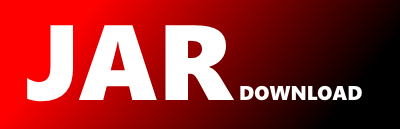
com.google.appengine.api.datastore.AutoValue_CloudDatastoreRemoteServiceConfig Maven / Gradle / Ivy
package com.google.appengine.api.datastore;
import com.google.common.collect.ImmutableSet;
import java.security.PrivateKey;
import java.util.Set;
import javax.annotation.Generated;
import org.checkerframework.checker.nullness.qual.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_CloudDatastoreRemoteServiceConfig extends CloudDatastoreRemoteServiceConfig {
private final CloudDatastoreRemoteServiceConfig.@Nullable AppId appId;
private final @Nullable String emulatorHost;
private final @Nullable String hostOverride;
private final @Nullable ImmutableSet additionalAppIds;
private final boolean installApiProxyEnvironment;
private final @Nullable String serviceAccount;
private final @Nullable PrivateKey privateKey;
private final @Nullable String accessToken;
private final boolean useComputeEngineCredential;
private final int maxRetries;
private final int httpConnectTimeoutMillis;
private final boolean asyncStackTraceCaptureEnabled;
private AutoValue_CloudDatastoreRemoteServiceConfig(
CloudDatastoreRemoteServiceConfig.@Nullable AppId appId,
@Nullable String emulatorHost,
@Nullable String hostOverride,
@Nullable ImmutableSet additionalAppIds,
boolean installApiProxyEnvironment,
@Nullable String serviceAccount,
@Nullable PrivateKey privateKey,
@Nullable String accessToken,
boolean useComputeEngineCredential,
int maxRetries,
int httpConnectTimeoutMillis,
boolean asyncStackTraceCaptureEnabled) {
this.appId = appId;
this.emulatorHost = emulatorHost;
this.hostOverride = hostOverride;
this.additionalAppIds = additionalAppIds;
this.installApiProxyEnvironment = installApiProxyEnvironment;
this.serviceAccount = serviceAccount;
this.privateKey = privateKey;
this.accessToken = accessToken;
this.useComputeEngineCredential = useComputeEngineCredential;
this.maxRetries = maxRetries;
this.httpConnectTimeoutMillis = httpConnectTimeoutMillis;
this.asyncStackTraceCaptureEnabled = asyncStackTraceCaptureEnabled;
}
@Override
CloudDatastoreRemoteServiceConfig.@Nullable AppId appId() {
return appId;
}
@Override
@Nullable String emulatorHost() {
return emulatorHost;
}
@Override
@Nullable String hostOverride() {
return hostOverride;
}
@Override
@Nullable ImmutableSet additionalAppIds() {
return additionalAppIds;
}
@Override
boolean installApiProxyEnvironment() {
return installApiProxyEnvironment;
}
@Override
@Nullable String serviceAccount() {
return serviceAccount;
}
@Override
@Nullable PrivateKey privateKey() {
return privateKey;
}
@Override
@Nullable String accessToken() {
return accessToken;
}
@Override
boolean useComputeEngineCredential() {
return useComputeEngineCredential;
}
@Override
int maxRetries() {
return maxRetries;
}
@Override
int httpConnectTimeoutMillis() {
return httpConnectTimeoutMillis;
}
@Override
boolean asyncStackTraceCaptureEnabled() {
return asyncStackTraceCaptureEnabled;
}
@Override
public String toString() {
return "CloudDatastoreRemoteServiceConfig{"
+ "appId=" + appId + ", "
+ "emulatorHost=" + emulatorHost + ", "
+ "hostOverride=" + hostOverride + ", "
+ "additionalAppIds=" + additionalAppIds + ", "
+ "installApiProxyEnvironment=" + installApiProxyEnvironment + ", "
+ "serviceAccount=" + serviceAccount + ", "
+ "privateKey=" + privateKey + ", "
+ "accessToken=" + accessToken + ", "
+ "useComputeEngineCredential=" + useComputeEngineCredential + ", "
+ "maxRetries=" + maxRetries + ", "
+ "httpConnectTimeoutMillis=" + httpConnectTimeoutMillis + ", "
+ "asyncStackTraceCaptureEnabled=" + asyncStackTraceCaptureEnabled
+ "}";
}
@Override
public boolean equals(@Nullable Object o) {
if (o == this) {
return true;
}
if (o instanceof CloudDatastoreRemoteServiceConfig) {
CloudDatastoreRemoteServiceConfig that = (CloudDatastoreRemoteServiceConfig) o;
return (this.appId == null ? that.appId() == null : this.appId.equals(that.appId()))
&& (this.emulatorHost == null ? that.emulatorHost() == null : this.emulatorHost.equals(that.emulatorHost()))
&& (this.hostOverride == null ? that.hostOverride() == null : this.hostOverride.equals(that.hostOverride()))
&& (this.additionalAppIds == null ? that.additionalAppIds() == null : this.additionalAppIds.equals(that.additionalAppIds()))
&& this.installApiProxyEnvironment == that.installApiProxyEnvironment()
&& (this.serviceAccount == null ? that.serviceAccount() == null : this.serviceAccount.equals(that.serviceAccount()))
&& (this.privateKey == null ? that.privateKey() == null : this.privateKey.equals(that.privateKey()))
&& (this.accessToken == null ? that.accessToken() == null : this.accessToken.equals(that.accessToken()))
&& this.useComputeEngineCredential == that.useComputeEngineCredential()
&& this.maxRetries == that.maxRetries()
&& this.httpConnectTimeoutMillis == that.httpConnectTimeoutMillis()
&& this.asyncStackTraceCaptureEnabled == that.asyncStackTraceCaptureEnabled();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (appId == null) ? 0 : appId.hashCode();
h$ *= 1000003;
h$ ^= (emulatorHost == null) ? 0 : emulatorHost.hashCode();
h$ *= 1000003;
h$ ^= (hostOverride == null) ? 0 : hostOverride.hashCode();
h$ *= 1000003;
h$ ^= (additionalAppIds == null) ? 0 : additionalAppIds.hashCode();
h$ *= 1000003;
h$ ^= installApiProxyEnvironment ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (serviceAccount == null) ? 0 : serviceAccount.hashCode();
h$ *= 1000003;
h$ ^= (privateKey == null) ? 0 : privateKey.hashCode();
h$ *= 1000003;
h$ ^= (accessToken == null) ? 0 : accessToken.hashCode();
h$ *= 1000003;
h$ ^= useComputeEngineCredential ? 1231 : 1237;
h$ *= 1000003;
h$ ^= maxRetries;
h$ *= 1000003;
h$ ^= httpConnectTimeoutMillis;
h$ *= 1000003;
h$ ^= asyncStackTraceCaptureEnabled ? 1231 : 1237;
return h$;
}
static final class Builder extends CloudDatastoreRemoteServiceConfig.Builder {
private CloudDatastoreRemoteServiceConfig.@Nullable AppId appId;
private @Nullable String emulatorHost;
private @Nullable String hostOverride;
private @Nullable ImmutableSet additionalAppIds;
private boolean installApiProxyEnvironment;
private @Nullable String serviceAccount;
private @Nullable PrivateKey privateKey;
private @Nullable String accessToken;
private boolean useComputeEngineCredential;
private int maxRetries;
private int httpConnectTimeoutMillis;
private boolean asyncStackTraceCaptureEnabled;
private byte set$0;
Builder() {
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder appId(CloudDatastoreRemoteServiceConfig.AppId appId) {
this.appId = appId;
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder emulatorHost(String emulatorHost) {
this.emulatorHost = emulatorHost;
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder hostOverride(String hostOverride) {
this.hostOverride = hostOverride;
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder additionalAppIds(Set additionalAppIds) {
this.additionalAppIds = (additionalAppIds == null ? null : ImmutableSet.copyOf(additionalAppIds));
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder installApiProxyEnvironment(boolean installApiProxyEnvironment) {
this.installApiProxyEnvironment = installApiProxyEnvironment;
set$0 |= (byte) 1;
return this;
}
@Override
CloudDatastoreRemoteServiceConfig.Builder serviceAccount(String serviceAccount) {
this.serviceAccount = serviceAccount;
return this;
}
@Override
CloudDatastoreRemoteServiceConfig.Builder privateKey(PrivateKey privateKey) {
this.privateKey = privateKey;
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder accessToken(String accessToken) {
this.accessToken = accessToken;
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder useComputeEngineCredential(boolean useComputeEngineCredential) {
this.useComputeEngineCredential = useComputeEngineCredential;
set$0 |= (byte) 2;
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder maxRetries(int maxRetries) {
this.maxRetries = maxRetries;
set$0 |= (byte) 4;
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder httpConnectTimeoutMillis(int httpConnectTimeoutMillis) {
this.httpConnectTimeoutMillis = httpConnectTimeoutMillis;
set$0 |= (byte) 8;
return this;
}
@Override
public CloudDatastoreRemoteServiceConfig.Builder asyncStackTraceCaptureEnabled(boolean asyncStackTraceCaptureEnabled) {
this.asyncStackTraceCaptureEnabled = asyncStackTraceCaptureEnabled;
set$0 |= (byte) 0x10;
return this;
}
@Override
CloudDatastoreRemoteServiceConfig autoBuild() {
if (set$0 != 0x1f) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" installApiProxyEnvironment");
}
if ((set$0 & 2) == 0) {
missing.append(" useComputeEngineCredential");
}
if ((set$0 & 4) == 0) {
missing.append(" maxRetries");
}
if ((set$0 & 8) == 0) {
missing.append(" httpConnectTimeoutMillis");
}
if ((set$0 & 0x10) == 0) {
missing.append(" asyncStackTraceCaptureEnabled");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CloudDatastoreRemoteServiceConfig(
this.appId,
this.emulatorHost,
this.hostOverride,
this.additionalAppIds,
this.installApiProxyEnvironment,
this.serviceAccount,
this.privateKey,
this.accessToken,
this.useComputeEngineCredential,
this.maxRetries,
this.httpConnectTimeoutMillis,
this.asyncStackTraceCaptureEnabled);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy