
- status or null
for none
-
getTrackKind
public String getTrackKind()
The caption track's type.
- Returns:
- value or
null
for none
-
setTrackKind
public CaptionSnippet setTrackKind(String trackKind)
The caption track's type.
- Parameters:
trackKind
- trackKind or null
for none
-
getVideoId
public String getVideoId()
The ID that YouTube uses to uniquely identify the video associated with the caption track.
- Returns:
- value or
null
for none
-
setVideoId
public CaptionSnippet setVideoId(String videoId)
The ID that YouTube uses to uniquely identify the video associated with the caption track.
- Parameters:
videoId
- videoId or null
for none
-
set
public CaptionSnippet set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public CaptionSnippet clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy