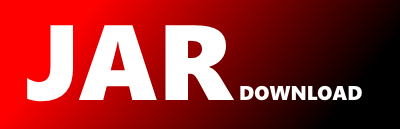
com.google.apphosting.base.protos.AppinfoPb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: appinfo.proto
package com.google.apphosting.base.protos;
public final class AppinfoPb {
private AppinfoPb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface HandlerOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.Handler)
com.google.protobuf.MessageOrBuilder {
/**
*
* Not really optional.
*
*
* optional int32 type = 1 [default = 1];
* @return Whether the type field is set.
*/
boolean hasType();
/**
*
* Not really optional.
*
*
* optional int32 type = 1 [default = 1];
* @return The type.
*/
int getType();
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return Whether the path field is set.
*/
boolean hasPath();
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return The path.
*/
java.lang.String getPath();
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
}
/**
*
* next-id: 17
*
*
* Protobuf type {@code java.apphosting.Handler}
*/
public static final class Handler extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.Handler)
HandlerOrBuilder {
private static final long serialVersionUID = 0L;
// Use Handler.newBuilder() to construct.
private Handler(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Handler() {
type_ = 1;
path_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Handler();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_Handler_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_Handler_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.Handler.class, com.google.apphosting.base.protos.AppinfoPb.Handler.Builder.class);
}
/**
*
* Specifies what sort of endpoint this is, which indicates how to
* handle it. Static file and cgi-bin are good examples. Some options
* may be language specific.
*
*
* Protobuf enum {@code java.apphosting.Handler.HANDLERTYPE}
*/
public enum HANDLERTYPE
implements com.google.protobuf.ProtocolMessageEnum {
/**
* STATIC = 0;
*/
STATIC(0),
/**
* CGI_BIN = 1;
*/
CGI_BIN(1),
/**
*
* SERVLET = 2; // DEPRECATED
*
*
* SHUTDOWN = 3;
*/
SHUTDOWN(3),
;
/**
* STATIC = 0;
*/
public static final int STATIC_VALUE = 0;
/**
* CGI_BIN = 1;
*/
public static final int CGI_BIN_VALUE = 1;
/**
*
* SERVLET = 2; // DEPRECATED
*
*
* SHUTDOWN = 3;
*/
public static final int SHUTDOWN_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static HANDLERTYPE valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static HANDLERTYPE forNumber(int value) {
switch (value) {
case 0: return STATIC;
case 1: return CGI_BIN;
case 3: return SHUTDOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
HANDLERTYPE> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public HANDLERTYPE findValueByNumber(int number) {
return HANDLERTYPE.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.Handler.getDescriptor().getEnumTypes().get(0);
}
private static final HANDLERTYPE[] VALUES = values();
public static HANDLERTYPE valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private HANDLERTYPE(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.Handler.HANDLERTYPE)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_ = 1;
/**
*
* Not really optional.
*
*
* optional int32 type = 1 [default = 1];
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Not really optional.
*
*
* optional int32 type = 1 [default = 1];
* @return The type.
*/
@java.lang.Override
public int getType() {
return type_;
}
public static final int PATH_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object path_ = "";
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return Whether the path field is set.
*/
@java.lang.Override
public boolean hasPath() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasPath()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, path_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, path_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.AppinfoPb.Handler)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.AppinfoPb.Handler other = (com.google.apphosting.base.protos.AppinfoPb.Handler) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (getType()
!= other.getType()) return false;
}
if (hasPath() != other.hasPath()) return false;
if (hasPath()) {
if (!getPath()
.equals(other.getPath())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType();
}
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.AppinfoPb.Handler prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* next-id: 17
*
*
* Protobuf type {@code java.apphosting.Handler}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.Handler)
com.google.apphosting.base.protos.AppinfoPb.HandlerOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_Handler_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_Handler_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.Handler.class, com.google.apphosting.base.protos.AppinfoPb.Handler.Builder.class);
}
// Construct using com.google.apphosting.base.protos.AppinfoPb.Handler.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
type_ = 1;
path_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_Handler_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.Handler getDefaultInstanceForType() {
return com.google.apphosting.base.protos.AppinfoPb.Handler.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.Handler build() {
com.google.apphosting.base.protos.AppinfoPb.Handler result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.Handler buildPartial() {
com.google.apphosting.base.protos.AppinfoPb.Handler result = new com.google.apphosting.base.protos.AppinfoPb.Handler(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.base.protos.AppinfoPb.Handler result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.type_ = type_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.path_ = path_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.AppinfoPb.Handler) {
return mergeFrom((com.google.apphosting.base.protos.AppinfoPb.Handler)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.AppinfoPb.Handler other) {
if (other == com.google.apphosting.base.protos.AppinfoPb.Handler.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasPath()) {
path_ = other.path_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasPath()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
type_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
path_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int type_ = 1;
/**
*
* Not really optional.
*
*
* optional int32 type = 1 [default = 1];
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Not really optional.
*
*
* optional int32 type = 1 [default = 1];
* @return The type.
*/
@java.lang.Override
public int getType() {
return type_;
}
/**
*
* Not really optional.
*
*
* optional int32 type = 1 [default = 1];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(int value) {
type_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Not really optional.
*
*
* optional int32 type = 1 [default = 1];
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 1;
onChanged();
return this;
}
private java.lang.Object path_ = "";
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return Whether the path field is set.
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
path_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* might be a file or a class name
*
*
* required string path = 2;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
path_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.Handler)
}
// @@protoc_insertion_point(class_scope:java.apphosting.Handler)
private static final com.google.apphosting.base.protos.AppinfoPb.Handler DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.AppinfoPb.Handler();
}
public static com.google.apphosting.base.protos.AppinfoPb.Handler getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Handler parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.Handler getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AppInfoOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.AppInfo)
com.google.protobuf.MessageOrBuilder {
/**
* required string app_id = 1;
* @return Whether the appId field is set.
*/
boolean hasAppId();
/**
* required string app_id = 1;
* @return The appId.
*/
java.lang.String getAppId();
/**
* required string app_id = 1;
* @return The bytes for appId.
*/
com.google.protobuf.ByteString
getAppIdBytes();
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return Whether the versionId field is set.
*/
boolean hasVersionId();
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return The versionId.
*/
java.lang.String getVersionId();
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return The bytes for versionId.
*/
com.google.protobuf.ByteString
getVersionIdBytes();
/**
*
* Time at which this AppInfo was written, in microseconds. This field should
* only be modified by the app_config_service jobs, as it is critical to
* operation of both schedulers:
* 1. CloneScheduler uses timestamp_usec to determine whether the AppInfo is
* newer than the GlobalConfig or vice versa (e.g. when determining instance
* class);
* 2. AppMaster2 uses timestamp_usec to determine whether an AppInfo was
* recently written (i.e. when deciding whether a config update warrants a
* zero-load ServingState expiration extension).
*
*
* optional int64 timestamp_usec = 90;
* @return Whether the timestampUsec field is set.
*/
boolean hasTimestampUsec();
/**
*
* Time at which this AppInfo was written, in microseconds. This field should
* only be modified by the app_config_service jobs, as it is critical to
* operation of both schedulers:
* 1. CloneScheduler uses timestamp_usec to determine whether the AppInfo is
* newer than the GlobalConfig or vice versa (e.g. when determining instance
* class);
* 2. AppMaster2 uses timestamp_usec to determine whether an AppInfo was
* recently written (i.e. when deciding whether a config update warrants a
* zero-load ServingState expiration extension).
*
*
* optional int64 timestamp_usec = 90;
* @return The timestampUsec.
*/
long getTimestampUsec();
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return Whether the runtimeId field is set.
*/
boolean hasRuntimeId();
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return The runtimeId.
*/
java.lang.String getRuntimeId();
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return The bytes for runtimeId.
*/
com.google.protobuf.ByteString
getRuntimeIdBytes();
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return Whether the runtimeSpecialization field is set.
*/
boolean hasRuntimeSpecialization();
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return The runtimeSpecialization.
*/
java.lang.String getRuntimeSpecialization();
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return The bytes for runtimeSpecialization.
*/
com.google.protobuf.ByteString
getRuntimeSpecializationBytes();
/**
*
* Enable App Engine APIs on Second Generation go/gen2-wormhole-phase-1
*
*
* optional bool gen2_app_engine_apis = 102;
* @return Whether the gen2AppEngineApis field is set.
*/
boolean hasGen2AppEngineApis();
/**
*
* Enable App Engine APIs on Second Generation go/gen2-wormhole-phase-1
*
*
* optional bool gen2_app_engine_apis = 102;
* @return The gen2AppEngineApis.
*/
boolean getGen2AppEngineApis();
/**
*
* The instance class of this app version. Taken from version_config (for
* frontends) or server_config (for backends). Currently populated only in
* AppInfoLite messages (i.e. is_appinfo_lite is true) via ReduceAppInfo. This
* field is used by Clone Scheduler when scheduling newly deployed versions.
* TODO: Consider populating this field directly, in Zeus.
*
*
* optional int32 instance_class = 91;
* @return Whether the instanceClass field is set.
*/
boolean hasInstanceClass();
/**
*
* The instance class of this app version. Taken from version_config (for
* frontends) or server_config (for backends). Currently populated only in
* AppInfoLite messages (i.e. is_appinfo_lite is true) via ReduceAppInfo. This
* field is used by Clone Scheduler when scheduling newly deployed versions.
* TODO: Consider populating this field directly, in Zeus.
*
*
* optional int32 instance_class = 91;
* @return The instanceClass.
*/
int getInstanceClass();
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return Whether the apiVersion field is set.
*/
boolean hasApiVersion();
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return The apiVersion.
*/
java.lang.String getApiVersion();
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return The bytes for apiVersion.
*/
com.google.protobuf.ByteString
getApiVersionBytes();
/**
* repeated group File = 6 { ... }
*/
java.util.List
getFileList();
/**
* repeated group File = 6 { ... }
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File getFile(int index);
/**
* repeated group File = 6 { ... }
*/
int getFileCount();
/**
* repeated group File = 6 { ... }
*/
java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder>
getFileOrBuilderList();
/**
* repeated group File = 6 { ... }
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder getFileOrBuilder(
int index);
/**
* repeated group Blob = 16 { ... }
*/
java.util.List
getBlobList();
/**
* repeated group Blob = 16 { ... }
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob getBlob(int index);
/**
* repeated group Blob = 16 { ... }
*/
int getBlobCount();
/**
* repeated group Blob = 16 { ... }
*/
java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder>
getBlobOrBuilderList();
/**
* repeated group Blob = 16 { ... }
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder getBlobOrBuilder(
int index);
/**
* repeated group URLMap = 9 { ... }
*/
java.util.List
getURLMapList();
/**
* repeated group URLMap = 9 { ... }
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap getURLMap(int index);
/**
* repeated group URLMap = 9 { ... }
*/
int getURLMapCount();
/**
* repeated group URLMap = 9 { ... }
*/
java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder>
getURLMapOrBuilderList();
/**
* repeated group URLMap = 9 { ... }
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder getURLMapOrBuilder(
int index);
/**
* optional group DerivedFiles = 39 { ... }
* @return Whether the derivedfiles field is set.
*/
boolean hasDerivedFiles();
/**
* optional group DerivedFiles = 39 { ... }
* @return The derivedfiles.
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles getDerivedFiles();
/**
* optional group DerivedFiles = 39 { ... }
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFilesOrBuilder getDerivedFilesOrBuilder();
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
java.util.List
getEnvironmentVariableList();
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable getEnvironmentVariable(int index);
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
int getEnvironmentVariableCount();
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder>
getEnvironmentVariableOrBuilderList();
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder getEnvironmentVariableOrBuilder(
int index);
}
/**
*
* Description of an application version. In the eyes of the
* AppServer, each version is basically a different app.
* AppInfos are immutable--they are not to be changed once created. If you
* need to add per-version data that can be changed while the version is
* running, use PerVersionConfig.
* Next Tag: 107
*
*
* Protobuf type {@code java.apphosting.AppInfo}
*/
public static final class AppInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.AppInfo)
AppInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use AppInfo.newBuilder() to construct.
private AppInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AppInfo() {
appId_ = "";
versionId_ = "1";
runtimeId_ = "python";
runtimeSpecialization_ = "";
apiVersion_ = "";
file_ = java.util.Collections.emptyList();
blob_ = java.util.Collections.emptyList();
uRLMap_ = java.util.Collections.emptyList();
environmentVariable_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AppInfo();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Builder.class);
}
public interface FileOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.AppInfo.File)
com.google.protobuf.MessageOrBuilder {
/**
* required string sha1_hash = 7;
* @return Whether the sha1Hash field is set.
*/
boolean hasSha1Hash();
/**
* required string sha1_hash = 7;
* @return The sha1Hash.
*/
java.lang.String getSha1Hash();
/**
* required string sha1_hash = 7;
* @return The bytes for sha1Hash.
*/
com.google.protobuf.ByteString
getSha1HashBytes();
/**
* required string path = 8;
* @return Whether the path field is set.
*/
boolean hasPath();
/**
* required string path = 8;
* @return The path.
*/
java.lang.String getPath();
/**
* required string path = 8;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
*
* file size in bytes
*
*
* required int64 file_size = 14;
* @return Whether the fileSize field is set.
*/
boolean hasFileSize();
/**
*
* file size in bytes
*
*
* required int64 file_size = 14;
* @return The fileSize.
*/
long getFileSize();
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return Whether the mimeType field is set.
*/
boolean hasMimeType();
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return The mimeType.
*/
java.lang.String getMimeType();
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return The bytes for mimeType.
*/
com.google.protobuf.ByteString
getMimeTypeBytes();
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return Whether the sourceUrl field is set.
*/
boolean hasSourceUrl();
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return The sourceUrl.
*/
java.lang.String getSourceUrl();
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return The bytes for sourceUrl.
*/
com.google.protobuf.ByteString
getSourceUrlBytes();
}
/**
*
* This should not be specified in the app.cfg file; it is computed
* automatically by appcfg.
* All files will live in bigtable and will be indexed by their
* sha1_hash. An AppServer can recreate the app's directory structure
* from each file's 'path'. The AppServer will copy any required
* files from bigtable to a shared directory on the machine. It will
* then create a tree of symlinks to reflect the directory structure
* that the developer created. This common directory will allow sharing
* of common files between apps.
*
*
* Protobuf type {@code java.apphosting.AppInfo.File}
*/
public static final class File extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.AppInfo.File)
FileOrBuilder {
private static final long serialVersionUID = 0L;
// Use File.newBuilder() to construct.
private File(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private File() {
sha1Hash_ = "";
path_ = "";
mimeType_ = "";
sourceUrl_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new File();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_File_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_File_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder.class);
}
private int bitField0_;
public static final int SHA1_HASH_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object sha1Hash_ = "";
/**
* required string sha1_hash = 7;
* @return Whether the sha1Hash field is set.
*/
@java.lang.Override
public boolean hasSha1Hash() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string sha1_hash = 7;
* @return The sha1Hash.
*/
@java.lang.Override
public java.lang.String getSha1Hash() {
java.lang.Object ref = sha1Hash_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sha1Hash_ = s;
}
return s;
}
}
/**
* required string sha1_hash = 7;
* @return The bytes for sha1Hash.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSha1HashBytes() {
java.lang.Object ref = sha1Hash_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sha1Hash_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PATH_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object path_ = "";
/**
* required string path = 8;
* @return Whether the path field is set.
*/
@java.lang.Override
public boolean hasPath() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string path = 8;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
* required string path = 8;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILE_SIZE_FIELD_NUMBER = 14;
private long fileSize_ = 0L;
/**
*
* file size in bytes
*
*
* required int64 file_size = 14;
* @return Whether the fileSize field is set.
*/
@java.lang.Override
public boolean hasFileSize() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* file size in bytes
*
*
* required int64 file_size = 14;
* @return The fileSize.
*/
@java.lang.Override
public long getFileSize() {
return fileSize_;
}
public static final int MIME_TYPE_FIELD_NUMBER = 15;
@SuppressWarnings("serial")
private volatile java.lang.Object mimeType_ = "";
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return Whether the mimeType field is set.
*/
@java.lang.Override
public boolean hasMimeType() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return The mimeType.
*/
@java.lang.Override
public java.lang.String getMimeType() {
java.lang.Object ref = mimeType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mimeType_ = s;
}
return s;
}
}
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return The bytes for mimeType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMimeTypeBytes() {
java.lang.Object ref = mimeType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mimeType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_URL_FIELD_NUMBER = 71;
@SuppressWarnings("serial")
private volatile java.lang.Object sourceUrl_ = "";
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return Whether the sourceUrl field is set.
*/
@java.lang.Override
public boolean hasSourceUrl() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return The sourceUrl.
*/
@java.lang.Override
public java.lang.String getSourceUrl() {
java.lang.Object ref = sourceUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sourceUrl_ = s;
}
return s;
}
}
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return The bytes for sourceUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSourceUrlBytes() {
java.lang.Object ref = sourceUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasSha1Hash()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPath()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasFileSize()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, sha1Hash_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, path_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(14, fileSize_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, mimeType_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 71, sourceUrl_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, sha1Hash_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, path_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(14, fileSize_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, mimeType_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(71, sourceUrl_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.File)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File other = (com.google.apphosting.base.protos.AppinfoPb.AppInfo.File) obj;
if (hasSha1Hash() != other.hasSha1Hash()) return false;
if (hasSha1Hash()) {
if (!getSha1Hash()
.equals(other.getSha1Hash())) return false;
}
if (hasPath() != other.hasPath()) return false;
if (hasPath()) {
if (!getPath()
.equals(other.getPath())) return false;
}
if (hasFileSize() != other.hasFileSize()) return false;
if (hasFileSize()) {
if (getFileSize()
!= other.getFileSize()) return false;
}
if (hasMimeType() != other.hasMimeType()) return false;
if (hasMimeType()) {
if (!getMimeType()
.equals(other.getMimeType())) return false;
}
if (hasSourceUrl() != other.hasSourceUrl()) return false;
if (hasSourceUrl()) {
if (!getSourceUrl()
.equals(other.getSourceUrl())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSha1Hash()) {
hash = (37 * hash) + SHA1_HASH_FIELD_NUMBER;
hash = (53 * hash) + getSha1Hash().hashCode();
}
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
if (hasFileSize()) {
hash = (37 * hash) + FILE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFileSize());
}
if (hasMimeType()) {
hash = (37 * hash) + MIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getMimeType().hashCode();
}
if (hasSourceUrl()) {
hash = (37 * hash) + SOURCE_URL_FIELD_NUMBER;
hash = (53 * hash) + getSourceUrl().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.AppinfoPb.AppInfo.File prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* This should not be specified in the app.cfg file; it is computed
* automatically by appcfg.
* All files will live in bigtable and will be indexed by their
* sha1_hash. An AppServer can recreate the app's directory structure
* from each file's 'path'. The AppServer will copy any required
* files from bigtable to a shared directory on the machine. It will
* then create a tree of symlinks to reflect the directory structure
* that the developer created. This common directory will allow sharing
* of common files between apps.
*
*
* Protobuf type {@code java.apphosting.AppInfo.File}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.AppInfo.File)
com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_File_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_File_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder.class);
}
// Construct using com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
sha1Hash_ = "";
path_ = "";
fileSize_ = 0L;
mimeType_ = "";
sourceUrl_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_File_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File getDefaultInstanceForType() {
return com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File build() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File buildPartial() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File result = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.File(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.base.protos.AppinfoPb.AppInfo.File result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.sha1Hash_ = sha1Hash_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.path_ = path_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.fileSize_ = fileSize_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.mimeType_ = mimeType_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.sourceUrl_ = sourceUrl_;
to_bitField0_ |= 0x00000010;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.File) {
return mergeFrom((com.google.apphosting.base.protos.AppinfoPb.AppInfo.File)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.AppinfoPb.AppInfo.File other) {
if (other == com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.getDefaultInstance()) return this;
if (other.hasSha1Hash()) {
sha1Hash_ = other.sha1Hash_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasPath()) {
path_ = other.path_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasFileSize()) {
setFileSize(other.getFileSize());
}
if (other.hasMimeType()) {
mimeType_ = other.mimeType_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasSourceUrl()) {
sourceUrl_ = other.sourceUrl_;
bitField0_ |= 0x00000010;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasSha1Hash()) {
return false;
}
if (!hasPath()) {
return false;
}
if (!hasFileSize()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 58: {
sha1Hash_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 58
case 66: {
path_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 66
case 112: {
fileSize_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 112
case 122: {
mimeType_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 122
case 570: {
sourceUrl_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 570
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object sha1Hash_ = "";
/**
* required string sha1_hash = 7;
* @return Whether the sha1Hash field is set.
*/
public boolean hasSha1Hash() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string sha1_hash = 7;
* @return The sha1Hash.
*/
public java.lang.String getSha1Hash() {
java.lang.Object ref = sha1Hash_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sha1Hash_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string sha1_hash = 7;
* @return The bytes for sha1Hash.
*/
public com.google.protobuf.ByteString
getSha1HashBytes() {
java.lang.Object ref = sha1Hash_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sha1Hash_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string sha1_hash = 7;
* @param value The sha1Hash to set.
* @return This builder for chaining.
*/
public Builder setSha1Hash(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
sha1Hash_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required string sha1_hash = 7;
* @return This builder for chaining.
*/
public Builder clearSha1Hash() {
sha1Hash_ = getDefaultInstance().getSha1Hash();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* required string sha1_hash = 7;
* @param value The bytes for sha1Hash to set.
* @return This builder for chaining.
*/
public Builder setSha1HashBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
sha1Hash_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object path_ = "";
/**
* required string path = 8;
* @return Whether the path field is set.
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string path = 8;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string path = 8;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string path = 8;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
path_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required string path = 8;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* required string path = 8;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
path_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private long fileSize_ ;
/**
*
* file size in bytes
*
*
* required int64 file_size = 14;
* @return Whether the fileSize field is set.
*/
@java.lang.Override
public boolean hasFileSize() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* file size in bytes
*
*
* required int64 file_size = 14;
* @return The fileSize.
*/
@java.lang.Override
public long getFileSize() {
return fileSize_;
}
/**
*
* file size in bytes
*
*
* required int64 file_size = 14;
* @param value The fileSize to set.
* @return This builder for chaining.
*/
public Builder setFileSize(long value) {
fileSize_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* file size in bytes
*
*
* required int64 file_size = 14;
* @return This builder for chaining.
*/
public Builder clearFileSize() {
bitField0_ = (bitField0_ & ~0x00000004);
fileSize_ = 0L;
onChanged();
return this;
}
private java.lang.Object mimeType_ = "";
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return Whether the mimeType field is set.
*/
public boolean hasMimeType() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return The mimeType.
*/
public java.lang.String getMimeType() {
java.lang.Object ref = mimeType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mimeType_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return The bytes for mimeType.
*/
public com.google.protobuf.ByteString
getMimeTypeBytes() {
java.lang.Object ref = mimeType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mimeType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @param value The mimeType to set.
* @return This builder for chaining.
*/
public Builder setMimeType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
mimeType_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @return This builder for chaining.
*/
public Builder clearMimeType() {
mimeType_ = getDefaultInstance().getMimeType();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* If non-empty, we will use this as the mime type. If empty, we will derive
* the mime type from the file extension of 'path'.
* N.B.(jonmac): This field is deprecated. Instead, static files should be
* included in Blob below.
*
*
* optional string mime_type = 15 [default = ""];
* @param value The bytes for mimeType to set.
* @return This builder for chaining.
*/
public Builder setMimeTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
mimeType_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object sourceUrl_ = "";
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return Whether the sourceUrl field is set.
*/
public boolean hasSourceUrl() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return The sourceUrl.
*/
public java.lang.String getSourceUrl() {
java.lang.Object ref = sourceUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
sourceUrl_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return The bytes for sourceUrl.
*/
public com.google.protobuf.ByteString
getSourceUrlBytes() {
java.lang.Object ref = sourceUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @param value The sourceUrl to set.
* @return This builder for chaining.
*/
public Builder setSourceUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
sourceUrl_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @return This builder for chaining.
*/
public Builder clearSourceUrl() {
sourceUrl_ = getDefaultInstance().getSourceUrl();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* The URL source where this file was fetched. This is populated
* for versions deployed through the Admin API. See
* http://cs/#piper///depot/google3/google/appengine/v1/deploy.proto?rcl=181115158&l=73
* for more details.
*
*
* optional string source_url = 71;
* @param value The bytes for sourceUrl to set.
* @return This builder for chaining.
*/
public Builder setSourceUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
sourceUrl_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.AppInfo.File)
}
// @@protoc_insertion_point(class_scope:java.apphosting.AppInfo.File)
private static final com.google.apphosting.base.protos.AppinfoPb.AppInfo.File DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.File();
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.File getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public File parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface BlobOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.AppInfo.Blob)
com.google.protobuf.MessageOrBuilder {
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return Whether the path field is set.
*/
boolean hasPath();
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return The path.
*/
java.lang.String getPath();
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return The bytes for path.
*/
com.google.protobuf.ByteString
getPathBytes();
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return Whether the mimeType field is set.
*/
boolean hasMimeType();
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return The mimeType.
*/
java.lang.String getMimeType();
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return The bytes for mimeType.
*/
com.google.protobuf.ByteString
getMimeTypeBytes();
}
/**
*
* This should not be specified in the app.cfg file; it is computed
* automatically by appcfg.
* All blobs will live in Blobstore. As opposed to files, they will not be
* made available to runtimes through a shared directory on the
* machine. Instead, blobs are for listing files that should be served as
* static content.
* When specifying blobs here, the expected procedure is that the client
* (e.g. appcfg) will first generate a BlobRef using AppConfigService. Then,
* the client will include the returned BlobRef here. Garbage collection of
* blobs will be handled by Prometheus and Blobstore. Sharing of common data
* between blobs (e.g. duplicate blobs) will be handled by Blobstore.
*
*
* Protobuf type {@code java.apphosting.AppInfo.Blob}
*/
public static final class Blob extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.AppInfo.Blob)
BlobOrBuilder {
private static final long serialVersionUID = 0L;
// Use Blob.newBuilder() to construct.
private Blob(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Blob() {
path_ = "";
mimeType_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Blob();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_Blob_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_Blob_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder.class);
}
private int bitField0_;
public static final int PATH_FIELD_NUMBER = 18;
@SuppressWarnings("serial")
private volatile java.lang.Object path_ = "";
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return Whether the path field is set.
*/
@java.lang.Override
public boolean hasPath() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return The path.
*/
@java.lang.Override
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return The bytes for path.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MIME_TYPE_FIELD_NUMBER = 35;
@SuppressWarnings("serial")
private volatile java.lang.Object mimeType_ = "";
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return Whether the mimeType field is set.
*/
@java.lang.Override
public boolean hasMimeType() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return The mimeType.
*/
@java.lang.Override
public java.lang.String getMimeType() {
java.lang.Object ref = mimeType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mimeType_ = s;
}
return s;
}
}
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return The bytes for mimeType.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMimeTypeBytes() {
java.lang.Object ref = mimeType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mimeType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasPath()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, path_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 35, mimeType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, path_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(35, mimeType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob other = (com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob) obj;
if (hasPath() != other.hasPath()) return false;
if (hasPath()) {
if (!getPath()
.equals(other.getPath())) return false;
}
if (hasMimeType() != other.hasMimeType()) return false;
if (hasMimeType()) {
if (!getMimeType()
.equals(other.getMimeType())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
if (hasMimeType()) {
hash = (37 * hash) + MIME_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getMimeType().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* This should not be specified in the app.cfg file; it is computed
* automatically by appcfg.
* All blobs will live in Blobstore. As opposed to files, they will not be
* made available to runtimes through a shared directory on the
* machine. Instead, blobs are for listing files that should be served as
* static content.
* When specifying blobs here, the expected procedure is that the client
* (e.g. appcfg) will first generate a BlobRef using AppConfigService. Then,
* the client will include the returned BlobRef here. Garbage collection of
* blobs will be handled by Prometheus and Blobstore. Sharing of common data
* between blobs (e.g. duplicate blobs) will be handled by Blobstore.
*
*
* Protobuf type {@code java.apphosting.AppInfo.Blob}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.AppInfo.Blob)
com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_Blob_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_Blob_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder.class);
}
// Construct using com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
path_ = "";
mimeType_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_Blob_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob getDefaultInstanceForType() {
return com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob build() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob buildPartial() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob result = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.path_ = path_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.mimeType_ = mimeType_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob) {
return mergeFrom((com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob other) {
if (other == com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.getDefaultInstance()) return this;
if (other.hasPath()) {
path_ = other.path_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasMimeType()) {
mimeType_ = other.mimeType_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasPath()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 146: {
path_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 146
case 282: {
mimeType_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 282
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object path_ = "";
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return Whether the path field is set.
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return The path.
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return The bytes for path.
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @param value The path to set.
* @return This builder for chaining.
*/
public Builder setPath(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
path_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @return This builder for chaining.
*/
public Builder clearPath() {
path_ = getDefaultInstance().getPath();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Used to support matching from Handler::path().
*
*
* required string path = 18;
* @param value The bytes for path to set.
* @return This builder for chaining.
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
path_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object mimeType_ = "";
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return Whether the mimeType field is set.
*/
public boolean hasMimeType() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return The mimeType.
*/
public java.lang.String getMimeType() {
java.lang.Object ref = mimeType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
mimeType_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return The bytes for mimeType.
*/
public com.google.protobuf.ByteString
getMimeTypeBytes() {
java.lang.Object ref = mimeType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mimeType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @param value The mimeType to set.
* @return This builder for chaining.
*/
public Builder setMimeType(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
mimeType_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @return This builder for chaining.
*/
public Builder clearMimeType() {
mimeType_ = getDefaultInstance().getMimeType();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* If non-empty, the mime_type of the blob.
*
*
* optional string mime_type = 35;
* @param value The bytes for mimeType to set.
* @return This builder for chaining.
*/
public Builder setMimeTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
mimeType_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.AppInfo.Blob)
}
// @@protoc_insertion_point(class_scope:java.apphosting.AppInfo.Blob)
private static final com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob();
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Blob parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface URLMapOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.AppInfo.URLMap)
com.google.protobuf.MessageOrBuilder {
/**
* optional string regex = 10;
* @return Whether the regex field is set.
*/
boolean hasRegex();
/**
* optional string regex = 10;
* @return The regex.
*/
java.lang.String getRegex();
/**
* optional string regex = 10;
* @return The bytes for regex.
*/
com.google.protobuf.ByteString
getRegexBytes();
/**
* optional .java.apphosting.Handler handler = 11;
* @return Whether the handler field is set.
*/
boolean hasHandler();
/**
* optional .java.apphosting.Handler handler = 11;
* @return The handler.
*/
com.google.apphosting.base.protos.AppinfoPb.Handler getHandler();
/**
* optional .java.apphosting.Handler handler = 11;
*/
com.google.apphosting.base.protos.AppinfoPb.HandlerOrBuilder getHandlerOrBuilder();
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return Whether the staticDir field is set.
*/
boolean hasStaticDir();
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return The staticDir.
*/
java.lang.String getStaticDir();
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return The bytes for staticDir.
*/
com.google.protobuf.ByteString
getStaticDirBytes();
/**
*
* If true, this URLMap entry does not match the request unless
* the file referenced by the handler also exists. If no such
* file exists, processing will continue with the next URLMap that
* matches the requested URL.
* This field is useful for applications that want to match a
* static file first (with a STATIC handler), but to continue on
* with dynamic processing if no static file matches.
* N.B.(schwardo): This functionality was added to facilitate
* dynamic translation of web.xml files for the Java runtime, and
* there are no plans to expose this to Python applications at
* this time.
*
*
* optional bool require_matching_file = 36 [default = false];
* @return Whether the requireMatchingFile field is set.
*/
boolean hasRequireMatchingFile();
/**
*
* If true, this URLMap entry does not match the request unless
* the file referenced by the handler also exists. If no such
* file exists, processing will continue with the next URLMap that
* matches the requested URL.
* This field is useful for applications that want to match a
* static file first (with a STATIC handler), but to continue on
* with dynamic processing if no static file matches.
* N.B.(schwardo): This functionality was added to facilitate
* dynamic translation of web.xml files for the Java runtime, and
* there are no plans to expose this to Python applications at
* this time.
*
*
* optional bool require_matching_file = 36 [default = false];
* @return The requireMatchingFile.
*/
boolean getRequireMatchingFile();
}
/**
*
* The regular expressions in this list are applied in order to the request
* URL until one matches, and then the associated handler will be used to
* process the request.
*
*
* Protobuf type {@code java.apphosting.AppInfo.URLMap}
*/
public static final class URLMap extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.AppInfo.URLMap)
URLMapOrBuilder {
private static final long serialVersionUID = 0L;
// Use URLMap.newBuilder() to construct.
private URLMap(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private URLMap() {
regex_ = "";
staticDir_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new URLMap();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_URLMap_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_URLMap_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder.class);
}
private int bitField0_;
public static final int REGEX_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private volatile java.lang.Object regex_ = "";
/**
* optional string regex = 10;
* @return Whether the regex field is set.
*/
@java.lang.Override
public boolean hasRegex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string regex = 10;
* @return The regex.
*/
@java.lang.Override
public java.lang.String getRegex() {
java.lang.Object ref = regex_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
regex_ = s;
}
return s;
}
}
/**
* optional string regex = 10;
* @return The bytes for regex.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRegexBytes() {
java.lang.Object ref = regex_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
regex_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HANDLER_FIELD_NUMBER = 11;
private com.google.apphosting.base.protos.AppinfoPb.Handler handler_;
/**
* optional .java.apphosting.Handler handler = 11;
* @return Whether the handler field is set.
*/
@java.lang.Override
public boolean hasHandler() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.Handler handler = 11;
* @return The handler.
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.Handler getHandler() {
return handler_ == null ? com.google.apphosting.base.protos.AppinfoPb.Handler.getDefaultInstance() : handler_;
}
/**
* optional .java.apphosting.Handler handler = 11;
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.HandlerOrBuilder getHandlerOrBuilder() {
return handler_ == null ? com.google.apphosting.base.protos.AppinfoPb.Handler.getDefaultInstance() : handler_;
}
public static final int STATIC_DIR_FIELD_NUMBER = 30;
@SuppressWarnings("serial")
private volatile java.lang.Object staticDir_ = "";
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return Whether the staticDir field is set.
*/
@java.lang.Override
public boolean hasStaticDir() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return The staticDir.
*/
@java.lang.Override
public java.lang.String getStaticDir() {
java.lang.Object ref = staticDir_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
staticDir_ = s;
}
return s;
}
}
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return The bytes for staticDir.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStaticDirBytes() {
java.lang.Object ref = staticDir_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
staticDir_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUIRE_MATCHING_FILE_FIELD_NUMBER = 36;
private boolean requireMatchingFile_ = false;
/**
*
* If true, this URLMap entry does not match the request unless
* the file referenced by the handler also exists. If no such
* file exists, processing will continue with the next URLMap that
* matches the requested URL.
* This field is useful for applications that want to match a
* static file first (with a STATIC handler), but to continue on
* with dynamic processing if no static file matches.
* N.B.(schwardo): This functionality was added to facilitate
* dynamic translation of web.xml files for the Java runtime, and
* there are no plans to expose this to Python applications at
* this time.
*
*
* optional bool require_matching_file = 36 [default = false];
* @return Whether the requireMatchingFile field is set.
*/
@java.lang.Override
public boolean hasRequireMatchingFile() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* If true, this URLMap entry does not match the request unless
* the file referenced by the handler also exists. If no such
* file exists, processing will continue with the next URLMap that
* matches the requested URL.
* This field is useful for applications that want to match a
* static file first (with a STATIC handler), but to continue on
* with dynamic processing if no static file matches.
* N.B.(schwardo): This functionality was added to facilitate
* dynamic translation of web.xml files for the Java runtime, and
* there are no plans to expose this to Python applications at
* this time.
*
*
* optional bool require_matching_file = 36 [default = false];
* @return The requireMatchingFile.
*/
@java.lang.Override
public boolean getRequireMatchingFile() {
return requireMatchingFile_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (hasHandler()) {
if (!getHandler().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, regex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(11, getHandler());
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, staticDir_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(36, requireMatchingFile_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, regex_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getHandler());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, staticDir_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(36, requireMatchingFile_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap other = (com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap) obj;
if (hasRegex() != other.hasRegex()) return false;
if (hasRegex()) {
if (!getRegex()
.equals(other.getRegex())) return false;
}
if (hasHandler() != other.hasHandler()) return false;
if (hasHandler()) {
if (!getHandler()
.equals(other.getHandler())) return false;
}
if (hasStaticDir() != other.hasStaticDir()) return false;
if (hasStaticDir()) {
if (!getStaticDir()
.equals(other.getStaticDir())) return false;
}
if (hasRequireMatchingFile() != other.hasRequireMatchingFile()) return false;
if (hasRequireMatchingFile()) {
if (getRequireMatchingFile()
!= other.getRequireMatchingFile()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRegex()) {
hash = (37 * hash) + REGEX_FIELD_NUMBER;
hash = (53 * hash) + getRegex().hashCode();
}
if (hasHandler()) {
hash = (37 * hash) + HANDLER_FIELD_NUMBER;
hash = (53 * hash) + getHandler().hashCode();
}
if (hasStaticDir()) {
hash = (37 * hash) + STATIC_DIR_FIELD_NUMBER;
hash = (53 * hash) + getStaticDir().hashCode();
}
if (hasRequireMatchingFile()) {
hash = (37 * hash) + REQUIRE_MATCHING_FILE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRequireMatchingFile());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The regular expressions in this list are applied in order to the request
* URL until one matches, and then the associated handler will be used to
* process the request.
*
*
* Protobuf type {@code java.apphosting.AppInfo.URLMap}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.AppInfo.URLMap)
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_URLMap_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_URLMap_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder.class);
}
// Construct using com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getHandlerFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
regex_ = "";
handler_ = null;
if (handlerBuilder_ != null) {
handlerBuilder_.dispose();
handlerBuilder_ = null;
}
staticDir_ = "";
requireMatchingFile_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_URLMap_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap getDefaultInstanceForType() {
return com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap build() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap buildPartial() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap result = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.regex_ = regex_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.handler_ = handlerBuilder_ == null
? handler_
: handlerBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.staticDir_ = staticDir_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.requireMatchingFile_ = requireMatchingFile_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap) {
return mergeFrom((com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap other) {
if (other == com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.getDefaultInstance()) return this;
if (other.hasRegex()) {
regex_ = other.regex_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasHandler()) {
mergeHandler(other.getHandler());
}
if (other.hasStaticDir()) {
staticDir_ = other.staticDir_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasRequireMatchingFile()) {
setRequireMatchingFile(other.getRequireMatchingFile());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (hasHandler()) {
if (!getHandler().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 82: {
regex_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 82
case 90: {
input.readMessage(
getHandlerFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 90
case 242: {
staticDir_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 242
case 288: {
requireMatchingFile_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 288
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object regex_ = "";
/**
* optional string regex = 10;
* @return Whether the regex field is set.
*/
public boolean hasRegex() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional string regex = 10;
* @return The regex.
*/
public java.lang.String getRegex() {
java.lang.Object ref = regex_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
regex_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string regex = 10;
* @return The bytes for regex.
*/
public com.google.protobuf.ByteString
getRegexBytes() {
java.lang.Object ref = regex_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
regex_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string regex = 10;
* @param value The regex to set.
* @return This builder for chaining.
*/
public Builder setRegex(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
regex_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* optional string regex = 10;
* @return This builder for chaining.
*/
public Builder clearRegex() {
regex_ = getDefaultInstance().getRegex();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* optional string regex = 10;
* @param value The bytes for regex to set.
* @return This builder for chaining.
*/
public Builder setRegexBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
regex_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.apphosting.base.protos.AppinfoPb.Handler handler_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.Handler, com.google.apphosting.base.protos.AppinfoPb.Handler.Builder, com.google.apphosting.base.protos.AppinfoPb.HandlerOrBuilder> handlerBuilder_;
/**
* optional .java.apphosting.Handler handler = 11;
* @return Whether the handler field is set.
*/
public boolean hasHandler() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .java.apphosting.Handler handler = 11;
* @return The handler.
*/
public com.google.apphosting.base.protos.AppinfoPb.Handler getHandler() {
if (handlerBuilder_ == null) {
return handler_ == null ? com.google.apphosting.base.protos.AppinfoPb.Handler.getDefaultInstance() : handler_;
} else {
return handlerBuilder_.getMessage();
}
}
/**
* optional .java.apphosting.Handler handler = 11;
*/
public Builder setHandler(com.google.apphosting.base.protos.AppinfoPb.Handler value) {
if (handlerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
handler_ = value;
} else {
handlerBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.Handler handler = 11;
*/
public Builder setHandler(
com.google.apphosting.base.protos.AppinfoPb.Handler.Builder builderForValue) {
if (handlerBuilder_ == null) {
handler_ = builderForValue.build();
} else {
handlerBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.Handler handler = 11;
*/
public Builder mergeHandler(com.google.apphosting.base.protos.AppinfoPb.Handler value) {
if (handlerBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
handler_ != null &&
handler_ != com.google.apphosting.base.protos.AppinfoPb.Handler.getDefaultInstance()) {
getHandlerBuilder().mergeFrom(value);
} else {
handler_ = value;
}
} else {
handlerBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* optional .java.apphosting.Handler handler = 11;
*/
public Builder clearHandler() {
bitField0_ = (bitField0_ & ~0x00000002);
handler_ = null;
if (handlerBuilder_ != null) {
handlerBuilder_.dispose();
handlerBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional .java.apphosting.Handler handler = 11;
*/
public com.google.apphosting.base.protos.AppinfoPb.Handler.Builder getHandlerBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getHandlerFieldBuilder().getBuilder();
}
/**
* optional .java.apphosting.Handler handler = 11;
*/
public com.google.apphosting.base.protos.AppinfoPb.HandlerOrBuilder getHandlerOrBuilder() {
if (handlerBuilder_ != null) {
return handlerBuilder_.getMessageOrBuilder();
} else {
return handler_ == null ?
com.google.apphosting.base.protos.AppinfoPb.Handler.getDefaultInstance() : handler_;
}
}
/**
* optional .java.apphosting.Handler handler = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.Handler, com.google.apphosting.base.protos.AppinfoPb.Handler.Builder, com.google.apphosting.base.protos.AppinfoPb.HandlerOrBuilder>
getHandlerFieldBuilder() {
if (handlerBuilder_ == null) {
handlerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.Handler, com.google.apphosting.base.protos.AppinfoPb.Handler.Builder, com.google.apphosting.base.protos.AppinfoPb.HandlerOrBuilder>(
getHandler(),
getParentForChildren(),
isClean());
handler_ = null;
}
return handlerBuilder_;
}
private java.lang.Object staticDir_ = "";
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return Whether the staticDir field is set.
*/
public boolean hasStaticDir() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return The staticDir.
*/
public java.lang.String getStaticDir() {
java.lang.Object ref = staticDir_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
staticDir_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return The bytes for staticDir.
*/
public com.google.protobuf.ByteString
getStaticDirBytes() {
java.lang.Object ref = staticDir_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
staticDir_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @param value The staticDir to set.
* @return This builder for chaining.
*/
public Builder setStaticDir(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
staticDir_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @return This builder for chaining.
*/
public Builder clearStaticDir() {
staticDir_ = getDefaultInstance().getStaticDir();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* For use in app.cfg only.
* This URLMap entry will be converted to serve static files from the
* specified directory, and we will additionally update StaticFileMap to
* consider all files in this directory as static files. More details at:
* http://g3doc/apphosting/g3doc/wiki-carryover/urlmap_change.md.
*
*
* optional string static_dir = 30;
* @param value The bytes for staticDir to set.
* @return This builder for chaining.
*/
public Builder setStaticDirBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
staticDir_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private boolean requireMatchingFile_ ;
/**
*
* If true, this URLMap entry does not match the request unless
* the file referenced by the handler also exists. If no such
* file exists, processing will continue with the next URLMap that
* matches the requested URL.
* This field is useful for applications that want to match a
* static file first (with a STATIC handler), but to continue on
* with dynamic processing if no static file matches.
* N.B.(schwardo): This functionality was added to facilitate
* dynamic translation of web.xml files for the Java runtime, and
* there are no plans to expose this to Python applications at
* this time.
*
*
* optional bool require_matching_file = 36 [default = false];
* @return Whether the requireMatchingFile field is set.
*/
@java.lang.Override
public boolean hasRequireMatchingFile() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* If true, this URLMap entry does not match the request unless
* the file referenced by the handler also exists. If no such
* file exists, processing will continue with the next URLMap that
* matches the requested URL.
* This field is useful for applications that want to match a
* static file first (with a STATIC handler), but to continue on
* with dynamic processing if no static file matches.
* N.B.(schwardo): This functionality was added to facilitate
* dynamic translation of web.xml files for the Java runtime, and
* there are no plans to expose this to Python applications at
* this time.
*
*
* optional bool require_matching_file = 36 [default = false];
* @return The requireMatchingFile.
*/
@java.lang.Override
public boolean getRequireMatchingFile() {
return requireMatchingFile_;
}
/**
*
* If true, this URLMap entry does not match the request unless
* the file referenced by the handler also exists. If no such
* file exists, processing will continue with the next URLMap that
* matches the requested URL.
* This field is useful for applications that want to match a
* static file first (with a STATIC handler), but to continue on
* with dynamic processing if no static file matches.
* N.B.(schwardo): This functionality was added to facilitate
* dynamic translation of web.xml files for the Java runtime, and
* there are no plans to expose this to Python applications at
* this time.
*
*
* optional bool require_matching_file = 36 [default = false];
* @param value The requireMatchingFile to set.
* @return This builder for chaining.
*/
public Builder setRequireMatchingFile(boolean value) {
requireMatchingFile_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* If true, this URLMap entry does not match the request unless
* the file referenced by the handler also exists. If no such
* file exists, processing will continue with the next URLMap that
* matches the requested URL.
* This field is useful for applications that want to match a
* static file first (with a STATIC handler), but to continue on
* with dynamic processing if no static file matches.
* N.B.(schwardo): This functionality was added to facilitate
* dynamic translation of web.xml files for the Java runtime, and
* there are no plans to expose this to Python applications at
* this time.
*
*
* optional bool require_matching_file = 36 [default = false];
* @return This builder for chaining.
*/
public Builder clearRequireMatchingFile() {
bitField0_ = (bitField0_ & ~0x00000008);
requireMatchingFile_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.AppInfo.URLMap)
}
// @@protoc_insertion_point(class_scope:java.apphosting.AppInfo.URLMap)
private static final com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap();
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public URLMap parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DerivedFilesOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.AppInfo.DerivedFiles)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @return A list containing the derivedFileType.
*/
java.util.List getDerivedFileTypeList();
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @return The count of derivedFileType.
*/
int getDerivedFileTypeCount();
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @param index The index of the element to return.
* @return The derivedFileType at the given index.
*/
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType getDerivedFileType(int index);
}
/**
*
* N.B.(schwardo): I wrapped this field an optional group to prevent
* an incompatibility when Python model process has this change but
* the Admin Console does not. Repeated fields in Python PB's don't
* like skew between their Python classes and swig library.
* These values must be kept in sync with those of DerivedFile::Type.
*
*
* Protobuf type {@code java.apphosting.AppInfo.DerivedFiles}
*/
public static final class DerivedFiles extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.AppInfo.DerivedFiles)
DerivedFilesOrBuilder {
private static final long serialVersionUID = 0L;
// Use DerivedFiles.newBuilder() to construct.
private DerivedFiles(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DerivedFiles() {
derivedFileType_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new DerivedFiles();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_DerivedFiles_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_DerivedFiles_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.Builder.class);
}
/**
* Protobuf enum {@code java.apphosting.AppInfo.DerivedFiles.DerivedFileType}
*/
public enum DerivedFileType
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Precompiled java bytecode.
*
*
* JAVA_PRECOMPILED = 1;
*/
JAVA_PRECOMPILED(1),
/**
*
* Precompiled Python bytecode.
*
*
* PYTHON_PRECOMPILED = 2;
*/
PYTHON_PRECOMPILED(2),
/**
*
* Compiled Go binary.
*
*
* GO_BINARY = 3;
*/
GO_BINARY(3),
;
/**
*
* Precompiled java bytecode.
*
*
* JAVA_PRECOMPILED = 1;
*/
public static final int JAVA_PRECOMPILED_VALUE = 1;
/**
*
* Precompiled Python bytecode.
*
*
* PYTHON_PRECOMPILED = 2;
*/
public static final int PYTHON_PRECOMPILED_VALUE = 2;
/**
*
* Compiled Go binary.
*
*
* GO_BINARY = 3;
*/
public static final int GO_BINARY_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DerivedFileType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static DerivedFileType forNumber(int value) {
switch (value) {
case 1: return JAVA_PRECOMPILED;
case 2: return PYTHON_PRECOMPILED;
case 3: return GO_BINARY;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
DerivedFileType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DerivedFileType findValueByNumber(int number) {
return DerivedFileType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.getDescriptor().getEnumTypes().get(0);
}
private static final DerivedFileType[] VALUES = values();
public static DerivedFileType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private DerivedFileType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.AppInfo.DerivedFiles.DerivedFileType)
}
public static final int DERIVED_FILE_TYPE_FIELD_NUMBER = 40;
@SuppressWarnings("serial")
private java.util.List derivedFileType_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType> derivedFileType_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType>() {
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType convert(java.lang.Integer from) {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType result = com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType.forNumber(from);
return result == null ? com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType.JAVA_PRECOMPILED : result;
}
};
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @return A list containing the derivedFileType.
*/
@java.lang.Override
public java.util.List getDerivedFileTypeList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType>(derivedFileType_, derivedFileType_converter_);
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @return The count of derivedFileType.
*/
@java.lang.Override
public int getDerivedFileTypeCount() {
return derivedFileType_.size();
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @param index The index of the element to return.
* @return The derivedFileType at the given index.
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType getDerivedFileType(int index) {
return derivedFileType_converter_.convert(derivedFileType_.get(index));
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < derivedFileType_.size(); i++) {
output.writeEnum(40, derivedFileType_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < derivedFileType_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(derivedFileType_.get(i));
}
size += dataSize;
size += 2 * derivedFileType_.size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles other = (com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles) obj;
if (!derivedFileType_.equals(other.derivedFileType_)) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getDerivedFileTypeCount() > 0) {
hash = (37 * hash) + DERIVED_FILE_TYPE_FIELD_NUMBER;
hash = (53 * hash) + derivedFileType_.hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* N.B.(schwardo): I wrapped this field an optional group to prevent
* an incompatibility when Python model process has this change but
* the Admin Console does not. Repeated fields in Python PB's don't
* like skew between their Python classes and swig library.
* These values must be kept in sync with those of DerivedFile::Type.
*
*
* Protobuf type {@code java.apphosting.AppInfo.DerivedFiles}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.AppInfo.DerivedFiles)
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFilesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_DerivedFiles_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_DerivedFiles_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.Builder.class);
}
// Construct using com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
derivedFileType_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_DerivedFiles_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles getDefaultInstanceForType() {
return com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles build() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles buildPartial() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles result = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles result) {
if (((bitField0_ & 0x00000001) != 0)) {
derivedFileType_ = java.util.Collections.unmodifiableList(derivedFileType_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.derivedFileType_ = derivedFileType_;
}
private void buildPartial0(com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles) {
return mergeFrom((com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles other) {
if (other == com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.getDefaultInstance()) return this;
if (!other.derivedFileType_.isEmpty()) {
if (derivedFileType_.isEmpty()) {
derivedFileType_ = other.derivedFileType_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureDerivedFileTypeIsMutable();
derivedFileType_.addAll(other.derivedFileType_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 320: {
int tmpRaw = input.readEnum();
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType tmpValue =
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(40, tmpRaw);
} else {
ensureDerivedFileTypeIsMutable();
derivedFileType_.add(tmpRaw);
}
break;
} // case 320
case 322: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType tmpValue =
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(40, tmpRaw);
} else {
ensureDerivedFileTypeIsMutable();
derivedFileType_.add(tmpRaw);
}
}
input.popLimit(oldLimit);
break;
} // case 322
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List derivedFileType_ =
java.util.Collections.emptyList();
private void ensureDerivedFileTypeIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
derivedFileType_ = new java.util.ArrayList(derivedFileType_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @return A list containing the derivedFileType.
*/
public java.util.List getDerivedFileTypeList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType>(derivedFileType_, derivedFileType_converter_);
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @return The count of derivedFileType.
*/
public int getDerivedFileTypeCount() {
return derivedFileType_.size();
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @param index The index of the element to return.
* @return The derivedFileType at the given index.
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType getDerivedFileType(int index) {
return derivedFileType_converter_.convert(derivedFileType_.get(index));
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @param index The index to set the value at.
* @param value The derivedFileType to set.
* @return This builder for chaining.
*/
public Builder setDerivedFileType(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType value) {
if (value == null) {
throw new NullPointerException();
}
ensureDerivedFileTypeIsMutable();
derivedFileType_.set(index, value.getNumber());
onChanged();
return this;
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @param value The derivedFileType to add.
* @return This builder for chaining.
*/
public Builder addDerivedFileType(com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType value) {
if (value == null) {
throw new NullPointerException();
}
ensureDerivedFileTypeIsMutable();
derivedFileType_.add(value.getNumber());
onChanged();
return this;
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @param values The derivedFileType to add.
* @return This builder for chaining.
*/
public Builder addAllDerivedFileType(
java.lang.Iterable extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType> values) {
ensureDerivedFileTypeIsMutable();
for (com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.DerivedFileType value : values) {
derivedFileType_.add(value.getNumber());
}
onChanged();
return this;
}
/**
* repeated .java.apphosting.AppInfo.DerivedFiles.DerivedFileType derived_file_type = 40;
* @return This builder for chaining.
*/
public Builder clearDerivedFileType() {
derivedFileType_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.AppInfo.DerivedFiles)
}
// @@protoc_insertion_point(class_scope:java.apphosting.AppInfo.DerivedFiles)
private static final com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles();
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DerivedFiles parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EnvironmentVariableOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.AppInfo.EnvironmentVariable)
com.google.protobuf.MessageOrBuilder {
/**
* required string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
* required string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* required string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* required string value = 2;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* required string value = 2;
* @return The value.
*/
java.lang.String getValue();
/**
* required string value = 2;
* @return The bytes for value.
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
* User defined environment variables to be set in each request.
*
*
* Protobuf type {@code java.apphosting.AppInfo.EnvironmentVariable}
*/
public static final class EnvironmentVariable extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.AppInfo.EnvironmentVariable)
EnvironmentVariableOrBuilder {
private static final long serialVersionUID = 0L;
// Use EnvironmentVariable.newBuilder() to construct.
private EnvironmentVariable(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EnvironmentVariable() {
name_ = "";
value_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new EnvironmentVariable();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_EnvironmentVariable_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_EnvironmentVariable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
* required string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* required string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object value_ = "";
/**
* required string value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string value = 2;
* @return The value.
*/
@java.lang.Override
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 2;
* @return The bytes for value.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasName()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable other = (com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* User defined environment variables to be set in each request.
*
*
* Protobuf type {@code java.apphosting.AppInfo.EnvironmentVariable}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.AppInfo.EnvironmentVariable)
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_EnvironmentVariable_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_EnvironmentVariable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder.class);
}
// Construct using com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
value_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_EnvironmentVariable_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable getDefaultInstanceForType() {
return com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable build() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable buildPartial() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable result = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable) {
return mergeFrom((com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable other) {
if (other == com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.getDefaultInstance()) return this;
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasValue()) {
value_ = other.value_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasName()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
value_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
* required string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* required string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object value_ = "";
/**
* required string value = 2;
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string value = 2;
* @return The value.
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 2;
* @return The bytes for value.
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required string value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* required string value = 2;
* @param value The bytes for value to set.
* @return This builder for chaining.
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.AppInfo.EnvironmentVariable)
}
// @@protoc_insertion_point(class_scope:java.apphosting.AppInfo.EnvironmentVariable)
private static final com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable();
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EnvironmentVariable parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int APP_ID_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object appId_ = "";
/**
* required string app_id = 1;
* @return Whether the appId field is set.
*/
@java.lang.Override
public boolean hasAppId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string app_id = 1;
* @return The appId.
*/
@java.lang.Override
public java.lang.String getAppId() {
java.lang.Object ref = appId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appId_ = s;
}
return s;
}
}
/**
* required string app_id = 1;
* @return The bytes for appId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAppIdBytes() {
java.lang.Object ref = appId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VERSION_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object versionId_ = "1";
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return Whether the versionId field is set.
*/
@java.lang.Override
public boolean hasVersionId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return The versionId.
*/
@java.lang.Override
public java.lang.String getVersionId() {
java.lang.Object ref = versionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
versionId_ = s;
}
return s;
}
}
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return The bytes for versionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVersionIdBytes() {
java.lang.Object ref = versionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
versionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMESTAMP_USEC_FIELD_NUMBER = 90;
private long timestampUsec_ = 0L;
/**
*
* Time at which this AppInfo was written, in microseconds. This field should
* only be modified by the app_config_service jobs, as it is critical to
* operation of both schedulers:
* 1. CloneScheduler uses timestamp_usec to determine whether the AppInfo is
* newer than the GlobalConfig or vice versa (e.g. when determining instance
* class);
* 2. AppMaster2 uses timestamp_usec to determine whether an AppInfo was
* recently written (i.e. when deciding whether a config update warrants a
* zero-load ServingState expiration extension).
*
*
* optional int64 timestamp_usec = 90;
* @return Whether the timestampUsec field is set.
*/
@java.lang.Override
public boolean hasTimestampUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Time at which this AppInfo was written, in microseconds. This field should
* only be modified by the app_config_service jobs, as it is critical to
* operation of both schedulers:
* 1. CloneScheduler uses timestamp_usec to determine whether the AppInfo is
* newer than the GlobalConfig or vice versa (e.g. when determining instance
* class);
* 2. AppMaster2 uses timestamp_usec to determine whether an AppInfo was
* recently written (i.e. when deciding whether a config update warrants a
* zero-load ServingState expiration extension).
*
*
* optional int64 timestamp_usec = 90;
* @return The timestampUsec.
*/
@java.lang.Override
public long getTimestampUsec() {
return timestampUsec_;
}
public static final int RUNTIME_ID_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object runtimeId_ = "python";
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return Whether the runtimeId field is set.
*/
@java.lang.Override
public boolean hasRuntimeId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return The runtimeId.
*/
@java.lang.Override
public java.lang.String getRuntimeId() {
java.lang.Object ref = runtimeId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
runtimeId_ = s;
}
return s;
}
}
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return The bytes for runtimeId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRuntimeIdBytes() {
java.lang.Object ref = runtimeId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runtimeId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RUNTIME_SPECIALIZATION_FIELD_NUMBER = 78;
@SuppressWarnings("serial")
private volatile java.lang.Object runtimeSpecialization_ = "";
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return Whether the runtimeSpecialization field is set.
*/
@java.lang.Override
public boolean hasRuntimeSpecialization() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return The runtimeSpecialization.
*/
@java.lang.Override
public java.lang.String getRuntimeSpecialization() {
java.lang.Object ref = runtimeSpecialization_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
runtimeSpecialization_ = s;
}
return s;
}
}
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return The bytes for runtimeSpecialization.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRuntimeSpecializationBytes() {
java.lang.Object ref = runtimeSpecialization_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runtimeSpecialization_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GEN2_APP_ENGINE_APIS_FIELD_NUMBER = 102;
private boolean gen2AppEngineApis_ = false;
/**
*
* Enable App Engine APIs on Second Generation go/gen2-wormhole-phase-1
*
*
* optional bool gen2_app_engine_apis = 102;
* @return Whether the gen2AppEngineApis field is set.
*/
@java.lang.Override
public boolean hasGen2AppEngineApis() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Enable App Engine APIs on Second Generation go/gen2-wormhole-phase-1
*
*
* optional bool gen2_app_engine_apis = 102;
* @return The gen2AppEngineApis.
*/
@java.lang.Override
public boolean getGen2AppEngineApis() {
return gen2AppEngineApis_;
}
public static final int INSTANCE_CLASS_FIELD_NUMBER = 91;
private int instanceClass_ = 0;
/**
*
* The instance class of this app version. Taken from version_config (for
* frontends) or server_config (for backends). Currently populated only in
* AppInfoLite messages (i.e. is_appinfo_lite is true) via ReduceAppInfo. This
* field is used by Clone Scheduler when scheduling newly deployed versions.
* TODO: Consider populating this field directly, in Zeus.
*
*
* optional int32 instance_class = 91;
* @return Whether the instanceClass field is set.
*/
@java.lang.Override
public boolean hasInstanceClass() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The instance class of this app version. Taken from version_config (for
* frontends) or server_config (for backends). Currently populated only in
* AppInfoLite messages (i.e. is_appinfo_lite is true) via ReduceAppInfo. This
* field is used by Clone Scheduler when scheduling newly deployed versions.
* TODO: Consider populating this field directly, in Zeus.
*
*
* optional int32 instance_class = 91;
* @return The instanceClass.
*/
@java.lang.Override
public int getInstanceClass() {
return instanceClass_;
}
public static final int API_VERSION_FIELD_NUMBER = 28;
@SuppressWarnings("serial")
private volatile java.lang.Object apiVersion_ = "";
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return Whether the apiVersion field is set.
*/
@java.lang.Override
public boolean hasApiVersion() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return The apiVersion.
*/
@java.lang.Override
public java.lang.String getApiVersion() {
java.lang.Object ref = apiVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
apiVersion_ = s;
}
return s;
}
}
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return The bytes for apiVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getApiVersionBytes() {
java.lang.Object ref = apiVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
apiVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILE_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List file_;
/**
* repeated group File = 6 { ... }
*/
@java.lang.Override
public java.util.List getFileList() {
return file_;
}
/**
* repeated group File = 6 { ... }
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder>
getFileOrBuilderList() {
return file_;
}
/**
* repeated group File = 6 { ... }
*/
@java.lang.Override
public int getFileCount() {
return file_.size();
}
/**
* repeated group File = 6 { ... }
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File getFile(int index) {
return file_.get(index);
}
/**
* repeated group File = 6 { ... }
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder getFileOrBuilder(
int index) {
return file_.get(index);
}
public static final int BLOB_FIELD_NUMBER = 16;
@SuppressWarnings("serial")
private java.util.List blob_;
/**
* repeated group Blob = 16 { ... }
*/
@java.lang.Override
public java.util.List getBlobList() {
return blob_;
}
/**
* repeated group Blob = 16 { ... }
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder>
getBlobOrBuilderList() {
return blob_;
}
/**
* repeated group Blob = 16 { ... }
*/
@java.lang.Override
public int getBlobCount() {
return blob_.size();
}
/**
* repeated group Blob = 16 { ... }
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob getBlob(int index) {
return blob_.get(index);
}
/**
* repeated group Blob = 16 { ... }
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder getBlobOrBuilder(
int index) {
return blob_.get(index);
}
public static final int URLMAP_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private java.util.List uRLMap_;
/**
* repeated group URLMap = 9 { ... }
*/
@java.lang.Override
public java.util.List getURLMapList() {
return uRLMap_;
}
/**
* repeated group URLMap = 9 { ... }
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder>
getURLMapOrBuilderList() {
return uRLMap_;
}
/**
* repeated group URLMap = 9 { ... }
*/
@java.lang.Override
public int getURLMapCount() {
return uRLMap_.size();
}
/**
* repeated group URLMap = 9 { ... }
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap getURLMap(int index) {
return uRLMap_.get(index);
}
/**
* repeated group URLMap = 9 { ... }
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder getURLMapOrBuilder(
int index) {
return uRLMap_.get(index);
}
public static final int DERIVEDFILES_FIELD_NUMBER = 39;
private com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles derivedFiles_;
/**
* optional group DerivedFiles = 39 { ... }
* @return Whether the derivedfiles field is set.
*/
@java.lang.Override
public boolean hasDerivedFiles() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional group DerivedFiles = 39 { ... }
* @return The derivedfiles.
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles getDerivedFiles() {
return derivedFiles_ == null ? com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.getDefaultInstance() : derivedFiles_;
}
/**
* optional group DerivedFiles = 39 { ... }
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFilesOrBuilder getDerivedFilesOrBuilder() {
return derivedFiles_ == null ? com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.getDefaultInstance() : derivedFiles_;
}
public static final int ENVIRONMENT_VARIABLE_FIELD_NUMBER = 61;
@SuppressWarnings("serial")
private java.util.List environmentVariable_;
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
@java.lang.Override
public java.util.List getEnvironmentVariableList() {
return environmentVariable_;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder>
getEnvironmentVariableOrBuilderList() {
return environmentVariable_;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
@java.lang.Override
public int getEnvironmentVariableCount() {
return environmentVariable_.size();
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable getEnvironmentVariable(int index) {
return environmentVariable_.get(index);
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder getEnvironmentVariableOrBuilder(
int index) {
return environmentVariable_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasAppId()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getFileCount(); i++) {
if (!getFile(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getBlobCount(); i++) {
if (!getBlob(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getURLMapCount(); i++) {
if (!getURLMap(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getEnvironmentVariableCount(); i++) {
if (!getEnvironmentVariable(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, appId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, versionId_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, runtimeId_);
}
for (int i = 0; i < file_.size(); i++) {
output.writeGroup(6, file_.get(i));
}
for (int i = 0; i < uRLMap_.size(); i++) {
output.writeGroup(9, uRLMap_.get(i));
}
for (int i = 0; i < blob_.size(); i++) {
output.writeGroup(16, blob_.get(i));
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 28, apiVersion_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeGroup(39, getDerivedFiles());
}
for (int i = 0; i < environmentVariable_.size(); i++) {
output.writeMessage(61, environmentVariable_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 78, runtimeSpecialization_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(90, timestampUsec_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt32(91, instanceClass_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBool(102, gen2AppEngineApis_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, appId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, versionId_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, runtimeId_);
}
for (int i = 0; i < file_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(6, file_.get(i));
}
for (int i = 0; i < uRLMap_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(9, uRLMap_.get(i));
}
for (int i = 0; i < blob_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(16, blob_.get(i));
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(28, apiVersion_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeGroupSize(39, getDerivedFiles());
}
for (int i = 0; i < environmentVariable_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(61, environmentVariable_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(78, runtimeSpecialization_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(90, timestampUsec_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(91, instanceClass_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(102, gen2AppEngineApis_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.AppinfoPb.AppInfo other = (com.google.apphosting.base.protos.AppinfoPb.AppInfo) obj;
if (hasAppId() != other.hasAppId()) return false;
if (hasAppId()) {
if (!getAppId()
.equals(other.getAppId())) return false;
}
if (hasVersionId() != other.hasVersionId()) return false;
if (hasVersionId()) {
if (!getVersionId()
.equals(other.getVersionId())) return false;
}
if (hasTimestampUsec() != other.hasTimestampUsec()) return false;
if (hasTimestampUsec()) {
if (getTimestampUsec()
!= other.getTimestampUsec()) return false;
}
if (hasRuntimeId() != other.hasRuntimeId()) return false;
if (hasRuntimeId()) {
if (!getRuntimeId()
.equals(other.getRuntimeId())) return false;
}
if (hasRuntimeSpecialization() != other.hasRuntimeSpecialization()) return false;
if (hasRuntimeSpecialization()) {
if (!getRuntimeSpecialization()
.equals(other.getRuntimeSpecialization())) return false;
}
if (hasGen2AppEngineApis() != other.hasGen2AppEngineApis()) return false;
if (hasGen2AppEngineApis()) {
if (getGen2AppEngineApis()
!= other.getGen2AppEngineApis()) return false;
}
if (hasInstanceClass() != other.hasInstanceClass()) return false;
if (hasInstanceClass()) {
if (getInstanceClass()
!= other.getInstanceClass()) return false;
}
if (hasApiVersion() != other.hasApiVersion()) return false;
if (hasApiVersion()) {
if (!getApiVersion()
.equals(other.getApiVersion())) return false;
}
if (!getFileList()
.equals(other.getFileList())) return false;
if (!getBlobList()
.equals(other.getBlobList())) return false;
if (!getURLMapList()
.equals(other.getURLMapList())) return false;
if (hasDerivedFiles() != other.hasDerivedFiles()) return false;
if (hasDerivedFiles()) {
if (!getDerivedFiles()
.equals(other.getDerivedFiles())) return false;
}
if (!getEnvironmentVariableList()
.equals(other.getEnvironmentVariableList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAppId()) {
hash = (37 * hash) + APP_ID_FIELD_NUMBER;
hash = (53 * hash) + getAppId().hashCode();
}
if (hasVersionId()) {
hash = (37 * hash) + VERSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getVersionId().hashCode();
}
if (hasTimestampUsec()) {
hash = (37 * hash) + TIMESTAMP_USEC_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimestampUsec());
}
if (hasRuntimeId()) {
hash = (37 * hash) + RUNTIME_ID_FIELD_NUMBER;
hash = (53 * hash) + getRuntimeId().hashCode();
}
if (hasRuntimeSpecialization()) {
hash = (37 * hash) + RUNTIME_SPECIALIZATION_FIELD_NUMBER;
hash = (53 * hash) + getRuntimeSpecialization().hashCode();
}
if (hasGen2AppEngineApis()) {
hash = (37 * hash) + GEN2_APP_ENGINE_APIS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getGen2AppEngineApis());
}
if (hasInstanceClass()) {
hash = (37 * hash) + INSTANCE_CLASS_FIELD_NUMBER;
hash = (53 * hash) + getInstanceClass();
}
if (hasApiVersion()) {
hash = (37 * hash) + API_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getApiVersion().hashCode();
}
if (getFileCount() > 0) {
hash = (37 * hash) + FILE_FIELD_NUMBER;
hash = (53 * hash) + getFileList().hashCode();
}
if (getBlobCount() > 0) {
hash = (37 * hash) + BLOB_FIELD_NUMBER;
hash = (53 * hash) + getBlobList().hashCode();
}
if (getURLMapCount() > 0) {
hash = (37 * hash) + URLMAP_FIELD_NUMBER;
hash = (53 * hash) + getURLMapList().hashCode();
}
if (hasDerivedFiles()) {
hash = (37 * hash) + DERIVEDFILES_FIELD_NUMBER;
hash = (53 * hash) + getDerivedFiles().hashCode();
}
if (getEnvironmentVariableCount() > 0) {
hash = (37 * hash) + ENVIRONMENT_VARIABLE_FIELD_NUMBER;
hash = (53 * hash) + getEnvironmentVariableList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.AppinfoPb.AppInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Description of an application version. In the eyes of the
* AppServer, each version is basically a different app.
* AppInfos are immutable--they are not to be changed once created. If you
* need to add per-version data that can be changed while the version is
* running, use PerVersionConfig.
* Next Tag: 107
*
*
* Protobuf type {@code java.apphosting.AppInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.AppInfo)
com.google.apphosting.base.protos.AppinfoPb.AppInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.class, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Builder.class);
}
// Construct using com.google.apphosting.base.protos.AppinfoPb.AppInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFileFieldBuilder();
getBlobFieldBuilder();
getURLMapFieldBuilder();
getDerivedFilesFieldBuilder();
getEnvironmentVariableFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
appId_ = "";
versionId_ = "1";
timestampUsec_ = 0L;
runtimeId_ = "python";
runtimeSpecialization_ = "";
gen2AppEngineApis_ = false;
instanceClass_ = 0;
apiVersion_ = "";
if (fileBuilder_ == null) {
file_ = java.util.Collections.emptyList();
} else {
file_ = null;
fileBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
if (blobBuilder_ == null) {
blob_ = java.util.Collections.emptyList();
} else {
blob_ = null;
blobBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000200);
if (uRLMapBuilder_ == null) {
uRLMap_ = java.util.Collections.emptyList();
} else {
uRLMap_ = null;
uRLMapBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000400);
derivedFiles_ = null;
if (derivedFilesBuilder_ != null) {
derivedFilesBuilder_.dispose();
derivedFilesBuilder_ = null;
}
if (environmentVariableBuilder_ == null) {
environmentVariable_ = java.util.Collections.emptyList();
} else {
environmentVariable_ = null;
environmentVariableBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.AppinfoPb.internal_static_java_apphosting_AppInfo_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo getDefaultInstanceForType() {
return com.google.apphosting.base.protos.AppinfoPb.AppInfo.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo build() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo buildPartial() {
com.google.apphosting.base.protos.AppinfoPb.AppInfo result = new com.google.apphosting.base.protos.AppinfoPb.AppInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.base.protos.AppinfoPb.AppInfo result) {
if (fileBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)) {
file_ = java.util.Collections.unmodifiableList(file_);
bitField0_ = (bitField0_ & ~0x00000100);
}
result.file_ = file_;
} else {
result.file_ = fileBuilder_.build();
}
if (blobBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)) {
blob_ = java.util.Collections.unmodifiableList(blob_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.blob_ = blob_;
} else {
result.blob_ = blobBuilder_.build();
}
if (uRLMapBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)) {
uRLMap_ = java.util.Collections.unmodifiableList(uRLMap_);
bitField0_ = (bitField0_ & ~0x00000400);
}
result.uRLMap_ = uRLMap_;
} else {
result.uRLMap_ = uRLMapBuilder_.build();
}
if (environmentVariableBuilder_ == null) {
if (((bitField0_ & 0x00001000) != 0)) {
environmentVariable_ = java.util.Collections.unmodifiableList(environmentVariable_);
bitField0_ = (bitField0_ & ~0x00001000);
}
result.environmentVariable_ = environmentVariable_;
} else {
result.environmentVariable_ = environmentVariableBuilder_.build();
}
}
private void buildPartial0(com.google.apphosting.base.protos.AppinfoPb.AppInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.appId_ = appId_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.versionId_ = versionId_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.timestampUsec_ = timestampUsec_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.runtimeId_ = runtimeId_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.runtimeSpecialization_ = runtimeSpecialization_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.gen2AppEngineApis_ = gen2AppEngineApis_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.instanceClass_ = instanceClass_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.apiVersion_ = apiVersion_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.derivedFiles_ = derivedFilesBuilder_ == null
? derivedFiles_
: derivedFilesBuilder_.build();
to_bitField0_ |= 0x00000100;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.AppinfoPb.AppInfo) {
return mergeFrom((com.google.apphosting.base.protos.AppinfoPb.AppInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.AppinfoPb.AppInfo other) {
if (other == com.google.apphosting.base.protos.AppinfoPb.AppInfo.getDefaultInstance()) return this;
if (other.hasAppId()) {
appId_ = other.appId_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasVersionId()) {
versionId_ = other.versionId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasTimestampUsec()) {
setTimestampUsec(other.getTimestampUsec());
}
if (other.hasRuntimeId()) {
runtimeId_ = other.runtimeId_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasRuntimeSpecialization()) {
runtimeSpecialization_ = other.runtimeSpecialization_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasGen2AppEngineApis()) {
setGen2AppEngineApis(other.getGen2AppEngineApis());
}
if (other.hasInstanceClass()) {
setInstanceClass(other.getInstanceClass());
}
if (other.hasApiVersion()) {
apiVersion_ = other.apiVersion_;
bitField0_ |= 0x00000080;
onChanged();
}
if (fileBuilder_ == null) {
if (!other.file_.isEmpty()) {
if (file_.isEmpty()) {
file_ = other.file_;
bitField0_ = (bitField0_ & ~0x00000100);
} else {
ensureFileIsMutable();
file_.addAll(other.file_);
}
onChanged();
}
} else {
if (!other.file_.isEmpty()) {
if (fileBuilder_.isEmpty()) {
fileBuilder_.dispose();
fileBuilder_ = null;
file_ = other.file_;
bitField0_ = (bitField0_ & ~0x00000100);
fileBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFileFieldBuilder() : null;
} else {
fileBuilder_.addAllMessages(other.file_);
}
}
}
if (blobBuilder_ == null) {
if (!other.blob_.isEmpty()) {
if (blob_.isEmpty()) {
blob_ = other.blob_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureBlobIsMutable();
blob_.addAll(other.blob_);
}
onChanged();
}
} else {
if (!other.blob_.isEmpty()) {
if (blobBuilder_.isEmpty()) {
blobBuilder_.dispose();
blobBuilder_ = null;
blob_ = other.blob_;
bitField0_ = (bitField0_ & ~0x00000200);
blobBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getBlobFieldBuilder() : null;
} else {
blobBuilder_.addAllMessages(other.blob_);
}
}
}
if (uRLMapBuilder_ == null) {
if (!other.uRLMap_.isEmpty()) {
if (uRLMap_.isEmpty()) {
uRLMap_ = other.uRLMap_;
bitField0_ = (bitField0_ & ~0x00000400);
} else {
ensureURLMapIsMutable();
uRLMap_.addAll(other.uRLMap_);
}
onChanged();
}
} else {
if (!other.uRLMap_.isEmpty()) {
if (uRLMapBuilder_.isEmpty()) {
uRLMapBuilder_.dispose();
uRLMapBuilder_ = null;
uRLMap_ = other.uRLMap_;
bitField0_ = (bitField0_ & ~0x00000400);
uRLMapBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getURLMapFieldBuilder() : null;
} else {
uRLMapBuilder_.addAllMessages(other.uRLMap_);
}
}
}
if (other.hasDerivedFiles()) {
mergeDerivedFiles(other.getDerivedFiles());
}
if (environmentVariableBuilder_ == null) {
if (!other.environmentVariable_.isEmpty()) {
if (environmentVariable_.isEmpty()) {
environmentVariable_ = other.environmentVariable_;
bitField0_ = (bitField0_ & ~0x00001000);
} else {
ensureEnvironmentVariableIsMutable();
environmentVariable_.addAll(other.environmentVariable_);
}
onChanged();
}
} else {
if (!other.environmentVariable_.isEmpty()) {
if (environmentVariableBuilder_.isEmpty()) {
environmentVariableBuilder_.dispose();
environmentVariableBuilder_ = null;
environmentVariable_ = other.environmentVariable_;
bitField0_ = (bitField0_ & ~0x00001000);
environmentVariableBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getEnvironmentVariableFieldBuilder() : null;
} else {
environmentVariableBuilder_.addAllMessages(other.environmentVariable_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasAppId()) {
return false;
}
for (int i = 0; i < getFileCount(); i++) {
if (!getFile(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getBlobCount(); i++) {
if (!getBlob(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getURLMapCount(); i++) {
if (!getURLMap(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getEnvironmentVariableCount(); i++) {
if (!getEnvironmentVariable(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
appId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
versionId_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 34: {
runtimeId_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
case 51: {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File m =
input.readGroup(6,
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.PARSER,
extensionRegistry);
if (fileBuilder_ == null) {
ensureFileIsMutable();
file_.add(m);
} else {
fileBuilder_.addMessage(m);
}
break;
} // case 51
case 75: {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap m =
input.readGroup(9,
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.PARSER,
extensionRegistry);
if (uRLMapBuilder_ == null) {
ensureURLMapIsMutable();
uRLMap_.add(m);
} else {
uRLMapBuilder_.addMessage(m);
}
break;
} // case 75
case 131: {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob m =
input.readGroup(16,
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.PARSER,
extensionRegistry);
if (blobBuilder_ == null) {
ensureBlobIsMutable();
blob_.add(m);
} else {
blobBuilder_.addMessage(m);
}
break;
} // case 131
case 226: {
apiVersion_ = input.readBytes();
bitField0_ |= 0x00000080;
break;
} // case 226
case 315: {
input.readGroup(39,
getDerivedFilesFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000800;
break;
} // case 315
case 490: {
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable m =
input.readMessage(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.PARSER,
extensionRegistry);
if (environmentVariableBuilder_ == null) {
ensureEnvironmentVariableIsMutable();
environmentVariable_.add(m);
} else {
environmentVariableBuilder_.addMessage(m);
}
break;
} // case 490
case 626: {
runtimeSpecialization_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 626
case 720: {
timestampUsec_ = input.readInt64();
bitField0_ |= 0x00000004;
break;
} // case 720
case 728: {
instanceClass_ = input.readInt32();
bitField0_ |= 0x00000040;
break;
} // case 728
case 816: {
gen2AppEngineApis_ = input.readBool();
bitField0_ |= 0x00000020;
break;
} // case 816
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object appId_ = "";
/**
* required string app_id = 1;
* @return Whether the appId field is set.
*/
public boolean hasAppId() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string app_id = 1;
* @return The appId.
*/
public java.lang.String getAppId() {
java.lang.Object ref = appId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string app_id = 1;
* @return The bytes for appId.
*/
public com.google.protobuf.ByteString
getAppIdBytes() {
java.lang.Object ref = appId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string app_id = 1;
* @param value The appId to set.
* @return This builder for chaining.
*/
public Builder setAppId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
appId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required string app_id = 1;
* @return This builder for chaining.
*/
public Builder clearAppId() {
appId_ = getDefaultInstance().getAppId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* required string app_id = 1;
* @param value The bytes for appId to set.
* @return This builder for chaining.
*/
public Builder setAppIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
appId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object versionId_ = "1";
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return Whether the versionId field is set.
*/
public boolean hasVersionId() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return The versionId.
*/
public java.lang.String getVersionId() {
java.lang.Object ref = versionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
versionId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return The bytes for versionId.
*/
public com.google.protobuf.ByteString
getVersionIdBytes() {
java.lang.Object ref = versionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
versionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @param value The versionId to set.
* @return This builder for chaining.
*/
public Builder setVersionId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
versionId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @return This builder for chaining.
*/
public Builder clearVersionId() {
versionId_ = getDefaultInstance().getVersionId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* This field encodes the version ID for this AppInfo. Due to historical
* reasons, this field can have two different formats, depending on the
* context. There is a storage format and a wire format. The storage format
* is the format that is generated when the AppInfo is written to
* storage. All subsequent reads for an already-created AppInfo use this
* format. The wire format is used by clients wishing to commit an AppInfo
* to persistent storage. We discuss these formats below.
* The storage format:
* -------------------
* The storage format is of the form
* [SERVICE_ID:]MAJOR_VERSION.MINOR_VERSION. Examples include
* "1.370530032149909029" and "my-service:1.370530032149909029".
* SERVICE_ID is the name of the service to which this version belongs. For
* versions that are deployed to the "default" service, SERVICE_ID is not
* populated. Internally, services have also been referred to as modules and
* engines.
* MAJOR_VERSION is a user-chosen string value, which happens to have some
* common values. The common values are "1" and the current time in the form
* YYYYMMDDthhmmss (e.g., "20170221t120140"). MAJOR_VERSION can be provided
* in app.yaml as the "version" field, or using the --version flag in
* "gcloud app deploy". If the version is deployed using gcloud, and no
* version is provided, gcloud generates one based on the current time in
* the format YYYYMMDDthhmmss. "1" happens to be a common value because our
* getting started documents that predate gcloud suggested using "1".
* Finally, MINOR_VERSION is an unsigned 64 bit integer, represented in its
* base-10 string form. This integer has the following properties:
* 1) Its 28 least-significant bits are randomly generated by the server
* committing the AppInfo to storage.
* 2) Its 36 most-significant bits represent the time around which the
* server committed the AppInfo to storage, in seconds since Epoch.
* The MINOR_VERSION format is *very* important. Many parts of our system
* rely on the ordering that is produced when sorting all minor versions
* within a major version. This ordering ensures that only the last deployed
* minor version is served, so we should probably never change the format of
* the minor version.
* The wire format:
* ----------------
* The wire format is the same as the storage format minus the
* MINOR_VERSION: [SERVICE_ID:]MAJOR_VERSION. Examples include "1" and
* "my-service:1".
* The minor version is missing from the wire format because it is the
* responsibility of AppConfigService implementation to generate the minor
* version based on the current time. If a client populates the
* MINOR_VERSION portion of version_id when creating an AppInfo, the
* AppConfigService implementation should discard it and generate its own
* MINOR_VERSION.
* * * *
* Interested in more? See http://go/app-engine-ids.
*
*
* optional string version_id = 2 [default = "1"];
* @param value The bytes for versionId to set.
* @return This builder for chaining.
*/
public Builder setVersionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
versionId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private long timestampUsec_ ;
/**
*
* Time at which this AppInfo was written, in microseconds. This field should
* only be modified by the app_config_service jobs, as it is critical to
* operation of both schedulers:
* 1. CloneScheduler uses timestamp_usec to determine whether the AppInfo is
* newer than the GlobalConfig or vice versa (e.g. when determining instance
* class);
* 2. AppMaster2 uses timestamp_usec to determine whether an AppInfo was
* recently written (i.e. when deciding whether a config update warrants a
* zero-load ServingState expiration extension).
*
*
* optional int64 timestamp_usec = 90;
* @return Whether the timestampUsec field is set.
*/
@java.lang.Override
public boolean hasTimestampUsec() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Time at which this AppInfo was written, in microseconds. This field should
* only be modified by the app_config_service jobs, as it is critical to
* operation of both schedulers:
* 1. CloneScheduler uses timestamp_usec to determine whether the AppInfo is
* newer than the GlobalConfig or vice versa (e.g. when determining instance
* class);
* 2. AppMaster2 uses timestamp_usec to determine whether an AppInfo was
* recently written (i.e. when deciding whether a config update warrants a
* zero-load ServingState expiration extension).
*
*
* optional int64 timestamp_usec = 90;
* @return The timestampUsec.
*/
@java.lang.Override
public long getTimestampUsec() {
return timestampUsec_;
}
/**
*
* Time at which this AppInfo was written, in microseconds. This field should
* only be modified by the app_config_service jobs, as it is critical to
* operation of both schedulers:
* 1. CloneScheduler uses timestamp_usec to determine whether the AppInfo is
* newer than the GlobalConfig or vice versa (e.g. when determining instance
* class);
* 2. AppMaster2 uses timestamp_usec to determine whether an AppInfo was
* recently written (i.e. when deciding whether a config update warrants a
* zero-load ServingState expiration extension).
*
*
* optional int64 timestamp_usec = 90;
* @param value The timestampUsec to set.
* @return This builder for chaining.
*/
public Builder setTimestampUsec(long value) {
timestampUsec_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Time at which this AppInfo was written, in microseconds. This field should
* only be modified by the app_config_service jobs, as it is critical to
* operation of both schedulers:
* 1. CloneScheduler uses timestamp_usec to determine whether the AppInfo is
* newer than the GlobalConfig or vice versa (e.g. when determining instance
* class);
* 2. AppMaster2 uses timestamp_usec to determine whether an AppInfo was
* recently written (i.e. when deciding whether a config update warrants a
* zero-load ServingState expiration extension).
*
*
* optional int64 timestamp_usec = 90;
* @return This builder for chaining.
*/
public Builder clearTimestampUsec() {
bitField0_ = (bitField0_ & ~0x00000004);
timestampUsec_ = 0L;
onChanged();
return this;
}
private java.lang.Object runtimeId_ = "python";
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return Whether the runtimeId field is set.
*/
public boolean hasRuntimeId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return The runtimeId.
*/
public java.lang.String getRuntimeId() {
java.lang.Object ref = runtimeId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
runtimeId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return The bytes for runtimeId.
*/
public com.google.protobuf.ByteString
getRuntimeIdBytes() {
java.lang.Object ref = runtimeId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runtimeId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @param value The runtimeId to set.
* @return This builder for chaining.
*/
public Builder setRuntimeId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
runtimeId_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @return This builder for chaining.
*/
public Builder clearRuntimeId() {
runtimeId_ = getDefaultInstance().getRuntimeId();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* Not really optional.
*
*
* optional string runtime_id = 4 [default = "python"];
* @param value The bytes for runtimeId to set.
* @return This builder for chaining.
*/
public Builder setRuntimeIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
runtimeId_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object runtimeSpecialization_ = "";
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return Whether the runtimeSpecialization field is set.
*/
public boolean hasRuntimeSpecialization() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return The runtimeSpecialization.
*/
public java.lang.String getRuntimeSpecialization() {
java.lang.Object ref = runtimeSpecialization_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
runtimeSpecialization_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return The bytes for runtimeSpecialization.
*/
public com.google.protobuf.ByteString
getRuntimeSpecializationBytes() {
java.lang.Object ref = runtimeSpecialization_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
runtimeSpecialization_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @param value The runtimeSpecialization to set.
* @return This builder for chaining.
*/
public Builder setRuntimeSpecialization(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
runtimeSpecialization_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @return This builder for chaining.
*/
public Builder clearRuntimeSpecialization() {
runtimeSpecialization_ = getDefaultInstance().getRuntimeSpecialization();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* Optional string that can be used to indicate a specialization within
* the runtime_id. For example, this field can be used to specialize
* within the runtime_id:container runtime.
* Important: This field should be populated only on consultation with
* the appengine<internal> team.
*
*
* optional string runtime_specialization = 78;
* @param value The bytes for runtimeSpecialization to set.
* @return This builder for chaining.
*/
public Builder setRuntimeSpecializationBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
runtimeSpecialization_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private boolean gen2AppEngineApis_ ;
/**
*
* Enable App Engine APIs on Second Generation go/gen2-wormhole-phase-1
*
*
* optional bool gen2_app_engine_apis = 102;
* @return Whether the gen2AppEngineApis field is set.
*/
@java.lang.Override
public boolean hasGen2AppEngineApis() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Enable App Engine APIs on Second Generation go/gen2-wormhole-phase-1
*
*
* optional bool gen2_app_engine_apis = 102;
* @return The gen2AppEngineApis.
*/
@java.lang.Override
public boolean getGen2AppEngineApis() {
return gen2AppEngineApis_;
}
/**
*
* Enable App Engine APIs on Second Generation go/gen2-wormhole-phase-1
*
*
* optional bool gen2_app_engine_apis = 102;
* @param value The gen2AppEngineApis to set.
* @return This builder for chaining.
*/
public Builder setGen2AppEngineApis(boolean value) {
gen2AppEngineApis_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Enable App Engine APIs on Second Generation go/gen2-wormhole-phase-1
*
*
* optional bool gen2_app_engine_apis = 102;
* @return This builder for chaining.
*/
public Builder clearGen2AppEngineApis() {
bitField0_ = (bitField0_ & ~0x00000020);
gen2AppEngineApis_ = false;
onChanged();
return this;
}
private int instanceClass_ ;
/**
*
* The instance class of this app version. Taken from version_config (for
* frontends) or server_config (for backends). Currently populated only in
* AppInfoLite messages (i.e. is_appinfo_lite is true) via ReduceAppInfo. This
* field is used by Clone Scheduler when scheduling newly deployed versions.
* TODO: Consider populating this field directly, in Zeus.
*
*
* optional int32 instance_class = 91;
* @return Whether the instanceClass field is set.
*/
@java.lang.Override
public boolean hasInstanceClass() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The instance class of this app version. Taken from version_config (for
* frontends) or server_config (for backends). Currently populated only in
* AppInfoLite messages (i.e. is_appinfo_lite is true) via ReduceAppInfo. This
* field is used by Clone Scheduler when scheduling newly deployed versions.
* TODO: Consider populating this field directly, in Zeus.
*
*
* optional int32 instance_class = 91;
* @return The instanceClass.
*/
@java.lang.Override
public int getInstanceClass() {
return instanceClass_;
}
/**
*
* The instance class of this app version. Taken from version_config (for
* frontends) or server_config (for backends). Currently populated only in
* AppInfoLite messages (i.e. is_appinfo_lite is true) via ReduceAppInfo. This
* field is used by Clone Scheduler when scheduling newly deployed versions.
* TODO: Consider populating this field directly, in Zeus.
*
*
* optional int32 instance_class = 91;
* @param value The instanceClass to set.
* @return This builder for chaining.
*/
public Builder setInstanceClass(int value) {
instanceClass_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The instance class of this app version. Taken from version_config (for
* frontends) or server_config (for backends). Currently populated only in
* AppInfoLite messages (i.e. is_appinfo_lite is true) via ReduceAppInfo. This
* field is used by Clone Scheduler when scheduling newly deployed versions.
* TODO: Consider populating this field directly, in Zeus.
*
*
* optional int32 instance_class = 91;
* @return This builder for chaining.
*/
public Builder clearInstanceClass() {
bitField0_ = (bitField0_ & ~0x00000040);
instanceClass_ = 0;
onChanged();
return this;
}
private java.lang.Object apiVersion_ = "";
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return Whether the apiVersion field is set.
*/
public boolean hasApiVersion() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return The apiVersion.
*/
public java.lang.String getApiVersion() {
java.lang.Object ref = apiVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
apiVersion_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return The bytes for apiVersion.
*/
public com.google.protobuf.ByteString
getApiVersionBytes() {
java.lang.Object ref = apiVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
apiVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @param value The apiVersion to set.
* @return This builder for chaining.
*/
public Builder setApiVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
apiVersion_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @return This builder for chaining.
*/
public Builder clearApiVersion() {
apiVersion_ = getDefaultInstance().getApiVersion();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
* Major API version to use for this application.
*
*
* optional string api_version = 28 [default = ""];
* @param value The bytes for apiVersion to set.
* @return This builder for chaining.
*/
public Builder setApiVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
apiVersion_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private java.util.List file_ =
java.util.Collections.emptyList();
private void ensureFileIsMutable() {
if (!((bitField0_ & 0x00000100) != 0)) {
file_ = new java.util.ArrayList(file_);
bitField0_ |= 0x00000100;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder> fileBuilder_;
/**
* repeated group File = 6 { ... }
*/
public java.util.List getFileList() {
if (fileBuilder_ == null) {
return java.util.Collections.unmodifiableList(file_);
} else {
return fileBuilder_.getMessageList();
}
}
/**
* repeated group File = 6 { ... }
*/
public int getFileCount() {
if (fileBuilder_ == null) {
return file_.size();
} else {
return fileBuilder_.getCount();
}
}
/**
* repeated group File = 6 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File getFile(int index) {
if (fileBuilder_ == null) {
return file_.get(index);
} else {
return fileBuilder_.getMessage(index);
}
}
/**
* repeated group File = 6 { ... }
*/
public Builder setFile(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File value) {
if (fileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFileIsMutable();
file_.set(index, value);
onChanged();
} else {
fileBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public Builder setFile(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder builderForValue) {
if (fileBuilder_ == null) {
ensureFileIsMutable();
file_.set(index, builderForValue.build());
onChanged();
} else {
fileBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public Builder addFile(com.google.apphosting.base.protos.AppinfoPb.AppInfo.File value) {
if (fileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFileIsMutable();
file_.add(value);
onChanged();
} else {
fileBuilder_.addMessage(value);
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public Builder addFile(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File value) {
if (fileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFileIsMutable();
file_.add(index, value);
onChanged();
} else {
fileBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public Builder addFile(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder builderForValue) {
if (fileBuilder_ == null) {
ensureFileIsMutable();
file_.add(builderForValue.build());
onChanged();
} else {
fileBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public Builder addFile(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder builderForValue) {
if (fileBuilder_ == null) {
ensureFileIsMutable();
file_.add(index, builderForValue.build());
onChanged();
} else {
fileBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public Builder addAllFile(
java.lang.Iterable extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.File> values) {
if (fileBuilder_ == null) {
ensureFileIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, file_);
onChanged();
} else {
fileBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public Builder clearFile() {
if (fileBuilder_ == null) {
file_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
} else {
fileBuilder_.clear();
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public Builder removeFile(int index) {
if (fileBuilder_ == null) {
ensureFileIsMutable();
file_.remove(index);
onChanged();
} else {
fileBuilder_.remove(index);
}
return this;
}
/**
* repeated group File = 6 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder getFileBuilder(
int index) {
return getFileFieldBuilder().getBuilder(index);
}
/**
* repeated group File = 6 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder getFileOrBuilder(
int index) {
if (fileBuilder_ == null) {
return file_.get(index); } else {
return fileBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated group File = 6 { ... }
*/
public java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder>
getFileOrBuilderList() {
if (fileBuilder_ != null) {
return fileBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(file_);
}
}
/**
* repeated group File = 6 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder addFileBuilder() {
return getFileFieldBuilder().addBuilder(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.getDefaultInstance());
}
/**
* repeated group File = 6 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder addFileBuilder(
int index) {
return getFileFieldBuilder().addBuilder(
index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.getDefaultInstance());
}
/**
* repeated group File = 6 { ... }
*/
public java.util.List
getFileBuilderList() {
return getFileFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder>
getFileFieldBuilder() {
if (fileBuilder_ == null) {
fileBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.File, com.google.apphosting.base.protos.AppinfoPb.AppInfo.File.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.FileOrBuilder>(
file_,
((bitField0_ & 0x00000100) != 0),
getParentForChildren(),
isClean());
file_ = null;
}
return fileBuilder_;
}
private java.util.List blob_ =
java.util.Collections.emptyList();
private void ensureBlobIsMutable() {
if (!((bitField0_ & 0x00000200) != 0)) {
blob_ = new java.util.ArrayList(blob_);
bitField0_ |= 0x00000200;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder> blobBuilder_;
/**
* repeated group Blob = 16 { ... }
*/
public java.util.List getBlobList() {
if (blobBuilder_ == null) {
return java.util.Collections.unmodifiableList(blob_);
} else {
return blobBuilder_.getMessageList();
}
}
/**
* repeated group Blob = 16 { ... }
*/
public int getBlobCount() {
if (blobBuilder_ == null) {
return blob_.size();
} else {
return blobBuilder_.getCount();
}
}
/**
* repeated group Blob = 16 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob getBlob(int index) {
if (blobBuilder_ == null) {
return blob_.get(index);
} else {
return blobBuilder_.getMessage(index);
}
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder setBlob(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob value) {
if (blobBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBlobIsMutable();
blob_.set(index, value);
onChanged();
} else {
blobBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder setBlob(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder builderForValue) {
if (blobBuilder_ == null) {
ensureBlobIsMutable();
blob_.set(index, builderForValue.build());
onChanged();
} else {
blobBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder addBlob(com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob value) {
if (blobBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBlobIsMutable();
blob_.add(value);
onChanged();
} else {
blobBuilder_.addMessage(value);
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder addBlob(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob value) {
if (blobBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBlobIsMutable();
blob_.add(index, value);
onChanged();
} else {
blobBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder addBlob(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder builderForValue) {
if (blobBuilder_ == null) {
ensureBlobIsMutable();
blob_.add(builderForValue.build());
onChanged();
} else {
blobBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder addBlob(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder builderForValue) {
if (blobBuilder_ == null) {
ensureBlobIsMutable();
blob_.add(index, builderForValue.build());
onChanged();
} else {
blobBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder addAllBlob(
java.lang.Iterable extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob> values) {
if (blobBuilder_ == null) {
ensureBlobIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, blob_);
onChanged();
} else {
blobBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder clearBlob() {
if (blobBuilder_ == null) {
blob_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
} else {
blobBuilder_.clear();
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public Builder removeBlob(int index) {
if (blobBuilder_ == null) {
ensureBlobIsMutable();
blob_.remove(index);
onChanged();
} else {
blobBuilder_.remove(index);
}
return this;
}
/**
* repeated group Blob = 16 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder getBlobBuilder(
int index) {
return getBlobFieldBuilder().getBuilder(index);
}
/**
* repeated group Blob = 16 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder getBlobOrBuilder(
int index) {
if (blobBuilder_ == null) {
return blob_.get(index); } else {
return blobBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated group Blob = 16 { ... }
*/
public java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder>
getBlobOrBuilderList() {
if (blobBuilder_ != null) {
return blobBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(blob_);
}
}
/**
* repeated group Blob = 16 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder addBlobBuilder() {
return getBlobFieldBuilder().addBuilder(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.getDefaultInstance());
}
/**
* repeated group Blob = 16 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder addBlobBuilder(
int index) {
return getBlobFieldBuilder().addBuilder(
index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.getDefaultInstance());
}
/**
* repeated group Blob = 16 { ... }
*/
public java.util.List
getBlobBuilderList() {
return getBlobFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder>
getBlobFieldBuilder() {
if (blobBuilder_ == null) {
blobBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob, com.google.apphosting.base.protos.AppinfoPb.AppInfo.Blob.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.BlobOrBuilder>(
blob_,
((bitField0_ & 0x00000200) != 0),
getParentForChildren(),
isClean());
blob_ = null;
}
return blobBuilder_;
}
private java.util.List uRLMap_ =
java.util.Collections.emptyList();
private void ensureURLMapIsMutable() {
if (!((bitField0_ & 0x00000400) != 0)) {
uRLMap_ = new java.util.ArrayList(uRLMap_);
bitField0_ |= 0x00000400;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder> uRLMapBuilder_;
/**
* repeated group URLMap = 9 { ... }
*/
public java.util.List getURLMapList() {
if (uRLMapBuilder_ == null) {
return java.util.Collections.unmodifiableList(uRLMap_);
} else {
return uRLMapBuilder_.getMessageList();
}
}
/**
* repeated group URLMap = 9 { ... }
*/
public int getURLMapCount() {
if (uRLMapBuilder_ == null) {
return uRLMap_.size();
} else {
return uRLMapBuilder_.getCount();
}
}
/**
* repeated group URLMap = 9 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap getURLMap(int index) {
if (uRLMapBuilder_ == null) {
return uRLMap_.get(index);
} else {
return uRLMapBuilder_.getMessage(index);
}
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder setURLMap(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap value) {
if (uRLMapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureURLMapIsMutable();
uRLMap_.set(index, value);
onChanged();
} else {
uRLMapBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder setURLMap(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder builderForValue) {
if (uRLMapBuilder_ == null) {
ensureURLMapIsMutable();
uRLMap_.set(index, builderForValue.build());
onChanged();
} else {
uRLMapBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder addURLMap(com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap value) {
if (uRLMapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureURLMapIsMutable();
uRLMap_.add(value);
onChanged();
} else {
uRLMapBuilder_.addMessage(value);
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder addURLMap(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap value) {
if (uRLMapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureURLMapIsMutable();
uRLMap_.add(index, value);
onChanged();
} else {
uRLMapBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder addURLMap(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder builderForValue) {
if (uRLMapBuilder_ == null) {
ensureURLMapIsMutable();
uRLMap_.add(builderForValue.build());
onChanged();
} else {
uRLMapBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder addURLMap(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder builderForValue) {
if (uRLMapBuilder_ == null) {
ensureURLMapIsMutable();
uRLMap_.add(index, builderForValue.build());
onChanged();
} else {
uRLMapBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder addAllURLMap(
java.lang.Iterable extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap> values) {
if (uRLMapBuilder_ == null) {
ensureURLMapIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, uRLMap_);
onChanged();
} else {
uRLMapBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder clearURLMap() {
if (uRLMapBuilder_ == null) {
uRLMap_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
onChanged();
} else {
uRLMapBuilder_.clear();
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public Builder removeURLMap(int index) {
if (uRLMapBuilder_ == null) {
ensureURLMapIsMutable();
uRLMap_.remove(index);
onChanged();
} else {
uRLMapBuilder_.remove(index);
}
return this;
}
/**
* repeated group URLMap = 9 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder getURLMapBuilder(
int index) {
return getURLMapFieldBuilder().getBuilder(index);
}
/**
* repeated group URLMap = 9 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder getURLMapOrBuilder(
int index) {
if (uRLMapBuilder_ == null) {
return uRLMap_.get(index); } else {
return uRLMapBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated group URLMap = 9 { ... }
*/
public java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder>
getURLMapOrBuilderList() {
if (uRLMapBuilder_ != null) {
return uRLMapBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(uRLMap_);
}
}
/**
* repeated group URLMap = 9 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder addURLMapBuilder() {
return getURLMapFieldBuilder().addBuilder(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.getDefaultInstance());
}
/**
* repeated group URLMap = 9 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder addURLMapBuilder(
int index) {
return getURLMapFieldBuilder().addBuilder(
index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.getDefaultInstance());
}
/**
* repeated group URLMap = 9 { ... }
*/
public java.util.List
getURLMapBuilderList() {
return getURLMapFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder>
getURLMapFieldBuilder() {
if (uRLMapBuilder_ == null) {
uRLMapBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMap.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.URLMapOrBuilder>(
uRLMap_,
((bitField0_ & 0x00000400) != 0),
getParentForChildren(),
isClean());
uRLMap_ = null;
}
return uRLMapBuilder_;
}
private com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles derivedFiles_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFilesOrBuilder> derivedFilesBuilder_;
/**
* optional group DerivedFiles = 39 { ... }
* @return Whether the derivedfiles field is set.
*/
public boolean hasDerivedFiles() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
* optional group DerivedFiles = 39 { ... }
* @return The derivedfiles.
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles getDerivedFiles() {
if (derivedFilesBuilder_ == null) {
return derivedFiles_ == null ? com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.getDefaultInstance() : derivedFiles_;
} else {
return derivedFilesBuilder_.getMessage();
}
}
/**
* optional group DerivedFiles = 39 { ... }
*/
public Builder setDerivedFiles(com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles value) {
if (derivedFilesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
derivedFiles_ = value;
} else {
derivedFilesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* optional group DerivedFiles = 39 { ... }
*/
public Builder setDerivedFiles(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.Builder builderForValue) {
if (derivedFilesBuilder_ == null) {
derivedFiles_ = builderForValue.build();
} else {
derivedFilesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* optional group DerivedFiles = 39 { ... }
*/
public Builder mergeDerivedFiles(com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles value) {
if (derivedFilesBuilder_ == null) {
if (((bitField0_ & 0x00000800) != 0) &&
derivedFiles_ != null &&
derivedFiles_ != com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.getDefaultInstance()) {
getDerivedFilesBuilder().mergeFrom(value);
} else {
derivedFiles_ = value;
}
} else {
derivedFilesBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* optional group DerivedFiles = 39 { ... }
*/
public Builder clearDerivedFiles() {
bitField0_ = (bitField0_ & ~0x00000800);
derivedFiles_ = null;
if (derivedFilesBuilder_ != null) {
derivedFilesBuilder_.dispose();
derivedFilesBuilder_ = null;
}
onChanged();
return this;
}
/**
* optional group DerivedFiles = 39 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.Builder getDerivedFilesBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getDerivedFilesFieldBuilder().getBuilder();
}
/**
* optional group DerivedFiles = 39 { ... }
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFilesOrBuilder getDerivedFilesOrBuilder() {
if (derivedFilesBuilder_ != null) {
return derivedFilesBuilder_.getMessageOrBuilder();
} else {
return derivedFiles_ == null ?
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.getDefaultInstance() : derivedFiles_;
}
}
/**
* optional group DerivedFiles = 39 { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFilesOrBuilder>
getDerivedFilesFieldBuilder() {
if (derivedFilesBuilder_ == null) {
derivedFilesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFiles.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.DerivedFilesOrBuilder>(
getDerivedFiles(),
getParentForChildren(),
isClean());
derivedFiles_ = null;
}
return derivedFilesBuilder_;
}
private java.util.List environmentVariable_ =
java.util.Collections.emptyList();
private void ensureEnvironmentVariableIsMutable() {
if (!((bitField0_ & 0x00001000) != 0)) {
environmentVariable_ = new java.util.ArrayList(environmentVariable_);
bitField0_ |= 0x00001000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder> environmentVariableBuilder_;
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public java.util.List getEnvironmentVariableList() {
if (environmentVariableBuilder_ == null) {
return java.util.Collections.unmodifiableList(environmentVariable_);
} else {
return environmentVariableBuilder_.getMessageList();
}
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public int getEnvironmentVariableCount() {
if (environmentVariableBuilder_ == null) {
return environmentVariable_.size();
} else {
return environmentVariableBuilder_.getCount();
}
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable getEnvironmentVariable(int index) {
if (environmentVariableBuilder_ == null) {
return environmentVariable_.get(index);
} else {
return environmentVariableBuilder_.getMessage(index);
}
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder setEnvironmentVariable(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable value) {
if (environmentVariableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnvironmentVariableIsMutable();
environmentVariable_.set(index, value);
onChanged();
} else {
environmentVariableBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder setEnvironmentVariable(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder builderForValue) {
if (environmentVariableBuilder_ == null) {
ensureEnvironmentVariableIsMutable();
environmentVariable_.set(index, builderForValue.build());
onChanged();
} else {
environmentVariableBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder addEnvironmentVariable(com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable value) {
if (environmentVariableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnvironmentVariableIsMutable();
environmentVariable_.add(value);
onChanged();
} else {
environmentVariableBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder addEnvironmentVariable(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable value) {
if (environmentVariableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEnvironmentVariableIsMutable();
environmentVariable_.add(index, value);
onChanged();
} else {
environmentVariableBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder addEnvironmentVariable(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder builderForValue) {
if (environmentVariableBuilder_ == null) {
ensureEnvironmentVariableIsMutable();
environmentVariable_.add(builderForValue.build());
onChanged();
} else {
environmentVariableBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder addEnvironmentVariable(
int index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder builderForValue) {
if (environmentVariableBuilder_ == null) {
ensureEnvironmentVariableIsMutable();
environmentVariable_.add(index, builderForValue.build());
onChanged();
} else {
environmentVariableBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder addAllEnvironmentVariable(
java.lang.Iterable extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable> values) {
if (environmentVariableBuilder_ == null) {
ensureEnvironmentVariableIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, environmentVariable_);
onChanged();
} else {
environmentVariableBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder clearEnvironmentVariable() {
if (environmentVariableBuilder_ == null) {
environmentVariable_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
} else {
environmentVariableBuilder_.clear();
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public Builder removeEnvironmentVariable(int index) {
if (environmentVariableBuilder_ == null) {
ensureEnvironmentVariableIsMutable();
environmentVariable_.remove(index);
onChanged();
} else {
environmentVariableBuilder_.remove(index);
}
return this;
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder getEnvironmentVariableBuilder(
int index) {
return getEnvironmentVariableFieldBuilder().getBuilder(index);
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder getEnvironmentVariableOrBuilder(
int index) {
if (environmentVariableBuilder_ == null) {
return environmentVariable_.get(index); } else {
return environmentVariableBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public java.util.List extends com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder>
getEnvironmentVariableOrBuilderList() {
if (environmentVariableBuilder_ != null) {
return environmentVariableBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(environmentVariable_);
}
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder addEnvironmentVariableBuilder() {
return getEnvironmentVariableFieldBuilder().addBuilder(
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.getDefaultInstance());
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder addEnvironmentVariableBuilder(
int index) {
return getEnvironmentVariableFieldBuilder().addBuilder(
index, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.getDefaultInstance());
}
/**
* repeated .java.apphosting.AppInfo.EnvironmentVariable environment_variable = 61;
*/
public java.util.List
getEnvironmentVariableBuilderList() {
return getEnvironmentVariableFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder>
getEnvironmentVariableFieldBuilder() {
if (environmentVariableBuilder_ == null) {
environmentVariableBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariable.Builder, com.google.apphosting.base.protos.AppinfoPb.AppInfo.EnvironmentVariableOrBuilder>(
environmentVariable_,
((bitField0_ & 0x00001000) != 0),
getParentForChildren(),
isClean());
environmentVariable_ = null;
}
return environmentVariableBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.AppInfo)
}
// @@protoc_insertion_point(class_scope:java.apphosting.AppInfo)
private static final com.google.apphosting.base.protos.AppinfoPb.AppInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.AppinfoPb.AppInfo();
}
public static com.google.apphosting.base.protos.AppinfoPb.AppInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AppInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.AppinfoPb.AppInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_Handler_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_Handler_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_AppInfo_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_AppInfo_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_AppInfo_File_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_AppInfo_File_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_AppInfo_Blob_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_AppInfo_Blob_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_AppInfo_URLMap_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_AppInfo_URLMap_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_AppInfo_DerivedFiles_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_AppInfo_DerivedFiles_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_AppInfo_EnvironmentVariable_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_AppInfo_EnvironmentVariable_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\rappinfo.proto\022\017java.apphosting\"^\n\007Hand" +
"ler\022\017\n\004type\030\001 \001(\005:\0011\022\014\n\004path\030\002 \002(\t\"4\n\013HA" +
"NDLERTYPE\022\n\n\006STATIC\020\000\022\013\n\007CGI_BIN\020\001\022\014\n\010SH" +
"UTDOWN\020\003\"\336\007\n\007AppInfo\022\016\n\006app_id\030\001 \002(\t\022\025\n\n" +
"version_id\030\002 \001(\t:\0011\022\026\n\016timestamp_usec\030Z " +
"\001(\003\022\032\n\nruntime_id\030\004 \001(\t:\006python\022\036\n\026runti" +
"me_specialization\030N \001(\t\022\034\n\024gen2_app_engi" +
"ne_apis\030f \001(\010\022\026\n\016instance_class\030[ \001(\005\022\025\n" +
"\013api_version\030\034 \001(\t:\000\022+\n\004file\030\006 \003(\n2\035.jav" +
"a.apphosting.AppInfo.File\022+\n\004blob\030\020 \003(\n2" +
"\035.java.apphosting.AppInfo.Blob\022/\n\006urlmap" +
"\030\t \003(\n2\037.java.apphosting.AppInfo.URLMap\022" +
";\n\014derivedfiles\030\' \001(\n2%.java.apphosting." +
"AppInfo.DerivedFiles\022J\n\024environment_vari" +
"able\030= \003(\0132,.java.apphosting.AppInfo.Env" +
"ironmentVariable\032c\n\004File\022\021\n\tsha1_hash\030\007 " +
"\002(\t\022\014\n\004path\030\010 \002(\t\022\021\n\tfile_size\030\016 \002(\003\022\023\n\t" +
"mime_type\030\017 \001(\t:\000\022\022\n\nsource_url\030G \001(\t\032\'\n" +
"\004Blob\022\014\n\004path\030\022 \002(\t\022\021\n\tmime_type\030# \001(\t\032|" +
"\n\006URLMap\022\r\n\005regex\030\n \001(\t\022)\n\007handler\030\013 \001(\013" +
"2\030.java.apphosting.Handler\022\022\n\nstatic_dir" +
"\030\036 \001(\t\022$\n\025require_matching_file\030$ \001(\010:\005f" +
"alse\032\260\001\n\014DerivedFiles\022P\n\021derived_file_ty" +
"pe\030( \003(\01625.java.apphosting.AppInfo.Deriv" +
"edFiles.DerivedFileType\"N\n\017DerivedFileTy" +
"pe\022\024\n\020JAVA_PRECOMPILED\020\001\022\026\n\022PYTHON_PRECO" +
"MPILED\020\002\022\r\n\tGO_BINARY\020\003\0322\n\023EnvironmentVa" +
"riable\022\014\n\004name\030\001 \002(\t\022\r\n\005value\030\002 \002(\tJ\004\010X\020" +
"YB1\n!com.google.apphosting.base.protosB\t" +
"AppinfoPb\370\001\001"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_java_apphosting_Handler_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_java_apphosting_Handler_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_Handler_descriptor,
new java.lang.String[] { "Type", "Path", });
internal_static_java_apphosting_AppInfo_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_java_apphosting_AppInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_AppInfo_descriptor,
new java.lang.String[] { "AppId", "VersionId", "TimestampUsec", "RuntimeId", "RuntimeSpecialization", "Gen2AppEngineApis", "InstanceClass", "ApiVersion", "File", "Blob", "URLMap", "DerivedFiles", "EnvironmentVariable", });
internal_static_java_apphosting_AppInfo_File_descriptor =
internal_static_java_apphosting_AppInfo_descriptor.getNestedTypes().get(0);
internal_static_java_apphosting_AppInfo_File_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_AppInfo_File_descriptor,
new java.lang.String[] { "Sha1Hash", "Path", "FileSize", "MimeType", "SourceUrl", });
internal_static_java_apphosting_AppInfo_Blob_descriptor =
internal_static_java_apphosting_AppInfo_descriptor.getNestedTypes().get(1);
internal_static_java_apphosting_AppInfo_Blob_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_AppInfo_Blob_descriptor,
new java.lang.String[] { "Path", "MimeType", });
internal_static_java_apphosting_AppInfo_URLMap_descriptor =
internal_static_java_apphosting_AppInfo_descriptor.getNestedTypes().get(2);
internal_static_java_apphosting_AppInfo_URLMap_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_AppInfo_URLMap_descriptor,
new java.lang.String[] { "Regex", "Handler", "StaticDir", "RequireMatchingFile", });
internal_static_java_apphosting_AppInfo_DerivedFiles_descriptor =
internal_static_java_apphosting_AppInfo_descriptor.getNestedTypes().get(3);
internal_static_java_apphosting_AppInfo_DerivedFiles_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_AppInfo_DerivedFiles_descriptor,
new java.lang.String[] { "DerivedFileType", });
internal_static_java_apphosting_AppInfo_EnvironmentVariable_descriptor =
internal_static_java_apphosting_AppInfo_descriptor.getNestedTypes().get(4);
internal_static_java_apphosting_AppInfo_EnvironmentVariable_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_AppInfo_EnvironmentVariable_descriptor,
new java.lang.String[] { "Name", "Value", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy