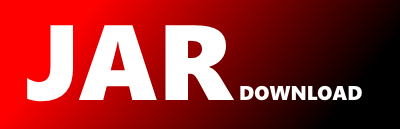
com.google.apphosting.executor.Task Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: task.proto
package com.google.apphosting.executor;
public final class Task {
private Task() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface TaskRefOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskRef)
com.google.protobuf.MessageOrBuilder {
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
* @return Whether the queueRef field is set.
*/
boolean hasQueueRef();
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
* @return The queueRef.
*/
com.google.apphosting.executor.Queue.QueueRef getQueueRef();
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
com.google.apphosting.executor.Queue.QueueRefOrBuilder getQueueRefOrBuilder();
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 2;
* @return The name.
*/
com.google.protobuf.ByteString getName();
}
/**
*
* http://g3doc/apphosting/g3doc/wiki-carryover/executor.md#Definition_of_a_Task
*
*
* Protobuf type {@code java.apphosting.TaskRef}
*/
public static final class TaskRef extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskRef)
TaskRefOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskRef.newBuilder() to construct.
private TaskRef(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskRef() {
name_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskRef();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRef_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRef.class, com.google.apphosting.executor.Task.TaskRef.Builder.class);
}
private int bitField0_;
public static final int QUEUE_REF_FIELD_NUMBER = 1;
private com.google.apphosting.executor.Queue.QueueRef queueRef_;
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
* @return Whether the queueRef field is set.
*/
@java.lang.Override
public boolean hasQueueRef() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
* @return The queueRef.
*/
@java.lang.Override
public com.google.apphosting.executor.Queue.QueueRef getQueueRef() {
return queueRef_ == null ? com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance() : queueRef_;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
@java.lang.Override
public com.google.apphosting.executor.Queue.QueueRefOrBuilder getQueueRefOrBuilder() {
return queueRef_ == null ? com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance() : queueRef_;
}
public static final int NAME_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 2;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getName() {
return name_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasQueueRef()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!getQueueRef().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getQueueRef());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, name_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getQueueRef());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, name_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskRef)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskRef other = (com.google.apphosting.executor.Task.TaskRef) obj;
if (hasQueueRef() != other.hasQueueRef()) return false;
if (hasQueueRef()) {
if (!getQueueRef()
.equals(other.getQueueRef())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasQueueRef()) {
hash = (37 * hash) + QUEUE_REF_FIELD_NUMBER;
hash = (53 * hash) + getQueueRef().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRef parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRef parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRef parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskRef prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* http://g3doc/apphosting/g3doc/wiki-carryover/executor.md#Definition_of_a_Task
*
*
* Protobuf type {@code java.apphosting.TaskRef}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskRef)
com.google.apphosting.executor.Task.TaskRefOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRef_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRef.class, com.google.apphosting.executor.Task.TaskRef.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskRef.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getQueueRefFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
queueRef_ = null;
if (queueRefBuilder_ != null) {
queueRefBuilder_.dispose();
queueRefBuilder_ = null;
}
name_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRef_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRef getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskRef.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRef build() {
com.google.apphosting.executor.Task.TaskRef result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRef buildPartial() {
com.google.apphosting.executor.Task.TaskRef result = new com.google.apphosting.executor.Task.TaskRef(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskRef result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.queueRef_ = queueRefBuilder_ == null
? queueRef_
: queueRefBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskRef) {
return mergeFrom((com.google.apphosting.executor.Task.TaskRef)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskRef other) {
if (other == com.google.apphosting.executor.Task.TaskRef.getDefaultInstance()) return this;
if (other.hasQueueRef()) {
mergeQueueRef(other.getQueueRef());
}
if (other.hasName()) {
setName(other.getName());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasQueueRef()) {
return false;
}
if (!hasName()) {
return false;
}
if (!getQueueRef().isInitialized()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getQueueRefFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
name_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.apphosting.executor.Queue.QueueRef queueRef_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Queue.QueueRef, com.google.apphosting.executor.Queue.QueueRef.Builder, com.google.apphosting.executor.Queue.QueueRefOrBuilder> queueRefBuilder_;
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
* @return Whether the queueRef field is set.
*/
public boolean hasQueueRef() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
* @return The queueRef.
*/
public com.google.apphosting.executor.Queue.QueueRef getQueueRef() {
if (queueRefBuilder_ == null) {
return queueRef_ == null ? com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance() : queueRef_;
} else {
return queueRefBuilder_.getMessage();
}
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
public Builder setQueueRef(com.google.apphosting.executor.Queue.QueueRef value) {
if (queueRefBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
queueRef_ = value;
} else {
queueRefBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
public Builder setQueueRef(
com.google.apphosting.executor.Queue.QueueRef.Builder builderForValue) {
if (queueRefBuilder_ == null) {
queueRef_ = builderForValue.build();
} else {
queueRefBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
public Builder mergeQueueRef(com.google.apphosting.executor.Queue.QueueRef value) {
if (queueRefBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
queueRef_ != null &&
queueRef_ != com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance()) {
getQueueRefBuilder().mergeFrom(value);
} else {
queueRef_ = value;
}
} else {
queueRefBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
public Builder clearQueueRef() {
bitField0_ = (bitField0_ & ~0x00000001);
queueRef_ = null;
if (queueRefBuilder_ != null) {
queueRefBuilder_.dispose();
queueRefBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
public com.google.apphosting.executor.Queue.QueueRef.Builder getQueueRefBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getQueueRefFieldBuilder().getBuilder();
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
public com.google.apphosting.executor.Queue.QueueRefOrBuilder getQueueRefOrBuilder() {
if (queueRefBuilder_ != null) {
return queueRefBuilder_.getMessageOrBuilder();
} else {
return queueRef_ == null ?
com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance() : queueRef_;
}
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Queue.QueueRef, com.google.apphosting.executor.Queue.QueueRef.Builder, com.google.apphosting.executor.Queue.QueueRefOrBuilder>
getQueueRefFieldBuilder() {
if (queueRefBuilder_ == null) {
queueRefBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Queue.QueueRef, com.google.apphosting.executor.Queue.QueueRef.Builder, com.google.apphosting.executor.Queue.QueueRefOrBuilder>(
getQueueRef(),
getParentForChildren(),
isClean());
queueRef_ = null;
}
return queueRefBuilder_;
}
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 2;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getName() {
return name_;
}
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskRef)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskRef)
private static final com.google.apphosting.executor.Task.TaskRef DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskRef();
}
public static com.google.apphosting.executor.Task.TaskRef getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskRef parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRef getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskIndexRefOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskIndexRef)
com.google.protobuf.MessageOrBuilder {
/**
*
* The shard of the sharded index to which this index ref belongs. During the
* transition period of the key reshard project we will maintain both
* sharded and non-sharded indexes. A minus one in this field indicates
* that this is an older-type non-sharded index ref.
*
*
* optional int32 shard_num = 1 [default = -1];
* @return Whether the shardNum field is set.
*/
boolean hasShardNum();
/**
*
* The shard of the sharded index to which this index ref belongs. During the
* transition period of the key reshard project we will maintain both
* sharded and non-sharded indexes. A minus one in this field indicates
* that this is an older-type non-sharded index ref.
*
*
* optional int32 shard_num = 1 [default = -1];
* @return The shardNum.
*/
int getShardNum();
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
* @return Whether the queueRef field is set.
*/
boolean hasQueueRef();
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
* @return The queueRef.
*/
com.google.apphosting.executor.Queue.QueueRef getQueueRef();
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
com.google.apphosting.executor.Queue.QueueRefOrBuilder getQueueRefOrBuilder();
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 3;
* @return Whether the etaUsec field is set.
*/
boolean hasEtaUsec();
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 3;
* @return The etaUsec.
*/
long getEtaUsec();
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 4;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 4;
* @return The name.
*/
com.google.protobuf.ByteString getName();
/**
*
* Optional user defined task tag.
*
*
* optional bytes tag = 5;
* @return Whether the tag field is set.
*/
boolean hasTag();
/**
*
* Optional user defined task tag.
*
*
* optional bytes tag = 5;
* @return The tag.
*/
com.google.protobuf.ByteString getTag();
}
/**
* Protobuf type {@code java.apphosting.TaskIndexRef}
*/
public static final class TaskIndexRef extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskIndexRef)
TaskIndexRefOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskIndexRef.newBuilder() to construct.
private TaskIndexRef(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskIndexRef() {
shardNum_ = -1;
name_ = com.google.protobuf.ByteString.EMPTY;
tag_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskIndexRef();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskIndexRef_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskIndexRef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskIndexRef.class, com.google.apphosting.executor.Task.TaskIndexRef.Builder.class);
}
private int bitField0_;
public static final int SHARD_NUM_FIELD_NUMBER = 1;
private int shardNum_ = -1;
/**
*
* The shard of the sharded index to which this index ref belongs. During the
* transition period of the key reshard project we will maintain both
* sharded and non-sharded indexes. A minus one in this field indicates
* that this is an older-type non-sharded index ref.
*
*
* optional int32 shard_num = 1 [default = -1];
* @return Whether the shardNum field is set.
*/
@java.lang.Override
public boolean hasShardNum() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The shard of the sharded index to which this index ref belongs. During the
* transition period of the key reshard project we will maintain both
* sharded and non-sharded indexes. A minus one in this field indicates
* that this is an older-type non-sharded index ref.
*
*
* optional int32 shard_num = 1 [default = -1];
* @return The shardNum.
*/
@java.lang.Override
public int getShardNum() {
return shardNum_;
}
public static final int QUEUE_REF_FIELD_NUMBER = 2;
private com.google.apphosting.executor.Queue.QueueRef queueRef_;
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
* @return Whether the queueRef field is set.
*/
@java.lang.Override
public boolean hasQueueRef() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
* @return The queueRef.
*/
@java.lang.Override
public com.google.apphosting.executor.Queue.QueueRef getQueueRef() {
return queueRef_ == null ? com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance() : queueRef_;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
@java.lang.Override
public com.google.apphosting.executor.Queue.QueueRefOrBuilder getQueueRefOrBuilder() {
return queueRef_ == null ? com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance() : queueRef_;
}
public static final int ETA_USEC_FIELD_NUMBER = 3;
private long etaUsec_ = 0L;
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 3;
* @return Whether the etaUsec field is set.
*/
@java.lang.Override
public boolean hasEtaUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 3;
* @return The etaUsec.
*/
@java.lang.Override
public long getEtaUsec() {
return etaUsec_;
}
public static final int NAME_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 4;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 4;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getName() {
return name_;
}
public static final int TAG_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString tag_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Optional user defined task tag.
*
*
* optional bytes tag = 5;
* @return Whether the tag field is set.
*/
@java.lang.Override
public boolean hasTag() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Optional user defined task tag.
*
*
* optional bytes tag = 5;
* @return The tag.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTag() {
return tag_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasQueueRef()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasEtaUsec()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!getQueueRef().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, shardNum_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getQueueRef());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(3, etaUsec_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBytes(4, name_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBytes(5, tag_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, shardNum_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getQueueRef());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, etaUsec_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, name_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, tag_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskIndexRef)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskIndexRef other = (com.google.apphosting.executor.Task.TaskIndexRef) obj;
if (hasShardNum() != other.hasShardNum()) return false;
if (hasShardNum()) {
if (getShardNum()
!= other.getShardNum()) return false;
}
if (hasQueueRef() != other.hasQueueRef()) return false;
if (hasQueueRef()) {
if (!getQueueRef()
.equals(other.getQueueRef())) return false;
}
if (hasEtaUsec() != other.hasEtaUsec()) return false;
if (hasEtaUsec()) {
if (getEtaUsec()
!= other.getEtaUsec()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasTag() != other.hasTag()) return false;
if (hasTag()) {
if (!getTag()
.equals(other.getTag())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasShardNum()) {
hash = (37 * hash) + SHARD_NUM_FIELD_NUMBER;
hash = (53 * hash) + getShardNum();
}
if (hasQueueRef()) {
hash = (37 * hash) + QUEUE_REF_FIELD_NUMBER;
hash = (53 * hash) + getQueueRef().hashCode();
}
if (hasEtaUsec()) {
hash = (37 * hash) + ETA_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getEtaUsec());
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasTag()) {
hash = (37 * hash) + TAG_FIELD_NUMBER;
hash = (53 * hash) + getTag().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskIndexRef parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskIndexRef prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.TaskIndexRef}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskIndexRef)
com.google.apphosting.executor.Task.TaskIndexRefOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskIndexRef_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskIndexRef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskIndexRef.class, com.google.apphosting.executor.Task.TaskIndexRef.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskIndexRef.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getQueueRefFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
shardNum_ = -1;
queueRef_ = null;
if (queueRefBuilder_ != null) {
queueRefBuilder_.dispose();
queueRefBuilder_ = null;
}
etaUsec_ = 0L;
name_ = com.google.protobuf.ByteString.EMPTY;
tag_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskIndexRef_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskIndexRef getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskIndexRef.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskIndexRef build() {
com.google.apphosting.executor.Task.TaskIndexRef result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskIndexRef buildPartial() {
com.google.apphosting.executor.Task.TaskIndexRef result = new com.google.apphosting.executor.Task.TaskIndexRef(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskIndexRef result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.shardNum_ = shardNum_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.queueRef_ = queueRefBuilder_ == null
? queueRef_
: queueRefBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.etaUsec_ = etaUsec_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.tag_ = tag_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskIndexRef) {
return mergeFrom((com.google.apphosting.executor.Task.TaskIndexRef)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskIndexRef other) {
if (other == com.google.apphosting.executor.Task.TaskIndexRef.getDefaultInstance()) return this;
if (other.hasShardNum()) {
setShardNum(other.getShardNum());
}
if (other.hasQueueRef()) {
mergeQueueRef(other.getQueueRef());
}
if (other.hasEtaUsec()) {
setEtaUsec(other.getEtaUsec());
}
if (other.hasName()) {
setName(other.getName());
}
if (other.hasTag()) {
setTag(other.getTag());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasQueueRef()) {
return false;
}
if (!hasEtaUsec()) {
return false;
}
if (!hasName()) {
return false;
}
if (!getQueueRef().isInitialized()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
shardNum_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
input.readMessage(
getQueueRefFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
etaUsec_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
name_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
tag_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int shardNum_ = -1;
/**
*
* The shard of the sharded index to which this index ref belongs. During the
* transition period of the key reshard project we will maintain both
* sharded and non-sharded indexes. A minus one in this field indicates
* that this is an older-type non-sharded index ref.
*
*
* optional int32 shard_num = 1 [default = -1];
* @return Whether the shardNum field is set.
*/
@java.lang.Override
public boolean hasShardNum() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The shard of the sharded index to which this index ref belongs. During the
* transition period of the key reshard project we will maintain both
* sharded and non-sharded indexes. A minus one in this field indicates
* that this is an older-type non-sharded index ref.
*
*
* optional int32 shard_num = 1 [default = -1];
* @return The shardNum.
*/
@java.lang.Override
public int getShardNum() {
return shardNum_;
}
/**
*
* The shard of the sharded index to which this index ref belongs. During the
* transition period of the key reshard project we will maintain both
* sharded and non-sharded indexes. A minus one in this field indicates
* that this is an older-type non-sharded index ref.
*
*
* optional int32 shard_num = 1 [default = -1];
* @param value The shardNum to set.
* @return This builder for chaining.
*/
public Builder setShardNum(int value) {
shardNum_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The shard of the sharded index to which this index ref belongs. During the
* transition period of the key reshard project we will maintain both
* sharded and non-sharded indexes. A minus one in this field indicates
* that this is an older-type non-sharded index ref.
*
*
* optional int32 shard_num = 1 [default = -1];
* @return This builder for chaining.
*/
public Builder clearShardNum() {
bitField0_ = (bitField0_ & ~0x00000001);
shardNum_ = -1;
onChanged();
return this;
}
private com.google.apphosting.executor.Queue.QueueRef queueRef_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Queue.QueueRef, com.google.apphosting.executor.Queue.QueueRef.Builder, com.google.apphosting.executor.Queue.QueueRefOrBuilder> queueRefBuilder_;
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
* @return Whether the queueRef field is set.
*/
public boolean hasQueueRef() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
* @return The queueRef.
*/
public com.google.apphosting.executor.Queue.QueueRef getQueueRef() {
if (queueRefBuilder_ == null) {
return queueRef_ == null ? com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance() : queueRef_;
} else {
return queueRefBuilder_.getMessage();
}
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
public Builder setQueueRef(com.google.apphosting.executor.Queue.QueueRef value) {
if (queueRefBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
queueRef_ = value;
} else {
queueRefBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
public Builder setQueueRef(
com.google.apphosting.executor.Queue.QueueRef.Builder builderForValue) {
if (queueRefBuilder_ == null) {
queueRef_ = builderForValue.build();
} else {
queueRefBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
public Builder mergeQueueRef(com.google.apphosting.executor.Queue.QueueRef value) {
if (queueRefBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
queueRef_ != null &&
queueRef_ != com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance()) {
getQueueRefBuilder().mergeFrom(value);
} else {
queueRef_ = value;
}
} else {
queueRefBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
public Builder clearQueueRef() {
bitField0_ = (bitField0_ & ~0x00000002);
queueRef_ = null;
if (queueRefBuilder_ != null) {
queueRefBuilder_.dispose();
queueRefBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
public com.google.apphosting.executor.Queue.QueueRef.Builder getQueueRefBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getQueueRefFieldBuilder().getBuilder();
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
public com.google.apphosting.executor.Queue.QueueRefOrBuilder getQueueRefOrBuilder() {
if (queueRefBuilder_ != null) {
return queueRefBuilder_.getMessageOrBuilder();
} else {
return queueRef_ == null ?
com.google.apphosting.executor.Queue.QueueRef.getDefaultInstance() : queueRef_;
}
}
/**
*
* Queue to which the task belongs.
*
*
* required .java.apphosting.QueueRef queue_ref = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Queue.QueueRef, com.google.apphosting.executor.Queue.QueueRef.Builder, com.google.apphosting.executor.Queue.QueueRefOrBuilder>
getQueueRefFieldBuilder() {
if (queueRefBuilder_ == null) {
queueRefBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Queue.QueueRef, com.google.apphosting.executor.Queue.QueueRef.Builder, com.google.apphosting.executor.Queue.QueueRefOrBuilder>(
getQueueRef(),
getParentForChildren(),
isClean());
queueRef_ = null;
}
return queueRefBuilder_;
}
private long etaUsec_ ;
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 3;
* @return Whether the etaUsec field is set.
*/
@java.lang.Override
public boolean hasEtaUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 3;
* @return The etaUsec.
*/
@java.lang.Override
public long getEtaUsec() {
return etaUsec_;
}
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 3;
* @param value The etaUsec to set.
* @return This builder for chaining.
*/
public Builder setEtaUsec(long value) {
etaUsec_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 3;
* @return This builder for chaining.
*/
public Builder clearEtaUsec() {
bitField0_ = (bitField0_ & ~0x00000004);
etaUsec_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 4;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 4;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getName() {
return name_;
}
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 4;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Unique identifier for the task within the queue.
*
*
* required bytes name = 4;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000008);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
private com.google.protobuf.ByteString tag_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Optional user defined task tag.
*
*
* optional bytes tag = 5;
* @return Whether the tag field is set.
*/
@java.lang.Override
public boolean hasTag() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Optional user defined task tag.
*
*
* optional bytes tag = 5;
* @return The tag.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTag() {
return tag_;
}
/**
*
* Optional user defined task tag.
*
*
* optional bytes tag = 5;
* @param value The tag to set.
* @return This builder for chaining.
*/
public Builder setTag(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
tag_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Optional user defined task tag.
*
*
* optional bytes tag = 5;
* @return This builder for chaining.
*/
public Builder clearTag() {
bitField0_ = (bitField0_ & ~0x00000010);
tag_ = getDefaultInstance().getTag();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskIndexRef)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskIndexRef)
private static final com.google.apphosting.executor.Task.TaskIndexRef DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskIndexRef();
}
public static com.google.apphosting.executor.Task.TaskIndexRef getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskIndexRef parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskIndexRef getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskRangeOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskRange)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* Generic task range (used for the scanning).
* All ranges are defined within a single queue.
* If start is unset, means the start of the queue (minus infinity).
* If limit is unset, means the end of the queue (positive infinity).
*
*
* Protobuf type {@code java.apphosting.TaskRange}
*/
public static final class TaskRange extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskRange)
TaskRangeOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskRange.newBuilder() to construct.
private TaskRange(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskRange() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskRange();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRange_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRange.class, com.google.apphosting.executor.Task.TaskRange.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeAsMessageSetTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSizeAsMessageSet();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskRange)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskRange other = (com.google.apphosting.executor.Task.TaskRange) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRange parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRange parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRange parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskRange prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Generic task range (used for the scanning).
* All ranges are defined within a single queue.
* If start is unset, means the start of the queue (minus infinity).
* If limit is unset, means the end of the queue (positive infinity).
*
*
* Protobuf type {@code java.apphosting.TaskRange}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskRange)
com.google.apphosting.executor.Task.TaskRangeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRange_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRange_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRange.class, com.google.apphosting.executor.Task.TaskRange.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskRange.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRange_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRange getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskRange.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRange build() {
com.google.apphosting.executor.Task.TaskRange result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRange buildPartial() {
com.google.apphosting.executor.Task.TaskRange result = new com.google.apphosting.executor.Task.TaskRange(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskRange) {
return mergeFrom((com.google.apphosting.executor.Task.TaskRange)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskRange other) {
if (other == com.google.apphosting.executor.Task.TaskRange.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskRange)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskRange)
private static final com.google.apphosting.executor.Task.TaskRange DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskRange();
}
public static com.google.apphosting.executor.Task.TaskRange getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskRange parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRange getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskEtaBorderOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskEtaBorder)
com.google.protobuf.MessageOrBuilder {
/**
* required int64 eta_usec = 1;
* @return Whether the etaUsec field is set.
*/
boolean hasEtaUsec();
/**
* required int64 eta_usec = 1;
* @return The etaUsec.
*/
long getEtaUsec();
/**
* optional bytes name = 2;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional bytes name = 2;
* @return The name.
*/
com.google.protobuf.ByteString getName();
}
/**
*
* Defines a border for paging over the tasks' eta index.
*
*
* Protobuf type {@code java.apphosting.TaskEtaBorder}
*/
public static final class TaskEtaBorder extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskEtaBorder)
TaskEtaBorderOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskEtaBorder.newBuilder() to construct.
private TaskEtaBorder(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskEtaBorder() {
name_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskEtaBorder();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskEtaBorder_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskEtaBorder_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskEtaBorder.class, com.google.apphosting.executor.Task.TaskEtaBorder.Builder.class);
}
private int bitField0_;
public static final int ETA_USEC_FIELD_NUMBER = 1;
private long etaUsec_ = 0L;
/**
* required int64 eta_usec = 1;
* @return Whether the etaUsec field is set.
*/
@java.lang.Override
public boolean hasEtaUsec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required int64 eta_usec = 1;
* @return The etaUsec.
*/
@java.lang.Override
public long getEtaUsec() {
return etaUsec_;
}
public static final int NAME_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes name = 2;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getName() {
return name_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasEtaUsec()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, etaUsec_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, name_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, etaUsec_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, name_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskEtaBorder)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskEtaBorder other = (com.google.apphosting.executor.Task.TaskEtaBorder) obj;
if (hasEtaUsec() != other.hasEtaUsec()) return false;
if (hasEtaUsec()) {
if (getEtaUsec()
!= other.getEtaUsec()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasEtaUsec()) {
hash = (37 * hash) + ETA_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getEtaUsec());
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskEtaBorder parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskEtaBorder prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Defines a border for paging over the tasks' eta index.
*
*
* Protobuf type {@code java.apphosting.TaskEtaBorder}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskEtaBorder)
com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskEtaBorder_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskEtaBorder_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskEtaBorder.class, com.google.apphosting.executor.Task.TaskEtaBorder.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskEtaBorder.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
etaUsec_ = 0L;
name_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskEtaBorder_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskEtaBorder getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskEtaBorder build() {
com.google.apphosting.executor.Task.TaskEtaBorder result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskEtaBorder buildPartial() {
com.google.apphosting.executor.Task.TaskEtaBorder result = new com.google.apphosting.executor.Task.TaskEtaBorder(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskEtaBorder result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.etaUsec_ = etaUsec_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskEtaBorder) {
return mergeFrom((com.google.apphosting.executor.Task.TaskEtaBorder)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskEtaBorder other) {
if (other == com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance()) return this;
if (other.hasEtaUsec()) {
setEtaUsec(other.getEtaUsec());
}
if (other.hasName()) {
setName(other.getName());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasEtaUsec()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
etaUsec_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
name_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long etaUsec_ ;
/**
* required int64 eta_usec = 1;
* @return Whether the etaUsec field is set.
*/
@java.lang.Override
public boolean hasEtaUsec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required int64 eta_usec = 1;
* @return The etaUsec.
*/
@java.lang.Override
public long getEtaUsec() {
return etaUsec_;
}
/**
* required int64 eta_usec = 1;
* @param value The etaUsec to set.
* @return This builder for chaining.
*/
public Builder setEtaUsec(long value) {
etaUsec_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required int64 eta_usec = 1;
* @return This builder for chaining.
*/
public Builder clearEtaUsec() {
bitField0_ = (bitField0_ & ~0x00000001);
etaUsec_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes name = 2;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes name = 2;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getName() {
return name_;
}
/**
* optional bytes name = 2;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional bytes name = 2;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000002);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskEtaBorder)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskEtaBorder)
private static final com.google.apphosting.executor.Task.TaskEtaBorder DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskEtaBorder();
}
public static com.google.apphosting.executor.Task.TaskEtaBorder getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskEtaBorder parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskEtaBorder getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskTagBorderOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskTagBorder)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes tag = 1;
* @return Whether the tag field is set.
*/
boolean hasTag();
/**
* required bytes tag = 1;
* @return The tag.
*/
com.google.protobuf.ByteString getTag();
/**
* optional int64 eta_usec = 2;
* @return Whether the etaUsec field is set.
*/
boolean hasEtaUsec();
/**
* optional int64 eta_usec = 2;
* @return The etaUsec.
*/
long getEtaUsec();
/**
* optional bytes name = 3;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* optional bytes name = 3;
* @return The name.
*/
com.google.protobuf.ByteString getName();
}
/**
*
* Defines a border for paging over the tasks' tag index.
*
*
* Protobuf type {@code java.apphosting.TaskTagBorder}
*/
public static final class TaskTagBorder extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskTagBorder)
TaskTagBorderOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskTagBorder.newBuilder() to construct.
private TaskTagBorder(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskTagBorder() {
tag_ = com.google.protobuf.ByteString.EMPTY;
name_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskTagBorder();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskTagBorder_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskTagBorder_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskTagBorder.class, com.google.apphosting.executor.Task.TaskTagBorder.Builder.class);
}
private int bitField0_;
public static final int TAG_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString tag_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes tag = 1;
* @return Whether the tag field is set.
*/
@java.lang.Override
public boolean hasTag() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes tag = 1;
* @return The tag.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTag() {
return tag_;
}
public static final int ETA_USEC_FIELD_NUMBER = 2;
private long etaUsec_ = 0L;
/**
* optional int64 eta_usec = 2;
* @return Whether the etaUsec field is set.
*/
@java.lang.Override
public boolean hasEtaUsec() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 eta_usec = 2;
* @return The etaUsec.
*/
@java.lang.Override
public long getEtaUsec() {
return etaUsec_;
}
public static final int NAME_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes name = 3;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes name = 3;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getName() {
return name_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTag()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, tag_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, etaUsec_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, name_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, tag_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, etaUsec_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, name_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskTagBorder)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskTagBorder other = (com.google.apphosting.executor.Task.TaskTagBorder) obj;
if (hasTag() != other.hasTag()) return false;
if (hasTag()) {
if (!getTag()
.equals(other.getTag())) return false;
}
if (hasEtaUsec() != other.hasEtaUsec()) return false;
if (hasEtaUsec()) {
if (getEtaUsec()
!= other.getEtaUsec()) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTag()) {
hash = (37 * hash) + TAG_FIELD_NUMBER;
hash = (53 * hash) + getTag().hashCode();
}
if (hasEtaUsec()) {
hash = (37 * hash) + ETA_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getEtaUsec());
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskTagBorder parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskTagBorder prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Defines a border for paging over the tasks' tag index.
*
*
* Protobuf type {@code java.apphosting.TaskTagBorder}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskTagBorder)
com.google.apphosting.executor.Task.TaskTagBorderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskTagBorder_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskTagBorder_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskTagBorder.class, com.google.apphosting.executor.Task.TaskTagBorder.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskTagBorder.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
tag_ = com.google.protobuf.ByteString.EMPTY;
etaUsec_ = 0L;
name_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskTagBorder_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskTagBorder getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskTagBorder build() {
com.google.apphosting.executor.Task.TaskTagBorder result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskTagBorder buildPartial() {
com.google.apphosting.executor.Task.TaskTagBorder result = new com.google.apphosting.executor.Task.TaskTagBorder(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskTagBorder result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.tag_ = tag_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.etaUsec_ = etaUsec_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskTagBorder) {
return mergeFrom((com.google.apphosting.executor.Task.TaskTagBorder)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskTagBorder other) {
if (other == com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance()) return this;
if (other.hasTag()) {
setTag(other.getTag());
}
if (other.hasEtaUsec()) {
setEtaUsec(other.getEtaUsec());
}
if (other.hasName()) {
setName(other.getName());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasTag()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
tag_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
etaUsec_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
name_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString tag_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes tag = 1;
* @return Whether the tag field is set.
*/
@java.lang.Override
public boolean hasTag() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes tag = 1;
* @return The tag.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTag() {
return tag_;
}
/**
* required bytes tag = 1;
* @param value The tag to set.
* @return This builder for chaining.
*/
public Builder setTag(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
tag_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required bytes tag = 1;
* @return This builder for chaining.
*/
public Builder clearTag() {
bitField0_ = (bitField0_ & ~0x00000001);
tag_ = getDefaultInstance().getTag();
onChanged();
return this;
}
private long etaUsec_ ;
/**
* optional int64 eta_usec = 2;
* @return Whether the etaUsec field is set.
*/
@java.lang.Override
public boolean hasEtaUsec() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 eta_usec = 2;
* @return The etaUsec.
*/
@java.lang.Override
public long getEtaUsec() {
return etaUsec_;
}
/**
* optional int64 eta_usec = 2;
* @param value The etaUsec to set.
* @return This builder for chaining.
*/
public Builder setEtaUsec(long value) {
etaUsec_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional int64 eta_usec = 2;
* @return This builder for chaining.
*/
public Builder clearEtaUsec() {
bitField0_ = (bitField0_ & ~0x00000002);
etaUsec_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString name_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes name = 3;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bytes name = 3;
* @return The name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getName() {
return name_;
}
/**
* optional bytes name = 3;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional bytes name = 3;
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000004);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskTagBorder)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskTagBorder)
private static final com.google.apphosting.executor.Task.TaskTagBorder DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskTagBorder();
}
public static com.google.apphosting.executor.Task.TaskTagBorder getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskTagBorder parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskTagBorder getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskRangeByEtaOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskRangeByEta)
com.google.protobuf.MessageOrBuilder {
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
* @return Whether the start field is set.
*/
boolean hasStart();
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
* @return The start.
*/
com.google.apphosting.executor.Task.TaskEtaBorder getStart();
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder getStartOrBuilder();
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
* @return Whether the limit field is set.
*/
boolean hasLimit();
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
* @return The limit.
*/
com.google.apphosting.executor.Task.TaskEtaBorder getLimit();
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder getLimitOrBuilder();
}
/**
*
* Range of tasks defined by tasks etas, defined within a single queue.
* If both ends are specified, start should be strictly less than limit.
* Note that a TaskRangeByEta is an abstraction that ignores the fact that
* the ETA index is sharded. When converting a TaskRangeByEta into concrete
* Bigtable row names, a single TaskRangeByEta will possibly generate multiple
* row ranges if the queue has multiple index shards.
*
*
* Protobuf type {@code java.apphosting.TaskRangeByEta}
*/
public static final class TaskRangeByEta extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskRangeByEta)
TaskRangeByEtaOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskRangeByEta.newBuilder() to construct.
private TaskRangeByEta(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskRangeByEta() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskRangeByEta();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByEta_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByEta_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRangeByEta.class, com.google.apphosting.executor.Task.TaskRangeByEta.Builder.class);
}
private int bitField0_;
public static final int START_FIELD_NUMBER = 1;
private com.google.apphosting.executor.Task.TaskEtaBorder start_;
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
* @return Whether the start field is set.
*/
@java.lang.Override
public boolean hasStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
* @return The start.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskEtaBorder getStart() {
return start_ == null ? com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance() : start_;
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder getStartOrBuilder() {
return start_ == null ? com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance() : start_;
}
public static final int LIMIT_FIELD_NUMBER = 2;
private com.google.apphosting.executor.Task.TaskEtaBorder limit_;
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
* @return Whether the limit field is set.
*/
@java.lang.Override
public boolean hasLimit() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
* @return The limit.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskEtaBorder getLimit() {
return limit_ == null ? com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance() : limit_;
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder getLimitOrBuilder() {
return limit_ == null ? com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance() : limit_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasStart()) {
if (!getStart().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasLimit()) {
if (!getLimit().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getStart());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getLimit());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStart());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getLimit());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskRangeByEta)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskRangeByEta other = (com.google.apphosting.executor.Task.TaskRangeByEta) obj;
if (hasStart() != other.hasStart()) return false;
if (hasStart()) {
if (!getStart()
.equals(other.getStart())) return false;
}
if (hasLimit() != other.hasLimit()) return false;
if (hasLimit()) {
if (!getLimit()
.equals(other.getLimit())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStart()) {
hash = (37 * hash) + START_FIELD_NUMBER;
hash = (53 * hash) + getStart().hashCode();
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByEta parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskRangeByEta prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Range of tasks defined by tasks etas, defined within a single queue.
* If both ends are specified, start should be strictly less than limit.
* Note that a TaskRangeByEta is an abstraction that ignores the fact that
* the ETA index is sharded. When converting a TaskRangeByEta into concrete
* Bigtable row names, a single TaskRangeByEta will possibly generate multiple
* row ranges if the queue has multiple index shards.
*
*
* Protobuf type {@code java.apphosting.TaskRangeByEta}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskRangeByEta)
com.google.apphosting.executor.Task.TaskRangeByEtaOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByEta_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByEta_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRangeByEta.class, com.google.apphosting.executor.Task.TaskRangeByEta.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskRangeByEta.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStartFieldBuilder();
getLimitFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
start_ = null;
if (startBuilder_ != null) {
startBuilder_.dispose();
startBuilder_ = null;
}
limit_ = null;
if (limitBuilder_ != null) {
limitBuilder_.dispose();
limitBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByEta_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByEta getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskRangeByEta.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByEta build() {
com.google.apphosting.executor.Task.TaskRangeByEta result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByEta buildPartial() {
com.google.apphosting.executor.Task.TaskRangeByEta result = new com.google.apphosting.executor.Task.TaskRangeByEta(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskRangeByEta result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.start_ = startBuilder_ == null
? start_
: startBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.limit_ = limitBuilder_ == null
? limit_
: limitBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskRangeByEta) {
return mergeFrom((com.google.apphosting.executor.Task.TaskRangeByEta)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskRangeByEta other) {
if (other == com.google.apphosting.executor.Task.TaskRangeByEta.getDefaultInstance()) return this;
if (other.hasStart()) {
mergeStart(other.getStart());
}
if (other.hasLimit()) {
mergeLimit(other.getLimit());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasStart()) {
if (!getStart().isInitialized()) {
return false;
}
}
if (hasLimit()) {
if (!getLimit().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getStartFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getLimitFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.apphosting.executor.Task.TaskEtaBorder start_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskEtaBorder, com.google.apphosting.executor.Task.TaskEtaBorder.Builder, com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder> startBuilder_;
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
* @return Whether the start field is set.
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
* @return The start.
*/
public com.google.apphosting.executor.Task.TaskEtaBorder getStart() {
if (startBuilder_ == null) {
return start_ == null ? com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance() : start_;
} else {
return startBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
public Builder setStart(com.google.apphosting.executor.Task.TaskEtaBorder value) {
if (startBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
start_ = value;
} else {
startBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
public Builder setStart(
com.google.apphosting.executor.Task.TaskEtaBorder.Builder builderForValue) {
if (startBuilder_ == null) {
start_ = builderForValue.build();
} else {
startBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
public Builder mergeStart(com.google.apphosting.executor.Task.TaskEtaBorder value) {
if (startBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
start_ != null &&
start_ != com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance()) {
getStartBuilder().mergeFrom(value);
} else {
start_ = value;
}
} else {
startBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
public Builder clearStart() {
bitField0_ = (bitField0_ & ~0x00000001);
start_ = null;
if (startBuilder_ != null) {
startBuilder_.dispose();
startBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
public com.google.apphosting.executor.Task.TaskEtaBorder.Builder getStartBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getStartFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
public com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder getStartOrBuilder() {
if (startBuilder_ != null) {
return startBuilder_.getMessageOrBuilder();
} else {
return start_ == null ?
com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance() : start_;
}
}
/**
* optional .java.apphosting.TaskEtaBorder start = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskEtaBorder, com.google.apphosting.executor.Task.TaskEtaBorder.Builder, com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder>
getStartFieldBuilder() {
if (startBuilder_ == null) {
startBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskEtaBorder, com.google.apphosting.executor.Task.TaskEtaBorder.Builder, com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder>(
getStart(),
getParentForChildren(),
isClean());
start_ = null;
}
return startBuilder_;
}
private com.google.apphosting.executor.Task.TaskEtaBorder limit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskEtaBorder, com.google.apphosting.executor.Task.TaskEtaBorder.Builder, com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder> limitBuilder_;
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
* @return Whether the limit field is set.
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
* @return The limit.
*/
public com.google.apphosting.executor.Task.TaskEtaBorder getLimit() {
if (limitBuilder_ == null) {
return limit_ == null ? com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance() : limit_;
} else {
return limitBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
public Builder setLimit(com.google.apphosting.executor.Task.TaskEtaBorder value) {
if (limitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
limit_ = value;
} else {
limitBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
public Builder setLimit(
com.google.apphosting.executor.Task.TaskEtaBorder.Builder builderForValue) {
if (limitBuilder_ == null) {
limit_ = builderForValue.build();
} else {
limitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
public Builder mergeLimit(com.google.apphosting.executor.Task.TaskEtaBorder value) {
if (limitBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
limit_ != null &&
limit_ != com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance()) {
getLimitBuilder().mergeFrom(value);
} else {
limit_ = value;
}
} else {
limitBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000002);
limit_ = null;
if (limitBuilder_ != null) {
limitBuilder_.dispose();
limitBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
public com.google.apphosting.executor.Task.TaskEtaBorder.Builder getLimitBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getLimitFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
public com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder getLimitOrBuilder() {
if (limitBuilder_ != null) {
return limitBuilder_.getMessageOrBuilder();
} else {
return limit_ == null ?
com.google.apphosting.executor.Task.TaskEtaBorder.getDefaultInstance() : limit_;
}
}
/**
* optional .java.apphosting.TaskEtaBorder limit = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskEtaBorder, com.google.apphosting.executor.Task.TaskEtaBorder.Builder, com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder>
getLimitFieldBuilder() {
if (limitBuilder_ == null) {
limitBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskEtaBorder, com.google.apphosting.executor.Task.TaskEtaBorder.Builder, com.google.apphosting.executor.Task.TaskEtaBorderOrBuilder>(
getLimit(),
getParentForChildren(),
isClean());
limit_ = null;
}
return limitBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskRangeByEta)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskRangeByEta)
private static final com.google.apphosting.executor.Task.TaskRangeByEta DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskRangeByEta();
}
public static com.google.apphosting.executor.Task.TaskRangeByEta getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskRangeByEta parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByEta getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskRangeByNameOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskRangeByName)
com.google.protobuf.MessageOrBuilder {
/**
* optional bytes start = 1;
* @return Whether the start field is set.
*/
boolean hasStart();
/**
* optional bytes start = 1;
* @return The start.
*/
com.google.protobuf.ByteString getStart();
/**
* optional bytes limit = 2;
* @return Whether the limit field is set.
*/
boolean hasLimit();
/**
* optional bytes limit = 2;
* @return The limit.
*/
com.google.protobuf.ByteString getLimit();
}
/**
*
* Range of tasks defined by tasks names, defined within a single queue.
* If both ends are specified, start should be strictly less than limit.
*
*
* Protobuf type {@code java.apphosting.TaskRangeByName}
*/
public static final class TaskRangeByName extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskRangeByName)
TaskRangeByNameOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskRangeByName.newBuilder() to construct.
private TaskRangeByName(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskRangeByName() {
start_ = com.google.protobuf.ByteString.EMPTY;
limit_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskRangeByName();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByName_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByName_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRangeByName.class, com.google.apphosting.executor.Task.TaskRangeByName.Builder.class);
}
private int bitField0_;
public static final int START_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString start_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes start = 1;
* @return Whether the start field is set.
*/
@java.lang.Override
public boolean hasStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes start = 1;
* @return The start.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStart() {
return start_;
}
public static final int LIMIT_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString limit_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes limit = 2;
* @return Whether the limit field is set.
*/
@java.lang.Override
public boolean hasLimit() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes limit = 2;
* @return The limit.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLimit() {
return limit_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, start_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, limit_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, start_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, limit_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskRangeByName)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskRangeByName other = (com.google.apphosting.executor.Task.TaskRangeByName) obj;
if (hasStart() != other.hasStart()) return false;
if (hasStart()) {
if (!getStart()
.equals(other.getStart())) return false;
}
if (hasLimit() != other.hasLimit()) return false;
if (hasLimit()) {
if (!getLimit()
.equals(other.getLimit())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStart()) {
hash = (37 * hash) + START_FIELD_NUMBER;
hash = (53 * hash) + getStart().hashCode();
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByName parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskRangeByName prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Range of tasks defined by tasks names, defined within a single queue.
* If both ends are specified, start should be strictly less than limit.
*
*
* Protobuf type {@code java.apphosting.TaskRangeByName}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskRangeByName)
com.google.apphosting.executor.Task.TaskRangeByNameOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByName_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByName_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRangeByName.class, com.google.apphosting.executor.Task.TaskRangeByName.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskRangeByName.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
start_ = com.google.protobuf.ByteString.EMPTY;
limit_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByName_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByName getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskRangeByName.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByName build() {
com.google.apphosting.executor.Task.TaskRangeByName result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByName buildPartial() {
com.google.apphosting.executor.Task.TaskRangeByName result = new com.google.apphosting.executor.Task.TaskRangeByName(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskRangeByName result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.start_ = start_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.limit_ = limit_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskRangeByName) {
return mergeFrom((com.google.apphosting.executor.Task.TaskRangeByName)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskRangeByName other) {
if (other == com.google.apphosting.executor.Task.TaskRangeByName.getDefaultInstance()) return this;
if (other.hasStart()) {
setStart(other.getStart());
}
if (other.hasLimit()) {
setLimit(other.getLimit());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
start_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
limit_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString start_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes start = 1;
* @return Whether the start field is set.
*/
@java.lang.Override
public boolean hasStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bytes start = 1;
* @return The start.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStart() {
return start_;
}
/**
* optional bytes start = 1;
* @param value The start to set.
* @return This builder for chaining.
*/
public Builder setStart(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
start_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional bytes start = 1;
* @return This builder for chaining.
*/
public Builder clearStart() {
bitField0_ = (bitField0_ & ~0x00000001);
start_ = getDefaultInstance().getStart();
onChanged();
return this;
}
private com.google.protobuf.ByteString limit_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes limit = 2;
* @return Whether the limit field is set.
*/
@java.lang.Override
public boolean hasLimit() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes limit = 2;
* @return The limit.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLimit() {
return limit_;
}
/**
* optional bytes limit = 2;
* @param value The limit to set.
* @return This builder for chaining.
*/
public Builder setLimit(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
limit_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional bytes limit = 2;
* @return This builder for chaining.
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000002);
limit_ = getDefaultInstance().getLimit();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskRangeByName)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskRangeByName)
private static final com.google.apphosting.executor.Task.TaskRangeByName DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskRangeByName();
}
public static com.google.apphosting.executor.Task.TaskRangeByName getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskRangeByName parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByName getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskRangeByTagOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskRangeByTag)
com.google.protobuf.MessageOrBuilder {
/**
* optional .java.apphosting.TaskTagBorder start = 1;
* @return Whether the start field is set.
*/
boolean hasStart();
/**
* optional .java.apphosting.TaskTagBorder start = 1;
* @return The start.
*/
com.google.apphosting.executor.Task.TaskTagBorder getStart();
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
com.google.apphosting.executor.Task.TaskTagBorderOrBuilder getStartOrBuilder();
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
* @return Whether the limit field is set.
*/
boolean hasLimit();
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
* @return The limit.
*/
com.google.apphosting.executor.Task.TaskTagBorder getLimit();
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
com.google.apphosting.executor.Task.TaskTagBorderOrBuilder getLimitOrBuilder();
}
/**
*
* Range of tasks defined by tasks tags, defined within a single queue.
* If both ends are specified, start should be strictly less than limit.
* Note that a TaskRangeByTag is an abstraction that ignores the fact that
* the Tag index is sharded. When converting a TaskRangeByTag into concrete
* Bigtable row names, a single TaskRangeByTag will possibly generate multiple
* row ranges if the queue has multiple index shards.
*
*
* Protobuf type {@code java.apphosting.TaskRangeByTag}
*/
public static final class TaskRangeByTag extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskRangeByTag)
TaskRangeByTagOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskRangeByTag.newBuilder() to construct.
private TaskRangeByTag(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskRangeByTag() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskRangeByTag();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByTag_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByTag_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRangeByTag.class, com.google.apphosting.executor.Task.TaskRangeByTag.Builder.class);
}
private int bitField0_;
public static final int START_FIELD_NUMBER = 1;
private com.google.apphosting.executor.Task.TaskTagBorder start_;
/**
* optional .java.apphosting.TaskTagBorder start = 1;
* @return Whether the start field is set.
*/
@java.lang.Override
public boolean hasStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
* @return The start.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskTagBorder getStart() {
return start_ == null ? com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance() : start_;
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskTagBorderOrBuilder getStartOrBuilder() {
return start_ == null ? com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance() : start_;
}
public static final int LIMIT_FIELD_NUMBER = 2;
private com.google.apphosting.executor.Task.TaskTagBorder limit_;
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
* @return Whether the limit field is set.
*/
@java.lang.Override
public boolean hasLimit() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
* @return The limit.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskTagBorder getLimit() {
return limit_ == null ? com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance() : limit_;
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskTagBorderOrBuilder getLimitOrBuilder() {
return limit_ == null ? com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance() : limit_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasStart()) {
if (!getStart().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasLimit()) {
if (!getLimit().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getStart());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getLimit());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStart());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getLimit());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskRangeByTag)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskRangeByTag other = (com.google.apphosting.executor.Task.TaskRangeByTag) obj;
if (hasStart() != other.hasStart()) return false;
if (hasStart()) {
if (!getStart()
.equals(other.getStart())) return false;
}
if (hasLimit() != other.hasLimit()) return false;
if (hasLimit()) {
if (!getLimit()
.equals(other.getLimit())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStart()) {
hash = (37 * hash) + START_FIELD_NUMBER;
hash = (53 * hash) + getStart().hashCode();
}
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRangeByTag parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskRangeByTag prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Range of tasks defined by tasks tags, defined within a single queue.
* If both ends are specified, start should be strictly less than limit.
* Note that a TaskRangeByTag is an abstraction that ignores the fact that
* the Tag index is sharded. When converting a TaskRangeByTag into concrete
* Bigtable row names, a single TaskRangeByTag will possibly generate multiple
* row ranges if the queue has multiple index shards.
*
*
* Protobuf type {@code java.apphosting.TaskRangeByTag}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskRangeByTag)
com.google.apphosting.executor.Task.TaskRangeByTagOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByTag_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByTag_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRangeByTag.class, com.google.apphosting.executor.Task.TaskRangeByTag.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskRangeByTag.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStartFieldBuilder();
getLimitFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
start_ = null;
if (startBuilder_ != null) {
startBuilder_.dispose();
startBuilder_ = null;
}
limit_ = null;
if (limitBuilder_ != null) {
limitBuilder_.dispose();
limitBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRangeByTag_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByTag getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskRangeByTag.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByTag build() {
com.google.apphosting.executor.Task.TaskRangeByTag result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByTag buildPartial() {
com.google.apphosting.executor.Task.TaskRangeByTag result = new com.google.apphosting.executor.Task.TaskRangeByTag(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskRangeByTag result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.start_ = startBuilder_ == null
? start_
: startBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.limit_ = limitBuilder_ == null
? limit_
: limitBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskRangeByTag) {
return mergeFrom((com.google.apphosting.executor.Task.TaskRangeByTag)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskRangeByTag other) {
if (other == com.google.apphosting.executor.Task.TaskRangeByTag.getDefaultInstance()) return this;
if (other.hasStart()) {
mergeStart(other.getStart());
}
if (other.hasLimit()) {
mergeLimit(other.getLimit());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasStart()) {
if (!getStart().isInitialized()) {
return false;
}
}
if (hasLimit()) {
if (!getLimit().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getStartFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getLimitFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.apphosting.executor.Task.TaskTagBorder start_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskTagBorder, com.google.apphosting.executor.Task.TaskTagBorder.Builder, com.google.apphosting.executor.Task.TaskTagBorderOrBuilder> startBuilder_;
/**
* optional .java.apphosting.TaskTagBorder start = 1;
* @return Whether the start field is set.
*/
public boolean hasStart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
* @return The start.
*/
public com.google.apphosting.executor.Task.TaskTagBorder getStart() {
if (startBuilder_ == null) {
return start_ == null ? com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance() : start_;
} else {
return startBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
public Builder setStart(com.google.apphosting.executor.Task.TaskTagBorder value) {
if (startBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
start_ = value;
} else {
startBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
public Builder setStart(
com.google.apphosting.executor.Task.TaskTagBorder.Builder builderForValue) {
if (startBuilder_ == null) {
start_ = builderForValue.build();
} else {
startBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
public Builder mergeStart(com.google.apphosting.executor.Task.TaskTagBorder value) {
if (startBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
start_ != null &&
start_ != com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance()) {
getStartBuilder().mergeFrom(value);
} else {
start_ = value;
}
} else {
startBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
public Builder clearStart() {
bitField0_ = (bitField0_ & ~0x00000001);
start_ = null;
if (startBuilder_ != null) {
startBuilder_.dispose();
startBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
public com.google.apphosting.executor.Task.TaskTagBorder.Builder getStartBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getStartFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
public com.google.apphosting.executor.Task.TaskTagBorderOrBuilder getStartOrBuilder() {
if (startBuilder_ != null) {
return startBuilder_.getMessageOrBuilder();
} else {
return start_ == null ?
com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance() : start_;
}
}
/**
* optional .java.apphosting.TaskTagBorder start = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskTagBorder, com.google.apphosting.executor.Task.TaskTagBorder.Builder, com.google.apphosting.executor.Task.TaskTagBorderOrBuilder>
getStartFieldBuilder() {
if (startBuilder_ == null) {
startBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskTagBorder, com.google.apphosting.executor.Task.TaskTagBorder.Builder, com.google.apphosting.executor.Task.TaskTagBorderOrBuilder>(
getStart(),
getParentForChildren(),
isClean());
start_ = null;
}
return startBuilder_;
}
private com.google.apphosting.executor.Task.TaskTagBorder limit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskTagBorder, com.google.apphosting.executor.Task.TaskTagBorder.Builder, com.google.apphosting.executor.Task.TaskTagBorderOrBuilder> limitBuilder_;
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
* @return Whether the limit field is set.
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
* @return The limit.
*/
public com.google.apphosting.executor.Task.TaskTagBorder getLimit() {
if (limitBuilder_ == null) {
return limit_ == null ? com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance() : limit_;
} else {
return limitBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
public Builder setLimit(com.google.apphosting.executor.Task.TaskTagBorder value) {
if (limitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
limit_ = value;
} else {
limitBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
public Builder setLimit(
com.google.apphosting.executor.Task.TaskTagBorder.Builder builderForValue) {
if (limitBuilder_ == null) {
limit_ = builderForValue.build();
} else {
limitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
public Builder mergeLimit(com.google.apphosting.executor.Task.TaskTagBorder value) {
if (limitBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
limit_ != null &&
limit_ != com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance()) {
getLimitBuilder().mergeFrom(value);
} else {
limit_ = value;
}
} else {
limitBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000002);
limit_ = null;
if (limitBuilder_ != null) {
limitBuilder_.dispose();
limitBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
public com.google.apphosting.executor.Task.TaskTagBorder.Builder getLimitBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getLimitFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
public com.google.apphosting.executor.Task.TaskTagBorderOrBuilder getLimitOrBuilder() {
if (limitBuilder_ != null) {
return limitBuilder_.getMessageOrBuilder();
} else {
return limit_ == null ?
com.google.apphosting.executor.Task.TaskTagBorder.getDefaultInstance() : limit_;
}
}
/**
* optional .java.apphosting.TaskTagBorder limit = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskTagBorder, com.google.apphosting.executor.Task.TaskTagBorder.Builder, com.google.apphosting.executor.Task.TaskTagBorderOrBuilder>
getLimitFieldBuilder() {
if (limitBuilder_ == null) {
limitBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskTagBorder, com.google.apphosting.executor.Task.TaskTagBorder.Builder, com.google.apphosting.executor.Task.TaskTagBorderOrBuilder>(
getLimit(),
getParentForChildren(),
isClean());
limit_ = null;
}
return limitBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskRangeByTag)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskRangeByTag)
private static final com.google.apphosting.executor.Task.TaskRangeByTag DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskRangeByTag();
}
public static com.google.apphosting.executor.Task.TaskRangeByTag getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskRangeByTag parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRangeByTag getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskRunnerInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskRunnerInfo)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* Generic task execution tracking information.
* The purpose is to help us/users with debugging of failing tasks,
* so we save the relevant info in bigtable.
*
*
* Protobuf type {@code java.apphosting.TaskRunnerInfo}
*/
public static final class TaskRunnerInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskRunnerInfo)
TaskRunnerInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskRunnerInfo.newBuilder() to construct.
private TaskRunnerInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskRunnerInfo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskRunnerInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRunnerInfo.class, com.google.apphosting.executor.Task.TaskRunnerInfo.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeAsMessageSetTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSizeAsMessageSet();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskRunnerInfo)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskRunnerInfo other = (com.google.apphosting.executor.Task.TaskRunnerInfo) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskRunnerInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Generic task execution tracking information.
* The purpose is to help us/users with debugging of failing tasks,
* so we save the relevant info in bigtable.
*
*
* Protobuf type {@code java.apphosting.TaskRunnerInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskRunnerInfo)
com.google.apphosting.executor.Task.TaskRunnerInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRunnerInfo.class, com.google.apphosting.executor.Task.TaskRunnerInfo.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskRunnerInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerInfo_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerInfo getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskRunnerInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerInfo build() {
com.google.apphosting.executor.Task.TaskRunnerInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerInfo buildPartial() {
com.google.apphosting.executor.Task.TaskRunnerInfo result = new com.google.apphosting.executor.Task.TaskRunnerInfo(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskRunnerInfo) {
return mergeFrom((com.google.apphosting.executor.Task.TaskRunnerInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskRunnerInfo other) {
if (other == com.google.apphosting.executor.Task.TaskRunnerInfo.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskRunnerInfo)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskRunnerInfo)
private static final com.google.apphosting.executor.Task.TaskRunnerInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskRunnerInfo();
}
public static com.google.apphosting.executor.Task.TaskRunnerInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskRunnerInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HttpTaskRunnerInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.HttpTaskRunnerInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Http response code received from the task acceptor.
*
*
* required int64 response_code = 1;
* @return Whether the responseCode field is set.
*/
boolean hasResponseCode();
/**
*
* Http response code received from the task acceptor.
*
*
* required int64 response_code = 1;
* @return The responseCode.
*/
long getResponseCode();
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return Whether the retryReason field is set.
*/
boolean hasRetryReason();
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return The retryReason.
*/
java.lang.String getRetryReason();
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return The bytes for retryReason.
*/
com.google.protobuf.ByteString
getRetryReasonBytes();
}
/**
*
* Statistics for HttpTaskRunner.
*
*
* Protobuf type {@code java.apphosting.HttpTaskRunnerInfo}
*/
public static final class HttpTaskRunnerInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.HttpTaskRunnerInfo)
HttpTaskRunnerInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use HttpTaskRunnerInfo.newBuilder() to construct.
private HttpTaskRunnerInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HttpTaskRunnerInfo() {
retryReason_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HttpTaskRunnerInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.HttpTaskRunnerInfo.class, com.google.apphosting.executor.Task.HttpTaskRunnerInfo.Builder.class);
}
private int bitField0_;
public static final int RESPONSE_CODE_FIELD_NUMBER = 1;
private long responseCode_ = 0L;
/**
*
* Http response code received from the task acceptor.
*
*
* required int64 response_code = 1;
* @return Whether the responseCode field is set.
*/
@java.lang.Override
public boolean hasResponseCode() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Http response code received from the task acceptor.
*
*
* required int64 response_code = 1;
* @return The responseCode.
*/
@java.lang.Override
public long getResponseCode() {
return responseCode_;
}
public static final int RETRY_REASON_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object retryReason_ = "";
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return Whether the retryReason field is set.
*/
@java.lang.Override
public boolean hasRetryReason() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return The retryReason.
*/
@java.lang.Override
public java.lang.String getRetryReason() {
java.lang.Object ref = retryReason_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
retryReason_ = s;
}
return s;
}
}
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return The bytes for retryReason.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRetryReasonBytes() {
java.lang.Object ref = retryReason_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
retryReason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasResponseCode()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, responseCode_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, retryReason_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, responseCode_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, retryReason_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.HttpTaskRunnerInfo)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.HttpTaskRunnerInfo other = (com.google.apphosting.executor.Task.HttpTaskRunnerInfo) obj;
if (hasResponseCode() != other.hasResponseCode()) return false;
if (hasResponseCode()) {
if (getResponseCode()
!= other.getResponseCode()) return false;
}
if (hasRetryReason() != other.hasRetryReason()) return false;
if (hasRetryReason()) {
if (!getRetryReason()
.equals(other.getRetryReason())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasResponseCode()) {
hash = (37 * hash) + RESPONSE_CODE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getResponseCode());
}
if (hasRetryReason()) {
hash = (37 * hash) + RETRY_REASON_FIELD_NUMBER;
hash = (53 * hash) + getRetryReason().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.HttpTaskRunnerInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Statistics for HttpTaskRunner.
*
*
* Protobuf type {@code java.apphosting.HttpTaskRunnerInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.HttpTaskRunnerInfo)
com.google.apphosting.executor.Task.HttpTaskRunnerInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.HttpTaskRunnerInfo.class, com.google.apphosting.executor.Task.HttpTaskRunnerInfo.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.HttpTaskRunnerInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
responseCode_ = 0L;
retryReason_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerInfo_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerInfo getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.HttpTaskRunnerInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerInfo build() {
com.google.apphosting.executor.Task.HttpTaskRunnerInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerInfo buildPartial() {
com.google.apphosting.executor.Task.HttpTaskRunnerInfo result = new com.google.apphosting.executor.Task.HttpTaskRunnerInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.HttpTaskRunnerInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.responseCode_ = responseCode_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.retryReason_ = retryReason_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.HttpTaskRunnerInfo) {
return mergeFrom((com.google.apphosting.executor.Task.HttpTaskRunnerInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.HttpTaskRunnerInfo other) {
if (other == com.google.apphosting.executor.Task.HttpTaskRunnerInfo.getDefaultInstance()) return this;
if (other.hasResponseCode()) {
setResponseCode(other.getResponseCode());
}
if (other.hasRetryReason()) {
retryReason_ = other.retryReason_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasResponseCode()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
responseCode_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
retryReason_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long responseCode_ ;
/**
*
* Http response code received from the task acceptor.
*
*
* required int64 response_code = 1;
* @return Whether the responseCode field is set.
*/
@java.lang.Override
public boolean hasResponseCode() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Http response code received from the task acceptor.
*
*
* required int64 response_code = 1;
* @return The responseCode.
*/
@java.lang.Override
public long getResponseCode() {
return responseCode_;
}
/**
*
* Http response code received from the task acceptor.
*
*
* required int64 response_code = 1;
* @param value The responseCode to set.
* @return This builder for chaining.
*/
public Builder setResponseCode(long value) {
responseCode_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Http response code received from the task acceptor.
*
*
* required int64 response_code = 1;
* @return This builder for chaining.
*/
public Builder clearResponseCode() {
bitField0_ = (bitField0_ & ~0x00000001);
responseCode_ = 0L;
onChanged();
return this;
}
private java.lang.Object retryReason_ = "";
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return Whether the retryReason field is set.
*/
public boolean hasRetryReason() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return The retryReason.
*/
public java.lang.String getRetryReason() {
java.lang.Object ref = retryReason_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
retryReason_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return The bytes for retryReason.
*/
public com.google.protobuf.ByteString
getRetryReasonBytes() {
java.lang.Object ref = retryReason_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
retryReason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @param value The retryReason to set.
* @return This builder for chaining.
*/
public Builder setRetryReason(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
retryReason_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @return This builder for chaining.
*/
public Builder clearRetryReason() {
retryReason_ = getDefaultInstance().getRetryReason();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Reason for retrying the task
*
*
* optional string retry_reason = 2;
* @param value The bytes for retryReason to set.
* @return This builder for chaining.
*/
public Builder setRetryReasonBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
retryReason_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.HttpTaskRunnerInfo)
}
// @@protoc_insertion_point(class_scope:java.apphosting.HttpTaskRunnerInfo)
private static final com.google.apphosting.executor.Task.HttpTaskRunnerInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.HttpTaskRunnerInfo();
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HttpTaskRunnerInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PubsubTaskRunnerInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.PubsubTaskRunnerInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* RPC status.
*
*
* optional int32 status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
*
* RPC status.
*
*
* optional int32 status = 1;
* @return The status.
*/
int getStatus();
}
/**
*
* Statistics for PubsubTaskRunner.
*
*
* Protobuf type {@code java.apphosting.PubsubTaskRunnerInfo}
*/
public static final class PubsubTaskRunnerInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.PubsubTaskRunnerInfo)
PubsubTaskRunnerInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use PubsubTaskRunnerInfo.newBuilder() to construct.
private PubsubTaskRunnerInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PubsubTaskRunnerInfo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PubsubTaskRunnerInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.PubsubTaskRunnerInfo.class, com.google.apphosting.executor.Task.PubsubTaskRunnerInfo.Builder.class);
}
private int bitField0_;
public static final int STATUS_FIELD_NUMBER = 1;
private int status_ = 0;
/**
*
* RPC status.
*
*
* optional int32 status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* RPC status.
*
*
* optional int32 status = 1;
* @return The status.
*/
@java.lang.Override
public int getStatus() {
return status_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, status_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, status_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.PubsubTaskRunnerInfo)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.PubsubTaskRunnerInfo other = (com.google.apphosting.executor.Task.PubsubTaskRunnerInfo) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (getStatus()
!= other.getStatus()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.PubsubTaskRunnerInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Statistics for PubsubTaskRunner.
*
*
* Protobuf type {@code java.apphosting.PubsubTaskRunnerInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.PubsubTaskRunnerInfo)
com.google.apphosting.executor.Task.PubsubTaskRunnerInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.PubsubTaskRunnerInfo.class, com.google.apphosting.executor.Task.PubsubTaskRunnerInfo.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.PubsubTaskRunnerInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
status_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerInfo_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PubsubTaskRunnerInfo getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.PubsubTaskRunnerInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.PubsubTaskRunnerInfo build() {
com.google.apphosting.executor.Task.PubsubTaskRunnerInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PubsubTaskRunnerInfo buildPartial() {
com.google.apphosting.executor.Task.PubsubTaskRunnerInfo result = new com.google.apphosting.executor.Task.PubsubTaskRunnerInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.PubsubTaskRunnerInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.status_ = status_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.PubsubTaskRunnerInfo) {
return mergeFrom((com.google.apphosting.executor.Task.PubsubTaskRunnerInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.PubsubTaskRunnerInfo other) {
if (other == com.google.apphosting.executor.Task.PubsubTaskRunnerInfo.getDefaultInstance()) return this;
if (other.hasStatus()) {
setStatus(other.getStatus());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
status_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int status_ ;
/**
*
* RPC status.
*
*
* optional int32 status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* RPC status.
*
*
* optional int32 status = 1;
* @return The status.
*/
@java.lang.Override
public int getStatus() {
return status_;
}
/**
*
* RPC status.
*
*
* optional int32 status = 1;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(int value) {
status_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* RPC status.
*
*
* optional int32 status = 1;
* @return This builder for chaining.
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000001);
status_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.PubsubTaskRunnerInfo)
}
// @@protoc_insertion_point(class_scope:java.apphosting.PubsubTaskRunnerInfo)
private static final com.google.apphosting.executor.Task.PubsubTaskRunnerInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.PubsubTaskRunnerInfo();
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PubsubTaskRunnerInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PubsubTaskRunnerInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ExternalHttpTaskRunnerInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.ExternalHttpTaskRunnerInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* Required. Canonical status code corresponding to HTTP response code
* received from the target or status received from internal intermediate.
*
*
* optional int32 status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
*
* Required. Canonical status code corresponding to HTTP response code
* received from the target or status received from internal intermediate.
*
*
* optional int32 status = 1;
* @return The status.
*/
int getStatus();
/**
*
* HTTP response code received from the target.
*
*
* optional int32 http_response_code = 2;
* @return Whether the httpResponseCode field is set.
*/
boolean hasHttpResponseCode();
/**
*
* HTTP response code received from the target.
*
*
* optional int32 http_response_code = 2;
* @return The httpResponseCode.
*/
int getHttpResponseCode();
}
/**
*
* Statistics for ExternalHttpTaskRunner.
*
*
* Protobuf type {@code java.apphosting.ExternalHttpTaskRunnerInfo}
*/
public static final class ExternalHttpTaskRunnerInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.ExternalHttpTaskRunnerInfo)
ExternalHttpTaskRunnerInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExternalHttpTaskRunnerInfo.newBuilder() to construct.
private ExternalHttpTaskRunnerInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExternalHttpTaskRunnerInfo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ExternalHttpTaskRunnerInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo.class, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo.Builder.class);
}
private int bitField0_;
public static final int STATUS_FIELD_NUMBER = 1;
private int status_ = 0;
/**
*
* Required. Canonical status code corresponding to HTTP response code
* received from the target or status received from internal intermediate.
*
*
* optional int32 status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required. Canonical status code corresponding to HTTP response code
* received from the target or status received from internal intermediate.
*
*
* optional int32 status = 1;
* @return The status.
*/
@java.lang.Override
public int getStatus() {
return status_;
}
public static final int HTTP_RESPONSE_CODE_FIELD_NUMBER = 2;
private int httpResponseCode_ = 0;
/**
*
* HTTP response code received from the target.
*
*
* optional int32 http_response_code = 2;
* @return Whether the httpResponseCode field is set.
*/
@java.lang.Override
public boolean hasHttpResponseCode() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* HTTP response code received from the target.
*
*
* optional int32 http_response_code = 2;
* @return The httpResponseCode.
*/
@java.lang.Override
public int getHttpResponseCode() {
return httpResponseCode_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, status_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, httpResponseCode_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, status_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, httpResponseCode_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo other = (com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (getStatus()
!= other.getStatus()) return false;
}
if (hasHttpResponseCode() != other.hasHttpResponseCode()) return false;
if (hasHttpResponseCode()) {
if (getHttpResponseCode()
!= other.getHttpResponseCode()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus();
}
if (hasHttpResponseCode()) {
hash = (37 * hash) + HTTP_RESPONSE_CODE_FIELD_NUMBER;
hash = (53 * hash) + getHttpResponseCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Statistics for ExternalHttpTaskRunner.
*
*
* Protobuf type {@code java.apphosting.ExternalHttpTaskRunnerInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.ExternalHttpTaskRunnerInfo)
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo.class, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
status_ = 0;
httpResponseCode_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo build() {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo buildPartial() {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo result = new com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.status_ = status_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.httpResponseCode_ = httpResponseCode_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo) {
return mergeFrom((com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo other) {
if (other == com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo.getDefaultInstance()) return this;
if (other.hasStatus()) {
setStatus(other.getStatus());
}
if (other.hasHttpResponseCode()) {
setHttpResponseCode(other.getHttpResponseCode());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
status_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
httpResponseCode_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int status_ ;
/**
*
* Required. Canonical status code corresponding to HTTP response code
* received from the target or status received from internal intermediate.
*
*
* optional int32 status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required. Canonical status code corresponding to HTTP response code
* received from the target or status received from internal intermediate.
*
*
* optional int32 status = 1;
* @return The status.
*/
@java.lang.Override
public int getStatus() {
return status_;
}
/**
*
* Required. Canonical status code corresponding to HTTP response code
* received from the target or status received from internal intermediate.
*
*
* optional int32 status = 1;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(int value) {
status_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Required. Canonical status code corresponding to HTTP response code
* received from the target or status received from internal intermediate.
*
*
* optional int32 status = 1;
* @return This builder for chaining.
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000001);
status_ = 0;
onChanged();
return this;
}
private int httpResponseCode_ ;
/**
*
* HTTP response code received from the target.
*
*
* optional int32 http_response_code = 2;
* @return Whether the httpResponseCode field is set.
*/
@java.lang.Override
public boolean hasHttpResponseCode() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* HTTP response code received from the target.
*
*
* optional int32 http_response_code = 2;
* @return The httpResponseCode.
*/
@java.lang.Override
public int getHttpResponseCode() {
return httpResponseCode_;
}
/**
*
* HTTP response code received from the target.
*
*
* optional int32 http_response_code = 2;
* @param value The httpResponseCode to set.
* @return This builder for chaining.
*/
public Builder setHttpResponseCode(int value) {
httpResponseCode_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* HTTP response code received from the target.
*
*
* optional int32 http_response_code = 2;
* @return This builder for chaining.
*/
public Builder clearHttpResponseCode() {
bitField0_ = (bitField0_ & ~0x00000002);
httpResponseCode_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.ExternalHttpTaskRunnerInfo)
}
// @@protoc_insertion_point(class_scope:java.apphosting.ExternalHttpTaskRunnerInfo)
private static final com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo();
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ExternalHttpTaskRunnerInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskRunnerStatsOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskRunnerStats)
com.google.protobuf.MessageOrBuilder {
/**
*
* When this run was started, in microseconds, and relative to
* midnight January 1, 1970 UTC.
*
*
* required int64 dispatched_usec = 1;
* @return Whether the dispatchedUsec field is set.
*/
boolean hasDispatchedUsec();
/**
*
* When this run was started, in microseconds, and relative to
* midnight January 1, 1970 UTC.
*
*
* required int64 dispatched_usec = 1;
* @return The dispatchedUsec.
*/
long getDispatchedUsec();
/**
*
* The latency in microseconds between the task schedule and
* actual dispatch time.
*
*
* required int64 lag_usec = 2;
* @return Whether the lagUsec field is set.
*/
boolean hasLagUsec();
/**
*
* The latency in microseconds between the task schedule and
* actual dispatch time.
*
*
* required int64 lag_usec = 2;
* @return The lagUsec.
*/
long getLagUsec();
/**
*
* The time this run took, in microseconds.
*
*
* required int64 elapsed_usec = 3;
* @return Whether the elapsedUsec field is set.
*/
boolean hasElapsedUsec();
/**
*
* The time this run took, in microseconds.
*
*
* required int64 elapsed_usec = 3;
* @return The elapsedUsec.
*/
long getElapsedUsec();
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
* @return Whether the info field is set.
*/
boolean hasInfo();
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
* @return The info.
*/
com.google.apphosting.executor.Task.TaskRunnerInfo getInfo();
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
com.google.apphosting.executor.Task.TaskRunnerInfoOrBuilder getInfoOrBuilder();
}
/**
*
* Information about an invocation of a task. Used for tracking task's progress.
*
*
* Protobuf type {@code java.apphosting.TaskRunnerStats}
*/
public static final class TaskRunnerStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskRunnerStats)
TaskRunnerStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskRunnerStats.newBuilder() to construct.
private TaskRunnerStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskRunnerStats() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskRunnerStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRunnerStats.class, com.google.apphosting.executor.Task.TaskRunnerStats.Builder.class);
}
private int bitField0_;
public static final int DISPATCHED_USEC_FIELD_NUMBER = 1;
private long dispatchedUsec_ = 0L;
/**
*
* When this run was started, in microseconds, and relative to
* midnight January 1, 1970 UTC.
*
*
* required int64 dispatched_usec = 1;
* @return Whether the dispatchedUsec field is set.
*/
@java.lang.Override
public boolean hasDispatchedUsec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* When this run was started, in microseconds, and relative to
* midnight January 1, 1970 UTC.
*
*
* required int64 dispatched_usec = 1;
* @return The dispatchedUsec.
*/
@java.lang.Override
public long getDispatchedUsec() {
return dispatchedUsec_;
}
public static final int LAG_USEC_FIELD_NUMBER = 2;
private long lagUsec_ = 0L;
/**
*
* The latency in microseconds between the task schedule and
* actual dispatch time.
*
*
* required int64 lag_usec = 2;
* @return Whether the lagUsec field is set.
*/
@java.lang.Override
public boolean hasLagUsec() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The latency in microseconds between the task schedule and
* actual dispatch time.
*
*
* required int64 lag_usec = 2;
* @return The lagUsec.
*/
@java.lang.Override
public long getLagUsec() {
return lagUsec_;
}
public static final int ELAPSED_USEC_FIELD_NUMBER = 3;
private long elapsedUsec_ = 0L;
/**
*
* The time this run took, in microseconds.
*
*
* required int64 elapsed_usec = 3;
* @return Whether the elapsedUsec field is set.
*/
@java.lang.Override
public boolean hasElapsedUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The time this run took, in microseconds.
*
*
* required int64 elapsed_usec = 3;
* @return The elapsedUsec.
*/
@java.lang.Override
public long getElapsedUsec() {
return elapsedUsec_;
}
public static final int INFO_FIELD_NUMBER = 4;
private com.google.apphosting.executor.Task.TaskRunnerInfo info_;
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
* @return Whether the info field is set.
*/
@java.lang.Override
public boolean hasInfo() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
* @return The info.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerInfo getInfo() {
return info_ == null ? com.google.apphosting.executor.Task.TaskRunnerInfo.getDefaultInstance() : info_;
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerInfoOrBuilder getInfoOrBuilder() {
return info_ == null ? com.google.apphosting.executor.Task.TaskRunnerInfo.getDefaultInstance() : info_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasDispatchedUsec()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasLagUsec()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasElapsedUsec()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, dispatchedUsec_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, lagUsec_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(3, elapsedUsec_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(4, getInfo());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, dispatchedUsec_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, lagUsec_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, elapsedUsec_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getInfo());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskRunnerStats)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskRunnerStats other = (com.google.apphosting.executor.Task.TaskRunnerStats) obj;
if (hasDispatchedUsec() != other.hasDispatchedUsec()) return false;
if (hasDispatchedUsec()) {
if (getDispatchedUsec()
!= other.getDispatchedUsec()) return false;
}
if (hasLagUsec() != other.hasLagUsec()) return false;
if (hasLagUsec()) {
if (getLagUsec()
!= other.getLagUsec()) return false;
}
if (hasElapsedUsec() != other.hasElapsedUsec()) return false;
if (hasElapsedUsec()) {
if (getElapsedUsec()
!= other.getElapsedUsec()) return false;
}
if (hasInfo() != other.hasInfo()) return false;
if (hasInfo()) {
if (!getInfo()
.equals(other.getInfo())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDispatchedUsec()) {
hash = (37 * hash) + DISPATCHED_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDispatchedUsec());
}
if (hasLagUsec()) {
hash = (37 * hash) + LAG_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLagUsec());
}
if (hasElapsedUsec()) {
hash = (37 * hash) + ELAPSED_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getElapsedUsec());
}
if (hasInfo()) {
hash = (37 * hash) + INFO_FIELD_NUMBER;
hash = (53 * hash) + getInfo().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskRunnerStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Information about an invocation of a task. Used for tracking task's progress.
*
*
* Protobuf type {@code java.apphosting.TaskRunnerStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskRunnerStats)
com.google.apphosting.executor.Task.TaskRunnerStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRunnerStats.class, com.google.apphosting.executor.Task.TaskRunnerStats.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskRunnerStats.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
dispatchedUsec_ = 0L;
lagUsec_ = 0L;
elapsedUsec_ = 0L;
info_ = null;
if (infoBuilder_ != null) {
infoBuilder_.dispose();
infoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerStats_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerStats getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskRunnerStats.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerStats build() {
com.google.apphosting.executor.Task.TaskRunnerStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerStats buildPartial() {
com.google.apphosting.executor.Task.TaskRunnerStats result = new com.google.apphosting.executor.Task.TaskRunnerStats(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskRunnerStats result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.dispatchedUsec_ = dispatchedUsec_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.lagUsec_ = lagUsec_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.elapsedUsec_ = elapsedUsec_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.info_ = infoBuilder_ == null
? info_
: infoBuilder_.build();
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskRunnerStats) {
return mergeFrom((com.google.apphosting.executor.Task.TaskRunnerStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskRunnerStats other) {
if (other == com.google.apphosting.executor.Task.TaskRunnerStats.getDefaultInstance()) return this;
if (other.hasDispatchedUsec()) {
setDispatchedUsec(other.getDispatchedUsec());
}
if (other.hasLagUsec()) {
setLagUsec(other.getLagUsec());
}
if (other.hasElapsedUsec()) {
setElapsedUsec(other.getElapsedUsec());
}
if (other.hasInfo()) {
mergeInfo(other.getInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasDispatchedUsec()) {
return false;
}
if (!hasLagUsec()) {
return false;
}
if (!hasElapsedUsec()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
dispatchedUsec_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
lagUsec_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
elapsedUsec_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34: {
input.readMessage(
getInfoFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long dispatchedUsec_ ;
/**
*
* When this run was started, in microseconds, and relative to
* midnight January 1, 1970 UTC.
*
*
* required int64 dispatched_usec = 1;
* @return Whether the dispatchedUsec field is set.
*/
@java.lang.Override
public boolean hasDispatchedUsec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* When this run was started, in microseconds, and relative to
* midnight January 1, 1970 UTC.
*
*
* required int64 dispatched_usec = 1;
* @return The dispatchedUsec.
*/
@java.lang.Override
public long getDispatchedUsec() {
return dispatchedUsec_;
}
/**
*
* When this run was started, in microseconds, and relative to
* midnight January 1, 1970 UTC.
*
*
* required int64 dispatched_usec = 1;
* @param value The dispatchedUsec to set.
* @return This builder for chaining.
*/
public Builder setDispatchedUsec(long value) {
dispatchedUsec_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* When this run was started, in microseconds, and relative to
* midnight January 1, 1970 UTC.
*
*
* required int64 dispatched_usec = 1;
* @return This builder for chaining.
*/
public Builder clearDispatchedUsec() {
bitField0_ = (bitField0_ & ~0x00000001);
dispatchedUsec_ = 0L;
onChanged();
return this;
}
private long lagUsec_ ;
/**
*
* The latency in microseconds between the task schedule and
* actual dispatch time.
*
*
* required int64 lag_usec = 2;
* @return Whether the lagUsec field is set.
*/
@java.lang.Override
public boolean hasLagUsec() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The latency in microseconds between the task schedule and
* actual dispatch time.
*
*
* required int64 lag_usec = 2;
* @return The lagUsec.
*/
@java.lang.Override
public long getLagUsec() {
return lagUsec_;
}
/**
*
* The latency in microseconds between the task schedule and
* actual dispatch time.
*
*
* required int64 lag_usec = 2;
* @param value The lagUsec to set.
* @return This builder for chaining.
*/
public Builder setLagUsec(long value) {
lagUsec_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The latency in microseconds between the task schedule and
* actual dispatch time.
*
*
* required int64 lag_usec = 2;
* @return This builder for chaining.
*/
public Builder clearLagUsec() {
bitField0_ = (bitField0_ & ~0x00000002);
lagUsec_ = 0L;
onChanged();
return this;
}
private long elapsedUsec_ ;
/**
*
* The time this run took, in microseconds.
*
*
* required int64 elapsed_usec = 3;
* @return Whether the elapsedUsec field is set.
*/
@java.lang.Override
public boolean hasElapsedUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The time this run took, in microseconds.
*
*
* required int64 elapsed_usec = 3;
* @return The elapsedUsec.
*/
@java.lang.Override
public long getElapsedUsec() {
return elapsedUsec_;
}
/**
*
* The time this run took, in microseconds.
*
*
* required int64 elapsed_usec = 3;
* @param value The elapsedUsec to set.
* @return This builder for chaining.
*/
public Builder setElapsedUsec(long value) {
elapsedUsec_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The time this run took, in microseconds.
*
*
* required int64 elapsed_usec = 3;
* @return This builder for chaining.
*/
public Builder clearElapsedUsec() {
bitField0_ = (bitField0_ & ~0x00000004);
elapsedUsec_ = 0L;
onChanged();
return this;
}
private com.google.apphosting.executor.Task.TaskRunnerInfo info_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerInfo, com.google.apphosting.executor.Task.TaskRunnerInfo.Builder, com.google.apphosting.executor.Task.TaskRunnerInfoOrBuilder> infoBuilder_;
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
* @return Whether the info field is set.
*/
public boolean hasInfo() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
* @return The info.
*/
public com.google.apphosting.executor.Task.TaskRunnerInfo getInfo() {
if (infoBuilder_ == null) {
return info_ == null ? com.google.apphosting.executor.Task.TaskRunnerInfo.getDefaultInstance() : info_;
} else {
return infoBuilder_.getMessage();
}
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
public Builder setInfo(com.google.apphosting.executor.Task.TaskRunnerInfo value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
info_ = value;
} else {
infoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
public Builder setInfo(
com.google.apphosting.executor.Task.TaskRunnerInfo.Builder builderForValue) {
if (infoBuilder_ == null) {
info_ = builderForValue.build();
} else {
infoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
public Builder mergeInfo(com.google.apphosting.executor.Task.TaskRunnerInfo value) {
if (infoBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
info_ != null &&
info_ != com.google.apphosting.executor.Task.TaskRunnerInfo.getDefaultInstance()) {
getInfoBuilder().mergeFrom(value);
} else {
info_ = value;
}
} else {
infoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
public Builder clearInfo() {
bitField0_ = (bitField0_ & ~0x00000008);
info_ = null;
if (infoBuilder_ != null) {
infoBuilder_.dispose();
infoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
public com.google.apphosting.executor.Task.TaskRunnerInfo.Builder getInfoBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getInfoFieldBuilder().getBuilder();
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
public com.google.apphosting.executor.Task.TaskRunnerInfoOrBuilder getInfoOrBuilder() {
if (infoBuilder_ != null) {
return infoBuilder_.getMessageOrBuilder();
} else {
return info_ == null ?
com.google.apphosting.executor.Task.TaskRunnerInfo.getDefaultInstance() : info_;
}
}
/**
*
* Last run details, specific for the task's runner.
*
*
* optional .java.apphosting.TaskRunnerInfo info = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerInfo, com.google.apphosting.executor.Task.TaskRunnerInfo.Builder, com.google.apphosting.executor.Task.TaskRunnerInfoOrBuilder>
getInfoFieldBuilder() {
if (infoBuilder_ == null) {
infoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerInfo, com.google.apphosting.executor.Task.TaskRunnerInfo.Builder, com.google.apphosting.executor.Task.TaskRunnerInfoOrBuilder>(
getInfo(),
getParentForChildren(),
isClean());
info_ = null;
}
return infoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskRunnerStats)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskRunnerStats)
private static final com.google.apphosting.executor.Task.TaskRunnerStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskRunnerStats();
}
public static com.google.apphosting.executor.Task.TaskRunnerStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskRunnerStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskRunnerPayloadOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskRunnerPayload)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* Generic payload type.
*
*
* Protobuf type {@code java.apphosting.TaskRunnerPayload}
*/
public static final class TaskRunnerPayload extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskRunnerPayload)
TaskRunnerPayloadOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskRunnerPayload.newBuilder() to construct.
private TaskRunnerPayload(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskRunnerPayload() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskRunnerPayload();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRunnerPayload.class, com.google.apphosting.executor.Task.TaskRunnerPayload.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeAsMessageSetTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSizeAsMessageSet();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskRunnerPayload)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskRunnerPayload other = (com.google.apphosting.executor.Task.TaskRunnerPayload) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskRunnerPayload prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Generic payload type.
*
*
* Protobuf type {@code java.apphosting.TaskRunnerPayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskRunnerPayload)
com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskRunnerPayload.class, com.google.apphosting.executor.Task.TaskRunnerPayload.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskRunnerPayload.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskRunnerPayload_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerPayload getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerPayload build() {
com.google.apphosting.executor.Task.TaskRunnerPayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerPayload buildPartial() {
com.google.apphosting.executor.Task.TaskRunnerPayload result = new com.google.apphosting.executor.Task.TaskRunnerPayload(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskRunnerPayload) {
return mergeFrom((com.google.apphosting.executor.Task.TaskRunnerPayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskRunnerPayload other) {
if (other == com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskRunnerPayload)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskRunnerPayload)
private static final com.google.apphosting.executor.Task.TaskRunnerPayload DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskRunnerPayload();
}
public static com.google.apphosting.executor.Task.TaskRunnerPayload getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskRunnerPayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerPayload getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HttpTaskRunnerPayloadOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.HttpTaskRunnerPayload)
com.google.protobuf.MessageOrBuilder {
/**
* optional .java.apphosting.HttpTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return Whether the method field is set.
*/
boolean hasMethod();
/**
* optional .java.apphosting.HttpTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return The method.
*/
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod getMethod();
/**
*
* Note that this stores the relative url. The host is derived from the app.
*
*
* required bytes url = 2;
* @return Whether the url field is set.
*/
boolean hasUrl();
/**
*
* Note that this stores the relative url. The host is derived from the app.
*
*
* required bytes url = 2;
* @return The url.
*/
com.google.protobuf.ByteString getUrl();
/**
* repeated group Header = 3 { ... }
*/
java.util.List
getHeaderList();
/**
* repeated group Header = 3 { ... }
*/
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header getHeader(int index);
/**
* repeated group Header = 3 { ... }
*/
int getHeaderCount();
/**
* repeated group Header = 3 { ... }
*/
java.util.List extends com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList();
/**
* repeated group Header = 3 { ... }
*/
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index);
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return Whether the body field is set.
*/
boolean hasBody();
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return The body.
*/
com.google.protobuf.ByteString getBody();
/**
*
* The dispatch_deadline is the time that Executor waits for the target,Adding
* with as few internal hops as possible.
* Note that when the request is cancelled, Cloud Tasks will stop listing for
* the response and will schedule the task to be retried, but whether the
* worker stops processing depends on how App Engine handles cancelled HTTP
* requests. Currently App Engine does not react to cancelled HTTP requests.
* Thus, this parameter controls how long Cloud Tasks will wait to retry the
* task, but it does not affect the work done by the App Engine instances.
*
*
* optional int32 dispatch_deadline_ms = 7;
* @return Whether the dispatchDeadlineMs field is set.
*/
boolean hasDispatchDeadlineMs();
/**
*
* The dispatch_deadline is the time that Executor waits for the target,Adding
* with as few internal hops as possible.
* Note that when the request is cancelled, Cloud Tasks will stop listing for
* the response and will schedule the task to be retried, but whether the
* worker stops processing depends on how App Engine handles cancelled HTTP
* requests. Currently App Engine does not react to cancelled HTTP requests.
* Thus, this parameter controls how long Cloud Tasks will wait to retry the
* task, but it does not affect the work done by the App Engine instances.
*
*
* optional int32 dispatch_deadline_ms = 7;
* @return The dispatchDeadlineMs.
*/
int getDispatchDeadlineMs();
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
* @return Whether the appEngineRouting field is set.
*/
boolean hasAppEngineRouting();
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
* @return The appEngineRouting.
*/
com.google.apphosting.executor.Task.AppEngineRouting getAppEngineRouting();
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
com.google.apphosting.executor.Task.AppEngineRoutingOrBuilder getAppEngineRoutingOrBuilder();
}
/**
*
* Payload type for HttpTaskRunner. This runner pushes to GAE.
*
*
* Protobuf type {@code java.apphosting.HttpTaskRunnerPayload}
*/
public static final class HttpTaskRunnerPayload extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.HttpTaskRunnerPayload)
HttpTaskRunnerPayloadOrBuilder {
private static final long serialVersionUID = 0L;
// Use HttpTaskRunnerPayload.newBuilder() to construct.
private HttpTaskRunnerPayload(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HttpTaskRunnerPayload() {
method_ = 2;
url_ = com.google.protobuf.ByteString.EMPTY;
header_ = java.util.Collections.emptyList();
body_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HttpTaskRunnerPayload();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.class, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.HttpTaskRunnerPayload.RequestMethod}
*/
public enum RequestMethod
implements com.google.protobuf.ProtocolMessageEnum {
/**
* GET = 1;
*/
GET(1),
/**
* POST = 2;
*/
POST(2),
/**
* HEAD = 3;
*/
HEAD(3),
/**
* PUT = 4;
*/
PUT(4),
/**
* DELETE = 5;
*/
DELETE(5),
/**
* PATCH = 6;
*/
PATCH(6),
/**
* OPTIONS = 7;
*/
OPTIONS(7),
;
/**
* GET = 1;
*/
public static final int GET_VALUE = 1;
/**
* POST = 2;
*/
public static final int POST_VALUE = 2;
/**
* HEAD = 3;
*/
public static final int HEAD_VALUE = 3;
/**
* PUT = 4;
*/
public static final int PUT_VALUE = 4;
/**
* DELETE = 5;
*/
public static final int DELETE_VALUE = 5;
/**
* PATCH = 6;
*/
public static final int PATCH_VALUE = 6;
/**
* OPTIONS = 7;
*/
public static final int OPTIONS_VALUE = 7;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RequestMethod valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static RequestMethod forNumber(int value) {
switch (value) {
case 1: return GET;
case 2: return POST;
case 3: return HEAD;
case 4: return PUT;
case 5: return DELETE;
case 6: return PATCH;
case 7: return OPTIONS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
RequestMethod> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RequestMethod findValueByNumber(int number) {
return RequestMethod.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.HttpTaskRunnerPayload.getDescriptor().getEnumTypes().get(0);
}
private static final RequestMethod[] VALUES = values();
public static RequestMethod valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private RequestMethod(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.HttpTaskRunnerPayload.RequestMethod)
}
public interface HeaderOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.HttpTaskRunnerPayload.Header)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes key = 4;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
* required bytes key = 4;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
* required bytes value = 5;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* required bytes value = 5;
* @return The value.
*/
com.google.protobuf.ByteString getValue();
}
/**
* Protobuf type {@code java.apphosting.HttpTaskRunnerPayload.Header}
*/
public static final class Header extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.HttpTaskRunnerPayload.Header)
HeaderOrBuilder {
private static final long serialVersionUID = 0L;
// Use Header.newBuilder() to construct.
private Header(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Header() {
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Header();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_Header_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.class, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 4;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes key = 4;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int VALUE_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes value = 5;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required bytes value = 5;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(4, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(5, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header other = (com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.HttpTaskRunnerPayload.Header}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.HttpTaskRunnerPayload.Header)
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_Header_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.class, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header build() {
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header buildPartial() {
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header result = new com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header) {
return mergeFrom((com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header other) {
if (other == com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 34: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 34
case 42: {
value_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 4;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes key = 4;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* required bytes key = 4;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required bytes key = 4;
* @return This builder for chaining.
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes value = 5;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required bytes value = 5;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* required bytes value = 5;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required bytes value = 5;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.HttpTaskRunnerPayload.Header)
}
// @@protoc_insertion_point(class_scope:java.apphosting.HttpTaskRunnerPayload.Header)
private static final com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header();
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Header parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int METHOD_FIELD_NUMBER = 1;
private int method_ = 2;
/**
* optional .java.apphosting.HttpTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return Whether the method field is set.
*/
@java.lang.Override public boolean hasMethod() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.HttpTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return The method.
*/
@java.lang.Override public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod getMethod() {
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod result = com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod.forNumber(method_);
return result == null ? com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod.POST : result;
}
public static final int URL_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString url_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Note that this stores the relative url. The host is derived from the app.
*
*
* required bytes url = 2;
* @return Whether the url field is set.
*/
@java.lang.Override
public boolean hasUrl() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Note that this stores the relative url. The host is derived from the app.
*
*
* required bytes url = 2;
* @return The url.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUrl() {
return url_;
}
public static final int HEADER_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List header_;
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public java.util.List getHeaderList() {
return header_;
}
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList() {
return header_;
}
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public int getHeaderCount() {
return header_.size();
}
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header getHeader(int index) {
return header_.get(index);
}
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index) {
return header_.get(index);
}
public static final int BODY_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString body_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return Whether the body field is set.
*/
@java.lang.Override
public boolean hasBody() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return The body.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBody() {
return body_;
}
public static final int DISPATCH_DEADLINE_MS_FIELD_NUMBER = 7;
private int dispatchDeadlineMs_ = 0;
/**
*
* The dispatch_deadline is the time that Executor waits for the target,Adding
* with as few internal hops as possible.
* Note that when the request is cancelled, Cloud Tasks will stop listing for
* the response and will schedule the task to be retried, but whether the
* worker stops processing depends on how App Engine handles cancelled HTTP
* requests. Currently App Engine does not react to cancelled HTTP requests.
* Thus, this parameter controls how long Cloud Tasks will wait to retry the
* task, but it does not affect the work done by the App Engine instances.
*
*
* optional int32 dispatch_deadline_ms = 7;
* @return Whether the dispatchDeadlineMs field is set.
*/
@java.lang.Override
public boolean hasDispatchDeadlineMs() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The dispatch_deadline is the time that Executor waits for the target,Adding
* with as few internal hops as possible.
* Note that when the request is cancelled, Cloud Tasks will stop listing for
* the response and will schedule the task to be retried, but whether the
* worker stops processing depends on how App Engine handles cancelled HTTP
* requests. Currently App Engine does not react to cancelled HTTP requests.
* Thus, this parameter controls how long Cloud Tasks will wait to retry the
* task, but it does not affect the work done by the App Engine instances.
*
*
* optional int32 dispatch_deadline_ms = 7;
* @return The dispatchDeadlineMs.
*/
@java.lang.Override
public int getDispatchDeadlineMs() {
return dispatchDeadlineMs_;
}
public static final int APP_ENGINE_ROUTING_FIELD_NUMBER = 8;
private com.google.apphosting.executor.Task.AppEngineRouting appEngineRouting_;
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
* @return Whether the appEngineRouting field is set.
*/
@java.lang.Override
public boolean hasAppEngineRouting() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
* @return The appEngineRouting.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.AppEngineRouting getAppEngineRouting() {
return appEngineRouting_ == null ? com.google.apphosting.executor.Task.AppEngineRouting.getDefaultInstance() : appEngineRouting_;
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.AppEngineRoutingOrBuilder getAppEngineRoutingOrBuilder() {
return appEngineRouting_ == null ? com.google.apphosting.executor.Task.AppEngineRouting.getDefaultInstance() : appEngineRouting_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasUrl()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getHeaderCount(); i++) {
if (!getHeader(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, method_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, url_);
}
for (int i = 0; i < header_.size(); i++) {
output.writeGroup(3, header_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(6, body_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(7, dispatchDeadlineMs_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(8, getAppEngineRouting());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, method_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, url_);
}
for (int i = 0; i < header_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(3, header_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, body_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(7, dispatchDeadlineMs_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getAppEngineRouting());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.HttpTaskRunnerPayload)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.HttpTaskRunnerPayload other = (com.google.apphosting.executor.Task.HttpTaskRunnerPayload) obj;
if (hasMethod() != other.hasMethod()) return false;
if (hasMethod()) {
if (method_ != other.method_) return false;
}
if (hasUrl() != other.hasUrl()) return false;
if (hasUrl()) {
if (!getUrl()
.equals(other.getUrl())) return false;
}
if (!getHeaderList()
.equals(other.getHeaderList())) return false;
if (hasBody() != other.hasBody()) return false;
if (hasBody()) {
if (!getBody()
.equals(other.getBody())) return false;
}
if (hasDispatchDeadlineMs() != other.hasDispatchDeadlineMs()) return false;
if (hasDispatchDeadlineMs()) {
if (getDispatchDeadlineMs()
!= other.getDispatchDeadlineMs()) return false;
}
if (hasAppEngineRouting() != other.hasAppEngineRouting()) return false;
if (hasAppEngineRouting()) {
if (!getAppEngineRouting()
.equals(other.getAppEngineRouting())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMethod()) {
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + method_;
}
if (hasUrl()) {
hash = (37 * hash) + URL_FIELD_NUMBER;
hash = (53 * hash) + getUrl().hashCode();
}
if (getHeaderCount() > 0) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeaderList().hashCode();
}
if (hasBody()) {
hash = (37 * hash) + BODY_FIELD_NUMBER;
hash = (53 * hash) + getBody().hashCode();
}
if (hasDispatchDeadlineMs()) {
hash = (37 * hash) + DISPATCH_DEADLINE_MS_FIELD_NUMBER;
hash = (53 * hash) + getDispatchDeadlineMs();
}
if (hasAppEngineRouting()) {
hash = (37 * hash) + APP_ENGINE_ROUTING_FIELD_NUMBER;
hash = (53 * hash) + getAppEngineRouting().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.HttpTaskRunnerPayload prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Payload type for HttpTaskRunner. This runner pushes to GAE.
*
*
* Protobuf type {@code java.apphosting.HttpTaskRunnerPayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.HttpTaskRunnerPayload)
com.google.apphosting.executor.Task.HttpTaskRunnerPayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.class, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.HttpTaskRunnerPayload.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getHeaderFieldBuilder();
getAppEngineRoutingFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
method_ = 2;
url_ = com.google.protobuf.ByteString.EMPTY;
if (headerBuilder_ == null) {
header_ = java.util.Collections.emptyList();
} else {
header_ = null;
headerBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
body_ = com.google.protobuf.ByteString.EMPTY;
dispatchDeadlineMs_ = 0;
appEngineRouting_ = null;
if (appEngineRoutingBuilder_ != null) {
appEngineRoutingBuilder_.dispose();
appEngineRoutingBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_HttpTaskRunnerPayload_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.HttpTaskRunnerPayload.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload build() {
com.google.apphosting.executor.Task.HttpTaskRunnerPayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload buildPartial() {
com.google.apphosting.executor.Task.HttpTaskRunnerPayload result = new com.google.apphosting.executor.Task.HttpTaskRunnerPayload(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.executor.Task.HttpTaskRunnerPayload result) {
if (headerBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
header_ = java.util.Collections.unmodifiableList(header_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
}
private void buildPartial0(com.google.apphosting.executor.Task.HttpTaskRunnerPayload result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.method_ = method_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.url_ = url_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.body_ = body_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.dispatchDeadlineMs_ = dispatchDeadlineMs_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.appEngineRouting_ = appEngineRoutingBuilder_ == null
? appEngineRouting_
: appEngineRoutingBuilder_.build();
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.HttpTaskRunnerPayload) {
return mergeFrom((com.google.apphosting.executor.Task.HttpTaskRunnerPayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.HttpTaskRunnerPayload other) {
if (other == com.google.apphosting.executor.Task.HttpTaskRunnerPayload.getDefaultInstance()) return this;
if (other.hasMethod()) {
setMethod(other.getMethod());
}
if (other.hasUrl()) {
setUrl(other.getUrl());
}
if (headerBuilder_ == null) {
if (!other.header_.isEmpty()) {
if (header_.isEmpty()) {
header_ = other.header_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureHeaderIsMutable();
header_.addAll(other.header_);
}
onChanged();
}
} else {
if (!other.header_.isEmpty()) {
if (headerBuilder_.isEmpty()) {
headerBuilder_.dispose();
headerBuilder_ = null;
header_ = other.header_;
bitField0_ = (bitField0_ & ~0x00000004);
headerBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getHeaderFieldBuilder() : null;
} else {
headerBuilder_.addAllMessages(other.header_);
}
}
}
if (other.hasBody()) {
setBody(other.getBody());
}
if (other.hasDispatchDeadlineMs()) {
setDispatchDeadlineMs(other.getDispatchDeadlineMs());
}
if (other.hasAppEngineRouting()) {
mergeAppEngineRouting(other.getAppEngineRouting());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasUrl()) {
return false;
}
for (int i = 0; i < getHeaderCount(); i++) {
if (!getHeader(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod tmpValue =
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
method_ = tmpRaw;
bitField0_ |= 0x00000001;
}
break;
} // case 8
case 18: {
url_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 27: {
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header m =
input.readGroup(3,
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.PARSER,
extensionRegistry);
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(m);
} else {
headerBuilder_.addMessage(m);
}
break;
} // case 27
case 50: {
body_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 50
case 56: {
dispatchDeadlineMs_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 56
case 66: {
input.readMessage(
getAppEngineRoutingFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 66
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int method_ = 2;
/**
* optional .java.apphosting.HttpTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return Whether the method field is set.
*/
@java.lang.Override public boolean hasMethod() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.HttpTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return The method.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod getMethod() {
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod result = com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod.forNumber(method_);
return result == null ? com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod.POST : result;
}
/**
* optional .java.apphosting.HttpTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @param value The method to set.
* @return This builder for chaining.
*/
public Builder setMethod(com.google.apphosting.executor.Task.HttpTaskRunnerPayload.RequestMethod value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
method_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.HttpTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return This builder for chaining.
*/
public Builder clearMethod() {
bitField0_ = (bitField0_ & ~0x00000001);
method_ = 2;
onChanged();
return this;
}
private com.google.protobuf.ByteString url_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Note that this stores the relative url. The host is derived from the app.
*
*
* required bytes url = 2;
* @return Whether the url field is set.
*/
@java.lang.Override
public boolean hasUrl() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Note that this stores the relative url. The host is derived from the app.
*
*
* required bytes url = 2;
* @return The url.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUrl() {
return url_;
}
/**
*
* Note that this stores the relative url. The host is derived from the app.
*
*
* required bytes url = 2;
* @param value The url to set.
* @return This builder for chaining.
*/
public Builder setUrl(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
url_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Note that this stores the relative url. The host is derived from the app.
*
*
* required bytes url = 2;
* @return This builder for chaining.
*/
public Builder clearUrl() {
bitField0_ = (bitField0_ & ~0x00000002);
url_ = getDefaultInstance().getUrl();
onChanged();
return this;
}
private java.util.List header_ =
java.util.Collections.emptyList();
private void ensureHeaderIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
header_ = new java.util.ArrayList(header_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder> headerBuilder_;
/**
* repeated group Header = 3 { ... }
*/
public java.util.List getHeaderList() {
if (headerBuilder_ == null) {
return java.util.Collections.unmodifiableList(header_);
} else {
return headerBuilder_.getMessageList();
}
}
/**
* repeated group Header = 3 { ... }
*/
public int getHeaderCount() {
if (headerBuilder_ == null) {
return header_.size();
} else {
return headerBuilder_.getCount();
}
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header getHeader(int index) {
if (headerBuilder_ == null) {
return header_.get(index);
} else {
return headerBuilder_.getMessage(index);
}
}
/**
* repeated group Header = 3 { ... }
*/
public Builder setHeader(
int index, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.set(index, value);
onChanged();
} else {
headerBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder setHeader(
int index, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.set(index, builderForValue.build());
onChanged();
} else {
headerBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addHeader(com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.add(value);
onChanged();
} else {
headerBuilder_.addMessage(value);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addHeader(
int index, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.add(index, value);
onChanged();
} else {
headerBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addHeader(
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(builderForValue.build());
onChanged();
} else {
headerBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addHeader(
int index, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(index, builderForValue.build());
onChanged();
} else {
headerBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addAllHeader(
java.lang.Iterable extends com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header> values) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, header_);
onChanged();
} else {
headerBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
headerBuilder_.clear();
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder removeHeader(int index) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.remove(index);
onChanged();
} else {
headerBuilder_.remove(index);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder getHeaderBuilder(
int index) {
return getHeaderFieldBuilder().getBuilder(index);
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index) {
if (headerBuilder_ == null) {
return header_.get(index); } else {
return headerBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated group Header = 3 { ... }
*/
public java.util.List extends com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(header_);
}
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder addHeaderBuilder() {
return getHeaderFieldBuilder().addBuilder(
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.getDefaultInstance());
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder addHeaderBuilder(
int index) {
return getHeaderFieldBuilder().addBuilder(
index, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.getDefaultInstance());
}
/**
* repeated group Header = 3 { ... }
*/
public java.util.List
getHeaderBuilderList() {
return getHeaderFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.HttpTaskRunnerPayload.HeaderOrBuilder>(
header_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private com.google.protobuf.ByteString body_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return Whether the body field is set.
*/
@java.lang.Override
public boolean hasBody() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return The body.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBody() {
return body_;
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @param value The body to set.
* @return This builder for chaining.
*/
public Builder setBody(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
body_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return This builder for chaining.
*/
public Builder clearBody() {
bitField0_ = (bitField0_ & ~0x00000008);
body_ = getDefaultInstance().getBody();
onChanged();
return this;
}
private int dispatchDeadlineMs_ ;
/**
*
* The dispatch_deadline is the time that Executor waits for the target,Adding
* with as few internal hops as possible.
* Note that when the request is cancelled, Cloud Tasks will stop listing for
* the response and will schedule the task to be retried, but whether the
* worker stops processing depends on how App Engine handles cancelled HTTP
* requests. Currently App Engine does not react to cancelled HTTP requests.
* Thus, this parameter controls how long Cloud Tasks will wait to retry the
* task, but it does not affect the work done by the App Engine instances.
*
*
* optional int32 dispatch_deadline_ms = 7;
* @return Whether the dispatchDeadlineMs field is set.
*/
@java.lang.Override
public boolean hasDispatchDeadlineMs() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The dispatch_deadline is the time that Executor waits for the target,Adding
* with as few internal hops as possible.
* Note that when the request is cancelled, Cloud Tasks will stop listing for
* the response and will schedule the task to be retried, but whether the
* worker stops processing depends on how App Engine handles cancelled HTTP
* requests. Currently App Engine does not react to cancelled HTTP requests.
* Thus, this parameter controls how long Cloud Tasks will wait to retry the
* task, but it does not affect the work done by the App Engine instances.
*
*
* optional int32 dispatch_deadline_ms = 7;
* @return The dispatchDeadlineMs.
*/
@java.lang.Override
public int getDispatchDeadlineMs() {
return dispatchDeadlineMs_;
}
/**
*
* The dispatch_deadline is the time that Executor waits for the target,Adding
* with as few internal hops as possible.
* Note that when the request is cancelled, Cloud Tasks will stop listing for
* the response and will schedule the task to be retried, but whether the
* worker stops processing depends on how App Engine handles cancelled HTTP
* requests. Currently App Engine does not react to cancelled HTTP requests.
* Thus, this parameter controls how long Cloud Tasks will wait to retry the
* task, but it does not affect the work done by the App Engine instances.
*
*
* optional int32 dispatch_deadline_ms = 7;
* @param value The dispatchDeadlineMs to set.
* @return This builder for chaining.
*/
public Builder setDispatchDeadlineMs(int value) {
dispatchDeadlineMs_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The dispatch_deadline is the time that Executor waits for the target,Adding
* with as few internal hops as possible.
* Note that when the request is cancelled, Cloud Tasks will stop listing for
* the response and will schedule the task to be retried, but whether the
* worker stops processing depends on how App Engine handles cancelled HTTP
* requests. Currently App Engine does not react to cancelled HTTP requests.
* Thus, this parameter controls how long Cloud Tasks will wait to retry the
* task, but it does not affect the work done by the App Engine instances.
*
*
* optional int32 dispatch_deadline_ms = 7;
* @return This builder for chaining.
*/
public Builder clearDispatchDeadlineMs() {
bitField0_ = (bitField0_ & ~0x00000010);
dispatchDeadlineMs_ = 0;
onChanged();
return this;
}
private com.google.apphosting.executor.Task.AppEngineRouting appEngineRouting_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.AppEngineRouting, com.google.apphosting.executor.Task.AppEngineRouting.Builder, com.google.apphosting.executor.Task.AppEngineRoutingOrBuilder> appEngineRoutingBuilder_;
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
* @return Whether the appEngineRouting field is set.
*/
public boolean hasAppEngineRouting() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
* @return The appEngineRouting.
*/
public com.google.apphosting.executor.Task.AppEngineRouting getAppEngineRouting() {
if (appEngineRoutingBuilder_ == null) {
return appEngineRouting_ == null ? com.google.apphosting.executor.Task.AppEngineRouting.getDefaultInstance() : appEngineRouting_;
} else {
return appEngineRoutingBuilder_.getMessage();
}
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
public Builder setAppEngineRouting(com.google.apphosting.executor.Task.AppEngineRouting value) {
if (appEngineRoutingBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
appEngineRouting_ = value;
} else {
appEngineRoutingBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
public Builder setAppEngineRouting(
com.google.apphosting.executor.Task.AppEngineRouting.Builder builderForValue) {
if (appEngineRoutingBuilder_ == null) {
appEngineRouting_ = builderForValue.build();
} else {
appEngineRoutingBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
public Builder mergeAppEngineRouting(com.google.apphosting.executor.Task.AppEngineRouting value) {
if (appEngineRoutingBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
appEngineRouting_ != null &&
appEngineRouting_ != com.google.apphosting.executor.Task.AppEngineRouting.getDefaultInstance()) {
getAppEngineRoutingBuilder().mergeFrom(value);
} else {
appEngineRouting_ = value;
}
} else {
appEngineRoutingBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
public Builder clearAppEngineRouting() {
bitField0_ = (bitField0_ & ~0x00000020);
appEngineRouting_ = null;
if (appEngineRoutingBuilder_ != null) {
appEngineRoutingBuilder_.dispose();
appEngineRoutingBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
public com.google.apphosting.executor.Task.AppEngineRouting.Builder getAppEngineRoutingBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getAppEngineRoutingFieldBuilder().getBuilder();
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
public com.google.apphosting.executor.Task.AppEngineRoutingOrBuilder getAppEngineRoutingOrBuilder() {
if (appEngineRoutingBuilder_ != null) {
return appEngineRoutingBuilder_.getMessageOrBuilder();
} else {
return appEngineRouting_ == null ?
com.google.apphosting.executor.Task.AppEngineRouting.getDefaultInstance() : appEngineRouting_;
}
}
/**
*
* app_engine_routing records the routing information for the payload.
*
*
* optional .java.apphosting.AppEngineRouting app_engine_routing = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.AppEngineRouting, com.google.apphosting.executor.Task.AppEngineRouting.Builder, com.google.apphosting.executor.Task.AppEngineRoutingOrBuilder>
getAppEngineRoutingFieldBuilder() {
if (appEngineRoutingBuilder_ == null) {
appEngineRoutingBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.AppEngineRouting, com.google.apphosting.executor.Task.AppEngineRouting.Builder, com.google.apphosting.executor.Task.AppEngineRoutingOrBuilder>(
getAppEngineRouting(),
getParentForChildren(),
isClean());
appEngineRouting_ = null;
}
return appEngineRoutingBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.HttpTaskRunnerPayload)
}
// @@protoc_insertion_point(class_scope:java.apphosting.HttpTaskRunnerPayload)
private static final com.google.apphosting.executor.Task.HttpTaskRunnerPayload DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.HttpTaskRunnerPayload();
}
public static com.google.apphosting.executor.Task.HttpTaskRunnerPayload getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HttpTaskRunnerPayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.HttpTaskRunnerPayload getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AppEngineRoutingOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.AppEngineRouting)
com.google.protobuf.MessageOrBuilder {
/**
* optional string service = 1;
* @return Whether the service field is set.
*/
boolean hasService();
/**
* optional string service = 1;
* @return The service.
*/
java.lang.String getService();
/**
* optional string service = 1;
* @return The bytes for service.
*/
com.google.protobuf.ByteString
getServiceBytes();
/**
* optional string version = 2;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
* optional string version = 2;
* @return The version.
*/
java.lang.String getVersion();
/**
* optional string version = 2;
* @return The bytes for version.
*/
com.google.protobuf.ByteString
getVersionBytes();
/**
* optional string instance = 3;
* @return Whether the instance field is set.
*/
boolean hasInstance();
/**
* optional string instance = 3;
* @return The instance.
*/
java.lang.String getInstance();
/**
* optional string instance = 3;
* @return The bytes for instance.
*/
com.google.protobuf.ByteString
getInstanceBytes();
/**
* optional string host = 4;
* @return Whether the host field is set.
*/
boolean hasHost();
/**
* optional string host = 4;
* @return The host.
*/
java.lang.String getHost();
/**
* optional string host = 4;
* @return The bytes for host.
*/
com.google.protobuf.ByteString
getHostBytes();
}
/**
*
* AppEngineRouting contains the routing information for the task, including the
* service, version, instance, and host that the task is sent to.
*
*
* Protobuf type {@code java.apphosting.AppEngineRouting}
*/
public static final class AppEngineRouting extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.AppEngineRouting)
AppEngineRoutingOrBuilder {
private static final long serialVersionUID = 0L;
// Use AppEngineRouting.newBuilder() to construct.
private AppEngineRouting(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AppEngineRouting() {
service_ = "";
version_ = "";
instance_ = "";
host_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AppEngineRouting();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_AppEngineRouting_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_AppEngineRouting_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.AppEngineRouting.class, com.google.apphosting.executor.Task.AppEngineRouting.Builder.class);
}
private int bitField0_;
public static final int SERVICE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object service_ = "";
/**
* optional string service = 1;
* @return Whether the service field is set.
*/
@java.lang.Override
public boolean hasService() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string service = 1;
* @return The service.
*/
@java.lang.Override
public java.lang.String getService() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
service_ = s;
}
return s;
}
}
/**
* optional string service = 1;
* @return The bytes for service.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VERSION_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object version_ = "";
/**
* optional string version = 2;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string version = 2;
* @return The version.
*/
@java.lang.Override
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
version_ = s;
}
return s;
}
}
/**
* optional string version = 2;
* @return The bytes for version.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTANCE_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object instance_ = "";
/**
* optional string instance = 3;
* @return Whether the instance field is set.
*/
@java.lang.Override
public boolean hasInstance() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string instance = 3;
* @return The instance.
*/
@java.lang.Override
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
}
}
/**
* optional string instance = 3;
* @return The bytes for instance.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HOST_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object host_ = "";
/**
* optional string host = 4;
* @return Whether the host field is set.
*/
@java.lang.Override
public boolean hasHost() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string host = 4;
* @return The host.
*/
@java.lang.Override
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
host_ = s;
}
return s;
}
}
/**
* optional string host = 4;
* @return The bytes for host.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, service_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, version_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, instance_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, host_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, service_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, version_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, instance_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, host_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.AppEngineRouting)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.AppEngineRouting other = (com.google.apphosting.executor.Task.AppEngineRouting) obj;
if (hasService() != other.hasService()) return false;
if (hasService()) {
if (!getService()
.equals(other.getService())) return false;
}
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (!getVersion()
.equals(other.getVersion())) return false;
}
if (hasInstance() != other.hasInstance()) return false;
if (hasInstance()) {
if (!getInstance()
.equals(other.getInstance())) return false;
}
if (hasHost() != other.hasHost()) return false;
if (hasHost()) {
if (!getHost()
.equals(other.getHost())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasService()) {
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
}
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
}
if (hasInstance()) {
hash = (37 * hash) + INSTANCE_FIELD_NUMBER;
hash = (53 * hash) + getInstance().hashCode();
}
if (hasHost()) {
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.AppEngineRouting parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.AppEngineRouting prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* AppEngineRouting contains the routing information for the task, including the
* service, version, instance, and host that the task is sent to.
*
*
* Protobuf type {@code java.apphosting.AppEngineRouting}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.AppEngineRouting)
com.google.apphosting.executor.Task.AppEngineRoutingOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_AppEngineRouting_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_AppEngineRouting_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.AppEngineRouting.class, com.google.apphosting.executor.Task.AppEngineRouting.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.AppEngineRouting.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
service_ = "";
version_ = "";
instance_ = "";
host_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_AppEngineRouting_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.AppEngineRouting getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.AppEngineRouting.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.AppEngineRouting build() {
com.google.apphosting.executor.Task.AppEngineRouting result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.AppEngineRouting buildPartial() {
com.google.apphosting.executor.Task.AppEngineRouting result = new com.google.apphosting.executor.Task.AppEngineRouting(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.AppEngineRouting result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.service_ = service_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.version_ = version_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.instance_ = instance_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.host_ = host_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.AppEngineRouting) {
return mergeFrom((com.google.apphosting.executor.Task.AppEngineRouting)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.AppEngineRouting other) {
if (other == com.google.apphosting.executor.Task.AppEngineRouting.getDefaultInstance()) return this;
if (other.hasService()) {
service_ = other.service_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasVersion()) {
version_ = other.version_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasInstance()) {
instance_ = other.instance_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasHost()) {
host_ = other.host_;
bitField0_ |= 0x00000008;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
service_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
version_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
instance_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
host_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object service_ = "";
/**
* optional string service = 1;
* @return Whether the service field is set.
*/
public boolean hasService() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string service = 1;
* @return The service.
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
service_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string service = 1;
* @return The bytes for service.
*/
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string service = 1;
* @param value The service to set.
* @return This builder for chaining.
*/
public Builder setService(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
service_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional string service = 1;
* @return This builder for chaining.
*/
public Builder clearService() {
service_ = getDefaultInstance().getService();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* optional string service = 1;
* @param value The bytes for service to set.
* @return This builder for chaining.
*/
public Builder setServiceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
service_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object version_ = "";
/**
* optional string version = 2;
* @return Whether the version field is set.
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string version = 2;
* @return The version.
*/
public java.lang.String getVersion() {
java.lang.Object ref = version_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
version_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string version = 2;
* @return The bytes for version.
*/
public com.google.protobuf.ByteString
getVersionBytes() {
java.lang.Object ref = version_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
version_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string version = 2;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
version_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional string version = 2;
* @return This builder for chaining.
*/
public Builder clearVersion() {
version_ = getDefaultInstance().getVersion();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* optional string version = 2;
* @param value The bytes for version to set.
* @return This builder for chaining.
*/
public Builder setVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
version_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object instance_ = "";
/**
* optional string instance = 3;
* @return Whether the instance field is set.
*/
public boolean hasInstance() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string instance = 3;
* @return The instance.
*/
public java.lang.String getInstance() {
java.lang.Object ref = instance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
instance_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string instance = 3;
* @return The bytes for instance.
*/
public com.google.protobuf.ByteString
getInstanceBytes() {
java.lang.Object ref = instance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string instance = 3;
* @param value The instance to set.
* @return This builder for chaining.
*/
public Builder setInstance(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
instance_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional string instance = 3;
* @return This builder for chaining.
*/
public Builder clearInstance() {
instance_ = getDefaultInstance().getInstance();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* optional string instance = 3;
* @param value The bytes for instance to set.
* @return This builder for chaining.
*/
public Builder setInstanceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
instance_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object host_ = "";
/**
* optional string host = 4;
* @return Whether the host field is set.
*/
public boolean hasHost() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string host = 4;
* @return The host.
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
host_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string host = 4;
* @return The bytes for host.
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string host = 4;
* @param value The host to set.
* @return This builder for chaining.
*/
public Builder setHost(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
host_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional string host = 4;
* @return This builder for chaining.
*/
public Builder clearHost() {
host_ = getDefaultInstance().getHost();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* optional string host = 4;
* @param value The bytes for host to set.
* @return This builder for chaining.
*/
public Builder setHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
host_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.AppEngineRouting)
}
// @@protoc_insertion_point(class_scope:java.apphosting.AppEngineRouting)
private static final com.google.apphosting.executor.Task.AppEngineRouting DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.AppEngineRouting();
}
public static com.google.apphosting.executor.Task.AppEngineRouting getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AppEngineRouting parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.AppEngineRouting getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CronTaskRunnerPayloadOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.CronTaskRunnerPayload)
com.google.protobuf.MessageOrBuilder {
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return Whether the schedule field is set.
*/
boolean hasSchedule();
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return The schedule.
*/
java.lang.String getSchedule();
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return The bytes for schedule.
*/
com.google.protobuf.ByteString
getScheduleBytes();
/**
* required string timezone = 2;
* @return Whether the timezone field is set.
*/
boolean hasTimezone();
/**
* required string timezone = 2;
* @return The timezone.
*/
java.lang.String getTimezone();
/**
* required string timezone = 2;
* @return The bytes for timezone.
*/
com.google.protobuf.ByteString
getTimezoneBytes();
/**
* optional .java.apphosting.CronTaskRunnerPayload.TimeSpecificationType time_specification_type = 5;
* @return Whether the timeSpecificationType field is set.
*/
boolean hasTimeSpecificationType();
/**
* optional .java.apphosting.CronTaskRunnerPayload.TimeSpecificationType time_specification_type = 5;
* @return The timeSpecificationType.
*/
com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType getTimeSpecificationType();
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
* @return Whether the delegatedPayload field is set.
*/
boolean hasDelegatedPayload();
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
* @return The delegatedPayload.
*/
com.google.apphosting.executor.Task.TaskRunnerPayload getDelegatedPayload();
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder getDelegatedPayloadOrBuilder();
/**
*
* User can optionally skip several first iterations.
*
*
* optional int64 skip_num_iterations = 4;
* @return Whether the skipNumIterations field is set.
*/
boolean hasSkipNumIterations();
/**
*
* User can optionally skip several first iterations.
*
*
* optional int64 skip_num_iterations = 4;
* @return The skipNumIterations.
*/
long getSkipNumIterations();
}
/**
*
* Payload type for CronTaskRunner.
* CronTaskRunner only handles scheduling and delegates the actual running
* of the task to another runner.
*
*
* Protobuf type {@code java.apphosting.CronTaskRunnerPayload}
*/
public static final class CronTaskRunnerPayload extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.CronTaskRunnerPayload)
CronTaskRunnerPayloadOrBuilder {
private static final long serialVersionUID = 0L;
// Use CronTaskRunnerPayload.newBuilder() to construct.
private CronTaskRunnerPayload(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CronTaskRunnerPayload() {
schedule_ = "";
timezone_ = "";
timeSpecificationType_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CronTaskRunnerPayload();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_CronTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_CronTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.CronTaskRunnerPayload.class, com.google.apphosting.executor.Task.CronTaskRunnerPayload.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.CronTaskRunnerPayload.TimeSpecificationType}
*/
public enum TimeSpecificationType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* TIME_SPECIFICATION_TYPE_UNSPECIFIED = 0;
*/
TIME_SPECIFICATION_TYPE_UNSPECIFIED(0),
/**
* GROC = 1;
*/
GROC(1),
/**
* CRONTAB = 2;
*/
CRONTAB(2),
;
/**
* TIME_SPECIFICATION_TYPE_UNSPECIFIED = 0;
*/
public static final int TIME_SPECIFICATION_TYPE_UNSPECIFIED_VALUE = 0;
/**
* GROC = 1;
*/
public static final int GROC_VALUE = 1;
/**
* CRONTAB = 2;
*/
public static final int CRONTAB_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TimeSpecificationType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static TimeSpecificationType forNumber(int value) {
switch (value) {
case 0: return TIME_SPECIFICATION_TYPE_UNSPECIFIED;
case 1: return GROC;
case 2: return CRONTAB;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TimeSpecificationType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TimeSpecificationType findValueByNumber(int number) {
return TimeSpecificationType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.CronTaskRunnerPayload.getDescriptor().getEnumTypes().get(0);
}
private static final TimeSpecificationType[] VALUES = values();
public static TimeSpecificationType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private TimeSpecificationType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.CronTaskRunnerPayload.TimeSpecificationType)
}
private int bitField0_;
public static final int SCHEDULE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object schedule_ = "";
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return Whether the schedule field is set.
*/
@java.lang.Override
public boolean hasSchedule() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return The schedule.
*/
@java.lang.Override
public java.lang.String getSchedule() {
java.lang.Object ref = schedule_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
schedule_ = s;
}
return s;
}
}
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return The bytes for schedule.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getScheduleBytes() {
java.lang.Object ref = schedule_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schedule_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMEZONE_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object timezone_ = "";
/**
* required string timezone = 2;
* @return Whether the timezone field is set.
*/
@java.lang.Override
public boolean hasTimezone() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string timezone = 2;
* @return The timezone.
*/
@java.lang.Override
public java.lang.String getTimezone() {
java.lang.Object ref = timezone_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
timezone_ = s;
}
return s;
}
}
/**
* required string timezone = 2;
* @return The bytes for timezone.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTimezoneBytes() {
java.lang.Object ref = timezone_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timezone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIME_SPECIFICATION_TYPE_FIELD_NUMBER = 5;
private int timeSpecificationType_ = 0;
/**
* optional .java.apphosting.CronTaskRunnerPayload.TimeSpecificationType time_specification_type = 5;
* @return Whether the timeSpecificationType field is set.
*/
@java.lang.Override public boolean hasTimeSpecificationType() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .java.apphosting.CronTaskRunnerPayload.TimeSpecificationType time_specification_type = 5;
* @return The timeSpecificationType.
*/
@java.lang.Override public com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType getTimeSpecificationType() {
com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType result = com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType.forNumber(timeSpecificationType_);
return result == null ? com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType.TIME_SPECIFICATION_TYPE_UNSPECIFIED : result;
}
public static final int DELEGATED_PAYLOAD_FIELD_NUMBER = 3;
private com.google.apphosting.executor.Task.TaskRunnerPayload delegatedPayload_;
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
* @return Whether the delegatedPayload field is set.
*/
@java.lang.Override
public boolean hasDelegatedPayload() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
* @return The delegatedPayload.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerPayload getDelegatedPayload() {
return delegatedPayload_ == null ? com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance() : delegatedPayload_;
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder getDelegatedPayloadOrBuilder() {
return delegatedPayload_ == null ? com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance() : delegatedPayload_;
}
public static final int SKIP_NUM_ITERATIONS_FIELD_NUMBER = 4;
private long skipNumIterations_ = 0L;
/**
*
* User can optionally skip several first iterations.
*
*
* optional int64 skip_num_iterations = 4;
* @return Whether the skipNumIterations field is set.
*/
@java.lang.Override
public boolean hasSkipNumIterations() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* User can optionally skip several first iterations.
*
*
* optional int64 skip_num_iterations = 4;
* @return The skipNumIterations.
*/
@java.lang.Override
public long getSkipNumIterations() {
return skipNumIterations_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasSchedule()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasTimezone()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasDelegatedPayload()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, schedule_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, timezone_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(3, getDelegatedPayload());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt64(4, skipNumIterations_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeEnum(5, timeSpecificationType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, schedule_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, timezone_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getDelegatedPayload());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, skipNumIterations_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, timeSpecificationType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.CronTaskRunnerPayload)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.CronTaskRunnerPayload other = (com.google.apphosting.executor.Task.CronTaskRunnerPayload) obj;
if (hasSchedule() != other.hasSchedule()) return false;
if (hasSchedule()) {
if (!getSchedule()
.equals(other.getSchedule())) return false;
}
if (hasTimezone() != other.hasTimezone()) return false;
if (hasTimezone()) {
if (!getTimezone()
.equals(other.getTimezone())) return false;
}
if (hasTimeSpecificationType() != other.hasTimeSpecificationType()) return false;
if (hasTimeSpecificationType()) {
if (timeSpecificationType_ != other.timeSpecificationType_) return false;
}
if (hasDelegatedPayload() != other.hasDelegatedPayload()) return false;
if (hasDelegatedPayload()) {
if (!getDelegatedPayload()
.equals(other.getDelegatedPayload())) return false;
}
if (hasSkipNumIterations() != other.hasSkipNumIterations()) return false;
if (hasSkipNumIterations()) {
if (getSkipNumIterations()
!= other.getSkipNumIterations()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSchedule()) {
hash = (37 * hash) + SCHEDULE_FIELD_NUMBER;
hash = (53 * hash) + getSchedule().hashCode();
}
if (hasTimezone()) {
hash = (37 * hash) + TIMEZONE_FIELD_NUMBER;
hash = (53 * hash) + getTimezone().hashCode();
}
if (hasTimeSpecificationType()) {
hash = (37 * hash) + TIME_SPECIFICATION_TYPE_FIELD_NUMBER;
hash = (53 * hash) + timeSpecificationType_;
}
if (hasDelegatedPayload()) {
hash = (37 * hash) + DELEGATED_PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getDelegatedPayload().hashCode();
}
if (hasSkipNumIterations()) {
hash = (37 * hash) + SKIP_NUM_ITERATIONS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSkipNumIterations());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.CronTaskRunnerPayload prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Payload type for CronTaskRunner.
* CronTaskRunner only handles scheduling and delegates the actual running
* of the task to another runner.
*
*
* Protobuf type {@code java.apphosting.CronTaskRunnerPayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.CronTaskRunnerPayload)
com.google.apphosting.executor.Task.CronTaskRunnerPayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_CronTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_CronTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.CronTaskRunnerPayload.class, com.google.apphosting.executor.Task.CronTaskRunnerPayload.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.CronTaskRunnerPayload.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDelegatedPayloadFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
schedule_ = "";
timezone_ = "";
timeSpecificationType_ = 0;
delegatedPayload_ = null;
if (delegatedPayloadBuilder_ != null) {
delegatedPayloadBuilder_.dispose();
delegatedPayloadBuilder_ = null;
}
skipNumIterations_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_CronTaskRunnerPayload_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.CronTaskRunnerPayload getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.CronTaskRunnerPayload.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.CronTaskRunnerPayload build() {
com.google.apphosting.executor.Task.CronTaskRunnerPayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.CronTaskRunnerPayload buildPartial() {
com.google.apphosting.executor.Task.CronTaskRunnerPayload result = new com.google.apphosting.executor.Task.CronTaskRunnerPayload(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.CronTaskRunnerPayload result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.schedule_ = schedule_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.timezone_ = timezone_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.timeSpecificationType_ = timeSpecificationType_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.delegatedPayload_ = delegatedPayloadBuilder_ == null
? delegatedPayload_
: delegatedPayloadBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.skipNumIterations_ = skipNumIterations_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.CronTaskRunnerPayload) {
return mergeFrom((com.google.apphosting.executor.Task.CronTaskRunnerPayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.CronTaskRunnerPayload other) {
if (other == com.google.apphosting.executor.Task.CronTaskRunnerPayload.getDefaultInstance()) return this;
if (other.hasSchedule()) {
schedule_ = other.schedule_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasTimezone()) {
timezone_ = other.timezone_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasTimeSpecificationType()) {
setTimeSpecificationType(other.getTimeSpecificationType());
}
if (other.hasDelegatedPayload()) {
mergeDelegatedPayload(other.getDelegatedPayload());
}
if (other.hasSkipNumIterations()) {
setSkipNumIterations(other.getSkipNumIterations());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasSchedule()) {
return false;
}
if (!hasTimezone()) {
return false;
}
if (!hasDelegatedPayload()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
schedule_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
timezone_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getDelegatedPayloadFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 26
case 32: {
skipNumIterations_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 32
case 40: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType tmpValue =
com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(5, tmpRaw);
} else {
timeSpecificationType_ = tmpRaw;
bitField0_ |= 0x00000004;
}
break;
} // case 40
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object schedule_ = "";
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return Whether the schedule field is set.
*/
public boolean hasSchedule() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return The schedule.
*/
public java.lang.String getSchedule() {
java.lang.Object ref = schedule_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
schedule_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return The bytes for schedule.
*/
public com.google.protobuf.ByteString
getScheduleBytes() {
java.lang.Object ref = schedule_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schedule_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @param value The schedule to set.
* @return This builder for chaining.
*/
public Builder setSchedule(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
schedule_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @return This builder for chaining.
*/
public Builder clearSchedule() {
schedule_ = getDefaultInstance().getSchedule();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Schedule for Cron task. This can be either:
* GROC: See //depot/google3/borg/borgcron/groc.g for details.
* or CRONTAB: http://en.wikipedia.org/wiki/Cron#Overview
* The type is decided based on time_specification_type field.
* If it is not specified or is TIME_SPECIFICATION_TYPE_UNSPECIFIED,
* GROC is assumed.
*
*
* required string schedule = 1;
* @param value The bytes for schedule to set.
* @return This builder for chaining.
*/
public Builder setScheduleBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
schedule_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object timezone_ = "";
/**
* required string timezone = 2;
* @return Whether the timezone field is set.
*/
public boolean hasTimezone() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string timezone = 2;
* @return The timezone.
*/
public java.lang.String getTimezone() {
java.lang.Object ref = timezone_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
timezone_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string timezone = 2;
* @return The bytes for timezone.
*/
public com.google.protobuf.ByteString
getTimezoneBytes() {
java.lang.Object ref = timezone_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timezone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string timezone = 2;
* @param value The timezone to set.
* @return This builder for chaining.
*/
public Builder setTimezone(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
timezone_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required string timezone = 2;
* @return This builder for chaining.
*/
public Builder clearTimezone() {
timezone_ = getDefaultInstance().getTimezone();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* required string timezone = 2;
* @param value The bytes for timezone to set.
* @return This builder for chaining.
*/
public Builder setTimezoneBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
timezone_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private int timeSpecificationType_ = 0;
/**
* optional .java.apphosting.CronTaskRunnerPayload.TimeSpecificationType time_specification_type = 5;
* @return Whether the timeSpecificationType field is set.
*/
@java.lang.Override public boolean hasTimeSpecificationType() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .java.apphosting.CronTaskRunnerPayload.TimeSpecificationType time_specification_type = 5;
* @return The timeSpecificationType.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType getTimeSpecificationType() {
com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType result = com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType.forNumber(timeSpecificationType_);
return result == null ? com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType.TIME_SPECIFICATION_TYPE_UNSPECIFIED : result;
}
/**
* optional .java.apphosting.CronTaskRunnerPayload.TimeSpecificationType time_specification_type = 5;
* @param value The timeSpecificationType to set.
* @return This builder for chaining.
*/
public Builder setTimeSpecificationType(com.google.apphosting.executor.Task.CronTaskRunnerPayload.TimeSpecificationType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
timeSpecificationType_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.CronTaskRunnerPayload.TimeSpecificationType time_specification_type = 5;
* @return This builder for chaining.
*/
public Builder clearTimeSpecificationType() {
bitField0_ = (bitField0_ & ~0x00000004);
timeSpecificationType_ = 0;
onChanged();
return this;
}
private com.google.apphosting.executor.Task.TaskRunnerPayload delegatedPayload_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerPayload, com.google.apphosting.executor.Task.TaskRunnerPayload.Builder, com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder> delegatedPayloadBuilder_;
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
* @return Whether the delegatedPayload field is set.
*/
public boolean hasDelegatedPayload() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
* @return The delegatedPayload.
*/
public com.google.apphosting.executor.Task.TaskRunnerPayload getDelegatedPayload() {
if (delegatedPayloadBuilder_ == null) {
return delegatedPayload_ == null ? com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance() : delegatedPayload_;
} else {
return delegatedPayloadBuilder_.getMessage();
}
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
public Builder setDelegatedPayload(com.google.apphosting.executor.Task.TaskRunnerPayload value) {
if (delegatedPayloadBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
delegatedPayload_ = value;
} else {
delegatedPayloadBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
public Builder setDelegatedPayload(
com.google.apphosting.executor.Task.TaskRunnerPayload.Builder builderForValue) {
if (delegatedPayloadBuilder_ == null) {
delegatedPayload_ = builderForValue.build();
} else {
delegatedPayloadBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
public Builder mergeDelegatedPayload(com.google.apphosting.executor.Task.TaskRunnerPayload value) {
if (delegatedPayloadBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
delegatedPayload_ != null &&
delegatedPayload_ != com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance()) {
getDelegatedPayloadBuilder().mergeFrom(value);
} else {
delegatedPayload_ = value;
}
} else {
delegatedPayloadBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
public Builder clearDelegatedPayload() {
bitField0_ = (bitField0_ & ~0x00000008);
delegatedPayload_ = null;
if (delegatedPayloadBuilder_ != null) {
delegatedPayloadBuilder_.dispose();
delegatedPayloadBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
public com.google.apphosting.executor.Task.TaskRunnerPayload.Builder getDelegatedPayloadBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getDelegatedPayloadFieldBuilder().getBuilder();
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
public com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder getDelegatedPayloadOrBuilder() {
if (delegatedPayloadBuilder_ != null) {
return delegatedPayloadBuilder_.getMessageOrBuilder();
} else {
return delegatedPayload_ == null ?
com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance() : delegatedPayload_;
}
}
/**
*
* Task payload which will be sent to the recipient.
* No recursion is supported, 'delegated_payload' must not be
* CronTaskRunnerPayload.
*
*
* required .java.apphosting.TaskRunnerPayload delegated_payload = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerPayload, com.google.apphosting.executor.Task.TaskRunnerPayload.Builder, com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder>
getDelegatedPayloadFieldBuilder() {
if (delegatedPayloadBuilder_ == null) {
delegatedPayloadBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerPayload, com.google.apphosting.executor.Task.TaskRunnerPayload.Builder, com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder>(
getDelegatedPayload(),
getParentForChildren(),
isClean());
delegatedPayload_ = null;
}
return delegatedPayloadBuilder_;
}
private long skipNumIterations_ ;
/**
*
* User can optionally skip several first iterations.
*
*
* optional int64 skip_num_iterations = 4;
* @return Whether the skipNumIterations field is set.
*/
@java.lang.Override
public boolean hasSkipNumIterations() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* User can optionally skip several first iterations.
*
*
* optional int64 skip_num_iterations = 4;
* @return The skipNumIterations.
*/
@java.lang.Override
public long getSkipNumIterations() {
return skipNumIterations_;
}
/**
*
* User can optionally skip several first iterations.
*
*
* optional int64 skip_num_iterations = 4;
* @param value The skipNumIterations to set.
* @return This builder for chaining.
*/
public Builder setSkipNumIterations(long value) {
skipNumIterations_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* User can optionally skip several first iterations.
*
*
* optional int64 skip_num_iterations = 4;
* @return This builder for chaining.
*/
public Builder clearSkipNumIterations() {
bitField0_ = (bitField0_ & ~0x00000010);
skipNumIterations_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.CronTaskRunnerPayload)
}
// @@protoc_insertion_point(class_scope:java.apphosting.CronTaskRunnerPayload)
private static final com.google.apphosting.executor.Task.CronTaskRunnerPayload DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.CronTaskRunnerPayload();
}
public static com.google.apphosting.executor.Task.CronTaskRunnerPayload getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CronTaskRunnerPayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.CronTaskRunnerPayload getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StubbyTaskRunnerPayloadOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.StubbyTaskRunnerPayload)
com.google.protobuf.MessageOrBuilder {
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
* @return Whether the channel field is set.
*/
boolean hasChannel();
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
* @return The channel.
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel getChannel();
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannelOrBuilder getChannelOrBuilder();
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return Whether the method field is set.
*/
boolean hasMethod();
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return The method.
*/
java.lang.String getMethod();
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return The bytes for method.
*/
com.google.protobuf.ByteString
getMethodBytes();
/**
*
* Protocol buffers to send to Method. Should be in binary.
* NB: proto2 doesn't support RawMessage.
*
*
* required bytes request = 3 [ctype = CORD];
* @return Whether the request field is set.
*/
boolean hasRequest();
/**
*
* Protocol buffers to send to Method. Should be in binary.
* NB: proto2 doesn't support RawMessage.
*
*
* required bytes request = 3 [ctype = CORD];
* @return The request.
*/
com.google.protobuf.ByteString getRequest();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
* @return Whether the rpcOptions field is set.
*/
boolean hasRpcOptions();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
* @return The rpcOptions.
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions getRpcOptions();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptionsOrBuilder getRpcOptionsOrBuilder();
/**
*
* If true, the enclosing task definition will be sent to Method,
* including this StubbyTaskRunnerPayload payload.
*
*
* optional bool request_task_definition = 5 [default = false];
* @return Whether the requestTaskDefinition field is set.
*/
boolean hasRequestTaskDefinition();
/**
*
* If true, the enclosing task definition will be sent to Method,
* including this StubbyTaskRunnerPayload payload.
*
*
* optional bool request_task_definition = 5 [default = false];
* @return The requestTaskDefinition.
*/
boolean getRequestTaskDefinition();
}
/**
*
* Payload type for StubbyTaskRunner.
* Initially based on apphosting/api/stubby/stubby_service.proto
*
*
* Protobuf type {@code java.apphosting.StubbyTaskRunnerPayload}
*/
public static final class StubbyTaskRunnerPayload extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.StubbyTaskRunnerPayload)
StubbyTaskRunnerPayloadOrBuilder {
private static final long serialVersionUID = 0L;
// Use StubbyTaskRunnerPayload.newBuilder() to construct.
private StubbyTaskRunnerPayload(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StubbyTaskRunnerPayload() {
method_ = "";
request_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StubbyTaskRunnerPayload();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.class, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.Builder.class);
}
public interface StubbyChannelOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.StubbyTaskRunnerPayload.StubbyChannel)
com.google.protobuf.MessageOrBuilder {
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return Whether the host field is set.
*/
boolean hasHost();
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return The host.
*/
java.lang.String getHost();
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return The bytes for host.
*/
com.google.protobuf.ByteString
getHostBytes();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy load_balancing_policy = 2 [default = LEAST_LOADED];
* @return Whether the loadBalancingPolicy field is set.
*/
boolean hasLoadBalancingPolicy();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy load_balancing_policy = 2 [default = LEAST_LOADED];
* @return The loadBalancingPolicy.
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy getLoadBalancingPolicy();
/**
* optional string security_protocol = 3 [default = "loas"];
* @return Whether the securityProtocol field is set.
*/
boolean hasSecurityProtocol();
/**
* optional string security_protocol = 3 [default = "loas"];
* @return The securityProtocol.
*/
java.lang.String getSecurityProtocol();
/**
* optional string security_protocol = 3 [default = "loas"];
* @return The bytes for securityProtocol.
*/
com.google.protobuf.ByteString
getSecurityProtocolBytes();
/**
*
* a SSLSecurityLevel value.
*
*
* optional int32 min_security_level = 4;
* @return Whether the minSecurityLevel field is set.
*/
boolean hasMinSecurityLevel();
/**
*
* a SSLSecurityLevel value.
*
*
* optional int32 min_security_level = 4;
* @return The minSecurityLevel.
*/
int getMinSecurityLevel();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy target_selection_policy = 5 [default = NO_FILTER];
* @return Whether the targetSelectionPolicy field is set.
*/
boolean hasTargetSelectionPolicy();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy target_selection_policy = 5 [default = NO_FILTER];
* @return The targetSelectionPolicy.
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy getTargetSelectionPolicy();
/**
*
* When using RANDOM_SUBSET or NETWORK_AWARE, use this as the max_targets
* parameter.
*
*
* optional int32 max_targets = 6 [default = 5];
* @return Whether the maxTargets field is set.
*/
boolean hasMaxTargets();
/**
*
* When using RANDOM_SUBSET or NETWORK_AWARE, use this as the max_targets
* parameter.
*
*
* optional int32 max_targets = 6 [default = 5];
* @return The maxTargets.
*/
int getMaxTargets();
/**
* optional int32 max_outstanding_rpcs = 7;
* @return Whether the maxOutstandingRpcs field is set.
*/
boolean hasMaxOutstandingRpcs();
/**
* optional int32 max_outstanding_rpcs = 7;
* @return The maxOutstandingRpcs.
*/
int getMaxOutstandingRpcs();
}
/**
*
* Describes a stubby channel to do a call on.
* Specifies all parameters for selecting the server and establishing connect
* to it.
*
*
* Protobuf type {@code java.apphosting.StubbyTaskRunnerPayload.StubbyChannel}
*/
public static final class StubbyChannel extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.StubbyTaskRunnerPayload.StubbyChannel)
StubbyChannelOrBuilder {
private static final long serialVersionUID = 0L;
// Use StubbyChannel.newBuilder() to construct.
private StubbyChannel(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StubbyChannel() {
host_ = "";
loadBalancingPolicy_ = 1;
securityProtocol_ = "loas";
targetSelectionPolicy_ = 1;
maxTargets_ = 5;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StubbyChannel();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.class, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy}
*/
public enum LoadBalancingPolicy
implements com.google.protobuf.ProtocolMessageEnum {
/**
* LEAST_LOADED = 1;
*/
LEAST_LOADED(1),
/**
* ROUND_ROBIN = 2;
*/
ROUND_ROBIN(2),
/**
* FIRST_REACHABLE = 3;
*/
FIRST_REACHABLE(3),
/**
* AFFINITY_SCHEDULING = 4;
*/
AFFINITY_SCHEDULING(4),
/**
* ERROR_ADVERSE = 5;
*/
ERROR_ADVERSE(5),
/**
* FIRST_LEAST_LOADED = 6;
*/
FIRST_LEAST_LOADED(6),
/**
* INVERSE_RTT = 7;
*/
INVERSE_RTT(7),
/**
* LATENCY_DRIVEN_RR = 8;
*/
LATENCY_DRIVEN_RR(8),
;
/**
* LEAST_LOADED = 1;
*/
public static final int LEAST_LOADED_VALUE = 1;
/**
* ROUND_ROBIN = 2;
*/
public static final int ROUND_ROBIN_VALUE = 2;
/**
* FIRST_REACHABLE = 3;
*/
public static final int FIRST_REACHABLE_VALUE = 3;
/**
* AFFINITY_SCHEDULING = 4;
*/
public static final int AFFINITY_SCHEDULING_VALUE = 4;
/**
* ERROR_ADVERSE = 5;
*/
public static final int ERROR_ADVERSE_VALUE = 5;
/**
* FIRST_LEAST_LOADED = 6;
*/
public static final int FIRST_LEAST_LOADED_VALUE = 6;
/**
* INVERSE_RTT = 7;
*/
public static final int INVERSE_RTT_VALUE = 7;
/**
* LATENCY_DRIVEN_RR = 8;
*/
public static final int LATENCY_DRIVEN_RR_VALUE = 8;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LoadBalancingPolicy valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static LoadBalancingPolicy forNumber(int value) {
switch (value) {
case 1: return LEAST_LOADED;
case 2: return ROUND_ROBIN;
case 3: return FIRST_REACHABLE;
case 4: return AFFINITY_SCHEDULING;
case 5: return ERROR_ADVERSE;
case 6: return FIRST_LEAST_LOADED;
case 7: return INVERSE_RTT;
case 8: return LATENCY_DRIVEN_RR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
LoadBalancingPolicy> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LoadBalancingPolicy findValueByNumber(int number) {
return LoadBalancingPolicy.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDescriptor().getEnumTypes().get(0);
}
private static final LoadBalancingPolicy[] VALUES = values();
public static LoadBalancingPolicy valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private LoadBalancingPolicy(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy)
}
/**
* Protobuf enum {@code java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy}
*/
public enum TargetSelectionPolicy
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Use rpc2::NoFilterSelectionPolicy
*
*
* NO_FILTER = 1;
*/
NO_FILTER(1),
/**
*
* Use rpc2::RandomSubsetTargetSelectionPolicy
*
*
* RANDOM_SUBSET = 2;
*/
RANDOM_SUBSET(2),
/**
*
* Use rpc2::NetworkAwareTargetSelectionPolicy
*
*
* NETWORK_AWARE = 3;
*/
NETWORK_AWARE(3),
;
/**
*
* Use rpc2::NoFilterSelectionPolicy
*
*
* NO_FILTER = 1;
*/
public static final int NO_FILTER_VALUE = 1;
/**
*
* Use rpc2::RandomSubsetTargetSelectionPolicy
*
*
* RANDOM_SUBSET = 2;
*/
public static final int RANDOM_SUBSET_VALUE = 2;
/**
*
* Use rpc2::NetworkAwareTargetSelectionPolicy
*
*
* NETWORK_AWARE = 3;
*/
public static final int NETWORK_AWARE_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TargetSelectionPolicy valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static TargetSelectionPolicy forNumber(int value) {
switch (value) {
case 1: return NO_FILTER;
case 2: return RANDOM_SUBSET;
case 3: return NETWORK_AWARE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TargetSelectionPolicy> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TargetSelectionPolicy findValueByNumber(int number) {
return TargetSelectionPolicy.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDescriptor().getEnumTypes().get(1);
}
private static final TargetSelectionPolicy[] VALUES = values();
public static TargetSelectionPolicy valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private TargetSelectionPolicy(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy)
}
private int bitField0_;
public static final int HOST_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object host_ = "";
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return Whether the host field is set.
*/
@java.lang.Override
public boolean hasHost() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return The host.
*/
@java.lang.Override
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
host_ = s;
}
return s;
}
}
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return The bytes for host.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOAD_BALANCING_POLICY_FIELD_NUMBER = 2;
private int loadBalancingPolicy_ = 1;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy load_balancing_policy = 2 [default = LEAST_LOADED];
* @return Whether the loadBalancingPolicy field is set.
*/
@java.lang.Override public boolean hasLoadBalancingPolicy() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy load_balancing_policy = 2 [default = LEAST_LOADED];
* @return The loadBalancingPolicy.
*/
@java.lang.Override public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy getLoadBalancingPolicy() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy.forNumber(loadBalancingPolicy_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy.LEAST_LOADED : result;
}
public static final int SECURITY_PROTOCOL_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object securityProtocol_ = "loas";
/**
* optional string security_protocol = 3 [default = "loas"];
* @return Whether the securityProtocol field is set.
*/
@java.lang.Override
public boolean hasSecurityProtocol() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string security_protocol = 3 [default = "loas"];
* @return The securityProtocol.
*/
@java.lang.Override
public java.lang.String getSecurityProtocol() {
java.lang.Object ref = securityProtocol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
securityProtocol_ = s;
}
return s;
}
}
/**
* optional string security_protocol = 3 [default = "loas"];
* @return The bytes for securityProtocol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSecurityProtocolBytes() {
java.lang.Object ref = securityProtocol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
securityProtocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MIN_SECURITY_LEVEL_FIELD_NUMBER = 4;
private int minSecurityLevel_ = 0;
/**
*
* a SSLSecurityLevel value.
*
*
* optional int32 min_security_level = 4;
* @return Whether the minSecurityLevel field is set.
*/
@java.lang.Override
public boolean hasMinSecurityLevel() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* a SSLSecurityLevel value.
*
*
* optional int32 min_security_level = 4;
* @return The minSecurityLevel.
*/
@java.lang.Override
public int getMinSecurityLevel() {
return minSecurityLevel_;
}
public static final int TARGET_SELECTION_POLICY_FIELD_NUMBER = 5;
private int targetSelectionPolicy_ = 1;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy target_selection_policy = 5 [default = NO_FILTER];
* @return Whether the targetSelectionPolicy field is set.
*/
@java.lang.Override public boolean hasTargetSelectionPolicy() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy target_selection_policy = 5 [default = NO_FILTER];
* @return The targetSelectionPolicy.
*/
@java.lang.Override public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy getTargetSelectionPolicy() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy.forNumber(targetSelectionPolicy_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy.NO_FILTER : result;
}
public static final int MAX_TARGETS_FIELD_NUMBER = 6;
private int maxTargets_ = 5;
/**
*
* When using RANDOM_SUBSET or NETWORK_AWARE, use this as the max_targets
* parameter.
*
*
* optional int32 max_targets = 6 [default = 5];
* @return Whether the maxTargets field is set.
*/
@java.lang.Override
public boolean hasMaxTargets() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* When using RANDOM_SUBSET or NETWORK_AWARE, use this as the max_targets
* parameter.
*
*
* optional int32 max_targets = 6 [default = 5];
* @return The maxTargets.
*/
@java.lang.Override
public int getMaxTargets() {
return maxTargets_;
}
public static final int MAX_OUTSTANDING_RPCS_FIELD_NUMBER = 7;
private int maxOutstandingRpcs_ = 0;
/**
* optional int32 max_outstanding_rpcs = 7;
* @return Whether the maxOutstandingRpcs field is set.
*/
@java.lang.Override
public boolean hasMaxOutstandingRpcs() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional int32 max_outstanding_rpcs = 7;
* @return The maxOutstandingRpcs.
*/
@java.lang.Override
public int getMaxOutstandingRpcs() {
return maxOutstandingRpcs_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasHost()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, host_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeEnum(2, loadBalancingPolicy_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, securityProtocol_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt32(4, minSecurityLevel_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeEnum(5, targetSelectionPolicy_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt32(6, maxTargets_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt32(7, maxOutstandingRpcs_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, host_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, loadBalancingPolicy_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, securityProtocol_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, minSecurityLevel_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, targetSelectionPolicy_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, maxTargets_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(7, maxOutstandingRpcs_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel other = (com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel) obj;
if (hasHost() != other.hasHost()) return false;
if (hasHost()) {
if (!getHost()
.equals(other.getHost())) return false;
}
if (hasLoadBalancingPolicy() != other.hasLoadBalancingPolicy()) return false;
if (hasLoadBalancingPolicy()) {
if (loadBalancingPolicy_ != other.loadBalancingPolicy_) return false;
}
if (hasSecurityProtocol() != other.hasSecurityProtocol()) return false;
if (hasSecurityProtocol()) {
if (!getSecurityProtocol()
.equals(other.getSecurityProtocol())) return false;
}
if (hasMinSecurityLevel() != other.hasMinSecurityLevel()) return false;
if (hasMinSecurityLevel()) {
if (getMinSecurityLevel()
!= other.getMinSecurityLevel()) return false;
}
if (hasTargetSelectionPolicy() != other.hasTargetSelectionPolicy()) return false;
if (hasTargetSelectionPolicy()) {
if (targetSelectionPolicy_ != other.targetSelectionPolicy_) return false;
}
if (hasMaxTargets() != other.hasMaxTargets()) return false;
if (hasMaxTargets()) {
if (getMaxTargets()
!= other.getMaxTargets()) return false;
}
if (hasMaxOutstandingRpcs() != other.hasMaxOutstandingRpcs()) return false;
if (hasMaxOutstandingRpcs()) {
if (getMaxOutstandingRpcs()
!= other.getMaxOutstandingRpcs()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHost()) {
hash = (37 * hash) + HOST_FIELD_NUMBER;
hash = (53 * hash) + getHost().hashCode();
}
if (hasLoadBalancingPolicy()) {
hash = (37 * hash) + LOAD_BALANCING_POLICY_FIELD_NUMBER;
hash = (53 * hash) + loadBalancingPolicy_;
}
if (hasSecurityProtocol()) {
hash = (37 * hash) + SECURITY_PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + getSecurityProtocol().hashCode();
}
if (hasMinSecurityLevel()) {
hash = (37 * hash) + MIN_SECURITY_LEVEL_FIELD_NUMBER;
hash = (53 * hash) + getMinSecurityLevel();
}
if (hasTargetSelectionPolicy()) {
hash = (37 * hash) + TARGET_SELECTION_POLICY_FIELD_NUMBER;
hash = (53 * hash) + targetSelectionPolicy_;
}
if (hasMaxTargets()) {
hash = (37 * hash) + MAX_TARGETS_FIELD_NUMBER;
hash = (53 * hash) + getMaxTargets();
}
if (hasMaxOutstandingRpcs()) {
hash = (37 * hash) + MAX_OUTSTANDING_RPCS_FIELD_NUMBER;
hash = (53 * hash) + getMaxOutstandingRpcs();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes a stubby channel to do a call on.
* Specifies all parameters for selecting the server and establishing connect
* to it.
*
*
* Protobuf type {@code java.apphosting.StubbyTaskRunnerPayload.StubbyChannel}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.StubbyTaskRunnerPayload.StubbyChannel)
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannelOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.class, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
host_ = "";
loadBalancingPolicy_ = 1;
securityProtocol_ = "loas";
minSecurityLevel_ = 0;
targetSelectionPolicy_ = 1;
maxTargets_ = 5;
maxOutstandingRpcs_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel build() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel buildPartial() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel result = new com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.host_ = host_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.loadBalancingPolicy_ = loadBalancingPolicy_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.securityProtocol_ = securityProtocol_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.minSecurityLevel_ = minSecurityLevel_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.targetSelectionPolicy_ = targetSelectionPolicy_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.maxTargets_ = maxTargets_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.maxOutstandingRpcs_ = maxOutstandingRpcs_;
to_bitField0_ |= 0x00000040;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel) {
return mergeFrom((com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel other) {
if (other == com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDefaultInstance()) return this;
if (other.hasHost()) {
host_ = other.host_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasLoadBalancingPolicy()) {
setLoadBalancingPolicy(other.getLoadBalancingPolicy());
}
if (other.hasSecurityProtocol()) {
securityProtocol_ = other.securityProtocol_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasMinSecurityLevel()) {
setMinSecurityLevel(other.getMinSecurityLevel());
}
if (other.hasTargetSelectionPolicy()) {
setTargetSelectionPolicy(other.getTargetSelectionPolicy());
}
if (other.hasMaxTargets()) {
setMaxTargets(other.getMaxTargets());
}
if (other.hasMaxOutstandingRpcs()) {
setMaxOutstandingRpcs(other.getMaxOutstandingRpcs());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasHost()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
host_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy tmpValue =
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(2, tmpRaw);
} else {
loadBalancingPolicy_ = tmpRaw;
bitField0_ |= 0x00000002;
}
break;
} // case 16
case 26: {
securityProtocol_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 32: {
minSecurityLevel_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy tmpValue =
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(5, tmpRaw);
} else {
targetSelectionPolicy_ = tmpRaw;
bitField0_ |= 0x00000010;
}
break;
} // case 40
case 48: {
maxTargets_ = input.readInt32();
bitField0_ |= 0x00000020;
break;
} // case 48
case 56: {
maxOutstandingRpcs_ = input.readInt32();
bitField0_ |= 0x00000040;
break;
} // case 56
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object host_ = "";
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return Whether the host field is set.
*/
public boolean hasHost() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return The host.
*/
public java.lang.String getHost() {
java.lang.Object ref = host_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
host_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return The bytes for host.
*/
public com.google.protobuf.ByteString
getHostBytes() {
java.lang.Object ref = host_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
host_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @param value The host to set.
* @return This builder for chaining.
*/
public Builder setHost(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
host_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @return This builder for chaining.
*/
public Builder clearHost() {
host_ = getDefaultInstance().getHost();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* A comma-separated list of any combination of the following: a BNS job
* prefix, a machine name, or the name of a Chubby file that contains the
* list of machine names.
*
*
* required string host = 1;
* @param value The bytes for host to set.
* @return This builder for chaining.
*/
public Builder setHostBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
host_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int loadBalancingPolicy_ = 1;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy load_balancing_policy = 2 [default = LEAST_LOADED];
* @return Whether the loadBalancingPolicy field is set.
*/
@java.lang.Override public boolean hasLoadBalancingPolicy() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy load_balancing_policy = 2 [default = LEAST_LOADED];
* @return The loadBalancingPolicy.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy getLoadBalancingPolicy() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy.forNumber(loadBalancingPolicy_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy.LEAST_LOADED : result;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy load_balancing_policy = 2 [default = LEAST_LOADED];
* @param value The loadBalancingPolicy to set.
* @return This builder for chaining.
*/
public Builder setLoadBalancingPolicy(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
loadBalancingPolicy_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.LoadBalancingPolicy load_balancing_policy = 2 [default = LEAST_LOADED];
* @return This builder for chaining.
*/
public Builder clearLoadBalancingPolicy() {
bitField0_ = (bitField0_ & ~0x00000002);
loadBalancingPolicy_ = 1;
onChanged();
return this;
}
private java.lang.Object securityProtocol_ = "loas";
/**
* optional string security_protocol = 3 [default = "loas"];
* @return Whether the securityProtocol field is set.
*/
public boolean hasSecurityProtocol() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional string security_protocol = 3 [default = "loas"];
* @return The securityProtocol.
*/
public java.lang.String getSecurityProtocol() {
java.lang.Object ref = securityProtocol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
securityProtocol_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string security_protocol = 3 [default = "loas"];
* @return The bytes for securityProtocol.
*/
public com.google.protobuf.ByteString
getSecurityProtocolBytes() {
java.lang.Object ref = securityProtocol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
securityProtocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string security_protocol = 3 [default = "loas"];
* @param value The securityProtocol to set.
* @return This builder for chaining.
*/
public Builder setSecurityProtocol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
securityProtocol_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional string security_protocol = 3 [default = "loas"];
* @return This builder for chaining.
*/
public Builder clearSecurityProtocol() {
securityProtocol_ = getDefaultInstance().getSecurityProtocol();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* optional string security_protocol = 3 [default = "loas"];
* @param value The bytes for securityProtocol to set.
* @return This builder for chaining.
*/
public Builder setSecurityProtocolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
securityProtocol_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private int minSecurityLevel_ ;
/**
*
* a SSLSecurityLevel value.
*
*
* optional int32 min_security_level = 4;
* @return Whether the minSecurityLevel field is set.
*/
@java.lang.Override
public boolean hasMinSecurityLevel() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* a SSLSecurityLevel value.
*
*
* optional int32 min_security_level = 4;
* @return The minSecurityLevel.
*/
@java.lang.Override
public int getMinSecurityLevel() {
return minSecurityLevel_;
}
/**
*
* a SSLSecurityLevel value.
*
*
* optional int32 min_security_level = 4;
* @param value The minSecurityLevel to set.
* @return This builder for chaining.
*/
public Builder setMinSecurityLevel(int value) {
minSecurityLevel_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* a SSLSecurityLevel value.
*
*
* optional int32 min_security_level = 4;
* @return This builder for chaining.
*/
public Builder clearMinSecurityLevel() {
bitField0_ = (bitField0_ & ~0x00000008);
minSecurityLevel_ = 0;
onChanged();
return this;
}
private int targetSelectionPolicy_ = 1;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy target_selection_policy = 5 [default = NO_FILTER];
* @return Whether the targetSelectionPolicy field is set.
*/
@java.lang.Override public boolean hasTargetSelectionPolicy() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy target_selection_policy = 5 [default = NO_FILTER];
* @return The targetSelectionPolicy.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy getTargetSelectionPolicy() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy.forNumber(targetSelectionPolicy_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy.NO_FILTER : result;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy target_selection_policy = 5 [default = NO_FILTER];
* @param value The targetSelectionPolicy to set.
* @return This builder for chaining.
*/
public Builder setTargetSelectionPolicy(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
targetSelectionPolicy_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel.TargetSelectionPolicy target_selection_policy = 5 [default = NO_FILTER];
* @return This builder for chaining.
*/
public Builder clearTargetSelectionPolicy() {
bitField0_ = (bitField0_ & ~0x00000010);
targetSelectionPolicy_ = 1;
onChanged();
return this;
}
private int maxTargets_ = 5;
/**
*
* When using RANDOM_SUBSET or NETWORK_AWARE, use this as the max_targets
* parameter.
*
*
* optional int32 max_targets = 6 [default = 5];
* @return Whether the maxTargets field is set.
*/
@java.lang.Override
public boolean hasMaxTargets() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* When using RANDOM_SUBSET or NETWORK_AWARE, use this as the max_targets
* parameter.
*
*
* optional int32 max_targets = 6 [default = 5];
* @return The maxTargets.
*/
@java.lang.Override
public int getMaxTargets() {
return maxTargets_;
}
/**
*
* When using RANDOM_SUBSET or NETWORK_AWARE, use this as the max_targets
* parameter.
*
*
* optional int32 max_targets = 6 [default = 5];
* @param value The maxTargets to set.
* @return This builder for chaining.
*/
public Builder setMaxTargets(int value) {
maxTargets_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* When using RANDOM_SUBSET or NETWORK_AWARE, use this as the max_targets
* parameter.
*
*
* optional int32 max_targets = 6 [default = 5];
* @return This builder for chaining.
*/
public Builder clearMaxTargets() {
bitField0_ = (bitField0_ & ~0x00000020);
maxTargets_ = 5;
onChanged();
return this;
}
private int maxOutstandingRpcs_ ;
/**
* optional int32 max_outstanding_rpcs = 7;
* @return Whether the maxOutstandingRpcs field is set.
*/
@java.lang.Override
public boolean hasMaxOutstandingRpcs() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional int32 max_outstanding_rpcs = 7;
* @return The maxOutstandingRpcs.
*/
@java.lang.Override
public int getMaxOutstandingRpcs() {
return maxOutstandingRpcs_;
}
/**
* optional int32 max_outstanding_rpcs = 7;
* @param value The maxOutstandingRpcs to set.
* @return This builder for chaining.
*/
public Builder setMaxOutstandingRpcs(int value) {
maxOutstandingRpcs_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* optional int32 max_outstanding_rpcs = 7;
* @return This builder for chaining.
*/
public Builder clearMaxOutstandingRpcs() {
bitField0_ = (bitField0_ & ~0x00000040);
maxOutstandingRpcs_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.StubbyTaskRunnerPayload.StubbyChannel)
}
// @@protoc_insertion_point(class_scope:java.apphosting.StubbyTaskRunnerPayload.StubbyChannel)
private static final com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel();
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StubbyChannel parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RpcOptionsOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.StubbyTaskRunnerPayload.RpcOptions)
com.google.protobuf.MessageOrBuilder {
/**
* optional double deadline = 1;
* @return Whether the deadline field is set.
*/
boolean hasDeadline();
/**
* optional double deadline = 1;
* @return The deadline.
*/
double getDeadline();
/**
* optional bool fail_fast = 2;
* @return Whether the failFast field is set.
*/
boolean hasFailFast();
/**
* optional bool fail_fast = 2;
* @return The failFast.
*/
boolean getFailFast();
/**
* optional bool duplicate_suppression = 3;
* @return Whether the duplicateSuppression field is set.
*/
boolean hasDuplicateSuppression();
/**
* optional bool duplicate_suppression = 3;
* @return The duplicateSuppression.
*/
boolean getDuplicateSuppression();
/**
* optional uint64 scheduling_hash = 4;
* @return Whether the schedulingHash field is set.
*/
boolean hasSchedulingHash();
/**
* optional uint64 scheduling_hash = 4;
* @return The schedulingHash.
*/
long getSchedulingHash();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol protocol = 5;
* @return Whether the protocol field is set.
*/
boolean hasProtocol();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol protocol = 5;
* @return The protocol.
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol getProtocol();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format response_format = 6;
* @return Whether the responseFormat field is set.
*/
boolean hasResponseFormat();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format response_format = 6;
* @return The responseFormat.
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format getResponseFormat();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format request_format = 7;
* @return Whether the requestFormat field is set.
*/
boolean hasRequestFormat();
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format request_format = 7;
* @return The requestFormat.
*/
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format getRequestFormat();
}
/**
*
* RPC options. For more background, see net/rpc/rpc.h.
*
*
* Protobuf type {@code java.apphosting.StubbyTaskRunnerPayload.RpcOptions}
*/
public static final class RpcOptions extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.StubbyTaskRunnerPayload.RpcOptions)
RpcOptionsOrBuilder {
private static final long serialVersionUID = 0L;
// Use RpcOptions.newBuilder() to construct.
private RpcOptions(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RpcOptions() {
protocol_ = 0;
responseFormat_ = 0;
requestFormat_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RpcOptions();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.class, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol}
*/
public enum RPCProtocol
implements com.google.protobuf.ProtocolMessageEnum {
/**
* TCP = 0;
*/
TCP(0),
/**
* UDP = 1;
*/
UDP(1),
;
/**
* TCP = 0;
*/
public static final int TCP_VALUE = 0;
/**
* UDP = 1;
*/
public static final int UDP_VALUE = 1;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RPCProtocol valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static RPCProtocol forNumber(int value) {
switch (value) {
case 0: return TCP;
case 1: return UDP;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
RPCProtocol> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RPCProtocol findValueByNumber(int number) {
return RPCProtocol.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDescriptor().getEnumTypes().get(0);
}
private static final RPCProtocol[] VALUES = values();
public static RPCProtocol valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private RPCProtocol(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol)
}
/**
* Protobuf enum {@code java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format}
*/
public enum Format
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNCOMPRESSED = 0;
*/
UNCOMPRESSED(0),
/**
* ZIPPY_COMPRESSED = 1;
*/
ZIPPY_COMPRESSED(1),
;
/**
* UNCOMPRESSED = 0;
*/
public static final int UNCOMPRESSED_VALUE = 0;
/**
* ZIPPY_COMPRESSED = 1;
*/
public static final int ZIPPY_COMPRESSED_VALUE = 1;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Format valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Format forNumber(int value) {
switch (value) {
case 0: return UNCOMPRESSED;
case 1: return ZIPPY_COMPRESSED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Format> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Format findValueByNumber(int number) {
return Format.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDescriptor().getEnumTypes().get(1);
}
private static final Format[] VALUES = values();
public static Format valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Format(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format)
}
private int bitField0_;
public static final int DEADLINE_FIELD_NUMBER = 1;
private double deadline_ = 0D;
/**
* optional double deadline = 1;
* @return Whether the deadline field is set.
*/
@java.lang.Override
public boolean hasDeadline() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional double deadline = 1;
* @return The deadline.
*/
@java.lang.Override
public double getDeadline() {
return deadline_;
}
public static final int FAIL_FAST_FIELD_NUMBER = 2;
private boolean failFast_ = false;
/**
* optional bool fail_fast = 2;
* @return Whether the failFast field is set.
*/
@java.lang.Override
public boolean hasFailFast() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bool fail_fast = 2;
* @return The failFast.
*/
@java.lang.Override
public boolean getFailFast() {
return failFast_;
}
public static final int DUPLICATE_SUPPRESSION_FIELD_NUMBER = 3;
private boolean duplicateSuppression_ = false;
/**
* optional bool duplicate_suppression = 3;
* @return Whether the duplicateSuppression field is set.
*/
@java.lang.Override
public boolean hasDuplicateSuppression() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool duplicate_suppression = 3;
* @return The duplicateSuppression.
*/
@java.lang.Override
public boolean getDuplicateSuppression() {
return duplicateSuppression_;
}
public static final int SCHEDULING_HASH_FIELD_NUMBER = 4;
private long schedulingHash_ = 0L;
/**
* optional uint64 scheduling_hash = 4;
* @return Whether the schedulingHash field is set.
*/
@java.lang.Override
public boolean hasSchedulingHash() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional uint64 scheduling_hash = 4;
* @return The schedulingHash.
*/
@java.lang.Override
public long getSchedulingHash() {
return schedulingHash_;
}
public static final int PROTOCOL_FIELD_NUMBER = 5;
private int protocol_ = 0;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol protocol = 5;
* @return Whether the protocol field is set.
*/
@java.lang.Override public boolean hasProtocol() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol protocol = 5;
* @return The protocol.
*/
@java.lang.Override public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol getProtocol() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol.forNumber(protocol_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol.TCP : result;
}
public static final int RESPONSE_FORMAT_FIELD_NUMBER = 6;
private int responseFormat_ = 0;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format response_format = 6;
* @return Whether the responseFormat field is set.
*/
@java.lang.Override public boolean hasResponseFormat() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format response_format = 6;
* @return The responseFormat.
*/
@java.lang.Override public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format getResponseFormat() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.forNumber(responseFormat_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.UNCOMPRESSED : result;
}
public static final int REQUEST_FORMAT_FIELD_NUMBER = 7;
private int requestFormat_ = 0;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format request_format = 7;
* @return Whether the requestFormat field is set.
*/
@java.lang.Override public boolean hasRequestFormat() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format request_format = 7;
* @return The requestFormat.
*/
@java.lang.Override public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format getRequestFormat() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.forNumber(requestFormat_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.UNCOMPRESSED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeDouble(1, deadline_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(2, failFast_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBool(3, duplicateSuppression_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeUInt64(4, schedulingHash_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeEnum(5, protocol_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeEnum(6, responseFormat_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeEnum(7, requestFormat_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(1, deadline_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, failFast_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, duplicateSuppression_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, schedulingHash_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, protocol_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, responseFormat_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, requestFormat_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions other = (com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions) obj;
if (hasDeadline() != other.hasDeadline()) return false;
if (hasDeadline()) {
if (java.lang.Double.doubleToLongBits(getDeadline())
!= java.lang.Double.doubleToLongBits(
other.getDeadline())) return false;
}
if (hasFailFast() != other.hasFailFast()) return false;
if (hasFailFast()) {
if (getFailFast()
!= other.getFailFast()) return false;
}
if (hasDuplicateSuppression() != other.hasDuplicateSuppression()) return false;
if (hasDuplicateSuppression()) {
if (getDuplicateSuppression()
!= other.getDuplicateSuppression()) return false;
}
if (hasSchedulingHash() != other.hasSchedulingHash()) return false;
if (hasSchedulingHash()) {
if (getSchedulingHash()
!= other.getSchedulingHash()) return false;
}
if (hasProtocol() != other.hasProtocol()) return false;
if (hasProtocol()) {
if (protocol_ != other.protocol_) return false;
}
if (hasResponseFormat() != other.hasResponseFormat()) return false;
if (hasResponseFormat()) {
if (responseFormat_ != other.responseFormat_) return false;
}
if (hasRequestFormat() != other.hasRequestFormat()) return false;
if (hasRequestFormat()) {
if (requestFormat_ != other.requestFormat_) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDeadline()) {
hash = (37 * hash) + DEADLINE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getDeadline()));
}
if (hasFailFast()) {
hash = (37 * hash) + FAIL_FAST_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getFailFast());
}
if (hasDuplicateSuppression()) {
hash = (37 * hash) + DUPLICATE_SUPPRESSION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDuplicateSuppression());
}
if (hasSchedulingHash()) {
hash = (37 * hash) + SCHEDULING_HASH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSchedulingHash());
}
if (hasProtocol()) {
hash = (37 * hash) + PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + protocol_;
}
if (hasResponseFormat()) {
hash = (37 * hash) + RESPONSE_FORMAT_FIELD_NUMBER;
hash = (53 * hash) + responseFormat_;
}
if (hasRequestFormat()) {
hash = (37 * hash) + REQUEST_FORMAT_FIELD_NUMBER;
hash = (53 * hash) + requestFormat_;
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* RPC options. For more background, see net/rpc/rpc.h.
*
*
* Protobuf type {@code java.apphosting.StubbyTaskRunnerPayload.RpcOptions}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.StubbyTaskRunnerPayload.RpcOptions)
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptionsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.class, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
deadline_ = 0D;
failFast_ = false;
duplicateSuppression_ = false;
schedulingHash_ = 0L;
protocol_ = 0;
responseFormat_ = 0;
requestFormat_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions build() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions buildPartial() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions result = new com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.deadline_ = deadline_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.failFast_ = failFast_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.duplicateSuppression_ = duplicateSuppression_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.schedulingHash_ = schedulingHash_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.protocol_ = protocol_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.responseFormat_ = responseFormat_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.requestFormat_ = requestFormat_;
to_bitField0_ |= 0x00000040;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions) {
return mergeFrom((com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions other) {
if (other == com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDefaultInstance()) return this;
if (other.hasDeadline()) {
setDeadline(other.getDeadline());
}
if (other.hasFailFast()) {
setFailFast(other.getFailFast());
}
if (other.hasDuplicateSuppression()) {
setDuplicateSuppression(other.getDuplicateSuppression());
}
if (other.hasSchedulingHash()) {
setSchedulingHash(other.getSchedulingHash());
}
if (other.hasProtocol()) {
setProtocol(other.getProtocol());
}
if (other.hasResponseFormat()) {
setResponseFormat(other.getResponseFormat());
}
if (other.hasRequestFormat()) {
setRequestFormat(other.getRequestFormat());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 9: {
deadline_ = input.readDouble();
bitField0_ |= 0x00000001;
break;
} // case 9
case 16: {
failFast_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
duplicateSuppression_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
schedulingHash_ = input.readUInt64();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol tmpValue =
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(5, tmpRaw);
} else {
protocol_ = tmpRaw;
bitField0_ |= 0x00000010;
}
break;
} // case 40
case 48: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format tmpValue =
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(6, tmpRaw);
} else {
responseFormat_ = tmpRaw;
bitField0_ |= 0x00000020;
}
break;
} // case 48
case 56: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format tmpValue =
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(7, tmpRaw);
} else {
requestFormat_ = tmpRaw;
bitField0_ |= 0x00000040;
}
break;
} // case 56
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private double deadline_ ;
/**
* optional double deadline = 1;
* @return Whether the deadline field is set.
*/
@java.lang.Override
public boolean hasDeadline() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional double deadline = 1;
* @return The deadline.
*/
@java.lang.Override
public double getDeadline() {
return deadline_;
}
/**
* optional double deadline = 1;
* @param value The deadline to set.
* @return This builder for chaining.
*/
public Builder setDeadline(double value) {
deadline_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional double deadline = 1;
* @return This builder for chaining.
*/
public Builder clearDeadline() {
bitField0_ = (bitField0_ & ~0x00000001);
deadline_ = 0D;
onChanged();
return this;
}
private boolean failFast_ ;
/**
* optional bool fail_fast = 2;
* @return Whether the failFast field is set.
*/
@java.lang.Override
public boolean hasFailFast() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bool fail_fast = 2;
* @return The failFast.
*/
@java.lang.Override
public boolean getFailFast() {
return failFast_;
}
/**
* optional bool fail_fast = 2;
* @param value The failFast to set.
* @return This builder for chaining.
*/
public Builder setFailFast(boolean value) {
failFast_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional bool fail_fast = 2;
* @return This builder for chaining.
*/
public Builder clearFailFast() {
bitField0_ = (bitField0_ & ~0x00000002);
failFast_ = false;
onChanged();
return this;
}
private boolean duplicateSuppression_ ;
/**
* optional bool duplicate_suppression = 3;
* @return Whether the duplicateSuppression field is set.
*/
@java.lang.Override
public boolean hasDuplicateSuppression() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional bool duplicate_suppression = 3;
* @return The duplicateSuppression.
*/
@java.lang.Override
public boolean getDuplicateSuppression() {
return duplicateSuppression_;
}
/**
* optional bool duplicate_suppression = 3;
* @param value The duplicateSuppression to set.
* @return This builder for chaining.
*/
public Builder setDuplicateSuppression(boolean value) {
duplicateSuppression_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional bool duplicate_suppression = 3;
* @return This builder for chaining.
*/
public Builder clearDuplicateSuppression() {
bitField0_ = (bitField0_ & ~0x00000004);
duplicateSuppression_ = false;
onChanged();
return this;
}
private long schedulingHash_ ;
/**
* optional uint64 scheduling_hash = 4;
* @return Whether the schedulingHash field is set.
*/
@java.lang.Override
public boolean hasSchedulingHash() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional uint64 scheduling_hash = 4;
* @return The schedulingHash.
*/
@java.lang.Override
public long getSchedulingHash() {
return schedulingHash_;
}
/**
* optional uint64 scheduling_hash = 4;
* @param value The schedulingHash to set.
* @return This builder for chaining.
*/
public Builder setSchedulingHash(long value) {
schedulingHash_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional uint64 scheduling_hash = 4;
* @return This builder for chaining.
*/
public Builder clearSchedulingHash() {
bitField0_ = (bitField0_ & ~0x00000008);
schedulingHash_ = 0L;
onChanged();
return this;
}
private int protocol_ = 0;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol protocol = 5;
* @return Whether the protocol field is set.
*/
@java.lang.Override public boolean hasProtocol() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol protocol = 5;
* @return The protocol.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol getProtocol() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol.forNumber(protocol_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol.TCP : result;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol protocol = 5;
* @param value The protocol to set.
* @return This builder for chaining.
*/
public Builder setProtocol(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
protocol_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.RPCProtocol protocol = 5;
* @return This builder for chaining.
*/
public Builder clearProtocol() {
bitField0_ = (bitField0_ & ~0x00000010);
protocol_ = 0;
onChanged();
return this;
}
private int responseFormat_ = 0;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format response_format = 6;
* @return Whether the responseFormat field is set.
*/
@java.lang.Override public boolean hasResponseFormat() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format response_format = 6;
* @return The responseFormat.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format getResponseFormat() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.forNumber(responseFormat_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.UNCOMPRESSED : result;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format response_format = 6;
* @param value The responseFormat to set.
* @return This builder for chaining.
*/
public Builder setResponseFormat(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
responseFormat_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format response_format = 6;
* @return This builder for chaining.
*/
public Builder clearResponseFormat() {
bitField0_ = (bitField0_ & ~0x00000020);
responseFormat_ = 0;
onChanged();
return this;
}
private int requestFormat_ = 0;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format request_format = 7;
* @return Whether the requestFormat field is set.
*/
@java.lang.Override public boolean hasRequestFormat() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format request_format = 7;
* @return The requestFormat.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format getRequestFormat() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format result = com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.forNumber(requestFormat_);
return result == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format.UNCOMPRESSED : result;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format request_format = 7;
* @param value The requestFormat to set.
* @return This builder for chaining.
*/
public Builder setRequestFormat(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Format value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
requestFormat_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions.Format request_format = 7;
* @return This builder for chaining.
*/
public Builder clearRequestFormat() {
bitField0_ = (bitField0_ & ~0x00000040);
requestFormat_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.StubbyTaskRunnerPayload.RpcOptions)
}
// @@protoc_insertion_point(class_scope:java.apphosting.StubbyTaskRunnerPayload.RpcOptions)
private static final com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions();
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RpcOptions parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int CHANNEL_FIELD_NUMBER = 1;
private com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel channel_;
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
* @return Whether the channel field is set.
*/
@java.lang.Override
public boolean hasChannel() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
* @return The channel.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel getChannel() {
return channel_ == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDefaultInstance() : channel_;
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannelOrBuilder getChannelOrBuilder() {
return channel_ == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDefaultInstance() : channel_;
}
public static final int METHOD_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object method_ = "";
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return Whether the method field is set.
*/
@java.lang.Override
public boolean hasMethod() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return The method.
*/
@java.lang.Override
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
method_ = s;
}
return s;
}
}
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return The bytes for method.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString request_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Protocol buffers to send to Method. Should be in binary.
* NB: proto2 doesn't support RawMessage.
*
*
* required bytes request = 3 [ctype = CORD];
* @return Whether the request field is set.
*/
@java.lang.Override
public boolean hasRequest() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Protocol buffers to send to Method. Should be in binary.
* NB: proto2 doesn't support RawMessage.
*
*
* required bytes request = 3 [ctype = CORD];
* @return The request.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRequest() {
return request_;
}
public static final int RPC_OPTIONS_FIELD_NUMBER = 4;
private com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions rpcOptions_;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
* @return Whether the rpcOptions field is set.
*/
@java.lang.Override
public boolean hasRpcOptions() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
* @return The rpcOptions.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions getRpcOptions() {
return rpcOptions_ == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDefaultInstance() : rpcOptions_;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptionsOrBuilder getRpcOptionsOrBuilder() {
return rpcOptions_ == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDefaultInstance() : rpcOptions_;
}
public static final int REQUEST_TASK_DEFINITION_FIELD_NUMBER = 5;
private boolean requestTaskDefinition_ = false;
/**
*
* If true, the enclosing task definition will be sent to Method,
* including this StubbyTaskRunnerPayload payload.
*
*
* optional bool request_task_definition = 5 [default = false];
* @return Whether the requestTaskDefinition field is set.
*/
@java.lang.Override
public boolean hasRequestTaskDefinition() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* If true, the enclosing task definition will be sent to Method,
* including this StubbyTaskRunnerPayload payload.
*
*
* optional bool request_task_definition = 5 [default = false];
* @return The requestTaskDefinition.
*/
@java.lang.Override
public boolean getRequestTaskDefinition() {
return requestTaskDefinition_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasChannel()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMethod()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasRequest()) {
memoizedIsInitialized = 0;
return false;
}
if (!getChannel().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getChannel());
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, method_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, request_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(4, getRpcOptions());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(5, requestTaskDefinition_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getChannel());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, method_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, request_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getRpcOptions());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, requestTaskDefinition_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.StubbyTaskRunnerPayload)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload other = (com.google.apphosting.executor.Task.StubbyTaskRunnerPayload) obj;
if (hasChannel() != other.hasChannel()) return false;
if (hasChannel()) {
if (!getChannel()
.equals(other.getChannel())) return false;
}
if (hasMethod() != other.hasMethod()) return false;
if (hasMethod()) {
if (!getMethod()
.equals(other.getMethod())) return false;
}
if (hasRequest() != other.hasRequest()) return false;
if (hasRequest()) {
if (!getRequest()
.equals(other.getRequest())) return false;
}
if (hasRpcOptions() != other.hasRpcOptions()) return false;
if (hasRpcOptions()) {
if (!getRpcOptions()
.equals(other.getRpcOptions())) return false;
}
if (hasRequestTaskDefinition() != other.hasRequestTaskDefinition()) return false;
if (hasRequestTaskDefinition()) {
if (getRequestTaskDefinition()
!= other.getRequestTaskDefinition()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasChannel()) {
hash = (37 * hash) + CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getChannel().hashCode();
}
if (hasMethod()) {
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + getMethod().hashCode();
}
if (hasRequest()) {
hash = (37 * hash) + REQUEST_FIELD_NUMBER;
hash = (53 * hash) + getRequest().hashCode();
}
if (hasRpcOptions()) {
hash = (37 * hash) + RPC_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getRpcOptions().hashCode();
}
if (hasRequestTaskDefinition()) {
hash = (37 * hash) + REQUEST_TASK_DEFINITION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRequestTaskDefinition());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Payload type for StubbyTaskRunner.
* Initially based on apphosting/api/stubby/stubby_service.proto
*
*
* Protobuf type {@code java.apphosting.StubbyTaskRunnerPayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.StubbyTaskRunnerPayload)
com.google.apphosting.executor.Task.StubbyTaskRunnerPayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.class, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getChannelFieldBuilder();
getRpcOptionsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
channel_ = null;
if (channelBuilder_ != null) {
channelBuilder_.dispose();
channelBuilder_ = null;
}
method_ = "";
request_ = com.google.protobuf.ByteString.EMPTY;
rpcOptions_ = null;
if (rpcOptionsBuilder_ != null) {
rpcOptionsBuilder_.dispose();
rpcOptionsBuilder_ = null;
}
requestTaskDefinition_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerPayload_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload build() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload buildPartial() {
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload result = new com.google.apphosting.executor.Task.StubbyTaskRunnerPayload(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.channel_ = channelBuilder_ == null
? channel_
: channelBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.method_ = method_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.request_ = request_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.rpcOptions_ = rpcOptionsBuilder_ == null
? rpcOptions_
: rpcOptionsBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.requestTaskDefinition_ = requestTaskDefinition_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.StubbyTaskRunnerPayload) {
return mergeFrom((com.google.apphosting.executor.Task.StubbyTaskRunnerPayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload other) {
if (other == com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.getDefaultInstance()) return this;
if (other.hasChannel()) {
mergeChannel(other.getChannel());
}
if (other.hasMethod()) {
method_ = other.method_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasRequest()) {
setRequest(other.getRequest());
}
if (other.hasRpcOptions()) {
mergeRpcOptions(other.getRpcOptions());
}
if (other.hasRequestTaskDefinition()) {
setRequestTaskDefinition(other.getRequestTaskDefinition());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasChannel()) {
return false;
}
if (!hasMethod()) {
return false;
}
if (!hasRequest()) {
return false;
}
if (!getChannel().isInitialized()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getChannelFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
method_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
request_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getRpcOptionsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 40: {
requestTaskDefinition_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 40
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel channel_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.Builder, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannelOrBuilder> channelBuilder_;
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
* @return Whether the channel field is set.
*/
public boolean hasChannel() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
* @return The channel.
*/
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel getChannel() {
if (channelBuilder_ == null) {
return channel_ == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDefaultInstance() : channel_;
} else {
return channelBuilder_.getMessage();
}
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
public Builder setChannel(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel value) {
if (channelBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
channel_ = value;
} else {
channelBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
public Builder setChannel(
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.Builder builderForValue) {
if (channelBuilder_ == null) {
channel_ = builderForValue.build();
} else {
channelBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
public Builder mergeChannel(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel value) {
if (channelBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
channel_ != null &&
channel_ != com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDefaultInstance()) {
getChannelBuilder().mergeFrom(value);
} else {
channel_ = value;
}
} else {
channelBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
public Builder clearChannel() {
bitField0_ = (bitField0_ & ~0x00000001);
channel_ = null;
if (channelBuilder_ != null) {
channelBuilder_.dispose();
channelBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.Builder getChannelBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getChannelFieldBuilder().getBuilder();
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannelOrBuilder getChannelOrBuilder() {
if (channelBuilder_ != null) {
return channelBuilder_.getMessageOrBuilder();
} else {
return channel_ == null ?
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.getDefaultInstance() : channel_;
}
}
/**
*
* Stubby channel to do a call on.
*
*
* required .java.apphosting.StubbyTaskRunnerPayload.StubbyChannel channel = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.Builder, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannelOrBuilder>
getChannelFieldBuilder() {
if (channelBuilder_ == null) {
channelBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannel.Builder, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.StubbyChannelOrBuilder>(
getChannel(),
getParentForChildren(),
isClean());
channel_ = null;
}
return channelBuilder_;
}
private java.lang.Object method_ = "";
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return Whether the method field is set.
*/
public boolean hasMethod() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return The method.
*/
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
method_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return The bytes for method.
*/
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @param value The method to set.
* @return This builder for chaining.
*/
public Builder setMethod(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
method_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @return This builder for chaining.
*/
public Builder clearMethod() {
method_ = getDefaultInstance().getMethod();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* String in the form: "/Server.Method" to indicate which method on which
* service to invoke.
*
*
* required string method = 2;
* @param value The bytes for method to set.
* @return This builder for chaining.
*/
public Builder setMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
method_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.ByteString request_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Protocol buffers to send to Method. Should be in binary.
* NB: proto2 doesn't support RawMessage.
*
*
* required bytes request = 3 [ctype = CORD];
* @return Whether the request field is set.
*/
@java.lang.Override
public boolean hasRequest() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Protocol buffers to send to Method. Should be in binary.
* NB: proto2 doesn't support RawMessage.
*
*
* required bytes request = 3 [ctype = CORD];
* @return The request.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRequest() {
return request_;
}
/**
*
* Protocol buffers to send to Method. Should be in binary.
* NB: proto2 doesn't support RawMessage.
*
*
* required bytes request = 3 [ctype = CORD];
* @param value The request to set.
* @return This builder for chaining.
*/
public Builder setRequest(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
request_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Protocol buffers to send to Method. Should be in binary.
* NB: proto2 doesn't support RawMessage.
*
*
* required bytes request = 3 [ctype = CORD];
* @return This builder for chaining.
*/
public Builder clearRequest() {
bitField0_ = (bitField0_ & ~0x00000004);
request_ = getDefaultInstance().getRequest();
onChanged();
return this;
}
private com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions rpcOptions_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Builder, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptionsOrBuilder> rpcOptionsBuilder_;
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
* @return Whether the rpcOptions field is set.
*/
public boolean hasRpcOptions() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
* @return The rpcOptions.
*/
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions getRpcOptions() {
if (rpcOptionsBuilder_ == null) {
return rpcOptions_ == null ? com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDefaultInstance() : rpcOptions_;
} else {
return rpcOptionsBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
public Builder setRpcOptions(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions value) {
if (rpcOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
rpcOptions_ = value;
} else {
rpcOptionsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
public Builder setRpcOptions(
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Builder builderForValue) {
if (rpcOptionsBuilder_ == null) {
rpcOptions_ = builderForValue.build();
} else {
rpcOptionsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
public Builder mergeRpcOptions(com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions value) {
if (rpcOptionsBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
rpcOptions_ != null &&
rpcOptions_ != com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDefaultInstance()) {
getRpcOptionsBuilder().mergeFrom(value);
} else {
rpcOptions_ = value;
}
} else {
rpcOptionsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
public Builder clearRpcOptions() {
bitField0_ = (bitField0_ & ~0x00000008);
rpcOptions_ = null;
if (rpcOptionsBuilder_ != null) {
rpcOptionsBuilder_.dispose();
rpcOptionsBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Builder getRpcOptionsBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getRpcOptionsFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptionsOrBuilder getRpcOptionsOrBuilder() {
if (rpcOptionsBuilder_ != null) {
return rpcOptionsBuilder_.getMessageOrBuilder();
} else {
return rpcOptions_ == null ?
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.getDefaultInstance() : rpcOptions_;
}
}
/**
* optional .java.apphosting.StubbyTaskRunnerPayload.RpcOptions rpc_options = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Builder, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptionsOrBuilder>
getRpcOptionsFieldBuilder() {
if (rpcOptionsBuilder_ == null) {
rpcOptionsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptions.Builder, com.google.apphosting.executor.Task.StubbyTaskRunnerPayload.RpcOptionsOrBuilder>(
getRpcOptions(),
getParentForChildren(),
isClean());
rpcOptions_ = null;
}
return rpcOptionsBuilder_;
}
private boolean requestTaskDefinition_ ;
/**
*
* If true, the enclosing task definition will be sent to Method,
* including this StubbyTaskRunnerPayload payload.
*
*
* optional bool request_task_definition = 5 [default = false];
* @return Whether the requestTaskDefinition field is set.
*/
@java.lang.Override
public boolean hasRequestTaskDefinition() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* If true, the enclosing task definition will be sent to Method,
* including this StubbyTaskRunnerPayload payload.
*
*
* optional bool request_task_definition = 5 [default = false];
* @return The requestTaskDefinition.
*/
@java.lang.Override
public boolean getRequestTaskDefinition() {
return requestTaskDefinition_;
}
/**
*
* If true, the enclosing task definition will be sent to Method,
* including this StubbyTaskRunnerPayload payload.
*
*
* optional bool request_task_definition = 5 [default = false];
* @param value The requestTaskDefinition to set.
* @return This builder for chaining.
*/
public Builder setRequestTaskDefinition(boolean value) {
requestTaskDefinition_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* If true, the enclosing task definition will be sent to Method,
* including this StubbyTaskRunnerPayload payload.
*
*
* optional bool request_task_definition = 5 [default = false];
* @return This builder for chaining.
*/
public Builder clearRequestTaskDefinition() {
bitField0_ = (bitField0_ & ~0x00000010);
requestTaskDefinition_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.StubbyTaskRunnerPayload)
}
// @@protoc_insertion_point(class_scope:java.apphosting.StubbyTaskRunnerPayload)
private static final com.google.apphosting.executor.Task.StubbyTaskRunnerPayload DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.StubbyTaskRunnerPayload();
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerPayload getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StubbyTaskRunnerPayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerPayload getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StubbyTaskRunnerInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.StubbyTaskRunnerInfo)
com.google.protobuf.MessageOrBuilder {
/**
*
* RPC status
*
*
* required int64 status = 1;
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
*
* RPC status
*
*
* required int64 status = 1;
* @return The status.
*/
long getStatus();
/**
*
* Application error, if any.
*
*
* optional int64 application_error = 2;
* @return Whether the applicationError field is set.
*/
boolean hasApplicationError();
/**
*
* Application error, if any.
*
*
* optional int64 application_error = 2;
* @return The applicationError.
*/
long getApplicationError();
/**
*
* Error message, if any.
*
*
* optional bytes error_message = 3;
* @return Whether the errorMessage field is set.
*/
boolean hasErrorMessage();
/**
*
* Error message, if any.
*
*
* optional bytes error_message = 3;
* @return The errorMessage.
*/
com.google.protobuf.ByteString getErrorMessage();
}
/**
*
* Statistics for StubbyTaskRunner.
*
*
* Protobuf type {@code java.apphosting.StubbyTaskRunnerInfo}
*/
public static final class StubbyTaskRunnerInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.StubbyTaskRunnerInfo)
StubbyTaskRunnerInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use StubbyTaskRunnerInfo.newBuilder() to construct.
private StubbyTaskRunnerInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StubbyTaskRunnerInfo() {
errorMessage_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new StubbyTaskRunnerInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.StubbyTaskRunnerInfo.class, com.google.apphosting.executor.Task.StubbyTaskRunnerInfo.Builder.class);
}
private int bitField0_;
public static final int STATUS_FIELD_NUMBER = 1;
private long status_ = 0L;
/**
*
* RPC status
*
*
* required int64 status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* RPC status
*
*
* required int64 status = 1;
* @return The status.
*/
@java.lang.Override
public long getStatus() {
return status_;
}
public static final int APPLICATION_ERROR_FIELD_NUMBER = 2;
private long applicationError_ = 0L;
/**
*
* Application error, if any.
*
*
* optional int64 application_error = 2;
* @return Whether the applicationError field is set.
*/
@java.lang.Override
public boolean hasApplicationError() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Application error, if any.
*
*
* optional int64 application_error = 2;
* @return The applicationError.
*/
@java.lang.Override
public long getApplicationError() {
return applicationError_;
}
public static final int ERROR_MESSAGE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString errorMessage_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Error message, if any.
*
*
* optional bytes error_message = 3;
* @return Whether the errorMessage field is set.
*/
@java.lang.Override
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Error message, if any.
*
*
* optional bytes error_message = 3;
* @return The errorMessage.
*/
@java.lang.Override
public com.google.protobuf.ByteString getErrorMessage() {
return errorMessage_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasStatus()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, status_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, applicationError_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(3, errorMessage_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, status_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, applicationError_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, errorMessage_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.StubbyTaskRunnerInfo)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.StubbyTaskRunnerInfo other = (com.google.apphosting.executor.Task.StubbyTaskRunnerInfo) obj;
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (getStatus()
!= other.getStatus()) return false;
}
if (hasApplicationError() != other.hasApplicationError()) return false;
if (hasApplicationError()) {
if (getApplicationError()
!= other.getApplicationError()) return false;
}
if (hasErrorMessage() != other.hasErrorMessage()) return false;
if (hasErrorMessage()) {
if (!getErrorMessage()
.equals(other.getErrorMessage())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStatus());
}
if (hasApplicationError()) {
hash = (37 * hash) + APPLICATION_ERROR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getApplicationError());
}
if (hasErrorMessage()) {
hash = (37 * hash) + ERROR_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getErrorMessage().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.StubbyTaskRunnerInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Statistics for StubbyTaskRunner.
*
*
* Protobuf type {@code java.apphosting.StubbyTaskRunnerInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.StubbyTaskRunnerInfo)
com.google.apphosting.executor.Task.StubbyTaskRunnerInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.StubbyTaskRunnerInfo.class, com.google.apphosting.executor.Task.StubbyTaskRunnerInfo.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.StubbyTaskRunnerInfo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
status_ = 0L;
applicationError_ = 0L;
errorMessage_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_StubbyTaskRunnerInfo_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerInfo getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.StubbyTaskRunnerInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerInfo build() {
com.google.apphosting.executor.Task.StubbyTaskRunnerInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerInfo buildPartial() {
com.google.apphosting.executor.Task.StubbyTaskRunnerInfo result = new com.google.apphosting.executor.Task.StubbyTaskRunnerInfo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.StubbyTaskRunnerInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.status_ = status_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.applicationError_ = applicationError_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.errorMessage_ = errorMessage_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.StubbyTaskRunnerInfo) {
return mergeFrom((com.google.apphosting.executor.Task.StubbyTaskRunnerInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.StubbyTaskRunnerInfo other) {
if (other == com.google.apphosting.executor.Task.StubbyTaskRunnerInfo.getDefaultInstance()) return this;
if (other.hasStatus()) {
setStatus(other.getStatus());
}
if (other.hasApplicationError()) {
setApplicationError(other.getApplicationError());
}
if (other.hasErrorMessage()) {
setErrorMessage(other.getErrorMessage());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasStatus()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
status_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
applicationError_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
errorMessage_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long status_ ;
/**
*
* RPC status
*
*
* required int64 status = 1;
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* RPC status
*
*
* required int64 status = 1;
* @return The status.
*/
@java.lang.Override
public long getStatus() {
return status_;
}
/**
*
* RPC status
*
*
* required int64 status = 1;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(long value) {
status_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* RPC status
*
*
* required int64 status = 1;
* @return This builder for chaining.
*/
public Builder clearStatus() {
bitField0_ = (bitField0_ & ~0x00000001);
status_ = 0L;
onChanged();
return this;
}
private long applicationError_ ;
/**
*
* Application error, if any.
*
*
* optional int64 application_error = 2;
* @return Whether the applicationError field is set.
*/
@java.lang.Override
public boolean hasApplicationError() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Application error, if any.
*
*
* optional int64 application_error = 2;
* @return The applicationError.
*/
@java.lang.Override
public long getApplicationError() {
return applicationError_;
}
/**
*
* Application error, if any.
*
*
* optional int64 application_error = 2;
* @param value The applicationError to set.
* @return This builder for chaining.
*/
public Builder setApplicationError(long value) {
applicationError_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Application error, if any.
*
*
* optional int64 application_error = 2;
* @return This builder for chaining.
*/
public Builder clearApplicationError() {
bitField0_ = (bitField0_ & ~0x00000002);
applicationError_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString errorMessage_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Error message, if any.
*
*
* optional bytes error_message = 3;
* @return Whether the errorMessage field is set.
*/
@java.lang.Override
public boolean hasErrorMessage() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Error message, if any.
*
*
* optional bytes error_message = 3;
* @return The errorMessage.
*/
@java.lang.Override
public com.google.protobuf.ByteString getErrorMessage() {
return errorMessage_;
}
/**
*
* Error message, if any.
*
*
* optional bytes error_message = 3;
* @param value The errorMessage to set.
* @return This builder for chaining.
*/
public Builder setErrorMessage(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
errorMessage_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Error message, if any.
*
*
* optional bytes error_message = 3;
* @return This builder for chaining.
*/
public Builder clearErrorMessage() {
bitField0_ = (bitField0_ & ~0x00000004);
errorMessage_ = getDefaultInstance().getErrorMessage();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.StubbyTaskRunnerInfo)
}
// @@protoc_insertion_point(class_scope:java.apphosting.StubbyTaskRunnerInfo)
private static final com.google.apphosting.executor.Task.StubbyTaskRunnerInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.StubbyTaskRunnerInfo();
}
public static com.google.apphosting.executor.Task.StubbyTaskRunnerInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StubbyTaskRunnerInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.StubbyTaskRunnerInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PullTaskPayloadOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.PullTaskPayload)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes content = 1 [ctype = CORD];
* @return Whether the content field is set.
*/
boolean hasContent();
/**
* required bytes content = 1 [ctype = CORD];
* @return The content.
*/
com.google.protobuf.ByteString getContent();
}
/**
*
* Payload for a pull task. The worker pulling the task is the runner and knows
* how to interpret its own payloads. We just have a bytes field that can be
* interpreted as a protocol buffer, for example.
*
*
* Protobuf type {@code java.apphosting.PullTaskPayload}
*/
public static final class PullTaskPayload extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.PullTaskPayload)
PullTaskPayloadOrBuilder {
private static final long serialVersionUID = 0L;
// Use PullTaskPayload.newBuilder() to construct.
private PullTaskPayload(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PullTaskPayload() {
content_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PullTaskPayload();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PullTaskPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PullTaskPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.PullTaskPayload.class, com.google.apphosting.executor.Task.PullTaskPayload.Builder.class);
}
private int bitField0_;
public static final int CONTENT_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString content_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes content = 1 [ctype = CORD];
* @return Whether the content field is set.
*/
@java.lang.Override
public boolean hasContent() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes content = 1 [ctype = CORD];
* @return The content.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContent() {
return content_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasContent()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, content_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, content_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.PullTaskPayload)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.PullTaskPayload other = (com.google.apphosting.executor.Task.PullTaskPayload) obj;
if (hasContent() != other.hasContent()) return false;
if (hasContent()) {
if (!getContent()
.equals(other.getContent())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasContent()) {
hash = (37 * hash) + CONTENT_FIELD_NUMBER;
hash = (53 * hash) + getContent().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PullTaskPayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.PullTaskPayload prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Payload for a pull task. The worker pulling the task is the runner and knows
* how to interpret its own payloads. We just have a bytes field that can be
* interpreted as a protocol buffer, for example.
*
*
* Protobuf type {@code java.apphosting.PullTaskPayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.PullTaskPayload)
com.google.apphosting.executor.Task.PullTaskPayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PullTaskPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PullTaskPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.PullTaskPayload.class, com.google.apphosting.executor.Task.PullTaskPayload.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.PullTaskPayload.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
content_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PullTaskPayload_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PullTaskPayload getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.PullTaskPayload.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.PullTaskPayload build() {
com.google.apphosting.executor.Task.PullTaskPayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PullTaskPayload buildPartial() {
com.google.apphosting.executor.Task.PullTaskPayload result = new com.google.apphosting.executor.Task.PullTaskPayload(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.PullTaskPayload result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.content_ = content_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.PullTaskPayload) {
return mergeFrom((com.google.apphosting.executor.Task.PullTaskPayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.PullTaskPayload other) {
if (other == com.google.apphosting.executor.Task.PullTaskPayload.getDefaultInstance()) return this;
if (other.hasContent()) {
setContent(other.getContent());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasContent()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
content_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString content_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes content = 1 [ctype = CORD];
* @return Whether the content field is set.
*/
@java.lang.Override
public boolean hasContent() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes content = 1 [ctype = CORD];
* @return The content.
*/
@java.lang.Override
public com.google.protobuf.ByteString getContent() {
return content_;
}
/**
* required bytes content = 1 [ctype = CORD];
* @param value The content to set.
* @return This builder for chaining.
*/
public Builder setContent(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
content_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required bytes content = 1 [ctype = CORD];
* @return This builder for chaining.
*/
public Builder clearContent() {
bitField0_ = (bitField0_ & ~0x00000001);
content_ = getDefaultInstance().getContent();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.PullTaskPayload)
}
// @@protoc_insertion_point(class_scope:java.apphosting.PullTaskPayload)
private static final com.google.apphosting.executor.Task.PullTaskPayload DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.PullTaskPayload();
}
public static com.google.apphosting.executor.Task.PullTaskPayload getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PullTaskPayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PullTaskPayload getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PubsubTaskRunnerPayloadOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.PubsubTaskRunnerPayload)
com.google.protobuf.MessageOrBuilder {
/**
*
* The payload should be a serialized [google.pubsub.v1beta2.PubsubMessage][].
*
*
* optional bytes pubsub_message = 1 [ctype = CORD];
* @return Whether the pubsubMessage field is set.
*/
boolean hasPubsubMessage();
/**
*
* The payload should be a serialized [google.pubsub.v1beta2.PubsubMessage][].
*
*
* optional bytes pubsub_message = 1 [ctype = CORD];
* @return The pubsubMessage.
*/
com.google.protobuf.ByteString getPubsubMessage();
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return Whether the internalTopicName field is set.
*/
boolean hasInternalTopicName();
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return The internalTopicName.
*/
java.lang.String getInternalTopicName();
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return The bytes for internalTopicName.
*/
com.google.protobuf.ByteString
getInternalTopicNameBytes();
}
/**
*
* Payload for a pub sub task. The topic name to post to comes from the queue
* definition.
*
*
* Protobuf type {@code java.apphosting.PubsubTaskRunnerPayload}
*/
public static final class PubsubTaskRunnerPayload extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.PubsubTaskRunnerPayload)
PubsubTaskRunnerPayloadOrBuilder {
private static final long serialVersionUID = 0L;
// Use PubsubTaskRunnerPayload.newBuilder() to construct.
private PubsubTaskRunnerPayload(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PubsubTaskRunnerPayload() {
pubsubMessage_ = com.google.protobuf.ByteString.EMPTY;
internalTopicName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PubsubTaskRunnerPayload();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.PubsubTaskRunnerPayload.class, com.google.apphosting.executor.Task.PubsubTaskRunnerPayload.Builder.class);
}
private int bitField0_;
public static final int PUBSUB_MESSAGE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString pubsubMessage_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The payload should be a serialized [google.pubsub.v1beta2.PubsubMessage][].
*
*
* optional bytes pubsub_message = 1 [ctype = CORD];
* @return Whether the pubsubMessage field is set.
*/
@java.lang.Override
public boolean hasPubsubMessage() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The payload should be a serialized [google.pubsub.v1beta2.PubsubMessage][].
*
*
* optional bytes pubsub_message = 1 [ctype = CORD];
* @return The pubsubMessage.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPubsubMessage() {
return pubsubMessage_;
}
public static final int INTERNAL_TOPIC_NAME_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object internalTopicName_ = "";
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return Whether the internalTopicName field is set.
*/
@java.lang.Override
public boolean hasInternalTopicName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return The internalTopicName.
*/
@java.lang.Override
public java.lang.String getInternalTopicName() {
java.lang.Object ref = internalTopicName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
internalTopicName_ = s;
}
return s;
}
}
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return The bytes for internalTopicName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInternalTopicNameBytes() {
java.lang.Object ref = internalTopicName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
internalTopicName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, pubsubMessage_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, internalTopicName_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, pubsubMessage_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, internalTopicName_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.PubsubTaskRunnerPayload)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.PubsubTaskRunnerPayload other = (com.google.apphosting.executor.Task.PubsubTaskRunnerPayload) obj;
if (hasPubsubMessage() != other.hasPubsubMessage()) return false;
if (hasPubsubMessage()) {
if (!getPubsubMessage()
.equals(other.getPubsubMessage())) return false;
}
if (hasInternalTopicName() != other.hasInternalTopicName()) return false;
if (hasInternalTopicName()) {
if (!getInternalTopicName()
.equals(other.getInternalTopicName())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPubsubMessage()) {
hash = (37 * hash) + PUBSUB_MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getPubsubMessage().hashCode();
}
if (hasInternalTopicName()) {
hash = (37 * hash) + INTERNAL_TOPIC_NAME_FIELD_NUMBER;
hash = (53 * hash) + getInternalTopicName().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.PubsubTaskRunnerPayload prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Payload for a pub sub task. The topic name to post to comes from the queue
* definition.
*
*
* Protobuf type {@code java.apphosting.PubsubTaskRunnerPayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.PubsubTaskRunnerPayload)
com.google.apphosting.executor.Task.PubsubTaskRunnerPayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.PubsubTaskRunnerPayload.class, com.google.apphosting.executor.Task.PubsubTaskRunnerPayload.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.PubsubTaskRunnerPayload.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
pubsubMessage_ = com.google.protobuf.ByteString.EMPTY;
internalTopicName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_PubsubTaskRunnerPayload_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PubsubTaskRunnerPayload getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.PubsubTaskRunnerPayload.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.PubsubTaskRunnerPayload build() {
com.google.apphosting.executor.Task.PubsubTaskRunnerPayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PubsubTaskRunnerPayload buildPartial() {
com.google.apphosting.executor.Task.PubsubTaskRunnerPayload result = new com.google.apphosting.executor.Task.PubsubTaskRunnerPayload(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.PubsubTaskRunnerPayload result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.pubsubMessage_ = pubsubMessage_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.internalTopicName_ = internalTopicName_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.PubsubTaskRunnerPayload) {
return mergeFrom((com.google.apphosting.executor.Task.PubsubTaskRunnerPayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.PubsubTaskRunnerPayload other) {
if (other == com.google.apphosting.executor.Task.PubsubTaskRunnerPayload.getDefaultInstance()) return this;
if (other.hasPubsubMessage()) {
setPubsubMessage(other.getPubsubMessage());
}
if (other.hasInternalTopicName()) {
internalTopicName_ = other.internalTopicName_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
pubsubMessage_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
internalTopicName_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString pubsubMessage_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The payload should be a serialized [google.pubsub.v1beta2.PubsubMessage][].
*
*
* optional bytes pubsub_message = 1 [ctype = CORD];
* @return Whether the pubsubMessage field is set.
*/
@java.lang.Override
public boolean hasPubsubMessage() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The payload should be a serialized [google.pubsub.v1beta2.PubsubMessage][].
*
*
* optional bytes pubsub_message = 1 [ctype = CORD];
* @return The pubsubMessage.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPubsubMessage() {
return pubsubMessage_;
}
/**
*
* The payload should be a serialized [google.pubsub.v1beta2.PubsubMessage][].
*
*
* optional bytes pubsub_message = 1 [ctype = CORD];
* @param value The pubsubMessage to set.
* @return This builder for chaining.
*/
public Builder setPubsubMessage(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
pubsubMessage_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The payload should be a serialized [google.pubsub.v1beta2.PubsubMessage][].
*
*
* optional bytes pubsub_message = 1 [ctype = CORD];
* @return This builder for chaining.
*/
public Builder clearPubsubMessage() {
bitField0_ = (bitField0_ & ~0x00000001);
pubsubMessage_ = getDefaultInstance().getPubsubMessage();
onChanged();
return this;
}
private java.lang.Object internalTopicName_ = "";
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return Whether the internalTopicName field is set.
*/
public boolean hasInternalTopicName() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return The internalTopicName.
*/
public java.lang.String getInternalTopicName() {
java.lang.Object ref = internalTopicName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
internalTopicName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return The bytes for internalTopicName.
*/
public com.google.protobuf.ByteString
getInternalTopicNameBytes() {
java.lang.Object ref = internalTopicName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
internalTopicName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @param value The internalTopicName to set.
* @return This builder for chaining.
*/
public Builder setInternalTopicName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
internalTopicName_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @return This builder for chaining.
*/
public Builder clearInternalTopicName() {
internalTopicName_ = getDefaultInstance().getInternalTopicName();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Internal queues (like cron queues) are allowed to send pubsub messages to
* multiple topics and must set the topic name per task. This field is
* required for cron tasks, but will be ignored for tasks belonging to regular
* queues.
*
*
* optional string internal_topic_name = 2;
* @param value The bytes for internalTopicName to set.
* @return This builder for chaining.
*/
public Builder setInternalTopicNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
internalTopicName_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.PubsubTaskRunnerPayload)
}
// @@protoc_insertion_point(class_scope:java.apphosting.PubsubTaskRunnerPayload)
private static final com.google.apphosting.executor.Task.PubsubTaskRunnerPayload DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.PubsubTaskRunnerPayload();
}
public static com.google.apphosting.executor.Task.PubsubTaskRunnerPayload getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PubsubTaskRunnerPayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.PubsubTaskRunnerPayload getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FunctionTaskRunnerPayloadOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.FunctionTaskRunnerPayload)
com.google.protobuf.MessageOrBuilder {
/**
* optional .java.apphosting.FunctionTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return Whether the method field is set.
*/
boolean hasMethod();
/**
* optional .java.apphosting.FunctionTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return The method.
*/
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod getMethod();
/**
* optional bytes url = 2;
* @return Whether the url field is set.
*/
boolean hasUrl();
/**
* optional bytes url = 2;
* @return The url.
*/
com.google.protobuf.ByteString getUrl();
/**
* repeated group Header = 3 { ... }
*/
java.util.List
getHeaderList();
/**
* repeated group Header = 3 { ... }
*/
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header getHeader(int index);
/**
* repeated group Header = 3 { ... }
*/
int getHeaderCount();
/**
* repeated group Header = 3 { ... }
*/
java.util.List extends com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList();
/**
* repeated group Header = 3 { ... }
*/
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index);
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return Whether the body field is set.
*/
boolean hasBody();
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return The body.
*/
com.google.protobuf.ByteString getBody();
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return Whether the functionName field is set.
*/
boolean hasFunctionName();
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return The functionName.
*/
java.lang.String getFunctionName();
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return The bytes for functionName.
*/
com.google.protobuf.ByteString
getFunctionNameBytes();
}
/**
* Protobuf type {@code java.apphosting.FunctionTaskRunnerPayload}
*/
public static final class FunctionTaskRunnerPayload extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.FunctionTaskRunnerPayload)
FunctionTaskRunnerPayloadOrBuilder {
private static final long serialVersionUID = 0L;
// Use FunctionTaskRunnerPayload.newBuilder() to construct.
private FunctionTaskRunnerPayload(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FunctionTaskRunnerPayload() {
method_ = 2;
url_ = com.google.protobuf.ByteString.EMPTY;
header_ = java.util.Collections.emptyList();
body_ = com.google.protobuf.ByteString.EMPTY;
functionName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FunctionTaskRunnerPayload();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.class, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.FunctionTaskRunnerPayload.RequestMethod}
*/
public enum RequestMethod
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
* GET = 1;
*/
GET(1),
/**
* POST = 2;
*/
POST(2),
/**
* HEAD = 3;
*/
HEAD(3),
/**
* PUT = 4;
*/
PUT(4),
/**
* DELETE = 5;
*/
DELETE(5),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* GET = 1;
*/
public static final int GET_VALUE = 1;
/**
* POST = 2;
*/
public static final int POST_VALUE = 2;
/**
* HEAD = 3;
*/
public static final int HEAD_VALUE = 3;
/**
* PUT = 4;
*/
public static final int PUT_VALUE = 4;
/**
* DELETE = 5;
*/
public static final int DELETE_VALUE = 5;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RequestMethod valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static RequestMethod forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return GET;
case 2: return POST;
case 3: return HEAD;
case 4: return PUT;
case 5: return DELETE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
RequestMethod> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RequestMethod findValueByNumber(int number) {
return RequestMethod.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.getDescriptor().getEnumTypes().get(0);
}
private static final RequestMethod[] VALUES = values();
public static RequestMethod valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private RequestMethod(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.FunctionTaskRunnerPayload.RequestMethod)
}
public interface HeaderOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.FunctionTaskRunnerPayload.Header)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes key = 4;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
* required bytes key = 4;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
* required bytes value = 5;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* required bytes value = 5;
* @return The value.
*/
com.google.protobuf.ByteString getValue();
}
/**
* Protobuf type {@code java.apphosting.FunctionTaskRunnerPayload.Header}
*/
public static final class Header extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.FunctionTaskRunnerPayload.Header)
HeaderOrBuilder {
private static final long serialVersionUID = 0L;
// Use Header.newBuilder() to construct.
private Header(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Header() {
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Header();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.class, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 4;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes key = 4;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int VALUE_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes value = 5;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required bytes value = 5;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(4, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(5, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header other = (com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.FunctionTaskRunnerPayload.Header}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.FunctionTaskRunnerPayload.Header)
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.class, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header build() {
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header buildPartial() {
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header result = new com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header) {
return mergeFrom((com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header other) {
if (other == com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 34: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 34
case 42: {
value_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 4;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes key = 4;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* required bytes key = 4;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required bytes key = 4;
* @return This builder for chaining.
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes value = 5;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required bytes value = 5;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* required bytes value = 5;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required bytes value = 5;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.FunctionTaskRunnerPayload.Header)
}
// @@protoc_insertion_point(class_scope:java.apphosting.FunctionTaskRunnerPayload.Header)
private static final com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header();
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Header parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int METHOD_FIELD_NUMBER = 1;
private int method_ = 2;
/**
* optional .java.apphosting.FunctionTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return Whether the method field is set.
*/
@java.lang.Override public boolean hasMethod() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.FunctionTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return The method.
*/
@java.lang.Override public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod getMethod() {
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod result = com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod.forNumber(method_);
return result == null ? com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod.POST : result;
}
public static final int URL_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString url_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes url = 2;
* @return Whether the url field is set.
*/
@java.lang.Override
public boolean hasUrl() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes url = 2;
* @return The url.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUrl() {
return url_;
}
public static final int HEADER_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List header_;
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public java.util.List getHeaderList() {
return header_;
}
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList() {
return header_;
}
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public int getHeaderCount() {
return header_.size();
}
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header getHeader(int index) {
return header_.get(index);
}
/**
* repeated group Header = 3 { ... }
*/
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index) {
return header_.get(index);
}
public static final int BODY_FIELD_NUMBER = 6;
private com.google.protobuf.ByteString body_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return Whether the body field is set.
*/
@java.lang.Override
public boolean hasBody() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return The body.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBody() {
return body_;
}
public static final int FUNCTION_NAME_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object functionName_ = "";
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return Whether the functionName field is set.
*/
@java.lang.Override
public boolean hasFunctionName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return The functionName.
*/
@java.lang.Override
public java.lang.String getFunctionName() {
java.lang.Object ref = functionName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
functionName_ = s;
}
return s;
}
}
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return The bytes for functionName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFunctionNameBytes() {
java.lang.Object ref = functionName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
functionName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getHeaderCount(); i++) {
if (!getHeader(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, method_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, url_);
}
for (int i = 0; i < header_.size(); i++) {
output.writeGroup(3, header_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(6, body_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, functionName_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, method_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, url_);
}
for (int i = 0; i < header_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(3, header_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, body_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, functionName_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.FunctionTaskRunnerPayload)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload other = (com.google.apphosting.executor.Task.FunctionTaskRunnerPayload) obj;
if (hasMethod() != other.hasMethod()) return false;
if (hasMethod()) {
if (method_ != other.method_) return false;
}
if (hasUrl() != other.hasUrl()) return false;
if (hasUrl()) {
if (!getUrl()
.equals(other.getUrl())) return false;
}
if (!getHeaderList()
.equals(other.getHeaderList())) return false;
if (hasBody() != other.hasBody()) return false;
if (hasBody()) {
if (!getBody()
.equals(other.getBody())) return false;
}
if (hasFunctionName() != other.hasFunctionName()) return false;
if (hasFunctionName()) {
if (!getFunctionName()
.equals(other.getFunctionName())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMethod()) {
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + method_;
}
if (hasUrl()) {
hash = (37 * hash) + URL_FIELD_NUMBER;
hash = (53 * hash) + getUrl().hashCode();
}
if (getHeaderCount() > 0) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeaderList().hashCode();
}
if (hasBody()) {
hash = (37 * hash) + BODY_FIELD_NUMBER;
hash = (53 * hash) + getBody().hashCode();
}
if (hasFunctionName()) {
hash = (37 * hash) + FUNCTION_NAME_FIELD_NUMBER;
hash = (53 * hash) + getFunctionName().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.FunctionTaskRunnerPayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.FunctionTaskRunnerPayload)
com.google.apphosting.executor.Task.FunctionTaskRunnerPayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.class, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
method_ = 2;
url_ = com.google.protobuf.ByteString.EMPTY;
if (headerBuilder_ == null) {
header_ = java.util.Collections.emptyList();
} else {
header_ = null;
headerBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
body_ = com.google.protobuf.ByteString.EMPTY;
functionName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_FunctionTaskRunnerPayload_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload build() {
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload buildPartial() {
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload result = new com.google.apphosting.executor.Task.FunctionTaskRunnerPayload(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload result) {
if (headerBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
header_ = java.util.Collections.unmodifiableList(header_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
}
private void buildPartial0(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.method_ = method_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.url_ = url_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.body_ = body_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.functionName_ = functionName_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.FunctionTaskRunnerPayload) {
return mergeFrom((com.google.apphosting.executor.Task.FunctionTaskRunnerPayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload other) {
if (other == com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.getDefaultInstance()) return this;
if (other.hasMethod()) {
setMethod(other.getMethod());
}
if (other.hasUrl()) {
setUrl(other.getUrl());
}
if (headerBuilder_ == null) {
if (!other.header_.isEmpty()) {
if (header_.isEmpty()) {
header_ = other.header_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureHeaderIsMutable();
header_.addAll(other.header_);
}
onChanged();
}
} else {
if (!other.header_.isEmpty()) {
if (headerBuilder_.isEmpty()) {
headerBuilder_.dispose();
headerBuilder_ = null;
header_ = other.header_;
bitField0_ = (bitField0_ & ~0x00000004);
headerBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getHeaderFieldBuilder() : null;
} else {
headerBuilder_.addAllMessages(other.header_);
}
}
}
if (other.hasBody()) {
setBody(other.getBody());
}
if (other.hasFunctionName()) {
functionName_ = other.functionName_;
bitField0_ |= 0x00000010;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getHeaderCount(); i++) {
if (!getHeader(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod tmpValue =
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
method_ = tmpRaw;
bitField0_ |= 0x00000001;
}
break;
} // case 8
case 18: {
url_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 27: {
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header m =
input.readGroup(3,
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.PARSER,
extensionRegistry);
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(m);
} else {
headerBuilder_.addMessage(m);
}
break;
} // case 27
case 50: {
body_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 50
case 58: {
functionName_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int method_ = 2;
/**
* optional .java.apphosting.FunctionTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return Whether the method field is set.
*/
@java.lang.Override public boolean hasMethod() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.FunctionTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return The method.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod getMethod() {
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod result = com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod.forNumber(method_);
return result == null ? com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod.POST : result;
}
/**
* optional .java.apphosting.FunctionTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @param value The method to set.
* @return This builder for chaining.
*/
public Builder setMethod(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.RequestMethod value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
method_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.FunctionTaskRunnerPayload.RequestMethod method = 1 [default = POST];
* @return This builder for chaining.
*/
public Builder clearMethod() {
bitField0_ = (bitField0_ & ~0x00000001);
method_ = 2;
onChanged();
return this;
}
private com.google.protobuf.ByteString url_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes url = 2;
* @return Whether the url field is set.
*/
@java.lang.Override
public boolean hasUrl() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes url = 2;
* @return The url.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUrl() {
return url_;
}
/**
* optional bytes url = 2;
* @param value The url to set.
* @return This builder for chaining.
*/
public Builder setUrl(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
url_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional bytes url = 2;
* @return This builder for chaining.
*/
public Builder clearUrl() {
bitField0_ = (bitField0_ & ~0x00000002);
url_ = getDefaultInstance().getUrl();
onChanged();
return this;
}
private java.util.List header_ =
java.util.Collections.emptyList();
private void ensureHeaderIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
header_ = new java.util.ArrayList(header_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder> headerBuilder_;
/**
* repeated group Header = 3 { ... }
*/
public java.util.List getHeaderList() {
if (headerBuilder_ == null) {
return java.util.Collections.unmodifiableList(header_);
} else {
return headerBuilder_.getMessageList();
}
}
/**
* repeated group Header = 3 { ... }
*/
public int getHeaderCount() {
if (headerBuilder_ == null) {
return header_.size();
} else {
return headerBuilder_.getCount();
}
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header getHeader(int index) {
if (headerBuilder_ == null) {
return header_.get(index);
} else {
return headerBuilder_.getMessage(index);
}
}
/**
* repeated group Header = 3 { ... }
*/
public Builder setHeader(
int index, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.set(index, value);
onChanged();
} else {
headerBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder setHeader(
int index, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.set(index, builderForValue.build());
onChanged();
} else {
headerBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addHeader(com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.add(value);
onChanged();
} else {
headerBuilder_.addMessage(value);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addHeader(
int index, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.add(index, value);
onChanged();
} else {
headerBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addHeader(
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(builderForValue.build());
onChanged();
} else {
headerBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addHeader(
int index, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(index, builderForValue.build());
onChanged();
} else {
headerBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder addAllHeader(
java.lang.Iterable extends com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header> values) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, header_);
onChanged();
} else {
headerBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
headerBuilder_.clear();
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public Builder removeHeader(int index) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.remove(index);
onChanged();
} else {
headerBuilder_.remove(index);
}
return this;
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder getHeaderBuilder(
int index) {
return getHeaderFieldBuilder().getBuilder(index);
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index) {
if (headerBuilder_ == null) {
return header_.get(index); } else {
return headerBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated group Header = 3 { ... }
*/
public java.util.List extends com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(header_);
}
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder addHeaderBuilder() {
return getHeaderFieldBuilder().addBuilder(
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.getDefaultInstance());
}
/**
* repeated group Header = 3 { ... }
*/
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder addHeaderBuilder(
int index) {
return getHeaderFieldBuilder().addBuilder(
index, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.getDefaultInstance());
}
/**
* repeated group Header = 3 { ... }
*/
public java.util.List
getHeaderBuilderList() {
return getHeaderFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.FunctionTaskRunnerPayload.HeaderOrBuilder>(
header_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private com.google.protobuf.ByteString body_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return Whether the body field is set.
*/
@java.lang.Override
public boolean hasBody() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return The body.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBody() {
return body_;
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @param value The body to set.
* @return This builder for chaining.
*/
public Builder setBody(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
body_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 6 [ctype = CORD];
* @return This builder for chaining.
*/
public Builder clearBody() {
bitField0_ = (bitField0_ & ~0x00000008);
body_ = getDefaultInstance().getBody();
onChanged();
return this;
}
private java.lang.Object functionName_ = "";
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return Whether the functionName field is set.
*/
public boolean hasFunctionName() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return The functionName.
*/
public java.lang.String getFunctionName() {
java.lang.Object ref = functionName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
functionName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return The bytes for functionName.
*/
public com.google.protobuf.ByteString
getFunctionNameBytes() {
java.lang.Object ref = functionName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
functionName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @param value The functionName to set.
* @return This builder for chaining.
*/
public Builder setFunctionName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
functionName_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @return This builder for chaining.
*/
public Builder clearFunctionName() {
functionName_ = getDefaultInstance().getFunctionName();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* Name of the Cloud Function to which this payload will be sent to.
*
*
* optional string function_name = 7;
* @param value The bytes for functionName to set.
* @return This builder for chaining.
*/
public Builder setFunctionNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
functionName_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.FunctionTaskRunnerPayload)
}
// @@protoc_insertion_point(class_scope:java.apphosting.FunctionTaskRunnerPayload)
private static final com.google.apphosting.executor.Task.FunctionTaskRunnerPayload DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.FunctionTaskRunnerPayload();
}
public static com.google.apphosting.executor.Task.FunctionTaskRunnerPayload getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FunctionTaskRunnerPayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.FunctionTaskRunnerPayload getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ExternalHttpTaskRunnerPayloadOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.ExternalHttpTaskRunnerPayload)
com.google.protobuf.MessageOrBuilder {
/**
* optional .java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod method = 1 [default = REQUEST_METHOD_UNSPECIFIED];
* @return Whether the method field is set.
*/
boolean hasMethod();
/**
* optional .java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod method = 1 [default = REQUEST_METHOD_UNSPECIFIED];
* @return The method.
*/
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod getMethod();
/**
*
* Required.
*
*
* optional bytes url = 2;
* @return Whether the url field is set.
*/
boolean hasUrl();
/**
*
* Required.
*
*
* optional bytes url = 2;
* @return The url.
*/
com.google.protobuf.ByteString getUrl();
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
java.util.List
getHeaderList();
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header getHeader(int index);
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
int getHeaderCount();
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
java.util.List extends com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList();
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index);
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 4 [ctype = CORD];
* @return Whether the body field is set.
*/
boolean hasBody();
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 4 [ctype = CORD];
* @return The body.
*/
com.google.protobuf.ByteString getBody();
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return Whether the requestorId field is set.
*/
boolean hasRequestorId();
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return The requestorId.
*/
java.lang.String getRequestorId();
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return The bytes for requestorId.
*/
com.google.protobuf.ByteString
getRequestorIdBytes();
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 request_deadline_ms = 6;
* @return Whether the requestDeadlineMs field is set.
*/
boolean hasRequestDeadlineMs();
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 request_deadline_ms = 6;
* @return The requestDeadlineMs.
*/
int getRequestDeadlineMs();
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 fetch_timeout_ms = 7;
* @return Whether the fetchTimeoutMs field is set.
*/
boolean hasFetchTimeoutMs();
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 fetch_timeout_ms = 7;
* @return The fetchTimeoutMs.
*/
int getFetchTimeoutMs();
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return Whether the userAgentToSend field is set.
*/
boolean hasUserAgentToSend();
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return The userAgentToSend.
*/
java.lang.String getUserAgentToSend();
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return The bytes for userAgentToSend.
*/
com.google.protobuf.ByteString
getUserAgentToSendBytes();
/**
*
* Optional. If set to true, the request fails if the server presents an
* invalid SSL certificate.
*
*
* optional bool fail_on_ssl_certificate_error = 9;
* @return Whether the failOnSslCertificateError field is set.
*/
boolean hasFailOnSslCertificateError();
/**
*
* Optional. If set to true, the request fails if the server presents an
* invalid SSL certificate.
*
*
* optional bool fail_on_ssl_certificate_error = 9;
* @return The failOnSslCertificateError.
*/
boolean getFailOnSslCertificateError();
}
/**
*
* Payload type for ExternalHttpTaskRunner.
*
*
* Protobuf type {@code java.apphosting.ExternalHttpTaskRunnerPayload}
*/
public static final class ExternalHttpTaskRunnerPayload extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.ExternalHttpTaskRunnerPayload)
ExternalHttpTaskRunnerPayloadOrBuilder {
private static final long serialVersionUID = 0L;
// Use ExternalHttpTaskRunnerPayload.newBuilder() to construct.
private ExternalHttpTaskRunnerPayload(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ExternalHttpTaskRunnerPayload() {
method_ = 0;
url_ = com.google.protobuf.ByteString.EMPTY;
header_ = java.util.Collections.emptyList();
body_ = com.google.protobuf.ByteString.EMPTY;
requestorId_ = "";
userAgentToSend_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ExternalHttpTaskRunnerPayload();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.class, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod}
*/
public enum RequestMethod
implements com.google.protobuf.ProtocolMessageEnum {
/**
* REQUEST_METHOD_UNSPECIFIED = 0;
*/
REQUEST_METHOD_UNSPECIFIED(0),
/**
* GET = 1;
*/
GET(1),
/**
* POST = 2;
*/
POST(2),
/**
* HEAD = 3;
*/
HEAD(3),
/**
* PUT = 4;
*/
PUT(4),
/**
* DELETE = 5;
*/
DELETE(5),
/**
* PATCH = 6;
*/
PATCH(6),
/**
* OPTIONS = 7;
*/
OPTIONS(7),
;
/**
* REQUEST_METHOD_UNSPECIFIED = 0;
*/
public static final int REQUEST_METHOD_UNSPECIFIED_VALUE = 0;
/**
* GET = 1;
*/
public static final int GET_VALUE = 1;
/**
* POST = 2;
*/
public static final int POST_VALUE = 2;
/**
* HEAD = 3;
*/
public static final int HEAD_VALUE = 3;
/**
* PUT = 4;
*/
public static final int PUT_VALUE = 4;
/**
* DELETE = 5;
*/
public static final int DELETE_VALUE = 5;
/**
* PATCH = 6;
*/
public static final int PATCH_VALUE = 6;
/**
* OPTIONS = 7;
*/
public static final int OPTIONS_VALUE = 7;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RequestMethod valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static RequestMethod forNumber(int value) {
switch (value) {
case 0: return REQUEST_METHOD_UNSPECIFIED;
case 1: return GET;
case 2: return POST;
case 3: return HEAD;
case 4: return PUT;
case 5: return DELETE;
case 6: return PATCH;
case 7: return OPTIONS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
RequestMethod> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public RequestMethod findValueByNumber(int number) {
return RequestMethod.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.getDescriptor().getEnumTypes().get(0);
}
private static final RequestMethod[] VALUES = values();
public static RequestMethod valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private RequestMethod(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod)
}
public interface HeaderOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.ExternalHttpTaskRunnerPayload.Header)
com.google.protobuf.MessageOrBuilder {
/**
*
* Required.
*
*
* optional bytes key = 1;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
*
* Required.
*
*
* optional bytes key = 1;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
* optional bytes value = 2;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* optional bytes value = 2;
* @return The value.
*/
com.google.protobuf.ByteString getValue();
}
/**
* Protobuf type {@code java.apphosting.ExternalHttpTaskRunnerPayload.Header}
*/
public static final class Header extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.ExternalHttpTaskRunnerPayload.Header)
HeaderOrBuilder {
private static final long serialVersionUID = 0L;
// Use Header.newBuilder() to construct.
private Header(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Header() {
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Header();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.class, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Required.
*
*
* optional bytes key = 1;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required.
*
*
* optional bytes key = 1;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int VALUE_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes value = 2;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header other = (com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.ExternalHttpTaskRunnerPayload.Header}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.ExternalHttpTaskRunnerPayload.Header)
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.class, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header build() {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header buildPartial() {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header result = new com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header) {
return mergeFrom((com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header other) {
if (other == com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
value_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Required.
*
*
* optional bytes key = 1;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required.
*
*
* optional bytes key = 1;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
*
* Required.
*
*
* optional bytes key = 1;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Required.
*
*
* optional bytes key = 1;
* @return This builder for chaining.
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional bytes value = 2;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* optional bytes value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional bytes value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.ExternalHttpTaskRunnerPayload.Header)
}
// @@protoc_insertion_point(class_scope:java.apphosting.ExternalHttpTaskRunnerPayload.Header)
private static final com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header();
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Header parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int METHOD_FIELD_NUMBER = 1;
private int method_ = 0;
/**
* optional .java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod method = 1 [default = REQUEST_METHOD_UNSPECIFIED];
* @return Whether the method field is set.
*/
@java.lang.Override public boolean hasMethod() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod method = 1 [default = REQUEST_METHOD_UNSPECIFIED];
* @return The method.
*/
@java.lang.Override public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod getMethod() {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod result = com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod.forNumber(method_);
return result == null ? com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod.REQUEST_METHOD_UNSPECIFIED : result;
}
public static final int URL_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString url_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Required.
*
*
* optional bytes url = 2;
* @return Whether the url field is set.
*/
@java.lang.Override
public boolean hasUrl() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Required.
*
*
* optional bytes url = 2;
* @return The url.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUrl() {
return url_;
}
public static final int HEADER_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List header_;
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
@java.lang.Override
public java.util.List getHeaderList() {
return header_;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList() {
return header_;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
@java.lang.Override
public int getHeaderCount() {
return header_.size();
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header getHeader(int index) {
return header_.get(index);
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index) {
return header_.get(index);
}
public static final int BODY_FIELD_NUMBER = 4;
private com.google.protobuf.ByteString body_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 4 [ctype = CORD];
* @return Whether the body field is set.
*/
@java.lang.Override
public boolean hasBody() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 4 [ctype = CORD];
* @return The body.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBody() {
return body_;
}
public static final int REQUESTOR_ID_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object requestorId_ = "";
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return Whether the requestorId field is set.
*/
@java.lang.Override
public boolean hasRequestorId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return The requestorId.
*/
@java.lang.Override
public java.lang.String getRequestorId() {
java.lang.Object ref = requestorId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
requestorId_ = s;
}
return s;
}
}
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return The bytes for requestorId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRequestorIdBytes() {
java.lang.Object ref = requestorId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestorId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_DEADLINE_MS_FIELD_NUMBER = 6;
private int requestDeadlineMs_ = 0;
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 request_deadline_ms = 6;
* @return Whether the requestDeadlineMs field is set.
*/
@java.lang.Override
public boolean hasRequestDeadlineMs() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 request_deadline_ms = 6;
* @return The requestDeadlineMs.
*/
@java.lang.Override
public int getRequestDeadlineMs() {
return requestDeadlineMs_;
}
public static final int FETCH_TIMEOUT_MS_FIELD_NUMBER = 7;
private int fetchTimeoutMs_ = 0;
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 fetch_timeout_ms = 7;
* @return Whether the fetchTimeoutMs field is set.
*/
@java.lang.Override
public boolean hasFetchTimeoutMs() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 fetch_timeout_ms = 7;
* @return The fetchTimeoutMs.
*/
@java.lang.Override
public int getFetchTimeoutMs() {
return fetchTimeoutMs_;
}
public static final int USER_AGENT_TO_SEND_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object userAgentToSend_ = "";
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return Whether the userAgentToSend field is set.
*/
@java.lang.Override
public boolean hasUserAgentToSend() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return The userAgentToSend.
*/
@java.lang.Override
public java.lang.String getUserAgentToSend() {
java.lang.Object ref = userAgentToSend_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userAgentToSend_ = s;
}
return s;
}
}
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return The bytes for userAgentToSend.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserAgentToSendBytes() {
java.lang.Object ref = userAgentToSend_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgentToSend_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FAIL_ON_SSL_CERTIFICATE_ERROR_FIELD_NUMBER = 9;
private boolean failOnSslCertificateError_ = false;
/**
*
* Optional. If set to true, the request fails if the server presents an
* invalid SSL certificate.
*
*
* optional bool fail_on_ssl_certificate_error = 9;
* @return Whether the failOnSslCertificateError field is set.
*/
@java.lang.Override
public boolean hasFailOnSslCertificateError() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Optional. If set to true, the request fails if the server presents an
* invalid SSL certificate.
*
*
* optional bool fail_on_ssl_certificate_error = 9;
* @return The failOnSslCertificateError.
*/
@java.lang.Override
public boolean getFailOnSslCertificateError() {
return failOnSslCertificateError_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, method_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, url_);
}
for (int i = 0; i < header_.size(); i++) {
output.writeMessage(3, header_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeBytes(4, body_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, requestorId_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(6, requestDeadlineMs_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt32(7, fetchTimeoutMs_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, userAgentToSend_);
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeBool(9, failOnSslCertificateError_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, method_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, url_);
}
for (int i = 0; i < header_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, header_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, body_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, requestorId_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, requestDeadlineMs_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(7, fetchTimeoutMs_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, userAgentToSend_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, failOnSslCertificateError_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload other = (com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload) obj;
if (hasMethod() != other.hasMethod()) return false;
if (hasMethod()) {
if (method_ != other.method_) return false;
}
if (hasUrl() != other.hasUrl()) return false;
if (hasUrl()) {
if (!getUrl()
.equals(other.getUrl())) return false;
}
if (!getHeaderList()
.equals(other.getHeaderList())) return false;
if (hasBody() != other.hasBody()) return false;
if (hasBody()) {
if (!getBody()
.equals(other.getBody())) return false;
}
if (hasRequestorId() != other.hasRequestorId()) return false;
if (hasRequestorId()) {
if (!getRequestorId()
.equals(other.getRequestorId())) return false;
}
if (hasRequestDeadlineMs() != other.hasRequestDeadlineMs()) return false;
if (hasRequestDeadlineMs()) {
if (getRequestDeadlineMs()
!= other.getRequestDeadlineMs()) return false;
}
if (hasFetchTimeoutMs() != other.hasFetchTimeoutMs()) return false;
if (hasFetchTimeoutMs()) {
if (getFetchTimeoutMs()
!= other.getFetchTimeoutMs()) return false;
}
if (hasUserAgentToSend() != other.hasUserAgentToSend()) return false;
if (hasUserAgentToSend()) {
if (!getUserAgentToSend()
.equals(other.getUserAgentToSend())) return false;
}
if (hasFailOnSslCertificateError() != other.hasFailOnSslCertificateError()) return false;
if (hasFailOnSslCertificateError()) {
if (getFailOnSslCertificateError()
!= other.getFailOnSslCertificateError()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMethod()) {
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + method_;
}
if (hasUrl()) {
hash = (37 * hash) + URL_FIELD_NUMBER;
hash = (53 * hash) + getUrl().hashCode();
}
if (getHeaderCount() > 0) {
hash = (37 * hash) + HEADER_FIELD_NUMBER;
hash = (53 * hash) + getHeaderList().hashCode();
}
if (hasBody()) {
hash = (37 * hash) + BODY_FIELD_NUMBER;
hash = (53 * hash) + getBody().hashCode();
}
if (hasRequestorId()) {
hash = (37 * hash) + REQUESTOR_ID_FIELD_NUMBER;
hash = (53 * hash) + getRequestorId().hashCode();
}
if (hasRequestDeadlineMs()) {
hash = (37 * hash) + REQUEST_DEADLINE_MS_FIELD_NUMBER;
hash = (53 * hash) + getRequestDeadlineMs();
}
if (hasFetchTimeoutMs()) {
hash = (37 * hash) + FETCH_TIMEOUT_MS_FIELD_NUMBER;
hash = (53 * hash) + getFetchTimeoutMs();
}
if (hasUserAgentToSend()) {
hash = (37 * hash) + USER_AGENT_TO_SEND_FIELD_NUMBER;
hash = (53 * hash) + getUserAgentToSend().hashCode();
}
if (hasFailOnSslCertificateError()) {
hash = (37 * hash) + FAIL_ON_SSL_CERTIFICATE_ERROR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getFailOnSslCertificateError());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Payload type for ExternalHttpTaskRunner.
*
*
* Protobuf type {@code java.apphosting.ExternalHttpTaskRunnerPayload}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.ExternalHttpTaskRunnerPayload)
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayloadOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.class, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
method_ = 0;
url_ = com.google.protobuf.ByteString.EMPTY;
if (headerBuilder_ == null) {
header_ = java.util.Collections.emptyList();
} else {
header_ = null;
headerBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
body_ = com.google.protobuf.ByteString.EMPTY;
requestorId_ = "";
requestDeadlineMs_ = 0;
fetchTimeoutMs_ = 0;
userAgentToSend_ = "";
failOnSslCertificateError_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload build() {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload buildPartial() {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload result = new com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload result) {
if (headerBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
header_ = java.util.Collections.unmodifiableList(header_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.header_ = header_;
} else {
result.header_ = headerBuilder_.build();
}
}
private void buildPartial0(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.method_ = method_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.url_ = url_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.body_ = body_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.requestorId_ = requestorId_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.requestDeadlineMs_ = requestDeadlineMs_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.fetchTimeoutMs_ = fetchTimeoutMs_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.userAgentToSend_ = userAgentToSend_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.failOnSslCertificateError_ = failOnSslCertificateError_;
to_bitField0_ |= 0x00000080;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload) {
return mergeFrom((com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload other) {
if (other == com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.getDefaultInstance()) return this;
if (other.hasMethod()) {
setMethod(other.getMethod());
}
if (other.hasUrl()) {
setUrl(other.getUrl());
}
if (headerBuilder_ == null) {
if (!other.header_.isEmpty()) {
if (header_.isEmpty()) {
header_ = other.header_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureHeaderIsMutable();
header_.addAll(other.header_);
}
onChanged();
}
} else {
if (!other.header_.isEmpty()) {
if (headerBuilder_.isEmpty()) {
headerBuilder_.dispose();
headerBuilder_ = null;
header_ = other.header_;
bitField0_ = (bitField0_ & ~0x00000004);
headerBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getHeaderFieldBuilder() : null;
} else {
headerBuilder_.addAllMessages(other.header_);
}
}
}
if (other.hasBody()) {
setBody(other.getBody());
}
if (other.hasRequestorId()) {
requestorId_ = other.requestorId_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasRequestDeadlineMs()) {
setRequestDeadlineMs(other.getRequestDeadlineMs());
}
if (other.hasFetchTimeoutMs()) {
setFetchTimeoutMs(other.getFetchTimeoutMs());
}
if (other.hasUserAgentToSend()) {
userAgentToSend_ = other.userAgentToSend_;
bitField0_ |= 0x00000080;
onChanged();
}
if (other.hasFailOnSslCertificateError()) {
setFailOnSslCertificateError(other.getFailOnSslCertificateError());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod tmpValue =
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
method_ = tmpRaw;
bitField0_ |= 0x00000001;
}
break;
} // case 8
case 18: {
url_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header m =
input.readMessage(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.PARSER,
extensionRegistry);
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(m);
} else {
headerBuilder_.addMessage(m);
}
break;
} // case 26
case 34: {
body_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
requestorId_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 42
case 48: {
requestDeadlineMs_ = input.readInt32();
bitField0_ |= 0x00000020;
break;
} // case 48
case 56: {
fetchTimeoutMs_ = input.readInt32();
bitField0_ |= 0x00000040;
break;
} // case 56
case 66: {
userAgentToSend_ = input.readBytes();
bitField0_ |= 0x00000080;
break;
} // case 66
case 72: {
failOnSslCertificateError_ = input.readBool();
bitField0_ |= 0x00000100;
break;
} // case 72
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int method_ = 0;
/**
* optional .java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod method = 1 [default = REQUEST_METHOD_UNSPECIFIED];
* @return Whether the method field is set.
*/
@java.lang.Override public boolean hasMethod() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod method = 1 [default = REQUEST_METHOD_UNSPECIFIED];
* @return The method.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod getMethod() {
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod result = com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod.forNumber(method_);
return result == null ? com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod.REQUEST_METHOD_UNSPECIFIED : result;
}
/**
* optional .java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod method = 1 [default = REQUEST_METHOD_UNSPECIFIED];
* @param value The method to set.
* @return This builder for chaining.
*/
public Builder setMethod(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.RequestMethod value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
method_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.ExternalHttpTaskRunnerPayload.RequestMethod method = 1 [default = REQUEST_METHOD_UNSPECIFIED];
* @return This builder for chaining.
*/
public Builder clearMethod() {
bitField0_ = (bitField0_ & ~0x00000001);
method_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString url_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Required.
*
*
* optional bytes url = 2;
* @return Whether the url field is set.
*/
@java.lang.Override
public boolean hasUrl() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Required.
*
*
* optional bytes url = 2;
* @return The url.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUrl() {
return url_;
}
/**
*
* Required.
*
*
* optional bytes url = 2;
* @param value The url to set.
* @return This builder for chaining.
*/
public Builder setUrl(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
url_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Required.
*
*
* optional bytes url = 2;
* @return This builder for chaining.
*/
public Builder clearUrl() {
bitField0_ = (bitField0_ & ~0x00000002);
url_ = getDefaultInstance().getUrl();
onChanged();
return this;
}
private java.util.List header_ =
java.util.Collections.emptyList();
private void ensureHeaderIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
header_ = new java.util.ArrayList(header_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder> headerBuilder_;
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public java.util.List getHeaderList() {
if (headerBuilder_ == null) {
return java.util.Collections.unmodifiableList(header_);
} else {
return headerBuilder_.getMessageList();
}
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public int getHeaderCount() {
if (headerBuilder_ == null) {
return header_.size();
} else {
return headerBuilder_.getCount();
}
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header getHeader(int index) {
if (headerBuilder_ == null) {
return header_.get(index);
} else {
return headerBuilder_.getMessage(index);
}
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder setHeader(
int index, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.set(index, value);
onChanged();
} else {
headerBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder setHeader(
int index, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.set(index, builderForValue.build());
onChanged();
} else {
headerBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder addHeader(com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.add(value);
onChanged();
} else {
headerBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder addHeader(
int index, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header value) {
if (headerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeaderIsMutable();
header_.add(index, value);
onChanged();
} else {
headerBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder addHeader(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(builderForValue.build());
onChanged();
} else {
headerBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder addHeader(
int index, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder builderForValue) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.add(index, builderForValue.build());
onChanged();
} else {
headerBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder addAllHeader(
java.lang.Iterable extends com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header> values) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, header_);
onChanged();
} else {
headerBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder clearHeader() {
if (headerBuilder_ == null) {
header_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
headerBuilder_.clear();
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public Builder removeHeader(int index) {
if (headerBuilder_ == null) {
ensureHeaderIsMutable();
header_.remove(index);
onChanged();
} else {
headerBuilder_.remove(index);
}
return this;
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder getHeaderBuilder(
int index) {
return getHeaderFieldBuilder().getBuilder(index);
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder getHeaderOrBuilder(
int index) {
if (headerBuilder_ == null) {
return header_.get(index); } else {
return headerBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public java.util.List extends com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder>
getHeaderOrBuilderList() {
if (headerBuilder_ != null) {
return headerBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(header_);
}
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder addHeaderBuilder() {
return getHeaderFieldBuilder().addBuilder(
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.getDefaultInstance());
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder addHeaderBuilder(
int index) {
return getHeaderFieldBuilder().addBuilder(
index, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.getDefaultInstance());
}
/**
* repeated .java.apphosting.ExternalHttpTaskRunnerPayload.Header header = 3;
*/
public java.util.List
getHeaderBuilderList() {
return getHeaderFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder>
getHeaderFieldBuilder() {
if (headerBuilder_ == null) {
headerBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.Header.Builder, com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload.HeaderOrBuilder>(
header_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
header_ = null;
}
return headerBuilder_;
}
private com.google.protobuf.ByteString body_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 4 [ctype = CORD];
* @return Whether the body field is set.
*/
@java.lang.Override
public boolean hasBody() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 4 [ctype = CORD];
* @return The body.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBody() {
return body_;
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 4 [ctype = CORD];
* @param value The body to set.
* @return This builder for chaining.
*/
public Builder setBody(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
body_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Body of the HTTP request (may contain binary data). Ignored unless method
* is POST or PUT.
*
*
* optional bytes body = 4 [ctype = CORD];
* @return This builder for chaining.
*/
public Builder clearBody() {
bitField0_ = (bitField0_ & ~0x00000008);
body_ = getDefaultInstance().getBody();
onChanged();
return this;
}
private java.lang.Object requestorId_ = "";
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return Whether the requestorId field is set.
*/
public boolean hasRequestorId() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return The requestorId.
*/
public java.lang.String getRequestorId() {
java.lang.Object ref = requestorId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
requestorId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return The bytes for requestorId.
*/
public com.google.protobuf.ByteString
getRequestorIdBytes() {
java.lang.Object ref = requestorId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestorId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @param value The requestorId to set.
* @return This builder for chaining.
*/
public Builder setRequestorId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
requestorId_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @return This builder for chaining.
*/
public Builder clearRequestorId() {
requestorId_ = getDefaultInstance().getRequestorId();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* Required.
*
*
* optional string requestor_id = 5;
* @param value The bytes for requestorId to set.
* @return This builder for chaining.
*/
public Builder setRequestorIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
requestorId_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private int requestDeadlineMs_ ;
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 request_deadline_ms = 6;
* @return Whether the requestDeadlineMs field is set.
*/
@java.lang.Override
public boolean hasRequestDeadlineMs() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 request_deadline_ms = 6;
* @return The requestDeadlineMs.
*/
@java.lang.Override
public int getRequestDeadlineMs() {
return requestDeadlineMs_;
}
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 request_deadline_ms = 6;
* @param value The requestDeadlineMs to set.
* @return This builder for chaining.
*/
public Builder setRequestDeadlineMs(int value) {
requestDeadlineMs_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 request_deadline_ms = 6;
* @return This builder for chaining.
*/
public Builder clearRequestDeadlineMs() {
bitField0_ = (bitField0_ & ~0x00000020);
requestDeadlineMs_ = 0;
onChanged();
return this;
}
private int fetchTimeoutMs_ ;
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 fetch_timeout_ms = 7;
* @return Whether the fetchTimeoutMs field is set.
*/
@java.lang.Override
public boolean hasFetchTimeoutMs() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 fetch_timeout_ms = 7;
* @return The fetchTimeoutMs.
*/
@java.lang.Override
public int getFetchTimeoutMs() {
return fetchTimeoutMs_;
}
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 fetch_timeout_ms = 7;
* @param value The fetchTimeoutMs to set.
* @return This builder for chaining.
*/
public Builder setFetchTimeoutMs(int value) {
fetchTimeoutMs_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Trawler timeout parameter. Explained here:
* http://g3doc/crawler/trawler/fetchclient/README.md#timeouts-in-trawler
*
*
* optional int32 fetch_timeout_ms = 7;
* @return This builder for chaining.
*/
public Builder clearFetchTimeoutMs() {
bitField0_ = (bitField0_ & ~0x00000040);
fetchTimeoutMs_ = 0;
onChanged();
return this;
}
private java.lang.Object userAgentToSend_ = "";
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return Whether the userAgentToSend field is set.
*/
public boolean hasUserAgentToSend() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return The userAgentToSend.
*/
public java.lang.String getUserAgentToSend() {
java.lang.Object ref = userAgentToSend_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userAgentToSend_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return The bytes for userAgentToSend.
*/
public com.google.protobuf.ByteString
getUserAgentToSendBytes() {
java.lang.Object ref = userAgentToSend_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userAgentToSend_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @param value The userAgentToSend to set.
* @return This builder for chaining.
*/
public Builder setUserAgentToSend(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
userAgentToSend_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @return This builder for chaining.
*/
public Builder clearUserAgentToSend() {
userAgentToSend_ = getDefaultInstance().getUserAgentToSend();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
* Required. User Agent to be specified in Harpoon request.
*
*
* optional string user_agent_to_send = 8;
* @param value The bytes for userAgentToSend to set.
* @return This builder for chaining.
*/
public Builder setUserAgentToSendBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
userAgentToSend_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private boolean failOnSslCertificateError_ ;
/**
*
* Optional. If set to true, the request fails if the server presents an
* invalid SSL certificate.
*
*
* optional bool fail_on_ssl_certificate_error = 9;
* @return Whether the failOnSslCertificateError field is set.
*/
@java.lang.Override
public boolean hasFailOnSslCertificateError() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Optional. If set to true, the request fails if the server presents an
* invalid SSL certificate.
*
*
* optional bool fail_on_ssl_certificate_error = 9;
* @return The failOnSslCertificateError.
*/
@java.lang.Override
public boolean getFailOnSslCertificateError() {
return failOnSslCertificateError_;
}
/**
*
* Optional. If set to true, the request fails if the server presents an
* invalid SSL certificate.
*
*
* optional bool fail_on_ssl_certificate_error = 9;
* @param value The failOnSslCertificateError to set.
* @return This builder for chaining.
*/
public Builder setFailOnSslCertificateError(boolean value) {
failOnSslCertificateError_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Optional. If set to true, the request fails if the server presents an
* invalid SSL certificate.
*
*
* optional bool fail_on_ssl_certificate_error = 9;
* @return This builder for chaining.
*/
public Builder clearFailOnSslCertificateError() {
bitField0_ = (bitField0_ & ~0x00000100);
failOnSslCertificateError_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.ExternalHttpTaskRunnerPayload)
}
// @@protoc_insertion_point(class_scope:java.apphosting.ExternalHttpTaskRunnerPayload)
private static final com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload();
}
public static com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ExternalHttpTaskRunnerPayload parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ExternalHttpTaskRunnerPayload getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ApiSourceOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.ApiSource)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code java.apphosting.ApiSource}
*/
public static final class ApiSource extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.ApiSource)
ApiSourceOrBuilder {
private static final long serialVersionUID = 0L;
// Use ApiSource.newBuilder() to construct.
private ApiSource(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ApiSource() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ApiSource();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ApiSource_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ApiSource_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.ApiSource.class, com.google.apphosting.executor.Task.ApiSource.Builder.class);
}
/**
*
* LINT.IfChange
*
*
* Protobuf enum {@code java.apphosting.ApiSource.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
* APP_ENGINE_RUNTIME = 1;
*/
APP_ENGINE_RUNTIME(1),
/**
* TASK_QUEUE_BRIDGE = 2;
*/
TASK_QUEUE_BRIDGE(2),
/**
* CLOUD_TASKS_FRONTEND = 3;
*/
CLOUD_TASKS_FRONTEND(3),
/**
* CLOUD_SCHEDULER_FRONTEND = 4;
*/
CLOUD_SCHEDULER_FRONTEND(4),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* APP_ENGINE_RUNTIME = 1;
*/
public static final int APP_ENGINE_RUNTIME_VALUE = 1;
/**
* TASK_QUEUE_BRIDGE = 2;
*/
public static final int TASK_QUEUE_BRIDGE_VALUE = 2;
/**
* CLOUD_TASKS_FRONTEND = 3;
*/
public static final int CLOUD_TASKS_FRONTEND_VALUE = 3;
/**
* CLOUD_SCHEDULER_FRONTEND = 4;
*/
public static final int CLOUD_SCHEDULER_FRONTEND_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Type forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return APP_ENGINE_RUNTIME;
case 2: return TASK_QUEUE_BRIDGE;
case 3: return CLOUD_TASKS_FRONTEND;
case 4: return CLOUD_SCHEDULER_FRONTEND;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.executor.Task.ApiSource.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.ApiSource.Type)
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.ApiSource)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.ApiSource other = (com.google.apphosting.executor.Task.ApiSource) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ApiSource parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ApiSource parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.ApiSource parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.ApiSource prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.ApiSource}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.ApiSource)
com.google.apphosting.executor.Task.ApiSourceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ApiSource_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ApiSource_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.ApiSource.class, com.google.apphosting.executor.Task.ApiSource.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.ApiSource.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_ApiSource_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ApiSource getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.ApiSource.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.ApiSource build() {
com.google.apphosting.executor.Task.ApiSource result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ApiSource buildPartial() {
com.google.apphosting.executor.Task.ApiSource result = new com.google.apphosting.executor.Task.ApiSource(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.ApiSource) {
return mergeFrom((com.google.apphosting.executor.Task.ApiSource)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.ApiSource other) {
if (other == com.google.apphosting.executor.Task.ApiSource.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.ApiSource)
}
// @@protoc_insertion_point(class_scope:java.apphosting.ApiSource)
private static final com.google.apphosting.executor.Task.ApiSource DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.ApiSource();
}
public static com.google.apphosting.executor.Task.ApiSource getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ApiSource parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.ApiSource getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskDefinitionOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskDefinition)
com.google.protobuf.MessageOrBuilder {
/**
* required .java.apphosting.TaskRef task_ref = 1;
* @return Whether the taskRef field is set.
*/
boolean hasTaskRef();
/**
* required .java.apphosting.TaskRef task_ref = 1;
* @return The taskRef.
*/
com.google.apphosting.executor.Task.TaskRef getTaskRef();
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
com.google.apphosting.executor.Task.TaskRefOrBuilder getTaskRefOrBuilder();
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 2;
* @return Whether the etaUsec field is set.
*/
boolean hasEtaUsec();
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 2;
* @return The etaUsec.
*/
long getEtaUsec();
/**
*
* The previous value of eta_usec. NOT written to BigTable.
* Currently only set:
* - When a pull task's lease is updated; eta_usec is set to the lease
* expiry time, and previous_eta_usec takes on eta_usec's previous value.
*
*
* optional int64 previous_eta_usec = 18;
* @return Whether the previousEtaUsec field is set.
*/
boolean hasPreviousEtaUsec();
/**
*
* The previous value of eta_usec. NOT written to BigTable.
* Currently only set:
* - When a pull task's lease is updated; eta_usec is set to the lease
* expiry time, and previous_eta_usec takes on eta_usec's previous value.
*
*
* optional int64 previous_eta_usec = 18;
* @return The previousEtaUsec.
*/
long getPreviousEtaUsec();
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
* @return Whether the payload field is set.
*/
boolean hasPayload();
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
* @return The payload.
*/
com.google.apphosting.executor.Task.TaskRunnerPayload getPayload();
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder getPayloadOrBuilder();
/**
*
* The number of times this task has been dispatched in its lifetime. This
* count includes attempts which have been dispatched but haven't received a
* response. This count also includes the first dispatch.
*
*
* optional int64 retry_count = 4 [default = 0];
* @return Whether the retryCount field is set.
*/
boolean hasRetryCount();
/**
*
* The number of times this task has been dispatched in its lifetime. This
* count includes attempts which have been dispatched but haven't received a
* response. This count also includes the first dispatch.
*
*
* optional int64 retry_count = 4 [default = 0];
* @return The retryCount.
*/
long getRetryCount();
/**
*
* Timestamp of when the task is written to the store. This is based on wall
* clock in addition to random lower order bits, in order to make it
* unique. Finally, it is inverted so that a higher value of store_timestamp
* actually means it was generated earlier in time. For more information, see
* StoreTimestampGenerator.
* store_timestamp is different from eta_usec. While eta_usec is specified by
* the client, this field is filled in by executor and its encoded form is an
* internal implementation detail. Cloud Tasks displays the decoded
* store_timestamp as task creation time.
*
*
* optional int64 store_timestamp = 5;
* @return Whether the storeTimestamp field is set.
*/
boolean hasStoreTimestamp();
/**
*
* Timestamp of when the task is written to the store. This is based on wall
* clock in addition to random lower order bits, in order to make it
* unique. Finally, it is inverted so that a higher value of store_timestamp
* actually means it was generated earlier in time. For more information, see
* StoreTimestampGenerator.
* store_timestamp is different from eta_usec. While eta_usec is specified by
* the client, this field is filled in by executor and its encoded form is an
* internal implementation detail. Cloud Tasks displays the decoded
* store_timestamp as task creation time.
*
*
* optional int64 store_timestamp = 5;
* @return The storeTimestamp.
*/
long getStoreTimestamp();
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
* @return Whether the lastInvocationStats field is set.
*/
boolean hasLastInvocationStats();
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
* @return The lastInvocationStats.
*/
com.google.apphosting.executor.Task.TaskRunnerStats getLastInvocationStats();
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
com.google.apphosting.executor.Task.TaskRunnerStatsOrBuilder getLastInvocationStatsOrBuilder();
/**
*
* Human readable description of the task.
*
*
* optional bytes description = 7;
* @return Whether the description field is set.
*/
boolean hasDescription();
/**
*
* Human readable description of the task.
*
*
* optional bytes description = 7;
* @return The description.
*/
com.google.protobuf.ByteString getDescription();
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
* @return Whether the retryParameters field is set.
*/
boolean hasRetryParameters();
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
* @return The retryParameters.
*/
com.google.apphosting.executor.Retry.RetryParameters getRetryParameters();
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
com.google.apphosting.executor.Retry.RetryParametersOrBuilder getRetryParametersOrBuilder();
/**
*
* UTC microseconds timestamp at which this task was first tried (i.e.
* dispatched to a task runner).
*
*
* optional int64 first_try_usec = 9;
* @return Whether the firstTryUsec field is set.
*/
boolean hasFirstTryUsec();
/**
*
* UTC microseconds timestamp at which this task was first tried (i.e.
* dispatched to a task runner).
*
*
* optional int64 first_try_usec = 9;
* @return The firstTryUsec.
*/
long getFirstTryUsec();
/**
*
* Tag associated with this task. This is used as for grouping tasks in a
* queue.
*
*
* optional bytes tag = 10;
* @return Whether the tag field is set.
*/
boolean hasTag();
/**
*
* Tag associated with this task. This is used as for grouping tasks in a
* queue.
*
*
* optional bytes tag = 10;
* @return The tag.
*/
com.google.protobuf.ByteString getTag();
/**
*
* The number of times this task has been dispatched and received a response
* from the execution target.
*
*
* optional int64 execution_count = 11;
* @return Whether the executionCount field is set.
*/
boolean hasExecutionCount();
/**
*
* The number of times this task has been dispatched and received a response
* from the execution target.
*
*
* optional int64 execution_count = 11;
* @return The executionCount.
*/
long getExecutionCount();
/**
*
* The shard number of the index in which this task's index entries are
* located. During the transition period of the Index Key Refactor Project,
* some tasks will have entries in the sharded indexes and have this field
* explicitly set to an index shard number and other tasks will not have
* entries in the sharded indexes and will not have this field explicitly
* set. By setting the default value of this field to zero we are asserting
* that all such tasks should be treated as belonging to index shard zero.
*
*
* optional int32 index_shard_number = 12 [default = 0];
* @return Whether the indexShardNumber field is set.
*/
boolean hasIndexShardNumber();
/**
*
* The shard number of the index in which this task's index entries are
* located. During the transition period of the Index Key Refactor Project,
* some tasks will have entries in the sharded indexes and have this field
* explicitly set to an index shard number and other tasks will not have
* entries in the sharded indexes and will not have this field explicitly
* set. By setting the default value of this field to zero we are asserting
* that all such tasks should be treated as belonging to index shard zero.
*
*
* optional int32 index_shard_number = 12 [default = 0];
* @return The indexShardNumber.
*/
int getIndexShardNumber();
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
* @return Whether the cronRetryParameters field is set.
*/
boolean hasCronRetryParameters();
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
* @return The cronRetryParameters.
*/
com.google.apphosting.executor.Retry.RetryParameters getCronRetryParameters();
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
com.google.apphosting.executor.Retry.RetryParametersOrBuilder getCronRetryParametersOrBuilder();
/**
* optional int64 cron_retry_count = 14 [default = 0];
* @return Whether the cronRetryCount field is set.
*/
boolean hasCronRetryCount();
/**
* optional int64 cron_retry_count = 14 [default = 0];
* @return The cronRetryCount.
*/
long getCronRetryCount();
/**
* optional int64 cron_run_start_usec = 15;
* @return Whether the cronRunStartUsec field is set.
*/
boolean hasCronRunStartUsec();
/**
* optional int64 cron_run_start_usec = 15;
* @return The cronRunStartUsec.
*/
long getCronRunStartUsec();
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
* @return Whether the notifyRecord field is set.
*/
boolean hasNotifyRecord();
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
* @return The notifyRecord.
*/
com.google.apphosting.executor.Task.NotifyRoutingRecord getNotifyRecord();
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
com.google.apphosting.executor.Task.NotifyRoutingRecordOrBuilder getNotifyRecordOrBuilder();
/**
*
* Indicates what API was used to create this task.
*
*
* optional .java.apphosting.ApiSource.Type creation_source_type = 17;
* @return Whether the creationSourceType field is set.
*/
boolean hasCreationSourceType();
/**
*
* Indicates what API was used to create this task.
*
*
* optional .java.apphosting.ApiSource.Type creation_source_type = 17;
* @return The creationSourceType.
*/
com.google.apphosting.executor.Task.ApiSource.Type getCreationSourceType();
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
* @return Whether the taskAuthorization field is set.
*/
boolean hasTaskAuthorization();
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
* @return The taskAuthorization.
*/
com.google.apphosting.executor.Target.TaskAuthorization getTaskAuthorization();
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
com.google.apphosting.executor.Target.TaskAuthorizationOrBuilder getTaskAuthorizationOrBuilder();
}
/**
*
* http://g3doc/apphosting/g3doc/wiki-carryover/executor.md#Definition_of_a_Task
* If adding fields to this message, be sure to update
* ExecutorProtoUtil::CopyTaskExceptPayload().
* NEXT_TAG = 20
*
*
* Protobuf type {@code java.apphosting.TaskDefinition}
*/
public static final class TaskDefinition extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskDefinition)
TaskDefinitionOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskDefinition.newBuilder() to construct.
private TaskDefinition(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskDefinition() {
description_ = com.google.protobuf.ByteString.EMPTY;
tag_ = com.google.protobuf.ByteString.EMPTY;
creationSourceType_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskDefinition();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskDefinition_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskDefinition_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskDefinition.class, com.google.apphosting.executor.Task.TaskDefinition.Builder.class);
}
private int bitField0_;
public static final int TASK_REF_FIELD_NUMBER = 1;
private com.google.apphosting.executor.Task.TaskRef taskRef_;
/**
* required .java.apphosting.TaskRef task_ref = 1;
* @return Whether the taskRef field is set.
*/
@java.lang.Override
public boolean hasTaskRef() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
* @return The taskRef.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRef getTaskRef() {
return taskRef_ == null ? com.google.apphosting.executor.Task.TaskRef.getDefaultInstance() : taskRef_;
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRefOrBuilder getTaskRefOrBuilder() {
return taskRef_ == null ? com.google.apphosting.executor.Task.TaskRef.getDefaultInstance() : taskRef_;
}
public static final int ETA_USEC_FIELD_NUMBER = 2;
private long etaUsec_ = 0L;
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 2;
* @return Whether the etaUsec field is set.
*/
@java.lang.Override
public boolean hasEtaUsec() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 2;
* @return The etaUsec.
*/
@java.lang.Override
public long getEtaUsec() {
return etaUsec_;
}
public static final int PREVIOUS_ETA_USEC_FIELD_NUMBER = 18;
private long previousEtaUsec_ = 0L;
/**
*
* The previous value of eta_usec. NOT written to BigTable.
* Currently only set:
* - When a pull task's lease is updated; eta_usec is set to the lease
* expiry time, and previous_eta_usec takes on eta_usec's previous value.
*
*
* optional int64 previous_eta_usec = 18;
* @return Whether the previousEtaUsec field is set.
*/
@java.lang.Override
public boolean hasPreviousEtaUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The previous value of eta_usec. NOT written to BigTable.
* Currently only set:
* - When a pull task's lease is updated; eta_usec is set to the lease
* expiry time, and previous_eta_usec takes on eta_usec's previous value.
*
*
* optional int64 previous_eta_usec = 18;
* @return The previousEtaUsec.
*/
@java.lang.Override
public long getPreviousEtaUsec() {
return previousEtaUsec_;
}
public static final int PAYLOAD_FIELD_NUMBER = 3;
private com.google.apphosting.executor.Task.TaskRunnerPayload payload_;
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
* @return Whether the payload field is set.
*/
@java.lang.Override
public boolean hasPayload() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
* @return The payload.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerPayload getPayload() {
return payload_ == null ? com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance() : payload_;
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder getPayloadOrBuilder() {
return payload_ == null ? com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance() : payload_;
}
public static final int RETRY_COUNT_FIELD_NUMBER = 4;
private long retryCount_ = 0L;
/**
*
* The number of times this task has been dispatched in its lifetime. This
* count includes attempts which have been dispatched but haven't received a
* response. This count also includes the first dispatch.
*
*
* optional int64 retry_count = 4 [default = 0];
* @return Whether the retryCount field is set.
*/
@java.lang.Override
public boolean hasRetryCount() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The number of times this task has been dispatched in its lifetime. This
* count includes attempts which have been dispatched but haven't received a
* response. This count also includes the first dispatch.
*
*
* optional int64 retry_count = 4 [default = 0];
* @return The retryCount.
*/
@java.lang.Override
public long getRetryCount() {
return retryCount_;
}
public static final int STORE_TIMESTAMP_FIELD_NUMBER = 5;
private long storeTimestamp_ = 0L;
/**
*
* Timestamp of when the task is written to the store. This is based on wall
* clock in addition to random lower order bits, in order to make it
* unique. Finally, it is inverted so that a higher value of store_timestamp
* actually means it was generated earlier in time. For more information, see
* StoreTimestampGenerator.
* store_timestamp is different from eta_usec. While eta_usec is specified by
* the client, this field is filled in by executor and its encoded form is an
* internal implementation detail. Cloud Tasks displays the decoded
* store_timestamp as task creation time.
*
*
* optional int64 store_timestamp = 5;
* @return Whether the storeTimestamp field is set.
*/
@java.lang.Override
public boolean hasStoreTimestamp() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Timestamp of when the task is written to the store. This is based on wall
* clock in addition to random lower order bits, in order to make it
* unique. Finally, it is inverted so that a higher value of store_timestamp
* actually means it was generated earlier in time. For more information, see
* StoreTimestampGenerator.
* store_timestamp is different from eta_usec. While eta_usec is specified by
* the client, this field is filled in by executor and its encoded form is an
* internal implementation detail. Cloud Tasks displays the decoded
* store_timestamp as task creation time.
*
*
* optional int64 store_timestamp = 5;
* @return The storeTimestamp.
*/
@java.lang.Override
public long getStoreTimestamp() {
return storeTimestamp_;
}
public static final int LAST_INVOCATION_STATS_FIELD_NUMBER = 6;
private com.google.apphosting.executor.Task.TaskRunnerStats lastInvocationStats_;
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
* @return Whether the lastInvocationStats field is set.
*/
@java.lang.Override
public boolean hasLastInvocationStats() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
* @return The lastInvocationStats.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerStats getLastInvocationStats() {
return lastInvocationStats_ == null ? com.google.apphosting.executor.Task.TaskRunnerStats.getDefaultInstance() : lastInvocationStats_;
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.TaskRunnerStatsOrBuilder getLastInvocationStatsOrBuilder() {
return lastInvocationStats_ == null ? com.google.apphosting.executor.Task.TaskRunnerStats.getDefaultInstance() : lastInvocationStats_;
}
public static final int DESCRIPTION_FIELD_NUMBER = 7;
private com.google.protobuf.ByteString description_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Human readable description of the task.
*
*
* optional bytes description = 7;
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Human readable description of the task.
*
*
* optional bytes description = 7;
* @return The description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescription() {
return description_;
}
public static final int RETRY_PARAMETERS_FIELD_NUMBER = 8;
private com.google.apphosting.executor.Retry.RetryParameters retryParameters_;
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
* @return Whether the retryParameters field is set.
*/
@java.lang.Override
public boolean hasRetryParameters() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
* @return The retryParameters.
*/
@java.lang.Override
public com.google.apphosting.executor.Retry.RetryParameters getRetryParameters() {
return retryParameters_ == null ? com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance() : retryParameters_;
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
@java.lang.Override
public com.google.apphosting.executor.Retry.RetryParametersOrBuilder getRetryParametersOrBuilder() {
return retryParameters_ == null ? com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance() : retryParameters_;
}
public static final int FIRST_TRY_USEC_FIELD_NUMBER = 9;
private long firstTryUsec_ = 0L;
/**
*
* UTC microseconds timestamp at which this task was first tried (i.e.
* dispatched to a task runner).
*
*
* optional int64 first_try_usec = 9;
* @return Whether the firstTryUsec field is set.
*/
@java.lang.Override
public boolean hasFirstTryUsec() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* UTC microseconds timestamp at which this task was first tried (i.e.
* dispatched to a task runner).
*
*
* optional int64 first_try_usec = 9;
* @return The firstTryUsec.
*/
@java.lang.Override
public long getFirstTryUsec() {
return firstTryUsec_;
}
public static final int TAG_FIELD_NUMBER = 10;
private com.google.protobuf.ByteString tag_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Tag associated with this task. This is used as for grouping tasks in a
* queue.
*
*
* optional bytes tag = 10;
* @return Whether the tag field is set.
*/
@java.lang.Override
public boolean hasTag() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Tag associated with this task. This is used as for grouping tasks in a
* queue.
*
*
* optional bytes tag = 10;
* @return The tag.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTag() {
return tag_;
}
public static final int EXECUTION_COUNT_FIELD_NUMBER = 11;
private long executionCount_ = 0L;
/**
*
* The number of times this task has been dispatched and received a response
* from the execution target.
*
*
* optional int64 execution_count = 11;
* @return Whether the executionCount field is set.
*/
@java.lang.Override
public boolean hasExecutionCount() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* The number of times this task has been dispatched and received a response
* from the execution target.
*
*
* optional int64 execution_count = 11;
* @return The executionCount.
*/
@java.lang.Override
public long getExecutionCount() {
return executionCount_;
}
public static final int INDEX_SHARD_NUMBER_FIELD_NUMBER = 12;
private int indexShardNumber_ = 0;
/**
*
* The shard number of the index in which this task's index entries are
* located. During the transition period of the Index Key Refactor Project,
* some tasks will have entries in the sharded indexes and have this field
* explicitly set to an index shard number and other tasks will not have
* entries in the sharded indexes and will not have this field explicitly
* set. By setting the default value of this field to zero we are asserting
* that all such tasks should be treated as belonging to index shard zero.
*
*
* optional int32 index_shard_number = 12 [default = 0];
* @return Whether the indexShardNumber field is set.
*/
@java.lang.Override
public boolean hasIndexShardNumber() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* The shard number of the index in which this task's index entries are
* located. During the transition period of the Index Key Refactor Project,
* some tasks will have entries in the sharded indexes and have this field
* explicitly set to an index shard number and other tasks will not have
* entries in the sharded indexes and will not have this field explicitly
* set. By setting the default value of this field to zero we are asserting
* that all such tasks should be treated as belonging to index shard zero.
*
*
* optional int32 index_shard_number = 12 [default = 0];
* @return The indexShardNumber.
*/
@java.lang.Override
public int getIndexShardNumber() {
return indexShardNumber_;
}
public static final int CRON_RETRY_PARAMETERS_FIELD_NUMBER = 13;
private com.google.apphosting.executor.Retry.RetryParameters cronRetryParameters_;
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
* @return Whether the cronRetryParameters field is set.
*/
@java.lang.Override
public boolean hasCronRetryParameters() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
* @return The cronRetryParameters.
*/
@java.lang.Override
public com.google.apphosting.executor.Retry.RetryParameters getCronRetryParameters() {
return cronRetryParameters_ == null ? com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance() : cronRetryParameters_;
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
@java.lang.Override
public com.google.apphosting.executor.Retry.RetryParametersOrBuilder getCronRetryParametersOrBuilder() {
return cronRetryParameters_ == null ? com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance() : cronRetryParameters_;
}
public static final int CRON_RETRY_COUNT_FIELD_NUMBER = 14;
private long cronRetryCount_ = 0L;
/**
* optional int64 cron_retry_count = 14 [default = 0];
* @return Whether the cronRetryCount field is set.
*/
@java.lang.Override
public boolean hasCronRetryCount() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
* optional int64 cron_retry_count = 14 [default = 0];
* @return The cronRetryCount.
*/
@java.lang.Override
public long getCronRetryCount() {
return cronRetryCount_;
}
public static final int CRON_RUN_START_USEC_FIELD_NUMBER = 15;
private long cronRunStartUsec_ = 0L;
/**
* optional int64 cron_run_start_usec = 15;
* @return Whether the cronRunStartUsec field is set.
*/
@java.lang.Override
public boolean hasCronRunStartUsec() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
* optional int64 cron_run_start_usec = 15;
* @return The cronRunStartUsec.
*/
@java.lang.Override
public long getCronRunStartUsec() {
return cronRunStartUsec_;
}
public static final int NOTIFY_RECORD_FIELD_NUMBER = 16;
private com.google.apphosting.executor.Task.NotifyRoutingRecord notifyRecord_;
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
* @return Whether the notifyRecord field is set.
*/
@java.lang.Override
public boolean hasNotifyRecord() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
* @return The notifyRecord.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.NotifyRoutingRecord getNotifyRecord() {
return notifyRecord_ == null ? com.google.apphosting.executor.Task.NotifyRoutingRecord.getDefaultInstance() : notifyRecord_;
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
@java.lang.Override
public com.google.apphosting.executor.Task.NotifyRoutingRecordOrBuilder getNotifyRecordOrBuilder() {
return notifyRecord_ == null ? com.google.apphosting.executor.Task.NotifyRoutingRecord.getDefaultInstance() : notifyRecord_;
}
public static final int CREATION_SOURCE_TYPE_FIELD_NUMBER = 17;
private int creationSourceType_ = 0;
/**
*
* Indicates what API was used to create this task.
*
*
* optional .java.apphosting.ApiSource.Type creation_source_type = 17;
* @return Whether the creationSourceType field is set.
*/
@java.lang.Override public boolean hasCreationSourceType() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* Indicates what API was used to create this task.
*
*
* optional .java.apphosting.ApiSource.Type creation_source_type = 17;
* @return The creationSourceType.
*/
@java.lang.Override public com.google.apphosting.executor.Task.ApiSource.Type getCreationSourceType() {
com.google.apphosting.executor.Task.ApiSource.Type result = com.google.apphosting.executor.Task.ApiSource.Type.forNumber(creationSourceType_);
return result == null ? com.google.apphosting.executor.Task.ApiSource.Type.UNKNOWN : result;
}
public static final int TASK_AUTHORIZATION_FIELD_NUMBER = 19;
private com.google.apphosting.executor.Target.TaskAuthorization taskAuthorization_;
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
* @return Whether the taskAuthorization field is set.
*/
@java.lang.Override
public boolean hasTaskAuthorization() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
* @return The taskAuthorization.
*/
@java.lang.Override
public com.google.apphosting.executor.Target.TaskAuthorization getTaskAuthorization() {
return taskAuthorization_ == null ? com.google.apphosting.executor.Target.TaskAuthorization.getDefaultInstance() : taskAuthorization_;
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
@java.lang.Override
public com.google.apphosting.executor.Target.TaskAuthorizationOrBuilder getTaskAuthorizationOrBuilder() {
return taskAuthorization_ == null ? com.google.apphosting.executor.Target.TaskAuthorization.getDefaultInstance() : taskAuthorization_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasTaskRef()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasEtaUsec()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPayload()) {
memoizedIsInitialized = 0;
return false;
}
if (!getTaskRef().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
if (hasLastInvocationStats()) {
if (!getLastInvocationStats().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getTaskRef());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, etaUsec_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(3, getPayload());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt64(4, retryCount_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt64(5, storeTimestamp_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(6, getLastInvocationStats());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeBytes(7, description_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(8, getRetryParameters());
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeInt64(9, firstTryUsec_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeBytes(10, tag_);
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeInt64(11, executionCount_);
}
if (((bitField0_ & 0x00001000) != 0)) {
output.writeInt32(12, indexShardNumber_);
}
if (((bitField0_ & 0x00002000) != 0)) {
output.writeMessage(13, getCronRetryParameters());
}
if (((bitField0_ & 0x00004000) != 0)) {
output.writeInt64(14, cronRetryCount_);
}
if (((bitField0_ & 0x00008000) != 0)) {
output.writeInt64(15, cronRunStartUsec_);
}
if (((bitField0_ & 0x00010000) != 0)) {
output.writeMessage(16, getNotifyRecord());
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeEnum(17, creationSourceType_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(18, previousEtaUsec_);
}
if (((bitField0_ & 0x00040000) != 0)) {
output.writeMessage(19, getTaskAuthorization());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getTaskRef());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, etaUsec_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPayload());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, retryCount_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, storeTimestamp_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getLastInvocationStats());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, description_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getRetryParameters());
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(9, firstTryUsec_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, tag_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, executionCount_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(12, indexShardNumber_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getCronRetryParameters());
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(14, cronRetryCount_);
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(15, cronRunStartUsec_);
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, getNotifyRecord());
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(17, creationSourceType_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(18, previousEtaUsec_);
}
if (((bitField0_ & 0x00040000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, getTaskAuthorization());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskDefinition)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskDefinition other = (com.google.apphosting.executor.Task.TaskDefinition) obj;
if (hasTaskRef() != other.hasTaskRef()) return false;
if (hasTaskRef()) {
if (!getTaskRef()
.equals(other.getTaskRef())) return false;
}
if (hasEtaUsec() != other.hasEtaUsec()) return false;
if (hasEtaUsec()) {
if (getEtaUsec()
!= other.getEtaUsec()) return false;
}
if (hasPreviousEtaUsec() != other.hasPreviousEtaUsec()) return false;
if (hasPreviousEtaUsec()) {
if (getPreviousEtaUsec()
!= other.getPreviousEtaUsec()) return false;
}
if (hasPayload() != other.hasPayload()) return false;
if (hasPayload()) {
if (!getPayload()
.equals(other.getPayload())) return false;
}
if (hasRetryCount() != other.hasRetryCount()) return false;
if (hasRetryCount()) {
if (getRetryCount()
!= other.getRetryCount()) return false;
}
if (hasStoreTimestamp() != other.hasStoreTimestamp()) return false;
if (hasStoreTimestamp()) {
if (getStoreTimestamp()
!= other.getStoreTimestamp()) return false;
}
if (hasLastInvocationStats() != other.hasLastInvocationStats()) return false;
if (hasLastInvocationStats()) {
if (!getLastInvocationStats()
.equals(other.getLastInvocationStats())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription()
.equals(other.getDescription())) return false;
}
if (hasRetryParameters() != other.hasRetryParameters()) return false;
if (hasRetryParameters()) {
if (!getRetryParameters()
.equals(other.getRetryParameters())) return false;
}
if (hasFirstTryUsec() != other.hasFirstTryUsec()) return false;
if (hasFirstTryUsec()) {
if (getFirstTryUsec()
!= other.getFirstTryUsec()) return false;
}
if (hasTag() != other.hasTag()) return false;
if (hasTag()) {
if (!getTag()
.equals(other.getTag())) return false;
}
if (hasExecutionCount() != other.hasExecutionCount()) return false;
if (hasExecutionCount()) {
if (getExecutionCount()
!= other.getExecutionCount()) return false;
}
if (hasIndexShardNumber() != other.hasIndexShardNumber()) return false;
if (hasIndexShardNumber()) {
if (getIndexShardNumber()
!= other.getIndexShardNumber()) return false;
}
if (hasCronRetryParameters() != other.hasCronRetryParameters()) return false;
if (hasCronRetryParameters()) {
if (!getCronRetryParameters()
.equals(other.getCronRetryParameters())) return false;
}
if (hasCronRetryCount() != other.hasCronRetryCount()) return false;
if (hasCronRetryCount()) {
if (getCronRetryCount()
!= other.getCronRetryCount()) return false;
}
if (hasCronRunStartUsec() != other.hasCronRunStartUsec()) return false;
if (hasCronRunStartUsec()) {
if (getCronRunStartUsec()
!= other.getCronRunStartUsec()) return false;
}
if (hasNotifyRecord() != other.hasNotifyRecord()) return false;
if (hasNotifyRecord()) {
if (!getNotifyRecord()
.equals(other.getNotifyRecord())) return false;
}
if (hasCreationSourceType() != other.hasCreationSourceType()) return false;
if (hasCreationSourceType()) {
if (creationSourceType_ != other.creationSourceType_) return false;
}
if (hasTaskAuthorization() != other.hasTaskAuthorization()) return false;
if (hasTaskAuthorization()) {
if (!getTaskAuthorization()
.equals(other.getTaskAuthorization())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasTaskRef()) {
hash = (37 * hash) + TASK_REF_FIELD_NUMBER;
hash = (53 * hash) + getTaskRef().hashCode();
}
if (hasEtaUsec()) {
hash = (37 * hash) + ETA_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getEtaUsec());
}
if (hasPreviousEtaUsec()) {
hash = (37 * hash) + PREVIOUS_ETA_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPreviousEtaUsec());
}
if (hasPayload()) {
hash = (37 * hash) + PAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getPayload().hashCode();
}
if (hasRetryCount()) {
hash = (37 * hash) + RETRY_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRetryCount());
}
if (hasStoreTimestamp()) {
hash = (37 * hash) + STORE_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStoreTimestamp());
}
if (hasLastInvocationStats()) {
hash = (37 * hash) + LAST_INVOCATION_STATS_FIELD_NUMBER;
hash = (53 * hash) + getLastInvocationStats().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasRetryParameters()) {
hash = (37 * hash) + RETRY_PARAMETERS_FIELD_NUMBER;
hash = (53 * hash) + getRetryParameters().hashCode();
}
if (hasFirstTryUsec()) {
hash = (37 * hash) + FIRST_TRY_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFirstTryUsec());
}
if (hasTag()) {
hash = (37 * hash) + TAG_FIELD_NUMBER;
hash = (53 * hash) + getTag().hashCode();
}
if (hasExecutionCount()) {
hash = (37 * hash) + EXECUTION_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getExecutionCount());
}
if (hasIndexShardNumber()) {
hash = (37 * hash) + INDEX_SHARD_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getIndexShardNumber();
}
if (hasCronRetryParameters()) {
hash = (37 * hash) + CRON_RETRY_PARAMETERS_FIELD_NUMBER;
hash = (53 * hash) + getCronRetryParameters().hashCode();
}
if (hasCronRetryCount()) {
hash = (37 * hash) + CRON_RETRY_COUNT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCronRetryCount());
}
if (hasCronRunStartUsec()) {
hash = (37 * hash) + CRON_RUN_START_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCronRunStartUsec());
}
if (hasNotifyRecord()) {
hash = (37 * hash) + NOTIFY_RECORD_FIELD_NUMBER;
hash = (53 * hash) + getNotifyRecord().hashCode();
}
if (hasCreationSourceType()) {
hash = (37 * hash) + CREATION_SOURCE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + creationSourceType_;
}
if (hasTaskAuthorization()) {
hash = (37 * hash) + TASK_AUTHORIZATION_FIELD_NUMBER;
hash = (53 * hash) + getTaskAuthorization().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskDefinition parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskDefinition prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* http://g3doc/apphosting/g3doc/wiki-carryover/executor.md#Definition_of_a_Task
* If adding fields to this message, be sure to update
* ExecutorProtoUtil::CopyTaskExceptPayload().
* NEXT_TAG = 20
*
*
* Protobuf type {@code java.apphosting.TaskDefinition}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskDefinition)
com.google.apphosting.executor.Task.TaskDefinitionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskDefinition_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskDefinition_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskDefinition.class, com.google.apphosting.executor.Task.TaskDefinition.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskDefinition.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTaskRefFieldBuilder();
getPayloadFieldBuilder();
getLastInvocationStatsFieldBuilder();
getRetryParametersFieldBuilder();
getCronRetryParametersFieldBuilder();
getNotifyRecordFieldBuilder();
getTaskAuthorizationFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
taskRef_ = null;
if (taskRefBuilder_ != null) {
taskRefBuilder_.dispose();
taskRefBuilder_ = null;
}
etaUsec_ = 0L;
previousEtaUsec_ = 0L;
payload_ = null;
if (payloadBuilder_ != null) {
payloadBuilder_.dispose();
payloadBuilder_ = null;
}
retryCount_ = 0L;
storeTimestamp_ = 0L;
lastInvocationStats_ = null;
if (lastInvocationStatsBuilder_ != null) {
lastInvocationStatsBuilder_.dispose();
lastInvocationStatsBuilder_ = null;
}
description_ = com.google.protobuf.ByteString.EMPTY;
retryParameters_ = null;
if (retryParametersBuilder_ != null) {
retryParametersBuilder_.dispose();
retryParametersBuilder_ = null;
}
firstTryUsec_ = 0L;
tag_ = com.google.protobuf.ByteString.EMPTY;
executionCount_ = 0L;
indexShardNumber_ = 0;
cronRetryParameters_ = null;
if (cronRetryParametersBuilder_ != null) {
cronRetryParametersBuilder_.dispose();
cronRetryParametersBuilder_ = null;
}
cronRetryCount_ = 0L;
cronRunStartUsec_ = 0L;
notifyRecord_ = null;
if (notifyRecordBuilder_ != null) {
notifyRecordBuilder_.dispose();
notifyRecordBuilder_ = null;
}
creationSourceType_ = 0;
taskAuthorization_ = null;
if (taskAuthorizationBuilder_ != null) {
taskAuthorizationBuilder_.dispose();
taskAuthorizationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskDefinition_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskDefinition getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskDefinition.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskDefinition build() {
com.google.apphosting.executor.Task.TaskDefinition result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskDefinition buildPartial() {
com.google.apphosting.executor.Task.TaskDefinition result = new com.google.apphosting.executor.Task.TaskDefinition(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskDefinition result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.taskRef_ = taskRefBuilder_ == null
? taskRef_
: taskRefBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.etaUsec_ = etaUsec_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.previousEtaUsec_ = previousEtaUsec_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.payload_ = payloadBuilder_ == null
? payload_
: payloadBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.retryCount_ = retryCount_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.storeTimestamp_ = storeTimestamp_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.lastInvocationStats_ = lastInvocationStatsBuilder_ == null
? lastInvocationStats_
: lastInvocationStatsBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.retryParameters_ = retryParametersBuilder_ == null
? retryParameters_
: retryParametersBuilder_.build();
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.firstTryUsec_ = firstTryUsec_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.tag_ = tag_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.executionCount_ = executionCount_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.indexShardNumber_ = indexShardNumber_;
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.cronRetryParameters_ = cronRetryParametersBuilder_ == null
? cronRetryParameters_
: cronRetryParametersBuilder_.build();
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.cronRetryCount_ = cronRetryCount_;
to_bitField0_ |= 0x00004000;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.cronRunStartUsec_ = cronRunStartUsec_;
to_bitField0_ |= 0x00008000;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.notifyRecord_ = notifyRecordBuilder_ == null
? notifyRecord_
: notifyRecordBuilder_.build();
to_bitField0_ |= 0x00010000;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.creationSourceType_ = creationSourceType_;
to_bitField0_ |= 0x00020000;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.taskAuthorization_ = taskAuthorizationBuilder_ == null
? taskAuthorization_
: taskAuthorizationBuilder_.build();
to_bitField0_ |= 0x00040000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskDefinition) {
return mergeFrom((com.google.apphosting.executor.Task.TaskDefinition)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskDefinition other) {
if (other == com.google.apphosting.executor.Task.TaskDefinition.getDefaultInstance()) return this;
if (other.hasTaskRef()) {
mergeTaskRef(other.getTaskRef());
}
if (other.hasEtaUsec()) {
setEtaUsec(other.getEtaUsec());
}
if (other.hasPreviousEtaUsec()) {
setPreviousEtaUsec(other.getPreviousEtaUsec());
}
if (other.hasPayload()) {
mergePayload(other.getPayload());
}
if (other.hasRetryCount()) {
setRetryCount(other.getRetryCount());
}
if (other.hasStoreTimestamp()) {
setStoreTimestamp(other.getStoreTimestamp());
}
if (other.hasLastInvocationStats()) {
mergeLastInvocationStats(other.getLastInvocationStats());
}
if (other.hasDescription()) {
setDescription(other.getDescription());
}
if (other.hasRetryParameters()) {
mergeRetryParameters(other.getRetryParameters());
}
if (other.hasFirstTryUsec()) {
setFirstTryUsec(other.getFirstTryUsec());
}
if (other.hasTag()) {
setTag(other.getTag());
}
if (other.hasExecutionCount()) {
setExecutionCount(other.getExecutionCount());
}
if (other.hasIndexShardNumber()) {
setIndexShardNumber(other.getIndexShardNumber());
}
if (other.hasCronRetryParameters()) {
mergeCronRetryParameters(other.getCronRetryParameters());
}
if (other.hasCronRetryCount()) {
setCronRetryCount(other.getCronRetryCount());
}
if (other.hasCronRunStartUsec()) {
setCronRunStartUsec(other.getCronRunStartUsec());
}
if (other.hasNotifyRecord()) {
mergeNotifyRecord(other.getNotifyRecord());
}
if (other.hasCreationSourceType()) {
setCreationSourceType(other.getCreationSourceType());
}
if (other.hasTaskAuthorization()) {
mergeTaskAuthorization(other.getTaskAuthorization());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasTaskRef()) {
return false;
}
if (!hasEtaUsec()) {
return false;
}
if (!hasPayload()) {
return false;
}
if (!getTaskRef().isInitialized()) {
return false;
}
if (hasLastInvocationStats()) {
if (!getLastInvocationStats().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getTaskRefFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
etaUsec_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
input.readMessage(
getPayloadFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 26
case 32: {
retryCount_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 32
case 40: {
storeTimestamp_ = input.readInt64();
bitField0_ |= 0x00000020;
break;
} // case 40
case 50: {
input.readMessage(
getLastInvocationStatsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 50
case 58: {
description_ = input.readBytes();
bitField0_ |= 0x00000080;
break;
} // case 58
case 66: {
input.readMessage(
getRetryParametersFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 66
case 72: {
firstTryUsec_ = input.readInt64();
bitField0_ |= 0x00000200;
break;
} // case 72
case 82: {
tag_ = input.readBytes();
bitField0_ |= 0x00000400;
break;
} // case 82
case 88: {
executionCount_ = input.readInt64();
bitField0_ |= 0x00000800;
break;
} // case 88
case 96: {
indexShardNumber_ = input.readInt32();
bitField0_ |= 0x00001000;
break;
} // case 96
case 106: {
input.readMessage(
getCronRetryParametersFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00002000;
break;
} // case 106
case 112: {
cronRetryCount_ = input.readInt64();
bitField0_ |= 0x00004000;
break;
} // case 112
case 120: {
cronRunStartUsec_ = input.readInt64();
bitField0_ |= 0x00008000;
break;
} // case 120
case 130: {
input.readMessage(
getNotifyRecordFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00010000;
break;
} // case 130
case 136: {
int tmpRaw = input.readEnum();
com.google.apphosting.executor.Task.ApiSource.Type tmpValue =
com.google.apphosting.executor.Task.ApiSource.Type.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(17, tmpRaw);
} else {
creationSourceType_ = tmpRaw;
bitField0_ |= 0x00020000;
}
break;
} // case 136
case 144: {
previousEtaUsec_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 144
case 154: {
input.readMessage(
getTaskAuthorizationFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00040000;
break;
} // case 154
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.apphosting.executor.Task.TaskRef taskRef_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRef, com.google.apphosting.executor.Task.TaskRef.Builder, com.google.apphosting.executor.Task.TaskRefOrBuilder> taskRefBuilder_;
/**
* required .java.apphosting.TaskRef task_ref = 1;
* @return Whether the taskRef field is set.
*/
public boolean hasTaskRef() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
* @return The taskRef.
*/
public com.google.apphosting.executor.Task.TaskRef getTaskRef() {
if (taskRefBuilder_ == null) {
return taskRef_ == null ? com.google.apphosting.executor.Task.TaskRef.getDefaultInstance() : taskRef_;
} else {
return taskRefBuilder_.getMessage();
}
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
public Builder setTaskRef(com.google.apphosting.executor.Task.TaskRef value) {
if (taskRefBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
taskRef_ = value;
} else {
taskRefBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
public Builder setTaskRef(
com.google.apphosting.executor.Task.TaskRef.Builder builderForValue) {
if (taskRefBuilder_ == null) {
taskRef_ = builderForValue.build();
} else {
taskRefBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
public Builder mergeTaskRef(com.google.apphosting.executor.Task.TaskRef value) {
if (taskRefBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
taskRef_ != null &&
taskRef_ != com.google.apphosting.executor.Task.TaskRef.getDefaultInstance()) {
getTaskRefBuilder().mergeFrom(value);
} else {
taskRef_ = value;
}
} else {
taskRefBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
public Builder clearTaskRef() {
bitField0_ = (bitField0_ & ~0x00000001);
taskRef_ = null;
if (taskRefBuilder_ != null) {
taskRefBuilder_.dispose();
taskRefBuilder_ = null;
}
onChanged();
return this;
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
public com.google.apphosting.executor.Task.TaskRef.Builder getTaskRefBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getTaskRefFieldBuilder().getBuilder();
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
public com.google.apphosting.executor.Task.TaskRefOrBuilder getTaskRefOrBuilder() {
if (taskRefBuilder_ != null) {
return taskRefBuilder_.getMessageOrBuilder();
} else {
return taskRef_ == null ?
com.google.apphosting.executor.Task.TaskRef.getDefaultInstance() : taskRef_;
}
}
/**
* required .java.apphosting.TaskRef task_ref = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRef, com.google.apphosting.executor.Task.TaskRef.Builder, com.google.apphosting.executor.Task.TaskRefOrBuilder>
getTaskRefFieldBuilder() {
if (taskRefBuilder_ == null) {
taskRefBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRef, com.google.apphosting.executor.Task.TaskRef.Builder, com.google.apphosting.executor.Task.TaskRefOrBuilder>(
getTaskRef(),
getParentForChildren(),
isClean());
taskRef_ = null;
}
return taskRefBuilder_;
}
private long etaUsec_ ;
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 2;
* @return Whether the etaUsec field is set.
*/
@java.lang.Override
public boolean hasEtaUsec() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 2;
* @return The etaUsec.
*/
@java.lang.Override
public long getEtaUsec() {
return etaUsec_;
}
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 2;
* @param value The etaUsec to set.
* @return This builder for chaining.
*/
public Builder setEtaUsec(long value) {
etaUsec_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Time to execute task, in microseconds since the unix epoch.
*
*
* required int64 eta_usec = 2;
* @return This builder for chaining.
*/
public Builder clearEtaUsec() {
bitField0_ = (bitField0_ & ~0x00000002);
etaUsec_ = 0L;
onChanged();
return this;
}
private long previousEtaUsec_ ;
/**
*
* The previous value of eta_usec. NOT written to BigTable.
* Currently only set:
* - When a pull task's lease is updated; eta_usec is set to the lease
* expiry time, and previous_eta_usec takes on eta_usec's previous value.
*
*
* optional int64 previous_eta_usec = 18;
* @return Whether the previousEtaUsec field is set.
*/
@java.lang.Override
public boolean hasPreviousEtaUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The previous value of eta_usec. NOT written to BigTable.
* Currently only set:
* - When a pull task's lease is updated; eta_usec is set to the lease
* expiry time, and previous_eta_usec takes on eta_usec's previous value.
*
*
* optional int64 previous_eta_usec = 18;
* @return The previousEtaUsec.
*/
@java.lang.Override
public long getPreviousEtaUsec() {
return previousEtaUsec_;
}
/**
*
* The previous value of eta_usec. NOT written to BigTable.
* Currently only set:
* - When a pull task's lease is updated; eta_usec is set to the lease
* expiry time, and previous_eta_usec takes on eta_usec's previous value.
*
*
* optional int64 previous_eta_usec = 18;
* @param value The previousEtaUsec to set.
* @return This builder for chaining.
*/
public Builder setPreviousEtaUsec(long value) {
previousEtaUsec_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The previous value of eta_usec. NOT written to BigTable.
* Currently only set:
* - When a pull task's lease is updated; eta_usec is set to the lease
* expiry time, and previous_eta_usec takes on eta_usec's previous value.
*
*
* optional int64 previous_eta_usec = 18;
* @return This builder for chaining.
*/
public Builder clearPreviousEtaUsec() {
bitField0_ = (bitField0_ & ~0x00000004);
previousEtaUsec_ = 0L;
onChanged();
return this;
}
private com.google.apphosting.executor.Task.TaskRunnerPayload payload_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerPayload, com.google.apphosting.executor.Task.TaskRunnerPayload.Builder, com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder> payloadBuilder_;
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
* @return Whether the payload field is set.
*/
public boolean hasPayload() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
* @return The payload.
*/
public com.google.apphosting.executor.Task.TaskRunnerPayload getPayload() {
if (payloadBuilder_ == null) {
return payload_ == null ? com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance() : payload_;
} else {
return payloadBuilder_.getMessage();
}
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
public Builder setPayload(com.google.apphosting.executor.Task.TaskRunnerPayload value) {
if (payloadBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
payload_ = value;
} else {
payloadBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
public Builder setPayload(
com.google.apphosting.executor.Task.TaskRunnerPayload.Builder builderForValue) {
if (payloadBuilder_ == null) {
payload_ = builderForValue.build();
} else {
payloadBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
public Builder mergePayload(com.google.apphosting.executor.Task.TaskRunnerPayload value) {
if (payloadBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
payload_ != null &&
payload_ != com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance()) {
getPayloadBuilder().mergeFrom(value);
} else {
payload_ = value;
}
} else {
payloadBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
public Builder clearPayload() {
bitField0_ = (bitField0_ & ~0x00000008);
payload_ = null;
if (payloadBuilder_ != null) {
payloadBuilder_.dispose();
payloadBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
public com.google.apphosting.executor.Task.TaskRunnerPayload.Builder getPayloadBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getPayloadFieldBuilder().getBuilder();
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
public com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder getPayloadOrBuilder() {
if (payloadBuilder_ != null) {
return payloadBuilder_.getMessageOrBuilder();
} else {
return payload_ == null ?
com.google.apphosting.executor.Task.TaskRunnerPayload.getDefaultInstance() : payload_;
}
}
/**
*
* Task payload.
*
*
* required .java.apphosting.TaskRunnerPayload payload = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerPayload, com.google.apphosting.executor.Task.TaskRunnerPayload.Builder, com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder>
getPayloadFieldBuilder() {
if (payloadBuilder_ == null) {
payloadBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerPayload, com.google.apphosting.executor.Task.TaskRunnerPayload.Builder, com.google.apphosting.executor.Task.TaskRunnerPayloadOrBuilder>(
getPayload(),
getParentForChildren(),
isClean());
payload_ = null;
}
return payloadBuilder_;
}
private long retryCount_ ;
/**
*
* The number of times this task has been dispatched in its lifetime. This
* count includes attempts which have been dispatched but haven't received a
* response. This count also includes the first dispatch.
*
*
* optional int64 retry_count = 4 [default = 0];
* @return Whether the retryCount field is set.
*/
@java.lang.Override
public boolean hasRetryCount() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The number of times this task has been dispatched in its lifetime. This
* count includes attempts which have been dispatched but haven't received a
* response. This count also includes the first dispatch.
*
*
* optional int64 retry_count = 4 [default = 0];
* @return The retryCount.
*/
@java.lang.Override
public long getRetryCount() {
return retryCount_;
}
/**
*
* The number of times this task has been dispatched in its lifetime. This
* count includes attempts which have been dispatched but haven't received a
* response. This count also includes the first dispatch.
*
*
* optional int64 retry_count = 4 [default = 0];
* @param value The retryCount to set.
* @return This builder for chaining.
*/
public Builder setRetryCount(long value) {
retryCount_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The number of times this task has been dispatched in its lifetime. This
* count includes attempts which have been dispatched but haven't received a
* response. This count also includes the first dispatch.
*
*
* optional int64 retry_count = 4 [default = 0];
* @return This builder for chaining.
*/
public Builder clearRetryCount() {
bitField0_ = (bitField0_ & ~0x00000010);
retryCount_ = 0L;
onChanged();
return this;
}
private long storeTimestamp_ ;
/**
*
* Timestamp of when the task is written to the store. This is based on wall
* clock in addition to random lower order bits, in order to make it
* unique. Finally, it is inverted so that a higher value of store_timestamp
* actually means it was generated earlier in time. For more information, see
* StoreTimestampGenerator.
* store_timestamp is different from eta_usec. While eta_usec is specified by
* the client, this field is filled in by executor and its encoded form is an
* internal implementation detail. Cloud Tasks displays the decoded
* store_timestamp as task creation time.
*
*
* optional int64 store_timestamp = 5;
* @return Whether the storeTimestamp field is set.
*/
@java.lang.Override
public boolean hasStoreTimestamp() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Timestamp of when the task is written to the store. This is based on wall
* clock in addition to random lower order bits, in order to make it
* unique. Finally, it is inverted so that a higher value of store_timestamp
* actually means it was generated earlier in time. For more information, see
* StoreTimestampGenerator.
* store_timestamp is different from eta_usec. While eta_usec is specified by
* the client, this field is filled in by executor and its encoded form is an
* internal implementation detail. Cloud Tasks displays the decoded
* store_timestamp as task creation time.
*
*
* optional int64 store_timestamp = 5;
* @return The storeTimestamp.
*/
@java.lang.Override
public long getStoreTimestamp() {
return storeTimestamp_;
}
/**
*
* Timestamp of when the task is written to the store. This is based on wall
* clock in addition to random lower order bits, in order to make it
* unique. Finally, it is inverted so that a higher value of store_timestamp
* actually means it was generated earlier in time. For more information, see
* StoreTimestampGenerator.
* store_timestamp is different from eta_usec. While eta_usec is specified by
* the client, this field is filled in by executor and its encoded form is an
* internal implementation detail. Cloud Tasks displays the decoded
* store_timestamp as task creation time.
*
*
* optional int64 store_timestamp = 5;
* @param value The storeTimestamp to set.
* @return This builder for chaining.
*/
public Builder setStoreTimestamp(long value) {
storeTimestamp_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Timestamp of when the task is written to the store. This is based on wall
* clock in addition to random lower order bits, in order to make it
* unique. Finally, it is inverted so that a higher value of store_timestamp
* actually means it was generated earlier in time. For more information, see
* StoreTimestampGenerator.
* store_timestamp is different from eta_usec. While eta_usec is specified by
* the client, this field is filled in by executor and its encoded form is an
* internal implementation detail. Cloud Tasks displays the decoded
* store_timestamp as task creation time.
*
*
* optional int64 store_timestamp = 5;
* @return This builder for chaining.
*/
public Builder clearStoreTimestamp() {
bitField0_ = (bitField0_ & ~0x00000020);
storeTimestamp_ = 0L;
onChanged();
return this;
}
private com.google.apphosting.executor.Task.TaskRunnerStats lastInvocationStats_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerStats, com.google.apphosting.executor.Task.TaskRunnerStats.Builder, com.google.apphosting.executor.Task.TaskRunnerStatsOrBuilder> lastInvocationStatsBuilder_;
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
* @return Whether the lastInvocationStats field is set.
*/
public boolean hasLastInvocationStats() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
* @return The lastInvocationStats.
*/
public com.google.apphosting.executor.Task.TaskRunnerStats getLastInvocationStats() {
if (lastInvocationStatsBuilder_ == null) {
return lastInvocationStats_ == null ? com.google.apphosting.executor.Task.TaskRunnerStats.getDefaultInstance() : lastInvocationStats_;
} else {
return lastInvocationStatsBuilder_.getMessage();
}
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
public Builder setLastInvocationStats(com.google.apphosting.executor.Task.TaskRunnerStats value) {
if (lastInvocationStatsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastInvocationStats_ = value;
} else {
lastInvocationStatsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
public Builder setLastInvocationStats(
com.google.apphosting.executor.Task.TaskRunnerStats.Builder builderForValue) {
if (lastInvocationStatsBuilder_ == null) {
lastInvocationStats_ = builderForValue.build();
} else {
lastInvocationStatsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
public Builder mergeLastInvocationStats(com.google.apphosting.executor.Task.TaskRunnerStats value) {
if (lastInvocationStatsBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
lastInvocationStats_ != null &&
lastInvocationStats_ != com.google.apphosting.executor.Task.TaskRunnerStats.getDefaultInstance()) {
getLastInvocationStatsBuilder().mergeFrom(value);
} else {
lastInvocationStats_ = value;
}
} else {
lastInvocationStatsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
public Builder clearLastInvocationStats() {
bitField0_ = (bitField0_ & ~0x00000040);
lastInvocationStats_ = null;
if (lastInvocationStatsBuilder_ != null) {
lastInvocationStatsBuilder_.dispose();
lastInvocationStatsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
public com.google.apphosting.executor.Task.TaskRunnerStats.Builder getLastInvocationStatsBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getLastInvocationStatsFieldBuilder().getBuilder();
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
public com.google.apphosting.executor.Task.TaskRunnerStatsOrBuilder getLastInvocationStatsOrBuilder() {
if (lastInvocationStatsBuilder_ != null) {
return lastInvocationStatsBuilder_.getMessageOrBuilder();
} else {
return lastInvocationStats_ == null ?
com.google.apphosting.executor.Task.TaskRunnerStats.getDefaultInstance() : lastInvocationStats_;
}
}
/**
*
* Information about last task's invocation.
*
*
* optional .java.apphosting.TaskRunnerStats last_invocation_stats = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerStats, com.google.apphosting.executor.Task.TaskRunnerStats.Builder, com.google.apphosting.executor.Task.TaskRunnerStatsOrBuilder>
getLastInvocationStatsFieldBuilder() {
if (lastInvocationStatsBuilder_ == null) {
lastInvocationStatsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.TaskRunnerStats, com.google.apphosting.executor.Task.TaskRunnerStats.Builder, com.google.apphosting.executor.Task.TaskRunnerStatsOrBuilder>(
getLastInvocationStats(),
getParentForChildren(),
isClean());
lastInvocationStats_ = null;
}
return lastInvocationStatsBuilder_;
}
private com.google.protobuf.ByteString description_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Human readable description of the task.
*
*
* optional bytes description = 7;
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Human readable description of the task.
*
*
* optional bytes description = 7;
* @return The description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescription() {
return description_;
}
/**
*
* Human readable description of the task.
*
*
* optional bytes description = 7;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Human readable description of the task.
*
*
* optional bytes description = 7;
* @return This builder for chaining.
*/
public Builder clearDescription() {
bitField0_ = (bitField0_ & ~0x00000080);
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
private com.google.apphosting.executor.Retry.RetryParameters retryParameters_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Retry.RetryParameters, com.google.apphosting.executor.Retry.RetryParameters.Builder, com.google.apphosting.executor.Retry.RetryParametersOrBuilder> retryParametersBuilder_;
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
* @return Whether the retryParameters field is set.
*/
public boolean hasRetryParameters() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
* @return The retryParameters.
*/
public com.google.apphosting.executor.Retry.RetryParameters getRetryParameters() {
if (retryParametersBuilder_ == null) {
return retryParameters_ == null ? com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance() : retryParameters_;
} else {
return retryParametersBuilder_.getMessage();
}
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
public Builder setRetryParameters(com.google.apphosting.executor.Retry.RetryParameters value) {
if (retryParametersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
retryParameters_ = value;
} else {
retryParametersBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
public Builder setRetryParameters(
com.google.apphosting.executor.Retry.RetryParameters.Builder builderForValue) {
if (retryParametersBuilder_ == null) {
retryParameters_ = builderForValue.build();
} else {
retryParametersBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
public Builder mergeRetryParameters(com.google.apphosting.executor.Retry.RetryParameters value) {
if (retryParametersBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0) &&
retryParameters_ != null &&
retryParameters_ != com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance()) {
getRetryParametersBuilder().mergeFrom(value);
} else {
retryParameters_ = value;
}
} else {
retryParametersBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
public Builder clearRetryParameters() {
bitField0_ = (bitField0_ & ~0x00000100);
retryParameters_ = null;
if (retryParametersBuilder_ != null) {
retryParametersBuilder_.dispose();
retryParametersBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
public com.google.apphosting.executor.Retry.RetryParameters.Builder getRetryParametersBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getRetryParametersFieldBuilder().getBuilder();
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
public com.google.apphosting.executor.Retry.RetryParametersOrBuilder getRetryParametersOrBuilder() {
if (retryParametersBuilder_ != null) {
return retryParametersBuilder_.getMessageOrBuilder();
} else {
return retryParameters_ == null ?
com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance() : retryParameters_;
}
}
/**
*
* Information about how to retry this task on failure. Overrides any
* retry_parameters on the task's queue.
*
*
* optional .java.apphosting.RetryParameters retry_parameters = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Retry.RetryParameters, com.google.apphosting.executor.Retry.RetryParameters.Builder, com.google.apphosting.executor.Retry.RetryParametersOrBuilder>
getRetryParametersFieldBuilder() {
if (retryParametersBuilder_ == null) {
retryParametersBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Retry.RetryParameters, com.google.apphosting.executor.Retry.RetryParameters.Builder, com.google.apphosting.executor.Retry.RetryParametersOrBuilder>(
getRetryParameters(),
getParentForChildren(),
isClean());
retryParameters_ = null;
}
return retryParametersBuilder_;
}
private long firstTryUsec_ ;
/**
*
* UTC microseconds timestamp at which this task was first tried (i.e.
* dispatched to a task runner).
*
*
* optional int64 first_try_usec = 9;
* @return Whether the firstTryUsec field is set.
*/
@java.lang.Override
public boolean hasFirstTryUsec() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* UTC microseconds timestamp at which this task was first tried (i.e.
* dispatched to a task runner).
*
*
* optional int64 first_try_usec = 9;
* @return The firstTryUsec.
*/
@java.lang.Override
public long getFirstTryUsec() {
return firstTryUsec_;
}
/**
*
* UTC microseconds timestamp at which this task was first tried (i.e.
* dispatched to a task runner).
*
*
* optional int64 first_try_usec = 9;
* @param value The firstTryUsec to set.
* @return This builder for chaining.
*/
public Builder setFirstTryUsec(long value) {
firstTryUsec_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* UTC microseconds timestamp at which this task was first tried (i.e.
* dispatched to a task runner).
*
*
* optional int64 first_try_usec = 9;
* @return This builder for chaining.
*/
public Builder clearFirstTryUsec() {
bitField0_ = (bitField0_ & ~0x00000200);
firstTryUsec_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString tag_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Tag associated with this task. This is used as for grouping tasks in a
* queue.
*
*
* optional bytes tag = 10;
* @return Whether the tag field is set.
*/
@java.lang.Override
public boolean hasTag() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Tag associated with this task. This is used as for grouping tasks in a
* queue.
*
*
* optional bytes tag = 10;
* @return The tag.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTag() {
return tag_;
}
/**
*
* Tag associated with this task. This is used as for grouping tasks in a
* queue.
*
*
* optional bytes tag = 10;
* @param value The tag to set.
* @return This builder for chaining.
*/
public Builder setTag(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
tag_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* Tag associated with this task. This is used as for grouping tasks in a
* queue.
*
*
* optional bytes tag = 10;
* @return This builder for chaining.
*/
public Builder clearTag() {
bitField0_ = (bitField0_ & ~0x00000400);
tag_ = getDefaultInstance().getTag();
onChanged();
return this;
}
private long executionCount_ ;
/**
*
* The number of times this task has been dispatched and received a response
* from the execution target.
*
*
* optional int64 execution_count = 11;
* @return Whether the executionCount field is set.
*/
@java.lang.Override
public boolean hasExecutionCount() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* The number of times this task has been dispatched and received a response
* from the execution target.
*
*
* optional int64 execution_count = 11;
* @return The executionCount.
*/
@java.lang.Override
public long getExecutionCount() {
return executionCount_;
}
/**
*
* The number of times this task has been dispatched and received a response
* from the execution target.
*
*
* optional int64 execution_count = 11;
* @param value The executionCount to set.
* @return This builder for chaining.
*/
public Builder setExecutionCount(long value) {
executionCount_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* The number of times this task has been dispatched and received a response
* from the execution target.
*
*
* optional int64 execution_count = 11;
* @return This builder for chaining.
*/
public Builder clearExecutionCount() {
bitField0_ = (bitField0_ & ~0x00000800);
executionCount_ = 0L;
onChanged();
return this;
}
private int indexShardNumber_ ;
/**
*
* The shard number of the index in which this task's index entries are
* located. During the transition period of the Index Key Refactor Project,
* some tasks will have entries in the sharded indexes and have this field
* explicitly set to an index shard number and other tasks will not have
* entries in the sharded indexes and will not have this field explicitly
* set. By setting the default value of this field to zero we are asserting
* that all such tasks should be treated as belonging to index shard zero.
*
*
* optional int32 index_shard_number = 12 [default = 0];
* @return Whether the indexShardNumber field is set.
*/
@java.lang.Override
public boolean hasIndexShardNumber() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* The shard number of the index in which this task's index entries are
* located. During the transition period of the Index Key Refactor Project,
* some tasks will have entries in the sharded indexes and have this field
* explicitly set to an index shard number and other tasks will not have
* entries in the sharded indexes and will not have this field explicitly
* set. By setting the default value of this field to zero we are asserting
* that all such tasks should be treated as belonging to index shard zero.
*
*
* optional int32 index_shard_number = 12 [default = 0];
* @return The indexShardNumber.
*/
@java.lang.Override
public int getIndexShardNumber() {
return indexShardNumber_;
}
/**
*
* The shard number of the index in which this task's index entries are
* located. During the transition period of the Index Key Refactor Project,
* some tasks will have entries in the sharded indexes and have this field
* explicitly set to an index shard number and other tasks will not have
* entries in the sharded indexes and will not have this field explicitly
* set. By setting the default value of this field to zero we are asserting
* that all such tasks should be treated as belonging to index shard zero.
*
*
* optional int32 index_shard_number = 12 [default = 0];
* @param value The indexShardNumber to set.
* @return This builder for chaining.
*/
public Builder setIndexShardNumber(int value) {
indexShardNumber_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* The shard number of the index in which this task's index entries are
* located. During the transition period of the Index Key Refactor Project,
* some tasks will have entries in the sharded indexes and have this field
* explicitly set to an index shard number and other tasks will not have
* entries in the sharded indexes and will not have this field explicitly
* set. By setting the default value of this field to zero we are asserting
* that all such tasks should be treated as belonging to index shard zero.
*
*
* optional int32 index_shard_number = 12 [default = 0];
* @return This builder for chaining.
*/
public Builder clearIndexShardNumber() {
bitField0_ = (bitField0_ & ~0x00001000);
indexShardNumber_ = 0;
onChanged();
return this;
}
private com.google.apphosting.executor.Retry.RetryParameters cronRetryParameters_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Retry.RetryParameters, com.google.apphosting.executor.Retry.RetryParameters.Builder, com.google.apphosting.executor.Retry.RetryParametersOrBuilder> cronRetryParametersBuilder_;
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
* @return Whether the cronRetryParameters field is set.
*/
public boolean hasCronRetryParameters() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
* @return The cronRetryParameters.
*/
public com.google.apphosting.executor.Retry.RetryParameters getCronRetryParameters() {
if (cronRetryParametersBuilder_ == null) {
return cronRetryParameters_ == null ? com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance() : cronRetryParameters_;
} else {
return cronRetryParametersBuilder_.getMessage();
}
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
public Builder setCronRetryParameters(com.google.apphosting.executor.Retry.RetryParameters value) {
if (cronRetryParametersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cronRetryParameters_ = value;
} else {
cronRetryParametersBuilder_.setMessage(value);
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
public Builder setCronRetryParameters(
com.google.apphosting.executor.Retry.RetryParameters.Builder builderForValue) {
if (cronRetryParametersBuilder_ == null) {
cronRetryParameters_ = builderForValue.build();
} else {
cronRetryParametersBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
public Builder mergeCronRetryParameters(com.google.apphosting.executor.Retry.RetryParameters value) {
if (cronRetryParametersBuilder_ == null) {
if (((bitField0_ & 0x00002000) != 0) &&
cronRetryParameters_ != null &&
cronRetryParameters_ != com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance()) {
getCronRetryParametersBuilder().mergeFrom(value);
} else {
cronRetryParameters_ = value;
}
} else {
cronRetryParametersBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
public Builder clearCronRetryParameters() {
bitField0_ = (bitField0_ & ~0x00002000);
cronRetryParameters_ = null;
if (cronRetryParametersBuilder_ != null) {
cronRetryParametersBuilder_.dispose();
cronRetryParametersBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
public com.google.apphosting.executor.Retry.RetryParameters.Builder getCronRetryParametersBuilder() {
bitField0_ |= 0x00002000;
onChanged();
return getCronRetryParametersFieldBuilder().getBuilder();
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
public com.google.apphosting.executor.Retry.RetryParametersOrBuilder getCronRetryParametersOrBuilder() {
if (cronRetryParametersBuilder_ != null) {
return cronRetryParametersBuilder_.getMessageOrBuilder();
} else {
return cronRetryParameters_ == null ?
com.google.apphosting.executor.Retry.RetryParameters.getDefaultInstance() : cronRetryParameters_;
}
}
/**
*
* Cron jobs are represented as Tasks in a special Cron queue.
* These Cron jobs maintain their own set of retry parameters that
* are used by the cron_task_runner. The paremeters apply to the
* current Cron job run (i.e. last time this task was scheduled and run).
* cron_retry_parameters
* Information about how to retry this cron job on failure.
* cron_retry_count
* The number of times this Cron job has been retried for this job run.
* cron_run_start_usec
* UTC microseconds timestamp at which this job was first attempted for
* the current job run.
*
*
* optional .java.apphosting.RetryParameters cron_retry_parameters = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Retry.RetryParameters, com.google.apphosting.executor.Retry.RetryParameters.Builder, com.google.apphosting.executor.Retry.RetryParametersOrBuilder>
getCronRetryParametersFieldBuilder() {
if (cronRetryParametersBuilder_ == null) {
cronRetryParametersBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Retry.RetryParameters, com.google.apphosting.executor.Retry.RetryParameters.Builder, com.google.apphosting.executor.Retry.RetryParametersOrBuilder>(
getCronRetryParameters(),
getParentForChildren(),
isClean());
cronRetryParameters_ = null;
}
return cronRetryParametersBuilder_;
}
private long cronRetryCount_ ;
/**
* optional int64 cron_retry_count = 14 [default = 0];
* @return Whether the cronRetryCount field is set.
*/
@java.lang.Override
public boolean hasCronRetryCount() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
* optional int64 cron_retry_count = 14 [default = 0];
* @return The cronRetryCount.
*/
@java.lang.Override
public long getCronRetryCount() {
return cronRetryCount_;
}
/**
* optional int64 cron_retry_count = 14 [default = 0];
* @param value The cronRetryCount to set.
* @return This builder for chaining.
*/
public Builder setCronRetryCount(long value) {
cronRetryCount_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
* optional int64 cron_retry_count = 14 [default = 0];
* @return This builder for chaining.
*/
public Builder clearCronRetryCount() {
bitField0_ = (bitField0_ & ~0x00004000);
cronRetryCount_ = 0L;
onChanged();
return this;
}
private long cronRunStartUsec_ ;
/**
* optional int64 cron_run_start_usec = 15;
* @return Whether the cronRunStartUsec field is set.
*/
@java.lang.Override
public boolean hasCronRunStartUsec() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
* optional int64 cron_run_start_usec = 15;
* @return The cronRunStartUsec.
*/
@java.lang.Override
public long getCronRunStartUsec() {
return cronRunStartUsec_;
}
/**
* optional int64 cron_run_start_usec = 15;
* @param value The cronRunStartUsec to set.
* @return This builder for chaining.
*/
public Builder setCronRunStartUsec(long value) {
cronRunStartUsec_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
* optional int64 cron_run_start_usec = 15;
* @return This builder for chaining.
*/
public Builder clearCronRunStartUsec() {
bitField0_ = (bitField0_ & ~0x00008000);
cronRunStartUsec_ = 0L;
onChanged();
return this;
}
private com.google.apphosting.executor.Task.NotifyRoutingRecord notifyRecord_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.NotifyRoutingRecord, com.google.apphosting.executor.Task.NotifyRoutingRecord.Builder, com.google.apphosting.executor.Task.NotifyRoutingRecordOrBuilder> notifyRecordBuilder_;
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
* @return Whether the notifyRecord field is set.
*/
public boolean hasNotifyRecord() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
* @return The notifyRecord.
*/
public com.google.apphosting.executor.Task.NotifyRoutingRecord getNotifyRecord() {
if (notifyRecordBuilder_ == null) {
return notifyRecord_ == null ? com.google.apphosting.executor.Task.NotifyRoutingRecord.getDefaultInstance() : notifyRecord_;
} else {
return notifyRecordBuilder_.getMessage();
}
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
public Builder setNotifyRecord(com.google.apphosting.executor.Task.NotifyRoutingRecord value) {
if (notifyRecordBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
notifyRecord_ = value;
} else {
notifyRecordBuilder_.setMessage(value);
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
public Builder setNotifyRecord(
com.google.apphosting.executor.Task.NotifyRoutingRecord.Builder builderForValue) {
if (notifyRecordBuilder_ == null) {
notifyRecord_ = builderForValue.build();
} else {
notifyRecordBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
public Builder mergeNotifyRecord(com.google.apphosting.executor.Task.NotifyRoutingRecord value) {
if (notifyRecordBuilder_ == null) {
if (((bitField0_ & 0x00010000) != 0) &&
notifyRecord_ != null &&
notifyRecord_ != com.google.apphosting.executor.Task.NotifyRoutingRecord.getDefaultInstance()) {
getNotifyRecordBuilder().mergeFrom(value);
} else {
notifyRecord_ = value;
}
} else {
notifyRecordBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
public Builder clearNotifyRecord() {
bitField0_ = (bitField0_ & ~0x00010000);
notifyRecord_ = null;
if (notifyRecordBuilder_ != null) {
notifyRecordBuilder_.dispose();
notifyRecordBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
public com.google.apphosting.executor.Task.NotifyRoutingRecord.Builder getNotifyRecordBuilder() {
bitField0_ |= 0x00010000;
onChanged();
return getNotifyRecordFieldBuilder().getBuilder();
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
public com.google.apphosting.executor.Task.NotifyRoutingRecordOrBuilder getNotifyRecordOrBuilder() {
if (notifyRecordBuilder_ != null) {
return notifyRecordBuilder_.getMessageOrBuilder();
} else {
return notifyRecord_ == null ?
com.google.apphosting.executor.Task.NotifyRoutingRecord.getDefaultInstance() : notifyRecord_;
}
}
/**
*
* Routing information about a Notify RPC call. When set, scanners can use
* this information and cooperate to avoid duplicate executions. Always set on
* newly-created tasks.
*
*
* optional .java.apphosting.NotifyRoutingRecord notify_record = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.NotifyRoutingRecord, com.google.apphosting.executor.Task.NotifyRoutingRecord.Builder, com.google.apphosting.executor.Task.NotifyRoutingRecordOrBuilder>
getNotifyRecordFieldBuilder() {
if (notifyRecordBuilder_ == null) {
notifyRecordBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Task.NotifyRoutingRecord, com.google.apphosting.executor.Task.NotifyRoutingRecord.Builder, com.google.apphosting.executor.Task.NotifyRoutingRecordOrBuilder>(
getNotifyRecord(),
getParentForChildren(),
isClean());
notifyRecord_ = null;
}
return notifyRecordBuilder_;
}
private int creationSourceType_ = 0;
/**
*
* Indicates what API was used to create this task.
*
*
* optional .java.apphosting.ApiSource.Type creation_source_type = 17;
* @return Whether the creationSourceType field is set.
*/
@java.lang.Override public boolean hasCreationSourceType() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* Indicates what API was used to create this task.
*
*
* optional .java.apphosting.ApiSource.Type creation_source_type = 17;
* @return The creationSourceType.
*/
@java.lang.Override
public com.google.apphosting.executor.Task.ApiSource.Type getCreationSourceType() {
com.google.apphosting.executor.Task.ApiSource.Type result = com.google.apphosting.executor.Task.ApiSource.Type.forNumber(creationSourceType_);
return result == null ? com.google.apphosting.executor.Task.ApiSource.Type.UNKNOWN : result;
}
/**
*
* Indicates what API was used to create this task.
*
*
* optional .java.apphosting.ApiSource.Type creation_source_type = 17;
* @param value The creationSourceType to set.
* @return This builder for chaining.
*/
public Builder setCreationSourceType(com.google.apphosting.executor.Task.ApiSource.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
creationSourceType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Indicates what API was used to create this task.
*
*
* optional .java.apphosting.ApiSource.Type creation_source_type = 17;
* @return This builder for chaining.
*/
public Builder clearCreationSourceType() {
bitField0_ = (bitField0_ & ~0x00020000);
creationSourceType_ = 0;
onChanged();
return this;
}
private com.google.apphosting.executor.Target.TaskAuthorization taskAuthorization_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Target.TaskAuthorization, com.google.apphosting.executor.Target.TaskAuthorization.Builder, com.google.apphosting.executor.Target.TaskAuthorizationOrBuilder> taskAuthorizationBuilder_;
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
* @return Whether the taskAuthorization field is set.
*/
public boolean hasTaskAuthorization() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
* @return The taskAuthorization.
*/
public com.google.apphosting.executor.Target.TaskAuthorization getTaskAuthorization() {
if (taskAuthorizationBuilder_ == null) {
return taskAuthorization_ == null ? com.google.apphosting.executor.Target.TaskAuthorization.getDefaultInstance() : taskAuthorization_;
} else {
return taskAuthorizationBuilder_.getMessage();
}
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
public Builder setTaskAuthorization(com.google.apphosting.executor.Target.TaskAuthorization value) {
if (taskAuthorizationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
taskAuthorization_ = value;
} else {
taskAuthorizationBuilder_.setMessage(value);
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
public Builder setTaskAuthorization(
com.google.apphosting.executor.Target.TaskAuthorization.Builder builderForValue) {
if (taskAuthorizationBuilder_ == null) {
taskAuthorization_ = builderForValue.build();
} else {
taskAuthorizationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
public Builder mergeTaskAuthorization(com.google.apphosting.executor.Target.TaskAuthorization value) {
if (taskAuthorizationBuilder_ == null) {
if (((bitField0_ & 0x00040000) != 0) &&
taskAuthorization_ != null &&
taskAuthorization_ != com.google.apphosting.executor.Target.TaskAuthorization.getDefaultInstance()) {
getTaskAuthorizationBuilder().mergeFrom(value);
} else {
taskAuthorization_ = value;
}
} else {
taskAuthorizationBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
public Builder clearTaskAuthorization() {
bitField0_ = (bitField0_ & ~0x00040000);
taskAuthorization_ = null;
if (taskAuthorizationBuilder_ != null) {
taskAuthorizationBuilder_.dispose();
taskAuthorizationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
public com.google.apphosting.executor.Target.TaskAuthorization.Builder getTaskAuthorizationBuilder() {
bitField0_ |= 0x00040000;
onChanged();
return getTaskAuthorizationFieldBuilder().getBuilder();
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
public com.google.apphosting.executor.Target.TaskAuthorizationOrBuilder getTaskAuthorizationOrBuilder() {
if (taskAuthorizationBuilder_ != null) {
return taskAuthorizationBuilder_.getMessageOrBuilder();
} else {
return taskAuthorization_ == null ?
com.google.apphosting.executor.Target.TaskAuthorization.getDefaultInstance() : taskAuthorization_;
}
}
/**
*
* Includes information for credentials to be used in requests when executing
* a task.
*
*
* optional .java.apphosting.TaskAuthorization task_authorization = 19;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Target.TaskAuthorization, com.google.apphosting.executor.Target.TaskAuthorization.Builder, com.google.apphosting.executor.Target.TaskAuthorizationOrBuilder>
getTaskAuthorizationFieldBuilder() {
if (taskAuthorizationBuilder_ == null) {
taskAuthorizationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.executor.Target.TaskAuthorization, com.google.apphosting.executor.Target.TaskAuthorization.Builder, com.google.apphosting.executor.Target.TaskAuthorizationOrBuilder>(
getTaskAuthorization(),
getParentForChildren(),
isClean());
taskAuthorization_ = null;
}
return taskAuthorizationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskDefinition)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskDefinition)
private static final com.google.apphosting.executor.Task.TaskDefinition DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskDefinition();
}
public static com.google.apphosting.executor.Task.TaskDefinition getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskDefinition parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskDefinition getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TaskGoldenVersionOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.TaskGoldenVersion)
com.google.protobuf.MessageOrBuilder {
/**
*
* See comments for TaskDefinition::store_timestamp and
* StoreTimestampGenerator.
*
*
* required int64 store_timestamp = 1;
* @return Whether the storeTimestamp field is set.
*/
boolean hasStoreTimestamp();
/**
*
* See comments for TaskDefinition::store_timestamp and
* StoreTimestampGenerator.
*
*
* required int64 store_timestamp = 1;
* @return The storeTimestamp.
*/
long getStoreTimestamp();
}
/**
*
* Specifies a golden version of a task. Will not always be present in Bigtable,
* but when it is it will be used to determine which version of the task to
* execute. When not present, we will instead use the task with the latest
* Bigtable cell timestamp, which will also be the first one written to
* Bigtable.
* A golden version exists when same task is unexpectedly added multiple times
* within a very short period of time (e.g. because the first bt write
* succeeded, but not before the deadline so the task was remotely retried).
* Retries and leases do not create a new task version -- the postponed task
* uses the same store_timestamp that the task had previously. For more
* information, see StoreTimestampGenerator.
*
*
* Protobuf type {@code java.apphosting.TaskGoldenVersion}
*/
public static final class TaskGoldenVersion extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.TaskGoldenVersion)
TaskGoldenVersionOrBuilder {
private static final long serialVersionUID = 0L;
// Use TaskGoldenVersion.newBuilder() to construct.
private TaskGoldenVersion(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TaskGoldenVersion() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TaskGoldenVersion();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskGoldenVersion_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskGoldenVersion_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskGoldenVersion.class, com.google.apphosting.executor.Task.TaskGoldenVersion.Builder.class);
}
private int bitField0_;
public static final int STORE_TIMESTAMP_FIELD_NUMBER = 1;
private long storeTimestamp_ = 0L;
/**
*
* See comments for TaskDefinition::store_timestamp and
* StoreTimestampGenerator.
*
*
* required int64 store_timestamp = 1;
* @return Whether the storeTimestamp field is set.
*/
@java.lang.Override
public boolean hasStoreTimestamp() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* See comments for TaskDefinition::store_timestamp and
* StoreTimestampGenerator.
*
*
* required int64 store_timestamp = 1;
* @return The storeTimestamp.
*/
@java.lang.Override
public long getStoreTimestamp() {
return storeTimestamp_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasStoreTimestamp()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, storeTimestamp_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, storeTimestamp_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.TaskGoldenVersion)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.TaskGoldenVersion other = (com.google.apphosting.executor.Task.TaskGoldenVersion) obj;
if (hasStoreTimestamp() != other.hasStoreTimestamp()) return false;
if (hasStoreTimestamp()) {
if (getStoreTimestamp()
!= other.getStoreTimestamp()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStoreTimestamp()) {
hash = (37 * hash) + STORE_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStoreTimestamp());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.TaskGoldenVersion prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a golden version of a task. Will not always be present in Bigtable,
* but when it is it will be used to determine which version of the task to
* execute. When not present, we will instead use the task with the latest
* Bigtable cell timestamp, which will also be the first one written to
* Bigtable.
* A golden version exists when same task is unexpectedly added multiple times
* within a very short period of time (e.g. because the first bt write
* succeeded, but not before the deadline so the task was remotely retried).
* Retries and leases do not create a new task version -- the postponed task
* uses the same store_timestamp that the task had previously. For more
* information, see StoreTimestampGenerator.
*
*
* Protobuf type {@code java.apphosting.TaskGoldenVersion}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.TaskGoldenVersion)
com.google.apphosting.executor.Task.TaskGoldenVersionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskGoldenVersion_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskGoldenVersion_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.TaskGoldenVersion.class, com.google.apphosting.executor.Task.TaskGoldenVersion.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.TaskGoldenVersion.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
storeTimestamp_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_TaskGoldenVersion_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskGoldenVersion getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.TaskGoldenVersion.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskGoldenVersion build() {
com.google.apphosting.executor.Task.TaskGoldenVersion result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskGoldenVersion buildPartial() {
com.google.apphosting.executor.Task.TaskGoldenVersion result = new com.google.apphosting.executor.Task.TaskGoldenVersion(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.TaskGoldenVersion result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.storeTimestamp_ = storeTimestamp_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.TaskGoldenVersion) {
return mergeFrom((com.google.apphosting.executor.Task.TaskGoldenVersion)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.TaskGoldenVersion other) {
if (other == com.google.apphosting.executor.Task.TaskGoldenVersion.getDefaultInstance()) return this;
if (other.hasStoreTimestamp()) {
setStoreTimestamp(other.getStoreTimestamp());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasStoreTimestamp()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
storeTimestamp_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long storeTimestamp_ ;
/**
*
* See comments for TaskDefinition::store_timestamp and
* StoreTimestampGenerator.
*
*
* required int64 store_timestamp = 1;
* @return Whether the storeTimestamp field is set.
*/
@java.lang.Override
public boolean hasStoreTimestamp() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* See comments for TaskDefinition::store_timestamp and
* StoreTimestampGenerator.
*
*
* required int64 store_timestamp = 1;
* @return The storeTimestamp.
*/
@java.lang.Override
public long getStoreTimestamp() {
return storeTimestamp_;
}
/**
*
* See comments for TaskDefinition::store_timestamp and
* StoreTimestampGenerator.
*
*
* required int64 store_timestamp = 1;
* @param value The storeTimestamp to set.
* @return This builder for chaining.
*/
public Builder setStoreTimestamp(long value) {
storeTimestamp_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* See comments for TaskDefinition::store_timestamp and
* StoreTimestampGenerator.
*
*
* required int64 store_timestamp = 1;
* @return This builder for chaining.
*/
public Builder clearStoreTimestamp() {
bitField0_ = (bitField0_ & ~0x00000001);
storeTimestamp_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.TaskGoldenVersion)
}
// @@protoc_insertion_point(class_scope:java.apphosting.TaskGoldenVersion)
private static final com.google.apphosting.executor.Task.TaskGoldenVersion DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.TaskGoldenVersion();
}
public static com.google.apphosting.executor.Task.TaskGoldenVersion getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TaskGoldenVersion parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.TaskGoldenVersion getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NotifyRoutingRecordOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.NotifyRoutingRecord)
com.google.protobuf.MessageOrBuilder {
/**
*
* Whether a Notify RPC call was sent. When false, explicitly indicates that
* no Notify message was sent - this rules out some duplicate execution corner
* cases, and might allow scanners to process the task faster.
*
*
* optional bool sent = 1 [default = true];
* @return Whether the sent field is set.
*/
boolean hasSent();
/**
*
* Whether a Notify RPC call was sent. When false, explicitly indicates that
* no Notify message was sent - this rules out some duplicate execution corner
* cases, and might allow scanners to process the task faster.
*
*
* optional bool sent = 1 [default = true];
* @return The sent.
*/
boolean getSent();
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return Whether the scannerBns field is set.
*/
boolean hasScannerBns();
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return The scannerBns.
*/
java.lang.String getScannerBns();
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return The bytes for scannerBns.
*/
com.google.protobuf.ByteString
getScannerBnsBytes();
/**
*
* The |timestamp_usec| field of the queue map that the sender used for
* routing. Must be set when sent=true.
*
*
* optional int64 queue_map_timestamp_usec = 3;
* @return Whether the queueMapTimestampUsec field is set.
*/
boolean hasQueueMapTimestampUsec();
/**
*
* The |timestamp_usec| field of the queue map that the sender used for
* routing. Must be set when sent=true.
*
*
* optional int64 queue_map_timestamp_usec = 3;
* @return The queueMapTimestampUsec.
*/
long getQueueMapTimestampUsec();
}
/**
*
* Stores routing information about a Notify RPC call.
*
*
* Protobuf type {@code java.apphosting.NotifyRoutingRecord}
*/
public static final class NotifyRoutingRecord extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.NotifyRoutingRecord)
NotifyRoutingRecordOrBuilder {
private static final long serialVersionUID = 0L;
// Use NotifyRoutingRecord.newBuilder() to construct.
private NotifyRoutingRecord(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NotifyRoutingRecord() {
sent_ = true;
scannerBns_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new NotifyRoutingRecord();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_NotifyRoutingRecord_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_NotifyRoutingRecord_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.NotifyRoutingRecord.class, com.google.apphosting.executor.Task.NotifyRoutingRecord.Builder.class);
}
private int bitField0_;
public static final int SENT_FIELD_NUMBER = 1;
private boolean sent_ = true;
/**
*
* Whether a Notify RPC call was sent. When false, explicitly indicates that
* no Notify message was sent - this rules out some duplicate execution corner
* cases, and might allow scanners to process the task faster.
*
*
* optional bool sent = 1 [default = true];
* @return Whether the sent field is set.
*/
@java.lang.Override
public boolean hasSent() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Whether a Notify RPC call was sent. When false, explicitly indicates that
* no Notify message was sent - this rules out some duplicate execution corner
* cases, and might allow scanners to process the task faster.
*
*
* optional bool sent = 1 [default = true];
* @return The sent.
*/
@java.lang.Override
public boolean getSent() {
return sent_;
}
public static final int SCANNER_BNS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object scannerBns_ = "";
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return Whether the scannerBns field is set.
*/
@java.lang.Override
public boolean hasScannerBns() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return The scannerBns.
*/
@java.lang.Override
public java.lang.String getScannerBns() {
java.lang.Object ref = scannerBns_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
scannerBns_ = s;
}
return s;
}
}
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return The bytes for scannerBns.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getScannerBnsBytes() {
java.lang.Object ref = scannerBns_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
scannerBns_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int QUEUE_MAP_TIMESTAMP_USEC_FIELD_NUMBER = 3;
private long queueMapTimestampUsec_ = 0L;
/**
*
* The |timestamp_usec| field of the queue map that the sender used for
* routing. Must be set when sent=true.
*
*
* optional int64 queue_map_timestamp_usec = 3;
* @return Whether the queueMapTimestampUsec field is set.
*/
@java.lang.Override
public boolean hasQueueMapTimestampUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The |timestamp_usec| field of the queue map that the sender used for
* routing. Must be set when sent=true.
*
*
* optional int64 queue_map_timestamp_usec = 3;
* @return The queueMapTimestampUsec.
*/
@java.lang.Override
public long getQueueMapTimestampUsec() {
return queueMapTimestampUsec_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBool(1, sent_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, scannerBns_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(3, queueMapTimestampUsec_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, sent_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, scannerBns_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, queueMapTimestampUsec_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.executor.Task.NotifyRoutingRecord)) {
return super.equals(obj);
}
com.google.apphosting.executor.Task.NotifyRoutingRecord other = (com.google.apphosting.executor.Task.NotifyRoutingRecord) obj;
if (hasSent() != other.hasSent()) return false;
if (hasSent()) {
if (getSent()
!= other.getSent()) return false;
}
if (hasScannerBns() != other.hasScannerBns()) return false;
if (hasScannerBns()) {
if (!getScannerBns()
.equals(other.getScannerBns())) return false;
}
if (hasQueueMapTimestampUsec() != other.hasQueueMapTimestampUsec()) return false;
if (hasQueueMapTimestampUsec()) {
if (getQueueMapTimestampUsec()
!= other.getQueueMapTimestampUsec()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSent()) {
hash = (37 * hash) + SENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSent());
}
if (hasScannerBns()) {
hash = (37 * hash) + SCANNER_BNS_FIELD_NUMBER;
hash = (53 * hash) + getScannerBns().hashCode();
}
if (hasQueueMapTimestampUsec()) {
hash = (37 * hash) + QUEUE_MAP_TIMESTAMP_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getQueueMapTimestampUsec());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.executor.Task.NotifyRoutingRecord prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Stores routing information about a Notify RPC call.
*
*
* Protobuf type {@code java.apphosting.NotifyRoutingRecord}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.NotifyRoutingRecord)
com.google.apphosting.executor.Task.NotifyRoutingRecordOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_NotifyRoutingRecord_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_NotifyRoutingRecord_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.executor.Task.NotifyRoutingRecord.class, com.google.apphosting.executor.Task.NotifyRoutingRecord.Builder.class);
}
// Construct using com.google.apphosting.executor.Task.NotifyRoutingRecord.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
sent_ = true;
scannerBns_ = "";
queueMapTimestampUsec_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.executor.Task.internal_static_java_apphosting_NotifyRoutingRecord_descriptor;
}
@java.lang.Override
public com.google.apphosting.executor.Task.NotifyRoutingRecord getDefaultInstanceForType() {
return com.google.apphosting.executor.Task.NotifyRoutingRecord.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.executor.Task.NotifyRoutingRecord build() {
com.google.apphosting.executor.Task.NotifyRoutingRecord result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.executor.Task.NotifyRoutingRecord buildPartial() {
com.google.apphosting.executor.Task.NotifyRoutingRecord result = new com.google.apphosting.executor.Task.NotifyRoutingRecord(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.executor.Task.NotifyRoutingRecord result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.sent_ = sent_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.scannerBns_ = scannerBns_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.queueMapTimestampUsec_ = queueMapTimestampUsec_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.executor.Task.NotifyRoutingRecord) {
return mergeFrom((com.google.apphosting.executor.Task.NotifyRoutingRecord)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.executor.Task.NotifyRoutingRecord other) {
if (other == com.google.apphosting.executor.Task.NotifyRoutingRecord.getDefaultInstance()) return this;
if (other.hasSent()) {
setSent(other.getSent());
}
if (other.hasScannerBns()) {
scannerBns_ = other.scannerBns_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasQueueMapTimestampUsec()) {
setQueueMapTimestampUsec(other.getQueueMapTimestampUsec());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
sent_ = input.readBool();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
scannerBns_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
queueMapTimestampUsec_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private boolean sent_ = true;
/**
*
* Whether a Notify RPC call was sent. When false, explicitly indicates that
* no Notify message was sent - this rules out some duplicate execution corner
* cases, and might allow scanners to process the task faster.
*
*
* optional bool sent = 1 [default = true];
* @return Whether the sent field is set.
*/
@java.lang.Override
public boolean hasSent() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Whether a Notify RPC call was sent. When false, explicitly indicates that
* no Notify message was sent - this rules out some duplicate execution corner
* cases, and might allow scanners to process the task faster.
*
*
* optional bool sent = 1 [default = true];
* @return The sent.
*/
@java.lang.Override
public boolean getSent() {
return sent_;
}
/**
*
* Whether a Notify RPC call was sent. When false, explicitly indicates that
* no Notify message was sent - this rules out some duplicate execution corner
* cases, and might allow scanners to process the task faster.
*
*
* optional bool sent = 1 [default = true];
* @param value The sent to set.
* @return This builder for chaining.
*/
public Builder setSent(boolean value) {
sent_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Whether a Notify RPC call was sent. When false, explicitly indicates that
* no Notify message was sent - this rules out some duplicate execution corner
* cases, and might allow scanners to process the task faster.
*
*
* optional bool sent = 1 [default = true];
* @return This builder for chaining.
*/
public Builder clearSent() {
bitField0_ = (bitField0_ & ~0x00000001);
sent_ = true;
onChanged();
return this;
}
private java.lang.Object scannerBns_ = "";
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return Whether the scannerBns field is set.
*/
public boolean hasScannerBns() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return The scannerBns.
*/
public java.lang.String getScannerBns() {
java.lang.Object ref = scannerBns_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
scannerBns_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return The bytes for scannerBns.
*/
public com.google.protobuf.ByteString
getScannerBnsBytes() {
java.lang.Object ref = scannerBns_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
scannerBns_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @param value The scannerBns to set.
* @return This builder for chaining.
*/
public Builder setScannerBns(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
scannerBns_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @return This builder for chaining.
*/
public Builder clearScannerBns() {
scannerBns_ = getDefaultInstance().getScannerBns();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* BNS address of the scanner that the Notify RPC call was sent to. Must be
* set when sent=true.
*
*
* optional string scanner_bns = 2;
* @param value The bytes for scannerBns to set.
* @return This builder for chaining.
*/
public Builder setScannerBnsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
scannerBns_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private long queueMapTimestampUsec_ ;
/**
*
* The |timestamp_usec| field of the queue map that the sender used for
* routing. Must be set when sent=true.
*
*
* optional int64 queue_map_timestamp_usec = 3;
* @return Whether the queueMapTimestampUsec field is set.
*/
@java.lang.Override
public boolean hasQueueMapTimestampUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The |timestamp_usec| field of the queue map that the sender used for
* routing. Must be set when sent=true.
*
*
* optional int64 queue_map_timestamp_usec = 3;
* @return The queueMapTimestampUsec.
*/
@java.lang.Override
public long getQueueMapTimestampUsec() {
return queueMapTimestampUsec_;
}
/**
*
* The |timestamp_usec| field of the queue map that the sender used for
* routing. Must be set when sent=true.
*
*
* optional int64 queue_map_timestamp_usec = 3;
* @param value The queueMapTimestampUsec to set.
* @return This builder for chaining.
*/
public Builder setQueueMapTimestampUsec(long value) {
queueMapTimestampUsec_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The |timestamp_usec| field of the queue map that the sender used for
* routing. Must be set when sent=true.
*
*
* optional int64 queue_map_timestamp_usec = 3;
* @return This builder for chaining.
*/
public Builder clearQueueMapTimestampUsec() {
bitField0_ = (bitField0_ & ~0x00000004);
queueMapTimestampUsec_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.NotifyRoutingRecord)
}
// @@protoc_insertion_point(class_scope:java.apphosting.NotifyRoutingRecord)
private static final com.google.apphosting.executor.Task.NotifyRoutingRecord DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.executor.Task.NotifyRoutingRecord();
}
public static com.google.apphosting.executor.Task.NotifyRoutingRecord getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public NotifyRoutingRecord parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.executor.Task.NotifyRoutingRecord getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskRef_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskRef_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskIndexRef_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskIndexRef_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskRange_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskRange_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskEtaBorder_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskEtaBorder_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskTagBorder_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskTagBorder_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskRangeByEta_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskRangeByEta_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskRangeByName_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskRangeByName_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskRangeByTag_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskRangeByTag_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskRunnerInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskRunnerInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_HttpTaskRunnerInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_HttpTaskRunnerInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_PubsubTaskRunnerInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_PubsubTaskRunnerInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskRunnerStats_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskRunnerStats_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskRunnerPayload_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskRunnerPayload_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_HttpTaskRunnerPayload_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_HttpTaskRunnerPayload_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_HttpTaskRunnerPayload_Header_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_HttpTaskRunnerPayload_Header_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_AppEngineRouting_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_AppEngineRouting_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_CronTaskRunnerPayload_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_CronTaskRunnerPayload_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_StubbyTaskRunnerPayload_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_StubbyTaskRunnerPayload_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_StubbyTaskRunnerInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_StubbyTaskRunnerInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_PullTaskPayload_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_PullTaskPayload_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_PubsubTaskRunnerPayload_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_PubsubTaskRunnerPayload_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_FunctionTaskRunnerPayload_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_FunctionTaskRunnerPayload_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_ApiSource_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_ApiSource_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskDefinition_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskDefinition_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_TaskGoldenVersion_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_TaskGoldenVersion_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_NotifyRoutingRecord_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_NotifyRoutingRecord_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\ntask.proto\022\017java.apphosting\032\013queue.pro" +
"to\032\013retry.proto\032\014target.proto\"E\n\007TaskRef" +
"\022,\n\tqueue_ref\030\001 \002(\0132\031.java.apphosting.Qu" +
"eueRef\022\014\n\004name\030\002 \002(\014\"\200\001\n\014TaskIndexRef\022\025\n" +
"\tshard_num\030\001 \001(\005:\002-1\022,\n\tqueue_ref\030\002 \002(\0132" +
"\031.java.apphosting.QueueRef\022\020\n\010eta_usec\030\003" +
" \002(\003\022\014\n\004name\030\004 \002(\014\022\013\n\003tag\030\005 \001(\014\"\017\n\tTaskR" +
"ange:\002\010\001\"/\n\rTaskEtaBorder\022\020\n\010eta_usec\030\001 " +
"\002(\003\022\014\n\004name\030\002 \001(\014\"<\n\rTaskTagBorder\022\013\n\003ta" +
"g\030\001 \002(\014\022\020\n\010eta_usec\030\002 \001(\003\022\014\n\004name\030\003 \001(\014\"" +
"n\n\016TaskRangeByEta\022-\n\005start\030\001 \001(\0132\036.java." +
"apphosting.TaskEtaBorder\022-\n\005limit\030\002 \001(\0132" +
"\036.java.apphosting.TaskEtaBorder\"/\n\017TaskR" +
"angeByName\022\r\n\005start\030\001 \001(\014\022\r\n\005limit\030\002 \001(\014" +
"\"n\n\016TaskRangeByTag\022-\n\005start\030\001 \001(\0132\036.java" +
".apphosting.TaskTagBorder\022-\n\005limit\030\002 \001(\013" +
"2\036.java.apphosting.TaskTagBorder\"\024\n\016Task" +
"RunnerInfo:\002\010\001\"A\n\022HttpTaskRunnerInfo\022\025\n\r" +
"response_code\030\001 \002(\003\022\024\n\014retry_reason\030\002 \001(" +
"\t\"&\n\024PubsubTaskRunnerInfo\022\016\n\006status\030\001 \001(" +
"\005\"H\n\032ExternalHttpTaskRunnerInfo\022\016\n\006statu" +
"s\030\001 \001(\005\022\032\n\022http_response_code\030\002 \001(\005\"\201\001\n\017" +
"TaskRunnerStats\022\027\n\017dispatched_usec\030\001 \002(\003" +
"\022\020\n\010lag_usec\030\002 \002(\003\022\024\n\014elapsed_usec\030\003 \002(\003" +
"\022-\n\004info\030\004 \001(\0132\037.java.apphosting.TaskRun" +
"nerInfo\"\027\n\021TaskRunnerPayload:\002\010\001\"\237\003\n\025Htt" +
"pTaskRunnerPayload\022J\n\006method\030\001 \001(\01624.jav" +
"a.apphosting.HttpTaskRunnerPayload.Reque" +
"stMethod:\004POST\022\013\n\003url\030\002 \002(\014\022=\n\006header\030\003 " +
"\003(\n2-.java.apphosting.HttpTaskRunnerPayl" +
"oad.Header\022\020\n\004body\030\006 \001(\014B\002\010\001\022\034\n\024dispatch" +
"_deadline_ms\030\007 \001(\005\022=\n\022app_engine_routing" +
"\030\010 \001(\0132!.java.apphosting.AppEngineRoutin" +
"g\032$\n\006Header\022\013\n\003key\030\004 \002(\014\022\r\n\005value\030\005 \002(\014\"" +
"Y\n\rRequestMethod\022\007\n\003GET\020\001\022\010\n\004POST\020\002\022\010\n\004H" +
"EAD\020\003\022\007\n\003PUT\020\004\022\n\n\006DELETE\020\005\022\t\n\005PATCH\020\006\022\013\n" +
"\007OPTIONS\020\007\"T\n\020AppEngineRouting\022\017\n\007servic" +
"e\030\001 \001(\t\022\017\n\007version\030\002 \001(\t\022\020\n\010instance\030\003 \001" +
"(\t\022\014\n\004host\030\004 \001(\t\"\317\002\n\025CronTaskRunnerPaylo" +
"ad\022\020\n\010schedule\030\001 \002(\t\022\020\n\010timezone\030\002 \002(\t\022]" +
"\n\027time_specification_type\030\005 \001(\0162<.java.a" +
"pphosting.CronTaskRunnerPayload.TimeSpec" +
"ificationType\022=\n\021delegated_payload\030\003 \002(\013" +
"2\".java.apphosting.TaskRunnerPayload\022\033\n\023" +
"skip_num_iterations\030\004 \001(\003\"W\n\025TimeSpecifi" +
"cationType\022\'\n#TIME_SPECIFICATION_TYPE_UN" +
"SPECIFIED\020\000\022\010\n\004GROC\020\001\022\013\n\007CRONTAB\020\002\"\304\n\n\027S" +
"tubbyTaskRunnerPayload\022G\n\007channel\030\001 \002(\0132" +
"6.java.apphosting.StubbyTaskRunnerPayloa" +
"d.StubbyChannel\022\016\n\006method\030\002 \002(\t\022\023\n\007reque" +
"st\030\003 \002(\014B\002\010\001\022H\n\013rpc_options\030\004 \001(\01323.java" +
".apphosting.StubbyTaskRunnerPayload.RpcO" +
"ptions\022&\n\027request_task_definition\030\005 \001(\010:" +
"\005false\032\215\005\n\rStubbyChannel\022\014\n\004host\030\001 \002(\t\022w" +
"\n\025load_balancing_policy\030\002 \001(\0162J.java.app" +
"hosting.StubbyTaskRunnerPayload.StubbyCh" +
"annel.LoadBalancingPolicy:\014LEAST_LOADED\022" +
"\037\n\021security_protocol\030\003 \001(\t:\004loas\022\032\n\022min_" +
"security_level\030\004 \001(\005\022x\n\027target_selection" +
"_policy\030\005 \001(\0162L.java.apphosting.StubbyTa" +
"skRunnerPayload.StubbyChannel.TargetSele" +
"ctionPolicy:\tNO_FILTER\022\026\n\013max_targets\030\006 " +
"\001(\005:\0015\022\034\n\024max_outstanding_rpcs\030\007 \001(\005\"\271\001\n" +
"\023LoadBalancingPolicy\022\020\n\014LEAST_LOADED\020\001\022\017" +
"\n\013ROUND_ROBIN\020\002\022\023\n\017FIRST_REACHABLE\020\003\022\027\n\023" +
"AFFINITY_SCHEDULING\020\004\022\021\n\rERROR_ADVERSE\020\005" +
"\022\026\n\022FIRST_LEAST_LOADED\020\006\022\017\n\013INVERSE_RTT\020" +
"\007\022\025\n\021LATENCY_DRIVEN_RR\020\010\"L\n\025TargetSelect" +
"ionPolicy\022\r\n\tNO_FILTER\020\001\022\021\n\rRANDOM_SUBSE" +
"T\020\002\022\021\n\rNETWORK_AWARE\020\003\032\270\003\n\nRpcOptions\022\020\n" +
"\010deadline\030\001 \001(\001\022\021\n\tfail_fast\030\002 \001(\010\022\035\n\025du" +
"plicate_suppression\030\003 \001(\010\022\027\n\017scheduling_" +
"hash\030\004 \001(\004\022Q\n\010protocol\030\005 \001(\0162?.java.apph" +
"osting.StubbyTaskRunnerPayload.RpcOption" +
"s.RPCProtocol\022S\n\017response_format\030\006 \001(\0162:" +
".java.apphosting.StubbyTaskRunnerPayload" +
".RpcOptions.Format\022R\n\016request_format\030\007 \001" +
"(\0162:.java.apphosting.StubbyTaskRunnerPay" +
"load.RpcOptions.Format\"\037\n\013RPCProtocol\022\007\n" +
"\003TCP\020\000\022\007\n\003UDP\020\001\"0\n\006Format\022\020\n\014UNCOMPRESSE" +
"D\020\000\022\024\n\020ZIPPY_COMPRESSED\020\001\"X\n\024StubbyTaskR" +
"unnerInfo\022\016\n\006status\030\001 \002(\003\022\031\n\021application" +
"_error\030\002 \001(\003\022\025\n\rerror_message\030\003 \001(\014\"&\n\017P" +
"ullTaskPayload\022\023\n\007content\030\001 \002(\014B\002\010\001\"R\n\027P" +
"ubsubTaskRunnerPayload\022\032\n\016pubsub_message" +
"\030\001 \001(\014B\002\010\001\022\033\n\023internal_topic_name\030\002 \001(\t\"" +
"\332\002\n\031FunctionTaskRunnerPayload\022N\n\006method\030" +
"\001 \001(\01628.java.apphosting.FunctionTaskRunn" +
"erPayload.RequestMethod:\004POST\022\013\n\003url\030\002 \001" +
"(\014\022A\n\006header\030\003 \003(\n21.java.apphosting.Fun" +
"ctionTaskRunnerPayload.Header\022\020\n\004body\030\006 " +
"\001(\014B\002\010\001\022\025\n\rfunction_name\030\007 \001(\t\032$\n\006Header" +
"\022\013\n\003key\030\004 \002(\014\022\r\n\005value\030\005 \002(\014\"N\n\rRequestM" +
"ethod\022\013\n\007UNKNOWN\020\000\022\007\n\003GET\020\001\022\010\n\004POST\020\002\022\010\n" +
"\004HEAD\020\003\022\007\n\003PUT\020\004\022\n\n\006DELETE\020\005\"\240\004\n\035Externa" +
"lHttpTaskRunnerPayload\022h\n\006method\030\001 \001(\0162<" +
".java.apphosting.ExternalHttpTaskRunnerP" +
"ayload.RequestMethod:\032REQUEST_METHOD_UNS" +
"PECIFIED\022\013\n\003url\030\002 \001(\014\022E\n\006header\030\003 \003(\01325." +
"java.apphosting.ExternalHttpTaskRunnerPa" +
"yload.Header\022\020\n\004body\030\004 \001(\014B\002\010\001\022\024\n\014reques" +
"tor_id\030\005 \001(\t\022\033\n\023request_deadline_ms\030\006 \001(" +
"\005\022\030\n\020fetch_timeout_ms\030\007 \001(\005\022\032\n\022user_agen" +
"t_to_send\030\010 \001(\t\022%\n\035fail_on_ssl_certifica" +
"te_error\030\t \001(\010\032$\n\006Header\022\013\n\003key\030\001 \001(\014\022\r\n" +
"\005value\030\002 \001(\014\"y\n\rRequestMethod\022\036\n\032REQUEST" +
"_METHOD_UNSPECIFIED\020\000\022\007\n\003GET\020\001\022\010\n\004POST\020\002" +
"\022\010\n\004HEAD\020\003\022\007\n\003PUT\020\004\022\n\n\006DELETE\020\005\022\t\n\005PATCH" +
"\020\006\022\013\n\007OPTIONS\020\007\"\207\001\n\tApiSource\"z\n\004Type\022\013\n" +
"\007UNKNOWN\020\000\022\026\n\022APP_ENGINE_RUNTIME\020\001\022\025\n\021TA" +
"SK_QUEUE_BRIDGE\020\002\022\030\n\024CLOUD_TASKS_FRONTEN" +
"D\020\003\022\034\n\030CLOUD_SCHEDULER_FRONTEND\020\004\"\365\005\n\016Ta" +
"skDefinition\022*\n\010task_ref\030\001 \002(\0132\030.java.ap" +
"phosting.TaskRef\022\020\n\010eta_usec\030\002 \002(\003\022\031\n\021pr" +
"evious_eta_usec\030\022 \001(\003\0223\n\007payload\030\003 \002(\0132\"" +
".java.apphosting.TaskRunnerPayload\022\026\n\013re" +
"try_count\030\004 \001(\003:\0010\022\027\n\017store_timestamp\030\005 " +
"\001(\003\022?\n\025last_invocation_stats\030\006 \001(\0132 .jav" +
"a.apphosting.TaskRunnerStats\022\023\n\013descript" +
"ion\030\007 \001(\014\022:\n\020retry_parameters\030\010 \001(\0132 .ja" +
"va.apphosting.RetryParameters\022\026\n\016first_t" +
"ry_usec\030\t \001(\003\022\013\n\003tag\030\n \001(\014\022\027\n\017execution_" +
"count\030\013 \001(\003\022\035\n\022index_shard_number\030\014 \001(\005:" +
"\0010\022?\n\025cron_retry_parameters\030\r \001(\0132 .java" +
".apphosting.RetryParameters\022\033\n\020cron_retr" +
"y_count\030\016 \001(\003:\0010\022\033\n\023cron_run_start_usec\030" +
"\017 \001(\003\022;\n\rnotify_record\030\020 \001(\0132$.java.apph" +
"osting.NotifyRoutingRecord\022=\n\024creation_s" +
"ource_type\030\021 \001(\0162\037.java.apphosting.ApiSo" +
"urce.Type\022>\n\022task_authorization\030\023 \001(\0132\"." +
"java.apphosting.TaskAuthorization\",\n\021Tas" +
"kGoldenVersion\022\027\n\017store_timestamp\030\001 \002(\003\"" +
"`\n\023NotifyRoutingRecord\022\022\n\004sent\030\001 \001(\010:\004tr" +
"ue\022\023\n\013scanner_bns\030\002 \001(\t\022 \n\030queue_map_tim" +
"estamp_usec\030\003 \001(\003B \n\036com.google.apphosti" +
"ng.executor"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.google.apphosting.executor.Queue.getDescriptor(),
com.google.apphosting.executor.Retry.getDescriptor(),
com.google.apphosting.executor.Target.getDescriptor(),
});
internal_static_java_apphosting_TaskRef_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_java_apphosting_TaskRef_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskRef_descriptor,
new java.lang.String[] { "QueueRef", "Name", });
internal_static_java_apphosting_TaskIndexRef_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_java_apphosting_TaskIndexRef_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskIndexRef_descriptor,
new java.lang.String[] { "ShardNum", "QueueRef", "EtaUsec", "Name", "Tag", });
internal_static_java_apphosting_TaskRange_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_java_apphosting_TaskRange_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskRange_descriptor,
new java.lang.String[] { });
internal_static_java_apphosting_TaskEtaBorder_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_java_apphosting_TaskEtaBorder_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskEtaBorder_descriptor,
new java.lang.String[] { "EtaUsec", "Name", });
internal_static_java_apphosting_TaskTagBorder_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_java_apphosting_TaskTagBorder_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskTagBorder_descriptor,
new java.lang.String[] { "Tag", "EtaUsec", "Name", });
internal_static_java_apphosting_TaskRangeByEta_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_java_apphosting_TaskRangeByEta_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskRangeByEta_descriptor,
new java.lang.String[] { "Start", "Limit", });
internal_static_java_apphosting_TaskRangeByName_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_java_apphosting_TaskRangeByName_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskRangeByName_descriptor,
new java.lang.String[] { "Start", "Limit", });
internal_static_java_apphosting_TaskRangeByTag_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_java_apphosting_TaskRangeByTag_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskRangeByTag_descriptor,
new java.lang.String[] { "Start", "Limit", });
internal_static_java_apphosting_TaskRunnerInfo_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_java_apphosting_TaskRunnerInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskRunnerInfo_descriptor,
new java.lang.String[] { });
internal_static_java_apphosting_HttpTaskRunnerInfo_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_java_apphosting_HttpTaskRunnerInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_HttpTaskRunnerInfo_descriptor,
new java.lang.String[] { "ResponseCode", "RetryReason", });
internal_static_java_apphosting_PubsubTaskRunnerInfo_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_java_apphosting_PubsubTaskRunnerInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_PubsubTaskRunnerInfo_descriptor,
new java.lang.String[] { "Status", });
internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_ExternalHttpTaskRunnerInfo_descriptor,
new java.lang.String[] { "Status", "HttpResponseCode", });
internal_static_java_apphosting_TaskRunnerStats_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_java_apphosting_TaskRunnerStats_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskRunnerStats_descriptor,
new java.lang.String[] { "DispatchedUsec", "LagUsec", "ElapsedUsec", "Info", });
internal_static_java_apphosting_TaskRunnerPayload_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_java_apphosting_TaskRunnerPayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskRunnerPayload_descriptor,
new java.lang.String[] { });
internal_static_java_apphosting_HttpTaskRunnerPayload_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_java_apphosting_HttpTaskRunnerPayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_HttpTaskRunnerPayload_descriptor,
new java.lang.String[] { "Method", "Url", "Header", "Body", "DispatchDeadlineMs", "AppEngineRouting", });
internal_static_java_apphosting_HttpTaskRunnerPayload_Header_descriptor =
internal_static_java_apphosting_HttpTaskRunnerPayload_descriptor.getNestedTypes().get(0);
internal_static_java_apphosting_HttpTaskRunnerPayload_Header_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_HttpTaskRunnerPayload_Header_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_java_apphosting_AppEngineRouting_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_java_apphosting_AppEngineRouting_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_AppEngineRouting_descriptor,
new java.lang.String[] { "Service", "Version", "Instance", "Host", });
internal_static_java_apphosting_CronTaskRunnerPayload_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_java_apphosting_CronTaskRunnerPayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_CronTaskRunnerPayload_descriptor,
new java.lang.String[] { "Schedule", "Timezone", "TimeSpecificationType", "DelegatedPayload", "SkipNumIterations", });
internal_static_java_apphosting_StubbyTaskRunnerPayload_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_java_apphosting_StubbyTaskRunnerPayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_StubbyTaskRunnerPayload_descriptor,
new java.lang.String[] { "Channel", "Method", "Request", "RpcOptions", "RequestTaskDefinition", });
internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_descriptor =
internal_static_java_apphosting_StubbyTaskRunnerPayload_descriptor.getNestedTypes().get(0);
internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_StubbyTaskRunnerPayload_StubbyChannel_descriptor,
new java.lang.String[] { "Host", "LoadBalancingPolicy", "SecurityProtocol", "MinSecurityLevel", "TargetSelectionPolicy", "MaxTargets", "MaxOutstandingRpcs", });
internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_descriptor =
internal_static_java_apphosting_StubbyTaskRunnerPayload_descriptor.getNestedTypes().get(1);
internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_StubbyTaskRunnerPayload_RpcOptions_descriptor,
new java.lang.String[] { "Deadline", "FailFast", "DuplicateSuppression", "SchedulingHash", "Protocol", "ResponseFormat", "RequestFormat", });
internal_static_java_apphosting_StubbyTaskRunnerInfo_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_java_apphosting_StubbyTaskRunnerInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_StubbyTaskRunnerInfo_descriptor,
new java.lang.String[] { "Status", "ApplicationError", "ErrorMessage", });
internal_static_java_apphosting_PullTaskPayload_descriptor =
getDescriptor().getMessageTypes().get(19);
internal_static_java_apphosting_PullTaskPayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_PullTaskPayload_descriptor,
new java.lang.String[] { "Content", });
internal_static_java_apphosting_PubsubTaskRunnerPayload_descriptor =
getDescriptor().getMessageTypes().get(20);
internal_static_java_apphosting_PubsubTaskRunnerPayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_PubsubTaskRunnerPayload_descriptor,
new java.lang.String[] { "PubsubMessage", "InternalTopicName", });
internal_static_java_apphosting_FunctionTaskRunnerPayload_descriptor =
getDescriptor().getMessageTypes().get(21);
internal_static_java_apphosting_FunctionTaskRunnerPayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_FunctionTaskRunnerPayload_descriptor,
new java.lang.String[] { "Method", "Url", "Header", "Body", "FunctionName", });
internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_descriptor =
internal_static_java_apphosting_FunctionTaskRunnerPayload_descriptor.getNestedTypes().get(0);
internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_FunctionTaskRunnerPayload_Header_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_descriptor =
getDescriptor().getMessageTypes().get(22);
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_descriptor,
new java.lang.String[] { "Method", "Url", "Header", "Body", "RequestorId", "RequestDeadlineMs", "FetchTimeoutMs", "UserAgentToSend", "FailOnSslCertificateError", });
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_descriptor =
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_descriptor.getNestedTypes().get(0);
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_ExternalHttpTaskRunnerPayload_Header_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_java_apphosting_ApiSource_descriptor =
getDescriptor().getMessageTypes().get(23);
internal_static_java_apphosting_ApiSource_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_ApiSource_descriptor,
new java.lang.String[] { });
internal_static_java_apphosting_TaskDefinition_descriptor =
getDescriptor().getMessageTypes().get(24);
internal_static_java_apphosting_TaskDefinition_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskDefinition_descriptor,
new java.lang.String[] { "TaskRef", "EtaUsec", "PreviousEtaUsec", "Payload", "RetryCount", "StoreTimestamp", "LastInvocationStats", "Description", "RetryParameters", "FirstTryUsec", "Tag", "ExecutionCount", "IndexShardNumber", "CronRetryParameters", "CronRetryCount", "CronRunStartUsec", "NotifyRecord", "CreationSourceType", "TaskAuthorization", });
internal_static_java_apphosting_TaskGoldenVersion_descriptor =
getDescriptor().getMessageTypes().get(25);
internal_static_java_apphosting_TaskGoldenVersion_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_TaskGoldenVersion_descriptor,
new java.lang.String[] { "StoreTimestamp", });
internal_static_java_apphosting_NotifyRoutingRecord_descriptor =
getDescriptor().getMessageTypes().get(26);
internal_static_java_apphosting_NotifyRoutingRecord_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_NotifyRoutingRecord_descriptor,
new java.lang.String[] { "Sent", "ScannerBns", "QueueMapTimestampUsec", });
com.google.apphosting.executor.Queue.getDescriptor();
com.google.apphosting.executor.Retry.getDescriptor();
com.google.apphosting.executor.Target.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy