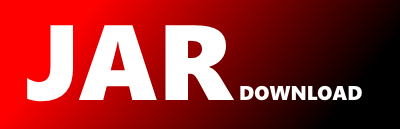
com.google.apphosting.api.search.DocumentPb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: document.proto
package com.google.apphosting.api.search;
public final class DocumentPb {
private DocumentPb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface FieldValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.FieldValue)
com.google.protobuf.MessageOrBuilder {
/**
*
* The type of content in this field value.
*
*
* optional .storage_onestore_v3.FieldValue.ContentType type = 1 [default = TEXT];
* @return Whether the type field is set.
*/
boolean hasType();
/**
*
* The type of content in this field value.
*
*
* optional .storage_onestore_v3.FieldValue.ContentType type = 1 [default = TEXT];
* @return The type.
*/
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType getType();
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return Whether the language field is set.
*/
boolean hasLanguage();
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The language.
*/
java.lang.String getLanguage();
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The bytes for language.
*/
com.google.protobuf.ByteString
getLanguageBytes();
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return Whether the stringValue field is set.
*/
boolean hasStringValue();
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return The stringValue.
*/
java.lang.String getStringValue();
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return The bytes for stringValue.
*/
com.google.protobuf.ByteString
getStringValueBytes();
/**
* optional group Geo = 4 { ... }
* @return Whether the geo field is set.
*/
boolean hasGeo();
/**
* optional group Geo = 4 { ... }
* @return The geo.
*/
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo getGeo();
/**
* optional group Geo = 4 { ... }
*/
com.google.apphosting.api.search.DocumentPb.FieldValue.GeoOrBuilder getGeoOrBuilder();
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @return A list containing the vectorValue.
*/
java.util.List getVectorValueList();
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @return The count of vectorValue.
*/
int getVectorValueCount();
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @param index The index of the element to return.
* @return The vectorValue at the given index.
*/
double getVectorValue(int index);
}
/**
*
* The value of a document field.
*
*
* Protobuf type {@code storage_onestore_v3.FieldValue}
*/
public static final class FieldValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.FieldValue)
FieldValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use FieldValue.newBuilder() to construct.
private FieldValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FieldValue() {
type_ = 0;
language_ = "en";
stringValue_ = "";
vectorValue_ = emptyDoubleList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FieldValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.FieldValue.class, com.google.apphosting.api.search.DocumentPb.FieldValue.Builder.class);
}
/**
*
* The type of content in the field value.
*
*
* Protobuf enum {@code storage_onestore_v3.FieldValue.ContentType}
*/
public enum ContentType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The content is plain text.
*
*
* TEXT = 0;
*/
TEXT(0),
/**
*
* The content is HTML.
*
*
* HTML = 1;
*/
HTML(1),
/**
*
* The content is indivisible text.
*
*
* ATOM = 2;
*/
ATOM(2),
/**
*
* The content is a date.
*
*
* DATE = 3;
*/
DATE(3),
/**
*
* The content is a number.
*
*
* NUMBER = 4;
*/
NUMBER(4),
/**
*
* The content is a location.
*
*
* GEO = 5;
*/
GEO(5),
/**
*
* The content is an untokenized prefix field.
*
*
* UNTOKENIZED_PREFIX = 6;
*/
UNTOKENIZED_PREFIX(6),
/**
*
* The content is a tokenized prefix field.
*
*
* TOKENIZED_PREFIX = 7;
*/
TOKENIZED_PREFIX(7),
/**
*
* The content is a vector of double numbers
*
*
* VECTOR = 8;
*/
VECTOR(8),
;
/**
*
* The content is plain text.
*
*
* TEXT = 0;
*/
public static final int TEXT_VALUE = 0;
/**
*
* The content is HTML.
*
*
* HTML = 1;
*/
public static final int HTML_VALUE = 1;
/**
*
* The content is indivisible text.
*
*
* ATOM = 2;
*/
public static final int ATOM_VALUE = 2;
/**
*
* The content is a date.
*
*
* DATE = 3;
*/
public static final int DATE_VALUE = 3;
/**
*
* The content is a number.
*
*
* NUMBER = 4;
*/
public static final int NUMBER_VALUE = 4;
/**
*
* The content is a location.
*
*
* GEO = 5;
*/
public static final int GEO_VALUE = 5;
/**
*
* The content is an untokenized prefix field.
*
*
* UNTOKENIZED_PREFIX = 6;
*/
public static final int UNTOKENIZED_PREFIX_VALUE = 6;
/**
*
* The content is a tokenized prefix field.
*
*
* TOKENIZED_PREFIX = 7;
*/
public static final int TOKENIZED_PREFIX_VALUE = 7;
/**
*
* The content is a vector of double numbers
*
*
* VECTOR = 8;
*/
public static final int VECTOR_VALUE = 8;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ContentType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ContentType forNumber(int value) {
switch (value) {
case 0: return TEXT;
case 1: return HTML;
case 2: return ATOM;
case 3: return DATE;
case 4: return NUMBER;
case 5: return GEO;
case 6: return UNTOKENIZED_PREFIX;
case 7: return TOKENIZED_PREFIX;
case 8: return VECTOR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ContentType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ContentType findValueByNumber(int number) {
return ContentType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.FieldValue.getDescriptor().getEnumTypes().get(0);
}
private static final ContentType[] VALUES = values();
public static ContentType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ContentType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:storage_onestore_v3.FieldValue.ContentType)
}
public interface GeoOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.FieldValue.Geo)
com.google.protobuf.MessageOrBuilder {
/**
* required double lat = 5;
* @return Whether the lat field is set.
*/
boolean hasLat();
/**
* required double lat = 5;
* @return The lat.
*/
double getLat();
/**
* required double lng = 6;
* @return Whether the lng field is set.
*/
boolean hasLng();
/**
* required double lng = 6;
* @return The lng.
*/
double getLng();
}
/**
*
* The field in which GEO values are stored
*
*
* Protobuf type {@code storage_onestore_v3.FieldValue.Geo}
*/
public static final class Geo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.FieldValue.Geo)
GeoOrBuilder {
private static final long serialVersionUID = 0L;
// Use Geo.newBuilder() to construct.
private Geo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Geo() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Geo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_Geo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_Geo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.class, com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.Builder.class);
}
private int bitField0_;
public static final int LAT_FIELD_NUMBER = 5;
private double lat_ = 0D;
/**
* required double lat = 5;
* @return Whether the lat field is set.
*/
@java.lang.Override
public boolean hasLat() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required double lat = 5;
* @return The lat.
*/
@java.lang.Override
public double getLat() {
return lat_;
}
public static final int LNG_FIELD_NUMBER = 6;
private double lng_ = 0D;
/**
* required double lng = 6;
* @return Whether the lng field is set.
*/
@java.lang.Override
public boolean hasLng() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required double lng = 6;
* @return The lng.
*/
@java.lang.Override
public double getLng() {
return lng_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasLat()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasLng()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeDouble(5, lat_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeDouble(6, lng_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, lat_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(6, lng_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.FieldValue.Geo)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo other = (com.google.apphosting.api.search.DocumentPb.FieldValue.Geo) obj;
if (hasLat() != other.hasLat()) return false;
if (hasLat()) {
if (java.lang.Double.doubleToLongBits(getLat())
!= java.lang.Double.doubleToLongBits(
other.getLat())) return false;
}
if (hasLng() != other.hasLng()) return false;
if (hasLng()) {
if (java.lang.Double.doubleToLongBits(getLng())
!= java.lang.Double.doubleToLongBits(
other.getLng())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasLat()) {
hash = (37 * hash) + LAT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getLat()));
}
if (hasLng()) {
hash = (37 * hash) + LNG_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getLng()));
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.FieldValue.Geo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The field in which GEO values are stored
*
*
* Protobuf type {@code storage_onestore_v3.FieldValue.Geo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.FieldValue.Geo)
com.google.apphosting.api.search.DocumentPb.FieldValue.GeoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_Geo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_Geo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.class, com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
lat_ = 0D;
lng_ = 0D;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_Geo_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue.Geo getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue.Geo build() {
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue.Geo buildPartial() {
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo result = new com.google.apphosting.api.search.DocumentPb.FieldValue.Geo(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.FieldValue.Geo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.lat_ = lat_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.lng_ = lng_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.FieldValue.Geo) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.FieldValue.Geo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.FieldValue.Geo other) {
if (other == com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.getDefaultInstance()) return this;
if (other.hasLat()) {
setLat(other.getLat());
}
if (other.hasLng()) {
setLng(other.getLng());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasLat()) {
return false;
}
if (!hasLng()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 41: {
lat_ = input.readDouble();
bitField0_ |= 0x00000001;
break;
} // case 41
case 49: {
lng_ = input.readDouble();
bitField0_ |= 0x00000002;
break;
} // case 49
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private double lat_ ;
/**
* required double lat = 5;
* @return Whether the lat field is set.
*/
@java.lang.Override
public boolean hasLat() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required double lat = 5;
* @return The lat.
*/
@java.lang.Override
public double getLat() {
return lat_;
}
/**
* required double lat = 5;
* @param value The lat to set.
* @return This builder for chaining.
*/
public Builder setLat(double value) {
lat_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required double lat = 5;
* @return This builder for chaining.
*/
public Builder clearLat() {
bitField0_ = (bitField0_ & ~0x00000001);
lat_ = 0D;
onChanged();
return this;
}
private double lng_ ;
/**
* required double lng = 6;
* @return Whether the lng field is set.
*/
@java.lang.Override
public boolean hasLng() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required double lng = 6;
* @return The lng.
*/
@java.lang.Override
public double getLng() {
return lng_;
}
/**
* required double lng = 6;
* @param value The lng to set.
* @return This builder for chaining.
*/
public Builder setLng(double value) {
lng_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required double lng = 6;
* @return This builder for chaining.
*/
public Builder clearLng() {
bitField0_ = (bitField0_ & ~0x00000002);
lng_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.FieldValue.Geo)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.FieldValue.Geo)
private static final com.google.apphosting.api.search.DocumentPb.FieldValue.Geo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.FieldValue.Geo();
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue.Geo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Geo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue.Geo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_ = 0;
/**
*
* The type of content in this field value.
*
*
* optional .storage_onestore_v3.FieldValue.ContentType type = 1 [default = TEXT];
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The type of content in this field value.
*
*
* optional .storage_onestore_v3.FieldValue.ContentType type = 1 [default = TEXT];
* @return The type.
*/
@java.lang.Override public com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType getType() {
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType result = com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.forNumber(type_);
return result == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.TEXT : result;
}
public static final int LANGUAGE_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object language_ = "en";
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return Whether the language field is set.
*/
@java.lang.Override
public boolean hasLanguage() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The language.
*/
@java.lang.Override
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
}
}
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The bytes for language.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STRING_VALUE_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object stringValue_ = "";
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return Whether the stringValue field is set.
*/
@java.lang.Override
public boolean hasStringValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return The stringValue.
*/
@java.lang.Override
public java.lang.String getStringValue() {
java.lang.Object ref = stringValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stringValue_ = s;
}
return s;
}
}
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return The bytes for stringValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = stringValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stringValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GEO_FIELD_NUMBER = 4;
private com.google.apphosting.api.search.DocumentPb.FieldValue.Geo geo_;
/**
* optional group Geo = 4 { ... }
* @return Whether the geo field is set.
*/
@java.lang.Override
public boolean hasGeo() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional group Geo = 4 { ... }
* @return The geo.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue.Geo getGeo() {
return geo_ == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.getDefaultInstance() : geo_;
}
/**
* optional group Geo = 4 { ... }
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue.GeoOrBuilder getGeoOrBuilder() {
return geo_ == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.getDefaultInstance() : geo_;
}
public static final int VECTOR_VALUE_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.DoubleList vectorValue_;
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @return A list containing the vectorValue.
*/
@java.lang.Override
public java.util.List
getVectorValueList() {
return vectorValue_;
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @return The count of vectorValue.
*/
public int getVectorValueCount() {
return vectorValue_.size();
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @param index The index of the element to return.
* @return The vectorValue at the given index.
*/
public double getVectorValue(int index) {
return vectorValue_.getDouble(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasGeo()) {
if (!getGeo().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, language_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, stringValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeGroup(4, getGeo());
}
for (int i = 0; i < vectorValue_.size(); i++) {
output.writeDouble(7, vectorValue_.getDouble(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, language_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, stringValue_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(4, getGeo());
}
{
int dataSize = 0;
dataSize = 8 * getVectorValueList().size();
size += dataSize;
size += 1 * getVectorValueList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.FieldValue)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.FieldValue other = (com.google.apphosting.api.search.DocumentPb.FieldValue) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasLanguage() != other.hasLanguage()) return false;
if (hasLanguage()) {
if (!getLanguage()
.equals(other.getLanguage())) return false;
}
if (hasStringValue() != other.hasStringValue()) return false;
if (hasStringValue()) {
if (!getStringValue()
.equals(other.getStringValue())) return false;
}
if (hasGeo() != other.hasGeo()) return false;
if (hasGeo()) {
if (!getGeo()
.equals(other.getGeo())) return false;
}
if (!getVectorValueList()
.equals(other.getVectorValueList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasLanguage()) {
hash = (37 * hash) + LANGUAGE_FIELD_NUMBER;
hash = (53 * hash) + getLanguage().hashCode();
}
if (hasStringValue()) {
hash = (37 * hash) + STRING_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getStringValue().hashCode();
}
if (hasGeo()) {
hash = (37 * hash) + GEO_FIELD_NUMBER;
hash = (53 * hash) + getGeo().hashCode();
}
if (getVectorValueCount() > 0) {
hash = (37 * hash) + VECTOR_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getVectorValueList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.FieldValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The value of a document field.
*
*
* Protobuf type {@code storage_onestore_v3.FieldValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.FieldValue)
com.google.apphosting.api.search.DocumentPb.FieldValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.FieldValue.class, com.google.apphosting.api.search.DocumentPb.FieldValue.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.FieldValue.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getGeoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
type_ = 0;
language_ = "en";
stringValue_ = "";
geo_ = null;
if (geoBuilder_ != null) {
geoBuilder_.dispose();
geoBuilder_ = null;
}
vectorValue_ = emptyDoubleList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldValue_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.FieldValue.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue build() {
com.google.apphosting.api.search.DocumentPb.FieldValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue buildPartial() {
com.google.apphosting.api.search.DocumentPb.FieldValue result = new com.google.apphosting.api.search.DocumentPb.FieldValue(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.api.search.DocumentPb.FieldValue result) {
if (((bitField0_ & 0x00000010) != 0)) {
vectorValue_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000010);
}
result.vectorValue_ = vectorValue_;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.FieldValue result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.type_ = type_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.language_ = language_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.stringValue_ = stringValue_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.geo_ = geoBuilder_ == null
? geo_
: geoBuilder_.build();
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.FieldValue) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.FieldValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.FieldValue other) {
if (other == com.google.apphosting.api.search.DocumentPb.FieldValue.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasLanguage()) {
language_ = other.language_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasStringValue()) {
stringValue_ = other.stringValue_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasGeo()) {
mergeGeo(other.getGeo());
}
if (!other.vectorValue_.isEmpty()) {
if (vectorValue_.isEmpty()) {
vectorValue_ = other.vectorValue_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureVectorValueIsMutable();
vectorValue_.addAll(other.vectorValue_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasGeo()) {
if (!getGeo().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int tmpRaw = input.readEnum();
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType tmpValue =
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
type_ = tmpRaw;
bitField0_ |= 0x00000001;
}
break;
} // case 8
case 18: {
language_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
stringValue_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 35: {
input.readGroup(4,
getGeoFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 35
case 57: {
double v = input.readDouble();
ensureVectorValueIsMutable();
vectorValue_.addDouble(v);
break;
} // case 57
case 58: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensureVectorValueIsMutable();
while (input.getBytesUntilLimit() > 0) {
vectorValue_.addDouble(input.readDouble());
}
input.popLimit(limit);
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int type_ = 0;
/**
*
* The type of content in this field value.
*
*
* optional .storage_onestore_v3.FieldValue.ContentType type = 1 [default = TEXT];
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The type of content in this field value.
*
*
* optional .storage_onestore_v3.FieldValue.ContentType type = 1 [default = TEXT];
* @return The type.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType getType() {
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType result = com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.forNumber(type_);
return result == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.TEXT : result;
}
/**
*
* The type of content in this field value.
*
*
* optional .storage_onestore_v3.FieldValue.ContentType type = 1 [default = TEXT];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The type of content in this field value.
*
*
* optional .storage_onestore_v3.FieldValue.ContentType type = 1 [default = TEXT];
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
private java.lang.Object language_ = "en";
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return Whether the language field is set.
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The language.
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The bytes for language.
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @param value The language to set.
* @return This builder for chaining.
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
language_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return This builder for chaining.
*/
public Builder clearLanguage() {
language_ = getDefaultInstance().getLanguage();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* The language of the content. The language must be a valid ISO 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @param value The bytes for language to set.
* @return This builder for chaining.
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
language_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object stringValue_ = "";
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return Whether the stringValue field is set.
*/
public boolean hasStringValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return The stringValue.
*/
public java.lang.String getStringValue() {
java.lang.Object ref = stringValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stringValue_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return The bytes for stringValue.
*/
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = stringValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stringValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @param value The stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValue(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
stringValue_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @return This builder for chaining.
*/
public Builder clearStringValue() {
stringValue_ = getDefaultInstance().getStringValue();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* The field in which HTML, TEXT, ATOM, DATE, NUMBER, UNTOKENIZED_PREFIX, and
* TOKENIZED_PREFIX values are stored. For DATE fields, it is the milliseconds
* since Unix epoch, formatted as what Java's Long.parseLong accepts.
* For NUMBER fields, it is formatted as what Java's Double.parseDouble
* accepts.
*
*
* optional string string_value = 3;
* @param value The bytes for stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
stringValue_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.apphosting.api.search.DocumentPb.FieldValue.Geo geo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo, com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.Builder, com.google.apphosting.api.search.DocumentPb.FieldValue.GeoOrBuilder> geoBuilder_;
/**
* optional group Geo = 4 { ... }
* @return Whether the geo field is set.
*/
public boolean hasGeo() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
* optional group Geo = 4 { ... }
* @return The geo.
*/
public com.google.apphosting.api.search.DocumentPb.FieldValue.Geo getGeo() {
if (geoBuilder_ == null) {
return geo_ == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.getDefaultInstance() : geo_;
} else {
return geoBuilder_.getMessage();
}
}
/**
* optional group Geo = 4 { ... }
*/
public Builder setGeo(com.google.apphosting.api.search.DocumentPb.FieldValue.Geo value) {
if (geoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
geo_ = value;
} else {
geoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional group Geo = 4 { ... }
*/
public Builder setGeo(
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.Builder builderForValue) {
if (geoBuilder_ == null) {
geo_ = builderForValue.build();
} else {
geoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional group Geo = 4 { ... }
*/
public Builder mergeGeo(com.google.apphosting.api.search.DocumentPb.FieldValue.Geo value) {
if (geoBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
geo_ != null &&
geo_ != com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.getDefaultInstance()) {
getGeoBuilder().mergeFrom(value);
} else {
geo_ = value;
}
} else {
geoBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* optional group Geo = 4 { ... }
*/
public Builder clearGeo() {
bitField0_ = (bitField0_ & ~0x00000008);
geo_ = null;
if (geoBuilder_ != null) {
geoBuilder_.dispose();
geoBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional group Geo = 4 { ... }
*/
public com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.Builder getGeoBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getGeoFieldBuilder().getBuilder();
}
/**
* optional group Geo = 4 { ... }
*/
public com.google.apphosting.api.search.DocumentPb.FieldValue.GeoOrBuilder getGeoOrBuilder() {
if (geoBuilder_ != null) {
return geoBuilder_.getMessageOrBuilder();
} else {
return geo_ == null ?
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.getDefaultInstance() : geo_;
}
}
/**
* optional group Geo = 4 { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo, com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.Builder, com.google.apphosting.api.search.DocumentPb.FieldValue.GeoOrBuilder>
getGeoFieldBuilder() {
if (geoBuilder_ == null) {
geoBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FieldValue.Geo, com.google.apphosting.api.search.DocumentPb.FieldValue.Geo.Builder, com.google.apphosting.api.search.DocumentPb.FieldValue.GeoOrBuilder>(
getGeo(),
getParentForChildren(),
isClean());
geo_ = null;
}
return geoBuilder_;
}
private com.google.protobuf.Internal.DoubleList vectorValue_ = emptyDoubleList();
private void ensureVectorValueIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
vectorValue_ = mutableCopy(vectorValue_);
bitField0_ |= 0x00000010;
}
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @return A list containing the vectorValue.
*/
public java.util.List
getVectorValueList() {
return ((bitField0_ & 0x00000010) != 0) ?
java.util.Collections.unmodifiableList(vectorValue_) : vectorValue_;
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @return The count of vectorValue.
*/
public int getVectorValueCount() {
return vectorValue_.size();
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @param index The index of the element to return.
* @return The vectorValue at the given index.
*/
public double getVectorValue(int index) {
return vectorValue_.getDouble(index);
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @param index The index to set the value at.
* @param value The vectorValue to set.
* @return This builder for chaining.
*/
public Builder setVectorValue(
int index, double value) {
ensureVectorValueIsMutable();
vectorValue_.setDouble(index, value);
onChanged();
return this;
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @param value The vectorValue to add.
* @return This builder for chaining.
*/
public Builder addVectorValue(double value) {
ensureVectorValueIsMutable();
vectorValue_.addDouble(value);
onChanged();
return this;
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @param values The vectorValue to add.
* @return This builder for chaining.
*/
public Builder addAllVectorValue(
java.lang.Iterable extends java.lang.Double> values) {
ensureVectorValueIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, vectorValue_);
onChanged();
return this;
}
/**
*
* The field in which VECTOR values are stored. Vector is defined as an
* ordered set of doubles.
*
*
* repeated double vector_value = 7;
* @return This builder for chaining.
*/
public Builder clearVectorValue() {
vectorValue_ = emptyDoubleList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.FieldValue)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.FieldValue)
private static final com.google.apphosting.api.search.DocumentPb.FieldValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.FieldValue();
}
public static com.google.apphosting.api.search.DocumentPb.FieldValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FieldValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FieldOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.Field)
com.google.protobuf.MessageOrBuilder {
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
* @return The value.
*/
com.google.apphosting.api.search.DocumentPb.FieldValue getValue();
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
com.google.apphosting.api.search.DocumentPb.FieldValueOrBuilder getValueOrBuilder();
}
/**
*
* A document field.
*
*
* Protobuf type {@code storage_onestore_v3.Field}
*/
public static final class Field extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.Field)
FieldOrBuilder {
private static final long serialVersionUID = 0L;
// Use Field.newBuilder() to construct.
private Field(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Field() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Field();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Field_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Field_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.Field.class, com.google.apphosting.api.search.DocumentPb.Field.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private com.google.apphosting.api.search.DocumentPb.FieldValue value_;
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
* @return The value.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue getValue() {
return value_ == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.getDefaultInstance() : value_;
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValueOrBuilder getValueOrBuilder() {
return value_ == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.getDefaultInstance() : value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
if (!getValue().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getValue());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.Field)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.Field other = (com.google.apphosting.api.search.DocumentPb.Field) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Field parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.Field prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A document field.
*
*
* Protobuf type {@code storage_onestore_v3.Field}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.Field)
com.google.apphosting.api.search.DocumentPb.FieldOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Field_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Field_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.Field.class, com.google.apphosting.api.search.DocumentPb.Field.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.Field.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
value_ = null;
if (valueBuilder_ != null) {
valueBuilder_.dispose();
valueBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Field_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Field getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.Field.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Field build() {
com.google.apphosting.api.search.DocumentPb.Field result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Field buildPartial() {
com.google.apphosting.api.search.DocumentPb.Field result = new com.google.apphosting.api.search.DocumentPb.Field(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.Field result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = valueBuilder_ == null
? value_
: valueBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.Field) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.Field)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.Field other) {
if (other == com.google.apphosting.api.search.DocumentPb.Field.getDefaultInstance()) return this;
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasValue()) {
return false;
}
if (!getValue().isInitialized()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getValueFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the field.
*
*
* required string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The name of the field.
*
*
* required string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The name of the field.
*
*
* required string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.apphosting.api.search.DocumentPb.FieldValue value_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FieldValue, com.google.apphosting.api.search.DocumentPb.FieldValue.Builder, com.google.apphosting.api.search.DocumentPb.FieldValueOrBuilder> valueBuilder_;
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
* @return The value.
*/
public com.google.apphosting.api.search.DocumentPb.FieldValue getValue() {
if (valueBuilder_ == null) {
return value_ == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.getDefaultInstance() : value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
public Builder setValue(com.google.apphosting.api.search.DocumentPb.FieldValue value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
} else {
valueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
public Builder setValue(
com.google.apphosting.api.search.DocumentPb.FieldValue.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
public Builder mergeValue(com.google.apphosting.api.search.DocumentPb.FieldValue value) {
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
value_ != null &&
value_ != com.google.apphosting.api.search.DocumentPb.FieldValue.getDefaultInstance()) {
getValueBuilder().mergeFrom(value);
} else {
value_ = value;
}
} else {
valueBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = null;
if (valueBuilder_ != null) {
valueBuilder_.dispose();
valueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
public com.google.apphosting.api.search.DocumentPb.FieldValue.Builder getValueBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
public com.google.apphosting.api.search.DocumentPb.FieldValueOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_ == null ?
com.google.apphosting.api.search.DocumentPb.FieldValue.getDefaultInstance() : value_;
}
}
/**
*
* The value of the field.
*
*
* required .storage_onestore_v3.FieldValue value = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FieldValue, com.google.apphosting.api.search.DocumentPb.FieldValue.Builder, com.google.apphosting.api.search.DocumentPb.FieldValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FieldValue, com.google.apphosting.api.search.DocumentPb.FieldValue.Builder, com.google.apphosting.api.search.DocumentPb.FieldValueOrBuilder>(
getValue(),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.Field)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.Field)
private static final com.google.apphosting.api.search.DocumentPb.Field DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.Field();
}
public static com.google.apphosting.api.search.DocumentPb.Field getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Field parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Field getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FieldTypesOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.FieldTypes)
com.google.protobuf.MessageOrBuilder {
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @return A list containing the type.
*/
java.util.List getTypeList();
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @return The count of type.
*/
int getTypeCount();
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @param index The index of the element to return.
* @return The type at the given index.
*/
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType getType(int index);
}
/**
*
* A mapping from a field name to known types associated with it.
*
*
* Protobuf type {@code storage_onestore_v3.FieldTypes}
*/
public static final class FieldTypes extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.FieldTypes)
FieldTypesOrBuilder {
private static final long serialVersionUID = 0L;
// Use FieldTypes.newBuilder() to construct.
private FieldTypes(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FieldTypes() {
name_ = "";
type_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FieldTypes();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldTypes_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldTypes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.FieldTypes.class, com.google.apphosting.api.search.DocumentPb.FieldTypes.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List type_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType> type_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType>() {
public com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType convert(java.lang.Integer from) {
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType result = com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.forNumber(from);
return result == null ? com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.TEXT : result;
}
};
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @return A list containing the type.
*/
@java.lang.Override
public java.util.List getTypeList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType>(type_, type_converter_);
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @return The count of type.
*/
@java.lang.Override
public int getTypeCount() {
return type_.size();
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @param index The index of the element to return.
* @return The type at the given index.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType getType(int index) {
return type_converter_.convert(type_.get(index));
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
for (int i = 0; i < type_.size(); i++) {
output.writeEnum(2, type_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
{
int dataSize = 0;
for (int i = 0; i < type_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(type_.get(i));
}
size += dataSize;
size += 1 * type_.size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.FieldTypes)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.FieldTypes other = (com.google.apphosting.api.search.DocumentPb.FieldTypes) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!type_.equals(other.type_)) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (getTypeCount() > 0) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_.hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.FieldTypes prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A mapping from a field name to known types associated with it.
*
*
* Protobuf type {@code storage_onestore_v3.FieldTypes}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.FieldTypes)
com.google.apphosting.api.search.DocumentPb.FieldTypesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldTypes_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldTypes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.FieldTypes.class, com.google.apphosting.api.search.DocumentPb.FieldTypes.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.FieldTypes.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
type_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FieldTypes_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldTypes getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.FieldTypes.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldTypes build() {
com.google.apphosting.api.search.DocumentPb.FieldTypes result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldTypes buildPartial() {
com.google.apphosting.api.search.DocumentPb.FieldTypes result = new com.google.apphosting.api.search.DocumentPb.FieldTypes(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.api.search.DocumentPb.FieldTypes result) {
if (((bitField0_ & 0x00000002) != 0)) {
type_ = java.util.Collections.unmodifiableList(type_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.type_ = type_;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.FieldTypes result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.FieldTypes) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.FieldTypes)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.FieldTypes other) {
if (other == com.google.apphosting.api.search.DocumentPb.FieldTypes.getDefaultInstance()) return this;
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.type_.isEmpty()) {
if (type_.isEmpty()) {
type_ = other.type_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureTypeIsMutable();
type_.addAll(other.type_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
int tmpRaw = input.readEnum();
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType tmpValue =
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(2, tmpRaw);
} else {
ensureTypeIsMutable();
type_.add(tmpRaw);
}
break;
} // case 16
case 18: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType tmpValue =
com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(2, tmpRaw);
} else {
ensureTypeIsMutable();
type_.add(tmpRaw);
}
}
input.popLimit(oldLimit);
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The user given field name.
*
*
* required string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The user given field name.
*
*
* required string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The user given field name.
*
*
* required string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.util.List type_ =
java.util.Collections.emptyList();
private void ensureTypeIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
type_ = new java.util.ArrayList(type_);
bitField0_ |= 0x00000002;
}
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @return A list containing the type.
*/
public java.util.List getTypeList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType>(type_, type_converter_);
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @return The count of type.
*/
public int getTypeCount() {
return type_.size();
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @param index The index of the element to return.
* @return The type at the given index.
*/
public com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType getType(int index) {
return type_converter_.convert(type_.get(index));
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @param index The index to set the value at.
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(
int index, com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType value) {
if (value == null) {
throw new NullPointerException();
}
ensureTypeIsMutable();
type_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @param value The type to add.
* @return This builder for chaining.
*/
public Builder addType(com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType value) {
if (value == null) {
throw new NullPointerException();
}
ensureTypeIsMutable();
type_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @param values The type to add.
* @return This builder for chaining.
*/
public Builder addAllType(
java.lang.Iterable extends com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType> values) {
ensureTypeIsMutable();
for (com.google.apphosting.api.search.DocumentPb.FieldValue.ContentType value : values) {
type_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* The types associated with the given field name.
*
*
* repeated .storage_onestore_v3.FieldValue.ContentType type = 2;
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.FieldTypes)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.FieldTypes)
private static final com.google.apphosting.api.search.DocumentPb.FieldTypes DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.FieldTypes();
}
public static com.google.apphosting.api.search.DocumentPb.FieldTypes getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FieldTypes parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldTypes getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IndexShardSettingsOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.IndexShardSettings)
com.google.protobuf.MessageOrBuilder {
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @return A list containing the prevNumShards.
*/
java.util.List getPrevNumShardsList();
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @return The count of prevNumShards.
*/
int getPrevNumShardsCount();
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @param index The index of the element to return.
* @return The prevNumShards at the given index.
*/
int getPrevNumShards(int index);
/**
*
* Current total number of shards for this index.
*
*
* required int32 num_shards = 2 [default = 1];
* @return Whether the numShards field is set.
*/
boolean hasNumShards();
/**
*
* Current total number of shards for this index.
*
*
* required int32 num_shards = 2 [default = 1];
* @return The numShards.
*/
int getNumShards();
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @return A list containing the prevNumShardsSearchFalse.
*/
java.util.List getPrevNumShardsSearchFalseList();
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @return The count of prevNumShardsSearchFalse.
*/
int getPrevNumShardsSearchFalseCount();
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @param index The index of the element to return.
* @return The prevNumShardsSearchFalse at the given index.
*/
int getPrevNumShardsSearchFalse(int index);
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return Whether the localReplica field is set.
*/
boolean hasLocalReplica();
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return The localReplica.
*/
java.lang.String getLocalReplica();
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return The bytes for localReplica.
*/
com.google.protobuf.ByteString
getLocalReplicaBytes();
}
/**
* Protobuf type {@code storage_onestore_v3.IndexShardSettings}
*/
public static final class IndexShardSettings extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.IndexShardSettings)
IndexShardSettingsOrBuilder {
private static final long serialVersionUID = 0L;
// Use IndexShardSettings.newBuilder() to construct.
private IndexShardSettings(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IndexShardSettings() {
prevNumShards_ = emptyIntList();
numShards_ = 1;
prevNumShardsSearchFalse_ = emptyIntList();
localReplica_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new IndexShardSettings();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexShardSettings_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexShardSettings_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.IndexShardSettings.class, com.google.apphosting.api.search.DocumentPb.IndexShardSettings.Builder.class);
}
private int bitField0_;
public static final int PREV_NUM_SHARDS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.IntList prevNumShards_;
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @return A list containing the prevNumShards.
*/
@java.lang.Override
public java.util.List
getPrevNumShardsList() {
return prevNumShards_;
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @return The count of prevNumShards.
*/
public int getPrevNumShardsCount() {
return prevNumShards_.size();
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @param index The index of the element to return.
* @return The prevNumShards at the given index.
*/
public int getPrevNumShards(int index) {
return prevNumShards_.getInt(index);
}
public static final int NUM_SHARDS_FIELD_NUMBER = 2;
private int numShards_ = 1;
/**
*
* Current total number of shards for this index.
*
*
* required int32 num_shards = 2 [default = 1];
* @return Whether the numShards field is set.
*/
@java.lang.Override
public boolean hasNumShards() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Current total number of shards for this index.
*
*
* required int32 num_shards = 2 [default = 1];
* @return The numShards.
*/
@java.lang.Override
public int getNumShards() {
return numShards_;
}
public static final int PREV_NUM_SHARDS_SEARCH_FALSE_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.IntList prevNumShardsSearchFalse_;
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @return A list containing the prevNumShardsSearchFalse.
*/
@java.lang.Override
public java.util.List
getPrevNumShardsSearchFalseList() {
return prevNumShardsSearchFalse_;
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @return The count of prevNumShardsSearchFalse.
*/
public int getPrevNumShardsSearchFalseCount() {
return prevNumShardsSearchFalse_.size();
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @param index The index of the element to return.
* @return The prevNumShardsSearchFalse at the given index.
*/
public int getPrevNumShardsSearchFalse(int index) {
return prevNumShardsSearchFalse_.getInt(index);
}
public static final int LOCAL_REPLICA_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object localReplica_ = "";
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return Whether the localReplica field is set.
*/
@java.lang.Override
public boolean hasLocalReplica() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return The localReplica.
*/
@java.lang.Override
public java.lang.String getLocalReplica() {
java.lang.Object ref = localReplica_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
localReplica_ = s;
}
return s;
}
}
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return The bytes for localReplica.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLocalReplicaBytes() {
java.lang.Object ref = localReplica_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
localReplica_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasNumShards()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < prevNumShards_.size(); i++) {
output.writeInt32(1, prevNumShards_.getInt(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(2, numShards_);
}
for (int i = 0; i < prevNumShardsSearchFalse_.size(); i++) {
output.writeInt32(3, prevNumShardsSearchFalse_.getInt(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, localReplica_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < prevNumShards_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(prevNumShards_.getInt(i));
}
size += dataSize;
size += 1 * getPrevNumShardsList().size();
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, numShards_);
}
{
int dataSize = 0;
for (int i = 0; i < prevNumShardsSearchFalse_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(prevNumShardsSearchFalse_.getInt(i));
}
size += dataSize;
size += 1 * getPrevNumShardsSearchFalseList().size();
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, localReplica_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.IndexShardSettings)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.IndexShardSettings other = (com.google.apphosting.api.search.DocumentPb.IndexShardSettings) obj;
if (!getPrevNumShardsList()
.equals(other.getPrevNumShardsList())) return false;
if (hasNumShards() != other.hasNumShards()) return false;
if (hasNumShards()) {
if (getNumShards()
!= other.getNumShards()) return false;
}
if (!getPrevNumShardsSearchFalseList()
.equals(other.getPrevNumShardsSearchFalseList())) return false;
if (hasLocalReplica() != other.hasLocalReplica()) return false;
if (hasLocalReplica()) {
if (!getLocalReplica()
.equals(other.getLocalReplica())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getPrevNumShardsCount() > 0) {
hash = (37 * hash) + PREV_NUM_SHARDS_FIELD_NUMBER;
hash = (53 * hash) + getPrevNumShardsList().hashCode();
}
if (hasNumShards()) {
hash = (37 * hash) + NUM_SHARDS_FIELD_NUMBER;
hash = (53 * hash) + getNumShards();
}
if (getPrevNumShardsSearchFalseCount() > 0) {
hash = (37 * hash) + PREV_NUM_SHARDS_SEARCH_FALSE_FIELD_NUMBER;
hash = (53 * hash) + getPrevNumShardsSearchFalseList().hashCode();
}
if (hasLocalReplica()) {
hash = (37 * hash) + LOCAL_REPLICA_FIELD_NUMBER;
hash = (53 * hash) + getLocalReplica().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.IndexShardSettings prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code storage_onestore_v3.IndexShardSettings}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.IndexShardSettings)
com.google.apphosting.api.search.DocumentPb.IndexShardSettingsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexShardSettings_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexShardSettings_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.IndexShardSettings.class, com.google.apphosting.api.search.DocumentPb.IndexShardSettings.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.IndexShardSettings.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
prevNumShards_ = emptyIntList();
numShards_ = 1;
prevNumShardsSearchFalse_ = emptyIntList();
localReplica_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexShardSettings_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexShardSettings getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.IndexShardSettings.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexShardSettings build() {
com.google.apphosting.api.search.DocumentPb.IndexShardSettings result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexShardSettings buildPartial() {
com.google.apphosting.api.search.DocumentPb.IndexShardSettings result = new com.google.apphosting.api.search.DocumentPb.IndexShardSettings(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.api.search.DocumentPb.IndexShardSettings result) {
if (((bitField0_ & 0x00000001) != 0)) {
prevNumShards_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.prevNumShards_ = prevNumShards_;
if (((bitField0_ & 0x00000004) != 0)) {
prevNumShardsSearchFalse_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.prevNumShardsSearchFalse_ = prevNumShardsSearchFalse_;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.IndexShardSettings result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.numShards_ = numShards_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.localReplica_ = localReplica_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.IndexShardSettings) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.IndexShardSettings)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.IndexShardSettings other) {
if (other == com.google.apphosting.api.search.DocumentPb.IndexShardSettings.getDefaultInstance()) return this;
if (!other.prevNumShards_.isEmpty()) {
if (prevNumShards_.isEmpty()) {
prevNumShards_ = other.prevNumShards_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensurePrevNumShardsIsMutable();
prevNumShards_.addAll(other.prevNumShards_);
}
onChanged();
}
if (other.hasNumShards()) {
setNumShards(other.getNumShards());
}
if (!other.prevNumShardsSearchFalse_.isEmpty()) {
if (prevNumShardsSearchFalse_.isEmpty()) {
prevNumShardsSearchFalse_ = other.prevNumShardsSearchFalse_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensurePrevNumShardsSearchFalseIsMutable();
prevNumShardsSearchFalse_.addAll(other.prevNumShardsSearchFalse_);
}
onChanged();
}
if (other.hasLocalReplica()) {
localReplica_ = other.localReplica_;
bitField0_ |= 0x00000008;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasNumShards()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int v = input.readInt32();
ensurePrevNumShardsIsMutable();
prevNumShards_.addInt(v);
break;
} // case 8
case 10: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensurePrevNumShardsIsMutable();
while (input.getBytesUntilLimit() > 0) {
prevNumShards_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
} // case 10
case 16: {
numShards_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
int v = input.readInt32();
ensurePrevNumShardsSearchFalseIsMutable();
prevNumShardsSearchFalse_.addInt(v);
break;
} // case 24
case 26: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
ensurePrevNumShardsSearchFalseIsMutable();
while (input.getBytesUntilLimit() > 0) {
prevNumShardsSearchFalse_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
} // case 26
case 34: {
localReplica_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.Internal.IntList prevNumShards_ = emptyIntList();
private void ensurePrevNumShardsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
prevNumShards_ = mutableCopy(prevNumShards_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @return A list containing the prevNumShards.
*/
public java.util.List
getPrevNumShardsList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(prevNumShards_) : prevNumShards_;
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @return The count of prevNumShards.
*/
public int getPrevNumShardsCount() {
return prevNumShards_.size();
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @param index The index of the element to return.
* @return The prevNumShards at the given index.
*/
public int getPrevNumShards(int index) {
return prevNumShards_.getInt(index);
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @param index The index to set the value at.
* @param value The prevNumShards to set.
* @return This builder for chaining.
*/
public Builder setPrevNumShards(
int index, int value) {
ensurePrevNumShardsIsMutable();
prevNumShards_.setInt(index, value);
onChanged();
return this;
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @param value The prevNumShards to add.
* @return This builder for chaining.
*/
public Builder addPrevNumShards(int value) {
ensurePrevNumShardsIsMutable();
prevNumShards_.addInt(value);
onChanged();
return this;
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @param values The prevNumShards to add.
* @return This builder for chaining.
*/
public Builder addAllPrevNumShards(
java.lang.Iterable extends java.lang.Integer> values) {
ensurePrevNumShardsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, prevNumShards_);
onChanged();
return this;
}
/**
*
* Total number of shards in the previous configuration.
*
*
* repeated int32 prev_num_shards = 1;
* @return This builder for chaining.
*/
public Builder clearPrevNumShards() {
prevNumShards_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private int numShards_ = 1;
/**
*
* Current total number of shards for this index.
*
*
* required int32 num_shards = 2 [default = 1];
* @return Whether the numShards field is set.
*/
@java.lang.Override
public boolean hasNumShards() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Current total number of shards for this index.
*
*
* required int32 num_shards = 2 [default = 1];
* @return The numShards.
*/
@java.lang.Override
public int getNumShards() {
return numShards_;
}
/**
*
* Current total number of shards for this index.
*
*
* required int32 num_shards = 2 [default = 1];
* @param value The numShards to set.
* @return This builder for chaining.
*/
public Builder setNumShards(int value) {
numShards_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Current total number of shards for this index.
*
*
* required int32 num_shards = 2 [default = 1];
* @return This builder for chaining.
*/
public Builder clearNumShards() {
bitField0_ = (bitField0_ & ~0x00000002);
numShards_ = 1;
onChanged();
return this;
}
private com.google.protobuf.Internal.IntList prevNumShardsSearchFalse_ = emptyIntList();
private void ensurePrevNumShardsSearchFalseIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
prevNumShardsSearchFalse_ = mutableCopy(prevNumShardsSearchFalse_);
bitField0_ |= 0x00000004;
}
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @return A list containing the prevNumShardsSearchFalse.
*/
public java.util.List
getPrevNumShardsSearchFalseList() {
return ((bitField0_ & 0x00000004) != 0) ?
java.util.Collections.unmodifiableList(prevNumShardsSearchFalse_) : prevNumShardsSearchFalse_;
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @return The count of prevNumShardsSearchFalse.
*/
public int getPrevNumShardsSearchFalseCount() {
return prevNumShardsSearchFalse_.size();
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @param index The index of the element to return.
* @return The prevNumShardsSearchFalse at the given index.
*/
public int getPrevNumShardsSearchFalse(int index) {
return prevNumShardsSearchFalse_.getInt(index);
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @param index The index to set the value at.
* @param value The prevNumShardsSearchFalse to set.
* @return This builder for chaining.
*/
public Builder setPrevNumShardsSearchFalse(
int index, int value) {
ensurePrevNumShardsSearchFalseIsMutable();
prevNumShardsSearchFalse_.setInt(index, value);
onChanged();
return this;
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @param value The prevNumShardsSearchFalse to add.
* @return This builder for chaining.
*/
public Builder addPrevNumShardsSearchFalse(int value) {
ensurePrevNumShardsSearchFalseIsMutable();
prevNumShardsSearchFalse_.addInt(value);
onChanged();
return this;
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @param values The prevNumShardsSearchFalse to add.
* @return This builder for chaining.
*/
public Builder addAllPrevNumShardsSearchFalse(
java.lang.Iterable extends java.lang.Integer> values) {
ensurePrevNumShardsSearchFalseIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, prevNumShardsSearchFalse_);
onChanged();
return this;
}
/**
*
* The set of prev_num_shards that are not searchable. This is a disjoint set
* relative to the prev_num_shards list.
*
*
* repeated int32 prev_num_shards_search_false = 3;
* @return This builder for chaining.
*/
public Builder clearPrevNumShardsSearchFalse() {
prevNumShardsSearchFalse_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
private java.lang.Object localReplica_ = "";
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return Whether the localReplica field is set.
*/
public boolean hasLocalReplica() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return The localReplica.
*/
public java.lang.String getLocalReplica() {
java.lang.Object ref = localReplica_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
localReplica_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return The bytes for localReplica.
*/
public com.google.protobuf.ByteString
getLocalReplicaBytes() {
java.lang.Object ref = localReplica_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
localReplica_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @param value The localReplica to set.
* @return This builder for chaining.
*/
public Builder setLocalReplica(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
localReplica_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @return This builder for chaining.
*/
public Builder clearLocalReplica() {
localReplica_ = getDefaultInstance().getLocalReplica();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* The local replica to use for searches
*
*
* optional string local_replica = 4 [default = ""];
* @param value The bytes for localReplica to set.
* @return This builder for chaining.
*/
public Builder setLocalReplicaBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
localReplica_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.IndexShardSettings)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.IndexShardSettings)
private static final com.google.apphosting.api.search.DocumentPb.IndexShardSettings DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.IndexShardSettings();
}
public static com.google.apphosting.api.search.DocumentPb.IndexShardSettings getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IndexShardSettings parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexShardSettings getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IndexMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.IndexMetadata)
com.google.protobuf.MessageOrBuilder {
/**
* optional bool is_over_field_number_threshold = 1 [default = false];
* @return Whether the isOverFieldNumberThreshold field is set.
*/
boolean hasIsOverFieldNumberThreshold();
/**
* optional bool is_over_field_number_threshold = 1 [default = false];
* @return The isOverFieldNumberThreshold.
*/
boolean getIsOverFieldNumberThreshold();
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
* @return Whether the indexShardSettings field is set.
*/
boolean hasIndexShardSettings();
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
* @return The indexShardSettings.
*/
com.google.apphosting.api.search.DocumentPb.IndexShardSettings getIndexShardSettings();
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
com.google.apphosting.api.search.DocumentPb.IndexShardSettingsOrBuilder getIndexShardSettingsOrBuilder();
/**
* optional .storage_onestore_v3.IndexMetadata.IndexState index_state = 3 [default = ACTIVE];
* @return Whether the indexState field is set.
*/
boolean hasIndexState();
/**
* optional .storage_onestore_v3.IndexMetadata.IndexState index_state = 3 [default = ACTIVE];
* @return The indexState.
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState getIndexState();
/**
*
* The scheduled deletion time, in milliseconds from 1970-01-01T00:00:00Z
* Present iff index_state is SOFT_DELETED or PURGING.
*
*
* optional int64 index_delete_time = 4;
* @return Whether the indexDeleteTime field is set.
*/
boolean hasIndexDeleteTime();
/**
*
* The scheduled deletion time, in milliseconds from 1970-01-01T00:00:00Z
* Present iff index_state is SOFT_DELETED or PURGING.
*
*
* optional int64 index_delete_time = 4;
* @return The indexDeleteTime.
*/
long getIndexDeleteTime();
/**
*
* The maximum size of the index (if quota override is present).
* Used to: recreate dynamic quota profiles and purge them during wipeout.
*
*
* optional int64 max_index_size_bytes = 5;
* @return Whether the maxIndexSizeBytes field is set.
*/
boolean hasMaxIndexSizeBytes();
/**
*
* The maximum size of the index (if quota override is present).
* Used to: recreate dynamic quota profiles and purge them during wipeout.
*
*
* optional int64 max_index_size_bytes = 5;
* @return The maxIndexSizeBytes.
*/
long getMaxIndexSizeBytes();
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
java.util.List
getReplicaDeletionList();
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails getReplicaDeletion(int index);
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
int getReplicaDeletionCount();
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
java.util.List extends com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder>
getReplicaDeletionOrBuilderList();
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder getReplicaDeletionOrBuilder(
int index);
}
/**
* Protobuf type {@code storage_onestore_v3.IndexMetadata}
*/
public static final class IndexMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.IndexMetadata)
IndexMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use IndexMetadata.newBuilder() to construct.
private IndexMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IndexMetadata() {
indexState_ = 0;
replicaDeletion_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new IndexMetadata();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.class, com.google.apphosting.api.search.DocumentPb.IndexMetadata.Builder.class);
}
/**
*
* The index state could be modified upon a user request or a scanner.
* When user issues a delete request, the state is set to SOFT_DELETED,
* while it is still possible for user to cancel that deletion.
* When the scanner finds out that index_delete_time has passed,
* or a Dexter instance finds out index_delete_time has passed upon a RPC,
* it sets the index's state to PURGING by writing to Megastore.
* User is now not able to cancel the deletion.
* When the scanner sees that all traces of the index are deleted,
* it removes the index metadata row.
* We transition between state as follows:
* Index
* +--------+ Created +---------+
* start----->| Non- |------------>| ACTIVE |
* |existent| | |
* Scanner +--------+ +---------+
* finds out ^ ^ |
* all deletes | User cancels | | User issues
* completed | index deletion | | index deletion
* | | v
* +--------+ +---------+
* | PURGE- |<------------| SOFT_ |
* | ING | Scanner/ | DELETED |
* +--------+ Dexter +---------+
* finds out
* index_delete_time
* passed
*
*
* Protobuf enum {@code storage_onestore_v3.IndexMetadata.IndexState}
*/
public enum IndexState
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Index is active.
*
*
* ACTIVE = 0;
*/
ACTIVE(0),
/**
*
* Index seen as marked deleted; purge trigger time not yet seen.
* The deletion could still be cancelled at this point.
*
*
* SOFT_DELETED = 1;
*/
SOFT_DELETED(1),
/**
*
* Purge trigger time seen, purge processes should run.
*
*
* PURGING = 2;
*/
PURGING(2),
;
/**
*
* Index is active.
*
*
* ACTIVE = 0;
*/
public static final int ACTIVE_VALUE = 0;
/**
*
* Index seen as marked deleted; purge trigger time not yet seen.
* The deletion could still be cancelled at this point.
*
*
* SOFT_DELETED = 1;
*/
public static final int SOFT_DELETED_VALUE = 1;
/**
*
* Purge trigger time seen, purge processes should run.
*
*
* PURGING = 2;
*/
public static final int PURGING_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static IndexState valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static IndexState forNumber(int value) {
switch (value) {
case 0: return ACTIVE;
case 1: return SOFT_DELETED;
case 2: return PURGING;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
IndexState> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public IndexState findValueByNumber(int number) {
return IndexState.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.IndexMetadata.getDescriptor().getEnumTypes().get(0);
}
private static final IndexState[] VALUES = values();
public static IndexState valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private IndexState(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:storage_onestore_v3.IndexMetadata.IndexState)
}
public interface DeletionStatusOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.IndexMetadata.DeletionStatus)
com.google.protobuf.MessageOrBuilder {
/**
* optional int64 started_time = 3;
* @return Whether the startedTime field is set.
*/
boolean hasStartedTime();
/**
* optional int64 started_time = 3;
* @return The startedTime.
*/
long getStartedTime();
/**
* optional int64 completed_time = 4;
* @return Whether the completedTime field is set.
*/
boolean hasCompletedTime();
/**
* optional int64 completed_time = 4;
* @return The completedTime.
*/
long getCompletedTime();
}
/**
* Protobuf type {@code storage_onestore_v3.IndexMetadata.DeletionStatus}
*/
public static final class DeletionStatus extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.IndexMetadata.DeletionStatus)
DeletionStatusOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeletionStatus.newBuilder() to construct.
private DeletionStatus(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeletionStatus() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DeletionStatus();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.class, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder.class);
}
private int bitField0_;
public static final int STARTED_TIME_FIELD_NUMBER = 3;
private long startedTime_ = 0L;
/**
* optional int64 started_time = 3;
* @return Whether the startedTime field is set.
*/
@java.lang.Override
public boolean hasStartedTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 started_time = 3;
* @return The startedTime.
*/
@java.lang.Override
public long getStartedTime() {
return startedTime_;
}
public static final int COMPLETED_TIME_FIELD_NUMBER = 4;
private long completedTime_ = 0L;
/**
* optional int64 completed_time = 4;
* @return Whether the completedTime field is set.
*/
@java.lang.Override
public boolean hasCompletedTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 completed_time = 4;
* @return The completedTime.
*/
@java.lang.Override
public long getCompletedTime() {
return completedTime_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(3, startedTime_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(4, completedTime_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, startedTime_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, completedTime_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus other = (com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus) obj;
if (hasStartedTime() != other.hasStartedTime()) return false;
if (hasStartedTime()) {
if (getStartedTime()
!= other.getStartedTime()) return false;
}
if (hasCompletedTime() != other.hasCompletedTime()) return false;
if (hasCompletedTime()) {
if (getCompletedTime()
!= other.getCompletedTime()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasStartedTime()) {
hash = (37 * hash) + STARTED_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getStartedTime());
}
if (hasCompletedTime()) {
hash = (37 * hash) + COMPLETED_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCompletedTime());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code storage_onestore_v3.IndexMetadata.DeletionStatus}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.IndexMetadata.DeletionStatus)
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.class, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
startedTime_ = 0L;
completedTime_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus build() {
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus buildPartial() {
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus result = new com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.startedTime_ = startedTime_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.completedTime_ = completedTime_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus other) {
if (other == com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance()) return this;
if (other.hasStartedTime()) {
setStartedTime(other.getStartedTime());
}
if (other.hasCompletedTime()) {
setCompletedTime(other.getCompletedTime());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 24: {
startedTime_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 24
case 32: {
completedTime_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 32
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long startedTime_ ;
/**
* optional int64 started_time = 3;
* @return Whether the startedTime field is set.
*/
@java.lang.Override
public boolean hasStartedTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional int64 started_time = 3;
* @return The startedTime.
*/
@java.lang.Override
public long getStartedTime() {
return startedTime_;
}
/**
* optional int64 started_time = 3;
* @param value The startedTime to set.
* @return This builder for chaining.
*/
public Builder setStartedTime(long value) {
startedTime_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional int64 started_time = 3;
* @return This builder for chaining.
*/
public Builder clearStartedTime() {
bitField0_ = (bitField0_ & ~0x00000001);
startedTime_ = 0L;
onChanged();
return this;
}
private long completedTime_ ;
/**
* optional int64 completed_time = 4;
* @return Whether the completedTime field is set.
*/
@java.lang.Override
public boolean hasCompletedTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional int64 completed_time = 4;
* @return The completedTime.
*/
@java.lang.Override
public long getCompletedTime() {
return completedTime_;
}
/**
* optional int64 completed_time = 4;
* @param value The completedTime to set.
* @return This builder for chaining.
*/
public Builder setCompletedTime(long value) {
completedTime_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional int64 completed_time = 4;
* @return This builder for chaining.
*/
public Builder clearCompletedTime() {
bitField0_ = (bitField0_ & ~0x00000002);
completedTime_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.IndexMetadata.DeletionStatus)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.IndexMetadata.DeletionStatus)
private static final com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus();
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DeletionStatus parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IndexDeletionDetailsOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.IndexMetadata.IndexDeletionDetails)
com.google.protobuf.MessageOrBuilder {
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return Whether the replicaName field is set.
*/
boolean hasReplicaName();
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return The replicaName.
*/
java.lang.String getReplicaName();
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return The bytes for replicaName.
*/
com.google.protobuf.ByteString
getReplicaNameBytes();
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
* @return Whether the precheck field is set.
*/
boolean hasPrecheck();
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
* @return The precheck.
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getPrecheck();
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getPrecheckOrBuilder();
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
* @return Whether the stBti field is set.
*/
boolean hasStBti();
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
* @return The stBti.
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getStBti();
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getStBtiOrBuilder();
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
* @return Whether the msDocs field is set.
*/
boolean hasMsDocs();
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
* @return The msDocs.
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getMsDocs();
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getMsDocsOrBuilder();
}
/**
*
* Tracks the status of each component that is part of the index deletion
* process. Each details represents the status of one replica.
*
*
* Protobuf type {@code storage_onestore_v3.IndexMetadata.IndexDeletionDetails}
*/
public static final class IndexDeletionDetails extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.IndexMetadata.IndexDeletionDetails)
IndexDeletionDetailsOrBuilder {
private static final long serialVersionUID = 0L;
// Use IndexDeletionDetails.newBuilder() to construct.
private IndexDeletionDetails(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IndexDeletionDetails() {
replicaName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new IndexDeletionDetails();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.class, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder.class);
}
private int bitField0_;
public static final int REPLICA_NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object replicaName_ = "";
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return Whether the replicaName field is set.
*/
@java.lang.Override
public boolean hasReplicaName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return The replicaName.
*/
@java.lang.Override
public java.lang.String getReplicaName() {
java.lang.Object ref = replicaName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
replicaName_ = s;
}
return s;
}
}
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return The bytes for replicaName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getReplicaNameBytes() {
java.lang.Object ref = replicaName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
replicaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRECHECK_FIELD_NUMBER = 2;
private com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus precheck_;
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
* @return Whether the precheck field is set.
*/
@java.lang.Override
public boolean hasPrecheck() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
* @return The precheck.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getPrecheck() {
return precheck_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : precheck_;
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getPrecheckOrBuilder() {
return precheck_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : precheck_;
}
public static final int ST_BTI_FIELD_NUMBER = 3;
private com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus stBti_;
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
* @return Whether the stBti field is set.
*/
@java.lang.Override
public boolean hasStBti() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
* @return The stBti.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getStBti() {
return stBti_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : stBti_;
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getStBtiOrBuilder() {
return stBti_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : stBti_;
}
public static final int MS_DOCS_FIELD_NUMBER = 4;
private com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus msDocs_;
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
* @return Whether the msDocs field is set.
*/
@java.lang.Override
public boolean hasMsDocs() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
* @return The msDocs.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getMsDocs() {
return msDocs_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : msDocs_;
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getMsDocsOrBuilder() {
return msDocs_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : msDocs_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasReplicaName()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, replicaName_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getPrecheck());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(3, getStBti());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(4, getMsDocs());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, replicaName_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPrecheck());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getStBti());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getMsDocs());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails other = (com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails) obj;
if (hasReplicaName() != other.hasReplicaName()) return false;
if (hasReplicaName()) {
if (!getReplicaName()
.equals(other.getReplicaName())) return false;
}
if (hasPrecheck() != other.hasPrecheck()) return false;
if (hasPrecheck()) {
if (!getPrecheck()
.equals(other.getPrecheck())) return false;
}
if (hasStBti() != other.hasStBti()) return false;
if (hasStBti()) {
if (!getStBti()
.equals(other.getStBti())) return false;
}
if (hasMsDocs() != other.hasMsDocs()) return false;
if (hasMsDocs()) {
if (!getMsDocs()
.equals(other.getMsDocs())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasReplicaName()) {
hash = (37 * hash) + REPLICA_NAME_FIELD_NUMBER;
hash = (53 * hash) + getReplicaName().hashCode();
}
if (hasPrecheck()) {
hash = (37 * hash) + PRECHECK_FIELD_NUMBER;
hash = (53 * hash) + getPrecheck().hashCode();
}
if (hasStBti()) {
hash = (37 * hash) + ST_BTI_FIELD_NUMBER;
hash = (53 * hash) + getStBti().hashCode();
}
if (hasMsDocs()) {
hash = (37 * hash) + MS_DOCS_FIELD_NUMBER;
hash = (53 * hash) + getMsDocs().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Tracks the status of each component that is part of the index deletion
* process. Each details represents the status of one replica.
*
*
* Protobuf type {@code storage_onestore_v3.IndexMetadata.IndexDeletionDetails}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.IndexMetadata.IndexDeletionDetails)
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.class, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPrecheckFieldBuilder();
getStBtiFieldBuilder();
getMsDocsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
replicaName_ = "";
precheck_ = null;
if (precheckBuilder_ != null) {
precheckBuilder_.dispose();
precheckBuilder_ = null;
}
stBti_ = null;
if (stBtiBuilder_ != null) {
stBtiBuilder_.dispose();
stBtiBuilder_ = null;
}
msDocs_ = null;
if (msDocsBuilder_ != null) {
msDocsBuilder_.dispose();
msDocsBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails build() {
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails buildPartial() {
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails result = new com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.replicaName_ = replicaName_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.precheck_ = precheckBuilder_ == null
? precheck_
: precheckBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.stBti_ = stBtiBuilder_ == null
? stBti_
: stBtiBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.msDocs_ = msDocsBuilder_ == null
? msDocs_
: msDocsBuilder_.build();
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails other) {
if (other == com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.getDefaultInstance()) return this;
if (other.hasReplicaName()) {
replicaName_ = other.replicaName_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasPrecheck()) {
mergePrecheck(other.getPrecheck());
}
if (other.hasStBti()) {
mergeStBti(other.getStBti());
}
if (other.hasMsDocs()) {
mergeMsDocs(other.getMsDocs());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasReplicaName()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
replicaName_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getPrecheckFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getStBtiFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getMsDocsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object replicaName_ = "";
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return Whether the replicaName field is set.
*/
public boolean hasReplicaName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return The replicaName.
*/
public java.lang.String getReplicaName() {
java.lang.Object ref = replicaName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
replicaName_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return The bytes for replicaName.
*/
public com.google.protobuf.ByteString
getReplicaNameBytes() {
java.lang.Object ref = replicaName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
replicaName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @param value The replicaName to set.
* @return This builder for chaining.
*/
public Builder setReplicaName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
replicaName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @return This builder for chaining.
*/
public Builder clearReplicaName() {
replicaName_ = getDefaultInstance().getReplicaName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Which replica these details describe
*
*
* required string replica_name = 1;
* @param value The bytes for replicaName to set.
* @return This builder for chaining.
*/
public Builder setReplicaNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
replicaName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus precheck_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder> precheckBuilder_;
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
* @return Whether the precheck field is set.
*/
public boolean hasPrecheck() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
* @return The precheck.
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getPrecheck() {
if (precheckBuilder_ == null) {
return precheck_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : precheck_;
} else {
return precheckBuilder_.getMessage();
}
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
public Builder setPrecheck(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus value) {
if (precheckBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
precheck_ = value;
} else {
precheckBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
public Builder setPrecheck(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder builderForValue) {
if (precheckBuilder_ == null) {
precheck_ = builderForValue.build();
} else {
precheckBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
public Builder mergePrecheck(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus value) {
if (precheckBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
precheck_ != null &&
precheck_ != com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance()) {
getPrecheckBuilder().mergeFrom(value);
} else {
precheck_ = value;
}
} else {
precheckBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
public Builder clearPrecheck() {
bitField0_ = (bitField0_ & ~0x00000002);
precheck_ = null;
if (precheckBuilder_ != null) {
precheckBuilder_.dispose();
precheckBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder getPrecheckBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getPrecheckFieldBuilder().getBuilder();
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getPrecheckOrBuilder() {
if (precheckBuilder_ != null) {
return precheckBuilder_.getMessageOrBuilder();
} else {
return precheck_ == null ?
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : precheck_;
}
}
/**
*
* Track if all Megastore writes have been applied
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus precheck = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder>
getPrecheckFieldBuilder() {
if (precheckBuilder_ == null) {
precheckBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder>(
getPrecheck(),
getParentForChildren(),
isClean());
precheck_ = null;
}
return precheckBuilder_;
}
private com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus stBti_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder> stBtiBuilder_;
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
* @return Whether the stBti field is set.
*/
public boolean hasStBti() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
* @return The stBti.
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getStBti() {
if (stBtiBuilder_ == null) {
return stBti_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : stBti_;
} else {
return stBtiBuilder_.getMessage();
}
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
public Builder setStBti(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus value) {
if (stBtiBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stBti_ = value;
} else {
stBtiBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
public Builder setStBti(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder builderForValue) {
if (stBtiBuilder_ == null) {
stBti_ = builderForValue.build();
} else {
stBtiBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
public Builder mergeStBti(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus value) {
if (stBtiBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
stBti_ != null &&
stBti_ != com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance()) {
getStBtiBuilder().mergeFrom(value);
} else {
stBti_ = value;
}
} else {
stBtiBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
public Builder clearStBti() {
bitField0_ = (bitField0_ & ~0x00000004);
stBti_ = null;
if (stBtiBuilder_ != null) {
stBtiBuilder_.dispose();
stBtiBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder getStBtiBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getStBtiFieldBuilder().getBuilder();
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getStBtiOrBuilder() {
if (stBtiBuilder_ != null) {
return stBtiBuilder_.getMessageOrBuilder();
} else {
return stBti_ == null ?
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : stBti_;
}
}
/**
*
* Tracks ST-BTI data deletion
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus st_bti = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder>
getStBtiFieldBuilder() {
if (stBtiBuilder_ == null) {
stBtiBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder>(
getStBti(),
getParentForChildren(),
isClean());
stBti_ = null;
}
return stBtiBuilder_;
}
private com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus msDocs_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder> msDocsBuilder_;
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
* @return Whether the msDocs field is set.
*/
public boolean hasMsDocs() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
* @return The msDocs.
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus getMsDocs() {
if (msDocsBuilder_ == null) {
return msDocs_ == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : msDocs_;
} else {
return msDocsBuilder_.getMessage();
}
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
public Builder setMsDocs(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus value) {
if (msDocsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
msDocs_ = value;
} else {
msDocsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
public Builder setMsDocs(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder builderForValue) {
if (msDocsBuilder_ == null) {
msDocs_ = builderForValue.build();
} else {
msDocsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
public Builder mergeMsDocs(com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus value) {
if (msDocsBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
msDocs_ != null &&
msDocs_ != com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance()) {
getMsDocsBuilder().mergeFrom(value);
} else {
msDocs_ = value;
}
} else {
msDocsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
public Builder clearMsDocs() {
bitField0_ = (bitField0_ & ~0x00000008);
msDocs_ = null;
if (msDocsBuilder_ != null) {
msDocsBuilder_.dispose();
msDocsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder getMsDocsBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getMsDocsFieldBuilder().getBuilder();
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder getMsDocsOrBuilder() {
if (msDocsBuilder_ != null) {
return msDocsBuilder_.getMessageOrBuilder();
} else {
return msDocs_ == null ?
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.getDefaultInstance() : msDocs_;
}
}
/**
*
* Tracks deletion from the Megastore CustomerDocument table
*
*
* optional .storage_onestore_v3.IndexMetadata.DeletionStatus ms_docs = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder>
getMsDocsFieldBuilder() {
if (msDocsBuilder_ == null) {
msDocsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatus.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.DeletionStatusOrBuilder>(
getMsDocs(),
getParentForChildren(),
isClean());
msDocs_ = null;
}
return msDocsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.IndexMetadata.IndexDeletionDetails)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.IndexMetadata.IndexDeletionDetails)
private static final com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails();
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IndexDeletionDetails parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int IS_OVER_FIELD_NUMBER_THRESHOLD_FIELD_NUMBER = 1;
private boolean isOverFieldNumberThreshold_ = false;
/**
* optional bool is_over_field_number_threshold = 1 [default = false];
* @return Whether the isOverFieldNumberThreshold field is set.
*/
@java.lang.Override
public boolean hasIsOverFieldNumberThreshold() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bool is_over_field_number_threshold = 1 [default = false];
* @return The isOverFieldNumberThreshold.
*/
@java.lang.Override
public boolean getIsOverFieldNumberThreshold() {
return isOverFieldNumberThreshold_;
}
public static final int INDEX_SHARD_SETTINGS_FIELD_NUMBER = 2;
private com.google.apphosting.api.search.DocumentPb.IndexShardSettings indexShardSettings_;
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
* @return Whether the indexShardSettings field is set.
*/
@java.lang.Override
public boolean hasIndexShardSettings() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
* @return The indexShardSettings.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexShardSettings getIndexShardSettings() {
return indexShardSettings_ == null ? com.google.apphosting.api.search.DocumentPb.IndexShardSettings.getDefaultInstance() : indexShardSettings_;
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexShardSettingsOrBuilder getIndexShardSettingsOrBuilder() {
return indexShardSettings_ == null ? com.google.apphosting.api.search.DocumentPb.IndexShardSettings.getDefaultInstance() : indexShardSettings_;
}
public static final int INDEX_STATE_FIELD_NUMBER = 3;
private int indexState_ = 0;
/**
* optional .storage_onestore_v3.IndexMetadata.IndexState index_state = 3 [default = ACTIVE];
* @return Whether the indexState field is set.
*/
@java.lang.Override public boolean hasIndexState() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .storage_onestore_v3.IndexMetadata.IndexState index_state = 3 [default = ACTIVE];
* @return The indexState.
*/
@java.lang.Override public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState getIndexState() {
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState result = com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState.forNumber(indexState_);
return result == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState.ACTIVE : result;
}
public static final int INDEX_DELETE_TIME_FIELD_NUMBER = 4;
private long indexDeleteTime_ = 0L;
/**
*
* The scheduled deletion time, in milliseconds from 1970-01-01T00:00:00Z
* Present iff index_state is SOFT_DELETED or PURGING.
*
*
* optional int64 index_delete_time = 4;
* @return Whether the indexDeleteTime field is set.
*/
@java.lang.Override
public boolean hasIndexDeleteTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The scheduled deletion time, in milliseconds from 1970-01-01T00:00:00Z
* Present iff index_state is SOFT_DELETED or PURGING.
*
*
* optional int64 index_delete_time = 4;
* @return The indexDeleteTime.
*/
@java.lang.Override
public long getIndexDeleteTime() {
return indexDeleteTime_;
}
public static final int MAX_INDEX_SIZE_BYTES_FIELD_NUMBER = 5;
private long maxIndexSizeBytes_ = 0L;
/**
*
* The maximum size of the index (if quota override is present).
* Used to: recreate dynamic quota profiles and purge them during wipeout.
*
*
* optional int64 max_index_size_bytes = 5;
* @return Whether the maxIndexSizeBytes field is set.
*/
@java.lang.Override
public boolean hasMaxIndexSizeBytes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The maximum size of the index (if quota override is present).
* Used to: recreate dynamic quota profiles and purge them during wipeout.
*
*
* optional int64 max_index_size_bytes = 5;
* @return The maxIndexSizeBytes.
*/
@java.lang.Override
public long getMaxIndexSizeBytes() {
return maxIndexSizeBytes_;
}
public static final int REPLICA_DELETION_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List replicaDeletion_;
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
@java.lang.Override
public java.util.List getReplicaDeletionList() {
return replicaDeletion_;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder>
getReplicaDeletionOrBuilderList() {
return replicaDeletion_;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
@java.lang.Override
public int getReplicaDeletionCount() {
return replicaDeletion_.size();
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails getReplicaDeletion(int index) {
return replicaDeletion_.get(index);
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder getReplicaDeletionOrBuilder(
int index) {
return replicaDeletion_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasIndexShardSettings()) {
if (!getIndexShardSettings().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getReplicaDeletionCount(); i++) {
if (!getReplicaDeletion(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBool(1, isOverFieldNumberThreshold_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getIndexShardSettings());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeEnum(3, indexState_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt64(4, indexDeleteTime_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt64(5, maxIndexSizeBytes_);
}
for (int i = 0; i < replicaDeletion_.size(); i++) {
output.writeMessage(6, replicaDeletion_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, isOverFieldNumberThreshold_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getIndexShardSettings());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, indexState_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, indexDeleteTime_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, maxIndexSizeBytes_);
}
for (int i = 0; i < replicaDeletion_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, replicaDeletion_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.IndexMetadata)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.IndexMetadata other = (com.google.apphosting.api.search.DocumentPb.IndexMetadata) obj;
if (hasIsOverFieldNumberThreshold() != other.hasIsOverFieldNumberThreshold()) return false;
if (hasIsOverFieldNumberThreshold()) {
if (getIsOverFieldNumberThreshold()
!= other.getIsOverFieldNumberThreshold()) return false;
}
if (hasIndexShardSettings() != other.hasIndexShardSettings()) return false;
if (hasIndexShardSettings()) {
if (!getIndexShardSettings()
.equals(other.getIndexShardSettings())) return false;
}
if (hasIndexState() != other.hasIndexState()) return false;
if (hasIndexState()) {
if (indexState_ != other.indexState_) return false;
}
if (hasIndexDeleteTime() != other.hasIndexDeleteTime()) return false;
if (hasIndexDeleteTime()) {
if (getIndexDeleteTime()
!= other.getIndexDeleteTime()) return false;
}
if (hasMaxIndexSizeBytes() != other.hasMaxIndexSizeBytes()) return false;
if (hasMaxIndexSizeBytes()) {
if (getMaxIndexSizeBytes()
!= other.getMaxIndexSizeBytes()) return false;
}
if (!getReplicaDeletionList()
.equals(other.getReplicaDeletionList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasIsOverFieldNumberThreshold()) {
hash = (37 * hash) + IS_OVER_FIELD_NUMBER_THRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsOverFieldNumberThreshold());
}
if (hasIndexShardSettings()) {
hash = (37 * hash) + INDEX_SHARD_SETTINGS_FIELD_NUMBER;
hash = (53 * hash) + getIndexShardSettings().hashCode();
}
if (hasIndexState()) {
hash = (37 * hash) + INDEX_STATE_FIELD_NUMBER;
hash = (53 * hash) + indexState_;
}
if (hasIndexDeleteTime()) {
hash = (37 * hash) + INDEX_DELETE_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getIndexDeleteTime());
}
if (hasMaxIndexSizeBytes()) {
hash = (37 * hash) + MAX_INDEX_SIZE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMaxIndexSizeBytes());
}
if (getReplicaDeletionCount() > 0) {
hash = (37 * hash) + REPLICA_DELETION_FIELD_NUMBER;
hash = (53 * hash) + getReplicaDeletionList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.IndexMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code storage_onestore_v3.IndexMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.IndexMetadata)
com.google.apphosting.api.search.DocumentPb.IndexMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.class, com.google.apphosting.api.search.DocumentPb.IndexMetadata.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.IndexMetadata.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getIndexShardSettingsFieldBuilder();
getReplicaDeletionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
isOverFieldNumberThreshold_ = false;
indexShardSettings_ = null;
if (indexShardSettingsBuilder_ != null) {
indexShardSettingsBuilder_.dispose();
indexShardSettingsBuilder_ = null;
}
indexState_ = 0;
indexDeleteTime_ = 0L;
maxIndexSizeBytes_ = 0L;
if (replicaDeletionBuilder_ == null) {
replicaDeletion_ = java.util.Collections.emptyList();
} else {
replicaDeletion_ = null;
replicaDeletionBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_IndexMetadata_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.IndexMetadata.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata build() {
com.google.apphosting.api.search.DocumentPb.IndexMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata buildPartial() {
com.google.apphosting.api.search.DocumentPb.IndexMetadata result = new com.google.apphosting.api.search.DocumentPb.IndexMetadata(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.api.search.DocumentPb.IndexMetadata result) {
if (replicaDeletionBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
replicaDeletion_ = java.util.Collections.unmodifiableList(replicaDeletion_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.replicaDeletion_ = replicaDeletion_;
} else {
result.replicaDeletion_ = replicaDeletionBuilder_.build();
}
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.IndexMetadata result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.isOverFieldNumberThreshold_ = isOverFieldNumberThreshold_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.indexShardSettings_ = indexShardSettingsBuilder_ == null
? indexShardSettings_
: indexShardSettingsBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.indexState_ = indexState_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.indexDeleteTime_ = indexDeleteTime_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.maxIndexSizeBytes_ = maxIndexSizeBytes_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.IndexMetadata) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.IndexMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.IndexMetadata other) {
if (other == com.google.apphosting.api.search.DocumentPb.IndexMetadata.getDefaultInstance()) return this;
if (other.hasIsOverFieldNumberThreshold()) {
setIsOverFieldNumberThreshold(other.getIsOverFieldNumberThreshold());
}
if (other.hasIndexShardSettings()) {
mergeIndexShardSettings(other.getIndexShardSettings());
}
if (other.hasIndexState()) {
setIndexState(other.getIndexState());
}
if (other.hasIndexDeleteTime()) {
setIndexDeleteTime(other.getIndexDeleteTime());
}
if (other.hasMaxIndexSizeBytes()) {
setMaxIndexSizeBytes(other.getMaxIndexSizeBytes());
}
if (replicaDeletionBuilder_ == null) {
if (!other.replicaDeletion_.isEmpty()) {
if (replicaDeletion_.isEmpty()) {
replicaDeletion_ = other.replicaDeletion_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureReplicaDeletionIsMutable();
replicaDeletion_.addAll(other.replicaDeletion_);
}
onChanged();
}
} else {
if (!other.replicaDeletion_.isEmpty()) {
if (replicaDeletionBuilder_.isEmpty()) {
replicaDeletionBuilder_.dispose();
replicaDeletionBuilder_ = null;
replicaDeletion_ = other.replicaDeletion_;
bitField0_ = (bitField0_ & ~0x00000020);
replicaDeletionBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getReplicaDeletionFieldBuilder() : null;
} else {
replicaDeletionBuilder_.addAllMessages(other.replicaDeletion_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasIndexShardSettings()) {
if (!getIndexShardSettings().isInitialized()) {
return false;
}
}
for (int i = 0; i < getReplicaDeletionCount(); i++) {
if (!getReplicaDeletion(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
isOverFieldNumberThreshold_ = input.readBool();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
input.readMessage(
getIndexShardSettingsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
int tmpRaw = input.readEnum();
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState tmpValue =
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(3, tmpRaw);
} else {
indexState_ = tmpRaw;
bitField0_ |= 0x00000004;
}
break;
} // case 24
case 32: {
indexDeleteTime_ = input.readInt64();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
maxIndexSizeBytes_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 40
case 50: {
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails m =
input.readMessage(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.PARSER,
extensionRegistry);
if (replicaDeletionBuilder_ == null) {
ensureReplicaDeletionIsMutable();
replicaDeletion_.add(m);
} else {
replicaDeletionBuilder_.addMessage(m);
}
break;
} // case 50
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private boolean isOverFieldNumberThreshold_ ;
/**
* optional bool is_over_field_number_threshold = 1 [default = false];
* @return Whether the isOverFieldNumberThreshold field is set.
*/
@java.lang.Override
public boolean hasIsOverFieldNumberThreshold() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional bool is_over_field_number_threshold = 1 [default = false];
* @return The isOverFieldNumberThreshold.
*/
@java.lang.Override
public boolean getIsOverFieldNumberThreshold() {
return isOverFieldNumberThreshold_;
}
/**
* optional bool is_over_field_number_threshold = 1 [default = false];
* @param value The isOverFieldNumberThreshold to set.
* @return This builder for chaining.
*/
public Builder setIsOverFieldNumberThreshold(boolean value) {
isOverFieldNumberThreshold_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional bool is_over_field_number_threshold = 1 [default = false];
* @return This builder for chaining.
*/
public Builder clearIsOverFieldNumberThreshold() {
bitField0_ = (bitField0_ & ~0x00000001);
isOverFieldNumberThreshold_ = false;
onChanged();
return this;
}
private com.google.apphosting.api.search.DocumentPb.IndexShardSettings indexShardSettings_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexShardSettings, com.google.apphosting.api.search.DocumentPb.IndexShardSettings.Builder, com.google.apphosting.api.search.DocumentPb.IndexShardSettingsOrBuilder> indexShardSettingsBuilder_;
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
* @return Whether the indexShardSettings field is set.
*/
public boolean hasIndexShardSettings() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
* @return The indexShardSettings.
*/
public com.google.apphosting.api.search.DocumentPb.IndexShardSettings getIndexShardSettings() {
if (indexShardSettingsBuilder_ == null) {
return indexShardSettings_ == null ? com.google.apphosting.api.search.DocumentPb.IndexShardSettings.getDefaultInstance() : indexShardSettings_;
} else {
return indexShardSettingsBuilder_.getMessage();
}
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
public Builder setIndexShardSettings(com.google.apphosting.api.search.DocumentPb.IndexShardSettings value) {
if (indexShardSettingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
indexShardSettings_ = value;
} else {
indexShardSettingsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
public Builder setIndexShardSettings(
com.google.apphosting.api.search.DocumentPb.IndexShardSettings.Builder builderForValue) {
if (indexShardSettingsBuilder_ == null) {
indexShardSettings_ = builderForValue.build();
} else {
indexShardSettingsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
public Builder mergeIndexShardSettings(com.google.apphosting.api.search.DocumentPb.IndexShardSettings value) {
if (indexShardSettingsBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
indexShardSettings_ != null &&
indexShardSettings_ != com.google.apphosting.api.search.DocumentPb.IndexShardSettings.getDefaultInstance()) {
getIndexShardSettingsBuilder().mergeFrom(value);
} else {
indexShardSettings_ = value;
}
} else {
indexShardSettingsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
public Builder clearIndexShardSettings() {
bitField0_ = (bitField0_ & ~0x00000002);
indexShardSettings_ = null;
if (indexShardSettingsBuilder_ != null) {
indexShardSettingsBuilder_.dispose();
indexShardSettingsBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
public com.google.apphosting.api.search.DocumentPb.IndexShardSettings.Builder getIndexShardSettingsBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getIndexShardSettingsFieldBuilder().getBuilder();
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
public com.google.apphosting.api.search.DocumentPb.IndexShardSettingsOrBuilder getIndexShardSettingsOrBuilder() {
if (indexShardSettingsBuilder_ != null) {
return indexShardSettingsBuilder_.getMessageOrBuilder();
} else {
return indexShardSettings_ == null ?
com.google.apphosting.api.search.DocumentPb.IndexShardSettings.getDefaultInstance() : indexShardSettings_;
}
}
/**
* optional .storage_onestore_v3.IndexShardSettings index_shard_settings = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexShardSettings, com.google.apphosting.api.search.DocumentPb.IndexShardSettings.Builder, com.google.apphosting.api.search.DocumentPb.IndexShardSettingsOrBuilder>
getIndexShardSettingsFieldBuilder() {
if (indexShardSettingsBuilder_ == null) {
indexShardSettingsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexShardSettings, com.google.apphosting.api.search.DocumentPb.IndexShardSettings.Builder, com.google.apphosting.api.search.DocumentPb.IndexShardSettingsOrBuilder>(
getIndexShardSettings(),
getParentForChildren(),
isClean());
indexShardSettings_ = null;
}
return indexShardSettingsBuilder_;
}
private int indexState_ = 0;
/**
* optional .storage_onestore_v3.IndexMetadata.IndexState index_state = 3 [default = ACTIVE];
* @return Whether the indexState field is set.
*/
@java.lang.Override public boolean hasIndexState() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
* optional .storage_onestore_v3.IndexMetadata.IndexState index_state = 3 [default = ACTIVE];
* @return The indexState.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState getIndexState() {
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState result = com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState.forNumber(indexState_);
return result == null ? com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState.ACTIVE : result;
}
/**
* optional .storage_onestore_v3.IndexMetadata.IndexState index_state = 3 [default = ACTIVE];
* @param value The indexState to set.
* @return This builder for chaining.
*/
public Builder setIndexState(com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexState value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
indexState_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .storage_onestore_v3.IndexMetadata.IndexState index_state = 3 [default = ACTIVE];
* @return This builder for chaining.
*/
public Builder clearIndexState() {
bitField0_ = (bitField0_ & ~0x00000004);
indexState_ = 0;
onChanged();
return this;
}
private long indexDeleteTime_ ;
/**
*
* The scheduled deletion time, in milliseconds from 1970-01-01T00:00:00Z
* Present iff index_state is SOFT_DELETED or PURGING.
*
*
* optional int64 index_delete_time = 4;
* @return Whether the indexDeleteTime field is set.
*/
@java.lang.Override
public boolean hasIndexDeleteTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The scheduled deletion time, in milliseconds from 1970-01-01T00:00:00Z
* Present iff index_state is SOFT_DELETED or PURGING.
*
*
* optional int64 index_delete_time = 4;
* @return The indexDeleteTime.
*/
@java.lang.Override
public long getIndexDeleteTime() {
return indexDeleteTime_;
}
/**
*
* The scheduled deletion time, in milliseconds from 1970-01-01T00:00:00Z
* Present iff index_state is SOFT_DELETED or PURGING.
*
*
* optional int64 index_delete_time = 4;
* @param value The indexDeleteTime to set.
* @return This builder for chaining.
*/
public Builder setIndexDeleteTime(long value) {
indexDeleteTime_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The scheduled deletion time, in milliseconds from 1970-01-01T00:00:00Z
* Present iff index_state is SOFT_DELETED or PURGING.
*
*
* optional int64 index_delete_time = 4;
* @return This builder for chaining.
*/
public Builder clearIndexDeleteTime() {
bitField0_ = (bitField0_ & ~0x00000008);
indexDeleteTime_ = 0L;
onChanged();
return this;
}
private long maxIndexSizeBytes_ ;
/**
*
* The maximum size of the index (if quota override is present).
* Used to: recreate dynamic quota profiles and purge them during wipeout.
*
*
* optional int64 max_index_size_bytes = 5;
* @return Whether the maxIndexSizeBytes field is set.
*/
@java.lang.Override
public boolean hasMaxIndexSizeBytes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The maximum size of the index (if quota override is present).
* Used to: recreate dynamic quota profiles and purge them during wipeout.
*
*
* optional int64 max_index_size_bytes = 5;
* @return The maxIndexSizeBytes.
*/
@java.lang.Override
public long getMaxIndexSizeBytes() {
return maxIndexSizeBytes_;
}
/**
*
* The maximum size of the index (if quota override is present).
* Used to: recreate dynamic quota profiles and purge them during wipeout.
*
*
* optional int64 max_index_size_bytes = 5;
* @param value The maxIndexSizeBytes to set.
* @return This builder for chaining.
*/
public Builder setMaxIndexSizeBytes(long value) {
maxIndexSizeBytes_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The maximum size of the index (if quota override is present).
* Used to: recreate dynamic quota profiles and purge them during wipeout.
*
*
* optional int64 max_index_size_bytes = 5;
* @return This builder for chaining.
*/
public Builder clearMaxIndexSizeBytes() {
bitField0_ = (bitField0_ & ~0x00000010);
maxIndexSizeBytes_ = 0L;
onChanged();
return this;
}
private java.util.List replicaDeletion_ =
java.util.Collections.emptyList();
private void ensureReplicaDeletionIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
replicaDeletion_ = new java.util.ArrayList(replicaDeletion_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder> replicaDeletionBuilder_;
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public java.util.List getReplicaDeletionList() {
if (replicaDeletionBuilder_ == null) {
return java.util.Collections.unmodifiableList(replicaDeletion_);
} else {
return replicaDeletionBuilder_.getMessageList();
}
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public int getReplicaDeletionCount() {
if (replicaDeletionBuilder_ == null) {
return replicaDeletion_.size();
} else {
return replicaDeletionBuilder_.getCount();
}
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails getReplicaDeletion(int index) {
if (replicaDeletionBuilder_ == null) {
return replicaDeletion_.get(index);
} else {
return replicaDeletionBuilder_.getMessage(index);
}
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder setReplicaDeletion(
int index, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails value) {
if (replicaDeletionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReplicaDeletionIsMutable();
replicaDeletion_.set(index, value);
onChanged();
} else {
replicaDeletionBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder setReplicaDeletion(
int index, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder builderForValue) {
if (replicaDeletionBuilder_ == null) {
ensureReplicaDeletionIsMutable();
replicaDeletion_.set(index, builderForValue.build());
onChanged();
} else {
replicaDeletionBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder addReplicaDeletion(com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails value) {
if (replicaDeletionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReplicaDeletionIsMutable();
replicaDeletion_.add(value);
onChanged();
} else {
replicaDeletionBuilder_.addMessage(value);
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder addReplicaDeletion(
int index, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails value) {
if (replicaDeletionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReplicaDeletionIsMutable();
replicaDeletion_.add(index, value);
onChanged();
} else {
replicaDeletionBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder addReplicaDeletion(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder builderForValue) {
if (replicaDeletionBuilder_ == null) {
ensureReplicaDeletionIsMutable();
replicaDeletion_.add(builderForValue.build());
onChanged();
} else {
replicaDeletionBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder addReplicaDeletion(
int index, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder builderForValue) {
if (replicaDeletionBuilder_ == null) {
ensureReplicaDeletionIsMutable();
replicaDeletion_.add(index, builderForValue.build());
onChanged();
} else {
replicaDeletionBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder addAllReplicaDeletion(
java.lang.Iterable extends com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails> values) {
if (replicaDeletionBuilder_ == null) {
ensureReplicaDeletionIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, replicaDeletion_);
onChanged();
} else {
replicaDeletionBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder clearReplicaDeletion() {
if (replicaDeletionBuilder_ == null) {
replicaDeletion_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
replicaDeletionBuilder_.clear();
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public Builder removeReplicaDeletion(int index) {
if (replicaDeletionBuilder_ == null) {
ensureReplicaDeletionIsMutable();
replicaDeletion_.remove(index);
onChanged();
} else {
replicaDeletionBuilder_.remove(index);
}
return this;
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder getReplicaDeletionBuilder(
int index) {
return getReplicaDeletionFieldBuilder().getBuilder(index);
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder getReplicaDeletionOrBuilder(
int index) {
if (replicaDeletionBuilder_ == null) {
return replicaDeletion_.get(index); } else {
return replicaDeletionBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public java.util.List extends com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder>
getReplicaDeletionOrBuilderList() {
if (replicaDeletionBuilder_ != null) {
return replicaDeletionBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(replicaDeletion_);
}
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder addReplicaDeletionBuilder() {
return getReplicaDeletionFieldBuilder().addBuilder(
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.getDefaultInstance());
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder addReplicaDeletionBuilder(
int index) {
return getReplicaDeletionFieldBuilder().addBuilder(
index, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.getDefaultInstance());
}
/**
*
* Details about which components have completed for index deletion,
* one per replica.
*
*
* repeated .storage_onestore_v3.IndexMetadata.IndexDeletionDetails replica_deletion = 6;
*/
public java.util.List
getReplicaDeletionBuilderList() {
return getReplicaDeletionFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder>
getReplicaDeletionFieldBuilder() {
if (replicaDeletionBuilder_ == null) {
replicaDeletionBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetails.Builder, com.google.apphosting.api.search.DocumentPb.IndexMetadata.IndexDeletionDetailsOrBuilder>(
replicaDeletion_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
replicaDeletion_ = null;
}
return replicaDeletionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.IndexMetadata)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.IndexMetadata)
private static final com.google.apphosting.api.search.DocumentPb.IndexMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.IndexMetadata();
}
public static com.google.apphosting.api.search.DocumentPb.IndexMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IndexMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.IndexMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FacetValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.FacetValue)
com.google.protobuf.MessageOrBuilder {
/**
*
* The type of content in this facet value.
*
*
* optional .storage_onestore_v3.FacetValue.ContentType type = 1 [default = ATOM];
* @return Whether the type field is set.
*/
boolean hasType();
/**
*
* The type of content in this facet value.
*
*
* optional .storage_onestore_v3.FacetValue.ContentType type = 1 [default = ATOM];
* @return The type.
*/
com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType getType();
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return Whether the stringValue field is set.
*/
boolean hasStringValue();
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return The stringValue.
*/
java.lang.String getStringValue();
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return The bytes for stringValue.
*/
com.google.protobuf.ByteString
getStringValueBytes();
}
/**
*
* The value of a document facet.
*
*
* Protobuf type {@code storage_onestore_v3.FacetValue}
*/
public static final class FacetValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.FacetValue)
FacetValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use FacetValue.newBuilder() to construct.
private FacetValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FacetValue() {
type_ = 2;
stringValue_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new FacetValue();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FacetValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FacetValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.FacetValue.class, com.google.apphosting.api.search.DocumentPb.FacetValue.Builder.class);
}
/**
*
* The type of content in the facet value.
* Keeping the numbering consistent with FieldType
*
*
* Protobuf enum {@code storage_onestore_v3.FacetValue.ContentType}
*/
public enum ContentType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The content is indivisible text.
*
*
* ATOM = 2;
*/
ATOM(2),
/**
*
* The content is a number.
*
*
* NUMBER = 4;
*/
NUMBER(4),
;
/**
*
* The content is indivisible text.
*
*
* ATOM = 2;
*/
public static final int ATOM_VALUE = 2;
/**
*
* The content is a number.
*
*
* NUMBER = 4;
*/
public static final int NUMBER_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ContentType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ContentType forNumber(int value) {
switch (value) {
case 2: return ATOM;
case 4: return NUMBER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ContentType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ContentType findValueByNumber(int number) {
return ContentType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.FacetValue.getDescriptor().getEnumTypes().get(0);
}
private static final ContentType[] VALUES = values();
public static ContentType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ContentType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:storage_onestore_v3.FacetValue.ContentType)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_ = 2;
/**
*
* The type of content in this facet value.
*
*
* optional .storage_onestore_v3.FacetValue.ContentType type = 1 [default = ATOM];
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The type of content in this facet value.
*
*
* optional .storage_onestore_v3.FacetValue.ContentType type = 1 [default = ATOM];
* @return The type.
*/
@java.lang.Override public com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType getType() {
com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType result = com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType.forNumber(type_);
return result == null ? com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType.ATOM : result;
}
public static final int STRING_VALUE_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object stringValue_ = "";
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return Whether the stringValue field is set.
*/
@java.lang.Override
public boolean hasStringValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return The stringValue.
*/
@java.lang.Override
public java.lang.String getStringValue() {
java.lang.Object ref = stringValue_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stringValue_ = s;
}
return s;
}
}
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return The bytes for stringValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = stringValue_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stringValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, stringValue_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, stringValue_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.FacetValue)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.FacetValue other = (com.google.apphosting.api.search.DocumentPb.FacetValue) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasStringValue() != other.hasStringValue()) return false;
if (hasStringValue()) {
if (!getStringValue()
.equals(other.getStringValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasStringValue()) {
hash = (37 * hash) + STRING_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getStringValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.FacetValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The value of a document facet.
*
*
* Protobuf type {@code storage_onestore_v3.FacetValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.FacetValue)
com.google.apphosting.api.search.DocumentPb.FacetValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FacetValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FacetValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.FacetValue.class, com.google.apphosting.api.search.DocumentPb.FacetValue.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.FacetValue.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
type_ = 2;
stringValue_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_FacetValue_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FacetValue getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.FacetValue.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FacetValue build() {
com.google.apphosting.api.search.DocumentPb.FacetValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FacetValue buildPartial() {
com.google.apphosting.api.search.DocumentPb.FacetValue result = new com.google.apphosting.api.search.DocumentPb.FacetValue(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.FacetValue result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.type_ = type_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.stringValue_ = stringValue_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.FacetValue) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.FacetValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.FacetValue other) {
if (other == com.google.apphosting.api.search.DocumentPb.FacetValue.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasStringValue()) {
stringValue_ = other.stringValue_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int tmpRaw = input.readEnum();
com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType tmpValue =
com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
type_ = tmpRaw;
bitField0_ |= 0x00000001;
}
break;
} // case 8
case 26: {
stringValue_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int type_ = 2;
/**
*
* The type of content in this facet value.
*
*
* optional .storage_onestore_v3.FacetValue.ContentType type = 1 [default = ATOM];
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The type of content in this facet value.
*
*
* optional .storage_onestore_v3.FacetValue.ContentType type = 1 [default = ATOM];
* @return The type.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType getType() {
com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType result = com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType.forNumber(type_);
return result == null ? com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType.ATOM : result;
}
/**
*
* The type of content in this facet value.
*
*
* optional .storage_onestore_v3.FacetValue.ContentType type = 1 [default = ATOM];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(com.google.apphosting.api.search.DocumentPb.FacetValue.ContentType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The type of content in this facet value.
*
*
* optional .storage_onestore_v3.FacetValue.ContentType type = 1 [default = ATOM];
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 2;
onChanged();
return this;
}
private java.lang.Object stringValue_ = "";
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return Whether the stringValue field is set.
*/
public boolean hasStringValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return The stringValue.
*/
public java.lang.String getStringValue() {
java.lang.Object ref = stringValue_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
stringValue_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return The bytes for stringValue.
*/
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = stringValue_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
stringValue_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @param value The stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValue(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
stringValue_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @return This builder for chaining.
*/
public Builder clearStringValue() {
stringValue_ = getDefaultInstance().getStringValue();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* The field in which ATOM or NUMBER values are stored.
* For NUMBER fields, it is formatted as what Java's
* Double.parseDouble accepts.
*
*
* optional string string_value = 3;
* @param value The bytes for stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
stringValue_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.FacetValue)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.FacetValue)
private static final com.google.apphosting.api.search.DocumentPb.FacetValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.FacetValue();
}
public static com.google.apphosting.api.search.DocumentPb.FacetValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FacetValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FacetValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FacetOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.Facet)
com.google.protobuf.MessageOrBuilder {
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
* @return The value.
*/
com.google.apphosting.api.search.DocumentPb.FacetValue getValue();
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
com.google.apphosting.api.search.DocumentPb.FacetValueOrBuilder getValueOrBuilder();
}
/**
*
* A document facet.
*
*
* Protobuf type {@code storage_onestore_v3.Facet}
*/
public static final class Facet extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.Facet)
FacetOrBuilder {
private static final long serialVersionUID = 0L;
// Use Facet.newBuilder() to construct.
private Facet(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Facet() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Facet();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Facet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Facet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.Facet.class, com.google.apphosting.api.search.DocumentPb.Facet.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private com.google.apphosting.api.search.DocumentPb.FacetValue value_;
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
* @return The value.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FacetValue getValue() {
return value_ == null ? com.google.apphosting.api.search.DocumentPb.FacetValue.getDefaultInstance() : value_;
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FacetValueOrBuilder getValueOrBuilder() {
return value_ == null ? com.google.apphosting.api.search.DocumentPb.FacetValue.getDefaultInstance() : value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getValue());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.Facet)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.Facet other = (com.google.apphosting.api.search.DocumentPb.Facet) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Facet parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.Facet prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A document facet.
*
*
* Protobuf type {@code storage_onestore_v3.Facet}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.Facet)
com.google.apphosting.api.search.DocumentPb.FacetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Facet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Facet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.Facet.class, com.google.apphosting.api.search.DocumentPb.Facet.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.Facet.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
value_ = null;
if (valueBuilder_ != null) {
valueBuilder_.dispose();
valueBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Facet_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Facet getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.Facet.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Facet build() {
com.google.apphosting.api.search.DocumentPb.Facet result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Facet buildPartial() {
com.google.apphosting.api.search.DocumentPb.Facet result = new com.google.apphosting.api.search.DocumentPb.Facet(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.Facet result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = valueBuilder_ == null
? value_
: valueBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.Facet) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.Facet)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.Facet other) {
if (other == com.google.apphosting.api.search.DocumentPb.Facet.getDefaultInstance()) return this;
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getValueFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The name of the facet.
*
*
* required string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.apphosting.api.search.DocumentPb.FacetValue value_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FacetValue, com.google.apphosting.api.search.DocumentPb.FacetValue.Builder, com.google.apphosting.api.search.DocumentPb.FacetValueOrBuilder> valueBuilder_;
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
* @return The value.
*/
public com.google.apphosting.api.search.DocumentPb.FacetValue getValue() {
if (valueBuilder_ == null) {
return value_ == null ? com.google.apphosting.api.search.DocumentPb.FacetValue.getDefaultInstance() : value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
public Builder setValue(com.google.apphosting.api.search.DocumentPb.FacetValue value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
} else {
valueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
public Builder setValue(
com.google.apphosting.api.search.DocumentPb.FacetValue.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
public Builder mergeValue(com.google.apphosting.api.search.DocumentPb.FacetValue value) {
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
value_ != null &&
value_ != com.google.apphosting.api.search.DocumentPb.FacetValue.getDefaultInstance()) {
getValueBuilder().mergeFrom(value);
} else {
value_ = value;
}
} else {
valueBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = null;
if (valueBuilder_ != null) {
valueBuilder_.dispose();
valueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
public com.google.apphosting.api.search.DocumentPb.FacetValue.Builder getValueBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
public com.google.apphosting.api.search.DocumentPb.FacetValueOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_ == null ?
com.google.apphosting.api.search.DocumentPb.FacetValue.getDefaultInstance() : value_;
}
}
/**
*
* The value of the facet.
*
*
* required .storage_onestore_v3.FacetValue value = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FacetValue, com.google.apphosting.api.search.DocumentPb.FacetValue.Builder, com.google.apphosting.api.search.DocumentPb.FacetValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.FacetValue, com.google.apphosting.api.search.DocumentPb.FacetValue.Builder, com.google.apphosting.api.search.DocumentPb.FacetValueOrBuilder>(
getValue(),
getParentForChildren(),
isClean());
value_ = null;
}
return valueBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.Facet)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.Facet)
private static final com.google.apphosting.api.search.DocumentPb.Facet DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.Facet();
}
public static com.google.apphosting.api.search.DocumentPb.Facet getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Facet parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Facet getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DocumentMetadataOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.DocumentMetadata)
com.google.protobuf.MessageOrBuilder {
/**
*
* The version number of this document. This should increment every time the
* document is modified. DocumentMetadata will stay around after deletes, so
* the version number will carry over after a delete and a document of the
* same name is indexed.
*
*
* optional int64 version = 1;
* @return Whether the version field is set.
*/
boolean hasVersion();
/**
*
* The version number of this document. This should increment every time the
* document is modified. DocumentMetadata will stay around after deletes, so
* the version number will carry over after a delete and a document of the
* same name is indexed.
*
*
* optional int64 version = 1;
* @return The version.
*/
long getVersion();
/**
*
* The highest version number of this document that has been written to the
* ST index.
*
*
* optional int64 committed_st_version = 2;
* @return Whether the committedStVersion field is set.
*/
boolean hasCommittedStVersion();
/**
*
* The highest version number of this document that has been written to the
* ST index.
*
*
* optional int64 committed_st_version = 2;
* @return The committedStVersion.
*/
long getCommittedStVersion();
}
/**
*
* The metadata of a document.
*
*
* Protobuf type {@code storage_onestore_v3.DocumentMetadata}
*/
public static final class DocumentMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.DocumentMetadata)
DocumentMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use DocumentMetadata.newBuilder() to construct.
private DocumentMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DocumentMetadata() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DocumentMetadata();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_DocumentMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_DocumentMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.DocumentMetadata.class, com.google.apphosting.api.search.DocumentPb.DocumentMetadata.Builder.class);
}
private int bitField0_;
public static final int VERSION_FIELD_NUMBER = 1;
private long version_ = 0L;
/**
*
* The version number of this document. This should increment every time the
* document is modified. DocumentMetadata will stay around after deletes, so
* the version number will carry over after a delete and a document of the
* same name is indexed.
*
*
* optional int64 version = 1;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The version number of this document. This should increment every time the
* document is modified. DocumentMetadata will stay around after deletes, so
* the version number will carry over after a delete and a document of the
* same name is indexed.
*
*
* optional int64 version = 1;
* @return The version.
*/
@java.lang.Override
public long getVersion() {
return version_;
}
public static final int COMMITTED_ST_VERSION_FIELD_NUMBER = 2;
private long committedStVersion_ = 0L;
/**
*
* The highest version number of this document that has been written to the
* ST index.
*
*
* optional int64 committed_st_version = 2;
* @return Whether the committedStVersion field is set.
*/
@java.lang.Override
public boolean hasCommittedStVersion() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The highest version number of this document that has been written to the
* ST index.
*
*
* optional int64 committed_st_version = 2;
* @return The committedStVersion.
*/
@java.lang.Override
public long getCommittedStVersion() {
return committedStVersion_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt64(1, version_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt64(2, committedStVersion_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, version_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, committedStVersion_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.DocumentMetadata)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.DocumentMetadata other = (com.google.apphosting.api.search.DocumentPb.DocumentMetadata) obj;
if (hasVersion() != other.hasVersion()) return false;
if (hasVersion()) {
if (getVersion()
!= other.getVersion()) return false;
}
if (hasCommittedStVersion() != other.hasCommittedStVersion()) return false;
if (hasCommittedStVersion()) {
if (getCommittedStVersion()
!= other.getCommittedStVersion()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getVersion());
}
if (hasCommittedStVersion()) {
hash = (37 * hash) + COMMITTED_ST_VERSION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCommittedStVersion());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.DocumentMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The metadata of a document.
*
*
* Protobuf type {@code storage_onestore_v3.DocumentMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.DocumentMetadata)
com.google.apphosting.api.search.DocumentPb.DocumentMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_DocumentMetadata_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_DocumentMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.DocumentMetadata.class, com.google.apphosting.api.search.DocumentPb.DocumentMetadata.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.DocumentMetadata.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
version_ = 0L;
committedStVersion_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_DocumentMetadata_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.DocumentMetadata getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.DocumentMetadata.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.DocumentMetadata build() {
com.google.apphosting.api.search.DocumentPb.DocumentMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.DocumentMetadata buildPartial() {
com.google.apphosting.api.search.DocumentPb.DocumentMetadata result = new com.google.apphosting.api.search.DocumentPb.DocumentMetadata(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.DocumentMetadata result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.version_ = version_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.committedStVersion_ = committedStVersion_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.DocumentMetadata) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.DocumentMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.DocumentMetadata other) {
if (other == com.google.apphosting.api.search.DocumentPb.DocumentMetadata.getDefaultInstance()) return this;
if (other.hasVersion()) {
setVersion(other.getVersion());
}
if (other.hasCommittedStVersion()) {
setCommittedStVersion(other.getCommittedStVersion());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
version_ = input.readInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
committedStVersion_ = input.readInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long version_ ;
/**
*
* The version number of this document. This should increment every time the
* document is modified. DocumentMetadata will stay around after deletes, so
* the version number will carry over after a delete and a document of the
* same name is indexed.
*
*
* optional int64 version = 1;
* @return Whether the version field is set.
*/
@java.lang.Override
public boolean hasVersion() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The version number of this document. This should increment every time the
* document is modified. DocumentMetadata will stay around after deletes, so
* the version number will carry over after a delete and a document of the
* same name is indexed.
*
*
* optional int64 version = 1;
* @return The version.
*/
@java.lang.Override
public long getVersion() {
return version_;
}
/**
*
* The version number of this document. This should increment every time the
* document is modified. DocumentMetadata will stay around after deletes, so
* the version number will carry over after a delete and a document of the
* same name is indexed.
*
*
* optional int64 version = 1;
* @param value The version to set.
* @return This builder for chaining.
*/
public Builder setVersion(long value) {
version_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The version number of this document. This should increment every time the
* document is modified. DocumentMetadata will stay around after deletes, so
* the version number will carry over after a delete and a document of the
* same name is indexed.
*
*
* optional int64 version = 1;
* @return This builder for chaining.
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000001);
version_ = 0L;
onChanged();
return this;
}
private long committedStVersion_ ;
/**
*
* The highest version number of this document that has been written to the
* ST index.
*
*
* optional int64 committed_st_version = 2;
* @return Whether the committedStVersion field is set.
*/
@java.lang.Override
public boolean hasCommittedStVersion() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The highest version number of this document that has been written to the
* ST index.
*
*
* optional int64 committed_st_version = 2;
* @return The committedStVersion.
*/
@java.lang.Override
public long getCommittedStVersion() {
return committedStVersion_;
}
/**
*
* The highest version number of this document that has been written to the
* ST index.
*
*
* optional int64 committed_st_version = 2;
* @param value The committedStVersion to set.
* @return This builder for chaining.
*/
public Builder setCommittedStVersion(long value) {
committedStVersion_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The highest version number of this document that has been written to the
* ST index.
*
*
* optional int64 committed_st_version = 2;
* @return This builder for chaining.
*/
public Builder clearCommittedStVersion() {
bitField0_ = (bitField0_ & ~0x00000002);
committedStVersion_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.DocumentMetadata)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.DocumentMetadata)
private static final com.google.apphosting.api.search.DocumentPb.DocumentMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.DocumentMetadata();
}
public static com.google.apphosting.api.search.DocumentPb.DocumentMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DocumentMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.DocumentMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DocumentOrBuilder extends
// @@protoc_insertion_point(interface_extends:storage_onestore_v3.Document)
com.google.protobuf.MessageOrBuilder {
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return Whether the id field is set.
*/
boolean hasId();
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return Whether the language field is set.
*/
boolean hasLanguage();
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The language.
*/
java.lang.String getLanguage();
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The bytes for language.
*/
com.google.protobuf.ByteString
getLanguageBytes();
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
java.util.List
getFieldList();
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
com.google.apphosting.api.search.DocumentPb.Field getField(int index);
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
int getFieldCount();
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
java.util.List extends com.google.apphosting.api.search.DocumentPb.FieldOrBuilder>
getFieldOrBuilderList();
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
com.google.apphosting.api.search.DocumentPb.FieldOrBuilder getFieldOrBuilder(
int index);
/**
*
* An id specified by the client, used to return documents in a defined
* order in search results. If it is not specified, then the number
* of seconds since 2011/1/1.
*
*
* optional int32 order_id = 4;
* @return Whether the orderId field is set.
*/
boolean hasOrderId();
/**
*
* An id specified by the client, used to return documents in a defined
* order in search results. If it is not specified, then the number
* of seconds since 2011/1/1.
*
*
* optional int32 order_id = 4;
* @return The orderId.
*/
int getOrderId();
/**
*
* Did we generate the order id ("rank") or did the client provide it?
*
*
* optional .storage_onestore_v3.Document.OrderIdSource order_id_source = 6 [default = SUPPLIED];
* @return Whether the orderIdSource field is set.
*/
boolean hasOrderIdSource();
/**
*
* Did we generate the order id ("rank") or did the client provide it?
*
*
* optional .storage_onestore_v3.Document.OrderIdSource order_id_source = 6 [default = SUPPLIED];
* @return The orderIdSource.
*/
com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource getOrderIdSource();
/**
*
* The storage type the index is built on.
*
*
* optional .storage_onestore_v3.Document.Storage storage = 5 [default = DISK];
* @return Whether the storage field is set.
*/
boolean hasStorage();
/**
*
* The storage type the index is built on.
*
*
* optional .storage_onestore_v3.Document.Storage storage = 5 [default = DISK];
* @return The storage.
*/
com.google.apphosting.api.search.DocumentPb.Document.Storage getStorage();
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
java.util.List
getFacetList();
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
com.google.apphosting.api.search.DocumentPb.Facet getFacet(int index);
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
int getFacetCount();
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
java.util.List extends com.google.apphosting.api.search.DocumentPb.FacetOrBuilder>
getFacetOrBuilderList();
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
com.google.apphosting.api.search.DocumentPb.FacetOrBuilder getFacetOrBuilder(
int index);
}
/**
*
* A document is a collection of fields.
*
*
* Protobuf type {@code storage_onestore_v3.Document}
*/
public static final class Document extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:storage_onestore_v3.Document)
DocumentOrBuilder {
private static final long serialVersionUID = 0L;
// Use Document.newBuilder() to construct.
private Document(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Document() {
id_ = "";
language_ = "en";
field_ = java.util.Collections.emptyList();
orderIdSource_ = 1;
storage_ = 0;
facet_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Document();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Document_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Document_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.Document.class, com.google.apphosting.api.search.DocumentPb.Document.Builder.class);
}
/**
* Protobuf enum {@code storage_onestore_v3.Document.OrderIdSource}
*/
public enum OrderIdSource
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* We generated an order ID.
*
*
* DEFAULTED = 0;
*/
DEFAULTED(0),
/**
*
* The client provided an explicit order ID.
*
*
* SUPPLIED = 1;
*/
SUPPLIED(1),
;
/**
*
* We generated an order ID.
*
*
* DEFAULTED = 0;
*/
public static final int DEFAULTED_VALUE = 0;
/**
*
* The client provided an explicit order ID.
*
*
* SUPPLIED = 1;
*/
public static final int SUPPLIED_VALUE = 1;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OrderIdSource valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static OrderIdSource forNumber(int value) {
switch (value) {
case 0: return DEFAULTED;
case 1: return SUPPLIED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OrderIdSource> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OrderIdSource findValueByNumber(int number) {
return OrderIdSource.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.Document.getDescriptor().getEnumTypes().get(0);
}
private static final OrderIdSource[] VALUES = values();
public static OrderIdSource valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private OrderIdSource(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:storage_onestore_v3.Document.OrderIdSource)
}
/**
*
* What sort of storage the index is built on.
*
*
* Protobuf enum {@code storage_onestore_v3.Document.Storage}
*/
public enum Storage
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Use a disk-based index.
*
*
* DISK = 0;
*/
DISK(0),
;
/**
*
* Use a disk-based index.
*
*
* DISK = 0;
*/
public static final int DISK_VALUE = 0;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Storage valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Storage forNumber(int value) {
switch (value) {
case 0: return DISK;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Storage> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Storage findValueByNumber(int number) {
return Storage.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.Document.getDescriptor().getEnumTypes().get(1);
}
private static final Storage[] VALUES = values();
public static Storage valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Storage(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:storage_onestore_v3.Document.Storage)
}
private int bitField0_;
public static final int ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object id_ = "";
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LANGUAGE_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object language_ = "en";
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return Whether the language field is set.
*/
@java.lang.Override
public boolean hasLanguage() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The language.
*/
@java.lang.Override
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
}
}
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The bytes for language.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FIELD_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List field_;
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
@java.lang.Override
public java.util.List getFieldList() {
return field_;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.api.search.DocumentPb.FieldOrBuilder>
getFieldOrBuilderList() {
return field_;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
@java.lang.Override
public int getFieldCount() {
return field_.size();
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Field getField(int index) {
return field_.get(index);
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FieldOrBuilder getFieldOrBuilder(
int index) {
return field_.get(index);
}
public static final int ORDER_ID_FIELD_NUMBER = 4;
private int orderId_ = 0;
/**
*
* An id specified by the client, used to return documents in a defined
* order in search results. If it is not specified, then the number
* of seconds since 2011/1/1.
*
*
* optional int32 order_id = 4;
* @return Whether the orderId field is set.
*/
@java.lang.Override
public boolean hasOrderId() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* An id specified by the client, used to return documents in a defined
* order in search results. If it is not specified, then the number
* of seconds since 2011/1/1.
*
*
* optional int32 order_id = 4;
* @return The orderId.
*/
@java.lang.Override
public int getOrderId() {
return orderId_;
}
public static final int ORDER_ID_SOURCE_FIELD_NUMBER = 6;
private int orderIdSource_ = 1;
/**
*
* Did we generate the order id ("rank") or did the client provide it?
*
*
* optional .storage_onestore_v3.Document.OrderIdSource order_id_source = 6 [default = SUPPLIED];
* @return Whether the orderIdSource field is set.
*/
@java.lang.Override public boolean hasOrderIdSource() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Did we generate the order id ("rank") or did the client provide it?
*
*
* optional .storage_onestore_v3.Document.OrderIdSource order_id_source = 6 [default = SUPPLIED];
* @return The orderIdSource.
*/
@java.lang.Override public com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource getOrderIdSource() {
com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource result = com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource.forNumber(orderIdSource_);
return result == null ? com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource.SUPPLIED : result;
}
public static final int STORAGE_FIELD_NUMBER = 5;
private int storage_ = 0;
/**
*
* The storage type the index is built on.
*
*
* optional .storage_onestore_v3.Document.Storage storage = 5 [default = DISK];
* @return Whether the storage field is set.
*/
@java.lang.Override public boolean hasStorage() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The storage type the index is built on.
*
*
* optional .storage_onestore_v3.Document.Storage storage = 5 [default = DISK];
* @return The storage.
*/
@java.lang.Override public com.google.apphosting.api.search.DocumentPb.Document.Storage getStorage() {
com.google.apphosting.api.search.DocumentPb.Document.Storage result = com.google.apphosting.api.search.DocumentPb.Document.Storage.forNumber(storage_);
return result == null ? com.google.apphosting.api.search.DocumentPb.Document.Storage.DISK : result;
}
public static final int FACET_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private java.util.List facet_;
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
@java.lang.Override
public java.util.List getFacetList() {
return facet_;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.api.search.DocumentPb.FacetOrBuilder>
getFacetOrBuilderList() {
return facet_;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
@java.lang.Override
public int getFacetCount() {
return facet_.size();
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Facet getFacet(int index) {
return facet_.get(index);
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.FacetOrBuilder getFacetOrBuilder(
int index) {
return facet_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
for (int i = 0; i < getFieldCount(); i++) {
if (!getField(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getFacetCount(); i++) {
if (!getFacet(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, language_);
}
for (int i = 0; i < field_.size(); i++) {
output.writeMessage(3, field_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt32(4, orderId_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeEnum(5, storage_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeEnum(6, orderIdSource_);
}
for (int i = 0; i < facet_.size(); i++) {
output.writeMessage(8, facet_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, language_);
}
for (int i = 0; i < field_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, field_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, orderId_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, storage_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, orderIdSource_);
}
for (int i = 0; i < facet_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, facet_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.api.search.DocumentPb.Document)) {
return super.equals(obj);
}
com.google.apphosting.api.search.DocumentPb.Document other = (com.google.apphosting.api.search.DocumentPb.Document) obj;
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (!getId()
.equals(other.getId())) return false;
}
if (hasLanguage() != other.hasLanguage()) return false;
if (hasLanguage()) {
if (!getLanguage()
.equals(other.getLanguage())) return false;
}
if (!getFieldList()
.equals(other.getFieldList())) return false;
if (hasOrderId() != other.hasOrderId()) return false;
if (hasOrderId()) {
if (getOrderId()
!= other.getOrderId()) return false;
}
if (hasOrderIdSource() != other.hasOrderIdSource()) return false;
if (hasOrderIdSource()) {
if (orderIdSource_ != other.orderIdSource_) return false;
}
if (hasStorage() != other.hasStorage()) return false;
if (hasStorage()) {
if (storage_ != other.storage_) return false;
}
if (!getFacetList()
.equals(other.getFacetList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
}
if (hasLanguage()) {
hash = (37 * hash) + LANGUAGE_FIELD_NUMBER;
hash = (53 * hash) + getLanguage().hashCode();
}
if (getFieldCount() > 0) {
hash = (37 * hash) + FIELD_FIELD_NUMBER;
hash = (53 * hash) + getFieldList().hashCode();
}
if (hasOrderId()) {
hash = (37 * hash) + ORDER_ID_FIELD_NUMBER;
hash = (53 * hash) + getOrderId();
}
if (hasOrderIdSource()) {
hash = (37 * hash) + ORDER_ID_SOURCE_FIELD_NUMBER;
hash = (53 * hash) + orderIdSource_;
}
if (hasStorage()) {
hash = (37 * hash) + STORAGE_FIELD_NUMBER;
hash = (53 * hash) + storage_;
}
if (getFacetCount() > 0) {
hash = (37 * hash) + FACET_FIELD_NUMBER;
hash = (53 * hash) + getFacetList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.api.search.DocumentPb.Document parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.api.search.DocumentPb.Document prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A document is a collection of fields.
*
*
* Protobuf type {@code storage_onestore_v3.Document}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:storage_onestore_v3.Document)
com.google.apphosting.api.search.DocumentPb.DocumentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Document_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Document_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.api.search.DocumentPb.Document.class, com.google.apphosting.api.search.DocumentPb.Document.Builder.class);
}
// Construct using com.google.apphosting.api.search.DocumentPb.Document.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
id_ = "";
language_ = "en";
if (fieldBuilder_ == null) {
field_ = java.util.Collections.emptyList();
} else {
field_ = null;
fieldBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
orderId_ = 0;
orderIdSource_ = 1;
storage_ = 0;
if (facetBuilder_ == null) {
facet_ = java.util.Collections.emptyList();
} else {
facet_ = null;
facetBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.api.search.DocumentPb.internal_static_storage_onestore_v3_Document_descriptor;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Document getDefaultInstanceForType() {
return com.google.apphosting.api.search.DocumentPb.Document.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Document build() {
com.google.apphosting.api.search.DocumentPb.Document result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Document buildPartial() {
com.google.apphosting.api.search.DocumentPb.Document result = new com.google.apphosting.api.search.DocumentPb.Document(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.api.search.DocumentPb.Document result) {
if (fieldBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
field_ = java.util.Collections.unmodifiableList(field_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.field_ = field_;
} else {
result.field_ = fieldBuilder_.build();
}
if (facetBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
facet_ = java.util.Collections.unmodifiableList(facet_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.facet_ = facet_;
} else {
result.facet_ = facetBuilder_.build();
}
}
private void buildPartial0(com.google.apphosting.api.search.DocumentPb.Document result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.language_ = language_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.orderId_ = orderId_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.orderIdSource_ = orderIdSource_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.storage_ = storage_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.api.search.DocumentPb.Document) {
return mergeFrom((com.google.apphosting.api.search.DocumentPb.Document)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.api.search.DocumentPb.Document other) {
if (other == com.google.apphosting.api.search.DocumentPb.Document.getDefaultInstance()) return this;
if (other.hasId()) {
id_ = other.id_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasLanguage()) {
language_ = other.language_;
bitField0_ |= 0x00000002;
onChanged();
}
if (fieldBuilder_ == null) {
if (!other.field_.isEmpty()) {
if (field_.isEmpty()) {
field_ = other.field_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureFieldIsMutable();
field_.addAll(other.field_);
}
onChanged();
}
} else {
if (!other.field_.isEmpty()) {
if (fieldBuilder_.isEmpty()) {
fieldBuilder_.dispose();
fieldBuilder_ = null;
field_ = other.field_;
bitField0_ = (bitField0_ & ~0x00000004);
fieldBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFieldFieldBuilder() : null;
} else {
fieldBuilder_.addAllMessages(other.field_);
}
}
}
if (other.hasOrderId()) {
setOrderId(other.getOrderId());
}
if (other.hasOrderIdSource()) {
setOrderIdSource(other.getOrderIdSource());
}
if (other.hasStorage()) {
setStorage(other.getStorage());
}
if (facetBuilder_ == null) {
if (!other.facet_.isEmpty()) {
if (facet_.isEmpty()) {
facet_ = other.facet_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureFacetIsMutable();
facet_.addAll(other.facet_);
}
onChanged();
}
} else {
if (!other.facet_.isEmpty()) {
if (facetBuilder_.isEmpty()) {
facetBuilder_.dispose();
facetBuilder_ = null;
facet_ = other.facet_;
bitField0_ = (bitField0_ & ~0x00000040);
facetBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFacetFieldBuilder() : null;
} else {
facetBuilder_.addAllMessages(other.facet_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
for (int i = 0; i < getFieldCount(); i++) {
if (!getField(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getFacetCount(); i++) {
if (!getFacet(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
id_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
language_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
com.google.apphosting.api.search.DocumentPb.Field m =
input.readMessage(
com.google.apphosting.api.search.DocumentPb.Field.PARSER,
extensionRegistry);
if (fieldBuilder_ == null) {
ensureFieldIsMutable();
field_.add(m);
} else {
fieldBuilder_.addMessage(m);
}
break;
} // case 26
case 32: {
orderId_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
int tmpRaw = input.readEnum();
com.google.apphosting.api.search.DocumentPb.Document.Storage tmpValue =
com.google.apphosting.api.search.DocumentPb.Document.Storage.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(5, tmpRaw);
} else {
storage_ = tmpRaw;
bitField0_ |= 0x00000020;
}
break;
} // case 40
case 48: {
int tmpRaw = input.readEnum();
com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource tmpValue =
com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(6, tmpRaw);
} else {
orderIdSource_ = tmpRaw;
bitField0_ |= 0x00000010;
}
break;
} // case 48
case 66: {
com.google.apphosting.api.search.DocumentPb.Facet m =
input.readMessage(
com.google.apphosting.api.search.DocumentPb.Facet.PARSER,
extensionRegistry);
if (facetBuilder_ == null) {
ensureFacetIsMutable();
facet_.add(m);
} else {
facetBuilder_.addMessage(m);
}
break;
} // case 66
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return Whether the id field is set.
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* An identifier for the document. If missing, a unique id will
* automatically be chosen for the document.
*
*
* optional string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
id_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object language_ = "en";
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return Whether the language field is set.
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The language.
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return The bytes for language.
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @param value The language to set.
* @return This builder for chaining.
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
language_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @return This builder for chaining.
*/
public Builder clearLanguage() {
language_ = getDefaultInstance().getLanguage();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* The language the document is written in. The language must be a valid ISO
* 639-1 code.
*
*
* optional string language = 2 [default = "en"];
* @param value The bytes for language to set.
* @return This builder for chaining.
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
language_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.util.List field_ =
java.util.Collections.emptyList();
private void ensureFieldIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
field_ = new java.util.ArrayList(field_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.Field, com.google.apphosting.api.search.DocumentPb.Field.Builder, com.google.apphosting.api.search.DocumentPb.FieldOrBuilder> fieldBuilder_;
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public java.util.List getFieldList() {
if (fieldBuilder_ == null) {
return java.util.Collections.unmodifiableList(field_);
} else {
return fieldBuilder_.getMessageList();
}
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public int getFieldCount() {
if (fieldBuilder_ == null) {
return field_.size();
} else {
return fieldBuilder_.getCount();
}
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public com.google.apphosting.api.search.DocumentPb.Field getField(int index) {
if (fieldBuilder_ == null) {
return field_.get(index);
} else {
return fieldBuilder_.getMessage(index);
}
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder setField(
int index, com.google.apphosting.api.search.DocumentPb.Field value) {
if (fieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldIsMutable();
field_.set(index, value);
onChanged();
} else {
fieldBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder setField(
int index, com.google.apphosting.api.search.DocumentPb.Field.Builder builderForValue) {
if (fieldBuilder_ == null) {
ensureFieldIsMutable();
field_.set(index, builderForValue.build());
onChanged();
} else {
fieldBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder addField(com.google.apphosting.api.search.DocumentPb.Field value) {
if (fieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldIsMutable();
field_.add(value);
onChanged();
} else {
fieldBuilder_.addMessage(value);
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder addField(
int index, com.google.apphosting.api.search.DocumentPb.Field value) {
if (fieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldIsMutable();
field_.add(index, value);
onChanged();
} else {
fieldBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder addField(
com.google.apphosting.api.search.DocumentPb.Field.Builder builderForValue) {
if (fieldBuilder_ == null) {
ensureFieldIsMutable();
field_.add(builderForValue.build());
onChanged();
} else {
fieldBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder addField(
int index, com.google.apphosting.api.search.DocumentPb.Field.Builder builderForValue) {
if (fieldBuilder_ == null) {
ensureFieldIsMutable();
field_.add(index, builderForValue.build());
onChanged();
} else {
fieldBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder addAllField(
java.lang.Iterable extends com.google.apphosting.api.search.DocumentPb.Field> values) {
if (fieldBuilder_ == null) {
ensureFieldIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, field_);
onChanged();
} else {
fieldBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder clearField() {
if (fieldBuilder_ == null) {
field_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
fieldBuilder_.clear();
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public Builder removeField(int index) {
if (fieldBuilder_ == null) {
ensureFieldIsMutable();
field_.remove(index);
onChanged();
} else {
fieldBuilder_.remove(index);
}
return this;
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public com.google.apphosting.api.search.DocumentPb.Field.Builder getFieldBuilder(
int index) {
return getFieldFieldBuilder().getBuilder(index);
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public com.google.apphosting.api.search.DocumentPb.FieldOrBuilder getFieldOrBuilder(
int index) {
if (fieldBuilder_ == null) {
return field_.get(index); } else {
return fieldBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public java.util.List extends com.google.apphosting.api.search.DocumentPb.FieldOrBuilder>
getFieldOrBuilderList() {
if (fieldBuilder_ != null) {
return fieldBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(field_);
}
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public com.google.apphosting.api.search.DocumentPb.Field.Builder addFieldBuilder() {
return getFieldFieldBuilder().addBuilder(
com.google.apphosting.api.search.DocumentPb.Field.getDefaultInstance());
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public com.google.apphosting.api.search.DocumentPb.Field.Builder addFieldBuilder(
int index) {
return getFieldFieldBuilder().addBuilder(
index, com.google.apphosting.api.search.DocumentPb.Field.getDefaultInstance());
}
/**
*
* The set of fields of the document.
*
*
* repeated .storage_onestore_v3.Field field = 3;
*/
public java.util.List
getFieldBuilderList() {
return getFieldFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.Field, com.google.apphosting.api.search.DocumentPb.Field.Builder, com.google.apphosting.api.search.DocumentPb.FieldOrBuilder>
getFieldFieldBuilder() {
if (fieldBuilder_ == null) {
fieldBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.Field, com.google.apphosting.api.search.DocumentPb.Field.Builder, com.google.apphosting.api.search.DocumentPb.FieldOrBuilder>(
field_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
field_ = null;
}
return fieldBuilder_;
}
private int orderId_ ;
/**
*
* An id specified by the client, used to return documents in a defined
* order in search results. If it is not specified, then the number
* of seconds since 2011/1/1.
*
*
* optional int32 order_id = 4;
* @return Whether the orderId field is set.
*/
@java.lang.Override
public boolean hasOrderId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* An id specified by the client, used to return documents in a defined
* order in search results. If it is not specified, then the number
* of seconds since 2011/1/1.
*
*
* optional int32 order_id = 4;
* @return The orderId.
*/
@java.lang.Override
public int getOrderId() {
return orderId_;
}
/**
*
* An id specified by the client, used to return documents in a defined
* order in search results. If it is not specified, then the number
* of seconds since 2011/1/1.
*
*
* optional int32 order_id = 4;
* @param value The orderId to set.
* @return This builder for chaining.
*/
public Builder setOrderId(int value) {
orderId_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* An id specified by the client, used to return documents in a defined
* order in search results. If it is not specified, then the number
* of seconds since 2011/1/1.
*
*
* optional int32 order_id = 4;
* @return This builder for chaining.
*/
public Builder clearOrderId() {
bitField0_ = (bitField0_ & ~0x00000008);
orderId_ = 0;
onChanged();
return this;
}
private int orderIdSource_ = 1;
/**
*
* Did we generate the order id ("rank") or did the client provide it?
*
*
* optional .storage_onestore_v3.Document.OrderIdSource order_id_source = 6 [default = SUPPLIED];
* @return Whether the orderIdSource field is set.
*/
@java.lang.Override public boolean hasOrderIdSource() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Did we generate the order id ("rank") or did the client provide it?
*
*
* optional .storage_onestore_v3.Document.OrderIdSource order_id_source = 6 [default = SUPPLIED];
* @return The orderIdSource.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource getOrderIdSource() {
com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource result = com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource.forNumber(orderIdSource_);
return result == null ? com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource.SUPPLIED : result;
}
/**
*
* Did we generate the order id ("rank") or did the client provide it?
*
*
* optional .storage_onestore_v3.Document.OrderIdSource order_id_source = 6 [default = SUPPLIED];
* @param value The orderIdSource to set.
* @return This builder for chaining.
*/
public Builder setOrderIdSource(com.google.apphosting.api.search.DocumentPb.Document.OrderIdSource value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
orderIdSource_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Did we generate the order id ("rank") or did the client provide it?
*
*
* optional .storage_onestore_v3.Document.OrderIdSource order_id_source = 6 [default = SUPPLIED];
* @return This builder for chaining.
*/
public Builder clearOrderIdSource() {
bitField0_ = (bitField0_ & ~0x00000010);
orderIdSource_ = 1;
onChanged();
return this;
}
private int storage_ = 0;
/**
*
* The storage type the index is built on.
*
*
* optional .storage_onestore_v3.Document.Storage storage = 5 [default = DISK];
* @return Whether the storage field is set.
*/
@java.lang.Override public boolean hasStorage() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* The storage type the index is built on.
*
*
* optional .storage_onestore_v3.Document.Storage storage = 5 [default = DISK];
* @return The storage.
*/
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Document.Storage getStorage() {
com.google.apphosting.api.search.DocumentPb.Document.Storage result = com.google.apphosting.api.search.DocumentPb.Document.Storage.forNumber(storage_);
return result == null ? com.google.apphosting.api.search.DocumentPb.Document.Storage.DISK : result;
}
/**
*
* The storage type the index is built on.
*
*
* optional .storage_onestore_v3.Document.Storage storage = 5 [default = DISK];
* @param value The storage to set.
* @return This builder for chaining.
*/
public Builder setStorage(com.google.apphosting.api.search.DocumentPb.Document.Storage value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
storage_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The storage type the index is built on.
*
*
* optional .storage_onestore_v3.Document.Storage storage = 5 [default = DISK];
* @return This builder for chaining.
*/
public Builder clearStorage() {
bitField0_ = (bitField0_ & ~0x00000020);
storage_ = 0;
onChanged();
return this;
}
private java.util.List facet_ =
java.util.Collections.emptyList();
private void ensureFacetIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
facet_ = new java.util.ArrayList(facet_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.Facet, com.google.apphosting.api.search.DocumentPb.Facet.Builder, com.google.apphosting.api.search.DocumentPb.FacetOrBuilder> facetBuilder_;
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public java.util.List getFacetList() {
if (facetBuilder_ == null) {
return java.util.Collections.unmodifiableList(facet_);
} else {
return facetBuilder_.getMessageList();
}
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public int getFacetCount() {
if (facetBuilder_ == null) {
return facet_.size();
} else {
return facetBuilder_.getCount();
}
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public com.google.apphosting.api.search.DocumentPb.Facet getFacet(int index) {
if (facetBuilder_ == null) {
return facet_.get(index);
} else {
return facetBuilder_.getMessage(index);
}
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder setFacet(
int index, com.google.apphosting.api.search.DocumentPb.Facet value) {
if (facetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetIsMutable();
facet_.set(index, value);
onChanged();
} else {
facetBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder setFacet(
int index, com.google.apphosting.api.search.DocumentPb.Facet.Builder builderForValue) {
if (facetBuilder_ == null) {
ensureFacetIsMutable();
facet_.set(index, builderForValue.build());
onChanged();
} else {
facetBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder addFacet(com.google.apphosting.api.search.DocumentPb.Facet value) {
if (facetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetIsMutable();
facet_.add(value);
onChanged();
} else {
facetBuilder_.addMessage(value);
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder addFacet(
int index, com.google.apphosting.api.search.DocumentPb.Facet value) {
if (facetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetIsMutable();
facet_.add(index, value);
onChanged();
} else {
facetBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder addFacet(
com.google.apphosting.api.search.DocumentPb.Facet.Builder builderForValue) {
if (facetBuilder_ == null) {
ensureFacetIsMutable();
facet_.add(builderForValue.build());
onChanged();
} else {
facetBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder addFacet(
int index, com.google.apphosting.api.search.DocumentPb.Facet.Builder builderForValue) {
if (facetBuilder_ == null) {
ensureFacetIsMutable();
facet_.add(index, builderForValue.build());
onChanged();
} else {
facetBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder addAllFacet(
java.lang.Iterable extends com.google.apphosting.api.search.DocumentPb.Facet> values) {
if (facetBuilder_ == null) {
ensureFacetIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, facet_);
onChanged();
} else {
facetBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder clearFacet() {
if (facetBuilder_ == null) {
facet_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
facetBuilder_.clear();
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public Builder removeFacet(int index) {
if (facetBuilder_ == null) {
ensureFacetIsMutable();
facet_.remove(index);
onChanged();
} else {
facetBuilder_.remove(index);
}
return this;
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public com.google.apphosting.api.search.DocumentPb.Facet.Builder getFacetBuilder(
int index) {
return getFacetFieldBuilder().getBuilder(index);
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public com.google.apphosting.api.search.DocumentPb.FacetOrBuilder getFacetOrBuilder(
int index) {
if (facetBuilder_ == null) {
return facet_.get(index); } else {
return facetBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public java.util.List extends com.google.apphosting.api.search.DocumentPb.FacetOrBuilder>
getFacetOrBuilderList() {
if (facetBuilder_ != null) {
return facetBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(facet_);
}
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public com.google.apphosting.api.search.DocumentPb.Facet.Builder addFacetBuilder() {
return getFacetFieldBuilder().addBuilder(
com.google.apphosting.api.search.DocumentPb.Facet.getDefaultInstance());
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public com.google.apphosting.api.search.DocumentPb.Facet.Builder addFacetBuilder(
int index) {
return getFacetFieldBuilder().addBuilder(
index, com.google.apphosting.api.search.DocumentPb.Facet.getDefaultInstance());
}
/**
*
* The set of facets/categories, the document belongs to.
*
*
* repeated .storage_onestore_v3.Facet facet = 8;
*/
public java.util.List
getFacetBuilderList() {
return getFacetFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.Facet, com.google.apphosting.api.search.DocumentPb.Facet.Builder, com.google.apphosting.api.search.DocumentPb.FacetOrBuilder>
getFacetFieldBuilder() {
if (facetBuilder_ == null) {
facetBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.api.search.DocumentPb.Facet, com.google.apphosting.api.search.DocumentPb.Facet.Builder, com.google.apphosting.api.search.DocumentPb.FacetOrBuilder>(
facet_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
facet_ = null;
}
return facetBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:storage_onestore_v3.Document)
}
// @@protoc_insertion_point(class_scope:storage_onestore_v3.Document)
private static final com.google.apphosting.api.search.DocumentPb.Document DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.api.search.DocumentPb.Document();
}
public static com.google.apphosting.api.search.DocumentPb.Document getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Document parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.api.search.DocumentPb.Document getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_FieldValue_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_FieldValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_FieldValue_Geo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_FieldValue_Geo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_Field_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_Field_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_FieldTypes_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_FieldTypes_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_IndexShardSettings_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_IndexShardSettings_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_IndexMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_IndexMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_FacetValue_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_FacetValue_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_Facet_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_Facet_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_DocumentMetadata_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_DocumentMetadata_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_storage_onestore_v3_Document_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_storage_onestore_v3_Document_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\016document.proto\022\023storage_onestore_v3\"\351\002" +
"\n\nFieldValue\022?\n\004type\030\001 \001(\0162+.storage_one" +
"store_v3.FieldValue.ContentType:\004TEXT\022\024\n" +
"\010language\030\002 \001(\t:\002en\022\024\n\014string_value\030\003 \001(" +
"\t\0220\n\003geo\030\004 \001(\n2#.storage_onestore_v3.Fie" +
"ldValue.Geo\022\024\n\014vector_value\030\007 \003(\001\032\037\n\003Geo" +
"\022\013\n\003lat\030\005 \002(\001\022\013\n\003lng\030\006 \002(\001\"\204\001\n\013ContentTy" +
"pe\022\010\n\004TEXT\020\000\022\010\n\004HTML\020\001\022\010\n\004ATOM\020\002\022\010\n\004DATE" +
"\020\003\022\n\n\006NUMBER\020\004\022\007\n\003GEO\020\005\022\026\n\022UNTOKENIZED_P" +
"REFIX\020\006\022\024\n\020TOKENIZED_PREFIX\020\007\022\n\n\006VECTOR\020" +
"\010\"E\n\005Field\022\014\n\004name\030\001 \002(\t\022.\n\005value\030\002 \002(\0132" +
"\037.storage_onestore_v3.FieldValue\"U\n\nFiel" +
"dTypes\022\014\n\004name\030\001 \002(\t\0229\n\004type\030\002 \003(\0162+.sto" +
"rage_onestore_v3.FieldValue.ContentType\"" +
"\203\001\n\022IndexShardSettings\022\027\n\017prev_num_shard" +
"s\030\001 \003(\005\022\025\n\nnum_shards\030\002 \002(\005:\0011\022$\n\034prev_n" +
"um_shards_search_false\030\003 \003(\005\022\027\n\rlocal_re" +
"plica\030\004 \001(\t:\000\"\321\005\n\rIndexMetadata\022-\n\036is_ov" +
"er_field_number_threshold\030\001 \001(\010:\005false\022E" +
"\n\024index_shard_settings\030\002 \001(\0132\'.storage_o" +
"nestore_v3.IndexShardSettings\022J\n\013index_s" +
"tate\030\003 \001(\0162-.storage_onestore_v3.IndexMe" +
"tadata.IndexState:\006ACTIVE\022\031\n\021index_delet" +
"e_time\030\004 \001(\003\022\034\n\024max_index_size_bytes\030\005 \001" +
"(\003\022Q\n\020replica_deletion\030\006 \003(\01327.storage_o" +
"nestore_v3.IndexMetadata.IndexDeletionDe" +
"tails\032>\n\016DeletionStatus\022\024\n\014started_time\030" +
"\003 \001(\003\022\026\n\016completed_time\030\004 \001(\003\032\370\001\n\024IndexD" +
"eletionDetails\022\024\n\014replica_name\030\001 \002(\t\022C\n\010" +
"precheck\030\002 \001(\01321.storage_onestore_v3.Ind" +
"exMetadata.DeletionStatus\022A\n\006st_bti\030\003 \001(" +
"\01321.storage_onestore_v3.IndexMetadata.De" +
"letionStatus\022B\n\007ms_docs\030\004 \001(\01321.storage_" +
"onestore_v3.IndexMetadata.DeletionStatus" +
"\"7\n\nIndexState\022\n\n\006ACTIVE\020\000\022\020\n\014SOFT_DELET" +
"ED\020\001\022\013\n\007PURGING\020\002\"\210\001\n\nFacetValue\022?\n\004type" +
"\030\001 \001(\0162+.storage_onestore_v3.FacetValue." +
"ContentType:\004ATOM\022\024\n\014string_value\030\003 \001(\t\"" +
"#\n\013ContentType\022\010\n\004ATOM\020\002\022\n\n\006NUMBER\020\004\"E\n\005" +
"Facet\022\014\n\004name\030\001 \002(\t\022.\n\005value\030\002 \002(\0132\037.sto" +
"rage_onestore_v3.FacetValue\"A\n\020DocumentM" +
"etadata\022\017\n\007version\030\001 \001(\003\022\034\n\024committed_st" +
"_version\030\002 \001(\003\"\345\002\n\010Document\022\n\n\002id\030\001 \001(\t\022" +
"\024\n\010language\030\002 \001(\t:\002en\022)\n\005field\030\003 \003(\0132\032.s" +
"torage_onestore_v3.Field\022\020\n\010order_id\030\004 \001" +
"(\005\022N\n\017order_id_source\030\006 \001(\0162+.storage_on" +
"estore_v3.Document.OrderIdSource:\010SUPPLI" +
"ED\022<\n\007storage\030\005 \001(\0162%.storage_onestore_v" +
"3.Document.Storage:\004DISK\022)\n\005facet\030\010 \003(\0132" +
"\032.storage_onestore_v3.Facet\",\n\rOrderIdSo" +
"urce\022\r\n\tDEFAULTED\020\000\022\014\n\010SUPPLIED\020\001\"\023\n\007Sto" +
"rage\022\010\n\004DISK\020\000B.\n com.google.apphosting." +
"api.searchB\nDocumentPb"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_storage_onestore_v3_FieldValue_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_storage_onestore_v3_FieldValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_FieldValue_descriptor,
new java.lang.String[] { "Type", "Language", "StringValue", "Geo", "VectorValue", });
internal_static_storage_onestore_v3_FieldValue_Geo_descriptor =
internal_static_storage_onestore_v3_FieldValue_descriptor.getNestedTypes().get(0);
internal_static_storage_onestore_v3_FieldValue_Geo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_FieldValue_Geo_descriptor,
new java.lang.String[] { "Lat", "Lng", });
internal_static_storage_onestore_v3_Field_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_storage_onestore_v3_Field_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_Field_descriptor,
new java.lang.String[] { "Name", "Value", });
internal_static_storage_onestore_v3_FieldTypes_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_storage_onestore_v3_FieldTypes_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_FieldTypes_descriptor,
new java.lang.String[] { "Name", "Type", });
internal_static_storage_onestore_v3_IndexShardSettings_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_storage_onestore_v3_IndexShardSettings_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_IndexShardSettings_descriptor,
new java.lang.String[] { "PrevNumShards", "NumShards", "PrevNumShardsSearchFalse", "LocalReplica", });
internal_static_storage_onestore_v3_IndexMetadata_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_storage_onestore_v3_IndexMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_IndexMetadata_descriptor,
new java.lang.String[] { "IsOverFieldNumberThreshold", "IndexShardSettings", "IndexState", "IndexDeleteTime", "MaxIndexSizeBytes", "ReplicaDeletion", });
internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_descriptor =
internal_static_storage_onestore_v3_IndexMetadata_descriptor.getNestedTypes().get(0);
internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_IndexMetadata_DeletionStatus_descriptor,
new java.lang.String[] { "StartedTime", "CompletedTime", });
internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_descriptor =
internal_static_storage_onestore_v3_IndexMetadata_descriptor.getNestedTypes().get(1);
internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_IndexMetadata_IndexDeletionDetails_descriptor,
new java.lang.String[] { "ReplicaName", "Precheck", "StBti", "MsDocs", });
internal_static_storage_onestore_v3_FacetValue_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_storage_onestore_v3_FacetValue_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_FacetValue_descriptor,
new java.lang.String[] { "Type", "StringValue", });
internal_static_storage_onestore_v3_Facet_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_storage_onestore_v3_Facet_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_Facet_descriptor,
new java.lang.String[] { "Name", "Value", });
internal_static_storage_onestore_v3_DocumentMetadata_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_storage_onestore_v3_DocumentMetadata_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_DocumentMetadata_descriptor,
new java.lang.String[] { "Version", "CommittedStVersion", });
internal_static_storage_onestore_v3_Document_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_storage_onestore_v3_Document_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_storage_onestore_v3_Document_descriptor,
new java.lang.String[] { "Id", "Language", "Field", "OrderId", "OrderIdSource", "Storage", "Facet", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy