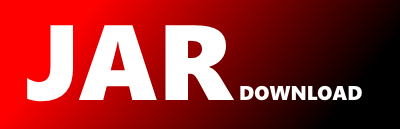
com.google.appengine.api.search.query.ExpressionLexer Maven / Gradle / Ivy
// $ANTLR 3.5.3 com/google/appengine/api/search/query/Expression.g 2024-06-06 12:43:20
package com.google.appengine.api.search.query;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class ExpressionLexer extends Lexer {
public static final int EOF=-1;
public static final int T__60=60;
public static final int ABS=4;
public static final int AND=5;
public static final int ASCII_LETTER=6;
public static final int ATOM=7;
public static final int COMMA=8;
public static final int COND=9;
public static final int COUNT=10;
public static final int DATE=11;
public static final int DIGIT=12;
public static final int DISTANCE=13;
public static final int DIV=14;
public static final int DOLLAR=15;
public static final int DOT=16;
public static final int EQ=17;
public static final int ESC_SEQ=18;
public static final int EXPONENT=19;
public static final int FLOAT=20;
public static final int GE=21;
public static final int GEO=22;
public static final int GEOPOINT=23;
public static final int GT=24;
public static final int HEX_DIGIT=25;
public static final int HTML=26;
public static final int INDEX=27;
public static final int INT=28;
public static final int LE=29;
public static final int LOG=30;
public static final int LPAREN=31;
public static final int LSQUARE=32;
public static final int LT=33;
public static final int MAX=34;
public static final int MIN=35;
public static final int MINUS=36;
public static final int NAME=37;
public static final int NAME_START=38;
public static final int NE=39;
public static final int NEG=40;
public static final int NOT=41;
public static final int NUMBER=42;
public static final int OCTAL_ESC=43;
public static final int OR=44;
public static final int PHRASE=45;
public static final int PLUS=46;
public static final int POW=47;
public static final int QUOTE=48;
public static final int RPAREN=49;
public static final int RSQUARE=50;
public static final int SNIPPET=51;
public static final int SWITCH=52;
public static final int TEXT=53;
public static final int TIMES=54;
public static final int UNDERSCORE=55;
public static final int UNICODE_ESC=56;
public static final int VECTOR=57;
public static final int WS=58;
public static final int XOR=59;
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public ExpressionLexer() {}
public ExpressionLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public ExpressionLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "com/google/appengine/api/search/query/Expression.g"; }
// $ANTLR start "T__60"
public final void mT__60() throws RecognitionException {
try {
int _type = T__60;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:6:7: ( '.' )
// com/google/appengine/api/search/query/Expression.g:6:9: '.'
{
match('.');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "T__60"
// $ANTLR start "ABS"
public final void mABS() throws RecognitionException {
try {
int _type = ABS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:187:3: ( 'abs' )
// com/google/appengine/api/search/query/Expression.g:187:5: 'abs'
{
match("abs");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ABS"
// $ANTLR start "COUNT"
public final void mCOUNT() throws RecognitionException {
try {
int _type = COUNT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:191:3: ( 'count' )
// com/google/appengine/api/search/query/Expression.g:191:5: 'count'
{
match("count");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COUNT"
// $ANTLR start "DISTANCE"
public final void mDISTANCE() throws RecognitionException {
try {
int _type = DISTANCE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:195:3: ( 'distance' )
// com/google/appengine/api/search/query/Expression.g:195:5: 'distance'
{
match("distance");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DISTANCE"
// $ANTLR start "GEOPOINT"
public final void mGEOPOINT() throws RecognitionException {
try {
int _type = GEOPOINT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:199:3: ( 'geopoint' )
// com/google/appengine/api/search/query/Expression.g:199:5: 'geopoint'
{
match("geopoint");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "GEOPOINT"
// $ANTLR start "LOG"
public final void mLOG() throws RecognitionException {
try {
int _type = LOG;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:203:3: ( 'log' )
// com/google/appengine/api/search/query/Expression.g:203:5: 'log'
{
match("log");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LOG"
// $ANTLR start "MAX"
public final void mMAX() throws RecognitionException {
try {
int _type = MAX;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:207:3: ( 'max' )
// com/google/appengine/api/search/query/Expression.g:207:5: 'max'
{
match("max");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MAX"
// $ANTLR start "MIN"
public final void mMIN() throws RecognitionException {
try {
int _type = MIN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:211:3: ( 'min' )
// com/google/appengine/api/search/query/Expression.g:211:5: 'min'
{
match("min");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MIN"
// $ANTLR start "POW"
public final void mPOW() throws RecognitionException {
try {
int _type = POW;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:215:3: ( 'pow' )
// com/google/appengine/api/search/query/Expression.g:215:5: 'pow'
{
match("pow");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "POW"
// $ANTLR start "AND"
public final void mAND() throws RecognitionException {
try {
int _type = AND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:219:3: ( 'AND' )
// com/google/appengine/api/search/query/Expression.g:219:5: 'AND'
{
match("AND");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AND"
// $ANTLR start "OR"
public final void mOR() throws RecognitionException {
try {
int _type = OR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:223:3: ( 'OR' )
// com/google/appengine/api/search/query/Expression.g:223:5: 'OR'
{
match("OR");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OR"
// $ANTLR start "XOR"
public final void mXOR() throws RecognitionException {
try {
int _type = XOR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:227:3: ( 'XOR' )
// com/google/appengine/api/search/query/Expression.g:227:5: 'XOR'
{
match("XOR");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "XOR"
// $ANTLR start "NOT"
public final void mNOT() throws RecognitionException {
try {
int _type = NOT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:231:3: ( 'NOT' )
// com/google/appengine/api/search/query/Expression.g:231:5: 'NOT'
{
match("NOT");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NOT"
// $ANTLR start "SNIPPET"
public final void mSNIPPET() throws RecognitionException {
try {
int _type = SNIPPET;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:235:2: ( 'snippet' )
// com/google/appengine/api/search/query/Expression.g:235:4: 'snippet'
{
match("snippet");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SNIPPET"
// $ANTLR start "SWITCH"
public final void mSWITCH() throws RecognitionException {
try {
int _type = SWITCH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:239:3: ( 'switch' )
// com/google/appengine/api/search/query/Expression.g:239:5: 'switch'
{
match("switch");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SWITCH"
// $ANTLR start "TEXT"
public final void mTEXT() throws RecognitionException {
try {
int _type = TEXT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:243:3: ( 'text' )
// com/google/appengine/api/search/query/Expression.g:243:5: 'text'
{
match("text");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TEXT"
// $ANTLR start "HTML"
public final void mHTML() throws RecognitionException {
try {
int _type = HTML;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:247:3: ( 'html' )
// com/google/appengine/api/search/query/Expression.g:247:5: 'html'
{
match("html");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HTML"
// $ANTLR start "ATOM"
public final void mATOM() throws RecognitionException {
try {
int _type = ATOM;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:251:3: ( 'atom' )
// com/google/appengine/api/search/query/Expression.g:251:5: 'atom'
{
match("atom");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ATOM"
// $ANTLR start "DATE"
public final void mDATE() throws RecognitionException {
try {
int _type = DATE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:255:3: ( 'date' )
// com/google/appengine/api/search/query/Expression.g:255:5: 'date'
{
match("date");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DATE"
// $ANTLR start "NUMBER"
public final void mNUMBER() throws RecognitionException {
try {
int _type = NUMBER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:259:3: ( 'number' )
// com/google/appengine/api/search/query/Expression.g:259:5: 'number'
{
match("number");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NUMBER"
// $ANTLR start "GEO"
public final void mGEO() throws RecognitionException {
try {
int _type = GEO;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:263:3: ( 'geo' )
// com/google/appengine/api/search/query/Expression.g:263:5: 'geo'
{
match("geo");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "GEO"
// $ANTLR start "DOT"
public final void mDOT() throws RecognitionException {
try {
int _type = DOT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:267:3: ( 'dot' )
// com/google/appengine/api/search/query/Expression.g:267:5: 'dot'
{
match("dot");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DOT"
// $ANTLR start "VECTOR"
public final void mVECTOR() throws RecognitionException {
try {
int _type = VECTOR;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:271:3: ( 'vector' )
// com/google/appengine/api/search/query/Expression.g:271:5: 'vector'
{
match("vector");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "VECTOR"
// $ANTLR start "INT"
public final void mINT() throws RecognitionException {
try {
int _type = INT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:275:3: ( ( DIGIT )+ )
// com/google/appengine/api/search/query/Expression.g:275:5: ( DIGIT )+
{
// com/google/appengine/api/search/query/Expression.g:275:5: ( DIGIT )+
int cnt1=0;
loop1:
while (true) {
int alt1=2;
int LA1_0 = input.LA(1);
if ( ((LA1_0 >= '0' && LA1_0 <= '9')) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt1 >= 1 ) break loop1;
EarlyExitException eee = new EarlyExitException(1, input);
throw eee;
}
cnt1++;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "INT"
// $ANTLR start "PHRASE"
public final void mPHRASE() throws RecognitionException {
try {
int _type = PHRASE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:279:3: ( QUOTE ( ESC_SEQ |~ ( '\"' | '\\\\' ) )* QUOTE )
// com/google/appengine/api/search/query/Expression.g:279:6: QUOTE ( ESC_SEQ |~ ( '\"' | '\\\\' ) )* QUOTE
{
mQUOTE();
// com/google/appengine/api/search/query/Expression.g:279:12: ( ESC_SEQ |~ ( '\"' | '\\\\' ) )*
loop2:
while (true) {
int alt2=3;
int LA2_0 = input.LA(1);
if ( (LA2_0=='\\') ) {
alt2=1;
}
else if ( ((LA2_0 >= '\u0000' && LA2_0 <= '!')||(LA2_0 >= '#' && LA2_0 <= '[')||(LA2_0 >= ']' && LA2_0 <= '\uFFFF')) ) {
alt2=2;
}
switch (alt2) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:279:13: ESC_SEQ
{
mESC_SEQ();
}
break;
case 2 :
// com/google/appengine/api/search/query/Expression.g:279:23: ~ ( '\"' | '\\\\' )
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '!')||(input.LA(1) >= '#' && input.LA(1) <= '[')||(input.LA(1) >= ']' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop2;
}
}
mQUOTE();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PHRASE"
// $ANTLR start "FLOAT"
public final void mFLOAT() throws RecognitionException {
try {
int _type = FLOAT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:283:3: ( ( DIGIT )+ '.' ( DIGIT )* ( EXPONENT )? | '.' ( DIGIT )+ ( EXPONENT )? | ( DIGIT )+ EXPONENT )
int alt9=3;
alt9 = dfa9.predict(input);
switch (alt9) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:283:5: ( DIGIT )+ '.' ( DIGIT )* ( EXPONENT )?
{
// com/google/appengine/api/search/query/Expression.g:283:5: ( DIGIT )+
int cnt3=0;
loop3:
while (true) {
int alt3=2;
int LA3_0 = input.LA(1);
if ( ((LA3_0 >= '0' && LA3_0 <= '9')) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt3 >= 1 ) break loop3;
EarlyExitException eee = new EarlyExitException(3, input);
throw eee;
}
cnt3++;
}
match('.');
// com/google/appengine/api/search/query/Expression.g:283:18: ( DIGIT )*
loop4:
while (true) {
int alt4=2;
int LA4_0 = input.LA(1);
if ( ((LA4_0 >= '0' && LA4_0 <= '9')) ) {
alt4=1;
}
switch (alt4) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop4;
}
}
// com/google/appengine/api/search/query/Expression.g:283:27: ( EXPONENT )?
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0=='E'||LA5_0=='e') ) {
alt5=1;
}
switch (alt5) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:283:27: EXPONENT
{
mEXPONENT();
}
break;
}
}
break;
case 2 :
// com/google/appengine/api/search/query/Expression.g:284:5: '.' ( DIGIT )+ ( EXPONENT )?
{
match('.');
// com/google/appengine/api/search/query/Expression.g:284:9: ( DIGIT )+
int cnt6=0;
loop6:
while (true) {
int alt6=2;
int LA6_0 = input.LA(1);
if ( ((LA6_0 >= '0' && LA6_0 <= '9')) ) {
alt6=1;
}
switch (alt6) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt6 >= 1 ) break loop6;
EarlyExitException eee = new EarlyExitException(6, input);
throw eee;
}
cnt6++;
}
// com/google/appengine/api/search/query/Expression.g:284:18: ( EXPONENT )?
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0=='E'||LA7_0=='e') ) {
alt7=1;
}
switch (alt7) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:284:18: EXPONENT
{
mEXPONENT();
}
break;
}
}
break;
case 3 :
// com/google/appengine/api/search/query/Expression.g:285:5: ( DIGIT )+ EXPONENT
{
// com/google/appengine/api/search/query/Expression.g:285:5: ( DIGIT )+
int cnt8=0;
loop8:
while (true) {
int alt8=2;
int LA8_0 = input.LA(1);
if ( ((LA8_0 >= '0' && LA8_0 <= '9')) ) {
alt8=1;
}
switch (alt8) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt8 >= 1 ) break loop8;
EarlyExitException eee = new EarlyExitException(8, input);
throw eee;
}
cnt8++;
}
mEXPONENT();
}
break;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FLOAT"
// $ANTLR start "NAME"
public final void mNAME() throws RecognitionException {
try {
int _type = NAME;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:289:3: ( NAME_START ( NAME_START | DIGIT )* )
// com/google/appengine/api/search/query/Expression.g:289:5: NAME_START ( NAME_START | DIGIT )*
{
mNAME_START();
// com/google/appengine/api/search/query/Expression.g:289:16: ( NAME_START | DIGIT )*
loop10:
while (true) {
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0=='$'||(LA10_0 >= '0' && LA10_0 <= '9')||(LA10_0 >= 'A' && LA10_0 <= 'Z')||LA10_0=='_'||(LA10_0 >= 'a' && LA10_0 <= 'z')) ) {
alt10=1;
}
switch (alt10) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( input.LA(1)=='$'||(input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop10;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NAME"
// $ANTLR start "LPAREN"
public final void mLPAREN() throws RecognitionException {
try {
int _type = LPAREN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:293:3: ( '(' )
// com/google/appengine/api/search/query/Expression.g:293:5: '('
{
match('(');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LPAREN"
// $ANTLR start "RPAREN"
public final void mRPAREN() throws RecognitionException {
try {
int _type = RPAREN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:297:3: ( ')' )
// com/google/appengine/api/search/query/Expression.g:297:5: ')'
{
match(')');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "RPAREN"
// $ANTLR start "LSQUARE"
public final void mLSQUARE() throws RecognitionException {
try {
int _type = LSQUARE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:301:3: ( '[' )
// com/google/appengine/api/search/query/Expression.g:301:5: '['
{
match('[');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LSQUARE"
// $ANTLR start "RSQUARE"
public final void mRSQUARE() throws RecognitionException {
try {
int _type = RSQUARE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:305:3: ( ']' )
// com/google/appengine/api/search/query/Expression.g:305:5: ']'
{
match(']');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "RSQUARE"
// $ANTLR start "PLUS"
public final void mPLUS() throws RecognitionException {
try {
int _type = PLUS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:309:3: ( '+' )
// com/google/appengine/api/search/query/Expression.g:309:5: '+'
{
match('+');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PLUS"
// $ANTLR start "MINUS"
public final void mMINUS() throws RecognitionException {
try {
int _type = MINUS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:313:3: ( '-' )
// com/google/appengine/api/search/query/Expression.g:313:5: '-'
{
match('-');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MINUS"
// $ANTLR start "TIMES"
public final void mTIMES() throws RecognitionException {
try {
int _type = TIMES;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:317:3: ( '*' )
// com/google/appengine/api/search/query/Expression.g:317:5: '*'
{
match('*');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TIMES"
// $ANTLR start "DIV"
public final void mDIV() throws RecognitionException {
try {
int _type = DIV;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:321:3: ( '/' )
// com/google/appengine/api/search/query/Expression.g:321:5: '/'
{
match('/');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DIV"
// $ANTLR start "LT"
public final void mLT() throws RecognitionException {
try {
int _type = LT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:325:3: ( '<' )
// com/google/appengine/api/search/query/Expression.g:325:5: '<'
{
match('<');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LT"
// $ANTLR start "LE"
public final void mLE() throws RecognitionException {
try {
int _type = LE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:329:3: ( '<=' )
// com/google/appengine/api/search/query/Expression.g:329:5: '<='
{
match("<=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LE"
// $ANTLR start "GT"
public final void mGT() throws RecognitionException {
try {
int _type = GT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:333:3: ( '>' )
// com/google/appengine/api/search/query/Expression.g:333:5: '>'
{
match('>');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "GT"
// $ANTLR start "GE"
public final void mGE() throws RecognitionException {
try {
int _type = GE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:337:3: ( '>=' )
// com/google/appengine/api/search/query/Expression.g:337:5: '>='
{
match(">=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "GE"
// $ANTLR start "EQ"
public final void mEQ() throws RecognitionException {
try {
int _type = EQ;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:341:3: ( '=' )
// com/google/appengine/api/search/query/Expression.g:341:5: '='
{
match('=');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EQ"
// $ANTLR start "NE"
public final void mNE() throws RecognitionException {
try {
int _type = NE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:345:3: ( '!=' )
// com/google/appengine/api/search/query/Expression.g:345:5: '!='
{
match("!=");
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NE"
// $ANTLR start "COND"
public final void mCOND() throws RecognitionException {
try {
int _type = COND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:349:3: ( '?' )
// com/google/appengine/api/search/query/Expression.g:349:5: '?'
{
match('?');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COND"
// $ANTLR start "QUOTE"
public final void mQUOTE() throws RecognitionException {
try {
int _type = QUOTE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:353:3: ( '\"' )
// com/google/appengine/api/search/query/Expression.g:353:5: '\"'
{
match('\"');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "QUOTE"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:357:3: ( ',' )
// com/google/appengine/api/search/query/Expression.g:357:5: ','
{
match(',');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMA"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/appengine/api/search/query/Expression.g:361:3: ( ( ' ' | '\\t' | '\\n' | '\\r' )+ )
// com/google/appengine/api/search/query/Expression.g:361:5: ( ' ' | '\\t' | '\\n' | '\\r' )+
{
// com/google/appengine/api/search/query/Expression.g:361:5: ( ' ' | '\\t' | '\\n' | '\\r' )+
int cnt11=0;
loop11:
while (true) {
int alt11=2;
int LA11_0 = input.LA(1);
if ( ((LA11_0 >= '\t' && LA11_0 <= '\n')||LA11_0=='\r'||LA11_0==' ') ) {
alt11=1;
}
switch (alt11) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '\t' && input.LA(1) <= '\n')||input.LA(1)=='\r'||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt11 >= 1 ) break loop11;
EarlyExitException eee = new EarlyExitException(11, input);
throw eee;
}
cnt11++;
}
_channel = HIDDEN;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WS"
// $ANTLR start "EXPONENT"
public final void mEXPONENT() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:365:3: ( ( 'e' | 'E' ) ( '+' | '-' )? ( DIGIT )+ )
// com/google/appengine/api/search/query/Expression.g:365:5: ( 'e' | 'E' ) ( '+' | '-' )? ( DIGIT )+
{
if ( input.LA(1)=='E'||input.LA(1)=='e' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
// com/google/appengine/api/search/query/Expression.g:365:15: ( '+' | '-' )?
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0=='+'||LA12_0=='-') ) {
alt12=1;
}
switch (alt12) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( input.LA(1)=='+'||input.LA(1)=='-' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
// com/google/appengine/api/search/query/Expression.g:365:26: ( DIGIT )+
int cnt13=0;
loop13:
while (true) {
int alt13=2;
int LA13_0 = input.LA(1);
if ( ((LA13_0 >= '0' && LA13_0 <= '9')) ) {
alt13=1;
}
switch (alt13) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt13 >= 1 ) break loop13;
EarlyExitException eee = new EarlyExitException(13, input);
throw eee;
}
cnt13++;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EXPONENT"
// $ANTLR start "NAME_START"
public final void mNAME_START() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:369:3: ( ( ASCII_LETTER | UNDERSCORE | DOLLAR ) )
// com/google/appengine/api/search/query/Expression.g:
{
if ( input.LA(1)=='$'||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NAME_START"
// $ANTLR start "ASCII_LETTER"
public final void mASCII_LETTER() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:373:3: ( 'a' .. 'z' | 'A' .. 'Z' )
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= 'A' && input.LA(1) <= 'Z')||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ASCII_LETTER"
// $ANTLR start "DIGIT"
public final void mDIGIT() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:378:3: ( '0' .. '9' )
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DIGIT"
// $ANTLR start "DOLLAR"
public final void mDOLLAR() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:382:3: ( '$' )
// com/google/appengine/api/search/query/Expression.g:382:5: '$'
{
match('$');
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DOLLAR"
// $ANTLR start "UNDERSCORE"
public final void mUNDERSCORE() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:386:3: ( '_' )
// com/google/appengine/api/search/query/Expression.g:386:5: '_'
{
match('_');
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "UNDERSCORE"
// $ANTLR start "HEX_DIGIT"
public final void mHEX_DIGIT() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:390:3: ( ( '0' .. '9' | 'a' .. 'f' | 'A' .. 'F' ) )
// com/google/appengine/api/search/query/Expression.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'F')||(input.LA(1) >= 'a' && input.LA(1) <= 'f') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HEX_DIGIT"
// $ANTLR start "ESC_SEQ"
public final void mESC_SEQ() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:394:3: ( '\\\\' ( 'b' | 't' | 'n' | 'f' | 'r' | '\"' | '\\'' | '\\\\' ) | UNICODE_ESC | OCTAL_ESC )
int alt14=3;
int LA14_0 = input.LA(1);
if ( (LA14_0=='\\') ) {
switch ( input.LA(2) ) {
case '\"':
case '\'':
case '\\':
case 'b':
case 'f':
case 'n':
case 'r':
case 't':
{
alt14=1;
}
break;
case 'u':
{
alt14=2;
}
break;
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
{
alt14=3;
}
break;
default:
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 14, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 14, 0, input);
throw nvae;
}
switch (alt14) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:394:5: '\\\\' ( 'b' | 't' | 'n' | 'f' | 'r' | '\"' | '\\'' | '\\\\' )
{
match('\\');
if ( input.LA(1)=='\"'||input.LA(1)=='\''||input.LA(1)=='\\'||input.LA(1)=='b'||input.LA(1)=='f'||input.LA(1)=='n'||input.LA(1)=='r'||input.LA(1)=='t' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
case 2 :
// com/google/appengine/api/search/query/Expression.g:395:5: UNICODE_ESC
{
mUNICODE_ESC();
}
break;
case 3 :
// com/google/appengine/api/search/query/Expression.g:396:5: OCTAL_ESC
{
mOCTAL_ESC();
}
break;
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ESC_SEQ"
// $ANTLR start "OCTAL_ESC"
public final void mOCTAL_ESC() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:400:3: ( '\\\\' ( '0' .. '3' ) ( '0' .. '7' ) ( '0' .. '7' ) | '\\\\' ( '0' .. '7' ) ( '0' .. '7' ) | '\\\\' ( '0' .. '7' ) )
int alt15=3;
int LA15_0 = input.LA(1);
if ( (LA15_0=='\\') ) {
int LA15_1 = input.LA(2);
if ( ((LA15_1 >= '0' && LA15_1 <= '3')) ) {
int LA15_2 = input.LA(3);
if ( ((LA15_2 >= '0' && LA15_2 <= '7')) ) {
int LA15_4 = input.LA(4);
if ( ((LA15_4 >= '0' && LA15_4 <= '7')) ) {
alt15=1;
}
else {
alt15=2;
}
}
else {
alt15=3;
}
}
else if ( ((LA15_1 >= '4' && LA15_1 <= '7')) ) {
int LA15_3 = input.LA(3);
if ( ((LA15_3 >= '0' && LA15_3 <= '7')) ) {
alt15=2;
}
else {
alt15=3;
}
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 15, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
NoViableAltException nvae =
new NoViableAltException("", 15, 0, input);
throw nvae;
}
switch (alt15) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:400:5: '\\\\' ( '0' .. '3' ) ( '0' .. '7' ) ( '0' .. '7' )
{
match('\\');
if ( (input.LA(1) >= '0' && input.LA(1) <= '3') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
if ( (input.LA(1) >= '0' && input.LA(1) <= '7') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
if ( (input.LA(1) >= '0' && input.LA(1) <= '7') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
case 2 :
// com/google/appengine/api/search/query/Expression.g:401:5: '\\\\' ( '0' .. '7' ) ( '0' .. '7' )
{
match('\\');
if ( (input.LA(1) >= '0' && input.LA(1) <= '7') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
if ( (input.LA(1) >= '0' && input.LA(1) <= '7') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
case 3 :
// com/google/appengine/api/search/query/Expression.g:402:5: '\\\\' ( '0' .. '7' )
{
match('\\');
if ( (input.LA(1) >= '0' && input.LA(1) <= '7') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OCTAL_ESC"
// $ANTLR start "UNICODE_ESC"
public final void mUNICODE_ESC() throws RecognitionException {
try {
// com/google/appengine/api/search/query/Expression.g:406:3: ( '\\\\' 'u' HEX_DIGIT HEX_DIGIT HEX_DIGIT HEX_DIGIT )
// com/google/appengine/api/search/query/Expression.g:406:6: '\\\\' 'u' HEX_DIGIT HEX_DIGIT HEX_DIGIT HEX_DIGIT
{
match('\\');
match('u');
mHEX_DIGIT();
mHEX_DIGIT();
mHEX_DIGIT();
mHEX_DIGIT();
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "UNICODE_ESC"
@Override
public void mTokens() throws RecognitionException {
// com/google/appengine/api/search/query/Expression.g:1:8: ( T__60 | ABS | COUNT | DISTANCE | GEOPOINT | LOG | MAX | MIN | POW | AND | OR | XOR | NOT | SNIPPET | SWITCH | TEXT | HTML | ATOM | DATE | NUMBER | GEO | DOT | VECTOR | INT | PHRASE | FLOAT | NAME | LPAREN | RPAREN | LSQUARE | RSQUARE | PLUS | MINUS | TIMES | DIV | LT | LE | GT | GE | EQ | NE | COND | QUOTE | COMMA | WS )
int alt16=45;
alt16 = dfa16.predict(input);
switch (alt16) {
case 1 :
// com/google/appengine/api/search/query/Expression.g:1:10: T__60
{
mT__60();
}
break;
case 2 :
// com/google/appengine/api/search/query/Expression.g:1:16: ABS
{
mABS();
}
break;
case 3 :
// com/google/appengine/api/search/query/Expression.g:1:20: COUNT
{
mCOUNT();
}
break;
case 4 :
// com/google/appengine/api/search/query/Expression.g:1:26: DISTANCE
{
mDISTANCE();
}
break;
case 5 :
// com/google/appengine/api/search/query/Expression.g:1:35: GEOPOINT
{
mGEOPOINT();
}
break;
case 6 :
// com/google/appengine/api/search/query/Expression.g:1:44: LOG
{
mLOG();
}
break;
case 7 :
// com/google/appengine/api/search/query/Expression.g:1:48: MAX
{
mMAX();
}
break;
case 8 :
// com/google/appengine/api/search/query/Expression.g:1:52: MIN
{
mMIN();
}
break;
case 9 :
// com/google/appengine/api/search/query/Expression.g:1:56: POW
{
mPOW();
}
break;
case 10 :
// com/google/appengine/api/search/query/Expression.g:1:60: AND
{
mAND();
}
break;
case 11 :
// com/google/appengine/api/search/query/Expression.g:1:64: OR
{
mOR();
}
break;
case 12 :
// com/google/appengine/api/search/query/Expression.g:1:67: XOR
{
mXOR();
}
break;
case 13 :
// com/google/appengine/api/search/query/Expression.g:1:71: NOT
{
mNOT();
}
break;
case 14 :
// com/google/appengine/api/search/query/Expression.g:1:75: SNIPPET
{
mSNIPPET();
}
break;
case 15 :
// com/google/appengine/api/search/query/Expression.g:1:83: SWITCH
{
mSWITCH();
}
break;
case 16 :
// com/google/appengine/api/search/query/Expression.g:1:90: TEXT
{
mTEXT();
}
break;
case 17 :
// com/google/appengine/api/search/query/Expression.g:1:95: HTML
{
mHTML();
}
break;
case 18 :
// com/google/appengine/api/search/query/Expression.g:1:100: ATOM
{
mATOM();
}
break;
case 19 :
// com/google/appengine/api/search/query/Expression.g:1:105: DATE
{
mDATE();
}
break;
case 20 :
// com/google/appengine/api/search/query/Expression.g:1:110: NUMBER
{
mNUMBER();
}
break;
case 21 :
// com/google/appengine/api/search/query/Expression.g:1:117: GEO
{
mGEO();
}
break;
case 22 :
// com/google/appengine/api/search/query/Expression.g:1:121: DOT
{
mDOT();
}
break;
case 23 :
// com/google/appengine/api/search/query/Expression.g:1:125: VECTOR
{
mVECTOR();
}
break;
case 24 :
// com/google/appengine/api/search/query/Expression.g:1:132: INT
{
mINT();
}
break;
case 25 :
// com/google/appengine/api/search/query/Expression.g:1:136: PHRASE
{
mPHRASE();
}
break;
case 26 :
// com/google/appengine/api/search/query/Expression.g:1:143: FLOAT
{
mFLOAT();
}
break;
case 27 :
// com/google/appengine/api/search/query/Expression.g:1:149: NAME
{
mNAME();
}
break;
case 28 :
// com/google/appengine/api/search/query/Expression.g:1:154: LPAREN
{
mLPAREN();
}
break;
case 29 :
// com/google/appengine/api/search/query/Expression.g:1:161: RPAREN
{
mRPAREN();
}
break;
case 30 :
// com/google/appengine/api/search/query/Expression.g:1:168: LSQUARE
{
mLSQUARE();
}
break;
case 31 :
// com/google/appengine/api/search/query/Expression.g:1:176: RSQUARE
{
mRSQUARE();
}
break;
case 32 :
// com/google/appengine/api/search/query/Expression.g:1:184: PLUS
{
mPLUS();
}
break;
case 33 :
// com/google/appengine/api/search/query/Expression.g:1:189: MINUS
{
mMINUS();
}
break;
case 34 :
// com/google/appengine/api/search/query/Expression.g:1:195: TIMES
{
mTIMES();
}
break;
case 35 :
// com/google/appengine/api/search/query/Expression.g:1:201: DIV
{
mDIV();
}
break;
case 36 :
// com/google/appengine/api/search/query/Expression.g:1:205: LT
{
mLT();
}
break;
case 37 :
// com/google/appengine/api/search/query/Expression.g:1:208: LE
{
mLE();
}
break;
case 38 :
// com/google/appengine/api/search/query/Expression.g:1:211: GT
{
mGT();
}
break;
case 39 :
// com/google/appengine/api/search/query/Expression.g:1:214: GE
{
mGE();
}
break;
case 40 :
// com/google/appengine/api/search/query/Expression.g:1:217: EQ
{
mEQ();
}
break;
case 41 :
// com/google/appengine/api/search/query/Expression.g:1:220: NE
{
mNE();
}
break;
case 42 :
// com/google/appengine/api/search/query/Expression.g:1:223: COND
{
mCOND();
}
break;
case 43 :
// com/google/appengine/api/search/query/Expression.g:1:228: QUOTE
{
mQUOTE();
}
break;
case 44 :
// com/google/appengine/api/search/query/Expression.g:1:234: COMMA
{
mCOMMA();
}
break;
case 45 :
// com/google/appengine/api/search/query/Expression.g:1:240: WS
{
mWS();
}
break;
}
}
protected DFA9 dfa9 = new DFA9(this);
protected DFA16 dfa16 = new DFA16(this);
static final String DFA9_eotS =
"\5\uffff";
static final String DFA9_eofS =
"\5\uffff";
static final String DFA9_minS =
"\2\56\3\uffff";
static final String DFA9_maxS =
"\1\71\1\145\3\uffff";
static final String DFA9_acceptS =
"\2\uffff\1\2\1\1\1\3";
static final String DFA9_specialS =
"\5\uffff}>";
static final String[] DFA9_transitionS = {
"\1\2\1\uffff\12\1",
"\1\3\1\uffff\12\1\13\uffff\1\4\37\uffff\1\4",
"",
"",
""
};
static final short[] DFA9_eot = DFA.unpackEncodedString(DFA9_eotS);
static final short[] DFA9_eof = DFA.unpackEncodedString(DFA9_eofS);
static final char[] DFA9_min = DFA.unpackEncodedStringToUnsignedChars(DFA9_minS);
static final char[] DFA9_max = DFA.unpackEncodedStringToUnsignedChars(DFA9_maxS);
static final short[] DFA9_accept = DFA.unpackEncodedString(DFA9_acceptS);
static final short[] DFA9_special = DFA.unpackEncodedString(DFA9_specialS);
static final short[][] DFA9_transition;
static {
int numStates = DFA9_transitionS.length;
DFA9_transition = new short[numStates][];
for (int i=0; i= '\u0000' && LA16_19 <= '\uFFFF')) ) {s = 61;}
else s = 60;
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 16, _s, input);
error(nvae);
throw nvae;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy