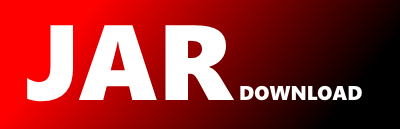
com.google.appengine.api.memcache.MemcacheServicePb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: memcache_service.proto
package com.google.appengine.api.memcache;
public final class MemcacheServicePb {
private MemcacheServicePb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface MemcacheServiceErrorOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheServiceError)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code java.apphosting.MemcacheServiceError}
*/
public static final class MemcacheServiceError extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheServiceError)
MemcacheServiceErrorOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheServiceError.newBuilder() to construct.
private MemcacheServiceError(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheServiceError() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheServiceError();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheServiceError_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheServiceError_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.MemcacheServiceError.ErrorCode}
*/
public enum ErrorCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* OK = 0;
*/
OK(0),
/**
*
* This could be any error including including misconfiguration,
* client-caused bad keys, and some types of temporary
* unavailability.
*
*
* UNSPECIFIED_ERROR = 1;
*/
UNSPECIFIED_ERROR(1),
/**
*
* Can currently (Mar 2014) only be returned by GrabTail, which is
* not exposed to customer.
*
*
* NAMESPACE_NOT_SET = 2;
*/
NAMESPACE_NOT_SET(2),
/**
*
* A non-superuser app attempted to use app_override.
*
*
* PERMISSION_DENIED = 3;
*/
PERMISSION_DENIED(3),
/**
*
* An attempt was made to increment a value that was not a valid
* number.
*
*
* INVALID_VALUE = 6;
*/
INVALID_VALUE(6),
/**
*
* The memcache_service is currently unavailable. This is a most
* likely a transient condition and may be corrected by retrying
* with a backoff. Possible reasons include not being able to
* connect to memcacheg backends because of shardmap changes or
* global chubby outages. For historical reasons the runtimes
* convert this into a capability-disabled exception or error.
*
*
* UNAVAILABLE = 9;
*/
UNAVAILABLE(9),
;
/**
* OK = 0;
*/
public static final int OK_VALUE = 0;
/**
*
* This could be any error including including misconfiguration,
* client-caused bad keys, and some types of temporary
* unavailability.
*
*
* UNSPECIFIED_ERROR = 1;
*/
public static final int UNSPECIFIED_ERROR_VALUE = 1;
/**
*
* Can currently (Mar 2014) only be returned by GrabTail, which is
* not exposed to customer.
*
*
* NAMESPACE_NOT_SET = 2;
*/
public static final int NAMESPACE_NOT_SET_VALUE = 2;
/**
*
* A non-superuser app attempted to use app_override.
*
*
* PERMISSION_DENIED = 3;
*/
public static final int PERMISSION_DENIED_VALUE = 3;
/**
*
* An attempt was made to increment a value that was not a valid
* number.
*
*
* INVALID_VALUE = 6;
*/
public static final int INVALID_VALUE_VALUE = 6;
/**
*
* The memcache_service is currently unavailable. This is a most
* likely a transient condition and may be corrected by retrying
* with a backoff. Possible reasons include not being able to
* connect to memcacheg backends because of shardmap changes or
* global chubby outages. For historical reasons the runtimes
* convert this into a capability-disabled exception or error.
*
*
* UNAVAILABLE = 9;
*/
public static final int UNAVAILABLE_VALUE = 9;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ErrorCode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ErrorCode forNumber(int value) {
switch (value) {
case 0: return OK;
case 1: return UNSPECIFIED_ERROR;
case 2: return NAMESPACE_NOT_SET;
case 3: return PERMISSION_DENIED;
case 6: return INVALID_VALUE;
case 9: return UNAVAILABLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ErrorCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ErrorCode findValueByNumber(int number) {
return ErrorCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError.getDescriptor().getEnumTypes().get(0);
}
private static final ErrorCode[] VALUES = values();
public static ErrorCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ErrorCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.MemcacheServiceError.ErrorCode)
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheServiceError}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheServiceError)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceErrorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheServiceError_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheServiceError_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheServiceError_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheServiceError)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheServiceError)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheServiceError parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheServiceError getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AppOverrideOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.AppOverride)
com.google.protobuf.MessageOrBuilder {
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return Whether the appId field is set.
*/
boolean hasAppId();
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return The appId.
*/
java.lang.String getAppId();
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return The bytes for appId.
*/
com.google.protobuf.ByteString
getAppIdBytes();
}
/**
*
* This message allows you to manipulate the memcache of a different
* application (not the memcache that belongs to the app initiating
* the request). If you are working with your own memcache (the usual case)
* you shouldn't specify it.
* Originally this mechanism required the client to pass along details
* of the backend configuration, since the appserver hosting the app
* performing the override would not have this info for the target app. The
* current memcache service implementation has been improved to obviate this,
* and the fields marked deprecated below will be ignored by the new server.
* TODO: Remove uses of deprecated fields once the new memcache
* service implementation is deployed.
*
*
* Protobuf type {@code java.apphosting.AppOverride}
*/
public static final class AppOverride extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.AppOverride)
AppOverrideOrBuilder {
private static final long serialVersionUID = 0L;
// Use AppOverride.newBuilder() to construct.
private AppOverride(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AppOverride() {
appId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AppOverride();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_AppOverride_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_AppOverride_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.class, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder.class);
}
private int bitField0_;
public static final int APP_ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object appId_ = "";
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return Whether the appId field is set.
*/
@java.lang.Override
public boolean hasAppId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return The appId.
*/
@java.lang.Override
public java.lang.String getAppId() {
java.lang.Object ref = appId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appId_ = s;
}
return s;
}
}
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return The bytes for appId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAppIdBytes() {
java.lang.Object ref = appId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasAppId()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, appId_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, appId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.AppOverride)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride other = (com.google.appengine.api.memcache.MemcacheServicePb.AppOverride) obj;
if (hasAppId() != other.hasAppId()) return false;
if (hasAppId()) {
if (!getAppId()
.equals(other.getAppId())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAppId()) {
hash = (37 * hash) + APP_ID_FIELD_NUMBER;
hash = (53 * hash) + getAppId().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* This message allows you to manipulate the memcache of a different
* application (not the memcache that belongs to the app initiating
* the request). If you are working with your own memcache (the usual case)
* you shouldn't specify it.
* Originally this mechanism required the client to pass along details
* of the backend configuration, since the appserver hosting the app
* performing the override would not have this info for the target app. The
* current memcache service implementation has been improved to obviate this,
* and the fields marked deprecated below will be ignored by the new server.
* TODO: Remove uses of deprecated fields once the new memcache
* service implementation is deployed.
*
*
* Protobuf type {@code java.apphosting.AppOverride}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.AppOverride)
com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_AppOverride_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_AppOverride_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.class, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
appId_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_AppOverride_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride build() {
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride result = new com.google.appengine.api.memcache.MemcacheServicePb.AppOverride(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.appId_ = appId_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.AppOverride) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.AppOverride)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance()) return this;
if (other.hasAppId()) {
appId_ = other.appId_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasAppId()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
appId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object appId_ = "";
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return Whether the appId field is set.
*/
public boolean hasAppId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return The appId.
*/
public java.lang.String getAppId() {
java.lang.Object ref = appId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return The bytes for appId.
*/
public com.google.protobuf.ByteString
getAppIdBytes() {
java.lang.Object ref = appId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @param value The appId to set.
* @return This builder for chaining.
*/
public Builder setAppId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
appId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @return This builder for chaining.
*/
public Builder clearAppId() {
appId_ = getDefaultInstance().getAppId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The app_id of the target memcache.
*
*
* required string app_id = 1;
* @param value The bytes for appId to set.
* @return This builder for chaining.
*/
public Builder setAppIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
appId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.AppOverride)
}
// @@protoc_insertion_point(class_scope:java.apphosting.AppOverride)
private static final com.google.appengine.api.memcache.MemcacheServicePb.AppOverride DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.AppOverride();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AppOverride parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheGetRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheGetRequest)
com.google.protobuf.MessageOrBuilder {
/**
* repeated bytes key = 1;
* @return A list containing the key.
*/
java.util.List getKeyList();
/**
* repeated bytes key = 1;
* @return The count of key.
*/
int getKeyCount();
/**
* repeated bytes key = 1;
* @param index The index of the element to return.
* @return The key at the given index.
*/
com.google.protobuf.ByteString getKey(int index);
/**
* optional string name_space = 2 [default = ""];
* @return Whether the nameSpace field is set.
*/
boolean hasNameSpace();
/**
* optional string name_space = 2 [default = ""];
* @return The nameSpace.
*/
java.lang.String getNameSpace();
/**
* optional string name_space = 2 [default = ""];
* @return The bytes for nameSpace.
*/
com.google.protobuf.ByteString
getNameSpaceBytes();
/**
*
* If set true, returned items will include a "cas_id" value needed
* to do a future atomic compare-and-swap operation. This allocates
* and initializes the cas_id on each returned item, even if it
* wasn't put into memcacheg initially with cas set. Because the
* cas_id takes memory in the server and bandwidth in the response,
* you should only set this if you're actually planning to use CAS.
*
*
* optional bool for_cas = 4;
* @return Whether the forCas field is set.
*/
boolean hasForCas();
/**
*
* If set true, returned items will include a "cas_id" value needed
* to do a future atomic compare-and-swap operation. This allocates
* and initializes the cas_id on each returned item, even if it
* wasn't put into memcacheg initially with cas set. Because the
* cas_id takes memory in the server and bandwidth in the response,
* you should only set this if you're actually planning to use CAS.
*
*
* optional bool for_cas = 4;
* @return The forCas.
*/
boolean getForCas();
/**
* optional .java.apphosting.AppOverride override = 5;
* @return Whether the override field is set.
*/
boolean hasOverride();
/**
* optional .java.apphosting.AppOverride override = 5;
* @return The override.
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride();
/**
* optional .java.apphosting.AppOverride override = 5;
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder();
/**
*
* If set true, returned items will include timestamps and indicate whether
* the item is delete_locked or not. Peeking at items will update stats, but
* will not alter the eviction order of the item.
*
*
* optional bool for_peek = 6;
* @return Whether the forPeek field is set.
*/
boolean hasForPeek();
/**
*
* If set true, returned items will include timestamps and indicate whether
* the item is delete_locked or not. Peeking at items will update stats, but
* will not alter the eviction order of the item.
*
*
* optional bool for_peek = 6;
* @return The forPeek.
*/
boolean getForPeek();
}
/**
*
* Next tag: 7
*
*
* Protobuf type {@code java.apphosting.MemcacheGetRequest}
*/
public static final class MemcacheGetRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheGetRequest)
MemcacheGetRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheGetRequest.newBuilder() to construct.
private MemcacheGetRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheGetRequest() {
key_ = java.util.Collections.emptyList();
nameSpace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheGetRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List key_;
/**
* repeated bytes key = 1;
* @return A list containing the key.
*/
@java.lang.Override
public java.util.List
getKeyList() {
return key_;
}
/**
* repeated bytes key = 1;
* @return The count of key.
*/
public int getKeyCount() {
return key_.size();
}
/**
* repeated bytes key = 1;
* @param index The index of the element to return.
* @return The key at the given index.
*/
public com.google.protobuf.ByteString getKey(int index) {
return key_.get(index);
}
public static final int NAME_SPACE_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 2 [default = ""];
* @return Whether the nameSpace field is set.
*/
@java.lang.Override
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name_space = 2 [default = ""];
* @return The nameSpace.
*/
@java.lang.Override
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
}
}
/**
* optional string name_space = 2 [default = ""];
* @return The bytes for nameSpace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FOR_CAS_FIELD_NUMBER = 4;
private boolean forCas_ = false;
/**
*
* If set true, returned items will include a "cas_id" value needed
* to do a future atomic compare-and-swap operation. This allocates
* and initializes the cas_id on each returned item, even if it
* wasn't put into memcacheg initially with cas set. Because the
* cas_id takes memory in the server and bandwidth in the response,
* you should only set this if you're actually planning to use CAS.
*
*
* optional bool for_cas = 4;
* @return Whether the forCas field is set.
*/
@java.lang.Override
public boolean hasForCas() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* If set true, returned items will include a "cas_id" value needed
* to do a future atomic compare-and-swap operation. This allocates
* and initializes the cas_id on each returned item, even if it
* wasn't put into memcacheg initially with cas set. Because the
* cas_id takes memory in the server and bandwidth in the response,
* you should only set this if you're actually planning to use CAS.
*
*
* optional bool for_cas = 4;
* @return The forCas.
*/
@java.lang.Override
public boolean getForCas() {
return forCas_;
}
public static final int OVERRIDE_FIELD_NUMBER = 5;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
/**
* optional .java.apphosting.AppOverride override = 5;
* @return Whether the override field is set.
*/
@java.lang.Override
public boolean hasOverride() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 5;
* @return The override.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
public static final int FOR_PEEK_FIELD_NUMBER = 6;
private boolean forPeek_ = false;
/**
*
* If set true, returned items will include timestamps and indicate whether
* the item is delete_locked or not. Peeking at items will update stats, but
* will not alter the eviction order of the item.
*
*
* optional bool for_peek = 6;
* @return Whether the forPeek field is set.
*/
@java.lang.Override
public boolean hasForPeek() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* If set true, returned items will include timestamps and indicate whether
* the item is delete_locked or not. Peeking at items will update stats, but
* will not alter the eviction order of the item.
*
*
* optional bool for_peek = 6;
* @return The forPeek.
*/
@java.lang.Override
public boolean getForPeek() {
return forPeek_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasOverride()) {
if (!getOverride().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < key_.size(); i++) {
output.writeBytes(1, key_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, nameSpace_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(4, forCas_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(5, getOverride());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(6, forPeek_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < key_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(key_.get(i));
}
size += dataSize;
size += 1 * getKeyList().size();
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, nameSpace_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, forCas_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getOverride());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, forPeek_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest) obj;
if (!getKeyList()
.equals(other.getKeyList())) return false;
if (hasNameSpace() != other.hasNameSpace()) return false;
if (hasNameSpace()) {
if (!getNameSpace()
.equals(other.getNameSpace())) return false;
}
if (hasForCas() != other.hasForCas()) return false;
if (hasForCas()) {
if (getForCas()
!= other.getForCas()) return false;
}
if (hasOverride() != other.hasOverride()) return false;
if (hasOverride()) {
if (!getOverride()
.equals(other.getOverride())) return false;
}
if (hasForPeek() != other.hasForPeek()) return false;
if (hasForPeek()) {
if (getForPeek()
!= other.getForPeek()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getKeyCount() > 0) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKeyList().hashCode();
}
if (hasNameSpace()) {
hash = (37 * hash) + NAME_SPACE_FIELD_NUMBER;
hash = (53 * hash) + getNameSpace().hashCode();
}
if (hasForCas()) {
hash = (37 * hash) + FOR_CAS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getForCas());
}
if (hasOverride()) {
hash = (37 * hash) + OVERRIDE_FIELD_NUMBER;
hash = (53 * hash) + getOverride().hashCode();
}
if (hasForPeek()) {
hash = (37 * hash) + FOR_PEEK_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getForPeek());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Next tag: 7
*
*
* Protobuf type {@code java.apphosting.MemcacheGetRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheGetRequest)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOverrideFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = java.util.Collections.emptyList();
nameSpace_ = "";
forCas_ = false;
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
forPeek_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetRequest_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest result) {
if (((bitField0_ & 0x00000001) != 0)) {
key_ = java.util.Collections.unmodifiableList(key_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.key_ = key_;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.nameSpace_ = nameSpace_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.forCas_ = forCas_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.override_ = overrideBuilder_ == null
? override_
: overrideBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.forPeek_ = forPeek_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest.getDefaultInstance()) return this;
if (!other.key_.isEmpty()) {
if (key_.isEmpty()) {
key_ = other.key_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureKeyIsMutable();
key_.addAll(other.key_);
}
onChanged();
}
if (other.hasNameSpace()) {
nameSpace_ = other.nameSpace_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasForCas()) {
setForCas(other.getForCas());
}
if (other.hasOverride()) {
mergeOverride(other.getOverride());
}
if (other.hasForPeek()) {
setForPeek(other.getForPeek());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasOverride()) {
if (!getOverride().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.ByteString v = input.readBytes();
ensureKeyIsMutable();
key_.add(v);
break;
} // case 10
case 18: {
nameSpace_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 32: {
forCas_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 32
case 42: {
input.readMessage(
getOverrideFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 42
case 48: {
forPeek_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 48
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List key_ = java.util.Collections.emptyList();
private void ensureKeyIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
key_ = new java.util.ArrayList(key_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated bytes key = 1;
* @return A list containing the key.
*/
public java.util.List
getKeyList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(key_) : key_;
}
/**
* repeated bytes key = 1;
* @return The count of key.
*/
public int getKeyCount() {
return key_.size();
}
/**
* repeated bytes key = 1;
* @param index The index of the element to return.
* @return The key at the given index.
*/
public com.google.protobuf.ByteString getKey(int index) {
return key_.get(index);
}
/**
* repeated bytes key = 1;
* @param index The index to set the value at.
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(
int index, com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
ensureKeyIsMutable();
key_.set(index, value);
onChanged();
return this;
}
/**
* repeated bytes key = 1;
* @param value The key to add.
* @return This builder for chaining.
*/
public Builder addKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
ensureKeyIsMutable();
key_.add(value);
onChanged();
return this;
}
/**
* repeated bytes key = 1;
* @param values The key to add.
* @return This builder for chaining.
*/
public Builder addAllKey(
java.lang.Iterable extends com.google.protobuf.ByteString> values) {
ensureKeyIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, key_);
onChanged();
return this;
}
/**
* repeated bytes key = 1;
* @return This builder for chaining.
*/
public Builder clearKey() {
key_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 2 [default = ""];
* @return Whether the nameSpace field is set.
*/
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string name_space = 2 [default = ""];
* @return The nameSpace.
*/
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name_space = 2 [default = ""];
* @return The bytes for nameSpace.
*/
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name_space = 2 [default = ""];
* @param value The nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpace(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional string name_space = 2 [default = ""];
* @return This builder for chaining.
*/
public Builder clearNameSpace() {
nameSpace_ = getDefaultInstance().getNameSpace();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* optional string name_space = 2 [default = ""];
* @param value The bytes for nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private boolean forCas_ ;
/**
*
* If set true, returned items will include a "cas_id" value needed
* to do a future atomic compare-and-swap operation. This allocates
* and initializes the cas_id on each returned item, even if it
* wasn't put into memcacheg initially with cas set. Because the
* cas_id takes memory in the server and bandwidth in the response,
* you should only set this if you're actually planning to use CAS.
*
*
* optional bool for_cas = 4;
* @return Whether the forCas field is set.
*/
@java.lang.Override
public boolean hasForCas() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* If set true, returned items will include a "cas_id" value needed
* to do a future atomic compare-and-swap operation. This allocates
* and initializes the cas_id on each returned item, even if it
* wasn't put into memcacheg initially with cas set. Because the
* cas_id takes memory in the server and bandwidth in the response,
* you should only set this if you're actually planning to use CAS.
*
*
* optional bool for_cas = 4;
* @return The forCas.
*/
@java.lang.Override
public boolean getForCas() {
return forCas_;
}
/**
*
* If set true, returned items will include a "cas_id" value needed
* to do a future atomic compare-and-swap operation. This allocates
* and initializes the cas_id on each returned item, even if it
* wasn't put into memcacheg initially with cas set. Because the
* cas_id takes memory in the server and bandwidth in the response,
* you should only set this if you're actually planning to use CAS.
*
*
* optional bool for_cas = 4;
* @param value The forCas to set.
* @return This builder for chaining.
*/
public Builder setForCas(boolean value) {
forCas_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* If set true, returned items will include a "cas_id" value needed
* to do a future atomic compare-and-swap operation. This allocates
* and initializes the cas_id on each returned item, even if it
* wasn't put into memcacheg initially with cas set. Because the
* cas_id takes memory in the server and bandwidth in the response,
* you should only set this if you're actually planning to use CAS.
*
*
* optional bool for_cas = 4;
* @return This builder for chaining.
*/
public Builder clearForCas() {
bitField0_ = (bitField0_ & ~0x00000004);
forCas_ = false;
onChanged();
return this;
}
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder> overrideBuilder_;
/**
* optional .java.apphosting.AppOverride override = 5;
* @return Whether the override field is set.
*/
public boolean hasOverride() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 5;
* @return The override.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
if (overrideBuilder_ == null) {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
} else {
return overrideBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public Builder setOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
override_ = value;
} else {
overrideBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public Builder setOverride(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder builderForValue) {
if (overrideBuilder_ == null) {
override_ = builderForValue.build();
} else {
overrideBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public Builder mergeOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
override_ != null &&
override_ != com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance()) {
getOverrideBuilder().mergeFrom(value);
} else {
override_ = value;
}
} else {
overrideBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public Builder clearOverride() {
bitField0_ = (bitField0_ & ~0x00000008);
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder getOverrideBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getOverrideFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
if (overrideBuilder_ != null) {
return overrideBuilder_.getMessageOrBuilder();
} else {
return override_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>
getOverrideFieldBuilder() {
if (overrideBuilder_ == null) {
overrideBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>(
getOverride(),
getParentForChildren(),
isClean());
override_ = null;
}
return overrideBuilder_;
}
private boolean forPeek_ ;
/**
*
* If set true, returned items will include timestamps and indicate whether
* the item is delete_locked or not. Peeking at items will update stats, but
* will not alter the eviction order of the item.
*
*
* optional bool for_peek = 6;
* @return Whether the forPeek field is set.
*/
@java.lang.Override
public boolean hasForPeek() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* If set true, returned items will include timestamps and indicate whether
* the item is delete_locked or not. Peeking at items will update stats, but
* will not alter the eviction order of the item.
*
*
* optional bool for_peek = 6;
* @return The forPeek.
*/
@java.lang.Override
public boolean getForPeek() {
return forPeek_;
}
/**
*
* If set true, returned items will include timestamps and indicate whether
* the item is delete_locked or not. Peeking at items will update stats, but
* will not alter the eviction order of the item.
*
*
* optional bool for_peek = 6;
* @param value The forPeek to set.
* @return This builder for chaining.
*/
public Builder setForPeek(boolean value) {
forPeek_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* If set true, returned items will include timestamps and indicate whether
* the item is delete_locked or not. Peeking at items will update stats, but
* will not alter the eviction order of the item.
*
*
* optional bool for_peek = 6;
* @return This builder for chaining.
*/
public Builder clearForPeek() {
bitField0_ = (bitField0_ & ~0x00000010);
forPeek_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheGetRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheGetRequest)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheGetRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ItemTimestampsOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.ItemTimestamps)
com.google.protobuf.MessageOrBuilder {
/**
*
* Absolute expiration timestamp of the item. Unset if this item has no
* expiration timestamp.
*
*
* optional int64 expiration_time_sec = 1;
* @return Whether the expirationTimeSec field is set.
*/
boolean hasExpirationTimeSec();
/**
*
* Absolute expiration timestamp of the item. Unset if this item has no
* expiration timestamp.
*
*
* optional int64 expiration_time_sec = 1;
* @return The expirationTimeSec.
*/
long getExpirationTimeSec();
/**
*
* Absolute last accessed timestamp of the item.
*
*
* optional int64 last_access_time_sec = 2;
* @return Whether the lastAccessTimeSec field is set.
*/
boolean hasLastAccessTimeSec();
/**
*
* Absolute last accessed timestamp of the item.
*
*
* optional int64 last_access_time_sec = 2;
* @return The lastAccessTimeSec.
*/
long getLastAccessTimeSec();
/**
*
* Absolute delete_time timestamp of the item. Unset if this item is not
* delete locked.
*
*
* optional int64 delete_lock_time_sec = 3;
* @return Whether the deleteLockTimeSec field is set.
*/
boolean hasDeleteLockTimeSec();
/**
*
* Absolute delete_time timestamp of the item. Unset if this item is not
* delete locked.
*
*
* optional int64 delete_lock_time_sec = 3;
* @return The deleteLockTimeSec.
*/
long getDeleteLockTimeSec();
}
/**
*
* Timestamps relevant to a memcache item.
* Next tag: 4
*
*
* Protobuf type {@code java.apphosting.ItemTimestamps}
*/
public static final class ItemTimestamps extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.ItemTimestamps)
ItemTimestampsOrBuilder {
private static final long serialVersionUID = 0L;
// Use ItemTimestamps.newBuilder() to construct.
private ItemTimestamps(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ItemTimestamps() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ItemTimestamps();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_ItemTimestamps_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_ItemTimestamps_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.class, com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.Builder.class);
}
private int bitField0_;
public static final int EXPIRATION_TIME_SEC_FIELD_NUMBER = 1;
private long expirationTimeSec_ = 0L;
/**
*
* Absolute expiration timestamp of the item. Unset if this item has no
* expiration timestamp.
*
*
* optional int64 expiration_time_sec = 1;
* @return Whether the expirationTimeSec field is set.
*/
@java.lang.Override
public boolean hasExpirationTimeSec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Absolute expiration timestamp of the item. Unset if this item has no
* expiration timestamp.
*
*
* optional int64 expiration_time_sec = 1;
* @return The expirationTimeSec.
*/
@java.lang.Override
public long getExpirationTimeSec() {
return expirationTimeSec_;
}
public static final int LAST_ACCESS_TIME_SEC_FIELD_NUMBER = 2;
private long lastAccessTimeSec_ = 0L;
/**
*
* Absolute last accessed timestamp of the item.
*
*
* optional int64 last_access_time_sec = 2;
* @return Whether the lastAccessTimeSec field is set.
*/
@java.lang.Override
public boolean hasLastAccessTimeSec() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Absolute last accessed timestamp of the item.
*
*
* optional int64 last_access_time_sec = 2;
* @return The lastAccessTimeSec.
*/
@java.lang.Override
public long getLastAccessTimeSec() {
return lastAccessTimeSec_;
}
public static final int DELETE_LOCK_TIME_SEC_FIELD_NUMBER = 3;
private long deleteLockTimeSec_ = 0L;
/**
*
* Absolute delete_time timestamp of the item. Unset if this item is not
* delete locked.
*
*
* optional int64 delete_lock_time_sec = 3;
* @return Whether the deleteLockTimeSec field is set.
*/
@java.lang.Override
public boolean hasDeleteLockTimeSec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Absolute delete_time timestamp of the item. Unset if this item is not
* delete locked.
*
*
* optional int64 delete_lock_time_sec = 3;
* @return The deleteLockTimeSec.
*/
@java.lang.Override
public long getDeleteLockTimeSec() {
return deleteLockTimeSec_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, expirationTimeSec_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, lastAccessTimeSec_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(3, deleteLockTimeSec_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, expirationTimeSec_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, lastAccessTimeSec_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, deleteLockTimeSec_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps other = (com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps) obj;
if (hasExpirationTimeSec() != other.hasExpirationTimeSec()) return false;
if (hasExpirationTimeSec()) {
if (getExpirationTimeSec()
!= other.getExpirationTimeSec()) return false;
}
if (hasLastAccessTimeSec() != other.hasLastAccessTimeSec()) return false;
if (hasLastAccessTimeSec()) {
if (getLastAccessTimeSec()
!= other.getLastAccessTimeSec()) return false;
}
if (hasDeleteLockTimeSec() != other.hasDeleteLockTimeSec()) return false;
if (hasDeleteLockTimeSec()) {
if (getDeleteLockTimeSec()
!= other.getDeleteLockTimeSec()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasExpirationTimeSec()) {
hash = (37 * hash) + EXPIRATION_TIME_SEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getExpirationTimeSec());
}
if (hasLastAccessTimeSec()) {
hash = (37 * hash) + LAST_ACCESS_TIME_SEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastAccessTimeSec());
}
if (hasDeleteLockTimeSec()) {
hash = (37 * hash) + DELETE_LOCK_TIME_SEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDeleteLockTimeSec());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Timestamps relevant to a memcache item.
* Next tag: 4
*
*
* Protobuf type {@code java.apphosting.ItemTimestamps}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.ItemTimestamps)
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestampsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_ItemTimestamps_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_ItemTimestamps_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.class, com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
expirationTimeSec_ = 0L;
lastAccessTimeSec_ = 0L;
deleteLockTimeSec_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_ItemTimestamps_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps build() {
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps result = new com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.expirationTimeSec_ = expirationTimeSec_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.lastAccessTimeSec_ = lastAccessTimeSec_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.deleteLockTimeSec_ = deleteLockTimeSec_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.getDefaultInstance()) return this;
if (other.hasExpirationTimeSec()) {
setExpirationTimeSec(other.getExpirationTimeSec());
}
if (other.hasLastAccessTimeSec()) {
setLastAccessTimeSec(other.getLastAccessTimeSec());
}
if (other.hasDeleteLockTimeSec()) {
setDeleteLockTimeSec(other.getDeleteLockTimeSec());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
expirationTimeSec_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
lastAccessTimeSec_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
deleteLockTimeSec_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long expirationTimeSec_ ;
/**
*
* Absolute expiration timestamp of the item. Unset if this item has no
* expiration timestamp.
*
*
* optional int64 expiration_time_sec = 1;
* @return Whether the expirationTimeSec field is set.
*/
@java.lang.Override
public boolean hasExpirationTimeSec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Absolute expiration timestamp of the item. Unset if this item has no
* expiration timestamp.
*
*
* optional int64 expiration_time_sec = 1;
* @return The expirationTimeSec.
*/
@java.lang.Override
public long getExpirationTimeSec() {
return expirationTimeSec_;
}
/**
*
* Absolute expiration timestamp of the item. Unset if this item has no
* expiration timestamp.
*
*
* optional int64 expiration_time_sec = 1;
* @param value The expirationTimeSec to set.
* @return This builder for chaining.
*/
public Builder setExpirationTimeSec(long value) {
expirationTimeSec_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Absolute expiration timestamp of the item. Unset if this item has no
* expiration timestamp.
*
*
* optional int64 expiration_time_sec = 1;
* @return This builder for chaining.
*/
public Builder clearExpirationTimeSec() {
bitField0_ = (bitField0_ & ~0x00000001);
expirationTimeSec_ = 0L;
onChanged();
return this;
}
private long lastAccessTimeSec_ ;
/**
*
* Absolute last accessed timestamp of the item.
*
*
* optional int64 last_access_time_sec = 2;
* @return Whether the lastAccessTimeSec field is set.
*/
@java.lang.Override
public boolean hasLastAccessTimeSec() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Absolute last accessed timestamp of the item.
*
*
* optional int64 last_access_time_sec = 2;
* @return The lastAccessTimeSec.
*/
@java.lang.Override
public long getLastAccessTimeSec() {
return lastAccessTimeSec_;
}
/**
*
* Absolute last accessed timestamp of the item.
*
*
* optional int64 last_access_time_sec = 2;
* @param value The lastAccessTimeSec to set.
* @return This builder for chaining.
*/
public Builder setLastAccessTimeSec(long value) {
lastAccessTimeSec_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Absolute last accessed timestamp of the item.
*
*
* optional int64 last_access_time_sec = 2;
* @return This builder for chaining.
*/
public Builder clearLastAccessTimeSec() {
bitField0_ = (bitField0_ & ~0x00000002);
lastAccessTimeSec_ = 0L;
onChanged();
return this;
}
private long deleteLockTimeSec_ ;
/**
*
* Absolute delete_time timestamp of the item. Unset if this item is not
* delete locked.
*
*
* optional int64 delete_lock_time_sec = 3;
* @return Whether the deleteLockTimeSec field is set.
*/
@java.lang.Override
public boolean hasDeleteLockTimeSec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Absolute delete_time timestamp of the item. Unset if this item is not
* delete locked.
*
*
* optional int64 delete_lock_time_sec = 3;
* @return The deleteLockTimeSec.
*/
@java.lang.Override
public long getDeleteLockTimeSec() {
return deleteLockTimeSec_;
}
/**
*
* Absolute delete_time timestamp of the item. Unset if this item is not
* delete locked.
*
*
* optional int64 delete_lock_time_sec = 3;
* @param value The deleteLockTimeSec to set.
* @return This builder for chaining.
*/
public Builder setDeleteLockTimeSec(long value) {
deleteLockTimeSec_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Absolute delete_time timestamp of the item. Unset if this item is not
* delete locked.
*
*
* optional int64 delete_lock_time_sec = 3;
* @return This builder for chaining.
*/
public Builder clearDeleteLockTimeSec() {
bitField0_ = (bitField0_ & ~0x00000004);
deleteLockTimeSec_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.ItemTimestamps)
}
// @@protoc_insertion_point(class_scope:java.apphosting.ItemTimestamps)
private static final com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ItemTimestamps parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheGetResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheGetResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated group Item = 1 { ... }
*/
java.util.List
getItemList();
/**
* repeated group Item = 1 { ... }
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item getItem(int index);
/**
* repeated group Item = 1 { ... }
*/
int getItemCount();
/**
* repeated group Item = 1 { ... }
*/
java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder>
getItemOrBuilderList();
/**
* repeated group Item = 1 { ... }
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder getItemOrBuilder(
int index);
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @return A list containing the getStatus.
*/
java.util.List getGetStatusList();
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @return The count of getStatus.
*/
int getGetStatusCount();
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @param index The index of the element to return.
* @return The getStatus at the given index.
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode getGetStatus(int index);
}
/**
*
* Next tag: 10
*
*
* Protobuf type {@code java.apphosting.MemcacheGetResponse}
*/
public static final class MemcacheGetResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheGetResponse)
MemcacheGetResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheGetResponse.newBuilder() to construct.
private MemcacheGetResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheGetResponse() {
item_ = java.util.Collections.emptyList();
getStatus_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheGetResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.MemcacheGetResponse.GetStatusCode}
*/
public enum GetStatusCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Key was found and value successfully returned.
*
*
* HIT = 1;
*/
HIT(1),
/**
*
* Key does not exist on the backend.
*
*
* MISS = 2;
*/
MISS(2),
/**
*
* Key was found, but value not returned because the response was truncated
* to fit into limited length of response.
* This is expected only if many keys were requested in a batch request;
* the default response size limit is 32 MiB, while items cannot be larger
* than 1 MiB.
*
*
* TRUNCATED = 3;
*/
TRUNCATED(3),
/**
*
* Response from backend not received in time. It's not known whether the
* key exists.
*
*
* DEADLINE_EXCEEDED = 4;
*/
DEADLINE_EXCEEDED(4),
/**
*
* Could not reach memcacheg backend (may be dead). It's not known whether
* the key exists.
*
*
* UNREACHABLE = 5;
*/
UNREACHABLE(5),
/**
*
* Other service error, e.g. other RPC errors, shardlock failure, missing
* config (ServingState). It's not known whether the key exists.
*
*
* OTHER_ERROR = 6;
*/
OTHER_ERROR(6),
;
/**
*
* Key was found and value successfully returned.
*
*
* HIT = 1;
*/
public static final int HIT_VALUE = 1;
/**
*
* Key does not exist on the backend.
*
*
* MISS = 2;
*/
public static final int MISS_VALUE = 2;
/**
*
* Key was found, but value not returned because the response was truncated
* to fit into limited length of response.
* This is expected only if many keys were requested in a batch request;
* the default response size limit is 32 MiB, while items cannot be larger
* than 1 MiB.
*
*
* TRUNCATED = 3;
*/
public static final int TRUNCATED_VALUE = 3;
/**
*
* Response from backend not received in time. It's not known whether the
* key exists.
*
*
* DEADLINE_EXCEEDED = 4;
*/
public static final int DEADLINE_EXCEEDED_VALUE = 4;
/**
*
* Could not reach memcacheg backend (may be dead). It's not known whether
* the key exists.
*
*
* UNREACHABLE = 5;
*/
public static final int UNREACHABLE_VALUE = 5;
/**
*
* Other service error, e.g. other RPC errors, shardlock failure, missing
* config (ServingState). It's not known whether the key exists.
*
*
* OTHER_ERROR = 6;
*/
public static final int OTHER_ERROR_VALUE = 6;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static GetStatusCode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static GetStatusCode forNumber(int value) {
switch (value) {
case 1: return HIT;
case 2: return MISS;
case 3: return TRUNCATED;
case 4: return DEADLINE_EXCEEDED;
case 5: return UNREACHABLE;
case 6: return OTHER_ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
GetStatusCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public GetStatusCode findValueByNumber(int number) {
return GetStatusCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.getDescriptor().getEnumTypes().get(0);
}
private static final GetStatusCode[] VALUES = values();
public static GetStatusCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private GetStatusCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.MemcacheGetResponse.GetStatusCode)
}
public interface ItemOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheGetResponse.Item)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes key = 2;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
* required bytes key = 2;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
* required bytes value = 3;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* required bytes value = 3;
* @return The value.
*/
com.google.protobuf.ByteString getValue();
/**
*
* server-opaque (app-owned) flags
*
*
* optional fixed32 flags = 4;
* @return Whether the flags field is set.
*/
boolean hasFlags();
/**
*
* server-opaque (app-owned) flags
*
*
* optional fixed32 flags = 4;
* @return The flags.
*/
int getFlags();
/**
*
* The compare-and-swap ID, if requested. Opaque to the client.
* All the client needs to know is that if they do a get request
* with "for_cas" set above, then get a cas_id, and then do a
* SetRequest with policy CAS, passing along the same opaque
* cas_id, it will only succeed if nobody else has replaced the
* value since then. The cas_ids won't be reused between versions
* of the value.
*
*
* optional fixed64 cas_id = 5;
* @return Whether the casId field is set.
*/
boolean hasCasId();
/**
*
* The compare-and-swap ID, if requested. Opaque to the client.
* All the client needs to know is that if they do a get request
* with "for_cas" set above, then get a cas_id, and then do a
* SetRequest with policy CAS, passing along the same opaque
* cas_id, it will only succeed if nobody else has replaced the
* value since then. The cas_ids won't be reused between versions
* of the value.
*
*
* optional fixed64 cas_id = 5;
* @return The casId.
*/
long getCasId();
/**
*
* Relative to now. Unset if this item has no expiration timestamp.
*
*
* optional int32 expires_in_seconds = 6;
* @return Whether the expiresInSeconds field is set.
*/
boolean hasExpiresInSeconds();
/**
*
* Relative to now. Unset if this item has no expiration timestamp.
*
*
* optional int32 expires_in_seconds = 6;
* @return The expiresInSeconds.
*/
int getExpiresInSeconds();
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
* @return Whether the timestamps field is set.
*/
boolean hasTimestamps();
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
* @return The timestamps.
*/
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps getTimestamps();
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestampsOrBuilder getTimestampsOrBuilder();
/**
*
* Item is delete_locked. Only returned if for_peek is set on the request.
*
*
* optional bool is_delete_locked = 9;
* @return Whether the isDeleteLocked field is set.
*/
boolean hasIsDeleteLocked();
/**
*
* Item is delete_locked. Only returned if for_peek is set on the request.
*
*
* optional bool is_delete_locked = 9;
* @return The isDeleteLocked.
*/
boolean getIsDeleteLocked();
}
/**
*
* One item will be returned for each HIT, i.e. where the value was found for
* the requested key. Order is not specified.
*
*
* Protobuf type {@code java.apphosting.MemcacheGetResponse.Item}
*/
public static final class Item extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheGetResponse.Item)
ItemOrBuilder {
private static final long serialVersionUID = 0L;
// Use Item.newBuilder() to construct.
private Item(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Item() {
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Item();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_Item_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_Item_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 2;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes key = 2;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int VALUE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes value = 3;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required bytes value = 3;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
public static final int FLAGS_FIELD_NUMBER = 4;
private int flags_ = 0;
/**
*
* server-opaque (app-owned) flags
*
*
* optional fixed32 flags = 4;
* @return Whether the flags field is set.
*/
@java.lang.Override
public boolean hasFlags() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* server-opaque (app-owned) flags
*
*
* optional fixed32 flags = 4;
* @return The flags.
*/
@java.lang.Override
public int getFlags() {
return flags_;
}
public static final int CAS_ID_FIELD_NUMBER = 5;
private long casId_ = 0L;
/**
*
* The compare-and-swap ID, if requested. Opaque to the client.
* All the client needs to know is that if they do a get request
* with "for_cas" set above, then get a cas_id, and then do a
* SetRequest with policy CAS, passing along the same opaque
* cas_id, it will only succeed if nobody else has replaced the
* value since then. The cas_ids won't be reused between versions
* of the value.
*
*
* optional fixed64 cas_id = 5;
* @return Whether the casId field is set.
*/
@java.lang.Override
public boolean hasCasId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The compare-and-swap ID, if requested. Opaque to the client.
* All the client needs to know is that if they do a get request
* with "for_cas" set above, then get a cas_id, and then do a
* SetRequest with policy CAS, passing along the same opaque
* cas_id, it will only succeed if nobody else has replaced the
* value since then. The cas_ids won't be reused between versions
* of the value.
*
*
* optional fixed64 cas_id = 5;
* @return The casId.
*/
@java.lang.Override
public long getCasId() {
return casId_;
}
public static final int EXPIRES_IN_SECONDS_FIELD_NUMBER = 6;
private int expiresInSeconds_ = 0;
/**
*
* Relative to now. Unset if this item has no expiration timestamp.
*
*
* optional int32 expires_in_seconds = 6;
* @return Whether the expiresInSeconds field is set.
*/
@java.lang.Override
public boolean hasExpiresInSeconds() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Relative to now. Unset if this item has no expiration timestamp.
*
*
* optional int32 expires_in_seconds = 6;
* @return The expiresInSeconds.
*/
@java.lang.Override
public int getExpiresInSeconds() {
return expiresInSeconds_;
}
public static final int TIMESTAMPS_FIELD_NUMBER = 8;
private com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps timestamps_;
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
* @return Whether the timestamps field is set.
*/
@java.lang.Override
public boolean hasTimestamps() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
* @return The timestamps.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps getTimestamps() {
return timestamps_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.getDefaultInstance() : timestamps_;
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestampsOrBuilder getTimestampsOrBuilder() {
return timestamps_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.getDefaultInstance() : timestamps_;
}
public static final int IS_DELETE_LOCKED_FIELD_NUMBER = 9;
private boolean isDeleteLocked_ = false;
/**
*
* Item is delete_locked. Only returned if for_peek is set on the request.
*
*
* optional bool is_delete_locked = 9;
* @return Whether the isDeleteLocked field is set.
*/
@java.lang.Override
public boolean hasIsDeleteLocked() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Item is delete_locked. Only returned if for_peek is set on the request.
*
*
* optional bool is_delete_locked = 9;
* @return The isDeleteLocked.
*/
@java.lang.Override
public boolean getIsDeleteLocked() {
return isDeleteLocked_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(2, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(3, value_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeFixed32(4, flags_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeFixed64(5, casId_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt32(6, expiresInSeconds_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(8, getTimestamps());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBool(9, isDeleteLocked_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, value_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed32Size(4, flags_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed64Size(5, casId_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(6, expiresInSeconds_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getTimestamps());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, isDeleteLocked_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (hasFlags() != other.hasFlags()) return false;
if (hasFlags()) {
if (getFlags()
!= other.getFlags()) return false;
}
if (hasCasId() != other.hasCasId()) return false;
if (hasCasId()) {
if (getCasId()
!= other.getCasId()) return false;
}
if (hasExpiresInSeconds() != other.hasExpiresInSeconds()) return false;
if (hasExpiresInSeconds()) {
if (getExpiresInSeconds()
!= other.getExpiresInSeconds()) return false;
}
if (hasTimestamps() != other.hasTimestamps()) return false;
if (hasTimestamps()) {
if (!getTimestamps()
.equals(other.getTimestamps())) return false;
}
if (hasIsDeleteLocked() != other.hasIsDeleteLocked()) return false;
if (hasIsDeleteLocked()) {
if (getIsDeleteLocked()
!= other.getIsDeleteLocked()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
if (hasFlags()) {
hash = (37 * hash) + FLAGS_FIELD_NUMBER;
hash = (53 * hash) + getFlags();
}
if (hasCasId()) {
hash = (37 * hash) + CAS_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCasId());
}
if (hasExpiresInSeconds()) {
hash = (37 * hash) + EXPIRES_IN_SECONDS_FIELD_NUMBER;
hash = (53 * hash) + getExpiresInSeconds();
}
if (hasTimestamps()) {
hash = (37 * hash) + TIMESTAMPS_FIELD_NUMBER;
hash = (53 * hash) + getTimestamps().hashCode();
}
if (hasIsDeleteLocked()) {
hash = (37 * hash) + IS_DELETE_LOCKED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsDeleteLocked());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* One item will be returned for each HIT, i.e. where the value was found for
* the requested key. Order is not specified.
*
*
* Protobuf type {@code java.apphosting.MemcacheGetResponse.Item}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheGetResponse.Item)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_Item_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_Item_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTimestampsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
flags_ = 0;
casId_ = 0L;
expiresInSeconds_ = 0;
timestamps_ = null;
if (timestampsBuilder_ != null) {
timestampsBuilder_.dispose();
timestampsBuilder_ = null;
}
isDeleteLocked_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_Item_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.flags_ = flags_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.casId_ = casId_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.expiresInSeconds_ = expiresInSeconds_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.timestamps_ = timestampsBuilder_ == null
? timestamps_
: timestampsBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.isDeleteLocked_ = isDeleteLocked_;
to_bitField0_ |= 0x00000040;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasValue()) {
setValue(other.getValue());
}
if (other.hasFlags()) {
setFlags(other.getFlags());
}
if (other.hasCasId()) {
setCasId(other.getCasId());
}
if (other.hasExpiresInSeconds()) {
setExpiresInSeconds(other.getExpiresInSeconds());
}
if (other.hasTimestamps()) {
mergeTimestamps(other.getTimestamps());
}
if (other.hasIsDeleteLocked()) {
setIsDeleteLocked(other.getIsDeleteLocked());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 18
case 26: {
value_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 26
case 37: {
flags_ = input.readFixed32();
bitField0_ |= 0x00000004;
break;
} // case 37
case 41: {
casId_ = input.readFixed64();
bitField0_ |= 0x00000008;
break;
} // case 41
case 48: {
expiresInSeconds_ = input.readInt32();
bitField0_ |= 0x00000010;
break;
} // case 48
case 66: {
input.readMessage(
getTimestampsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 66
case 72: {
isDeleteLocked_ = input.readBool();
bitField0_ |= 0x00000040;
break;
} // case 72
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes key = 2;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required bytes key = 2;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
* required bytes key = 2;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required bytes key = 2;
* @return This builder for chaining.
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes value = 3;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required bytes value = 3;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* required bytes value = 3;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required bytes value = 3;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
private int flags_ ;
/**
*
* server-opaque (app-owned) flags
*
*
* optional fixed32 flags = 4;
* @return Whether the flags field is set.
*/
@java.lang.Override
public boolean hasFlags() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* server-opaque (app-owned) flags
*
*
* optional fixed32 flags = 4;
* @return The flags.
*/
@java.lang.Override
public int getFlags() {
return flags_;
}
/**
*
* server-opaque (app-owned) flags
*
*
* optional fixed32 flags = 4;
* @param value The flags to set.
* @return This builder for chaining.
*/
public Builder setFlags(int value) {
flags_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* server-opaque (app-owned) flags
*
*
* optional fixed32 flags = 4;
* @return This builder for chaining.
*/
public Builder clearFlags() {
bitField0_ = (bitField0_ & ~0x00000004);
flags_ = 0;
onChanged();
return this;
}
private long casId_ ;
/**
*
* The compare-and-swap ID, if requested. Opaque to the client.
* All the client needs to know is that if they do a get request
* with "for_cas" set above, then get a cas_id, and then do a
* SetRequest with policy CAS, passing along the same opaque
* cas_id, it will only succeed if nobody else has replaced the
* value since then. The cas_ids won't be reused between versions
* of the value.
*
*
* optional fixed64 cas_id = 5;
* @return Whether the casId field is set.
*/
@java.lang.Override
public boolean hasCasId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The compare-and-swap ID, if requested. Opaque to the client.
* All the client needs to know is that if they do a get request
* with "for_cas" set above, then get a cas_id, and then do a
* SetRequest with policy CAS, passing along the same opaque
* cas_id, it will only succeed if nobody else has replaced the
* value since then. The cas_ids won't be reused between versions
* of the value.
*
*
* optional fixed64 cas_id = 5;
* @return The casId.
*/
@java.lang.Override
public long getCasId() {
return casId_;
}
/**
*
* The compare-and-swap ID, if requested. Opaque to the client.
* All the client needs to know is that if they do a get request
* with "for_cas" set above, then get a cas_id, and then do a
* SetRequest with policy CAS, passing along the same opaque
* cas_id, it will only succeed if nobody else has replaced the
* value since then. The cas_ids won't be reused between versions
* of the value.
*
*
* optional fixed64 cas_id = 5;
* @param value The casId to set.
* @return This builder for chaining.
*/
public Builder setCasId(long value) {
casId_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The compare-and-swap ID, if requested. Opaque to the client.
* All the client needs to know is that if they do a get request
* with "for_cas" set above, then get a cas_id, and then do a
* SetRequest with policy CAS, passing along the same opaque
* cas_id, it will only succeed if nobody else has replaced the
* value since then. The cas_ids won't be reused between versions
* of the value.
*
*
* optional fixed64 cas_id = 5;
* @return This builder for chaining.
*/
public Builder clearCasId() {
bitField0_ = (bitField0_ & ~0x00000008);
casId_ = 0L;
onChanged();
return this;
}
private int expiresInSeconds_ ;
/**
*
* Relative to now. Unset if this item has no expiration timestamp.
*
*
* optional int32 expires_in_seconds = 6;
* @return Whether the expiresInSeconds field is set.
*/
@java.lang.Override
public boolean hasExpiresInSeconds() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Relative to now. Unset if this item has no expiration timestamp.
*
*
* optional int32 expires_in_seconds = 6;
* @return The expiresInSeconds.
*/
@java.lang.Override
public int getExpiresInSeconds() {
return expiresInSeconds_;
}
/**
*
* Relative to now. Unset if this item has no expiration timestamp.
*
*
* optional int32 expires_in_seconds = 6;
* @param value The expiresInSeconds to set.
* @return This builder for chaining.
*/
public Builder setExpiresInSeconds(int value) {
expiresInSeconds_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Relative to now. Unset if this item has no expiration timestamp.
*
*
* optional int32 expires_in_seconds = 6;
* @return This builder for chaining.
*/
public Builder clearExpiresInSeconds() {
bitField0_ = (bitField0_ & ~0x00000010);
expiresInSeconds_ = 0;
onChanged();
return this;
}
private com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps timestamps_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps, com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.Builder, com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestampsOrBuilder> timestampsBuilder_;
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
* @return Whether the timestamps field is set.
*/
public boolean hasTimestamps() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
* @return The timestamps.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps getTimestamps() {
if (timestampsBuilder_ == null) {
return timestamps_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.getDefaultInstance() : timestamps_;
} else {
return timestampsBuilder_.getMessage();
}
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
public Builder setTimestamps(com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps value) {
if (timestampsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
timestamps_ = value;
} else {
timestampsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
public Builder setTimestamps(
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.Builder builderForValue) {
if (timestampsBuilder_ == null) {
timestamps_ = builderForValue.build();
} else {
timestampsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
public Builder mergeTimestamps(com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps value) {
if (timestampsBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
timestamps_ != null &&
timestamps_ != com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.getDefaultInstance()) {
getTimestampsBuilder().mergeFrom(value);
} else {
timestamps_ = value;
}
} else {
timestampsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
public Builder clearTimestamps() {
bitField0_ = (bitField0_ & ~0x00000020);
timestamps_ = null;
if (timestampsBuilder_ != null) {
timestampsBuilder_.dispose();
timestampsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.Builder getTimestampsBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getTimestampsFieldBuilder().getBuilder();
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestampsOrBuilder getTimestampsOrBuilder() {
if (timestampsBuilder_ != null) {
return timestampsBuilder_.getMessageOrBuilder();
} else {
return timestamps_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.getDefaultInstance() : timestamps_;
}
}
/**
*
* Item timestamps. Only returned if for_peek is set on the request.
*
*
* optional .java.apphosting.ItemTimestamps timestamps = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps, com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.Builder, com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestampsOrBuilder>
getTimestampsFieldBuilder() {
if (timestampsBuilder_ == null) {
timestampsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps, com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestamps.Builder, com.google.appengine.api.memcache.MemcacheServicePb.ItemTimestampsOrBuilder>(
getTimestamps(),
getParentForChildren(),
isClean());
timestamps_ = null;
}
return timestampsBuilder_;
}
private boolean isDeleteLocked_ ;
/**
*
* Item is delete_locked. Only returned if for_peek is set on the request.
*
*
* optional bool is_delete_locked = 9;
* @return Whether the isDeleteLocked field is set.
*/
@java.lang.Override
public boolean hasIsDeleteLocked() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Item is delete_locked. Only returned if for_peek is set on the request.
*
*
* optional bool is_delete_locked = 9;
* @return The isDeleteLocked.
*/
@java.lang.Override
public boolean getIsDeleteLocked() {
return isDeleteLocked_;
}
/**
*
* Item is delete_locked. Only returned if for_peek is set on the request.
*
*
* optional bool is_delete_locked = 9;
* @param value The isDeleteLocked to set.
* @return This builder for chaining.
*/
public Builder setIsDeleteLocked(boolean value) {
isDeleteLocked_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Item is delete_locked. Only returned if for_peek is set on the request.
*
*
* optional bool is_delete_locked = 9;
* @return This builder for chaining.
*/
public Builder clearIsDeleteLocked() {
bitField0_ = (bitField0_ & ~0x00000040);
isDeleteLocked_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheGetResponse.Item)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheGetResponse.Item)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser-
PARSER = new com.google.protobuf.AbstractParser
- () {
@java.lang.Override
public Item parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser
- parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser
- getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int ITEM_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List
item_;
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public java.util.List getItemList() {
return item_;
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder>
getItemOrBuilderList() {
return item_;
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public int getItemCount() {
return item_.size();
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item getItem(int index) {
return item_.get(index);
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder getItemOrBuilder(
int index) {
return item_.get(index);
}
public static final int GET_STATUS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List getStatus_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode> getStatus_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode>() {
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode convert(java.lang.Integer from) {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode.forNumber(from);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode.HIT : result;
}
};
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @return A list containing the getStatus.
*/
@java.lang.Override
public java.util.List getGetStatusList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode>(getStatus_, getStatus_converter_);
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @return The count of getStatus.
*/
@java.lang.Override
public int getGetStatusCount() {
return getStatus_.size();
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @param index The index of the element to return.
* @return The getStatus at the given index.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode getGetStatus(int index) {
return getStatus_converter_.convert(getStatus_.get(index));
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getItemCount(); i++) {
if (!getItem(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < item_.size(); i++) {
output.writeGroup(1, item_.get(i));
}
for (int i = 0; i < getStatus_.size(); i++) {
output.writeEnum(7, getStatus_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < item_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(1, item_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < getStatus_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(getStatus_.get(i));
}
size += dataSize;
size += 1 * getStatus_.size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse) obj;
if (!getItemList()
.equals(other.getItemList())) return false;
if (!getStatus_.equals(other.getStatus_)) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getItemCount() > 0) {
hash = (37 * hash) + ITEM_FIELD_NUMBER;
hash = (53 * hash) + getItemList().hashCode();
}
if (getGetStatusCount() > 0) {
hash = (37 * hash) + GET_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus_.hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Next tag: 10
*
*
* Protobuf type {@code java.apphosting.MemcacheGetResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheGetResponse)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
} else {
item_ = null;
itemBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
getStatus_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheGetResponse_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse result) {
if (itemBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
if (((bitField0_ & 0x00000002) != 0)) {
getStatus_ = java.util.Collections.unmodifiableList(getStatus_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.getStatus_ = getStatus_;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.getDefaultInstance()) return this;
if (itemBuilder_ == null) {
if (!other.item_.isEmpty()) {
if (item_.isEmpty()) {
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureItemIsMutable();
item_.addAll(other.item_);
}
onChanged();
}
} else {
if (!other.item_.isEmpty()) {
if (itemBuilder_.isEmpty()) {
itemBuilder_.dispose();
itemBuilder_ = null;
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000001);
itemBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getItemFieldBuilder() : null;
} else {
itemBuilder_.addAllMessages(other.item_);
}
}
}
if (!other.getStatus_.isEmpty()) {
if (getStatus_.isEmpty()) {
getStatus_ = other.getStatus_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureGetStatusIsMutable();
getStatus_.addAll(other.getStatus_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getItemCount(); i++) {
if (!getItem(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 11: {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item m =
input.readGroup(1,
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.PARSER,
extensionRegistry);
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(m);
} else {
itemBuilder_.addMessage(m);
}
break;
} // case 11
case 56: {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(7, tmpRaw);
} else {
ensureGetStatusIsMutable();
getStatus_.add(tmpRaw);
}
break;
} // case 56
case 58: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(7, tmpRaw);
} else {
ensureGetStatusIsMutable();
getStatus_.add(tmpRaw);
}
}
input.popLimit(oldLimit);
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List item_ =
java.util.Collections.emptyList();
private void ensureItemIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
item_ = new java.util.ArrayList(item_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder> itemBuilder_;
/**
* repeated group Item = 1 { ... }
*/
public java.util.List getItemList() {
if (itemBuilder_ == null) {
return java.util.Collections.unmodifiableList(item_);
} else {
return itemBuilder_.getMessageList();
}
}
/**
* repeated group Item = 1 { ... }
*/
public int getItemCount() {
if (itemBuilder_ == null) {
return item_.size();
} else {
return itemBuilder_.getCount();
}
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item getItem(int index) {
if (itemBuilder_ == null) {
return item_.get(index);
} else {
return itemBuilder_.getMessage(index);
}
}
/**
* repeated group Item = 1 { ... }
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.set(index, value);
onChanged();
} else {
itemBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.set(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(value);
onChanged();
} else {
itemBuilder_.addMessage(value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(index, value);
onChanged();
} else {
itemBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addAllItem(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item> values) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, item_);
onChanged();
} else {
itemBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
itemBuilder_.clear();
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder removeItem(int index) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.remove(index);
onChanged();
} else {
itemBuilder_.remove(index);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder getItemBuilder(
int index) {
return getItemFieldBuilder().getBuilder(index);
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder getItemOrBuilder(
int index) {
if (itemBuilder_ == null) {
return item_.get(index); } else {
return itemBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated group Item = 1 { ... }
*/
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder>
getItemOrBuilderList() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(item_);
}
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder addItemBuilder() {
return getItemFieldBuilder().addBuilder(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.getDefaultInstance());
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder addItemBuilder(
int index) {
return getItemFieldBuilder().addBuilder(
index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.getDefaultInstance());
}
/**
* repeated group Item = 1 { ... }
*/
public java.util.List
getItemBuilderList() {
return getItemFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.ItemOrBuilder>(
item_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
private java.util.List getStatus_ =
java.util.Collections.emptyList();
private void ensureGetStatusIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
getStatus_ = new java.util.ArrayList(getStatus_);
bitField0_ |= 0x00000002;
}
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @return A list containing the getStatus.
*/
public java.util.List getGetStatusList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode>(getStatus_, getStatus_converter_);
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @return The count of getStatus.
*/
public int getGetStatusCount() {
return getStatus_.size();
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @param index The index of the element to return.
* @return The getStatus at the given index.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode getGetStatus(int index) {
return getStatus_converter_.convert(getStatus_.get(index));
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @param index The index to set the value at.
* @param value The getStatus to set.
* @return This builder for chaining.
*/
public Builder setGetStatus(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode value) {
if (value == null) {
throw new NullPointerException();
}
ensureGetStatusIsMutable();
getStatus_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @param value The getStatus to add.
* @return This builder for chaining.
*/
public Builder addGetStatus(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode value) {
if (value == null) {
throw new NullPointerException();
}
ensureGetStatusIsMutable();
getStatus_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @param values The getStatus to add.
* @return This builder for chaining.
*/
public Builder addAllGetStatus(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode> values) {
ensureGetStatusIsMutable();
for (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse.GetStatusCode value : values) {
getStatus_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* One get_status will be returned for each requested key, in the same order
* that the requests were in.
* TODO(b/290098535) This can return the wrong status code when there's a mix
* of hit/miss.
*
*
* repeated .java.apphosting.MemcacheGetResponse.GetStatusCode get_status = 7;
* @return This builder for chaining.
*/
public Builder clearGetStatus() {
getStatus_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheGetResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheGetResponse)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheGetResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheGetResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheSetRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheSetRequest)
com.google.protobuf.MessageOrBuilder {
/**
* repeated group Item = 1 { ... }
*/
java.util.List
getItemList();
/**
* repeated group Item = 1 { ... }
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item getItem(int index);
/**
* repeated group Item = 1 { ... }
*/
int getItemCount();
/**
* repeated group Item = 1 { ... }
*/
java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder>
getItemOrBuilderList();
/**
* repeated group Item = 1 { ... }
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder getItemOrBuilder(
int index);
/**
* optional string name_space = 7 [default = ""];
* @return Whether the nameSpace field is set.
*/
boolean hasNameSpace();
/**
* optional string name_space = 7 [default = ""];
* @return The nameSpace.
*/
java.lang.String getNameSpace();
/**
* optional string name_space = 7 [default = ""];
* @return The bytes for nameSpace.
*/
com.google.protobuf.ByteString
getNameSpaceBytes();
/**
* optional .java.apphosting.AppOverride override = 10;
* @return Whether the override field is set.
*/
boolean hasOverride();
/**
* optional .java.apphosting.AppOverride override = 10;
* @return The override.
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride();
/**
* optional .java.apphosting.AppOverride override = 10;
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder();
}
/**
* Protobuf type {@code java.apphosting.MemcacheSetRequest}
*/
public static final class MemcacheSetRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheSetRequest)
MemcacheSetRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheSetRequest.newBuilder() to construct.
private MemcacheSetRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheSetRequest() {
item_ = java.util.Collections.emptyList();
nameSpace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheSetRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.MemcacheSetRequest.SetPolicy}
*/
public enum SetPolicy
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* set value in memcached, unconditionally
*
*
* SET = 1;
*/
SET(1),
/**
*
* add to memcached, if it doesn't already exist
*
*
* ADD = 2;
*/
ADD(2),
/**
*
* put it in memcached, but only if it's already in there
*
*
* REPLACE = 3;
*/
REPLACE(3),
/**
*
* Compare-and-swap. If using this policy, the 'cas_id' optional
* field is required, else NOT_STORED will be returned. This
* policy is like REPLACE, but a smart replace that only updates
* the value if nobody else has between your Get and your Set.
* See the docs in MemcacheGetRequest and MemcacheGetResponse.
*
*
* CAS = 4;
*/
CAS(4),
;
/**
*
* set value in memcached, unconditionally
*
*
* SET = 1;
*/
public static final int SET_VALUE = 1;
/**
*
* add to memcached, if it doesn't already exist
*
*
* ADD = 2;
*/
public static final int ADD_VALUE = 2;
/**
*
* put it in memcached, but only if it's already in there
*
*
* REPLACE = 3;
*/
public static final int REPLACE_VALUE = 3;
/**
*
* Compare-and-swap. If using this policy, the 'cas_id' optional
* field is required, else NOT_STORED will be returned. This
* policy is like REPLACE, but a smart replace that only updates
* the value if nobody else has between your Get and your Set.
* See the docs in MemcacheGetRequest and MemcacheGetResponse.
*
*
* CAS = 4;
*/
public static final int CAS_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SetPolicy valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static SetPolicy forNumber(int value) {
switch (value) {
case 1: return SET;
case 2: return ADD;
case 3: return REPLACE;
case 4: return CAS;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
SetPolicy> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SetPolicy findValueByNumber(int number) {
return SetPolicy.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.getDescriptor().getEnumTypes().get(0);
}
private static final SetPolicy[] VALUES = values();
public static SetPolicy valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private SetPolicy(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.MemcacheSetRequest.SetPolicy)
}
public interface ItemOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheSetRequest.Item)
com.google.protobuf.MessageOrBuilder {
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
* required bytes value = 3;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* required bytes value = 3;
* @return The value.
*/
com.google.protobuf.ByteString getValue();
/**
*
* From the docs above:
* <flags> is an arbitrary 32-bit unsigned integer that the server
* stores along with the data and sends back when the item is
* retrieved. Clients may use this as a bit field to store
* data-specific information; this field is opaque to the server.
*
*
* optional fixed32 flags = 4;
* @return Whether the flags field is set.
*/
boolean hasFlags();
/**
*
* From the docs above:
* <flags> is an arbitrary 32-bit unsigned integer that the server
* stores along with the data and sends back when the item is
* retrieved. Clients may use this as a bit field to store
* data-specific information; this field is opaque to the server.
*
*
* optional fixed32 flags = 4;
* @return The flags.
*/
int getFlags();
/**
* optional .java.apphosting.MemcacheSetRequest.SetPolicy set_policy = 5 [default = SET];
* @return Whether the setPolicy field is set.
*/
boolean hasSetPolicy();
/**
* optional .java.apphosting.MemcacheSetRequest.SetPolicy set_policy = 5 [default = SET];
* @return The setPolicy.
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy getSetPolicy();
/**
*
* unixtime to expire key. 0 or unset means "no expiration"
* From the memcached documentation:
* <exptime> is expiration time. If it's 0, the item never expires
* (although it may be deleted from the cache to make place for
* other items). If it's non-zero (either Unix time or offset in
* seconds from current time), it is guaranteed that clients will
* not be able to retrieve this item after the expiration time
* arrives (measured by server time).
*
*
* optional fixed32 expiration_time = 6 [default = 0];
* @return Whether the expirationTime field is set.
*/
boolean hasExpirationTime();
/**
*
* unixtime to expire key. 0 or unset means "no expiration"
* From the memcached documentation:
* <exptime> is expiration time. If it's 0, the item never expires
* (although it may be deleted from the cache to make place for
* other items). If it's non-zero (either Unix time or offset in
* seconds from current time), it is guaranteed that clients will
* not be able to retrieve this item after the expiration time
* arrives (measured by server time).
*
*
* optional fixed32 expiration_time = 6 [default = 0];
* @return The expirationTime.
*/
int getExpirationTime();
/**
*
* Required if using the CAS (compare & swap) set_policy. This is
* the cas_id as returned in MemcacheGetResponse, requested in
* MemacheGetResponse. The value, opaque to the client, is the handle
* the guarantees that nobody modified the value from the time you
* requested it until the time you replaced it with CAS. Holding a
* a cas_id is not a lock though: anybody can beat you to the CAS.
* If you lose the race, you'll get the status code EXISTS returned.
* See docs in MemcacheSetResponse.
*
*
* optional fixed64 cas_id = 8;
* @return Whether the casId field is set.
*/
boolean hasCasId();
/**
*
* Required if using the CAS (compare & swap) set_policy. This is
* the cas_id as returned in MemcacheGetResponse, requested in
* MemacheGetResponse. The value, opaque to the client, is the handle
* the guarantees that nobody modified the value from the time you
* requested it until the time you replaced it with CAS. Holding a
* a cas_id is not a lock though: anybody can beat you to the CAS.
* If you lose the race, you'll get the status code EXISTS returned.
* See docs in MemcacheSetResponse.
*
*
* optional fixed64 cas_id = 8;
* @return The casId.
*/
long getCasId();
/**
*
* Optionally set this when doing a SET or ADD if you plan to do a
* CAS operation on this item in the future, which will cause the
* item's internal cas_id to be allocated early, rather than the
* server upgrading it to include a cas_id in the future when you
* do a Get. You may still do a CAS operation in the future on
* any key without setting this. This is purely an optimization
* hint to the server.
*
*
* optional bool for_cas = 9;
* @return Whether the forCas field is set.
*/
boolean hasForCas();
/**
*
* Optionally set this when doing a SET or ADD if you plan to do a
* CAS operation on this item in the future, which will cause the
* item's internal cas_id to be allocated early, rather than the
* server upgrading it to include a cas_id in the future when you
* do a Get. You may still do a CAS operation in the future on
* any key without setting this. This is purely an optimization
* hint to the server.
*
*
* optional bool for_cas = 9;
* @return The forCas.
*/
boolean getForCas();
}
/**
* Protobuf type {@code java.apphosting.MemcacheSetRequest.Item}
*/
public static final class Item extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheSetRequest.Item)
ItemOrBuilder {
private static final long serialVersionUID = 0L;
// Use Item.newBuilder() to construct.
private Item(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Item() {
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
setPolicy_ = 1;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Item();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_Item_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_Item_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int VALUE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes value = 3;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required bytes value = 3;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
public static final int FLAGS_FIELD_NUMBER = 4;
private int flags_ = 0;
/**
*
* From the docs above:
* <flags> is an arbitrary 32-bit unsigned integer that the server
* stores along with the data and sends back when the item is
* retrieved. Clients may use this as a bit field to store
* data-specific information; this field is opaque to the server.
*
*
* optional fixed32 flags = 4;
* @return Whether the flags field is set.
*/
@java.lang.Override
public boolean hasFlags() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* From the docs above:
* <flags> is an arbitrary 32-bit unsigned integer that the server
* stores along with the data and sends back when the item is
* retrieved. Clients may use this as a bit field to store
* data-specific information; this field is opaque to the server.
*
*
* optional fixed32 flags = 4;
* @return The flags.
*/
@java.lang.Override
public int getFlags() {
return flags_;
}
public static final int SET_POLICY_FIELD_NUMBER = 5;
private int setPolicy_ = 1;
/**
* optional .java.apphosting.MemcacheSetRequest.SetPolicy set_policy = 5 [default = SET];
* @return Whether the setPolicy field is set.
*/
@java.lang.Override public boolean hasSetPolicy() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional .java.apphosting.MemcacheSetRequest.SetPolicy set_policy = 5 [default = SET];
* @return The setPolicy.
*/
@java.lang.Override public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy getSetPolicy() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy.forNumber(setPolicy_);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy.SET : result;
}
public static final int EXPIRATION_TIME_FIELD_NUMBER = 6;
private int expirationTime_ = 0;
/**
*
* unixtime to expire key. 0 or unset means "no expiration"
* From the memcached documentation:
* <exptime> is expiration time. If it's 0, the item never expires
* (although it may be deleted from the cache to make place for
* other items). If it's non-zero (either Unix time or offset in
* seconds from current time), it is guaranteed that clients will
* not be able to retrieve this item after the expiration time
* arrives (measured by server time).
*
*
* optional fixed32 expiration_time = 6 [default = 0];
* @return Whether the expirationTime field is set.
*/
@java.lang.Override
public boolean hasExpirationTime() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* unixtime to expire key. 0 or unset means "no expiration"
* From the memcached documentation:
* <exptime> is expiration time. If it's 0, the item never expires
* (although it may be deleted from the cache to make place for
* other items). If it's non-zero (either Unix time or offset in
* seconds from current time), it is guaranteed that clients will
* not be able to retrieve this item after the expiration time
* arrives (measured by server time).
*
*
* optional fixed32 expiration_time = 6 [default = 0];
* @return The expirationTime.
*/
@java.lang.Override
public int getExpirationTime() {
return expirationTime_;
}
public static final int CAS_ID_FIELD_NUMBER = 8;
private long casId_ = 0L;
/**
*
* Required if using the CAS (compare & swap) set_policy. This is
* the cas_id as returned in MemcacheGetResponse, requested in
* MemacheGetResponse. The value, opaque to the client, is the handle
* the guarantees that nobody modified the value from the time you
* requested it until the time you replaced it with CAS. Holding a
* a cas_id is not a lock though: anybody can beat you to the CAS.
* If you lose the race, you'll get the status code EXISTS returned.
* See docs in MemcacheSetResponse.
*
*
* optional fixed64 cas_id = 8;
* @return Whether the casId field is set.
*/
@java.lang.Override
public boolean hasCasId() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Required if using the CAS (compare & swap) set_policy. This is
* the cas_id as returned in MemcacheGetResponse, requested in
* MemacheGetResponse. The value, opaque to the client, is the handle
* the guarantees that nobody modified the value from the time you
* requested it until the time you replaced it with CAS. Holding a
* a cas_id is not a lock though: anybody can beat you to the CAS.
* If you lose the race, you'll get the status code EXISTS returned.
* See docs in MemcacheSetResponse.
*
*
* optional fixed64 cas_id = 8;
* @return The casId.
*/
@java.lang.Override
public long getCasId() {
return casId_;
}
public static final int FOR_CAS_FIELD_NUMBER = 9;
private boolean forCas_ = false;
/**
*
* Optionally set this when doing a SET or ADD if you plan to do a
* CAS operation on this item in the future, which will cause the
* item's internal cas_id to be allocated early, rather than the
* server upgrading it to include a cas_id in the future when you
* do a Get. You may still do a CAS operation in the future on
* any key without setting this. This is purely an optimization
* hint to the server.
*
*
* optional bool for_cas = 9;
* @return Whether the forCas field is set.
*/
@java.lang.Override
public boolean hasForCas() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Optionally set this when doing a SET or ADD if you plan to do a
* CAS operation on this item in the future, which will cause the
* item's internal cas_id to be allocated early, rather than the
* server upgrading it to include a cas_id in the future when you
* do a Get. You may still do a CAS operation in the future on
* any key without setting this. This is purely an optimization
* hint to the server.
*
*
* optional bool for_cas = 9;
* @return The forCas.
*/
@java.lang.Override
public boolean getForCas() {
return forCas_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(2, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(3, value_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeFixed32(4, flags_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeEnum(5, setPolicy_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeFixed32(6, expirationTime_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeFixed64(8, casId_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBool(9, forCas_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, value_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed32Size(4, flags_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, setPolicy_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed32Size(6, expirationTime_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed64Size(8, casId_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, forCas_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (hasFlags() != other.hasFlags()) return false;
if (hasFlags()) {
if (getFlags()
!= other.getFlags()) return false;
}
if (hasSetPolicy() != other.hasSetPolicy()) return false;
if (hasSetPolicy()) {
if (setPolicy_ != other.setPolicy_) return false;
}
if (hasExpirationTime() != other.hasExpirationTime()) return false;
if (hasExpirationTime()) {
if (getExpirationTime()
!= other.getExpirationTime()) return false;
}
if (hasCasId() != other.hasCasId()) return false;
if (hasCasId()) {
if (getCasId()
!= other.getCasId()) return false;
}
if (hasForCas() != other.hasForCas()) return false;
if (hasForCas()) {
if (getForCas()
!= other.getForCas()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
if (hasFlags()) {
hash = (37 * hash) + FLAGS_FIELD_NUMBER;
hash = (53 * hash) + getFlags();
}
if (hasSetPolicy()) {
hash = (37 * hash) + SET_POLICY_FIELD_NUMBER;
hash = (53 * hash) + setPolicy_;
}
if (hasExpirationTime()) {
hash = (37 * hash) + EXPIRATION_TIME_FIELD_NUMBER;
hash = (53 * hash) + getExpirationTime();
}
if (hasCasId()) {
hash = (37 * hash) + CAS_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCasId());
}
if (hasForCas()) {
hash = (37 * hash) + FOR_CAS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getForCas());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheSetRequest.Item}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheSetRequest.Item)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_Item_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_Item_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = com.google.protobuf.ByteString.EMPTY;
value_ = com.google.protobuf.ByteString.EMPTY;
flags_ = 0;
setPolicy_ = 1;
expirationTime_ = 0;
casId_ = 0L;
forCas_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_Item_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.flags_ = flags_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.setPolicy_ = setPolicy_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.expirationTime_ = expirationTime_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.casId_ = casId_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.forCas_ = forCas_;
to_bitField0_ |= 0x00000040;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasValue()) {
setValue(other.getValue());
}
if (other.hasFlags()) {
setFlags(other.getFlags());
}
if (other.hasSetPolicy()) {
setSetPolicy(other.getSetPolicy());
}
if (other.hasExpirationTime()) {
setExpirationTime(other.getExpirationTime());
}
if (other.hasCasId()) {
setCasId(other.getCasId());
}
if (other.hasForCas()) {
setForCas(other.getForCas());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 18
case 26: {
value_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 26
case 37: {
flags_ = input.readFixed32();
bitField0_ |= 0x00000004;
break;
} // case 37
case 40: {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(5, tmpRaw);
} else {
setPolicy_ = tmpRaw;
bitField0_ |= 0x00000008;
}
break;
} // case 40
case 53: {
expirationTime_ = input.readFixed32();
bitField0_ |= 0x00000010;
break;
} // case 53
case 65: {
casId_ = input.readFixed64();
bitField0_ |= 0x00000020;
break;
} // case 65
case 72: {
forCas_ = input.readBool();
bitField0_ |= 0x00000040;
break;
} // case 72
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return This builder for chaining.
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private com.google.protobuf.ByteString value_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes value = 3;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required bytes value = 3;
* @return The value.
*/
@java.lang.Override
public com.google.protobuf.ByteString getValue() {
return value_;
}
/**
* required bytes value = 3;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required bytes value = 3;
* @return This builder for chaining.
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
private int flags_ ;
/**
*
* From the docs above:
* <flags> is an arbitrary 32-bit unsigned integer that the server
* stores along with the data and sends back when the item is
* retrieved. Clients may use this as a bit field to store
* data-specific information; this field is opaque to the server.
*
*
* optional fixed32 flags = 4;
* @return Whether the flags field is set.
*/
@java.lang.Override
public boolean hasFlags() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* From the docs above:
* <flags> is an arbitrary 32-bit unsigned integer that the server
* stores along with the data and sends back when the item is
* retrieved. Clients may use this as a bit field to store
* data-specific information; this field is opaque to the server.
*
*
* optional fixed32 flags = 4;
* @return The flags.
*/
@java.lang.Override
public int getFlags() {
return flags_;
}
/**
*
* From the docs above:
* <flags> is an arbitrary 32-bit unsigned integer that the server
* stores along with the data and sends back when the item is
* retrieved. Clients may use this as a bit field to store
* data-specific information; this field is opaque to the server.
*
*
* optional fixed32 flags = 4;
* @param value The flags to set.
* @return This builder for chaining.
*/
public Builder setFlags(int value) {
flags_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* From the docs above:
* <flags> is an arbitrary 32-bit unsigned integer that the server
* stores along with the data and sends back when the item is
* retrieved. Clients may use this as a bit field to store
* data-specific information; this field is opaque to the server.
*
*
* optional fixed32 flags = 4;
* @return This builder for chaining.
*/
public Builder clearFlags() {
bitField0_ = (bitField0_ & ~0x00000004);
flags_ = 0;
onChanged();
return this;
}
private int setPolicy_ = 1;
/**
* optional .java.apphosting.MemcacheSetRequest.SetPolicy set_policy = 5 [default = SET];
* @return Whether the setPolicy field is set.
*/
@java.lang.Override public boolean hasSetPolicy() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional .java.apphosting.MemcacheSetRequest.SetPolicy set_policy = 5 [default = SET];
* @return The setPolicy.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy getSetPolicy() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy.forNumber(setPolicy_);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy.SET : result;
}
/**
* optional .java.apphosting.MemcacheSetRequest.SetPolicy set_policy = 5 [default = SET];
* @param value The setPolicy to set.
* @return This builder for chaining.
*/
public Builder setSetPolicy(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.SetPolicy value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
setPolicy_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.MemcacheSetRequest.SetPolicy set_policy = 5 [default = SET];
* @return This builder for chaining.
*/
public Builder clearSetPolicy() {
bitField0_ = (bitField0_ & ~0x00000008);
setPolicy_ = 1;
onChanged();
return this;
}
private int expirationTime_ ;
/**
*
* unixtime to expire key. 0 or unset means "no expiration"
* From the memcached documentation:
* <exptime> is expiration time. If it's 0, the item never expires
* (although it may be deleted from the cache to make place for
* other items). If it's non-zero (either Unix time or offset in
* seconds from current time), it is guaranteed that clients will
* not be able to retrieve this item after the expiration time
* arrives (measured by server time).
*
*
* optional fixed32 expiration_time = 6 [default = 0];
* @return Whether the expirationTime field is set.
*/
@java.lang.Override
public boolean hasExpirationTime() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* unixtime to expire key. 0 or unset means "no expiration"
* From the memcached documentation:
* <exptime> is expiration time. If it's 0, the item never expires
* (although it may be deleted from the cache to make place for
* other items). If it's non-zero (either Unix time or offset in
* seconds from current time), it is guaranteed that clients will
* not be able to retrieve this item after the expiration time
* arrives (measured by server time).
*
*
* optional fixed32 expiration_time = 6 [default = 0];
* @return The expirationTime.
*/
@java.lang.Override
public int getExpirationTime() {
return expirationTime_;
}
/**
*
* unixtime to expire key. 0 or unset means "no expiration"
* From the memcached documentation:
* <exptime> is expiration time. If it's 0, the item never expires
* (although it may be deleted from the cache to make place for
* other items). If it's non-zero (either Unix time or offset in
* seconds from current time), it is guaranteed that clients will
* not be able to retrieve this item after the expiration time
* arrives (measured by server time).
*
*
* optional fixed32 expiration_time = 6 [default = 0];
* @param value The expirationTime to set.
* @return This builder for chaining.
*/
public Builder setExpirationTime(int value) {
expirationTime_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* unixtime to expire key. 0 or unset means "no expiration"
* From the memcached documentation:
* <exptime> is expiration time. If it's 0, the item never expires
* (although it may be deleted from the cache to make place for
* other items). If it's non-zero (either Unix time or offset in
* seconds from current time), it is guaranteed that clients will
* not be able to retrieve this item after the expiration time
* arrives (measured by server time).
*
*
* optional fixed32 expiration_time = 6 [default = 0];
* @return This builder for chaining.
*/
public Builder clearExpirationTime() {
bitField0_ = (bitField0_ & ~0x00000010);
expirationTime_ = 0;
onChanged();
return this;
}
private long casId_ ;
/**
*
* Required if using the CAS (compare & swap) set_policy. This is
* the cas_id as returned in MemcacheGetResponse, requested in
* MemacheGetResponse. The value, opaque to the client, is the handle
* the guarantees that nobody modified the value from the time you
* requested it until the time you replaced it with CAS. Holding a
* a cas_id is not a lock though: anybody can beat you to the CAS.
* If you lose the race, you'll get the status code EXISTS returned.
* See docs in MemcacheSetResponse.
*
*
* optional fixed64 cas_id = 8;
* @return Whether the casId field is set.
*/
@java.lang.Override
public boolean hasCasId() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Required if using the CAS (compare & swap) set_policy. This is
* the cas_id as returned in MemcacheGetResponse, requested in
* MemacheGetResponse. The value, opaque to the client, is the handle
* the guarantees that nobody modified the value from the time you
* requested it until the time you replaced it with CAS. Holding a
* a cas_id is not a lock though: anybody can beat you to the CAS.
* If you lose the race, you'll get the status code EXISTS returned.
* See docs in MemcacheSetResponse.
*
*
* optional fixed64 cas_id = 8;
* @return The casId.
*/
@java.lang.Override
public long getCasId() {
return casId_;
}
/**
*
* Required if using the CAS (compare & swap) set_policy. This is
* the cas_id as returned in MemcacheGetResponse, requested in
* MemacheGetResponse. The value, opaque to the client, is the handle
* the guarantees that nobody modified the value from the time you
* requested it until the time you replaced it with CAS. Holding a
* a cas_id is not a lock though: anybody can beat you to the CAS.
* If you lose the race, you'll get the status code EXISTS returned.
* See docs in MemcacheSetResponse.
*
*
* optional fixed64 cas_id = 8;
* @param value The casId to set.
* @return This builder for chaining.
*/
public Builder setCasId(long value) {
casId_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Required if using the CAS (compare & swap) set_policy. This is
* the cas_id as returned in MemcacheGetResponse, requested in
* MemacheGetResponse. The value, opaque to the client, is the handle
* the guarantees that nobody modified the value from the time you
* requested it until the time you replaced it with CAS. Holding a
* a cas_id is not a lock though: anybody can beat you to the CAS.
* If you lose the race, you'll get the status code EXISTS returned.
* See docs in MemcacheSetResponse.
*
*
* optional fixed64 cas_id = 8;
* @return This builder for chaining.
*/
public Builder clearCasId() {
bitField0_ = (bitField0_ & ~0x00000020);
casId_ = 0L;
onChanged();
return this;
}
private boolean forCas_ ;
/**
*
* Optionally set this when doing a SET or ADD if you plan to do a
* CAS operation on this item in the future, which will cause the
* item's internal cas_id to be allocated early, rather than the
* server upgrading it to include a cas_id in the future when you
* do a Get. You may still do a CAS operation in the future on
* any key without setting this. This is purely an optimization
* hint to the server.
*
*
* optional bool for_cas = 9;
* @return Whether the forCas field is set.
*/
@java.lang.Override
public boolean hasForCas() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Optionally set this when doing a SET or ADD if you plan to do a
* CAS operation on this item in the future, which will cause the
* item's internal cas_id to be allocated early, rather than the
* server upgrading it to include a cas_id in the future when you
* do a Get. You may still do a CAS operation in the future on
* any key without setting this. This is purely an optimization
* hint to the server.
*
*
* optional bool for_cas = 9;
* @return The forCas.
*/
@java.lang.Override
public boolean getForCas() {
return forCas_;
}
/**
*
* Optionally set this when doing a SET or ADD if you plan to do a
* CAS operation on this item in the future, which will cause the
* item's internal cas_id to be allocated early, rather than the
* server upgrading it to include a cas_id in the future when you
* do a Get. You may still do a CAS operation in the future on
* any key without setting this. This is purely an optimization
* hint to the server.
*
*
* optional bool for_cas = 9;
* @param value The forCas to set.
* @return This builder for chaining.
*/
public Builder setForCas(boolean value) {
forCas_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Optionally set this when doing a SET or ADD if you plan to do a
* CAS operation on this item in the future, which will cause the
* item's internal cas_id to be allocated early, rather than the
* server upgrading it to include a cas_id in the future when you
* do a Get. You may still do a CAS operation in the future on
* any key without setting this. This is purely an optimization
* hint to the server.
*
*
* optional bool for_cas = 9;
* @return This builder for chaining.
*/
public Builder clearForCas() {
bitField0_ = (bitField0_ & ~0x00000040);
forCas_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheSetRequest.Item)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheSetRequest.Item)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser-
PARSER = new com.google.protobuf.AbstractParser
- () {
@java.lang.Override
public Item parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser
- parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser
- getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int ITEM_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List
item_;
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public java.util.List getItemList() {
return item_;
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder>
getItemOrBuilderList() {
return item_;
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public int getItemCount() {
return item_.size();
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item getItem(int index) {
return item_.get(index);
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder getItemOrBuilder(
int index) {
return item_.get(index);
}
public static final int NAME_SPACE_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 7 [default = ""];
* @return Whether the nameSpace field is set.
*/
@java.lang.Override
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name_space = 7 [default = ""];
* @return The nameSpace.
*/
@java.lang.Override
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
}
}
/**
* optional string name_space = 7 [default = ""];
* @return The bytes for nameSpace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OVERRIDE_FIELD_NUMBER = 10;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
/**
* optional .java.apphosting.AppOverride override = 10;
* @return Whether the override field is set.
*/
@java.lang.Override
public boolean hasOverride() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 10;
* @return The override.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
/**
* optional .java.apphosting.AppOverride override = 10;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getItemCount(); i++) {
if (!getItem(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasOverride()) {
if (!getOverride().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < item_.size(); i++) {
output.writeGroup(1, item_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, nameSpace_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(10, getOverride());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < item_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(1, item_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, nameSpace_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getOverride());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest) obj;
if (!getItemList()
.equals(other.getItemList())) return false;
if (hasNameSpace() != other.hasNameSpace()) return false;
if (hasNameSpace()) {
if (!getNameSpace()
.equals(other.getNameSpace())) return false;
}
if (hasOverride() != other.hasOverride()) return false;
if (hasOverride()) {
if (!getOverride()
.equals(other.getOverride())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getItemCount() > 0) {
hash = (37 * hash) + ITEM_FIELD_NUMBER;
hash = (53 * hash) + getItemList().hashCode();
}
if (hasNameSpace()) {
hash = (37 * hash) + NAME_SPACE_FIELD_NUMBER;
hash = (53 * hash) + getNameSpace().hashCode();
}
if (hasOverride()) {
hash = (37 * hash) + OVERRIDE_FIELD_NUMBER;
hash = (53 * hash) + getOverride().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheSetRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheSetRequest)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getItemFieldBuilder();
getOverrideFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
} else {
item_ = null;
itemBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
nameSpace_ = "";
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetRequest_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest result) {
if (itemBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.nameSpace_ = nameSpace_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.override_ = overrideBuilder_ == null
? override_
: overrideBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.getDefaultInstance()) return this;
if (itemBuilder_ == null) {
if (!other.item_.isEmpty()) {
if (item_.isEmpty()) {
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureItemIsMutable();
item_.addAll(other.item_);
}
onChanged();
}
} else {
if (!other.item_.isEmpty()) {
if (itemBuilder_.isEmpty()) {
itemBuilder_.dispose();
itemBuilder_ = null;
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000001);
itemBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getItemFieldBuilder() : null;
} else {
itemBuilder_.addAllMessages(other.item_);
}
}
}
if (other.hasNameSpace()) {
nameSpace_ = other.nameSpace_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasOverride()) {
mergeOverride(other.getOverride());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getItemCount(); i++) {
if (!getItem(i).isInitialized()) {
return false;
}
}
if (hasOverride()) {
if (!getOverride().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 11: {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item m =
input.readGroup(1,
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.PARSER,
extensionRegistry);
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(m);
} else {
itemBuilder_.addMessage(m);
}
break;
} // case 11
case 58: {
nameSpace_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 58
case 82: {
input.readMessage(
getOverrideFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 82
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List item_ =
java.util.Collections.emptyList();
private void ensureItemIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
item_ = new java.util.ArrayList(item_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder> itemBuilder_;
/**
* repeated group Item = 1 { ... }
*/
public java.util.List getItemList() {
if (itemBuilder_ == null) {
return java.util.Collections.unmodifiableList(item_);
} else {
return itemBuilder_.getMessageList();
}
}
/**
* repeated group Item = 1 { ... }
*/
public int getItemCount() {
if (itemBuilder_ == null) {
return item_.size();
} else {
return itemBuilder_.getCount();
}
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item getItem(int index) {
if (itemBuilder_ == null) {
return item_.get(index);
} else {
return itemBuilder_.getMessage(index);
}
}
/**
* repeated group Item = 1 { ... }
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.set(index, value);
onChanged();
} else {
itemBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.set(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(value);
onChanged();
} else {
itemBuilder_.addMessage(value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(index, value);
onChanged();
} else {
itemBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addAllItem(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item> values) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, item_);
onChanged();
} else {
itemBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
itemBuilder_.clear();
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder removeItem(int index) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.remove(index);
onChanged();
} else {
itemBuilder_.remove(index);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder getItemBuilder(
int index) {
return getItemFieldBuilder().getBuilder(index);
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder getItemOrBuilder(
int index) {
if (itemBuilder_ == null) {
return item_.get(index); } else {
return itemBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated group Item = 1 { ... }
*/
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder>
getItemOrBuilderList() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(item_);
}
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder addItemBuilder() {
return getItemFieldBuilder().addBuilder(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.getDefaultInstance());
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder addItemBuilder(
int index) {
return getItemFieldBuilder().addBuilder(
index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.getDefaultInstance());
}
/**
* repeated group Item = 1 { ... }
*/
public java.util.List
getItemBuilderList() {
return getItemFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest.ItemOrBuilder>(
item_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
private java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 7 [default = ""];
* @return Whether the nameSpace field is set.
*/
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string name_space = 7 [default = ""];
* @return The nameSpace.
*/
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name_space = 7 [default = ""];
* @return The bytes for nameSpace.
*/
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name_space = 7 [default = ""];
* @param value The nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpace(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional string name_space = 7 [default = ""];
* @return This builder for chaining.
*/
public Builder clearNameSpace() {
nameSpace_ = getDefaultInstance().getNameSpace();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* optional string name_space = 7 [default = ""];
* @param value The bytes for nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder> overrideBuilder_;
/**
* optional .java.apphosting.AppOverride override = 10;
* @return Whether the override field is set.
*/
public boolean hasOverride() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 10;
* @return The override.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
if (overrideBuilder_ == null) {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
} else {
return overrideBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.AppOverride override = 10;
*/
public Builder setOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
override_ = value;
} else {
overrideBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 10;
*/
public Builder setOverride(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder builderForValue) {
if (overrideBuilder_ == null) {
override_ = builderForValue.build();
} else {
overrideBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 10;
*/
public Builder mergeOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
override_ != null &&
override_ != com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance()) {
getOverrideBuilder().mergeFrom(value);
} else {
override_ = value;
}
} else {
overrideBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 10;
*/
public Builder clearOverride() {
bitField0_ = (bitField0_ & ~0x00000004);
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 10;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder getOverrideBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getOverrideFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.AppOverride override = 10;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
if (overrideBuilder_ != null) {
return overrideBuilder_.getMessageOrBuilder();
} else {
return override_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
}
/**
* optional .java.apphosting.AppOverride override = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>
getOverrideFieldBuilder() {
if (overrideBuilder_ == null) {
overrideBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>(
getOverride(),
getParentForChildren(),
isClean());
override_ = null;
}
return overrideBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheSetRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheSetRequest)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheSetRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheSetResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheSetResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @return A list containing the setStatus.
*/
java.util.List getSetStatusList();
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @return The count of setStatus.
*/
int getSetStatusCount();
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @param index The index of the element to return.
* @return The setStatus at the given index.
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode getSetStatus(int index);
}
/**
* Protobuf type {@code java.apphosting.MemcacheSetResponse}
*/
public static final class MemcacheSetResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheSetResponse)
MemcacheSetResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheSetResponse.newBuilder() to construct.
private MemcacheSetResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheSetResponse() {
setStatus_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheSetResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.MemcacheSetResponse.SetStatusCode}
*/
public enum SetStatusCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Key-value pair was successfully stored.
*
*
* STORED = 1;
*/
STORED(1),
/**
*
* Not stored for policy reasons (e.g. policy=ADD but item exists),
* not error reasons.
*
*
* NOT_STORED = 2;
*/
NOT_STORED(2),
/**
*
* Not stored due to bad request, e.g. invalid key, item too large, ...
*
*
* ERROR = 3;
*/
ERROR(3),
/**
*
* If you lost a CAS race. From the memcached docs: "to indicate
* that the item you are trying to store with a CAS command has
* been modified since you last fetched it." If instead the item
* being CAS'd has fallen out of memcacheg, NOT_STORED will be
* returned instead.
*
*
* EXISTS = 4;
*/
EXISTS(4),
/**
*
* Response from backend not received in time. It's not known whether the
* item was stored.
*
*
* DEADLINE_EXCEEDED = 5;
*/
DEADLINE_EXCEEDED(5),
/**
*
* Could not reach memcacheg backend (may be dead). The item was
* definitively not stored.
*
*
* UNREACHABLE = 6;
*/
UNREACHABLE(6),
/**
*
* Other service error, e.g. other RPC errors, shardlock failure, missing
* config (ServingState). It's not known whether the item was stored or
* not.
*
*
* OTHER_ERROR = 7;
*/
OTHER_ERROR(7),
;
/**
*
* Key-value pair was successfully stored.
*
*
* STORED = 1;
*/
public static final int STORED_VALUE = 1;
/**
*
* Not stored for policy reasons (e.g. policy=ADD but item exists),
* not error reasons.
*
*
* NOT_STORED = 2;
*/
public static final int NOT_STORED_VALUE = 2;
/**
*
* Not stored due to bad request, e.g. invalid key, item too large, ...
*
*
* ERROR = 3;
*/
public static final int ERROR_VALUE = 3;
/**
*
* If you lost a CAS race. From the memcached docs: "to indicate
* that the item you are trying to store with a CAS command has
* been modified since you last fetched it." If instead the item
* being CAS'd has fallen out of memcacheg, NOT_STORED will be
* returned instead.
*
*
* EXISTS = 4;
*/
public static final int EXISTS_VALUE = 4;
/**
*
* Response from backend not received in time. It's not known whether the
* item was stored.
*
*
* DEADLINE_EXCEEDED = 5;
*/
public static final int DEADLINE_EXCEEDED_VALUE = 5;
/**
*
* Could not reach memcacheg backend (may be dead). The item was
* definitively not stored.
*
*
* UNREACHABLE = 6;
*/
public static final int UNREACHABLE_VALUE = 6;
/**
*
* Other service error, e.g. other RPC errors, shardlock failure, missing
* config (ServingState). It's not known whether the item was stored or
* not.
*
*
* OTHER_ERROR = 7;
*/
public static final int OTHER_ERROR_VALUE = 7;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SetStatusCode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static SetStatusCode forNumber(int value) {
switch (value) {
case 1: return STORED;
case 2: return NOT_STORED;
case 3: return ERROR;
case 4: return EXISTS;
case 5: return DEADLINE_EXCEEDED;
case 6: return UNREACHABLE;
case 7: return OTHER_ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
SetStatusCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SetStatusCode findValueByNumber(int number) {
return SetStatusCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.getDescriptor().getEnumTypes().get(0);
}
private static final SetStatusCode[] VALUES = values();
public static SetStatusCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private SetStatusCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.MemcacheSetResponse.SetStatusCode)
}
public static final int SET_STATUS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List setStatus_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode> setStatus_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode>() {
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode convert(java.lang.Integer from) {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode.forNumber(from);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode.STORED : result;
}
};
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @return A list containing the setStatus.
*/
@java.lang.Override
public java.util.List getSetStatusList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode>(setStatus_, setStatus_converter_);
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @return The count of setStatus.
*/
@java.lang.Override
public int getSetStatusCount() {
return setStatus_.size();
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @param index The index of the element to return.
* @return The setStatus at the given index.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode getSetStatus(int index) {
return setStatus_converter_.convert(setStatus_.get(index));
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < setStatus_.size(); i++) {
output.writeEnum(1, setStatus_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < setStatus_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(setStatus_.get(i));
}
size += dataSize;
size += 1 * setStatus_.size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse) obj;
if (!setStatus_.equals(other.setStatus_)) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSetStatusCount() > 0) {
hash = (37 * hash) + SET_STATUS_FIELD_NUMBER;
hash = (53 * hash) + setStatus_.hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheSetResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheSetResponse)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
setStatus_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheSetResponse_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse result) {
if (((bitField0_ & 0x00000001) != 0)) {
setStatus_ = java.util.Collections.unmodifiableList(setStatus_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.setStatus_ = setStatus_;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.getDefaultInstance()) return this;
if (!other.setStatus_.isEmpty()) {
if (setStatus_.isEmpty()) {
setStatus_ = other.setStatus_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSetStatusIsMutable();
setStatus_.addAll(other.setStatus_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
ensureSetStatusIsMutable();
setStatus_.add(tmpRaw);
}
break;
} // case 8
case 10: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
ensureSetStatusIsMutable();
setStatus_.add(tmpRaw);
}
}
input.popLimit(oldLimit);
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List setStatus_ =
java.util.Collections.emptyList();
private void ensureSetStatusIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
setStatus_ = new java.util.ArrayList(setStatus_);
bitField0_ |= 0x00000001;
}
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @return A list containing the setStatus.
*/
public java.util.List getSetStatusList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode>(setStatus_, setStatus_converter_);
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @return The count of setStatus.
*/
public int getSetStatusCount() {
return setStatus_.size();
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @param index The index of the element to return.
* @return The setStatus at the given index.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode getSetStatus(int index) {
return setStatus_converter_.convert(setStatus_.get(index));
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @param index The index to set the value at.
* @param value The setStatus to set.
* @return This builder for chaining.
*/
public Builder setSetStatus(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode value) {
if (value == null) {
throw new NullPointerException();
}
ensureSetStatusIsMutable();
setStatus_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @param value The setStatus to add.
* @return This builder for chaining.
*/
public Builder addSetStatus(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode value) {
if (value == null) {
throw new NullPointerException();
}
ensureSetStatusIsMutable();
setStatus_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @param values The setStatus to add.
* @return This builder for chaining.
*/
public Builder addAllSetStatus(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode> values) {
ensureSetStatusIsMutable();
for (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse.SetStatusCode value : values) {
setStatus_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* One set_status will be returned for each set key, in the same
* order that the requests were in.
*
*
* repeated .java.apphosting.MemcacheSetResponse.SetStatusCode set_status = 1;
* @return This builder for chaining.
*/
public Builder clearSetStatus() {
setStatus_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheSetResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheSetResponse)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheSetResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheSetResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheDeleteRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheDeleteRequest)
com.google.protobuf.MessageOrBuilder {
/**
* repeated group Item = 1 { ... }
*/
java.util.List
getItemList();
/**
* repeated group Item = 1 { ... }
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item getItem(int index);
/**
* repeated group Item = 1 { ... }
*/
int getItemCount();
/**
* repeated group Item = 1 { ... }
*/
java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder>
getItemOrBuilderList();
/**
* repeated group Item = 1 { ... }
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder getItemOrBuilder(
int index);
/**
* optional string name_space = 4 [default = ""];
* @return Whether the nameSpace field is set.
*/
boolean hasNameSpace();
/**
* optional string name_space = 4 [default = ""];
* @return The nameSpace.
*/
java.lang.String getNameSpace();
/**
* optional string name_space = 4 [default = ""];
* @return The bytes for nameSpace.
*/
com.google.protobuf.ByteString
getNameSpaceBytes();
/**
* optional .java.apphosting.AppOverride override = 5;
* @return Whether the override field is set.
*/
boolean hasOverride();
/**
* optional .java.apphosting.AppOverride override = 5;
* @return The override.
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride();
/**
* optional .java.apphosting.AppOverride override = 5;
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder();
}
/**
* Protobuf type {@code java.apphosting.MemcacheDeleteRequest}
*/
public static final class MemcacheDeleteRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheDeleteRequest)
MemcacheDeleteRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheDeleteRequest.newBuilder() to construct.
private MemcacheDeleteRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheDeleteRequest() {
item_ = java.util.Collections.emptyList();
nameSpace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheDeleteRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Builder.class);
}
public interface ItemOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheDeleteRequest.Item)
com.google.protobuf.MessageOrBuilder {
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
*
* There's no limit to what this value may be, outside of the limit of
* it being a 32-bit int.
*
*
* optional fixed32 delete_time = 3 [default = 0];
* @return Whether the deleteTime field is set.
*/
boolean hasDeleteTime();
/**
*
* There's no limit to what this value may be, outside of the limit of
* it being a 32-bit int.
*
*
* optional fixed32 delete_time = 3 [default = 0];
* @return The deleteTime.
*/
int getDeleteTime();
}
/**
* Protobuf type {@code java.apphosting.MemcacheDeleteRequest.Item}
*/
public static final class Item extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheDeleteRequest.Item)
ItemOrBuilder {
private static final long serialVersionUID = 0L;
// Use Item.newBuilder() to construct.
private Item(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Item() {
key_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Item();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_Item_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_Item_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int DELETE_TIME_FIELD_NUMBER = 3;
private int deleteTime_ = 0;
/**
*
* There's no limit to what this value may be, outside of the limit of
* it being a 32-bit int.
*
*
* optional fixed32 delete_time = 3 [default = 0];
* @return Whether the deleteTime field is set.
*/
@java.lang.Override
public boolean hasDeleteTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* There's no limit to what this value may be, outside of the limit of
* it being a 32-bit int.
*
*
* optional fixed32 delete_time = 3 [default = 0];
* @return The deleteTime.
*/
@java.lang.Override
public int getDeleteTime() {
return deleteTime_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(2, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeFixed32(3, deleteTime_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed32Size(3, deleteTime_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasDeleteTime() != other.hasDeleteTime()) return false;
if (hasDeleteTime()) {
if (getDeleteTime()
!= other.getDeleteTime()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasDeleteTime()) {
hash = (37 * hash) + DELETE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getDeleteTime();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheDeleteRequest.Item}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheDeleteRequest.Item)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_Item_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_Item_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = com.google.protobuf.ByteString.EMPTY;
deleteTime_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_Item_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.deleteTime_ = deleteTime_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasDeleteTime()) {
setDeleteTime(other.getDeleteTime());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 18
case 29: {
deleteTime_ = input.readFixed32();
bitField0_ |= 0x00000002;
break;
} // case 29
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 2;
* @return This builder for chaining.
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private int deleteTime_ ;
/**
*
* There's no limit to what this value may be, outside of the limit of
* it being a 32-bit int.
*
*
* optional fixed32 delete_time = 3 [default = 0];
* @return Whether the deleteTime field is set.
*/
@java.lang.Override
public boolean hasDeleteTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* There's no limit to what this value may be, outside of the limit of
* it being a 32-bit int.
*
*
* optional fixed32 delete_time = 3 [default = 0];
* @return The deleteTime.
*/
@java.lang.Override
public int getDeleteTime() {
return deleteTime_;
}
/**
*
* There's no limit to what this value may be, outside of the limit of
* it being a 32-bit int.
*
*
* optional fixed32 delete_time = 3 [default = 0];
* @param value The deleteTime to set.
* @return This builder for chaining.
*/
public Builder setDeleteTime(int value) {
deleteTime_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* There's no limit to what this value may be, outside of the limit of
* it being a 32-bit int.
*
*
* optional fixed32 delete_time = 3 [default = 0];
* @return This builder for chaining.
*/
public Builder clearDeleteTime() {
bitField0_ = (bitField0_ & ~0x00000002);
deleteTime_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheDeleteRequest.Item)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheDeleteRequest.Item)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser-
PARSER = new com.google.protobuf.AbstractParser
- () {
@java.lang.Override
public Item parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser
- parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser
- getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int ITEM_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List
item_;
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public java.util.List getItemList() {
return item_;
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder>
getItemOrBuilderList() {
return item_;
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public int getItemCount() {
return item_.size();
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item getItem(int index) {
return item_.get(index);
}
/**
* repeated group Item = 1 { ... }
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder getItemOrBuilder(
int index) {
return item_.get(index);
}
public static final int NAME_SPACE_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 4 [default = ""];
* @return Whether the nameSpace field is set.
*/
@java.lang.Override
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name_space = 4 [default = ""];
* @return The nameSpace.
*/
@java.lang.Override
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
}
}
/**
* optional string name_space = 4 [default = ""];
* @return The bytes for nameSpace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OVERRIDE_FIELD_NUMBER = 5;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
/**
* optional .java.apphosting.AppOverride override = 5;
* @return Whether the override field is set.
*/
@java.lang.Override
public boolean hasOverride() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 5;
* @return The override.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getItemCount(); i++) {
if (!getItem(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasOverride()) {
if (!getOverride().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < item_.size(); i++) {
output.writeGroup(1, item_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, nameSpace_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(5, getOverride());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < item_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(1, item_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, nameSpace_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getOverride());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest) obj;
if (!getItemList()
.equals(other.getItemList())) return false;
if (hasNameSpace() != other.hasNameSpace()) return false;
if (hasNameSpace()) {
if (!getNameSpace()
.equals(other.getNameSpace())) return false;
}
if (hasOverride() != other.hasOverride()) return false;
if (hasOverride()) {
if (!getOverride()
.equals(other.getOverride())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getItemCount() > 0) {
hash = (37 * hash) + ITEM_FIELD_NUMBER;
hash = (53 * hash) + getItemList().hashCode();
}
if (hasNameSpace()) {
hash = (37 * hash) + NAME_SPACE_FIELD_NUMBER;
hash = (53 * hash) + getNameSpace().hashCode();
}
if (hasOverride()) {
hash = (37 * hash) + OVERRIDE_FIELD_NUMBER;
hash = (53 * hash) + getOverride().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheDeleteRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheDeleteRequest)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getItemFieldBuilder();
getOverrideFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
} else {
item_ = null;
itemBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
nameSpace_ = "";
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteRequest_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest result) {
if (itemBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.nameSpace_ = nameSpace_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.override_ = overrideBuilder_ == null
? override_
: overrideBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.getDefaultInstance()) return this;
if (itemBuilder_ == null) {
if (!other.item_.isEmpty()) {
if (item_.isEmpty()) {
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureItemIsMutable();
item_.addAll(other.item_);
}
onChanged();
}
} else {
if (!other.item_.isEmpty()) {
if (itemBuilder_.isEmpty()) {
itemBuilder_.dispose();
itemBuilder_ = null;
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000001);
itemBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getItemFieldBuilder() : null;
} else {
itemBuilder_.addAllMessages(other.item_);
}
}
}
if (other.hasNameSpace()) {
nameSpace_ = other.nameSpace_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasOverride()) {
mergeOverride(other.getOverride());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getItemCount(); i++) {
if (!getItem(i).isInitialized()) {
return false;
}
}
if (hasOverride()) {
if (!getOverride().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 11: {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item m =
input.readGroup(1,
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.PARSER,
extensionRegistry);
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(m);
} else {
itemBuilder_.addMessage(m);
}
break;
} // case 11
case 34: {
nameSpace_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 34
case 42: {
input.readMessage(
getOverrideFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List item_ =
java.util.Collections.emptyList();
private void ensureItemIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
item_ = new java.util.ArrayList(item_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder> itemBuilder_;
/**
* repeated group Item = 1 { ... }
*/
public java.util.List getItemList() {
if (itemBuilder_ == null) {
return java.util.Collections.unmodifiableList(item_);
} else {
return itemBuilder_.getMessageList();
}
}
/**
* repeated group Item = 1 { ... }
*/
public int getItemCount() {
if (itemBuilder_ == null) {
return item_.size();
} else {
return itemBuilder_.getCount();
}
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item getItem(int index) {
if (itemBuilder_ == null) {
return item_.get(index);
} else {
return itemBuilder_.getMessage(index);
}
}
/**
* repeated group Item = 1 { ... }
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.set(index, value);
onChanged();
} else {
itemBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.set(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(value);
onChanged();
} else {
itemBuilder_.addMessage(value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(index, value);
onChanged();
} else {
itemBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder addAllItem(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item> values) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, item_);
onChanged();
} else {
itemBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
itemBuilder_.clear();
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public Builder removeItem(int index) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.remove(index);
onChanged();
} else {
itemBuilder_.remove(index);
}
return this;
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder getItemBuilder(
int index) {
return getItemFieldBuilder().getBuilder(index);
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder getItemOrBuilder(
int index) {
if (itemBuilder_ == null) {
return item_.get(index); } else {
return itemBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated group Item = 1 { ... }
*/
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder>
getItemOrBuilderList() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(item_);
}
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder addItemBuilder() {
return getItemFieldBuilder().addBuilder(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.getDefaultInstance());
}
/**
* repeated group Item = 1 { ... }
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder addItemBuilder(
int index) {
return getItemFieldBuilder().addBuilder(
index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.getDefaultInstance());
}
/**
* repeated group Item = 1 { ... }
*/
public java.util.List
getItemBuilderList() {
return getItemFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.Item.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest.ItemOrBuilder>(
item_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
private java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 4 [default = ""];
* @return Whether the nameSpace field is set.
*/
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string name_space = 4 [default = ""];
* @return The nameSpace.
*/
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name_space = 4 [default = ""];
* @return The bytes for nameSpace.
*/
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name_space = 4 [default = ""];
* @param value The nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpace(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional string name_space = 4 [default = ""];
* @return This builder for chaining.
*/
public Builder clearNameSpace() {
nameSpace_ = getDefaultInstance().getNameSpace();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* optional string name_space = 4 [default = ""];
* @param value The bytes for nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder> overrideBuilder_;
/**
* optional .java.apphosting.AppOverride override = 5;
* @return Whether the override field is set.
*/
public boolean hasOverride() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 5;
* @return The override.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
if (overrideBuilder_ == null) {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
} else {
return overrideBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public Builder setOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
override_ = value;
} else {
overrideBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public Builder setOverride(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder builderForValue) {
if (overrideBuilder_ == null) {
override_ = builderForValue.build();
} else {
overrideBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public Builder mergeOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
override_ != null &&
override_ != com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance()) {
getOverrideBuilder().mergeFrom(value);
} else {
override_ = value;
}
} else {
overrideBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public Builder clearOverride() {
bitField0_ = (bitField0_ & ~0x00000004);
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder getOverrideBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getOverrideFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
if (overrideBuilder_ != null) {
return overrideBuilder_.getMessageOrBuilder();
} else {
return override_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
}
/**
* optional .java.apphosting.AppOverride override = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>
getOverrideFieldBuilder() {
if (overrideBuilder_ == null) {
overrideBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>(
getOverride(),
getParentForChildren(),
isClean());
override_ = null;
}
return overrideBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheDeleteRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheDeleteRequest)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheDeleteRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheDeleteResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheDeleteResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @return A list containing the deleteStatus.
*/
java.util.List getDeleteStatusList();
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @return The count of deleteStatus.
*/
int getDeleteStatusCount();
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @param index The index of the element to return.
* @return The deleteStatus at the given index.
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode getDeleteStatus(int index);
}
/**
* Protobuf type {@code java.apphosting.MemcacheDeleteResponse}
*/
public static final class MemcacheDeleteResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheDeleteResponse)
MemcacheDeleteResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheDeleteResponse.newBuilder() to construct.
private MemcacheDeleteResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheDeleteResponse() {
deleteStatus_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheDeleteResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.MemcacheDeleteResponse.DeleteStatusCode}
*/
public enum DeleteStatusCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The key existed and was deleted.
*
*
* DELETED = 1;
*/
DELETED(1),
/**
*
* The key didn't exist and therefore didn't need to be deleted.
*
*
* NOT_FOUND = 2;
*/
NOT_FOUND(2),
/**
*
* Response from backend not received in time. It's not known whether the
* item existed and it's also not known whether it was deleted.
*
*
* DEADLINE_EXCEEDED = 3;
*/
DEADLINE_EXCEEDED(3),
/**
*
* Could not reach memcacheg backend (may be dead). It's not known whether
* the item existed, but it definitively was not deleted.
*
*
* UNREACHABLE = 4;
*/
UNREACHABLE(4),
/**
*
* Other service error, e.g. other RPC errors, shardlock failure, missing
* config (ServingState). It's not known whether the item existed and
* it's also not known whether it was deleted.
*
*
* OTHER_ERROR = 5;
*/
OTHER_ERROR(5),
;
/**
*
* The key existed and was deleted.
*
*
* DELETED = 1;
*/
public static final int DELETED_VALUE = 1;
/**
*
* The key didn't exist and therefore didn't need to be deleted.
*
*
* NOT_FOUND = 2;
*/
public static final int NOT_FOUND_VALUE = 2;
/**
*
* Response from backend not received in time. It's not known whether the
* item existed and it's also not known whether it was deleted.
*
*
* DEADLINE_EXCEEDED = 3;
*/
public static final int DEADLINE_EXCEEDED_VALUE = 3;
/**
*
* Could not reach memcacheg backend (may be dead). It's not known whether
* the item existed, but it definitively was not deleted.
*
*
* UNREACHABLE = 4;
*/
public static final int UNREACHABLE_VALUE = 4;
/**
*
* Other service error, e.g. other RPC errors, shardlock failure, missing
* config (ServingState). It's not known whether the item existed and
* it's also not known whether it was deleted.
*
*
* OTHER_ERROR = 5;
*/
public static final int OTHER_ERROR_VALUE = 5;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DeleteStatusCode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static DeleteStatusCode forNumber(int value) {
switch (value) {
case 1: return DELETED;
case 2: return NOT_FOUND;
case 3: return DEADLINE_EXCEEDED;
case 4: return UNREACHABLE;
case 5: return OTHER_ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
DeleteStatusCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DeleteStatusCode findValueByNumber(int number) {
return DeleteStatusCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.getDescriptor().getEnumTypes().get(0);
}
private static final DeleteStatusCode[] VALUES = values();
public static DeleteStatusCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private DeleteStatusCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.MemcacheDeleteResponse.DeleteStatusCode)
}
public static final int DELETE_STATUS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List deleteStatus_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode> deleteStatus_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode>() {
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode convert(java.lang.Integer from) {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode.forNumber(from);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode.DELETED : result;
}
};
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @return A list containing the deleteStatus.
*/
@java.lang.Override
public java.util.List getDeleteStatusList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode>(deleteStatus_, deleteStatus_converter_);
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @return The count of deleteStatus.
*/
@java.lang.Override
public int getDeleteStatusCount() {
return deleteStatus_.size();
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @param index The index of the element to return.
* @return The deleteStatus at the given index.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode getDeleteStatus(int index) {
return deleteStatus_converter_.convert(deleteStatus_.get(index));
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < deleteStatus_.size(); i++) {
output.writeEnum(1, deleteStatus_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < deleteStatus_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(deleteStatus_.get(i));
}
size += dataSize;
size += 1 * deleteStatus_.size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse) obj;
if (!deleteStatus_.equals(other.deleteStatus_)) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getDeleteStatusCount() > 0) {
hash = (37 * hash) + DELETE_STATUS_FIELD_NUMBER;
hash = (53 * hash) + deleteStatus_.hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheDeleteResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheDeleteResponse)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
deleteStatus_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheDeleteResponse_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse result) {
if (((bitField0_ & 0x00000001) != 0)) {
deleteStatus_ = java.util.Collections.unmodifiableList(deleteStatus_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.deleteStatus_ = deleteStatus_;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.getDefaultInstance()) return this;
if (!other.deleteStatus_.isEmpty()) {
if (deleteStatus_.isEmpty()) {
deleteStatus_ = other.deleteStatus_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDeleteStatusIsMutable();
deleteStatus_.addAll(other.deleteStatus_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
ensureDeleteStatusIsMutable();
deleteStatus_.add(tmpRaw);
}
break;
} // case 8
case 10: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
ensureDeleteStatusIsMutable();
deleteStatus_.add(tmpRaw);
}
}
input.popLimit(oldLimit);
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List deleteStatus_ =
java.util.Collections.emptyList();
private void ensureDeleteStatusIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
deleteStatus_ = new java.util.ArrayList(deleteStatus_);
bitField0_ |= 0x00000001;
}
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @return A list containing the deleteStatus.
*/
public java.util.List getDeleteStatusList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode>(deleteStatus_, deleteStatus_converter_);
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @return The count of deleteStatus.
*/
public int getDeleteStatusCount() {
return deleteStatus_.size();
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @param index The index of the element to return.
* @return The deleteStatus at the given index.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode getDeleteStatus(int index) {
return deleteStatus_converter_.convert(deleteStatus_.get(index));
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @param index The index to set the value at.
* @param value The deleteStatus to set.
* @return This builder for chaining.
*/
public Builder setDeleteStatus(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode value) {
if (value == null) {
throw new NullPointerException();
}
ensureDeleteStatusIsMutable();
deleteStatus_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @param value The deleteStatus to add.
* @return This builder for chaining.
*/
public Builder addDeleteStatus(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode value) {
if (value == null) {
throw new NullPointerException();
}
ensureDeleteStatusIsMutable();
deleteStatus_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @param values The deleteStatus to add.
* @return This builder for chaining.
*/
public Builder addAllDeleteStatus(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode> values) {
ensureDeleteStatusIsMutable();
for (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse.DeleteStatusCode value : values) {
deleteStatus_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* one set_status will be returned for each set key, matching the
* order of the requested items to delete.
*
*
* repeated .java.apphosting.MemcacheDeleteResponse.DeleteStatusCode delete_status = 1;
* @return This builder for chaining.
*/
public Builder clearDeleteStatus() {
deleteStatus_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheDeleteResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheDeleteResponse)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheDeleteResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheDeleteResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheIncrementRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheIncrementRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 1;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 1;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
* optional string name_space = 4 [default = ""];
* @return Whether the nameSpace field is set.
*/
boolean hasNameSpace();
/**
* optional string name_space = 4 [default = ""];
* @return The nameSpace.
*/
java.lang.String getNameSpace();
/**
* optional string name_space = 4 [default = ""];
* @return The bytes for nameSpace.
*/
com.google.protobuf.ByteString
getNameSpaceBytes();
/**
*
* The amount to increment/decrement the value by, if it already
* exists in the cache. Note that this does not implicitly create a
* new counter starting at the specified delta. To initialize a new
* counter, the client must Set an initial value. (which they send
* as a decimal string, just like memcached).
*
*
* optional uint64 delta = 2 [default = 1];
* @return Whether the delta field is set.
*/
boolean hasDelta();
/**
*
* The amount to increment/decrement the value by, if it already
* exists in the cache. Note that this does not implicitly create a
* new counter starting at the specified delta. To initialize a new
* counter, the client must Set an initial value. (which they send
* as a decimal string, just like memcached).
*
*
* optional uint64 delta = 2 [default = 1];
* @return The delta.
*/
long getDelta();
/**
* optional .java.apphosting.MemcacheIncrementRequest.Direction direction = 3 [default = INCREMENT];
* @return Whether the direction field is set.
*/
boolean hasDirection();
/**
* optional .java.apphosting.MemcacheIncrementRequest.Direction direction = 3 [default = INCREMENT];
* @return The direction.
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction getDirection();
/**
*
* If set (even if set to 0), traditional memcached semantics around
* needing to set the value in the cache before doing an increment
* are ignored and the value is instead initialized to this value if
* it's not already in the cache. If you want this before, you'll
* probably want to set this to zero, where most apps count from.
*
*
* optional uint64 initial_value = 5;
* @return Whether the initialValue field is set.
*/
boolean hasInitialValue();
/**
*
* If set (even if set to 0), traditional memcached semantics around
* needing to set the value in the cache before doing an increment
* are ignored and the value is instead initialized to this value if
* it's not already in the cache. If you want this before, you'll
* probably want to set this to zero, where most apps count from.
*
*
* optional uint64 initial_value = 5;
* @return The initialValue.
*/
long getInitialValue();
/**
*
* If set, determines the flags that are used for a value
* initialized from initial_value.
*
*
* optional fixed32 initial_flags = 6;
* @return Whether the initialFlags field is set.
*/
boolean hasInitialFlags();
/**
*
* If set, determines the flags that are used for a value
* initialized from initial_value.
*
*
* optional fixed32 initial_flags = 6;
* @return The initialFlags.
*/
int getInitialFlags();
/**
* optional .java.apphosting.AppOverride override = 7;
* @return Whether the override field is set.
*/
boolean hasOverride();
/**
* optional .java.apphosting.AppOverride override = 7;
* @return The override.
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride();
/**
* optional .java.apphosting.AppOverride override = 7;
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder();
}
/**
*
* The memcached protocol spec defines the deltas for both "incr"
* and "decr" as uint64 values. Since we're lumping these together
* as one RPC, we also need the optional direction to specify decrementing.
* By default the delta is '1' and direction is increment (the common use case).
* Note that this mutation request doesn't support multiple operations
* at once, intentionally, as that's a rare operation.
*
*
* Protobuf type {@code java.apphosting.MemcacheIncrementRequest}
*/
public static final class MemcacheIncrementRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheIncrementRequest)
MemcacheIncrementRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheIncrementRequest.newBuilder() to construct.
private MemcacheIncrementRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheIncrementRequest() {
key_ = com.google.protobuf.ByteString.EMPTY;
nameSpace_ = "";
delta_ = 1L;
direction_ = 1;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheIncrementRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.MemcacheIncrementRequest.Direction}
*/
public enum Direction
implements com.google.protobuf.ProtocolMessageEnum {
/**
* INCREMENT = 1;
*/
INCREMENT(1),
/**
* DECREMENT = 2;
*/
DECREMENT(2),
;
/**
* INCREMENT = 1;
*/
public static final int INCREMENT_VALUE = 1;
/**
* DECREMENT = 2;
*/
public static final int DECREMENT_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Direction valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Direction forNumber(int value) {
switch (value) {
case 1: return INCREMENT;
case 2: return DECREMENT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Direction> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Direction findValueByNumber(int number) {
return Direction.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.getDescriptor().getEnumTypes().get(0);
}
private static final Direction[] VALUES = values();
public static Direction valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Direction(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.MemcacheIncrementRequest.Direction)
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 1;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 1;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int NAME_SPACE_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 4 [default = ""];
* @return Whether the nameSpace field is set.
*/
@java.lang.Override
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string name_space = 4 [default = ""];
* @return The nameSpace.
*/
@java.lang.Override
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
}
}
/**
* optional string name_space = 4 [default = ""];
* @return The bytes for nameSpace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DELTA_FIELD_NUMBER = 2;
private long delta_ = 1L;
/**
*
* The amount to increment/decrement the value by, if it already
* exists in the cache. Note that this does not implicitly create a
* new counter starting at the specified delta. To initialize a new
* counter, the client must Set an initial value. (which they send
* as a decimal string, just like memcached).
*
*
* optional uint64 delta = 2 [default = 1];
* @return Whether the delta field is set.
*/
@java.lang.Override
public boolean hasDelta() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The amount to increment/decrement the value by, if it already
* exists in the cache. Note that this does not implicitly create a
* new counter starting at the specified delta. To initialize a new
* counter, the client must Set an initial value. (which they send
* as a decimal string, just like memcached).
*
*
* optional uint64 delta = 2 [default = 1];
* @return The delta.
*/
@java.lang.Override
public long getDelta() {
return delta_;
}
public static final int DIRECTION_FIELD_NUMBER = 3;
private int direction_ = 1;
/**
* optional .java.apphosting.MemcacheIncrementRequest.Direction direction = 3 [default = INCREMENT];
* @return Whether the direction field is set.
*/
@java.lang.Override public boolean hasDirection() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional .java.apphosting.MemcacheIncrementRequest.Direction direction = 3 [default = INCREMENT];
* @return The direction.
*/
@java.lang.Override public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction getDirection() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction.forNumber(direction_);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction.INCREMENT : result;
}
public static final int INITIAL_VALUE_FIELD_NUMBER = 5;
private long initialValue_ = 0L;
/**
*
* If set (even if set to 0), traditional memcached semantics around
* needing to set the value in the cache before doing an increment
* are ignored and the value is instead initialized to this value if
* it's not already in the cache. If you want this before, you'll
* probably want to set this to zero, where most apps count from.
*
*
* optional uint64 initial_value = 5;
* @return Whether the initialValue field is set.
*/
@java.lang.Override
public boolean hasInitialValue() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* If set (even if set to 0), traditional memcached semantics around
* needing to set the value in the cache before doing an increment
* are ignored and the value is instead initialized to this value if
* it's not already in the cache. If you want this before, you'll
* probably want to set this to zero, where most apps count from.
*
*
* optional uint64 initial_value = 5;
* @return The initialValue.
*/
@java.lang.Override
public long getInitialValue() {
return initialValue_;
}
public static final int INITIAL_FLAGS_FIELD_NUMBER = 6;
private int initialFlags_ = 0;
/**
*
* If set, determines the flags that are used for a value
* initialized from initial_value.
*
*
* optional fixed32 initial_flags = 6;
* @return Whether the initialFlags field is set.
*/
@java.lang.Override
public boolean hasInitialFlags() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* If set, determines the flags that are used for a value
* initialized from initial_value.
*
*
* optional fixed32 initial_flags = 6;
* @return The initialFlags.
*/
@java.lang.Override
public int getInitialFlags() {
return initialFlags_;
}
public static final int OVERRIDE_FIELD_NUMBER = 7;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
/**
* optional .java.apphosting.AppOverride override = 7;
* @return Whether the override field is set.
*/
@java.lang.Override
public boolean hasOverride() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 7;
* @return The override.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
/**
* optional .java.apphosting.AppOverride override = 7;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (hasOverride()) {
if (!getOverride().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, key_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeUInt64(2, delta_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeEnum(3, direction_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, nameSpace_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeUInt64(5, initialValue_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeFixed32(6, initialFlags_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(7, getOverride());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, key_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, delta_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, direction_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, nameSpace_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, initialValue_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed32Size(6, initialFlags_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getOverride());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasNameSpace() != other.hasNameSpace()) return false;
if (hasNameSpace()) {
if (!getNameSpace()
.equals(other.getNameSpace())) return false;
}
if (hasDelta() != other.hasDelta()) return false;
if (hasDelta()) {
if (getDelta()
!= other.getDelta()) return false;
}
if (hasDirection() != other.hasDirection()) return false;
if (hasDirection()) {
if (direction_ != other.direction_) return false;
}
if (hasInitialValue() != other.hasInitialValue()) return false;
if (hasInitialValue()) {
if (getInitialValue()
!= other.getInitialValue()) return false;
}
if (hasInitialFlags() != other.hasInitialFlags()) return false;
if (hasInitialFlags()) {
if (getInitialFlags()
!= other.getInitialFlags()) return false;
}
if (hasOverride() != other.hasOverride()) return false;
if (hasOverride()) {
if (!getOverride()
.equals(other.getOverride())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasNameSpace()) {
hash = (37 * hash) + NAME_SPACE_FIELD_NUMBER;
hash = (53 * hash) + getNameSpace().hashCode();
}
if (hasDelta()) {
hash = (37 * hash) + DELTA_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDelta());
}
if (hasDirection()) {
hash = (37 * hash) + DIRECTION_FIELD_NUMBER;
hash = (53 * hash) + direction_;
}
if (hasInitialValue()) {
hash = (37 * hash) + INITIAL_VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getInitialValue());
}
if (hasInitialFlags()) {
hash = (37 * hash) + INITIAL_FLAGS_FIELD_NUMBER;
hash = (53 * hash) + getInitialFlags();
}
if (hasOverride()) {
hash = (37 * hash) + OVERRIDE_FIELD_NUMBER;
hash = (53 * hash) + getOverride().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The memcached protocol spec defines the deltas for both "incr"
* and "decr" as uint64 values. Since we're lumping these together
* as one RPC, we also need the optional direction to specify decrementing.
* By default the delta is '1' and direction is increment (the common use case).
* Note that this mutation request doesn't support multiple operations
* at once, intentionally, as that's a rare operation.
*
*
* Protobuf type {@code java.apphosting.MemcacheIncrementRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheIncrementRequest)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOverrideFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = com.google.protobuf.ByteString.EMPTY;
nameSpace_ = "";
delta_ = 1L;
direction_ = 1;
initialValue_ = 0L;
initialFlags_ = 0;
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementRequest_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.nameSpace_ = nameSpace_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.delta_ = delta_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.direction_ = direction_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.initialValue_ = initialValue_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.initialFlags_ = initialFlags_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.override_ = overrideBuilder_ == null
? override_
: overrideBuilder_.build();
to_bitField0_ |= 0x00000040;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasNameSpace()) {
nameSpace_ = other.nameSpace_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasDelta()) {
setDelta(other.getDelta());
}
if (other.hasDirection()) {
setDirection(other.getDirection());
}
if (other.hasInitialValue()) {
setInitialValue(other.getInitialValue());
}
if (other.hasInitialFlags()) {
setInitialFlags(other.getInitialFlags());
}
if (other.hasOverride()) {
mergeOverride(other.getOverride());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
if (hasOverride()) {
if (!getOverride().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
delta_ = input.readUInt64();
bitField0_ |= 0x00000004;
break;
} // case 16
case 24: {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(3, tmpRaw);
} else {
direction_ = tmpRaw;
bitField0_ |= 0x00000008;
}
break;
} // case 24
case 34: {
nameSpace_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 34
case 40: {
initialValue_ = input.readUInt64();
bitField0_ |= 0x00000010;
break;
} // case 40
case 53: {
initialFlags_ = input.readFixed32();
bitField0_ |= 0x00000020;
break;
} // case 53
case 58: {
input.readMessage(
getOverrideFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 1;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 1;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 1;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* max 250 bytes, per upstream spec
*
*
* required bytes key = 1;
* @return This builder for chaining.
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 4 [default = ""];
* @return Whether the nameSpace field is set.
*/
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string name_space = 4 [default = ""];
* @return The nameSpace.
*/
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name_space = 4 [default = ""];
* @return The bytes for nameSpace.
*/
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name_space = 4 [default = ""];
* @param value The nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpace(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional string name_space = 4 [default = ""];
* @return This builder for chaining.
*/
public Builder clearNameSpace() {
nameSpace_ = getDefaultInstance().getNameSpace();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* optional string name_space = 4 [default = ""];
* @param value The bytes for nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private long delta_ = 1L;
/**
*
* The amount to increment/decrement the value by, if it already
* exists in the cache. Note that this does not implicitly create a
* new counter starting at the specified delta. To initialize a new
* counter, the client must Set an initial value. (which they send
* as a decimal string, just like memcached).
*
*
* optional uint64 delta = 2 [default = 1];
* @return Whether the delta field is set.
*/
@java.lang.Override
public boolean hasDelta() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The amount to increment/decrement the value by, if it already
* exists in the cache. Note that this does not implicitly create a
* new counter starting at the specified delta. To initialize a new
* counter, the client must Set an initial value. (which they send
* as a decimal string, just like memcached).
*
*
* optional uint64 delta = 2 [default = 1];
* @return The delta.
*/
@java.lang.Override
public long getDelta() {
return delta_;
}
/**
*
* The amount to increment/decrement the value by, if it already
* exists in the cache. Note that this does not implicitly create a
* new counter starting at the specified delta. To initialize a new
* counter, the client must Set an initial value. (which they send
* as a decimal string, just like memcached).
*
*
* optional uint64 delta = 2 [default = 1];
* @param value The delta to set.
* @return This builder for chaining.
*/
public Builder setDelta(long value) {
delta_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The amount to increment/decrement the value by, if it already
* exists in the cache. Note that this does not implicitly create a
* new counter starting at the specified delta. To initialize a new
* counter, the client must Set an initial value. (which they send
* as a decimal string, just like memcached).
*
*
* optional uint64 delta = 2 [default = 1];
* @return This builder for chaining.
*/
public Builder clearDelta() {
bitField0_ = (bitField0_ & ~0x00000004);
delta_ = 1L;
onChanged();
return this;
}
private int direction_ = 1;
/**
* optional .java.apphosting.MemcacheIncrementRequest.Direction direction = 3 [default = INCREMENT];
* @return Whether the direction field is set.
*/
@java.lang.Override public boolean hasDirection() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional .java.apphosting.MemcacheIncrementRequest.Direction direction = 3 [default = INCREMENT];
* @return The direction.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction getDirection() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction.forNumber(direction_);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction.INCREMENT : result;
}
/**
* optional .java.apphosting.MemcacheIncrementRequest.Direction direction = 3 [default = INCREMENT];
* @param value The direction to set.
* @return This builder for chaining.
*/
public Builder setDirection(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Direction value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
direction_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .java.apphosting.MemcacheIncrementRequest.Direction direction = 3 [default = INCREMENT];
* @return This builder for chaining.
*/
public Builder clearDirection() {
bitField0_ = (bitField0_ & ~0x00000008);
direction_ = 1;
onChanged();
return this;
}
private long initialValue_ ;
/**
*
* If set (even if set to 0), traditional memcached semantics around
* needing to set the value in the cache before doing an increment
* are ignored and the value is instead initialized to this value if
* it's not already in the cache. If you want this before, you'll
* probably want to set this to zero, where most apps count from.
*
*
* optional uint64 initial_value = 5;
* @return Whether the initialValue field is set.
*/
@java.lang.Override
public boolean hasInitialValue() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* If set (even if set to 0), traditional memcached semantics around
* needing to set the value in the cache before doing an increment
* are ignored and the value is instead initialized to this value if
* it's not already in the cache. If you want this before, you'll
* probably want to set this to zero, where most apps count from.
*
*
* optional uint64 initial_value = 5;
* @return The initialValue.
*/
@java.lang.Override
public long getInitialValue() {
return initialValue_;
}
/**
*
* If set (even if set to 0), traditional memcached semantics around
* needing to set the value in the cache before doing an increment
* are ignored and the value is instead initialized to this value if
* it's not already in the cache. If you want this before, you'll
* probably want to set this to zero, where most apps count from.
*
*
* optional uint64 initial_value = 5;
* @param value The initialValue to set.
* @return This builder for chaining.
*/
public Builder setInitialValue(long value) {
initialValue_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* If set (even if set to 0), traditional memcached semantics around
* needing to set the value in the cache before doing an increment
* are ignored and the value is instead initialized to this value if
* it's not already in the cache. If you want this before, you'll
* probably want to set this to zero, where most apps count from.
*
*
* optional uint64 initial_value = 5;
* @return This builder for chaining.
*/
public Builder clearInitialValue() {
bitField0_ = (bitField0_ & ~0x00000010);
initialValue_ = 0L;
onChanged();
return this;
}
private int initialFlags_ ;
/**
*
* If set, determines the flags that are used for a value
* initialized from initial_value.
*
*
* optional fixed32 initial_flags = 6;
* @return Whether the initialFlags field is set.
*/
@java.lang.Override
public boolean hasInitialFlags() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* If set, determines the flags that are used for a value
* initialized from initial_value.
*
*
* optional fixed32 initial_flags = 6;
* @return The initialFlags.
*/
@java.lang.Override
public int getInitialFlags() {
return initialFlags_;
}
/**
*
* If set, determines the flags that are used for a value
* initialized from initial_value.
*
*
* optional fixed32 initial_flags = 6;
* @param value The initialFlags to set.
* @return This builder for chaining.
*/
public Builder setInitialFlags(int value) {
initialFlags_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* If set, determines the flags that are used for a value
* initialized from initial_value.
*
*
* optional fixed32 initial_flags = 6;
* @return This builder for chaining.
*/
public Builder clearInitialFlags() {
bitField0_ = (bitField0_ & ~0x00000020);
initialFlags_ = 0;
onChanged();
return this;
}
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder> overrideBuilder_;
/**
* optional .java.apphosting.AppOverride override = 7;
* @return Whether the override field is set.
*/
public boolean hasOverride() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 7;
* @return The override.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
if (overrideBuilder_ == null) {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
} else {
return overrideBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.AppOverride override = 7;
*/
public Builder setOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
override_ = value;
} else {
overrideBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 7;
*/
public Builder setOverride(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder builderForValue) {
if (overrideBuilder_ == null) {
override_ = builderForValue.build();
} else {
overrideBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 7;
*/
public Builder mergeOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
override_ != null &&
override_ != com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance()) {
getOverrideBuilder().mergeFrom(value);
} else {
override_ = value;
}
} else {
overrideBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 7;
*/
public Builder clearOverride() {
bitField0_ = (bitField0_ & ~0x00000040);
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 7;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder getOverrideBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getOverrideFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.AppOverride override = 7;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
if (overrideBuilder_ != null) {
return overrideBuilder_.getMessageOrBuilder();
} else {
return override_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
}
/**
* optional .java.apphosting.AppOverride override = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>
getOverrideFieldBuilder() {
if (overrideBuilder_ == null) {
overrideBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>(
getOverride(),
getParentForChildren(),
isClean());
override_ = null;
}
return overrideBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheIncrementRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheIncrementRequest)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheIncrementRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheIncrementResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheIncrementResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* The new value, only set if the item was found. Per the spec,
* underflow is capped at zero, but overflow wraps around.
*
*
* optional uint64 new_value = 1;
* @return Whether the newValue field is set.
*/
boolean hasNewValue();
/**
*
* The new value, only set if the item was found. Per the spec,
* underflow is capped at zero, but overflow wraps around.
*
*
* optional uint64 new_value = 1;
* @return The newValue.
*/
long getNewValue();
/**
*
* Always set, unlike cacheserving_memcacheg::MemcacheIncrementResponse which
* is set only if part of BatchIncrement() call.
*
*
* optional .java.apphosting.MemcacheIncrementResponse.IncrementStatusCode increment_status = 2;
* @return Whether the incrementStatus field is set.
*/
boolean hasIncrementStatus();
/**
*
* Always set, unlike cacheserving_memcacheg::MemcacheIncrementResponse which
* is set only if part of BatchIncrement() call.
*
*
* optional .java.apphosting.MemcacheIncrementResponse.IncrementStatusCode increment_status = 2;
* @return The incrementStatus.
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode getIncrementStatus();
}
/**
* Protobuf type {@code java.apphosting.MemcacheIncrementResponse}
*/
public static final class MemcacheIncrementResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheIncrementResponse)
MemcacheIncrementResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheIncrementResponse.newBuilder() to construct.
private MemcacheIncrementResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheIncrementResponse() {
incrementStatus_ = 1;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheIncrementResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.MemcacheIncrementResponse.IncrementStatusCode}
*/
public enum IncrementStatusCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Key was found and incremented.
*
*
* OK = 1;
*/
OK(1),
/**
*
* For policy reasons, e.g. key doesn't exist and initial value was not
* specified, existing value wasn't a number, or invalid request.
*
*
* NOT_CHANGED = 2;
*/
NOT_CHANGED(2),
/**
*
* May or may not have been incremented due to some server error.
* DEPRECATED in favor of the 3 status codes below. As of April 2017, only
* ERROR is returned. Once we change all clients to understand the new codes
* below, we'll flip --memcache_return_better_errors and ERROR will never be
* returned (tracked in b/25965653).
*
*
* ERROR = 3;
*/
ERROR(3),
/**
*
* Response from backend not received in time. It's not known whether the
* key exists and it's also not known whether it was incremented.
*
*
* DEADLINE_EXCEEDED = 4;
*/
DEADLINE_EXCEEDED(4),
/**
*
* Could not reach memcacheg backend (may be dead). It's not known whether
* the key exists, but it definitively was not incremented.
*
*
* UNREACHABLE = 5;
*/
UNREACHABLE(5),
/**
*
* Other service error, e.g. other RPC errors, shardlock failure, missing
* config (ServingState). It's not known whether the key exists and it's
* also not known whether it was incremented.
*
*
* OTHER_ERROR = 6;
*/
OTHER_ERROR(6),
;
/**
*
* Key was found and incremented.
*
*
* OK = 1;
*/
public static final int OK_VALUE = 1;
/**
*
* For policy reasons, e.g. key doesn't exist and initial value was not
* specified, existing value wasn't a number, or invalid request.
*
*
* NOT_CHANGED = 2;
*/
public static final int NOT_CHANGED_VALUE = 2;
/**
*
* May or may not have been incremented due to some server error.
* DEPRECATED in favor of the 3 status codes below. As of April 2017, only
* ERROR is returned. Once we change all clients to understand the new codes
* below, we'll flip --memcache_return_better_errors and ERROR will never be
* returned (tracked in b/25965653).
*
*
* ERROR = 3;
*/
public static final int ERROR_VALUE = 3;
/**
*
* Response from backend not received in time. It's not known whether the
* key exists and it's also not known whether it was incremented.
*
*
* DEADLINE_EXCEEDED = 4;
*/
public static final int DEADLINE_EXCEEDED_VALUE = 4;
/**
*
* Could not reach memcacheg backend (may be dead). It's not known whether
* the key exists, but it definitively was not incremented.
*
*
* UNREACHABLE = 5;
*/
public static final int UNREACHABLE_VALUE = 5;
/**
*
* Other service error, e.g. other RPC errors, shardlock failure, missing
* config (ServingState). It's not known whether the key exists and it's
* also not known whether it was incremented.
*
*
* OTHER_ERROR = 6;
*/
public static final int OTHER_ERROR_VALUE = 6;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static IncrementStatusCode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static IncrementStatusCode forNumber(int value) {
switch (value) {
case 1: return OK;
case 2: return NOT_CHANGED;
case 3: return ERROR;
case 4: return DEADLINE_EXCEEDED;
case 5: return UNREACHABLE;
case 6: return OTHER_ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
IncrementStatusCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public IncrementStatusCode findValueByNumber(int number) {
return IncrementStatusCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.getDescriptor().getEnumTypes().get(0);
}
private static final IncrementStatusCode[] VALUES = values();
public static IncrementStatusCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private IncrementStatusCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.MemcacheIncrementResponse.IncrementStatusCode)
}
private int bitField0_;
public static final int NEW_VALUE_FIELD_NUMBER = 1;
private long newValue_ = 0L;
/**
*
* The new value, only set if the item was found. Per the spec,
* underflow is capped at zero, but overflow wraps around.
*
*
* optional uint64 new_value = 1;
* @return Whether the newValue field is set.
*/
@java.lang.Override
public boolean hasNewValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The new value, only set if the item was found. Per the spec,
* underflow is capped at zero, but overflow wraps around.
*
*
* optional uint64 new_value = 1;
* @return The newValue.
*/
@java.lang.Override
public long getNewValue() {
return newValue_;
}
public static final int INCREMENT_STATUS_FIELD_NUMBER = 2;
private int incrementStatus_ = 1;
/**
*
* Always set, unlike cacheserving_memcacheg::MemcacheIncrementResponse which
* is set only if part of BatchIncrement() call.
*
*
* optional .java.apphosting.MemcacheIncrementResponse.IncrementStatusCode increment_status = 2;
* @return Whether the incrementStatus field is set.
*/
@java.lang.Override public boolean hasIncrementStatus() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Always set, unlike cacheserving_memcacheg::MemcacheIncrementResponse which
* is set only if part of BatchIncrement() call.
*
*
* optional .java.apphosting.MemcacheIncrementResponse.IncrementStatusCode increment_status = 2;
* @return The incrementStatus.
*/
@java.lang.Override public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode getIncrementStatus() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode.forNumber(incrementStatus_);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode.OK : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeUInt64(1, newValue_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeEnum(2, incrementStatus_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, newValue_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, incrementStatus_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse) obj;
if (hasNewValue() != other.hasNewValue()) return false;
if (hasNewValue()) {
if (getNewValue()
!= other.getNewValue()) return false;
}
if (hasIncrementStatus() != other.hasIncrementStatus()) return false;
if (hasIncrementStatus()) {
if (incrementStatus_ != other.incrementStatus_) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasNewValue()) {
hash = (37 * hash) + NEW_VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNewValue());
}
if (hasIncrementStatus()) {
hash = (37 * hash) + INCREMENT_STATUS_FIELD_NUMBER;
hash = (53 * hash) + incrementStatus_;
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheIncrementResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheIncrementResponse)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
newValue_ = 0L;
incrementStatus_ = 1;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheIncrementResponse_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.newValue_ = newValue_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.incrementStatus_ = incrementStatus_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.getDefaultInstance()) return this;
if (other.hasNewValue()) {
setNewValue(other.getNewValue());
}
if (other.hasIncrementStatus()) {
setIncrementStatus(other.getIncrementStatus());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
newValue_ = input.readUInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
int tmpRaw = input.readEnum();
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode tmpValue =
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(2, tmpRaw);
} else {
incrementStatus_ = tmpRaw;
bitField0_ |= 0x00000002;
}
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long newValue_ ;
/**
*
* The new value, only set if the item was found. Per the spec,
* underflow is capped at zero, but overflow wraps around.
*
*
* optional uint64 new_value = 1;
* @return Whether the newValue field is set.
*/
@java.lang.Override
public boolean hasNewValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The new value, only set if the item was found. Per the spec,
* underflow is capped at zero, but overflow wraps around.
*
*
* optional uint64 new_value = 1;
* @return The newValue.
*/
@java.lang.Override
public long getNewValue() {
return newValue_;
}
/**
*
* The new value, only set if the item was found. Per the spec,
* underflow is capped at zero, but overflow wraps around.
*
*
* optional uint64 new_value = 1;
* @param value The newValue to set.
* @return This builder for chaining.
*/
public Builder setNewValue(long value) {
newValue_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The new value, only set if the item was found. Per the spec,
* underflow is capped at zero, but overflow wraps around.
*
*
* optional uint64 new_value = 1;
* @return This builder for chaining.
*/
public Builder clearNewValue() {
bitField0_ = (bitField0_ & ~0x00000001);
newValue_ = 0L;
onChanged();
return this;
}
private int incrementStatus_ = 1;
/**
*
* Always set, unlike cacheserving_memcacheg::MemcacheIncrementResponse which
* is set only if part of BatchIncrement() call.
*
*
* optional .java.apphosting.MemcacheIncrementResponse.IncrementStatusCode increment_status = 2;
* @return Whether the incrementStatus field is set.
*/
@java.lang.Override public boolean hasIncrementStatus() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Always set, unlike cacheserving_memcacheg::MemcacheIncrementResponse which
* is set only if part of BatchIncrement() call.
*
*
* optional .java.apphosting.MemcacheIncrementResponse.IncrementStatusCode increment_status = 2;
* @return The incrementStatus.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode getIncrementStatus() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode result = com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode.forNumber(incrementStatus_);
return result == null ? com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode.OK : result;
}
/**
*
* Always set, unlike cacheserving_memcacheg::MemcacheIncrementResponse which
* is set only if part of BatchIncrement() call.
*
*
* optional .java.apphosting.MemcacheIncrementResponse.IncrementStatusCode increment_status = 2;
* @param value The incrementStatus to set.
* @return This builder for chaining.
*/
public Builder setIncrementStatus(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.IncrementStatusCode value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
incrementStatus_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Always set, unlike cacheserving_memcacheg::MemcacheIncrementResponse which
* is set only if part of BatchIncrement() call.
*
*
* optional .java.apphosting.MemcacheIncrementResponse.IncrementStatusCode increment_status = 2;
* @return This builder for chaining.
*/
public Builder clearIncrementStatus() {
bitField0_ = (bitField0_ & ~0x00000002);
incrementStatus_ = 1;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheIncrementResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheIncrementResponse)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheIncrementResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheBatchIncrementRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheBatchIncrementRequest)
com.google.protobuf.MessageOrBuilder {
/**
* optional string name_space = 1 [default = ""];
* @return Whether the nameSpace field is set.
*/
boolean hasNameSpace();
/**
* optional string name_space = 1 [default = ""];
* @return The nameSpace.
*/
java.lang.String getNameSpace();
/**
* optional string name_space = 1 [default = ""];
* @return The bytes for nameSpace.
*/
com.google.protobuf.ByteString
getNameSpaceBytes();
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
java.util.List
getItemList();
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest getItem(int index);
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
int getItemCount();
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder>
getItemOrBuilderList();
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder getItemOrBuilder(
int index);
/**
* optional .java.apphosting.AppOverride override = 3;
* @return Whether the override field is set.
*/
boolean hasOverride();
/**
* optional .java.apphosting.AppOverride override = 3;
* @return The override.
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride();
/**
* optional .java.apphosting.AppOverride override = 3;
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder();
}
/**
*
* Allow for multiple increment/decrements in parallel. Combined with the
* 'initial_value' field of the MemcacheIncrementRequest message, this can
* be used to track a large set of counters with low-latency.
*
*
* Protobuf type {@code java.apphosting.MemcacheBatchIncrementRequest}
*/
public static final class MemcacheBatchIncrementRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheBatchIncrementRequest)
MemcacheBatchIncrementRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheBatchIncrementRequest.newBuilder() to construct.
private MemcacheBatchIncrementRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheBatchIncrementRequest() {
nameSpace_ = "";
item_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheBatchIncrementRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest.Builder.class);
}
private int bitField0_;
public static final int NAME_SPACE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 1 [default = ""];
* @return Whether the nameSpace field is set.
*/
@java.lang.Override
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name_space = 1 [default = ""];
* @return The nameSpace.
*/
@java.lang.Override
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
}
}
/**
* optional string name_space = 1 [default = ""];
* @return The bytes for nameSpace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ITEM_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List item_;
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
@java.lang.Override
public java.util.List getItemList() {
return item_;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
@java.lang.Override
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder>
getItemOrBuilderList() {
return item_;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
@java.lang.Override
public int getItemCount() {
return item_.size();
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest getItem(int index) {
return item_.get(index);
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder getItemOrBuilder(
int index) {
return item_.get(index);
}
public static final int OVERRIDE_FIELD_NUMBER = 3;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
/**
* optional .java.apphosting.AppOverride override = 3;
* @return Whether the override field is set.
*/
@java.lang.Override
public boolean hasOverride() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 3;
* @return The override.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
/**
* optional .java.apphosting.AppOverride override = 3;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getItemCount(); i++) {
if (!getItem(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasOverride()) {
if (!getOverride().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, nameSpace_);
}
for (int i = 0; i < item_.size(); i++) {
output.writeMessage(2, item_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getOverride());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, nameSpace_);
}
for (int i = 0; i < item_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, item_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getOverride());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest) obj;
if (hasNameSpace() != other.hasNameSpace()) return false;
if (hasNameSpace()) {
if (!getNameSpace()
.equals(other.getNameSpace())) return false;
}
if (!getItemList()
.equals(other.getItemList())) return false;
if (hasOverride() != other.hasOverride()) return false;
if (hasOverride()) {
if (!getOverride()
.equals(other.getOverride())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasNameSpace()) {
hash = (37 * hash) + NAME_SPACE_FIELD_NUMBER;
hash = (53 * hash) + getNameSpace().hashCode();
}
if (getItemCount() > 0) {
hash = (37 * hash) + ITEM_FIELD_NUMBER;
hash = (53 * hash) + getItemList().hashCode();
}
if (hasOverride()) {
hash = (37 * hash) + OVERRIDE_FIELD_NUMBER;
hash = (53 * hash) + getOverride().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Allow for multiple increment/decrements in parallel. Combined with the
* 'initial_value' field of the MemcacheIncrementRequest message, this can
* be used to track a large set of counters with low-latency.
*
*
* Protobuf type {@code java.apphosting.MemcacheBatchIncrementRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheBatchIncrementRequest)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getItemFieldBuilder();
getOverrideFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
nameSpace_ = "";
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
} else {
item_ = null;
itemBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementRequest_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest result) {
if (itemBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.nameSpace_ = nameSpace_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.override_ = overrideBuilder_ == null
? override_
: overrideBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest.getDefaultInstance()) return this;
if (other.hasNameSpace()) {
nameSpace_ = other.nameSpace_;
bitField0_ |= 0x00000001;
onChanged();
}
if (itemBuilder_ == null) {
if (!other.item_.isEmpty()) {
if (item_.isEmpty()) {
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureItemIsMutable();
item_.addAll(other.item_);
}
onChanged();
}
} else {
if (!other.item_.isEmpty()) {
if (itemBuilder_.isEmpty()) {
itemBuilder_.dispose();
itemBuilder_ = null;
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000002);
itemBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getItemFieldBuilder() : null;
} else {
itemBuilder_.addAllMessages(other.item_);
}
}
}
if (other.hasOverride()) {
mergeOverride(other.getOverride());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getItemCount(); i++) {
if (!getItem(i).isInitialized()) {
return false;
}
}
if (hasOverride()) {
if (!getOverride().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
nameSpace_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest m =
input.readMessage(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.PARSER,
extensionRegistry);
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(m);
} else {
itemBuilder_.addMessage(m);
}
break;
} // case 18
case 26: {
input.readMessage(
getOverrideFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object nameSpace_ = "";
/**
* optional string name_space = 1 [default = ""];
* @return Whether the nameSpace field is set.
*/
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string name_space = 1 [default = ""];
* @return The nameSpace.
*/
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name_space = 1 [default = ""];
* @return The bytes for nameSpace.
*/
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name_space = 1 [default = ""];
* @param value The nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpace(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional string name_space = 1 [default = ""];
* @return This builder for chaining.
*/
public Builder clearNameSpace() {
nameSpace_ = getDefaultInstance().getNameSpace();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* optional string name_space = 1 [default = ""];
* @param value The bytes for nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.util.List item_ =
java.util.Collections.emptyList();
private void ensureItemIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
item_ = new java.util.ArrayList(item_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder> itemBuilder_;
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public java.util.List getItemList() {
if (itemBuilder_ == null) {
return java.util.Collections.unmodifiableList(item_);
} else {
return itemBuilder_.getMessageList();
}
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public int getItemCount() {
if (itemBuilder_ == null) {
return item_.size();
} else {
return itemBuilder_.getCount();
}
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest getItem(int index) {
if (itemBuilder_ == null) {
return item_.get(index);
} else {
return itemBuilder_.getMessage(index);
}
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.set(index, value);
onChanged();
} else {
itemBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.set(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder addItem(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(value);
onChanged();
} else {
itemBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(index, value);
onChanged();
} else {
itemBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder addItem(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder addAllItem(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest> values) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, item_);
onChanged();
} else {
itemBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
itemBuilder_.clear();
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public Builder removeItem(int index) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.remove(index);
onChanged();
} else {
itemBuilder_.remove(index);
}
return this;
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder getItemBuilder(
int index) {
return getItemFieldBuilder().getBuilder(index);
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder getItemOrBuilder(
int index) {
if (itemBuilder_ == null) {
return item_.get(index); } else {
return itemBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder>
getItemOrBuilderList() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(item_);
}
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder addItemBuilder() {
return getItemFieldBuilder().addBuilder(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.getDefaultInstance());
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder addItemBuilder(
int index) {
return getItemFieldBuilder().addBuilder(
index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.getDefaultInstance());
}
/**
* repeated .java.apphosting.MemcacheIncrementRequest item = 2;
*/
public java.util.List
getItemBuilderList() {
return getItemFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequest.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementRequestOrBuilder>(
item_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder> overrideBuilder_;
/**
* optional .java.apphosting.AppOverride override = 3;
* @return Whether the override field is set.
*/
public boolean hasOverride() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .java.apphosting.AppOverride override = 3;
* @return The override.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
if (overrideBuilder_ == null) {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
} else {
return overrideBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.AppOverride override = 3;
*/
public Builder setOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
override_ = value;
} else {
overrideBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 3;
*/
public Builder setOverride(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder builderForValue) {
if (overrideBuilder_ == null) {
override_ = builderForValue.build();
} else {
overrideBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 3;
*/
public Builder mergeOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
override_ != null &&
override_ != com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance()) {
getOverrideBuilder().mergeFrom(value);
} else {
override_ = value;
}
} else {
overrideBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 3;
*/
public Builder clearOverride() {
bitField0_ = (bitField0_ & ~0x00000004);
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.AppOverride override = 3;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder getOverrideBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getOverrideFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.AppOverride override = 3;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
if (overrideBuilder_ != null) {
return overrideBuilder_.getMessageOrBuilder();
} else {
return override_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
}
/**
* optional .java.apphosting.AppOverride override = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>
getOverrideFieldBuilder() {
if (overrideBuilder_ == null) {
overrideBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>(
getOverride(),
getParentForChildren(),
isClean());
override_ = null;
}
return overrideBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheBatchIncrementRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheBatchIncrementRequest)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheBatchIncrementRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheBatchIncrementResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheBatchIncrementResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
java.util.List
getItemList();
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse getItem(int index);
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
int getItemCount();
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder>
getItemOrBuilderList();
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder getItemOrBuilder(
int index);
}
/**
* Protobuf type {@code java.apphosting.MemcacheBatchIncrementResponse}
*/
public static final class MemcacheBatchIncrementResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheBatchIncrementResponse)
MemcacheBatchIncrementResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheBatchIncrementResponse.newBuilder() to construct.
private MemcacheBatchIncrementResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheBatchIncrementResponse() {
item_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheBatchIncrementResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse.Builder.class);
}
public static final int ITEM_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List item_;
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
@java.lang.Override
public java.util.List getItemList() {
return item_;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
@java.lang.Override
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder>
getItemOrBuilderList() {
return item_;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
@java.lang.Override
public int getItemCount() {
return item_.size();
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse getItem(int index) {
return item_.get(index);
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder getItemOrBuilder(
int index) {
return item_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < item_.size(); i++) {
output.writeMessage(1, item_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < item_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, item_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse) obj;
if (!getItemList()
.equals(other.getItemList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getItemCount() > 0) {
hash = (37 * hash) + ITEM_FIELD_NUMBER;
hash = (53 * hash) + getItemList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheBatchIncrementResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheBatchIncrementResponse)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
} else {
item_ = null;
itemBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheBatchIncrementResponse_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse result) {
if (itemBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
item_ = java.util.Collections.unmodifiableList(item_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.item_ = item_;
} else {
result.item_ = itemBuilder_.build();
}
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse.getDefaultInstance()) return this;
if (itemBuilder_ == null) {
if (!other.item_.isEmpty()) {
if (item_.isEmpty()) {
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureItemIsMutable();
item_.addAll(other.item_);
}
onChanged();
}
} else {
if (!other.item_.isEmpty()) {
if (itemBuilder_.isEmpty()) {
itemBuilder_.dispose();
itemBuilder_ = null;
item_ = other.item_;
bitField0_ = (bitField0_ & ~0x00000001);
itemBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getItemFieldBuilder() : null;
} else {
itemBuilder_.addAllMessages(other.item_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse m =
input.readMessage(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.PARSER,
extensionRegistry);
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(m);
} else {
itemBuilder_.addMessage(m);
}
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List item_ =
java.util.Collections.emptyList();
private void ensureItemIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
item_ = new java.util.ArrayList(item_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder> itemBuilder_;
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public java.util.List getItemList() {
if (itemBuilder_ == null) {
return java.util.Collections.unmodifiableList(item_);
} else {
return itemBuilder_.getMessageList();
}
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public int getItemCount() {
if (itemBuilder_ == null) {
return item_.size();
} else {
return itemBuilder_.getCount();
}
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse getItem(int index) {
if (itemBuilder_ == null) {
return item_.get(index);
} else {
return itemBuilder_.getMessage(index);
}
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.set(index, value);
onChanged();
} else {
itemBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder setItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.set(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder addItem(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(value);
onChanged();
} else {
itemBuilder_.addMessage(value);
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse value) {
if (itemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureItemIsMutable();
item_.add(index, value);
onChanged();
} else {
itemBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder addItem(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder addItem(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder builderForValue) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.add(index, builderForValue.build());
onChanged();
} else {
itemBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder addAllItem(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse> values) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, item_);
onChanged();
} else {
itemBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder clearItem() {
if (itemBuilder_ == null) {
item_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
itemBuilder_.clear();
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public Builder removeItem(int index) {
if (itemBuilder_ == null) {
ensureItemIsMutable();
item_.remove(index);
onChanged();
} else {
itemBuilder_.remove(index);
}
return this;
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder getItemBuilder(
int index) {
return getItemFieldBuilder().getBuilder(index);
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder getItemOrBuilder(
int index) {
if (itemBuilder_ == null) {
return item_.get(index); } else {
return itemBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder>
getItemOrBuilderList() {
if (itemBuilder_ != null) {
return itemBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(item_);
}
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder addItemBuilder() {
return getItemFieldBuilder().addBuilder(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.getDefaultInstance());
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder addItemBuilder(
int index) {
return getItemFieldBuilder().addBuilder(
index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.getDefaultInstance());
}
/**
*
* One 'item' will always be returned in the response for each 'item' in the
* request, with no exceptions.
*
*
* repeated .java.apphosting.MemcacheIncrementResponse item = 1;
*/
public java.util.List
getItemBuilderList() {
return getItemFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder>
getItemFieldBuilder() {
if (itemBuilder_ == null) {
itemBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponse.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheIncrementResponseOrBuilder>(
item_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
item_ = null;
}
return itemBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheBatchIncrementResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheBatchIncrementResponse)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheBatchIncrementResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheBatchIncrementResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheFlushRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheFlushRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return Whether the override field is set.
*/
boolean hasOverride();
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return The override.
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride();
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder();
}
/**
* Protobuf type {@code java.apphosting.MemcacheFlushRequest}
*/
public static final class MemcacheFlushRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheFlushRequest)
MemcacheFlushRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheFlushRequest.newBuilder() to construct.
private MemcacheFlushRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheFlushRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheFlushRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest.Builder.class);
}
private int bitField0_;
public static final int OVERRIDE_FIELD_NUMBER = 1;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return Whether the override field is set.
*/
@java.lang.Override
public boolean hasOverride() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return The override.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasOverride()) {
if (!getOverride().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getOverride());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getOverride());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest) obj;
if (hasOverride() != other.hasOverride()) return false;
if (hasOverride()) {
if (!getOverride()
.equals(other.getOverride())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasOverride()) {
hash = (37 * hash) + OVERRIDE_FIELD_NUMBER;
hash = (53 * hash) + getOverride().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheFlushRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheFlushRequest)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOverrideFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushRequest_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.override_ = overrideBuilder_ == null
? override_
: overrideBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest.getDefaultInstance()) return this;
if (other.hasOverride()) {
mergeOverride(other.getOverride());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasOverride()) {
if (!getOverride().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getOverrideFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder> overrideBuilder_;
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return Whether the override field is set.
*/
public boolean hasOverride() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return The override.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
if (overrideBuilder_ == null) {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
} else {
return overrideBuilder_.getMessage();
}
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public Builder setOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
override_ = value;
} else {
overrideBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public Builder setOverride(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder builderForValue) {
if (overrideBuilder_ == null) {
override_ = builderForValue.build();
} else {
overrideBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public Builder mergeOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
override_ != null &&
override_ != com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance()) {
getOverrideBuilder().mergeFrom(value);
} else {
override_ = value;
}
} else {
overrideBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public Builder clearOverride() {
bitField0_ = (bitField0_ & ~0x00000001);
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder getOverrideBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getOverrideFieldBuilder().getBuilder();
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
if (overrideBuilder_ != null) {
return overrideBuilder_.getMessageOrBuilder();
} else {
return override_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
}
/**
*
* Note: There is no name_space parameter. This request flushes
* all memcache data for an app.
* Note: we don't support upstream's flush_all 'time' parameter
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>
getOverrideFieldBuilder() {
if (overrideBuilder_ == null) {
overrideBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>(
getOverride(),
getParentForChildren(),
isClean());
override_ = null;
}
return overrideBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheFlushRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheFlushRequest)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheFlushRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheFlushResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheFlushResponse)
com.google.protobuf.MessageOrBuilder {
}
/**
*
* This space intentionally left blank. Reserved for future
* expansion.
*
*
* Protobuf type {@code java.apphosting.MemcacheFlushResponse}
*/
public static final class MemcacheFlushResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheFlushResponse)
MemcacheFlushResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheFlushResponse.newBuilder() to construct.
private MemcacheFlushResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheFlushResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheFlushResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse.Builder.class);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* This space intentionally left blank. Reserved for future
* expansion.
*
*
* Protobuf type {@code java.apphosting.MemcacheFlushResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheFlushResponse)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheFlushResponse_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheFlushResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheFlushResponse)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheFlushResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheFlushResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheStatsRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheStatsRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return Whether the override field is set.
*/
boolean hasOverride();
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return The override.
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride();
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder();
/**
*
* Number of hot key requested.
*
*
* optional int32 max_hotkey_count = 2 [default = 0];
* @return Whether the maxHotkeyCount field is set.
*/
boolean hasMaxHotkeyCount();
/**
*
* Number of hot key requested.
*
*
* optional int32 max_hotkey_count = 2 [default = 0];
* @return The maxHotkeyCount.
*/
int getMaxHotkeyCount();
}
/**
* Protobuf type {@code java.apphosting.MemcacheStatsRequest}
*/
public static final class MemcacheStatsRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheStatsRequest)
MemcacheStatsRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheStatsRequest.newBuilder() to construct.
private MemcacheStatsRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheStatsRequest() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheStatsRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest.Builder.class);
}
private int bitField0_;
public static final int OVERRIDE_FIELD_NUMBER = 1;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return Whether the override field is set.
*/
@java.lang.Override
public boolean hasOverride() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return The override.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
public static final int MAX_HOTKEY_COUNT_FIELD_NUMBER = 2;
private int maxHotkeyCount_ = 0;
/**
*
* Number of hot key requested.
*
*
* optional int32 max_hotkey_count = 2 [default = 0];
* @return Whether the maxHotkeyCount field is set.
*/
@java.lang.Override
public boolean hasMaxHotkeyCount() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Number of hot key requested.
*
*
* optional int32 max_hotkey_count = 2 [default = 0];
* @return The maxHotkeyCount.
*/
@java.lang.Override
public int getMaxHotkeyCount() {
return maxHotkeyCount_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasOverride()) {
if (!getOverride().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getOverride());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(2, maxHotkeyCount_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getOverride());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, maxHotkeyCount_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest) obj;
if (hasOverride() != other.hasOverride()) return false;
if (hasOverride()) {
if (!getOverride()
.equals(other.getOverride())) return false;
}
if (hasMaxHotkeyCount() != other.hasMaxHotkeyCount()) return false;
if (hasMaxHotkeyCount()) {
if (getMaxHotkeyCount()
!= other.getMaxHotkeyCount()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasOverride()) {
hash = (37 * hash) + OVERRIDE_FIELD_NUMBER;
hash = (53 * hash) + getOverride().hashCode();
}
if (hasMaxHotkeyCount()) {
hash = (37 * hash) + MAX_HOTKEY_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getMaxHotkeyCount();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheStatsRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheStatsRequest)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getOverrideFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
maxHotkeyCount_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsRequest_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.override_ = overrideBuilder_ == null
? override_
: overrideBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.maxHotkeyCount_ = maxHotkeyCount_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest.getDefaultInstance()) return this;
if (other.hasOverride()) {
mergeOverride(other.getOverride());
}
if (other.hasMaxHotkeyCount()) {
setMaxHotkeyCount(other.getMaxHotkeyCount());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasOverride()) {
if (!getOverride().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getOverrideFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
maxHotkeyCount_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.appengine.api.memcache.MemcacheServicePb.AppOverride override_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder> overrideBuilder_;
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return Whether the override field is set.
*/
public boolean hasOverride() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
* @return The override.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride getOverride() {
if (overrideBuilder_ == null) {
return override_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
} else {
return overrideBuilder_.getMessage();
}
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public Builder setOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
override_ = value;
} else {
overrideBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public Builder setOverride(
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder builderForValue) {
if (overrideBuilder_ == null) {
override_ = builderForValue.build();
} else {
overrideBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public Builder mergeOverride(com.google.appengine.api.memcache.MemcacheServicePb.AppOverride value) {
if (overrideBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
override_ != null &&
override_ != com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance()) {
getOverrideBuilder().mergeFrom(value);
} else {
override_ = value;
}
} else {
overrideBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public Builder clearOverride() {
bitField0_ = (bitField0_ & ~0x00000001);
override_ = null;
if (overrideBuilder_ != null) {
overrideBuilder_.dispose();
overrideBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder getOverrideBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getOverrideFieldBuilder().getBuilder();
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder getOverrideOrBuilder() {
if (overrideBuilder_ != null) {
return overrideBuilder_.getMessageOrBuilder();
} else {
return override_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.getDefaultInstance() : override_;
}
}
/**
*
* N.B.(jackkelly): Stats for the entire app are returned, regardless of which
* namespaces (if any) are used.
*
*
* optional .java.apphosting.AppOverride override = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>
getOverrideFieldBuilder() {
if (overrideBuilder_ == null) {
overrideBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.AppOverride, com.google.appengine.api.memcache.MemcacheServicePb.AppOverride.Builder, com.google.appengine.api.memcache.MemcacheServicePb.AppOverrideOrBuilder>(
getOverride(),
getParentForChildren(),
isClean());
override_ = null;
}
return overrideBuilder_;
}
private int maxHotkeyCount_ ;
/**
*
* Number of hot key requested.
*
*
* optional int32 max_hotkey_count = 2 [default = 0];
* @return Whether the maxHotkeyCount field is set.
*/
@java.lang.Override
public boolean hasMaxHotkeyCount() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Number of hot key requested.
*
*
* optional int32 max_hotkey_count = 2 [default = 0];
* @return The maxHotkeyCount.
*/
@java.lang.Override
public int getMaxHotkeyCount() {
return maxHotkeyCount_;
}
/**
*
* Number of hot key requested.
*
*
* optional int32 max_hotkey_count = 2 [default = 0];
* @param value The maxHotkeyCount to set.
* @return This builder for chaining.
*/
public Builder setMaxHotkeyCount(int value) {
maxHotkeyCount_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Number of hot key requested.
*
*
* optional int32 max_hotkey_count = 2 [default = 0];
* @return This builder for chaining.
*/
public Builder clearMaxHotkeyCount() {
bitField0_ = (bitField0_ & ~0x00000002);
maxHotkeyCount_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheStatsRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheStatsRequest)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheStatsRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MergedNamespaceStatsOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MergedNamespaceStats)
com.google.protobuf.MessageOrBuilder {
/**
*
* Counters: (only increase, except when stats reset)
*
*
* required uint64 hits = 1;
* @return Whether the hits field is set.
*/
boolean hasHits();
/**
*
* Counters: (only increase, except when stats reset)
*
*
* required uint64 hits = 1;
* @return The hits.
*/
long getHits();
/**
* required uint64 misses = 2;
* @return Whether the misses field is set.
*/
boolean hasMisses();
/**
* required uint64 misses = 2;
* @return The misses.
*/
long getMisses();
/**
*
* bytes transferred on gets
*
*
* required uint64 byte_hits = 3;
* @return Whether the byteHits field is set.
*/
boolean hasByteHits();
/**
*
* bytes transferred on gets
*
*
* required uint64 byte_hits = 3;
* @return The byteHits.
*/
long getByteHits();
/**
*
* Not counters:
*
*
* required uint64 items = 4;
* @return Whether the items field is set.
*/
boolean hasItems();
/**
*
* Not counters:
*
*
* required uint64 items = 4;
* @return The items.
*/
long getItems();
/**
* required uint64 bytes = 5;
* @return Whether the bytes field is set.
*/
boolean hasBytes();
/**
* required uint64 bytes = 5;
* @return The bytes.
*/
long getBytes();
/**
*
* How long (in seconds) it's been since the oldest item in the
* namespace's LRU chain has been accessed. This is how long a new
* item can currently be put in the cache and survive without being
* accessed. This is _not_ about the time since the item was
* created, but how long it's been since it was accessed.
*
*
* required fixed32 oldest_item_age = 6;
* @return Whether the oldestItemAge field is set.
*/
boolean hasOldestItemAge();
/**
*
* How long (in seconds) it's been since the oldest item in the
* namespace's LRU chain has been accessed. This is how long a new
* item can currently be put in the cache and survive without being
* accessed. This is _not_ about the time since the item was
* created, but how long it's been since it was accessed.
*
*
* required fixed32 oldest_item_age = 6;
* @return The oldestItemAge.
*/
int getOldestItemAge();
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
java.util.List
getHotkeysList();
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey getHotkeys(int index);
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
int getHotkeysCount();
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder>
getHotkeysOrBuilderList();
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder getHotkeysOrBuilder(
int index);
}
/**
*
* This is a merge of all the NamespaceStats for each of the namespaces owned by
* the requesting application.
*
*
* Protobuf type {@code java.apphosting.MergedNamespaceStats}
*/
public static final class MergedNamespaceStats extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MergedNamespaceStats)
MergedNamespaceStatsOrBuilder {
private static final long serialVersionUID = 0L;
// Use MergedNamespaceStats.newBuilder() to construct.
private MergedNamespaceStats(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MergedNamespaceStats() {
hotkeys_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MergedNamespaceStats();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MergedNamespaceStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MergedNamespaceStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.class, com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.Builder.class);
}
private int bitField0_;
public static final int HITS_FIELD_NUMBER = 1;
private long hits_ = 0L;
/**
*
* Counters: (only increase, except when stats reset)
*
*
* required uint64 hits = 1;
* @return Whether the hits field is set.
*/
@java.lang.Override
public boolean hasHits() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Counters: (only increase, except when stats reset)
*
*
* required uint64 hits = 1;
* @return The hits.
*/
@java.lang.Override
public long getHits() {
return hits_;
}
public static final int MISSES_FIELD_NUMBER = 2;
private long misses_ = 0L;
/**
* required uint64 misses = 2;
* @return Whether the misses field is set.
*/
@java.lang.Override
public boolean hasMisses() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required uint64 misses = 2;
* @return The misses.
*/
@java.lang.Override
public long getMisses() {
return misses_;
}
public static final int BYTE_HITS_FIELD_NUMBER = 3;
private long byteHits_ = 0L;
/**
*
* bytes transferred on gets
*
*
* required uint64 byte_hits = 3;
* @return Whether the byteHits field is set.
*/
@java.lang.Override
public boolean hasByteHits() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* bytes transferred on gets
*
*
* required uint64 byte_hits = 3;
* @return The byteHits.
*/
@java.lang.Override
public long getByteHits() {
return byteHits_;
}
public static final int ITEMS_FIELD_NUMBER = 4;
private long items_ = 0L;
/**
*
* Not counters:
*
*
* required uint64 items = 4;
* @return Whether the items field is set.
*/
@java.lang.Override
public boolean hasItems() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Not counters:
*
*
* required uint64 items = 4;
* @return The items.
*/
@java.lang.Override
public long getItems() {
return items_;
}
public static final int BYTES_FIELD_NUMBER = 5;
private long bytes_ = 0L;
/**
* required uint64 bytes = 5;
* @return Whether the bytes field is set.
*/
@java.lang.Override
public boolean hasBytes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* required uint64 bytes = 5;
* @return The bytes.
*/
@java.lang.Override
public long getBytes() {
return bytes_;
}
public static final int OLDEST_ITEM_AGE_FIELD_NUMBER = 6;
private int oldestItemAge_ = 0;
/**
*
* How long (in seconds) it's been since the oldest item in the
* namespace's LRU chain has been accessed. This is how long a new
* item can currently be put in the cache and survive without being
* accessed. This is _not_ about the time since the item was
* created, but how long it's been since it was accessed.
*
*
* required fixed32 oldest_item_age = 6;
* @return Whether the oldestItemAge field is set.
*/
@java.lang.Override
public boolean hasOldestItemAge() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* How long (in seconds) it's been since the oldest item in the
* namespace's LRU chain has been accessed. This is how long a new
* item can currently be put in the cache and survive without being
* accessed. This is _not_ about the time since the item was
* created, but how long it's been since it was accessed.
*
*
* required fixed32 oldest_item_age = 6;
* @return The oldestItemAge.
*/
@java.lang.Override
public int getOldestItemAge() {
return oldestItemAge_;
}
public static final int HOTKEYS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List hotkeys_;
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
@java.lang.Override
public java.util.List getHotkeysList() {
return hotkeys_;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
@java.lang.Override
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder>
getHotkeysOrBuilderList() {
return hotkeys_;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
@java.lang.Override
public int getHotkeysCount() {
return hotkeys_.size();
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey getHotkeys(int index) {
return hotkeys_.get(index);
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder getHotkeysOrBuilder(
int index) {
return hotkeys_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasHits()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasMisses()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasByteHits()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasItems()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasBytes()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasOldestItemAge()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getHotkeysCount(); i++) {
if (!getHotkeys(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeUInt64(1, hits_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeUInt64(2, misses_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeUInt64(3, byteHits_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeUInt64(4, items_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeUInt64(5, bytes_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeFixed32(6, oldestItemAge_);
}
for (int i = 0; i < hotkeys_.size(); i++) {
output.writeMessage(7, hotkeys_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(1, hits_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, misses_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, byteHits_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(4, items_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(5, bytes_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed32Size(6, oldestItemAge_);
}
for (int i = 0; i < hotkeys_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, hotkeys_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats other = (com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats) obj;
if (hasHits() != other.hasHits()) return false;
if (hasHits()) {
if (getHits()
!= other.getHits()) return false;
}
if (hasMisses() != other.hasMisses()) return false;
if (hasMisses()) {
if (getMisses()
!= other.getMisses()) return false;
}
if (hasByteHits() != other.hasByteHits()) return false;
if (hasByteHits()) {
if (getByteHits()
!= other.getByteHits()) return false;
}
if (hasItems() != other.hasItems()) return false;
if (hasItems()) {
if (getItems()
!= other.getItems()) return false;
}
if (hasBytes() != other.hasBytes()) return false;
if (hasBytes()) {
if (getBytes()
!= other.getBytes()) return false;
}
if (hasOldestItemAge() != other.hasOldestItemAge()) return false;
if (hasOldestItemAge()) {
if (getOldestItemAge()
!= other.getOldestItemAge()) return false;
}
if (!getHotkeysList()
.equals(other.getHotkeysList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasHits()) {
hash = (37 * hash) + HITS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getHits());
}
if (hasMisses()) {
hash = (37 * hash) + MISSES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMisses());
}
if (hasByteHits()) {
hash = (37 * hash) + BYTE_HITS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getByteHits());
}
if (hasItems()) {
hash = (37 * hash) + ITEMS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getItems());
}
if (hasBytes()) {
hash = (37 * hash) + BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBytes());
}
if (hasOldestItemAge()) {
hash = (37 * hash) + OLDEST_ITEM_AGE_FIELD_NUMBER;
hash = (53 * hash) + getOldestItemAge();
}
if (getHotkeysCount() > 0) {
hash = (37 * hash) + HOTKEYS_FIELD_NUMBER;
hash = (53 * hash) + getHotkeysList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* This is a merge of all the NamespaceStats for each of the namespaces owned by
* the requesting application.
*
*
* Protobuf type {@code java.apphosting.MergedNamespaceStats}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MergedNamespaceStats)
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStatsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MergedNamespaceStats_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MergedNamespaceStats_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.class, com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
hits_ = 0L;
misses_ = 0L;
byteHits_ = 0L;
items_ = 0L;
bytes_ = 0L;
oldestItemAge_ = 0;
if (hotkeysBuilder_ == null) {
hotkeys_ = java.util.Collections.emptyList();
} else {
hotkeys_ = null;
hotkeysBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MergedNamespaceStats_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats build() {
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats result = new com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats result) {
if (hotkeysBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
hotkeys_ = java.util.Collections.unmodifiableList(hotkeys_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.hotkeys_ = hotkeys_;
} else {
result.hotkeys_ = hotkeysBuilder_.build();
}
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.hits_ = hits_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.misses_ = misses_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.byteHits_ = byteHits_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.items_ = items_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.bytes_ = bytes_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.oldestItemAge_ = oldestItemAge_;
to_bitField0_ |= 0x00000020;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.getDefaultInstance()) return this;
if (other.hasHits()) {
setHits(other.getHits());
}
if (other.hasMisses()) {
setMisses(other.getMisses());
}
if (other.hasByteHits()) {
setByteHits(other.getByteHits());
}
if (other.hasItems()) {
setItems(other.getItems());
}
if (other.hasBytes()) {
setBytes(other.getBytes());
}
if (other.hasOldestItemAge()) {
setOldestItemAge(other.getOldestItemAge());
}
if (hotkeysBuilder_ == null) {
if (!other.hotkeys_.isEmpty()) {
if (hotkeys_.isEmpty()) {
hotkeys_ = other.hotkeys_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureHotkeysIsMutable();
hotkeys_.addAll(other.hotkeys_);
}
onChanged();
}
} else {
if (!other.hotkeys_.isEmpty()) {
if (hotkeysBuilder_.isEmpty()) {
hotkeysBuilder_.dispose();
hotkeysBuilder_ = null;
hotkeys_ = other.hotkeys_;
bitField0_ = (bitField0_ & ~0x00000040);
hotkeysBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getHotkeysFieldBuilder() : null;
} else {
hotkeysBuilder_.addAllMessages(other.hotkeys_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasHits()) {
return false;
}
if (!hasMisses()) {
return false;
}
if (!hasByteHits()) {
return false;
}
if (!hasItems()) {
return false;
}
if (!hasBytes()) {
return false;
}
if (!hasOldestItemAge()) {
return false;
}
for (int i = 0; i < getHotkeysCount(); i++) {
if (!getHotkeys(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
hits_ = input.readUInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
misses_ = input.readUInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
byteHits_ = input.readUInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
items_ = input.readUInt64();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
bytes_ = input.readUInt64();
bitField0_ |= 0x00000010;
break;
} // case 40
case 53: {
oldestItemAge_ = input.readFixed32();
bitField0_ |= 0x00000020;
break;
} // case 53
case 58: {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey m =
input.readMessage(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.PARSER,
extensionRegistry);
if (hotkeysBuilder_ == null) {
ensureHotkeysIsMutable();
hotkeys_.add(m);
} else {
hotkeysBuilder_.addMessage(m);
}
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long hits_ ;
/**
*
* Counters: (only increase, except when stats reset)
*
*
* required uint64 hits = 1;
* @return Whether the hits field is set.
*/
@java.lang.Override
public boolean hasHits() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Counters: (only increase, except when stats reset)
*
*
* required uint64 hits = 1;
* @return The hits.
*/
@java.lang.Override
public long getHits() {
return hits_;
}
/**
*
* Counters: (only increase, except when stats reset)
*
*
* required uint64 hits = 1;
* @param value The hits to set.
* @return This builder for chaining.
*/
public Builder setHits(long value) {
hits_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Counters: (only increase, except when stats reset)
*
*
* required uint64 hits = 1;
* @return This builder for chaining.
*/
public Builder clearHits() {
bitField0_ = (bitField0_ & ~0x00000001);
hits_ = 0L;
onChanged();
return this;
}
private long misses_ ;
/**
* required uint64 misses = 2;
* @return Whether the misses field is set.
*/
@java.lang.Override
public boolean hasMisses() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required uint64 misses = 2;
* @return The misses.
*/
@java.lang.Override
public long getMisses() {
return misses_;
}
/**
* required uint64 misses = 2;
* @param value The misses to set.
* @return This builder for chaining.
*/
public Builder setMisses(long value) {
misses_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required uint64 misses = 2;
* @return This builder for chaining.
*/
public Builder clearMisses() {
bitField0_ = (bitField0_ & ~0x00000002);
misses_ = 0L;
onChanged();
return this;
}
private long byteHits_ ;
/**
*
* bytes transferred on gets
*
*
* required uint64 byte_hits = 3;
* @return Whether the byteHits field is set.
*/
@java.lang.Override
public boolean hasByteHits() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* bytes transferred on gets
*
*
* required uint64 byte_hits = 3;
* @return The byteHits.
*/
@java.lang.Override
public long getByteHits() {
return byteHits_;
}
/**
*
* bytes transferred on gets
*
*
* required uint64 byte_hits = 3;
* @param value The byteHits to set.
* @return This builder for chaining.
*/
public Builder setByteHits(long value) {
byteHits_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* bytes transferred on gets
*
*
* required uint64 byte_hits = 3;
* @return This builder for chaining.
*/
public Builder clearByteHits() {
bitField0_ = (bitField0_ & ~0x00000004);
byteHits_ = 0L;
onChanged();
return this;
}
private long items_ ;
/**
*
* Not counters:
*
*
* required uint64 items = 4;
* @return Whether the items field is set.
*/
@java.lang.Override
public boolean hasItems() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Not counters:
*
*
* required uint64 items = 4;
* @return The items.
*/
@java.lang.Override
public long getItems() {
return items_;
}
/**
*
* Not counters:
*
*
* required uint64 items = 4;
* @param value The items to set.
* @return This builder for chaining.
*/
public Builder setItems(long value) {
items_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Not counters:
*
*
* required uint64 items = 4;
* @return This builder for chaining.
*/
public Builder clearItems() {
bitField0_ = (bitField0_ & ~0x00000008);
items_ = 0L;
onChanged();
return this;
}
private long bytes_ ;
/**
* required uint64 bytes = 5;
* @return Whether the bytes field is set.
*/
@java.lang.Override
public boolean hasBytes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* required uint64 bytes = 5;
* @return The bytes.
*/
@java.lang.Override
public long getBytes() {
return bytes_;
}
/**
* required uint64 bytes = 5;
* @param value The bytes to set.
* @return This builder for chaining.
*/
public Builder setBytes(long value) {
bytes_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* required uint64 bytes = 5;
* @return This builder for chaining.
*/
public Builder clearBytes() {
bitField0_ = (bitField0_ & ~0x00000010);
bytes_ = 0L;
onChanged();
return this;
}
private int oldestItemAge_ ;
/**
*
* How long (in seconds) it's been since the oldest item in the
* namespace's LRU chain has been accessed. This is how long a new
* item can currently be put in the cache and survive without being
* accessed. This is _not_ about the time since the item was
* created, but how long it's been since it was accessed.
*
*
* required fixed32 oldest_item_age = 6;
* @return Whether the oldestItemAge field is set.
*/
@java.lang.Override
public boolean hasOldestItemAge() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* How long (in seconds) it's been since the oldest item in the
* namespace's LRU chain has been accessed. This is how long a new
* item can currently be put in the cache and survive without being
* accessed. This is _not_ about the time since the item was
* created, but how long it's been since it was accessed.
*
*
* required fixed32 oldest_item_age = 6;
* @return The oldestItemAge.
*/
@java.lang.Override
public int getOldestItemAge() {
return oldestItemAge_;
}
/**
*
* How long (in seconds) it's been since the oldest item in the
* namespace's LRU chain has been accessed. This is how long a new
* item can currently be put in the cache and survive without being
* accessed. This is _not_ about the time since the item was
* created, but how long it's been since it was accessed.
*
*
* required fixed32 oldest_item_age = 6;
* @param value The oldestItemAge to set.
* @return This builder for chaining.
*/
public Builder setOldestItemAge(int value) {
oldestItemAge_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* How long (in seconds) it's been since the oldest item in the
* namespace's LRU chain has been accessed. This is how long a new
* item can currently be put in the cache and survive without being
* accessed. This is _not_ about the time since the item was
* created, but how long it's been since it was accessed.
*
*
* required fixed32 oldest_item_age = 6;
* @return This builder for chaining.
*/
public Builder clearOldestItemAge() {
bitField0_ = (bitField0_ & ~0x00000020);
oldestItemAge_ = 0;
onChanged();
return this;
}
private java.util.List hotkeys_ =
java.util.Collections.emptyList();
private void ensureHotkeysIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
hotkeys_ = new java.util.ArrayList(hotkeys_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder> hotkeysBuilder_;
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public java.util.List getHotkeysList() {
if (hotkeysBuilder_ == null) {
return java.util.Collections.unmodifiableList(hotkeys_);
} else {
return hotkeysBuilder_.getMessageList();
}
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public int getHotkeysCount() {
if (hotkeysBuilder_ == null) {
return hotkeys_.size();
} else {
return hotkeysBuilder_.getCount();
}
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey getHotkeys(int index) {
if (hotkeysBuilder_ == null) {
return hotkeys_.get(index);
} else {
return hotkeysBuilder_.getMessage(index);
}
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder setHotkeys(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey value) {
if (hotkeysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHotkeysIsMutable();
hotkeys_.set(index, value);
onChanged();
} else {
hotkeysBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder setHotkeys(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder builderForValue) {
if (hotkeysBuilder_ == null) {
ensureHotkeysIsMutable();
hotkeys_.set(index, builderForValue.build());
onChanged();
} else {
hotkeysBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder addHotkeys(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey value) {
if (hotkeysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHotkeysIsMutable();
hotkeys_.add(value);
onChanged();
} else {
hotkeysBuilder_.addMessage(value);
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder addHotkeys(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey value) {
if (hotkeysBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHotkeysIsMutable();
hotkeys_.add(index, value);
onChanged();
} else {
hotkeysBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder addHotkeys(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder builderForValue) {
if (hotkeysBuilder_ == null) {
ensureHotkeysIsMutable();
hotkeys_.add(builderForValue.build());
onChanged();
} else {
hotkeysBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder addHotkeys(
int index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder builderForValue) {
if (hotkeysBuilder_ == null) {
ensureHotkeysIsMutable();
hotkeys_.add(index, builderForValue.build());
onChanged();
} else {
hotkeysBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder addAllHotkeys(
java.lang.Iterable extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey> values) {
if (hotkeysBuilder_ == null) {
ensureHotkeysIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, hotkeys_);
onChanged();
} else {
hotkeysBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder clearHotkeys() {
if (hotkeysBuilder_ == null) {
hotkeys_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
hotkeysBuilder_.clear();
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public Builder removeHotkeys(int index) {
if (hotkeysBuilder_ == null) {
ensureHotkeysIsMutable();
hotkeys_.remove(index);
onChanged();
} else {
hotkeysBuilder_.remove(index);
}
return this;
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder getHotkeysBuilder(
int index) {
return getHotkeysFieldBuilder().getBuilder(index);
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder getHotkeysOrBuilder(
int index) {
if (hotkeysBuilder_ == null) {
return hotkeys_.get(index); } else {
return hotkeysBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public java.util.List extends com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder>
getHotkeysOrBuilderList() {
if (hotkeysBuilder_ != null) {
return hotkeysBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(hotkeys_);
}
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder addHotkeysBuilder() {
return getHotkeysFieldBuilder().addBuilder(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.getDefaultInstance());
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder addHotkeysBuilder(
int index) {
return getHotkeysFieldBuilder().addBuilder(
index, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.getDefaultInstance());
}
/**
*
* Only set when hot keys are present and requested through stats.
*
*
* repeated .java.apphosting.MemcacheHotKey hotkeys = 7;
*/
public java.util.List
getHotkeysBuilderList() {
return getHotkeysFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder>
getHotkeysFieldBuilder() {
if (hotkeysBuilder_ == null) {
hotkeysBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder>(
hotkeys_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
hotkeys_ = null;
}
return hotkeysBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MergedNamespaceStats)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MergedNamespaceStats)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MergedNamespaceStats parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheHotKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheHotKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* Max 250 bytes.
*
*
* required bytes key = 1;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
*
* Max 250 bytes.
*
*
* required bytes key = 1;
* @return The key.
*/
com.google.protobuf.ByteString getKey();
/**
*
* A query is defined as an individual key operation, i.e. a single GET
* operation on one particular key. A memcache API call can contain multiple
* key operations in a single call.
*
*
* required double qps = 2;
* @return Whether the qps field is set.
*/
boolean hasQps();
/**
*
* A query is defined as an individual key operation, i.e. a single GET
* operation on one particular key. A memcache API call can contain multiple
* key operations in a single call.
*
*
* required double qps = 2;
* @return The qps.
*/
double getQps();
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return Whether the nameSpace field is set.
*/
boolean hasNameSpace();
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return The nameSpace.
*/
java.lang.String getNameSpace();
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return The bytes for nameSpace.
*/
com.google.protobuf.ByteString
getNameSpaceBytes();
}
/**
*
* Memcache key that has a hit rate higher than a certain threshold. This
* threshold is controlled by the flag --hotkey_threshold_qps in memcacheg.
*
*
* Protobuf type {@code java.apphosting.MemcacheHotKey}
*/
public static final class MemcacheHotKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheHotKey)
MemcacheHotKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheHotKey.newBuilder() to construct.
private MemcacheHotKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheHotKey() {
key_ = com.google.protobuf.ByteString.EMPTY;
nameSpace_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheHotKey();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheHotKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheHotKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Max 250 bytes.
*
*
* required bytes key = 1;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Max 250 bytes.
*
*
* required bytes key = 1;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
public static final int QPS_FIELD_NUMBER = 2;
private double qps_ = 0D;
/**
*
* A query is defined as an individual key operation, i.e. a single GET
* operation on one particular key. A memcache API call can contain multiple
* key operations in a single call.
*
*
* required double qps = 2;
* @return Whether the qps field is set.
*/
@java.lang.Override
public boolean hasQps() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* A query is defined as an individual key operation, i.e. a single GET
* operation on one particular key. A memcache API call can contain multiple
* key operations in a single call.
*
*
* required double qps = 2;
* @return The qps.
*/
@java.lang.Override
public double getQps() {
return qps_;
}
public static final int NAME_SPACE_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object nameSpace_ = "";
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return Whether the nameSpace field is set.
*/
@java.lang.Override
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return The nameSpace.
*/
@java.lang.Override
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
}
}
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return The bytes for nameSpace.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasQps()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBytes(1, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeDouble(2, qps_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, nameSpace_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, qps_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, nameSpace_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasQps() != other.hasQps()) return false;
if (hasQps()) {
if (java.lang.Double.doubleToLongBits(getQps())
!= java.lang.Double.doubleToLongBits(
other.getQps())) return false;
}
if (hasNameSpace() != other.hasNameSpace()) return false;
if (hasNameSpace()) {
if (!getNameSpace()
.equals(other.getNameSpace())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasQps()) {
hash = (37 * hash) + QPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getQps()));
}
if (hasNameSpace()) {
hash = (37 * hash) + NAME_SPACE_FIELD_NUMBER;
hash = (53 * hash) + getNameSpace().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Memcache key that has a hit rate higher than a certain threshold. This
* threshold is controlled by the flag --hotkey_threshold_qps in memcacheg.
*
*
* Protobuf type {@code java.apphosting.MemcacheHotKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheHotKey)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheHotKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheHotKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = com.google.protobuf.ByteString.EMPTY;
qps_ = 0D;
nameSpace_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheHotKey_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.qps_ = qps_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.nameSpace_ = nameSpace_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey.getDefaultInstance()) return this;
if (other.hasKey()) {
setKey(other.getKey());
}
if (other.hasQps()) {
setQps(other.getQps());
}
if (other.hasNameSpace()) {
nameSpace_ = other.nameSpace_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
if (!hasQps()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 17: {
qps_ = input.readDouble();
bitField0_ |= 0x00000002;
break;
} // case 17
case 26: {
nameSpace_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString key_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Max 250 bytes.
*
*
* required bytes key = 1;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Max 250 bytes.
*
*
* required bytes key = 1;
* @return The key.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKey() {
return key_;
}
/**
*
* Max 250 bytes.
*
*
* required bytes key = 1;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Max 250 bytes.
*
*
* required bytes key = 1;
* @return This builder for chaining.
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
private double qps_ ;
/**
*
* A query is defined as an individual key operation, i.e. a single GET
* operation on one particular key. A memcache API call can contain multiple
* key operations in a single call.
*
*
* required double qps = 2;
* @return Whether the qps field is set.
*/
@java.lang.Override
public boolean hasQps() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* A query is defined as an individual key operation, i.e. a single GET
* operation on one particular key. A memcache API call can contain multiple
* key operations in a single call.
*
*
* required double qps = 2;
* @return The qps.
*/
@java.lang.Override
public double getQps() {
return qps_;
}
/**
*
* A query is defined as an individual key operation, i.e. a single GET
* operation on one particular key. A memcache API call can contain multiple
* key operations in a single call.
*
*
* required double qps = 2;
* @param value The qps to set.
* @return This builder for chaining.
*/
public Builder setQps(double value) {
qps_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* A query is defined as an individual key operation, i.e. a single GET
* operation on one particular key. A memcache API call can contain multiple
* key operations in a single call.
*
*
* required double qps = 2;
* @return This builder for chaining.
*/
public Builder clearQps() {
bitField0_ = (bitField0_ & ~0x00000002);
qps_ = 0D;
onChanged();
return this;
}
private java.lang.Object nameSpace_ = "";
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return Whether the nameSpace field is set.
*/
public boolean hasNameSpace() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return The nameSpace.
*/
public java.lang.String getNameSpace() {
java.lang.Object ref = nameSpace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
nameSpace_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return The bytes for nameSpace.
*/
public com.google.protobuf.ByteString
getNameSpaceBytes() {
java.lang.Object ref = nameSpace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
nameSpace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @param value The nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpace(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @return This builder for chaining.
*/
public Builder clearNameSpace() {
nameSpace_ = getDefaultInstance().getNameSpace();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* The namespace this key belongs to.
*
*
* optional string name_space = 3;
* @param value The bytes for nameSpace to set.
* @return This builder for chaining.
*/
public Builder setNameSpaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
nameSpace_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheHotKey)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheHotKey)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheHotKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheHotKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MemcacheStatsResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.MemcacheStatsResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
* @return Whether the stats field is set.
*/
boolean hasStats();
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
* @return The stats.
*/
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats getStats();
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStatsOrBuilder getStatsOrBuilder();
}
/**
* Protobuf type {@code java.apphosting.MemcacheStatsResponse}
*/
public static final class MemcacheStatsResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.MemcacheStatsResponse)
MemcacheStatsResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use MemcacheStatsResponse.newBuilder() to construct.
private MemcacheStatsResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MemcacheStatsResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MemcacheStatsResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse.Builder.class);
}
private int bitField0_;
public static final int STATS_FIELD_NUMBER = 1;
private com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats stats_;
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
* @return Whether the stats field is set.
*/
@java.lang.Override
public boolean hasStats() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
* @return The stats.
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats getStats() {
return stats_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.getDefaultInstance() : stats_;
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStatsOrBuilder getStatsOrBuilder() {
return stats_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.getDefaultInstance() : stats_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasStats()) {
if (!getStats().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getStats());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getStats());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse)) {
return super.equals(obj);
}
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse other = (com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse) obj;
if (hasStats() != other.hasStats()) return false;
if (hasStats()) {
if (!getStats()
.equals(other.getStats())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStats()) {
hash = (37 * hash) + STATS_FIELD_NUMBER;
hash = (53 * hash) + getStats().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.MemcacheStatsResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.MemcacheStatsResponse)
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse.class, com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse.Builder.class);
}
// Construct using com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStatsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
stats_ = null;
if (statsBuilder_ != null) {
statsBuilder_.dispose();
statsBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.internal_static_java_apphosting_MemcacheStatsResponse_descriptor;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse getDefaultInstanceForType() {
return com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse build() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse buildPartial() {
com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse result = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.stats_ = statsBuilder_ == null
? stats_
: statsBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse) {
return mergeFrom((com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse other) {
if (other == com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse.getDefaultInstance()) return this;
if (other.hasStats()) {
mergeStats(other.getStats());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasStats()) {
if (!getStats().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getStatsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats stats_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats, com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStatsOrBuilder> statsBuilder_;
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
* @return Whether the stats field is set.
*/
public boolean hasStats() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
* @return The stats.
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats getStats() {
if (statsBuilder_ == null) {
return stats_ == null ? com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.getDefaultInstance() : stats_;
} else {
return statsBuilder_.getMessage();
}
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
public Builder setStats(com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats value) {
if (statsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stats_ = value;
} else {
statsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
public Builder setStats(
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.Builder builderForValue) {
if (statsBuilder_ == null) {
stats_ = builderForValue.build();
} else {
statsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
public Builder mergeStats(com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats value) {
if (statsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
stats_ != null &&
stats_ != com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.getDefaultInstance()) {
getStatsBuilder().mergeFrom(value);
} else {
stats_ = value;
}
} else {
statsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
public Builder clearStats() {
bitField0_ = (bitField0_ & ~0x00000001);
stats_ = null;
if (statsBuilder_ != null) {
statsBuilder_.dispose();
statsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.Builder getStatsBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getStatsFieldBuilder().getBuilder();
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
public com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStatsOrBuilder getStatsOrBuilder() {
if (statsBuilder_ != null) {
return statsBuilder_.getMessageOrBuilder();
} else {
return stats_ == null ?
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.getDefaultInstance() : stats_;
}
}
/**
*
* This is set if the namespace was found:
*
*
* optional .java.apphosting.MergedNamespaceStats stats = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats, com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStatsOrBuilder>
getStatsFieldBuilder() {
if (statsBuilder_ == null) {
statsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats, com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStats.Builder, com.google.appengine.api.memcache.MemcacheServicePb.MergedNamespaceStatsOrBuilder>(
getStats(),
getParentForChildren(),
isClean());
stats_ = null;
}
return statsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.MemcacheStatsResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.MemcacheStatsResponse)
private static final com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse();
}
public static com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MemcacheStatsResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.appengine.api.memcache.MemcacheServicePb.MemcacheStatsResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheServiceError_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheServiceError_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_AppOverride_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_AppOverride_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheGetRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheGetRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_ItemTimestamps_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_ItemTimestamps_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheGetResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheGetResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheGetResponse_Item_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheGetResponse_Item_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheSetRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheSetRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheSetRequest_Item_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheSetRequest_Item_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheSetResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheSetResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheDeleteRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheDeleteRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheDeleteRequest_Item_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheDeleteRequest_Item_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheDeleteResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheDeleteResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheIncrementRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheIncrementRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheIncrementResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheIncrementResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheBatchIncrementRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheBatchIncrementRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheBatchIncrementResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheBatchIncrementResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheFlushRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheFlushRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheFlushResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheFlushResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheStatsRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheStatsRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MergedNamespaceStats_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MergedNamespaceStats_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheHotKey_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheHotKey_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_MemcacheStatsResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_MemcacheStatsResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\026memcache_service.proto\022\017java.apphostin" +
"g\"\224\001\n\024MemcacheServiceError\"|\n\tErrorCode\022" +
"\006\n\002OK\020\000\022\025\n\021UNSPECIFIED_ERROR\020\001\022\025\n\021NAMESP" +
"ACE_NOT_SET\020\002\022\025\n\021PERMISSION_DENIED\020\003\022\021\n\r" +
"INVALID_VALUE\020\006\022\017\n\013UNAVAILABLE\020\t\"\035\n\013AppO" +
"verride\022\016\n\006app_id\030\001 \002(\t\"\212\001\n\022MemcacheGetR" +
"equest\022\013\n\003key\030\001 \003(\014\022\024\n\nname_space\030\002 \001(\t:" +
"\000\022\017\n\007for_cas\030\004 \001(\010\022.\n\010override\030\005 \001(\0132\034.j" +
"ava.apphosting.AppOverride\022\020\n\010for_peek\030\006" +
" \001(\010\"i\n\016ItemTimestamps\022\033\n\023expiration_tim" +
"e_sec\030\001 \001(\003\022\034\n\024last_access_time_sec\030\002 \001(" +
"\003\022\034\n\024delete_lock_time_sec\030\003 \001(\003\"\261\003\n\023Memc" +
"acheGetResponse\0227\n\004item\030\001 \003(\n2).java.app" +
"hosting.MemcacheGetResponse.Item\022F\n\nget_" +
"status\030\007 \003(\01622.java.apphosting.MemcacheG" +
"etResponse.GetStatusCode\032\254\001\n\004Item\022\013\n\003key" +
"\030\002 \002(\014\022\r\n\005value\030\003 \002(\014\022\r\n\005flags\030\004 \001(\007\022\016\n\006" +
"cas_id\030\005 \001(\006\022\032\n\022expires_in_seconds\030\006 \001(\005" +
"\0223\n\ntimestamps\030\010 \001(\0132\037.java.apphosting.I" +
"temTimestamps\022\030\n\020is_delete_locked\030\t \001(\010\"" +
"j\n\rGetStatusCode\022\007\n\003HIT\020\001\022\010\n\004MISS\020\002\022\r\n\tT" +
"RUNCATED\020\003\022\025\n\021DEADLINE_EXCEEDED\020\004\022\017\n\013UNR" +
"EACHABLE\020\005\022\017\n\013OTHER_ERROR\020\006\"\200\003\n\022Memcache" +
"SetRequest\0226\n\004item\030\001 \003(\n2(.java.apphosti" +
"ng.MemcacheSetRequest.Item\022\024\n\nname_space" +
"\030\007 \001(\t:\000\022.\n\010override\030\n \001(\0132\034.java.apphos" +
"ting.AppOverride\032\266\001\n\004Item\022\013\n\003key\030\002 \002(\014\022\r" +
"\n\005value\030\003 \002(\014\022\r\n\005flags\030\004 \001(\007\022F\n\nset_poli" +
"cy\030\005 \001(\0162-.java.apphosting.MemcacheSetRe" +
"quest.SetPolicy:\003SET\022\032\n\017expiration_time\030" +
"\006 \001(\007:\0010\022\016\n\006cas_id\030\010 \001(\006\022\017\n\007for_cas\030\t \001(" +
"\010\"3\n\tSetPolicy\022\007\n\003SET\020\001\022\007\n\003ADD\020\002\022\013\n\007REPL" +
"ACE\020\003\022\007\n\003CAS\020\004\"\332\001\n\023MemcacheSetResponse\022F" +
"\n\nset_status\030\001 \003(\01622.java.apphosting.Mem" +
"cacheSetResponse.SetStatusCode\"{\n\rSetSta" +
"tusCode\022\n\n\006STORED\020\001\022\016\n\nNOT_STORED\020\002\022\t\n\005E" +
"RROR\020\003\022\n\n\006EXISTS\020\004\022\025\n\021DEADLINE_EXCEEDED\020" +
"\005\022\017\n\013UNREACHABLE\020\006\022\017\n\013OTHER_ERROR\020\007\"\305\001\n\025" +
"MemcacheDeleteRequest\0229\n\004item\030\001 \003(\n2+.ja" +
"va.apphosting.MemcacheDeleteRequest.Item" +
"\022\024\n\nname_space\030\004 \001(\t:\000\022.\n\010override\030\005 \001(\013" +
"2\034.java.apphosting.AppOverride\032+\n\004Item\022\013" +
"\n\003key\030\002 \002(\014\022\026\n\013delete_time\030\003 \001(\007:\0010\"\322\001\n\026" +
"MemcacheDeleteResponse\022O\n\rdelete_status\030" +
"\001 \003(\01628.java.apphosting.MemcacheDeleteRe" +
"sponse.DeleteStatusCode\"g\n\020DeleteStatusC" +
"ode\022\013\n\007DELETED\020\001\022\r\n\tNOT_FOUND\020\002\022\025\n\021DEADL" +
"INE_EXCEEDED\020\003\022\017\n\013UNREACHABLE\020\004\022\017\n\013OTHER" +
"_ERROR\020\005\"\253\002\n\030MemcacheIncrementRequest\022\013\n" +
"\003key\030\001 \002(\014\022\024\n\nname_space\030\004 \001(\t:\000\022\020\n\005delt" +
"a\030\002 \001(\004:\0011\022Q\n\tdirection\030\003 \001(\01623.java.app" +
"hosting.MemcacheIncrementRequest.Directi" +
"on:\tINCREMENT\022\025\n\rinitial_value\030\005 \001(\004\022\025\n\r" +
"initial_flags\030\006 \001(\007\022.\n\010override\030\007 \001(\0132\034." +
"java.apphosting.AppOverride\")\n\tDirection" +
"\022\r\n\tINCREMENT\020\001\022\r\n\tDECREMENT\020\002\"\374\001\n\031Memca" +
"cheIncrementResponse\022\021\n\tnew_value\030\001 \001(\004\022" +
"X\n\020increment_status\030\002 \001(\0162>.java.apphost" +
"ing.MemcacheIncrementResponse.IncrementS" +
"tatusCode\"r\n\023IncrementStatusCode\022\006\n\002OK\020\001" +
"\022\017\n\013NOT_CHANGED\020\002\022\t\n\005ERROR\020\003\022\025\n\021DEADLINE" +
"_EXCEEDED\020\004\022\017\n\013UNREACHABLE\020\005\022\017\n\013OTHER_ER" +
"ROR\020\006\"\236\001\n\035MemcacheBatchIncrementRequest\022" +
"\024\n\nname_space\030\001 \001(\t:\000\0227\n\004item\030\002 \003(\0132).ja" +
"va.apphosting.MemcacheIncrementRequest\022." +
"\n\010override\030\003 \001(\0132\034.java.apphosting.AppOv" +
"erride\"Z\n\036MemcacheBatchIncrementResponse" +
"\0228\n\004item\030\001 \003(\0132*.java.apphosting.Memcach" +
"eIncrementResponse\"F\n\024MemcacheFlushReque" +
"st\022.\n\010override\030\001 \001(\0132\034.java.apphosting.A" +
"ppOverride\"\027\n\025MemcacheFlushResponse\"c\n\024M" +
"emcacheStatsRequest\022.\n\010override\030\001 \001(\0132\034." +
"java.apphosting.AppOverride\022\033\n\020max_hotke" +
"y_count\030\002 \001(\005:\0010\"\260\001\n\024MergedNamespaceStat" +
"s\022\014\n\004hits\030\001 \002(\004\022\016\n\006misses\030\002 \002(\004\022\021\n\tbyte_" +
"hits\030\003 \002(\004\022\r\n\005items\030\004 \002(\004\022\r\n\005bytes\030\005 \002(\004" +
"\022\027\n\017oldest_item_age\030\006 \002(\007\0220\n\007hotkeys\030\007 \003" +
"(\0132\037.java.apphosting.MemcacheHotKey\">\n\016M" +
"emcacheHotKey\022\013\n\003key\030\001 \002(\014\022\013\n\003qps\030\002 \002(\001\022" +
"\022\n\nname_space\030\003 \001(\t\"M\n\025MemcacheStatsResp" +
"onse\0224\n\005stats\030\001 \001(\0132%.java.apphosting.Me" +
"rgedNamespaceStatsB9\n!com.google.appengi" +
"ne.api.memcacheB\021MemcacheServicePb\210\001\001"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_java_apphosting_MemcacheServiceError_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_java_apphosting_MemcacheServiceError_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheServiceError_descriptor,
new java.lang.String[] { });
internal_static_java_apphosting_AppOverride_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_java_apphosting_AppOverride_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_AppOverride_descriptor,
new java.lang.String[] { "AppId", });
internal_static_java_apphosting_MemcacheGetRequest_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_java_apphosting_MemcacheGetRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheGetRequest_descriptor,
new java.lang.String[] { "Key", "NameSpace", "ForCas", "Override", "ForPeek", });
internal_static_java_apphosting_ItemTimestamps_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_java_apphosting_ItemTimestamps_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_ItemTimestamps_descriptor,
new java.lang.String[] { "ExpirationTimeSec", "LastAccessTimeSec", "DeleteLockTimeSec", });
internal_static_java_apphosting_MemcacheGetResponse_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_java_apphosting_MemcacheGetResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheGetResponse_descriptor,
new java.lang.String[] { "Item", "GetStatus", });
internal_static_java_apphosting_MemcacheGetResponse_Item_descriptor =
internal_static_java_apphosting_MemcacheGetResponse_descriptor.getNestedTypes().get(0);
internal_static_java_apphosting_MemcacheGetResponse_Item_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheGetResponse_Item_descriptor,
new java.lang.String[] { "Key", "Value", "Flags", "CasId", "ExpiresInSeconds", "Timestamps", "IsDeleteLocked", });
internal_static_java_apphosting_MemcacheSetRequest_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_java_apphosting_MemcacheSetRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheSetRequest_descriptor,
new java.lang.String[] { "Item", "NameSpace", "Override", });
internal_static_java_apphosting_MemcacheSetRequest_Item_descriptor =
internal_static_java_apphosting_MemcacheSetRequest_descriptor.getNestedTypes().get(0);
internal_static_java_apphosting_MemcacheSetRequest_Item_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheSetRequest_Item_descriptor,
new java.lang.String[] { "Key", "Value", "Flags", "SetPolicy", "ExpirationTime", "CasId", "ForCas", });
internal_static_java_apphosting_MemcacheSetResponse_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_java_apphosting_MemcacheSetResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheSetResponse_descriptor,
new java.lang.String[] { "SetStatus", });
internal_static_java_apphosting_MemcacheDeleteRequest_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_java_apphosting_MemcacheDeleteRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheDeleteRequest_descriptor,
new java.lang.String[] { "Item", "NameSpace", "Override", });
internal_static_java_apphosting_MemcacheDeleteRequest_Item_descriptor =
internal_static_java_apphosting_MemcacheDeleteRequest_descriptor.getNestedTypes().get(0);
internal_static_java_apphosting_MemcacheDeleteRequest_Item_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheDeleteRequest_Item_descriptor,
new java.lang.String[] { "Key", "DeleteTime", });
internal_static_java_apphosting_MemcacheDeleteResponse_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_java_apphosting_MemcacheDeleteResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheDeleteResponse_descriptor,
new java.lang.String[] { "DeleteStatus", });
internal_static_java_apphosting_MemcacheIncrementRequest_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_java_apphosting_MemcacheIncrementRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheIncrementRequest_descriptor,
new java.lang.String[] { "Key", "NameSpace", "Delta", "Direction", "InitialValue", "InitialFlags", "Override", });
internal_static_java_apphosting_MemcacheIncrementResponse_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_java_apphosting_MemcacheIncrementResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheIncrementResponse_descriptor,
new java.lang.String[] { "NewValue", "IncrementStatus", });
internal_static_java_apphosting_MemcacheBatchIncrementRequest_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_java_apphosting_MemcacheBatchIncrementRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheBatchIncrementRequest_descriptor,
new java.lang.String[] { "NameSpace", "Item", "Override", });
internal_static_java_apphosting_MemcacheBatchIncrementResponse_descriptor =
getDescriptor().getMessageTypes().get(12);
internal_static_java_apphosting_MemcacheBatchIncrementResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheBatchIncrementResponse_descriptor,
new java.lang.String[] { "Item", });
internal_static_java_apphosting_MemcacheFlushRequest_descriptor =
getDescriptor().getMessageTypes().get(13);
internal_static_java_apphosting_MemcacheFlushRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheFlushRequest_descriptor,
new java.lang.String[] { "Override", });
internal_static_java_apphosting_MemcacheFlushResponse_descriptor =
getDescriptor().getMessageTypes().get(14);
internal_static_java_apphosting_MemcacheFlushResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheFlushResponse_descriptor,
new java.lang.String[] { });
internal_static_java_apphosting_MemcacheStatsRequest_descriptor =
getDescriptor().getMessageTypes().get(15);
internal_static_java_apphosting_MemcacheStatsRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheStatsRequest_descriptor,
new java.lang.String[] { "Override", "MaxHotkeyCount", });
internal_static_java_apphosting_MergedNamespaceStats_descriptor =
getDescriptor().getMessageTypes().get(16);
internal_static_java_apphosting_MergedNamespaceStats_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MergedNamespaceStats_descriptor,
new java.lang.String[] { "Hits", "Misses", "ByteHits", "Items", "Bytes", "OldestItemAge", "Hotkeys", });
internal_static_java_apphosting_MemcacheHotKey_descriptor =
getDescriptor().getMessageTypes().get(17);
internal_static_java_apphosting_MemcacheHotKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheHotKey_descriptor,
new java.lang.String[] { "Key", "Qps", "NameSpace", });
internal_static_java_apphosting_MemcacheStatsResponse_descriptor =
getDescriptor().getMessageTypes().get(18);
internal_static_java_apphosting_MemcacheStatsResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_MemcacheStatsResponse_descriptor,
new java.lang.String[] { "Stats", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy