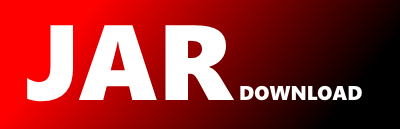
com.google.apphosting.api.UserServicePb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: user_service.proto
package com.google.apphosting.api;
public final class UserServicePb {
private UserServicePb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface UserServiceErrorOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.UserServiceError)
com.google.protobuf.MessageOrBuilder {
}
/**
* Protobuf type {@code java.apphosting.UserServiceError}
*/
public static final class UserServiceError extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.UserServiceError)
UserServiceErrorOrBuilder {
private static final long serialVersionUID = 0L;
// Use UserServiceError.newBuilder() to construct.
private UserServiceError(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserServiceError() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new UserServiceError();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_UserServiceError_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_UserServiceError_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.UserServiceError.class, com.google.apphosting.api.UserServicePb.UserServiceError.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.UserServiceError.ErrorCode}
*/
public enum ErrorCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* OK = 0;
*/
OK(0),
/**
* REDIRECT_URL_TOO_LONG = 1;
*/
REDIRECT_URL_TOO_LONG(1),
/**
* NOT_ALLOWED = 2;
*/
NOT_ALLOWED(2),
/**
* OAUTH_INVALID_TOKEN = 3;
*/
OAUTH_INVALID_TOKEN(3),
/**
* OAUTH_INVALID_REQUEST = 4;
*/
OAUTH_INVALID_REQUEST(4),
/**
* OAUTH_ERROR = 5;
*/
OAUTH_ERROR(5),
;
/**
* OK = 0;
*/
public static final int OK_VALUE = 0;
/**
* REDIRECT_URL_TOO_LONG = 1;
*/
public static final int REDIRECT_URL_TOO_LONG_VALUE = 1;
/**
* NOT_ALLOWED = 2;
*/
public static final int NOT_ALLOWED_VALUE = 2;
/**
* OAUTH_INVALID_TOKEN = 3;
*/
public static final int OAUTH_INVALID_TOKEN_VALUE = 3;
/**
* OAUTH_INVALID_REQUEST = 4;
*/
public static final int OAUTH_INVALID_REQUEST_VALUE = 4;
/**
* OAUTH_ERROR = 5;
*/
public static final int OAUTH_ERROR_VALUE = 5;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ErrorCode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ErrorCode forNumber(int value) {
switch (value) {
case 0: return OK;
case 1: return REDIRECT_URL_TOO_LONG;
case 2: return NOT_ALLOWED;
case 3: return OAUTH_INVALID_TOKEN;
case 4: return OAUTH_INVALID_REQUEST;
case 5: return OAUTH_ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ErrorCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ErrorCode findValueByNumber(int number) {
return ErrorCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.UserServiceError.getDescriptor().getEnumTypes().get(0);
}
private static final ErrorCode[] VALUES = values();
public static ErrorCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ErrorCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.UserServiceError.ErrorCode)
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.UserServicePb.UserServiceError)) {
return super.equals(obj);
}
com.google.apphosting.api.UserServicePb.UserServiceError other = (com.google.apphosting.api.UserServicePb.UserServiceError) obj;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.UserServiceError parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.UserServicePb.UserServiceError prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.UserServiceError}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.UserServiceError)
com.google.apphosting.api.UserServicePb.UserServiceErrorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_UserServiceError_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_UserServiceError_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.UserServiceError.class, com.google.apphosting.api.UserServicePb.UserServiceError.Builder.class);
}
// Construct using com.google.apphosting.api.UserServicePb.UserServiceError.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_UserServiceError_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.UserServiceError getDefaultInstanceForType() {
return com.google.apphosting.api.UserServicePb.UserServiceError.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.UserServiceError build() {
com.google.apphosting.api.UserServicePb.UserServiceError result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.UserServiceError buildPartial() {
com.google.apphosting.api.UserServicePb.UserServiceError result = new com.google.apphosting.api.UserServicePb.UserServiceError(this);
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.UserServicePb.UserServiceError) {
return mergeFrom((com.google.apphosting.api.UserServicePb.UserServiceError)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.UserServicePb.UserServiceError other) {
if (other == com.google.apphosting.api.UserServicePb.UserServiceError.getDefaultInstance()) return this;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.UserServiceError)
}
// @@protoc_insertion_point(class_scope:java.apphosting.UserServiceError)
private static final com.google.apphosting.api.UserServicePb.UserServiceError DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.UserServicePb.UserServiceError();
}
public static com.google.apphosting.api.UserServicePb.UserServiceError getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UserServiceError parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.UserServiceError getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateLoginURLRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.CreateLoginURLRequest)
com.google.protobuf.MessageOrBuilder {
/**
* required string destination_url = 1;
* @return Whether the destinationUrl field is set.
*/
boolean hasDestinationUrl();
/**
* required string destination_url = 1;
* @return The destinationUrl.
*/
java.lang.String getDestinationUrl();
/**
* required string destination_url = 1;
* @return The bytes for destinationUrl.
*/
com.google.protobuf.ByteString
getDestinationUrlBytes();
/**
* optional string auth_domain = 2;
* @return Whether the authDomain field is set.
*/
boolean hasAuthDomain();
/**
* optional string auth_domain = 2;
* @return The authDomain.
*/
java.lang.String getAuthDomain();
/**
* optional string auth_domain = 2;
* @return The bytes for authDomain.
*/
com.google.protobuf.ByteString
getAuthDomainBytes();
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return Whether the federatedIdentity field is set.
*/
boolean hasFederatedIdentity();
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return The federatedIdentity.
*/
java.lang.String getFederatedIdentity();
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return The bytes for federatedIdentity.
*/
com.google.protobuf.ByteString
getFederatedIdentityBytes();
}
/**
* Protobuf type {@code java.apphosting.CreateLoginURLRequest}
*/
public static final class CreateLoginURLRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.CreateLoginURLRequest)
CreateLoginURLRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateLoginURLRequest.newBuilder() to construct.
private CreateLoginURLRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateLoginURLRequest() {
destinationUrl_ = "";
authDomain_ = "";
federatedIdentity_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateLoginURLRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.CreateLoginURLRequest.class, com.google.apphosting.api.UserServicePb.CreateLoginURLRequest.Builder.class);
}
private int bitField0_;
public static final int DESTINATION_URL_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object destinationUrl_ = "";
/**
* required string destination_url = 1;
* @return Whether the destinationUrl field is set.
*/
@java.lang.Override
public boolean hasDestinationUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string destination_url = 1;
* @return The destinationUrl.
*/
@java.lang.Override
public java.lang.String getDestinationUrl() {
java.lang.Object ref = destinationUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
destinationUrl_ = s;
}
return s;
}
}
/**
* required string destination_url = 1;
* @return The bytes for destinationUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDestinationUrlBytes() {
java.lang.Object ref = destinationUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
destinationUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AUTH_DOMAIN_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object authDomain_ = "";
/**
* optional string auth_domain = 2;
* @return Whether the authDomain field is set.
*/
@java.lang.Override
public boolean hasAuthDomain() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string auth_domain = 2;
* @return The authDomain.
*/
@java.lang.Override
public java.lang.String getAuthDomain() {
java.lang.Object ref = authDomain_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
authDomain_ = s;
}
return s;
}
}
/**
* optional string auth_domain = 2;
* @return The bytes for authDomain.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAuthDomainBytes() {
java.lang.Object ref = authDomain_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
authDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FEDERATED_IDENTITY_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object federatedIdentity_ = "";
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return Whether the federatedIdentity field is set.
*/
@java.lang.Override
public boolean hasFederatedIdentity() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return The federatedIdentity.
*/
@java.lang.Override
public java.lang.String getFederatedIdentity() {
java.lang.Object ref = federatedIdentity_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
federatedIdentity_ = s;
}
return s;
}
}
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return The bytes for federatedIdentity.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFederatedIdentityBytes() {
java.lang.Object ref = federatedIdentity_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
federatedIdentity_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasDestinationUrl()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, destinationUrl_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, authDomain_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, federatedIdentity_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, destinationUrl_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, authDomain_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, federatedIdentity_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.UserServicePb.CreateLoginURLRequest)) {
return super.equals(obj);
}
com.google.apphosting.api.UserServicePb.CreateLoginURLRequest other = (com.google.apphosting.api.UserServicePb.CreateLoginURLRequest) obj;
if (hasDestinationUrl() != other.hasDestinationUrl()) return false;
if (hasDestinationUrl()) {
if (!getDestinationUrl()
.equals(other.getDestinationUrl())) return false;
}
if (hasAuthDomain() != other.hasAuthDomain()) return false;
if (hasAuthDomain()) {
if (!getAuthDomain()
.equals(other.getAuthDomain())) return false;
}
if (hasFederatedIdentity() != other.hasFederatedIdentity()) return false;
if (hasFederatedIdentity()) {
if (!getFederatedIdentity()
.equals(other.getFederatedIdentity())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDestinationUrl()) {
hash = (37 * hash) + DESTINATION_URL_FIELD_NUMBER;
hash = (53 * hash) + getDestinationUrl().hashCode();
}
if (hasAuthDomain()) {
hash = (37 * hash) + AUTH_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getAuthDomain().hashCode();
}
if (hasFederatedIdentity()) {
hash = (37 * hash) + FEDERATED_IDENTITY_FIELD_NUMBER;
hash = (53 * hash) + getFederatedIdentity().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.UserServicePb.CreateLoginURLRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.CreateLoginURLRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.CreateLoginURLRequest)
com.google.apphosting.api.UserServicePb.CreateLoginURLRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.CreateLoginURLRequest.class, com.google.apphosting.api.UserServicePb.CreateLoginURLRequest.Builder.class);
}
// Construct using com.google.apphosting.api.UserServicePb.CreateLoginURLRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
destinationUrl_ = "";
authDomain_ = "";
federatedIdentity_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLRequest_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLoginURLRequest getDefaultInstanceForType() {
return com.google.apphosting.api.UserServicePb.CreateLoginURLRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLoginURLRequest build() {
com.google.apphosting.api.UserServicePb.CreateLoginURLRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLoginURLRequest buildPartial() {
com.google.apphosting.api.UserServicePb.CreateLoginURLRequest result = new com.google.apphosting.api.UserServicePb.CreateLoginURLRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.UserServicePb.CreateLoginURLRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.destinationUrl_ = destinationUrl_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.authDomain_ = authDomain_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.federatedIdentity_ = federatedIdentity_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.UserServicePb.CreateLoginURLRequest) {
return mergeFrom((com.google.apphosting.api.UserServicePb.CreateLoginURLRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.UserServicePb.CreateLoginURLRequest other) {
if (other == com.google.apphosting.api.UserServicePb.CreateLoginURLRequest.getDefaultInstance()) return this;
if (other.hasDestinationUrl()) {
destinationUrl_ = other.destinationUrl_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasAuthDomain()) {
authDomain_ = other.authDomain_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasFederatedIdentity()) {
federatedIdentity_ = other.federatedIdentity_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasDestinationUrl()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
destinationUrl_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
authDomain_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
federatedIdentity_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object destinationUrl_ = "";
/**
* required string destination_url = 1;
* @return Whether the destinationUrl field is set.
*/
public boolean hasDestinationUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string destination_url = 1;
* @return The destinationUrl.
*/
public java.lang.String getDestinationUrl() {
java.lang.Object ref = destinationUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
destinationUrl_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string destination_url = 1;
* @return The bytes for destinationUrl.
*/
public com.google.protobuf.ByteString
getDestinationUrlBytes() {
java.lang.Object ref = destinationUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
destinationUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string destination_url = 1;
* @param value The destinationUrl to set.
* @return This builder for chaining.
*/
public Builder setDestinationUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
destinationUrl_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required string destination_url = 1;
* @return This builder for chaining.
*/
public Builder clearDestinationUrl() {
destinationUrl_ = getDefaultInstance().getDestinationUrl();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* required string destination_url = 1;
* @param value The bytes for destinationUrl to set.
* @return This builder for chaining.
*/
public Builder setDestinationUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
destinationUrl_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object authDomain_ = "";
/**
* optional string auth_domain = 2;
* @return Whether the authDomain field is set.
*/
public boolean hasAuthDomain() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string auth_domain = 2;
* @return The authDomain.
*/
public java.lang.String getAuthDomain() {
java.lang.Object ref = authDomain_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
authDomain_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string auth_domain = 2;
* @return The bytes for authDomain.
*/
public com.google.protobuf.ByteString
getAuthDomainBytes() {
java.lang.Object ref = authDomain_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
authDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string auth_domain = 2;
* @param value The authDomain to set.
* @return This builder for chaining.
*/
public Builder setAuthDomain(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
authDomain_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional string auth_domain = 2;
* @return This builder for chaining.
*/
public Builder clearAuthDomain() {
authDomain_ = getDefaultInstance().getAuthDomain();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* optional string auth_domain = 2;
* @param value The bytes for authDomain to set.
* @return This builder for chaining.
*/
public Builder setAuthDomainBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
authDomain_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object federatedIdentity_ = "";
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return Whether the federatedIdentity field is set.
*/
public boolean hasFederatedIdentity() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return The federatedIdentity.
*/
public java.lang.String getFederatedIdentity() {
java.lang.Object ref = federatedIdentity_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
federatedIdentity_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return The bytes for federatedIdentity.
*/
public com.google.protobuf.ByteString
getFederatedIdentityBytes() {
java.lang.Object ref = federatedIdentity_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
federatedIdentity_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @param value The federatedIdentity to set.
* @return This builder for chaining.
*/
public Builder setFederatedIdentity(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
federatedIdentity_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @return This builder for chaining.
*/
public Builder clearFederatedIdentity() {
federatedIdentity_ = getDefaultInstance().getFederatedIdentity();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* federated_identity is used to do OpenID login. string type default is "".
*
*
* optional string federated_identity = 3;
* @param value The bytes for federatedIdentity to set.
* @return This builder for chaining.
*/
public Builder setFederatedIdentityBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
federatedIdentity_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.CreateLoginURLRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.CreateLoginURLRequest)
private static final com.google.apphosting.api.UserServicePb.CreateLoginURLRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.UserServicePb.CreateLoginURLRequest();
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateLoginURLRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLoginURLRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateLoginURLResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.CreateLoginURLResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return Whether the loginUrl field is set.
*/
boolean hasLoginUrl();
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return The loginUrl.
*/
java.lang.String getLoginUrl();
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return The bytes for loginUrl.
*/
com.google.protobuf.ByteString
getLoginUrlBytes();
}
/**
* Protobuf type {@code java.apphosting.CreateLoginURLResponse}
*/
public static final class CreateLoginURLResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.CreateLoginURLResponse)
CreateLoginURLResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateLoginURLResponse.newBuilder() to construct.
private CreateLoginURLResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateLoginURLResponse() {
loginUrl_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateLoginURLResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.CreateLoginURLResponse.class, com.google.apphosting.api.UserServicePb.CreateLoginURLResponse.Builder.class);
}
private int bitField0_;
public static final int LOGIN_URL_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object loginUrl_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return Whether the loginUrl field is set.
*/
@java.lang.Override
public boolean hasLoginUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return The loginUrl.
*/
@java.lang.Override
public java.lang.String getLoginUrl() {
java.lang.Object ref = loginUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
loginUrl_ = s;
}
return s;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return The bytes for loginUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLoginUrlBytes() {
java.lang.Object ref = loginUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loginUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, loginUrl_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, loginUrl_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.UserServicePb.CreateLoginURLResponse)) {
return super.equals(obj);
}
com.google.apphosting.api.UserServicePb.CreateLoginURLResponse other = (com.google.apphosting.api.UserServicePb.CreateLoginURLResponse) obj;
if (hasLoginUrl() != other.hasLoginUrl()) return false;
if (hasLoginUrl()) {
if (!getLoginUrl()
.equals(other.getLoginUrl())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLoginUrl()) {
hash = (37 * hash) + LOGIN_URL_FIELD_NUMBER;
hash = (53 * hash) + getLoginUrl().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.UserServicePb.CreateLoginURLResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.CreateLoginURLResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.CreateLoginURLResponse)
com.google.apphosting.api.UserServicePb.CreateLoginURLResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.CreateLoginURLResponse.class, com.google.apphosting.api.UserServicePb.CreateLoginURLResponse.Builder.class);
}
// Construct using com.google.apphosting.api.UserServicePb.CreateLoginURLResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
loginUrl_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLoginURLResponse_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLoginURLResponse getDefaultInstanceForType() {
return com.google.apphosting.api.UserServicePb.CreateLoginURLResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLoginURLResponse build() {
com.google.apphosting.api.UserServicePb.CreateLoginURLResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLoginURLResponse buildPartial() {
com.google.apphosting.api.UserServicePb.CreateLoginURLResponse result = new com.google.apphosting.api.UserServicePb.CreateLoginURLResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.UserServicePb.CreateLoginURLResponse result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.loginUrl_ = loginUrl_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.UserServicePb.CreateLoginURLResponse) {
return mergeFrom((com.google.apphosting.api.UserServicePb.CreateLoginURLResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.UserServicePb.CreateLoginURLResponse other) {
if (other == com.google.apphosting.api.UserServicePb.CreateLoginURLResponse.getDefaultInstance()) return this;
if (other.hasLoginUrl()) {
loginUrl_ = other.loginUrl_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
loginUrl_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object loginUrl_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return Whether the loginUrl field is set.
*/
public boolean hasLoginUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return The loginUrl.
*/
public java.lang.String getLoginUrl() {
java.lang.Object ref = loginUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
loginUrl_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return The bytes for loginUrl.
*/
public com.google.protobuf.ByteString
getLoginUrlBytes() {
java.lang.Object ref = loginUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
loginUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @param value The loginUrl to set.
* @return This builder for chaining.
*/
public Builder setLoginUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
loginUrl_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @return This builder for chaining.
*/
public Builder clearLoginUrl() {
loginUrl_ = getDefaultInstance().getLoginUrl();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string login_url = 1;
* @param value The bytes for loginUrl to set.
* @return This builder for chaining.
*/
public Builder setLoginUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
loginUrl_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.CreateLoginURLResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.CreateLoginURLResponse)
private static final com.google.apphosting.api.UserServicePb.CreateLoginURLResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.UserServicePb.CreateLoginURLResponse();
}
public static com.google.apphosting.api.UserServicePb.CreateLoginURLResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateLoginURLResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLoginURLResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateLogoutURLRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.CreateLogoutURLRequest)
com.google.protobuf.MessageOrBuilder {
/**
* required string destination_url = 1;
* @return Whether the destinationUrl field is set.
*/
boolean hasDestinationUrl();
/**
* required string destination_url = 1;
* @return The destinationUrl.
*/
java.lang.String getDestinationUrl();
/**
* required string destination_url = 1;
* @return The bytes for destinationUrl.
*/
com.google.protobuf.ByteString
getDestinationUrlBytes();
/**
* optional string auth_domain = 2;
* @return Whether the authDomain field is set.
*/
boolean hasAuthDomain();
/**
* optional string auth_domain = 2;
* @return The authDomain.
*/
java.lang.String getAuthDomain();
/**
* optional string auth_domain = 2;
* @return The bytes for authDomain.
*/
com.google.protobuf.ByteString
getAuthDomainBytes();
}
/**
* Protobuf type {@code java.apphosting.CreateLogoutURLRequest}
*/
public static final class CreateLogoutURLRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.CreateLogoutURLRequest)
CreateLogoutURLRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateLogoutURLRequest.newBuilder() to construct.
private CreateLogoutURLRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateLogoutURLRequest() {
destinationUrl_ = "";
authDomain_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateLogoutURLRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest.class, com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest.Builder.class);
}
private int bitField0_;
public static final int DESTINATION_URL_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object destinationUrl_ = "";
/**
* required string destination_url = 1;
* @return Whether the destinationUrl field is set.
*/
@java.lang.Override
public boolean hasDestinationUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string destination_url = 1;
* @return The destinationUrl.
*/
@java.lang.Override
public java.lang.String getDestinationUrl() {
java.lang.Object ref = destinationUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
destinationUrl_ = s;
}
return s;
}
}
/**
* required string destination_url = 1;
* @return The bytes for destinationUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDestinationUrlBytes() {
java.lang.Object ref = destinationUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
destinationUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AUTH_DOMAIN_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object authDomain_ = "";
/**
* optional string auth_domain = 2;
* @return Whether the authDomain field is set.
*/
@java.lang.Override
public boolean hasAuthDomain() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string auth_domain = 2;
* @return The authDomain.
*/
@java.lang.Override
public java.lang.String getAuthDomain() {
java.lang.Object ref = authDomain_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
authDomain_ = s;
}
return s;
}
}
/**
* optional string auth_domain = 2;
* @return The bytes for authDomain.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAuthDomainBytes() {
java.lang.Object ref = authDomain_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
authDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasDestinationUrl()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, destinationUrl_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, authDomain_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, destinationUrl_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, authDomain_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest)) {
return super.equals(obj);
}
com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest other = (com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest) obj;
if (hasDestinationUrl() != other.hasDestinationUrl()) return false;
if (hasDestinationUrl()) {
if (!getDestinationUrl()
.equals(other.getDestinationUrl())) return false;
}
if (hasAuthDomain() != other.hasAuthDomain()) return false;
if (hasAuthDomain()) {
if (!getAuthDomain()
.equals(other.getAuthDomain())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasDestinationUrl()) {
hash = (37 * hash) + DESTINATION_URL_FIELD_NUMBER;
hash = (53 * hash) + getDestinationUrl().hashCode();
}
if (hasAuthDomain()) {
hash = (37 * hash) + AUTH_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getAuthDomain().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.CreateLogoutURLRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.CreateLogoutURLRequest)
com.google.apphosting.api.UserServicePb.CreateLogoutURLRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest.class, com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest.Builder.class);
}
// Construct using com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
destinationUrl_ = "";
authDomain_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLRequest_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest getDefaultInstanceForType() {
return com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest build() {
com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest buildPartial() {
com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest result = new com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.destinationUrl_ = destinationUrl_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.authDomain_ = authDomain_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest) {
return mergeFrom((com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest other) {
if (other == com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest.getDefaultInstance()) return this;
if (other.hasDestinationUrl()) {
destinationUrl_ = other.destinationUrl_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasAuthDomain()) {
authDomain_ = other.authDomain_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasDestinationUrl()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
destinationUrl_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
authDomain_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object destinationUrl_ = "";
/**
* required string destination_url = 1;
* @return Whether the destinationUrl field is set.
*/
public boolean hasDestinationUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string destination_url = 1;
* @return The destinationUrl.
*/
public java.lang.String getDestinationUrl() {
java.lang.Object ref = destinationUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
destinationUrl_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string destination_url = 1;
* @return The bytes for destinationUrl.
*/
public com.google.protobuf.ByteString
getDestinationUrlBytes() {
java.lang.Object ref = destinationUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
destinationUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string destination_url = 1;
* @param value The destinationUrl to set.
* @return This builder for chaining.
*/
public Builder setDestinationUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
destinationUrl_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required string destination_url = 1;
* @return This builder for chaining.
*/
public Builder clearDestinationUrl() {
destinationUrl_ = getDefaultInstance().getDestinationUrl();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* required string destination_url = 1;
* @param value The bytes for destinationUrl to set.
* @return This builder for chaining.
*/
public Builder setDestinationUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
destinationUrl_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object authDomain_ = "";
/**
* optional string auth_domain = 2;
* @return Whether the authDomain field is set.
*/
public boolean hasAuthDomain() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional string auth_domain = 2;
* @return The authDomain.
*/
public java.lang.String getAuthDomain() {
java.lang.Object ref = authDomain_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
authDomain_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string auth_domain = 2;
* @return The bytes for authDomain.
*/
public com.google.protobuf.ByteString
getAuthDomainBytes() {
java.lang.Object ref = authDomain_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
authDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string auth_domain = 2;
* @param value The authDomain to set.
* @return This builder for chaining.
*/
public Builder setAuthDomain(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
authDomain_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional string auth_domain = 2;
* @return This builder for chaining.
*/
public Builder clearAuthDomain() {
authDomain_ = getDefaultInstance().getAuthDomain();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* optional string auth_domain = 2;
* @param value The bytes for authDomain to set.
* @return This builder for chaining.
*/
public Builder setAuthDomainBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
authDomain_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.CreateLogoutURLRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.CreateLogoutURLRequest)
private static final com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest();
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateLogoutURLRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLogoutURLRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CreateLogoutURLResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.CreateLogoutURLResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return Whether the logoutUrl field is set.
*/
boolean hasLogoutUrl();
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return The logoutUrl.
*/
java.lang.String getLogoutUrl();
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return The bytes for logoutUrl.
*/
com.google.protobuf.ByteString
getLogoutUrlBytes();
}
/**
* Protobuf type {@code java.apphosting.CreateLogoutURLResponse}
*/
public static final class CreateLogoutURLResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.CreateLogoutURLResponse)
CreateLogoutURLResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CreateLogoutURLResponse.newBuilder() to construct.
private CreateLogoutURLResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CreateLogoutURLResponse() {
logoutUrl_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CreateLogoutURLResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse.class, com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse.Builder.class);
}
private int bitField0_;
public static final int LOGOUT_URL_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object logoutUrl_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return Whether the logoutUrl field is set.
*/
@java.lang.Override
public boolean hasLogoutUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return The logoutUrl.
*/
@java.lang.Override
public java.lang.String getLogoutUrl() {
java.lang.Object ref = logoutUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
logoutUrl_ = s;
}
return s;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return The bytes for logoutUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLogoutUrlBytes() {
java.lang.Object ref = logoutUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
logoutUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, logoutUrl_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, logoutUrl_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse)) {
return super.equals(obj);
}
com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse other = (com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse) obj;
if (hasLogoutUrl() != other.hasLogoutUrl()) return false;
if (hasLogoutUrl()) {
if (!getLogoutUrl()
.equals(other.getLogoutUrl())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLogoutUrl()) {
hash = (37 * hash) + LOGOUT_URL_FIELD_NUMBER;
hash = (53 * hash) + getLogoutUrl().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.CreateLogoutURLResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.CreateLogoutURLResponse)
com.google.apphosting.api.UserServicePb.CreateLogoutURLResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse.class, com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse.Builder.class);
}
// Construct using com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
logoutUrl_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_CreateLogoutURLResponse_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse getDefaultInstanceForType() {
return com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse build() {
com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse buildPartial() {
com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse result = new com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.logoutUrl_ = logoutUrl_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse) {
return mergeFrom((com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse other) {
if (other == com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse.getDefaultInstance()) return this;
if (other.hasLogoutUrl()) {
logoutUrl_ = other.logoutUrl_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
logoutUrl_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object logoutUrl_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return Whether the logoutUrl field is set.
*/
public boolean hasLogoutUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return The logoutUrl.
*/
public java.lang.String getLogoutUrl() {
java.lang.Object ref = logoutUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
logoutUrl_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return The bytes for logoutUrl.
*/
public com.google.protobuf.ByteString
getLogoutUrlBytes() {
java.lang.Object ref = logoutUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
logoutUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @param value The logoutUrl to set.
* @return This builder for chaining.
*/
public Builder setLogoutUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
logoutUrl_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @return This builder for chaining.
*/
public Builder clearLogoutUrl() {
logoutUrl_ = getDefaultInstance().getLogoutUrl();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string logout_url = 1;
* @param value The bytes for logoutUrl to set.
* @return This builder for chaining.
*/
public Builder setLogoutUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
logoutUrl_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.CreateLogoutURLResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.CreateLogoutURLResponse)
private static final com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse();
}
public static com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CreateLogoutURLResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.CreateLogoutURLResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetOAuthUserRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.GetOAuthUserRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return Whether the scope field is set.
*/
boolean hasScope();
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return The scope.
*/
java.lang.String getScope();
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return The bytes for scope.
*/
com.google.protobuf.ByteString
getScopeBytes();
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @return A list containing the scopes.
*/
java.util.List
getScopesList();
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @return The count of scopes.
*/
int getScopesCount();
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param index The index of the element to return.
* @return The scopes at the given index.
*/
java.lang.String getScopes(int index);
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param index The index of the value to return.
* @return The bytes of the scopes at the given index.
*/
com.google.protobuf.ByteString
getScopesBytes(int index);
/**
*
* Deprecated. Does nothing.
*
*
* optional bool request_writer_permission = 3 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserRequest.request_writer_permission is deprecated.
* See user_service.proto;l=74
* @return Whether the requestWriterPermission field is set.
*/
@java.lang.Deprecated boolean hasRequestWriterPermission();
/**
*
* Deprecated. Does nothing.
*
*
* optional bool request_writer_permission = 3 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserRequest.request_writer_permission is deprecated.
* See user_service.proto;l=74
* @return The requestWriterPermission.
*/
@java.lang.Deprecated boolean getRequestWriterPermission();
}
/**
* Protobuf type {@code java.apphosting.GetOAuthUserRequest}
*/
public static final class GetOAuthUserRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.GetOAuthUserRequest)
GetOAuthUserRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetOAuthUserRequest.newBuilder() to construct.
private GetOAuthUserRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetOAuthUserRequest() {
scope_ = "";
scopes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetOAuthUserRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.GetOAuthUserRequest.class, com.google.apphosting.api.UserServicePb.GetOAuthUserRequest.Builder.class);
}
private int bitField0_;
public static final int SCOPE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object scope_ = "";
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return Whether the scope field is set.
*/
@java.lang.Override
public boolean hasScope() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return The scope.
*/
@java.lang.Override
public java.lang.String getScope() {
java.lang.Object ref = scope_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
scope_ = s;
}
return s;
}
}
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return The bytes for scope.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getScopeBytes() {
java.lang.Object ref = scope_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
scope_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCOPES_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringList scopes_;
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @return A list containing the scopes.
*/
public com.google.protobuf.ProtocolStringList
getScopesList() {
return scopes_;
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @return The count of scopes.
*/
public int getScopesCount() {
return scopes_.size();
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param index The index of the element to return.
* @return The scopes at the given index.
*/
public java.lang.String getScopes(int index) {
return scopes_.get(index);
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param index The index of the value to return.
* @return The bytes of the scopes at the given index.
*/
public com.google.protobuf.ByteString
getScopesBytes(int index) {
return scopes_.getByteString(index);
}
public static final int REQUEST_WRITER_PERMISSION_FIELD_NUMBER = 3;
private boolean requestWriterPermission_ = false;
/**
*
* Deprecated. Does nothing.
*
*
* optional bool request_writer_permission = 3 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserRequest.request_writer_permission is deprecated.
* See user_service.proto;l=74
* @return Whether the requestWriterPermission field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasRequestWriterPermission() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Deprecated. Does nothing.
*
*
* optional bool request_writer_permission = 3 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserRequest.request_writer_permission is deprecated.
* See user_service.proto;l=74
* @return The requestWriterPermission.
*/
@java.lang.Override
@java.lang.Deprecated public boolean getRequestWriterPermission() {
return requestWriterPermission_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, scope_);
}
for (int i = 0; i < scopes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, scopes_.getRaw(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(3, requestWriterPermission_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, scope_);
}
{
int dataSize = 0;
for (int i = 0; i < scopes_.size(); i++) {
dataSize += computeStringSizeNoTag(scopes_.getRaw(i));
}
size += dataSize;
size += 1 * getScopesList().size();
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, requestWriterPermission_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.UserServicePb.GetOAuthUserRequest)) {
return super.equals(obj);
}
com.google.apphosting.api.UserServicePb.GetOAuthUserRequest other = (com.google.apphosting.api.UserServicePb.GetOAuthUserRequest) obj;
if (hasScope() != other.hasScope()) return false;
if (hasScope()) {
if (!getScope()
.equals(other.getScope())) return false;
}
if (!getScopesList()
.equals(other.getScopesList())) return false;
if (hasRequestWriterPermission() != other.hasRequestWriterPermission()) return false;
if (hasRequestWriterPermission()) {
if (getRequestWriterPermission()
!= other.getRequestWriterPermission()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasScope()) {
hash = (37 * hash) + SCOPE_FIELD_NUMBER;
hash = (53 * hash) + getScope().hashCode();
}
if (getScopesCount() > 0) {
hash = (37 * hash) + SCOPES_FIELD_NUMBER;
hash = (53 * hash) + getScopesList().hashCode();
}
if (hasRequestWriterPermission()) {
hash = (37 * hash) + REQUEST_WRITER_PERMISSION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRequestWriterPermission());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.UserServicePb.GetOAuthUserRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.GetOAuthUserRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.GetOAuthUserRequest)
com.google.apphosting.api.UserServicePb.GetOAuthUserRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.GetOAuthUserRequest.class, com.google.apphosting.api.UserServicePb.GetOAuthUserRequest.Builder.class);
}
// Construct using com.google.apphosting.api.UserServicePb.GetOAuthUserRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
scope_ = "";
scopes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
requestWriterPermission_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserRequest_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.GetOAuthUserRequest getDefaultInstanceForType() {
return com.google.apphosting.api.UserServicePb.GetOAuthUserRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.GetOAuthUserRequest build() {
com.google.apphosting.api.UserServicePb.GetOAuthUserRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.GetOAuthUserRequest buildPartial() {
com.google.apphosting.api.UserServicePb.GetOAuthUserRequest result = new com.google.apphosting.api.UserServicePb.GetOAuthUserRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.api.UserServicePb.GetOAuthUserRequest result) {
if (((bitField0_ & 0x00000002) != 0)) {
scopes_ = scopes_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.scopes_ = scopes_;
}
private void buildPartial0(com.google.apphosting.api.UserServicePb.GetOAuthUserRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.scope_ = scope_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.requestWriterPermission_ = requestWriterPermission_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.UserServicePb.GetOAuthUserRequest) {
return mergeFrom((com.google.apphosting.api.UserServicePb.GetOAuthUserRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.UserServicePb.GetOAuthUserRequest other) {
if (other == com.google.apphosting.api.UserServicePb.GetOAuthUserRequest.getDefaultInstance()) return this;
if (other.hasScope()) {
scope_ = other.scope_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.scopes_.isEmpty()) {
if (scopes_.isEmpty()) {
scopes_ = other.scopes_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureScopesIsMutable();
scopes_.addAll(other.scopes_);
}
onChanged();
}
if (other.hasRequestWriterPermission()) {
setRequestWriterPermission(other.getRequestWriterPermission());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
scope_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
com.google.protobuf.ByteString bs = input.readBytes();
ensureScopesIsMutable();
scopes_.add(bs);
break;
} // case 18
case 24: {
requestWriterPermission_ = input.readBool();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object scope_ = "";
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return Whether the scope field is set.
*/
public boolean hasScope() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return The scope.
*/
public java.lang.String getScope() {
java.lang.Object ref = scope_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
scope_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return The bytes for scope.
*/
public com.google.protobuf.ByteString
getScopeBytes() {
java.lang.Object ref = scope_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
scope_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @param value The scope to set.
* @return This builder for chaining.
*/
public Builder setScope(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
scope_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @return This builder for chaining.
*/
public Builder clearScope() {
scope_ = getDefaultInstance().getScope();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The scope of the OAuth token to check for, e.g.
* "https://www.googleapis.com/auth/appengine.admin".
* Used if present and "scopes" is unset.
* If both "scope" and "scopes" are not specified, defaults to checking for
* app-specific token as described in
* http://g3doc/apphosting/g3doc/wiki-carryover/oauth_integration.md
*
*
* optional string scope = 1;
* @param value The bytes for scope to set.
* @return This builder for chaining.
*/
public Builder setScopeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
scope_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList scopes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureScopesIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
scopes_ = new com.google.protobuf.LazyStringArrayList(scopes_);
bitField0_ |= 0x00000002;
}
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @return A list containing the scopes.
*/
public com.google.protobuf.ProtocolStringList
getScopesList() {
return scopes_.getUnmodifiableView();
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @return The count of scopes.
*/
public int getScopesCount() {
return scopes_.size();
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param index The index of the element to return.
* @return The scopes at the given index.
*/
public java.lang.String getScopes(int index) {
return scopes_.get(index);
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param index The index of the value to return.
* @return The bytes of the scopes at the given index.
*/
public com.google.protobuf.ByteString
getScopesBytes(int index) {
return scopes_.getByteString(index);
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param index The index to set the value at.
* @param value The scopes to set.
* @return This builder for chaining.
*/
public Builder setScopes(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureScopesIsMutable();
scopes_.set(index, value);
onChanged();
return this;
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param value The scopes to add.
* @return This builder for chaining.
*/
public Builder addScopes(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureScopesIsMutable();
scopes_.add(value);
onChanged();
return this;
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param values The scopes to add.
* @return This builder for chaining.
*/
public Builder addAllScopes(
java.lang.Iterable values) {
ensureScopesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, scopes_);
onChanged();
return this;
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @return This builder for chaining.
*/
public Builder clearScopes() {
scopes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* List of scopes, one of them must be present in authentication credentials
* for the request to succeed.
* If present, "scope" is ignored.
*
*
* repeated string scopes = 2;
* @param value The bytes of the scopes to add.
* @return This builder for chaining.
*/
public Builder addScopesBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
ensureScopesIsMutable();
scopes_.add(value);
onChanged();
return this;
}
private boolean requestWriterPermission_ ;
/**
*
* Deprecated. Does nothing.
*
*
* optional bool request_writer_permission = 3 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserRequest.request_writer_permission is deprecated.
* See user_service.proto;l=74
* @return Whether the requestWriterPermission field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasRequestWriterPermission() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Deprecated. Does nothing.
*
*
* optional bool request_writer_permission = 3 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserRequest.request_writer_permission is deprecated.
* See user_service.proto;l=74
* @return The requestWriterPermission.
*/
@java.lang.Override
@java.lang.Deprecated public boolean getRequestWriterPermission() {
return requestWriterPermission_;
}
/**
*
* Deprecated. Does nothing.
*
*
* optional bool request_writer_permission = 3 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserRequest.request_writer_permission is deprecated.
* See user_service.proto;l=74
* @param value The requestWriterPermission to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setRequestWriterPermission(boolean value) {
requestWriterPermission_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Deprecated. Does nothing.
*
*
* optional bool request_writer_permission = 3 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserRequest.request_writer_permission is deprecated.
* See user_service.proto;l=74
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearRequestWriterPermission() {
bitField0_ = (bitField0_ & ~0x00000004);
requestWriterPermission_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.GetOAuthUserRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.GetOAuthUserRequest)
private static final com.google.apphosting.api.UserServicePb.GetOAuthUserRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.UserServicePb.GetOAuthUserRequest();
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetOAuthUserRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.GetOAuthUserRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetOAuthUserResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.GetOAuthUserResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return Whether the email field is set.
*/
boolean hasEmail();
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return The email.
*/
java.lang.String getEmail();
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return The bytes for email.
*/
com.google.protobuf.ByteString
getEmailBytes();
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return Whether the userId field is set.
*/
boolean hasUserId();
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return The userId.
*/
java.lang.String getUserId();
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return The bytes for userId.
*/
com.google.protobuf.ByteString
getUserIdBytes();
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return Whether the authDomain field is set.
*/
boolean hasAuthDomain();
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return The authDomain.
*/
java.lang.String getAuthDomain();
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return The bytes for authDomain.
*/
com.google.protobuf.ByteString
getAuthDomainBytes();
/**
* optional string user_organization = 4;
* @return Whether the userOrganization field is set.
*/
boolean hasUserOrganization();
/**
* optional string user_organization = 4;
* @return The userOrganization.
*/
java.lang.String getUserOrganization();
/**
* optional string user_organization = 4;
* @return The bytes for userOrganization.
*/
com.google.protobuf.ByteString
getUserOrganizationBytes();
/**
* optional bool is_admin = 5;
* @return Whether the isAdmin field is set.
*/
boolean hasIsAdmin();
/**
* optional bool is_admin = 5;
* @return The isAdmin.
*/
boolean getIsAdmin();
/**
* optional string client_id = 6;
* @return Whether the clientId field is set.
*/
boolean hasClientId();
/**
* optional string client_id = 6;
* @return The clientId.
*/
java.lang.String getClientId();
/**
* optional string client_id = 6;
* @return The bytes for clientId.
*/
com.google.protobuf.ByteString
getClientIdBytes();
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @return A list containing the scopes.
*/
java.util.List
getScopesList();
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @return The count of scopes.
*/
int getScopesCount();
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param index The index of the element to return.
* @return The scopes at the given index.
*/
java.lang.String getScopes(int index);
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param index The index of the value to return.
* @return The bytes of the scopes at the given index.
*/
com.google.protobuf.ByteString
getScopesBytes(int index);
/**
*
* Deprecated. Never set.
*
*
* optional bool is_project_writer = 8 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserResponse.is_project_writer is deprecated.
* See user_service.proto;l=89
* @return Whether the isProjectWriter field is set.
*/
@java.lang.Deprecated boolean hasIsProjectWriter();
/**
*
* Deprecated. Never set.
*
*
* optional bool is_project_writer = 8 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserResponse.is_project_writer is deprecated.
* See user_service.proto;l=89
* @return The isProjectWriter.
*/
@java.lang.Deprecated boolean getIsProjectWriter();
}
/**
* Protobuf type {@code java.apphosting.GetOAuthUserResponse}
*/
public static final class GetOAuthUserResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.GetOAuthUserResponse)
GetOAuthUserResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetOAuthUserResponse.newBuilder() to construct.
private GetOAuthUserResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetOAuthUserResponse() {
email_ = "";
userId_ = "";
authDomain_ = "";
userOrganization_ = "";
clientId_ = "";
scopes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetOAuthUserResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.GetOAuthUserResponse.class, com.google.apphosting.api.UserServicePb.GetOAuthUserResponse.Builder.class);
}
private int bitField0_;
public static final int EMAIL_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object email_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return Whether the email field is set.
*/
@java.lang.Override
public boolean hasEmail() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return The email.
*/
@java.lang.Override
public java.lang.String getEmail() {
java.lang.Object ref = email_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
email_ = s;
}
return s;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return The bytes for email.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getEmailBytes() {
java.lang.Object ref = email_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
email_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USER_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object userId_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return Whether the userId field is set.
*/
@java.lang.Override
public boolean hasUserId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return The userId.
*/
@java.lang.Override
public java.lang.String getUserId() {
java.lang.Object ref = userId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userId_ = s;
}
return s;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return The bytes for userId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserIdBytes() {
java.lang.Object ref = userId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int AUTH_DOMAIN_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object authDomain_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return Whether the authDomain field is set.
*/
@java.lang.Override
public boolean hasAuthDomain() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return The authDomain.
*/
@java.lang.Override
public java.lang.String getAuthDomain() {
java.lang.Object ref = authDomain_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
authDomain_ = s;
}
return s;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return The bytes for authDomain.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAuthDomainBytes() {
java.lang.Object ref = authDomain_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
authDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USER_ORGANIZATION_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object userOrganization_ = "";
/**
* optional string user_organization = 4;
* @return Whether the userOrganization field is set.
*/
@java.lang.Override
public boolean hasUserOrganization() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string user_organization = 4;
* @return The userOrganization.
*/
@java.lang.Override
public java.lang.String getUserOrganization() {
java.lang.Object ref = userOrganization_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userOrganization_ = s;
}
return s;
}
}
/**
* optional string user_organization = 4;
* @return The bytes for userOrganization.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserOrganizationBytes() {
java.lang.Object ref = userOrganization_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userOrganization_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IS_ADMIN_FIELD_NUMBER = 5;
private boolean isAdmin_ = false;
/**
* optional bool is_admin = 5;
* @return Whether the isAdmin field is set.
*/
@java.lang.Override
public boolean hasIsAdmin() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional bool is_admin = 5;
* @return The isAdmin.
*/
@java.lang.Override
public boolean getIsAdmin() {
return isAdmin_;
}
public static final int CLIENT_ID_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object clientId_ = "";
/**
* optional string client_id = 6;
* @return Whether the clientId field is set.
*/
@java.lang.Override
public boolean hasClientId() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional string client_id = 6;
* @return The clientId.
*/
@java.lang.Override
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientId_ = s;
}
return s;
}
}
/**
* optional string client_id = 6;
* @return The bytes for clientId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCOPES_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringList scopes_;
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @return A list containing the scopes.
*/
public com.google.protobuf.ProtocolStringList
getScopesList() {
return scopes_;
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @return The count of scopes.
*/
public int getScopesCount() {
return scopes_.size();
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param index The index of the element to return.
* @return The scopes at the given index.
*/
public java.lang.String getScopes(int index) {
return scopes_.get(index);
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param index The index of the value to return.
* @return The bytes of the scopes at the given index.
*/
public com.google.protobuf.ByteString
getScopesBytes(int index) {
return scopes_.getByteString(index);
}
public static final int IS_PROJECT_WRITER_FIELD_NUMBER = 8;
private boolean isProjectWriter_ = false;
/**
*
* Deprecated. Never set.
*
*
* optional bool is_project_writer = 8 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserResponse.is_project_writer is deprecated.
* See user_service.proto;l=89
* @return Whether the isProjectWriter field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasIsProjectWriter() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Deprecated. Never set.
*
*
* optional bool is_project_writer = 8 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserResponse.is_project_writer is deprecated.
* See user_service.proto;l=89
* @return The isProjectWriter.
*/
@java.lang.Override
@java.lang.Deprecated public boolean getIsProjectWriter() {
return isProjectWriter_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, email_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, userId_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, authDomain_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, userOrganization_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(5, isAdmin_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, clientId_);
}
for (int i = 0; i < scopes_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, scopes_.getRaw(i));
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeBool(8, isProjectWriter_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, email_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, userId_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, authDomain_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, userOrganization_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, isAdmin_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, clientId_);
}
{
int dataSize = 0;
for (int i = 0; i < scopes_.size(); i++) {
dataSize += computeStringSizeNoTag(scopes_.getRaw(i));
}
size += dataSize;
size += 1 * getScopesList().size();
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, isProjectWriter_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.UserServicePb.GetOAuthUserResponse)) {
return super.equals(obj);
}
com.google.apphosting.api.UserServicePb.GetOAuthUserResponse other = (com.google.apphosting.api.UserServicePb.GetOAuthUserResponse) obj;
if (hasEmail() != other.hasEmail()) return false;
if (hasEmail()) {
if (!getEmail()
.equals(other.getEmail())) return false;
}
if (hasUserId() != other.hasUserId()) return false;
if (hasUserId()) {
if (!getUserId()
.equals(other.getUserId())) return false;
}
if (hasAuthDomain() != other.hasAuthDomain()) return false;
if (hasAuthDomain()) {
if (!getAuthDomain()
.equals(other.getAuthDomain())) return false;
}
if (hasUserOrganization() != other.hasUserOrganization()) return false;
if (hasUserOrganization()) {
if (!getUserOrganization()
.equals(other.getUserOrganization())) return false;
}
if (hasIsAdmin() != other.hasIsAdmin()) return false;
if (hasIsAdmin()) {
if (getIsAdmin()
!= other.getIsAdmin()) return false;
}
if (hasClientId() != other.hasClientId()) return false;
if (hasClientId()) {
if (!getClientId()
.equals(other.getClientId())) return false;
}
if (!getScopesList()
.equals(other.getScopesList())) return false;
if (hasIsProjectWriter() != other.hasIsProjectWriter()) return false;
if (hasIsProjectWriter()) {
if (getIsProjectWriter()
!= other.getIsProjectWriter()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasEmail()) {
hash = (37 * hash) + EMAIL_FIELD_NUMBER;
hash = (53 * hash) + getEmail().hashCode();
}
if (hasUserId()) {
hash = (37 * hash) + USER_ID_FIELD_NUMBER;
hash = (53 * hash) + getUserId().hashCode();
}
if (hasAuthDomain()) {
hash = (37 * hash) + AUTH_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getAuthDomain().hashCode();
}
if (hasUserOrganization()) {
hash = (37 * hash) + USER_ORGANIZATION_FIELD_NUMBER;
hash = (53 * hash) + getUserOrganization().hashCode();
}
if (hasIsAdmin()) {
hash = (37 * hash) + IS_ADMIN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsAdmin());
}
if (hasClientId()) {
hash = (37 * hash) + CLIENT_ID_FIELD_NUMBER;
hash = (53 * hash) + getClientId().hashCode();
}
if (getScopesCount() > 0) {
hash = (37 * hash) + SCOPES_FIELD_NUMBER;
hash = (53 * hash) + getScopesList().hashCode();
}
if (hasIsProjectWriter()) {
hash = (37 * hash) + IS_PROJECT_WRITER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsProjectWriter());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.UserServicePb.GetOAuthUserResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code java.apphosting.GetOAuthUserResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.GetOAuthUserResponse)
com.google.apphosting.api.UserServicePb.GetOAuthUserResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.UserServicePb.GetOAuthUserResponse.class, com.google.apphosting.api.UserServicePb.GetOAuthUserResponse.Builder.class);
}
// Construct using com.google.apphosting.api.UserServicePb.GetOAuthUserResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
email_ = "";
userId_ = "";
authDomain_ = "";
userOrganization_ = "";
isAdmin_ = false;
clientId_ = "";
scopes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
isProjectWriter_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.UserServicePb.internal_static_java_apphosting_GetOAuthUserResponse_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.GetOAuthUserResponse getDefaultInstanceForType() {
return com.google.apphosting.api.UserServicePb.GetOAuthUserResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.GetOAuthUserResponse build() {
com.google.apphosting.api.UserServicePb.GetOAuthUserResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.GetOAuthUserResponse buildPartial() {
com.google.apphosting.api.UserServicePb.GetOAuthUserResponse result = new com.google.apphosting.api.UserServicePb.GetOAuthUserResponse(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.api.UserServicePb.GetOAuthUserResponse result) {
if (((bitField0_ & 0x00000040) != 0)) {
scopes_ = scopes_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000040);
}
result.scopes_ = scopes_;
}
private void buildPartial0(com.google.apphosting.api.UserServicePb.GetOAuthUserResponse result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.email_ = email_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.userId_ = userId_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.authDomain_ = authDomain_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.userOrganization_ = userOrganization_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.isAdmin_ = isAdmin_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.clientId_ = clientId_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.isProjectWriter_ = isProjectWriter_;
to_bitField0_ |= 0x00000040;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.UserServicePb.GetOAuthUserResponse) {
return mergeFrom((com.google.apphosting.api.UserServicePb.GetOAuthUserResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.UserServicePb.GetOAuthUserResponse other) {
if (other == com.google.apphosting.api.UserServicePb.GetOAuthUserResponse.getDefaultInstance()) return this;
if (other.hasEmail()) {
email_ = other.email_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasUserId()) {
userId_ = other.userId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasAuthDomain()) {
authDomain_ = other.authDomain_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasUserOrganization()) {
userOrganization_ = other.userOrganization_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasIsAdmin()) {
setIsAdmin(other.getIsAdmin());
}
if (other.hasClientId()) {
clientId_ = other.clientId_;
bitField0_ |= 0x00000020;
onChanged();
}
if (!other.scopes_.isEmpty()) {
if (scopes_.isEmpty()) {
scopes_ = other.scopes_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureScopesIsMutable();
scopes_.addAll(other.scopes_);
}
onChanged();
}
if (other.hasIsProjectWriter()) {
setIsProjectWriter(other.getIsProjectWriter());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
email_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
userId_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
authDomain_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
userOrganization_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
case 40: {
isAdmin_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 40
case 50: {
clientId_ = input.readBytes();
bitField0_ |= 0x00000020;
break;
} // case 50
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
ensureScopesIsMutable();
scopes_.add(bs);
break;
} // case 58
case 64: {
isProjectWriter_ = input.readBool();
bitField0_ |= 0x00000080;
break;
} // case 64
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object email_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return Whether the email field is set.
*/
public boolean hasEmail() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return The email.
*/
public java.lang.String getEmail() {
java.lang.Object ref = email_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
email_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return The bytes for email.
*/
public com.google.protobuf.ByteString
getEmailBytes() {
java.lang.Object ref = email_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
email_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @param value The email to set.
* @return This builder for chaining.
*/
public Builder setEmail(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
email_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @return This builder for chaining.
*/
public Builder clearEmail() {
email_ = getDefaultInstance().getEmail();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string email = 1;
* @param value The bytes for email to set.
* @return This builder for chaining.
*/
public Builder setEmailBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
email_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object userId_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return Whether the userId field is set.
*/
public boolean hasUserId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return The userId.
*/
public java.lang.String getUserId() {
java.lang.Object ref = userId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return The bytes for userId.
*/
public com.google.protobuf.ByteString
getUserIdBytes() {
java.lang.Object ref = userId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @param value The userId to set.
* @return This builder for chaining.
*/
public Builder setUserId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
userId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @return This builder for chaining.
*/
public Builder clearUserId() {
userId_ = getDefaultInstance().getUserId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string user_id = 2;
* @param value The bytes for userId to set.
* @return This builder for chaining.
*/
public Builder setUserIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
userId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object authDomain_ = "";
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return Whether the authDomain field is set.
*/
public boolean hasAuthDomain() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return The authDomain.
*/
public java.lang.String getAuthDomain() {
java.lang.Object ref = authDomain_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
authDomain_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return The bytes for authDomain.
*/
public com.google.protobuf.ByteString
getAuthDomainBytes() {
java.lang.Object ref = authDomain_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
authDomain_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @param value The authDomain to set.
* @return This builder for chaining.
*/
public Builder setAuthDomain(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
authDomain_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @return This builder for chaining.
*/
public Builder clearAuthDomain() {
authDomain_ = getDefaultInstance().getAuthDomain();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Required, but not proto enforced.
*
*
* optional string auth_domain = 3;
* @param value The bytes for authDomain to set.
* @return This builder for chaining.
*/
public Builder setAuthDomainBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
authDomain_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object userOrganization_ = "";
/**
* optional string user_organization = 4;
* @return Whether the userOrganization field is set.
*/
public boolean hasUserOrganization() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional string user_organization = 4;
* @return The userOrganization.
*/
public java.lang.String getUserOrganization() {
java.lang.Object ref = userOrganization_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userOrganization_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string user_organization = 4;
* @return The bytes for userOrganization.
*/
public com.google.protobuf.ByteString
getUserOrganizationBytes() {
java.lang.Object ref = userOrganization_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userOrganization_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string user_organization = 4;
* @param value The userOrganization to set.
* @return This builder for chaining.
*/
public Builder setUserOrganization(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
userOrganization_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional string user_organization = 4;
* @return This builder for chaining.
*/
public Builder clearUserOrganization() {
userOrganization_ = getDefaultInstance().getUserOrganization();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* optional string user_organization = 4;
* @param value The bytes for userOrganization to set.
* @return This builder for chaining.
*/
public Builder setUserOrganizationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
userOrganization_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private boolean isAdmin_ ;
/**
* optional bool is_admin = 5;
* @return Whether the isAdmin field is set.
*/
@java.lang.Override
public boolean hasIsAdmin() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
* optional bool is_admin = 5;
* @return The isAdmin.
*/
@java.lang.Override
public boolean getIsAdmin() {
return isAdmin_;
}
/**
* optional bool is_admin = 5;
* @param value The isAdmin to set.
* @return This builder for chaining.
*/
public Builder setIsAdmin(boolean value) {
isAdmin_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* optional bool is_admin = 5;
* @return This builder for chaining.
*/
public Builder clearIsAdmin() {
bitField0_ = (bitField0_ & ~0x00000010);
isAdmin_ = false;
onChanged();
return this;
}
private java.lang.Object clientId_ = "";
/**
* optional string client_id = 6;
* @return Whether the clientId field is set.
*/
public boolean hasClientId() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
* optional string client_id = 6;
* @return The clientId.
*/
public java.lang.String getClientId() {
java.lang.Object ref = clientId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
clientId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string client_id = 6;
* @return The bytes for clientId.
*/
public com.google.protobuf.ByteString
getClientIdBytes() {
java.lang.Object ref = clientId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clientId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string client_id = 6;
* @param value The clientId to set.
* @return This builder for chaining.
*/
public Builder setClientId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
clientId_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* optional string client_id = 6;
* @return This builder for chaining.
*/
public Builder clearClientId() {
clientId_ = getDefaultInstance().getClientId();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
* optional string client_id = 6;
* @param value The bytes for clientId to set.
* @return This builder for chaining.
*/
public Builder setClientIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
clientId_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList scopes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureScopesIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
scopes_ = new com.google.protobuf.LazyStringArrayList(scopes_);
bitField0_ |= 0x00000040;
}
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @return A list containing the scopes.
*/
public com.google.protobuf.ProtocolStringList
getScopesList() {
return scopes_.getUnmodifiableView();
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @return The count of scopes.
*/
public int getScopesCount() {
return scopes_.size();
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param index The index of the element to return.
* @return The scopes at the given index.
*/
public java.lang.String getScopes(int index) {
return scopes_.get(index);
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param index The index of the value to return.
* @return The bytes of the scopes at the given index.
*/
public com.google.protobuf.ByteString
getScopesBytes(int index) {
return scopes_.getByteString(index);
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param index The index to set the value at.
* @param value The scopes to set.
* @return This builder for chaining.
*/
public Builder setScopes(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureScopesIsMutable();
scopes_.set(index, value);
onChanged();
return this;
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param value The scopes to add.
* @return This builder for chaining.
*/
public Builder addScopes(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureScopesIsMutable();
scopes_.add(value);
onChanged();
return this;
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param values The scopes to add.
* @return This builder for chaining.
*/
public Builder addAllScopes(
java.lang.Iterable values) {
ensureScopesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, scopes_);
onChanged();
return this;
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @return This builder for chaining.
*/
public Builder clearScopes() {
scopes_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* Authorized scopes from GetOAuthUserRequest.
*
*
* repeated string scopes = 7;
* @param value The bytes of the scopes to add.
* @return This builder for chaining.
*/
public Builder addScopesBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
ensureScopesIsMutable();
scopes_.add(value);
onChanged();
return this;
}
private boolean isProjectWriter_ ;
/**
*
* Deprecated. Never set.
*
*
* optional bool is_project_writer = 8 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserResponse.is_project_writer is deprecated.
* See user_service.proto;l=89
* @return Whether the isProjectWriter field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasIsProjectWriter() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Deprecated. Never set.
*
*
* optional bool is_project_writer = 8 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserResponse.is_project_writer is deprecated.
* See user_service.proto;l=89
* @return The isProjectWriter.
*/
@java.lang.Override
@java.lang.Deprecated public boolean getIsProjectWriter() {
return isProjectWriter_;
}
/**
*
* Deprecated. Never set.
*
*
* optional bool is_project_writer = 8 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserResponse.is_project_writer is deprecated.
* See user_service.proto;l=89
* @param value The isProjectWriter to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setIsProjectWriter(boolean value) {
isProjectWriter_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Deprecated. Never set.
*
*
* optional bool is_project_writer = 8 [deprecated = true];
* @deprecated java.apphosting.GetOAuthUserResponse.is_project_writer is deprecated.
* See user_service.proto;l=89
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearIsProjectWriter() {
bitField0_ = (bitField0_ & ~0x00000080);
isProjectWriter_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.GetOAuthUserResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.GetOAuthUserResponse)
private static final com.google.apphosting.api.UserServicePb.GetOAuthUserResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.UserServicePb.GetOAuthUserResponse();
}
public static com.google.apphosting.api.UserServicePb.GetOAuthUserResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetOAuthUserResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.UserServicePb.GetOAuthUserResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_UserServiceError_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_UserServiceError_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_CreateLoginURLRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_CreateLoginURLRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_CreateLoginURLResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_CreateLoginURLResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_CreateLogoutURLRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_CreateLogoutURLRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_CreateLogoutURLResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_CreateLogoutURLResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_GetOAuthUserRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_GetOAuthUserRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_GetOAuthUserResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_GetOAuthUserResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\022user_service.proto\022\017java.apphosting\"\231\001" +
"\n\020UserServiceError\"\204\001\n\tErrorCode\022\006\n\002OK\020\000" +
"\022\031\n\025REDIRECT_URL_TOO_LONG\020\001\022\017\n\013NOT_ALLOW" +
"ED\020\002\022\027\n\023OAUTH_INVALID_TOKEN\020\003\022\031\n\025OAUTH_I" +
"NVALID_REQUEST\020\004\022\017\n\013OAUTH_ERROR\020\005\"a\n\025Cre" +
"ateLoginURLRequest\022\027\n\017destination_url\030\001 " +
"\002(\t\022\023\n\013auth_domain\030\002 \001(\t\022\032\n\022federated_id" +
"entity\030\003 \001(\t\"+\n\026CreateLoginURLResponse\022\021" +
"\n\tlogin_url\030\001 \001(\t\"F\n\026CreateLogoutURLRequ" +
"est\022\027\n\017destination_url\030\001 \002(\t\022\023\n\013auth_dom" +
"ain\030\002 \001(\t\"-\n\027CreateLogoutURLResponse\022\022\n\n" +
"logout_url\030\001 \001(\t\"[\n\023GetOAuthUserRequest\022" +
"\r\n\005scope\030\001 \001(\t\022\016\n\006scopes\030\002 \003(\t\022%\n\031reques" +
"t_writer_permission\030\003 \001(\010B\002\030\001\"\272\001\n\024GetOAu" +
"thUserResponse\022\r\n\005email\030\001 \001(\t\022\017\n\007user_id" +
"\030\002 \001(\t\022\023\n\013auth_domain\030\003 \001(\t\022\031\n\021user_orga" +
"nization\030\004 \001(\t\022\020\n\010is_admin\030\005 \001(\010\022\021\n\tclie" +
"nt_id\030\006 \001(\t\022\016\n\006scopes\030\007 \003(\t\022\035\n\021is_projec" +
"t_writer\030\010 \001(\010B\002\030\001B*\n\031com.google.apphost" +
"ing.apiB\rUserServicePb"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_java_apphosting_UserServiceError_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_java_apphosting_UserServiceError_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_UserServiceError_descriptor,
new java.lang.String[] { });
internal_static_java_apphosting_CreateLoginURLRequest_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_java_apphosting_CreateLoginURLRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_CreateLoginURLRequest_descriptor,
new java.lang.String[] { "DestinationUrl", "AuthDomain", "FederatedIdentity", });
internal_static_java_apphosting_CreateLoginURLResponse_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_java_apphosting_CreateLoginURLResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_CreateLoginURLResponse_descriptor,
new java.lang.String[] { "LoginUrl", });
internal_static_java_apphosting_CreateLogoutURLRequest_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_java_apphosting_CreateLogoutURLRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_CreateLogoutURLRequest_descriptor,
new java.lang.String[] { "DestinationUrl", "AuthDomain", });
internal_static_java_apphosting_CreateLogoutURLResponse_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_java_apphosting_CreateLogoutURLResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_CreateLogoutURLResponse_descriptor,
new java.lang.String[] { "LogoutUrl", });
internal_static_java_apphosting_GetOAuthUserRequest_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_java_apphosting_GetOAuthUserRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_GetOAuthUserRequest_descriptor,
new java.lang.String[] { "Scope", "Scopes", "RequestWriterPermission", });
internal_static_java_apphosting_GetOAuthUserResponse_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_java_apphosting_GetOAuthUserResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_GetOAuthUserResponse_descriptor,
new java.lang.String[] { "Email", "UserId", "AuthDomain", "UserOrganization", "IsAdmin", "ClientId", "Scopes", "IsProjectWriter", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy