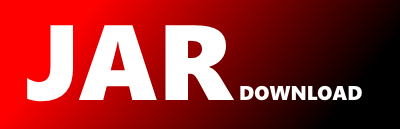
com.google.apphosting.base.protos.HttpPb Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: http.proto
package com.google.apphosting.base.protos;
public final class HttpPb {
private HttpPb() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code java.apphosting.ServingDestination}
*/
public enum ServingDestination
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Served by worker code, default value.
*
*
* WORKER = 0;
*/
WORKER(0),
/**
*
* Served by the supervisor.
*
*
* TITANIUM_SUPERVISOR = 1;
*/
TITANIUM_SUPERVISOR(1),
;
/**
*
* Served by worker code, default value.
*
*
* WORKER = 0;
*/
public static final int WORKER_VALUE = 0;
/**
*
* Served by the supervisor.
*
*
* TITANIUM_SUPERVISOR = 1;
*/
public static final int TITANIUM_SUPERVISOR_VALUE = 1;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ServingDestination valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ServingDestination forNumber(int value) {
switch (value) {
case 0: return WORKER;
case 1: return TITANIUM_SUPERVISOR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
ServingDestination> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ServingDestination findValueByNumber(int number) {
return ServingDestination.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.apphosting.base.protos.HttpPb.getDescriptor().getEnumTypes().get(0);
}
private static final ServingDestination[] VALUES = values();
public static ServingDestination valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private ServingDestination(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:java.apphosting.ServingDestination)
}
public interface ParsedHttpHeaderOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.ParsedHttpHeader)
com.google.protobuf.MessageOrBuilder {
/**
* required string key = 1;
* @return Whether the key field is set.
*/
boolean hasKey();
/**
* required string key = 1;
* @return The key.
*/
java.lang.String getKey();
/**
* required string key = 1;
* @return The bytes for key.
*/
com.google.protobuf.ByteString
getKeyBytes();
/**
* required string value = 2;
* @return Whether the value field is set.
*/
boolean hasValue();
/**
* required string value = 2;
* @return The value.
*/
java.lang.String getValue();
/**
* required string value = 2;
* @return The bytes for value.
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
*
* A parsed copy of the HTTP headers, except it doesn't have first
* line of the request.
*
*
* Protobuf type {@code java.apphosting.ParsedHttpHeader}
*/
public static final class ParsedHttpHeader extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.ParsedHttpHeader)
ParsedHttpHeaderOrBuilder {
private static final long serialVersionUID = 0L;
// Use ParsedHttpHeader.newBuilder() to construct.
private ParsedHttpHeader(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ParsedHttpHeader() {
key_ = "";
value_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ParsedHttpHeader();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_ParsedHttpHeader_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_ParsedHttpHeader_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.class, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder.class);
}
private int bitField0_;
public static final int KEY_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object key_ = "";
/**
* required string key = 1;
* @return Whether the key field is set.
*/
@java.lang.Override
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string key = 1;
* @return The key.
*/
@java.lang.Override
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
}
}
/**
* required string key = 1;
* @return The bytes for key.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object value_ = "";
/**
* required string value = 2;
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string value = 2;
* @return The value.
*/
@java.lang.Override
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* required string value = 2;
* @return The bytes for value.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasKey()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasValue()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, value_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, key_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, value_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader other = (com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader) obj;
if (hasKey() != other.hasKey()) return false;
if (hasKey()) {
if (!getKey()
.equals(other.getKey())) return false;
}
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue()
.equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A parsed copy of the HTTP headers, except it doesn't have first
* line of the request.
*
*
* Protobuf type {@code java.apphosting.ParsedHttpHeader}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.ParsedHttpHeader)
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_ParsedHttpHeader_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_ParsedHttpHeader_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.class, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder.class);
}
// Construct using com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
key_ = "";
value_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_ParsedHttpHeader_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getDefaultInstanceForType() {
return com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader build() {
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader buildPartial() {
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader result = new com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.key_ = key_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.value_ = value_;
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader) {
return mergeFrom((com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader other) {
if (other == com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.getDefaultInstance()) return this;
if (other.hasKey()) {
key_ = other.key_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasValue()) {
value_ = other.value_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasKey()) {
return false;
}
if (!hasValue()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
key_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
value_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object key_ = "";
/**
* required string key = 1;
* @return Whether the key field is set.
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string key = 1;
* @return The key.
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string key = 1;
* @return The bytes for key.
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string key = 1;
* @param value The key to set.
* @return This builder for chaining.
*/
public Builder setKey(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required string key = 1;
* @return This builder for chaining.
*/
public Builder clearKey() {
key_ = getDefaultInstance().getKey();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* required string key = 1;
* @param value The bytes for key to set.
* @return This builder for chaining.
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
key_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object value_ = "";
/**
* required string value = 2;
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* required string value = 2;
* @return The value.
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string value = 2;
* @return The bytes for value.
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* required string value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* required string value = 2;
* @param value The bytes for value to set.
* @return This builder for chaining.
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
value_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.ParsedHttpHeader)
}
// @@protoc_insertion_point(class_scope:java.apphosting.ParsedHttpHeader)
private static final com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader();
}
public static com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ParsedHttpHeader parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HttpRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.HttpRequest)
com.google.protobuf.MessageOrBuilder {
/**
* required string url = 1;
* @return Whether the url field is set.
*/
boolean hasUrl();
/**
* required string url = 1;
* @return The url.
*/
java.lang.String getUrl();
/**
* required string url = 1;
* @return The bytes for url.
*/
com.google.protobuf.ByteString
getUrlBytes();
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* optional bytes postdata = 2 [ctype = CORD];
* @return Whether the postdata field is set.
*/
boolean hasPostdata();
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* optional bytes postdata = 2 [ctype = CORD];
* @return The postdata.
*/
com.google.protobuf.ByteString getPostdata();
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
java.util.List
getHeadersList();
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getHeaders(int index);
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
int getHeadersCount();
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getHeadersOrBuilderList();
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getHeadersOrBuilder(
int index);
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return Whether the userIp field is set.
*/
boolean hasUserIp();
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return The userIp.
*/
java.lang.String getUserIp();
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return The bytes for userIp.
*/
com.google.protobuf.ByteString
getUserIpBytes();
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return Whether the serverIp field is set.
*/
boolean hasServerIp();
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return The serverIp.
*/
java.lang.String getServerIp();
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return The bytes for serverIp.
*/
com.google.protobuf.ByteString
getServerIpBytes();
/**
*
* from HTTPServerRequest
*
*
* optional bool trusted = 5 [default = false];
* @return Whether the trusted field is set.
*/
boolean hasTrusted();
/**
*
* from HTTPServerRequest
*
*
* optional bool trusted = 5 [default = false];
* @return The trusted.
*/
boolean getTrusted();
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return Whether the protocol field is set.
*/
boolean hasProtocol();
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return The protocol.
*/
java.lang.String getProtocol();
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return The bytes for protocol.
*/
com.google.protobuf.ByteString
getProtocolBytes();
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return Whether the originalRequestMethod field is set.
*/
boolean hasOriginalRequestMethod();
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return The originalRequestMethod.
*/
java.lang.String getOriginalRequestMethod();
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return The bytes for originalRequestMethod.
*/
com.google.protobuf.ByteString
getOriginalRequestMethodBytes();
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return Whether the httpVersion field is set.
*/
boolean hasHttpVersion();
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return The httpVersion.
*/
java.lang.String getHttpVersion();
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return The bytes for httpVersion.
*/
com.google.protobuf.ByteString
getHttpVersionBytes();
/**
*
* Did this request come to Google (GFE) over HTTPS/SSL rather than HTTP?
*
*
* optional bool is_https = 8 [default = false];
* @return Whether the isHttps field is set.
*/
boolean hasIsHttps();
/**
*
* Did this request come to Google (GFE) over HTTPS/SSL rather than HTTP?
*
*
* optional bool is_https = 8 [default = false];
* @return The isHttps.
*/
boolean getIsHttps();
/**
*
* The request came from Executor. Note: this also includes cron requests
* since they are sent by Executor.
*
*
* optional bool is_offline = 9 [default = false];
* @return Whether the isOffline field is set.
*/
boolean hasIsOffline();
/**
*
* The request came from Executor. Note: this also includes cron requests
* since they are sent by Executor.
*
*
* optional bool is_offline = 9 [default = false];
* @return The isOffline.
*/
boolean getIsOffline();
/**
*
* Whether this request can go on the pending queue.
*
*
* optional bool enable_pending_queue = 10 [default = true];
* @return Whether the enablePendingQueue field is set.
*/
boolean hasEnablePendingQueue();
/**
*
* Whether this request can go on the pending queue.
*
*
* optional bool enable_pending_queue = 10 [default = true];
* @return The enablePendingQueue.
*/
boolean getEnablePendingQueue();
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return Whether the appserverBns field is set.
*/
boolean hasAppserverBns();
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return The appserverBns.
*/
java.lang.String getAppserverBns();
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return The bytes for appserverBns.
*/
com.google.protobuf.ByteString
getAppserverBnsBytes();
/**
*
* If true this request will always load a new clone.
*
*
* optional bool warming_request = 12 [default = false];
* @return Whether the warmingRequest field is set.
*/
boolean hasWarmingRequest();
/**
*
* If true this request will always load a new clone.
*
*
* optional bool warming_request = 12 [default = false];
* @return The warmingRequest.
*/
boolean getWarmingRequest();
/**
*
* If set, this request was issued by an appserver and this field identifies
* the request to the appserver. An id specified by an appserver will be
* non-negative.
*
*
* optional int64 warming_request_id = 22 [default = -1];
* @return Whether the warmingRequestId field is set.
*/
boolean hasWarmingRequestId();
/**
*
* If set, this request was issued by an appserver and this field identifies
* the request to the appserver. An id specified by an appserver will be
* non-negative.
*
*
* optional int64 warming_request_id = 22 [default = -1];
* @return The warmingRequestId.
*/
long getWarmingRequestId();
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return Whether the shutdownRequestCloneId field is set.
*/
boolean hasShutdownRequestCloneId();
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return The shutdownRequestCloneId.
*/
java.lang.String getShutdownRequestCloneId();
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return The bytes for shutdownRequestCloneId.
*/
com.google.protobuf.ByteString
getShutdownRequestCloneIdBytes();
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return Whether the backgroundRequestCloneId field is set.
*/
boolean hasBackgroundRequestCloneId();
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return The backgroundRequestCloneId.
*/
java.lang.String getBackgroundRequestCloneId();
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return The bytes for backgroundRequestCloneId.
*/
com.google.protobuf.ByteString
getBackgroundRequestCloneIdBytes();
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return Whether the cloneId field is set.
*/
boolean hasCloneId();
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return The cloneId.
*/
java.lang.String getCloneId();
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return The bytes for cloneId.
*/
com.google.protobuf.ByteString
getCloneIdBytes();
/**
*
* If true, "gzip(gfe)" was stripped from the User-Agent: value in 'headers'.
*
*
* optional bool gzip_gfe = 15 [default = false];
* @return Whether the gzipGfe field is set.
*/
boolean hasGzipGfe();
/**
*
* If true, "gzip(gfe)" was stripped from the User-Agent: value in 'headers'.
*
*
* optional bool gzip_gfe = 15 [default = false];
* @return The gzipGfe.
*/
boolean getGzipGfe();
/**
*
* Component within a clone that should serve the request.
*
*
* optional .java.apphosting.ServingDestination serving_destination = 19;
* @return Whether the servingDestination field is set.
*/
boolean hasServingDestination();
/**
*
* Component within a clone that should serve the request.
*
*
* optional .java.apphosting.ServingDestination serving_destination = 19;
* @return The servingDestination.
*/
com.google.apphosting.base.protos.HttpPb.ServingDestination getServingDestination();
/**
*
* If set, this request is a QFE node selection request.
* It is a "control plane" request for:
* 1) finding an available AppServer and clone to serve the incoming
* request, so that the it can instruct the QFE to route the request
* to this "selected" clone through Node Envoy.
* 2) reserving the clone until the actual request is complete so that
* AppServer would not kill the clone early.
*
*
* optional bool qfe_node_selection_request = 23;
* @return Whether the qfeNodeSelectionRequest field is set.
*/
boolean hasQfeNodeSelectionRequest();
/**
*
* If set, this request is a QFE node selection request.
* It is a "control plane" request for:
* 1) finding an available AppServer and clone to serve the incoming
* request, so that the it can instruct the QFE to route the request
* to this "selected" clone through Node Envoy.
* 2) reserving the clone until the actual request is complete so that
* AppServer would not kill the clone early.
*
*
* optional bool qfe_node_selection_request = 23;
* @return The qfeNodeSelectionRequest.
*/
boolean getQfeNodeSelectionRequest();
}
/**
*
* Representation of an entire HTTP request, as a PB.
*
*
* Protobuf type {@code java.apphosting.HttpRequest}
*/
public static final class HttpRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.HttpRequest)
HttpRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use HttpRequest.newBuilder() to construct.
private HttpRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HttpRequest() {
url_ = "";
postdata_ = com.google.protobuf.ByteString.EMPTY;
headers_ = java.util.Collections.emptyList();
userIp_ = "";
serverIp_ = "";
protocol_ = "";
originalRequestMethod_ = "";
httpVersion_ = "HTTP/1.1";
enablePendingQueue_ = true;
appserverBns_ = "";
warmingRequestId_ = -1L;
shutdownRequestCloneId_ = "";
backgroundRequestCloneId_ = "";
cloneId_ = "";
servingDestination_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HttpRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.HttpPb.HttpRequest.class, com.google.apphosting.base.protos.HttpPb.HttpRequest.Builder.class);
}
private int bitField0_;
public static final int URL_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object url_ = "";
/**
* required string url = 1;
* @return Whether the url field is set.
*/
@java.lang.Override
public boolean hasUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string url = 1;
* @return The url.
*/
@java.lang.Override
public java.lang.String getUrl() {
java.lang.Object ref = url_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
url_ = s;
}
return s;
}
}
/**
* required string url = 1;
* @return The bytes for url.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUrlBytes() {
java.lang.Object ref = url_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
url_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POSTDATA_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString postdata_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* optional bytes postdata = 2 [ctype = CORD];
* @return Whether the postdata field is set.
*/
@java.lang.Override
public boolean hasPostdata() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* optional bytes postdata = 2 [ctype = CORD];
* @return The postdata.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPostdata() {
return postdata_;
}
public static final int HEADERS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List headers_;
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
@java.lang.Override
public java.util.List getHeadersList() {
return headers_;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getHeadersOrBuilderList() {
return headers_;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
@java.lang.Override
public int getHeadersCount() {
return headers_.size();
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getHeaders(int index) {
return headers_.get(index);
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getHeadersOrBuilder(
int index) {
return headers_.get(index);
}
public static final int USER_IP_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object userIp_ = "";
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return Whether the userIp field is set.
*/
@java.lang.Override
public boolean hasUserIp() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return The userIp.
*/
@java.lang.Override
public java.lang.String getUserIp() {
java.lang.Object ref = userIp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userIp_ = s;
}
return s;
}
}
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return The bytes for userIp.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUserIpBytes() {
java.lang.Object ref = userIp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userIp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVER_IP_FIELD_NUMBER = 16;
@SuppressWarnings("serial")
private volatile java.lang.Object serverIp_ = "";
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return Whether the serverIp field is set.
*/
@java.lang.Override
public boolean hasServerIp() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return The serverIp.
*/
@java.lang.Override
public java.lang.String getServerIp() {
java.lang.Object ref = serverIp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
serverIp_ = s;
}
return s;
}
}
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return The bytes for serverIp.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getServerIpBytes() {
java.lang.Object ref = serverIp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serverIp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRUSTED_FIELD_NUMBER = 5;
private boolean trusted_ = false;
/**
*
* from HTTPServerRequest
*
*
* optional bool trusted = 5 [default = false];
* @return Whether the trusted field is set.
*/
@java.lang.Override
public boolean hasTrusted() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* from HTTPServerRequest
*
*
* optional bool trusted = 5 [default = false];
* @return The trusted.
*/
@java.lang.Override
public boolean getTrusted() {
return trusted_;
}
public static final int PROTOCOL_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object protocol_ = "";
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return Whether the protocol field is set.
*/
@java.lang.Override
public boolean hasProtocol() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return The protocol.
*/
@java.lang.Override
public java.lang.String getProtocol() {
java.lang.Object ref = protocol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
protocol_ = s;
}
return s;
}
}
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return The bytes for protocol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProtocolBytes() {
java.lang.Object ref = protocol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ORIGINAL_REQUEST_METHOD_FIELD_NUMBER = 24;
@SuppressWarnings("serial")
private volatile java.lang.Object originalRequestMethod_ = "";
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return Whether the originalRequestMethod field is set.
*/
@java.lang.Override
public boolean hasOriginalRequestMethod() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return The originalRequestMethod.
*/
@java.lang.Override
public java.lang.String getOriginalRequestMethod() {
java.lang.Object ref = originalRequestMethod_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
originalRequestMethod_ = s;
}
return s;
}
}
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return The bytes for originalRequestMethod.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getOriginalRequestMethodBytes() {
java.lang.Object ref = originalRequestMethod_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
originalRequestMethod_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HTTP_VERSION_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object httpVersion_ = "HTTP/1.1";
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return Whether the httpVersion field is set.
*/
@java.lang.Override
public boolean hasHttpVersion() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return The httpVersion.
*/
@java.lang.Override
public java.lang.String getHttpVersion() {
java.lang.Object ref = httpVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
httpVersion_ = s;
}
return s;
}
}
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return The bytes for httpVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getHttpVersionBytes() {
java.lang.Object ref = httpVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
httpVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IS_HTTPS_FIELD_NUMBER = 8;
private boolean isHttps_ = false;
/**
*
* Did this request come to Google (GFE) over HTTPS/SSL rather than HTTP?
*
*
* optional bool is_https = 8 [default = false];
* @return Whether the isHttps field is set.
*/
@java.lang.Override
public boolean hasIsHttps() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Did this request come to Google (GFE) over HTTPS/SSL rather than HTTP?
*
*
* optional bool is_https = 8 [default = false];
* @return The isHttps.
*/
@java.lang.Override
public boolean getIsHttps() {
return isHttps_;
}
public static final int IS_OFFLINE_FIELD_NUMBER = 9;
private boolean isOffline_ = false;
/**
*
* The request came from Executor. Note: this also includes cron requests
* since they are sent by Executor.
*
*
* optional bool is_offline = 9 [default = false];
* @return Whether the isOffline field is set.
*/
@java.lang.Override
public boolean hasIsOffline() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* The request came from Executor. Note: this also includes cron requests
* since they are sent by Executor.
*
*
* optional bool is_offline = 9 [default = false];
* @return The isOffline.
*/
@java.lang.Override
public boolean getIsOffline() {
return isOffline_;
}
public static final int ENABLE_PENDING_QUEUE_FIELD_NUMBER = 10;
private boolean enablePendingQueue_ = true;
/**
*
* Whether this request can go on the pending queue.
*
*
* optional bool enable_pending_queue = 10 [default = true];
* @return Whether the enablePendingQueue field is set.
*/
@java.lang.Override
public boolean hasEnablePendingQueue() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* Whether this request can go on the pending queue.
*
*
* optional bool enable_pending_queue = 10 [default = true];
* @return The enablePendingQueue.
*/
@java.lang.Override
public boolean getEnablePendingQueue() {
return enablePendingQueue_;
}
public static final int APPSERVER_BNS_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private volatile java.lang.Object appserverBns_ = "";
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return Whether the appserverBns field is set.
*/
@java.lang.Override
public boolean hasAppserverBns() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return The appserverBns.
*/
@java.lang.Override
public java.lang.String getAppserverBns() {
java.lang.Object ref = appserverBns_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appserverBns_ = s;
}
return s;
}
}
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return The bytes for appserverBns.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAppserverBnsBytes() {
java.lang.Object ref = appserverBns_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appserverBns_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int WARMING_REQUEST_FIELD_NUMBER = 12;
private boolean warmingRequest_ = false;
/**
*
* If true this request will always load a new clone.
*
*
* optional bool warming_request = 12 [default = false];
* @return Whether the warmingRequest field is set.
*/
@java.lang.Override
public boolean hasWarmingRequest() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* If true this request will always load a new clone.
*
*
* optional bool warming_request = 12 [default = false];
* @return The warmingRequest.
*/
@java.lang.Override
public boolean getWarmingRequest() {
return warmingRequest_;
}
public static final int WARMING_REQUEST_ID_FIELD_NUMBER = 22;
private long warmingRequestId_ = -1L;
/**
*
* If set, this request was issued by an appserver and this field identifies
* the request to the appserver. An id specified by an appserver will be
* non-negative.
*
*
* optional int64 warming_request_id = 22 [default = -1];
* @return Whether the warmingRequestId field is set.
*/
@java.lang.Override
public boolean hasWarmingRequestId() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* If set, this request was issued by an appserver and this field identifies
* the request to the appserver. An id specified by an appserver will be
* non-negative.
*
*
* optional int64 warming_request_id = 22 [default = -1];
* @return The warmingRequestId.
*/
@java.lang.Override
public long getWarmingRequestId() {
return warmingRequestId_;
}
public static final int SHUTDOWN_REQUEST_CLONE_ID_FIELD_NUMBER = 17;
@SuppressWarnings("serial")
private volatile java.lang.Object shutdownRequestCloneId_ = "";
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return Whether the shutdownRequestCloneId field is set.
*/
@java.lang.Override
public boolean hasShutdownRequestCloneId() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return The shutdownRequestCloneId.
*/
@java.lang.Override
public java.lang.String getShutdownRequestCloneId() {
java.lang.Object ref = shutdownRequestCloneId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shutdownRequestCloneId_ = s;
}
return s;
}
}
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return The bytes for shutdownRequestCloneId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getShutdownRequestCloneIdBytes() {
java.lang.Object ref = shutdownRequestCloneId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shutdownRequestCloneId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BACKGROUND_REQUEST_CLONE_ID_FIELD_NUMBER = 18;
@SuppressWarnings("serial")
private volatile java.lang.Object backgroundRequestCloneId_ = "";
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return Whether the backgroundRequestCloneId field is set.
*/
@java.lang.Override
public boolean hasBackgroundRequestCloneId() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return The backgroundRequestCloneId.
*/
@java.lang.Override
public java.lang.String getBackgroundRequestCloneId() {
java.lang.Object ref = backgroundRequestCloneId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
backgroundRequestCloneId_ = s;
}
return s;
}
}
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return The bytes for backgroundRequestCloneId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBackgroundRequestCloneIdBytes() {
java.lang.Object ref = backgroundRequestCloneId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
backgroundRequestCloneId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLONE_ID_FIELD_NUMBER = 21;
@SuppressWarnings("serial")
private volatile java.lang.Object cloneId_ = "";
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return Whether the cloneId field is set.
*/
@java.lang.Override
public boolean hasCloneId() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return The cloneId.
*/
@java.lang.Override
public java.lang.String getCloneId() {
java.lang.Object ref = cloneId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cloneId_ = s;
}
return s;
}
}
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return The bytes for cloneId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCloneIdBytes() {
java.lang.Object ref = cloneId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cloneId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GZIP_GFE_FIELD_NUMBER = 15;
private boolean gzipGfe_ = false;
/**
*
* If true, "gzip(gfe)" was stripped from the User-Agent: value in 'headers'.
*
*
* optional bool gzip_gfe = 15 [default = false];
* @return Whether the gzipGfe field is set.
*/
@java.lang.Override
public boolean hasGzipGfe() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* If true, "gzip(gfe)" was stripped from the User-Agent: value in 'headers'.
*
*
* optional bool gzip_gfe = 15 [default = false];
* @return The gzipGfe.
*/
@java.lang.Override
public boolean getGzipGfe() {
return gzipGfe_;
}
public static final int SERVING_DESTINATION_FIELD_NUMBER = 19;
private int servingDestination_ = 0;
/**
*
* Component within a clone that should serve the request.
*
*
* optional .java.apphosting.ServingDestination serving_destination = 19;
* @return Whether the servingDestination field is set.
*/
@java.lang.Override public boolean hasServingDestination() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* Component within a clone that should serve the request.
*
*
* optional .java.apphosting.ServingDestination serving_destination = 19;
* @return The servingDestination.
*/
@java.lang.Override public com.google.apphosting.base.protos.HttpPb.ServingDestination getServingDestination() {
com.google.apphosting.base.protos.HttpPb.ServingDestination result = com.google.apphosting.base.protos.HttpPb.ServingDestination.forNumber(servingDestination_);
return result == null ? com.google.apphosting.base.protos.HttpPb.ServingDestination.WORKER : result;
}
public static final int QFE_NODE_SELECTION_REQUEST_FIELD_NUMBER = 23;
private boolean qfeNodeSelectionRequest_ = false;
/**
*
* If set, this request is a QFE node selection request.
* It is a "control plane" request for:
* 1) finding an available AppServer and clone to serve the incoming
* request, so that the it can instruct the QFE to route the request
* to this "selected" clone through Node Envoy.
* 2) reserving the clone until the actual request is complete so that
* AppServer would not kill the clone early.
*
*
* optional bool qfe_node_selection_request = 23;
* @return Whether the qfeNodeSelectionRequest field is set.
*/
@java.lang.Override
public boolean hasQfeNodeSelectionRequest() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
* If set, this request is a QFE node selection request.
* It is a "control plane" request for:
* 1) finding an available AppServer and clone to serve the incoming
* request, so that the it can instruct the QFE to route the request
* to this "selected" clone through Node Envoy.
* 2) reserving the clone until the actual request is complete so that
* AppServer would not kill the clone early.
*
*
* optional bool qfe_node_selection_request = 23;
* @return The qfeNodeSelectionRequest.
*/
@java.lang.Override
public boolean getQfeNodeSelectionRequest() {
return qfeNodeSelectionRequest_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasUrl()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasUserIp()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasProtocol()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getHeadersCount(); i++) {
if (!getHeaders(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, url_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(2, postdata_);
}
for (int i = 0; i < headers_.size(); i++) {
output.writeMessage(3, headers_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, userIp_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeBool(5, trusted_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, protocol_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, httpVersion_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeBool(8, isHttps_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeBool(9, isOffline_);
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeBool(10, enablePendingQueue_);
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, appserverBns_);
}
if (((bitField0_ & 0x00001000) != 0)) {
output.writeBool(12, warmingRequest_);
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeBool(15, gzipGfe_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, serverIp_);
}
if (((bitField0_ & 0x00004000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, shutdownRequestCloneId_);
}
if (((bitField0_ & 0x00008000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 18, backgroundRequestCloneId_);
}
if (((bitField0_ & 0x00040000) != 0)) {
output.writeEnum(19, servingDestination_);
}
if (((bitField0_ & 0x00010000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, cloneId_);
}
if (((bitField0_ & 0x00002000) != 0)) {
output.writeInt64(22, warmingRequestId_);
}
if (((bitField0_ & 0x00080000) != 0)) {
output.writeBool(23, qfeNodeSelectionRequest_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 24, originalRequestMethod_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, url_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, postdata_);
}
for (int i = 0; i < headers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, headers_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, userIp_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, trusted_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, protocol_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, httpVersion_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, isHttps_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(9, isOffline_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(10, enablePendingQueue_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, appserverBns_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(12, warmingRequest_);
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, gzipGfe_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, serverIp_);
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, shutdownRequestCloneId_);
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(18, backgroundRequestCloneId_);
}
if (((bitField0_ & 0x00040000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(19, servingDestination_);
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, cloneId_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(22, warmingRequestId_);
}
if (((bitField0_ & 0x00080000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(23, qfeNodeSelectionRequest_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(24, originalRequestMethod_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.HttpPb.HttpRequest)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.HttpPb.HttpRequest other = (com.google.apphosting.base.protos.HttpPb.HttpRequest) obj;
if (hasUrl() != other.hasUrl()) return false;
if (hasUrl()) {
if (!getUrl()
.equals(other.getUrl())) return false;
}
if (hasPostdata() != other.hasPostdata()) return false;
if (hasPostdata()) {
if (!getPostdata()
.equals(other.getPostdata())) return false;
}
if (!getHeadersList()
.equals(other.getHeadersList())) return false;
if (hasUserIp() != other.hasUserIp()) return false;
if (hasUserIp()) {
if (!getUserIp()
.equals(other.getUserIp())) return false;
}
if (hasServerIp() != other.hasServerIp()) return false;
if (hasServerIp()) {
if (!getServerIp()
.equals(other.getServerIp())) return false;
}
if (hasTrusted() != other.hasTrusted()) return false;
if (hasTrusted()) {
if (getTrusted()
!= other.getTrusted()) return false;
}
if (hasProtocol() != other.hasProtocol()) return false;
if (hasProtocol()) {
if (!getProtocol()
.equals(other.getProtocol())) return false;
}
if (hasOriginalRequestMethod() != other.hasOriginalRequestMethod()) return false;
if (hasOriginalRequestMethod()) {
if (!getOriginalRequestMethod()
.equals(other.getOriginalRequestMethod())) return false;
}
if (hasHttpVersion() != other.hasHttpVersion()) return false;
if (hasHttpVersion()) {
if (!getHttpVersion()
.equals(other.getHttpVersion())) return false;
}
if (hasIsHttps() != other.hasIsHttps()) return false;
if (hasIsHttps()) {
if (getIsHttps()
!= other.getIsHttps()) return false;
}
if (hasIsOffline() != other.hasIsOffline()) return false;
if (hasIsOffline()) {
if (getIsOffline()
!= other.getIsOffline()) return false;
}
if (hasEnablePendingQueue() != other.hasEnablePendingQueue()) return false;
if (hasEnablePendingQueue()) {
if (getEnablePendingQueue()
!= other.getEnablePendingQueue()) return false;
}
if (hasAppserverBns() != other.hasAppserverBns()) return false;
if (hasAppserverBns()) {
if (!getAppserverBns()
.equals(other.getAppserverBns())) return false;
}
if (hasWarmingRequest() != other.hasWarmingRequest()) return false;
if (hasWarmingRequest()) {
if (getWarmingRequest()
!= other.getWarmingRequest()) return false;
}
if (hasWarmingRequestId() != other.hasWarmingRequestId()) return false;
if (hasWarmingRequestId()) {
if (getWarmingRequestId()
!= other.getWarmingRequestId()) return false;
}
if (hasShutdownRequestCloneId() != other.hasShutdownRequestCloneId()) return false;
if (hasShutdownRequestCloneId()) {
if (!getShutdownRequestCloneId()
.equals(other.getShutdownRequestCloneId())) return false;
}
if (hasBackgroundRequestCloneId() != other.hasBackgroundRequestCloneId()) return false;
if (hasBackgroundRequestCloneId()) {
if (!getBackgroundRequestCloneId()
.equals(other.getBackgroundRequestCloneId())) return false;
}
if (hasCloneId() != other.hasCloneId()) return false;
if (hasCloneId()) {
if (!getCloneId()
.equals(other.getCloneId())) return false;
}
if (hasGzipGfe() != other.hasGzipGfe()) return false;
if (hasGzipGfe()) {
if (getGzipGfe()
!= other.getGzipGfe()) return false;
}
if (hasServingDestination() != other.hasServingDestination()) return false;
if (hasServingDestination()) {
if (servingDestination_ != other.servingDestination_) return false;
}
if (hasQfeNodeSelectionRequest() != other.hasQfeNodeSelectionRequest()) return false;
if (hasQfeNodeSelectionRequest()) {
if (getQfeNodeSelectionRequest()
!= other.getQfeNodeSelectionRequest()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasUrl()) {
hash = (37 * hash) + URL_FIELD_NUMBER;
hash = (53 * hash) + getUrl().hashCode();
}
if (hasPostdata()) {
hash = (37 * hash) + POSTDATA_FIELD_NUMBER;
hash = (53 * hash) + getPostdata().hashCode();
}
if (getHeadersCount() > 0) {
hash = (37 * hash) + HEADERS_FIELD_NUMBER;
hash = (53 * hash) + getHeadersList().hashCode();
}
if (hasUserIp()) {
hash = (37 * hash) + USER_IP_FIELD_NUMBER;
hash = (53 * hash) + getUserIp().hashCode();
}
if (hasServerIp()) {
hash = (37 * hash) + SERVER_IP_FIELD_NUMBER;
hash = (53 * hash) + getServerIp().hashCode();
}
if (hasTrusted()) {
hash = (37 * hash) + TRUSTED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTrusted());
}
if (hasProtocol()) {
hash = (37 * hash) + PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + getProtocol().hashCode();
}
if (hasOriginalRequestMethod()) {
hash = (37 * hash) + ORIGINAL_REQUEST_METHOD_FIELD_NUMBER;
hash = (53 * hash) + getOriginalRequestMethod().hashCode();
}
if (hasHttpVersion()) {
hash = (37 * hash) + HTTP_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getHttpVersion().hashCode();
}
if (hasIsHttps()) {
hash = (37 * hash) + IS_HTTPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsHttps());
}
if (hasIsOffline()) {
hash = (37 * hash) + IS_OFFLINE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsOffline());
}
if (hasEnablePendingQueue()) {
hash = (37 * hash) + ENABLE_PENDING_QUEUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getEnablePendingQueue());
}
if (hasAppserverBns()) {
hash = (37 * hash) + APPSERVER_BNS_FIELD_NUMBER;
hash = (53 * hash) + getAppserverBns().hashCode();
}
if (hasWarmingRequest()) {
hash = (37 * hash) + WARMING_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getWarmingRequest());
}
if (hasWarmingRequestId()) {
hash = (37 * hash) + WARMING_REQUEST_ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getWarmingRequestId());
}
if (hasShutdownRequestCloneId()) {
hash = (37 * hash) + SHUTDOWN_REQUEST_CLONE_ID_FIELD_NUMBER;
hash = (53 * hash) + getShutdownRequestCloneId().hashCode();
}
if (hasBackgroundRequestCloneId()) {
hash = (37 * hash) + BACKGROUND_REQUEST_CLONE_ID_FIELD_NUMBER;
hash = (53 * hash) + getBackgroundRequestCloneId().hashCode();
}
if (hasCloneId()) {
hash = (37 * hash) + CLONE_ID_FIELD_NUMBER;
hash = (53 * hash) + getCloneId().hashCode();
}
if (hasGzipGfe()) {
hash = (37 * hash) + GZIP_GFE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getGzipGfe());
}
if (hasServingDestination()) {
hash = (37 * hash) + SERVING_DESTINATION_FIELD_NUMBER;
hash = (53 * hash) + servingDestination_;
}
if (hasQfeNodeSelectionRequest()) {
hash = (37 * hash) + QFE_NODE_SELECTION_REQUEST_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getQfeNodeSelectionRequest());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.HttpPb.HttpRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Representation of an entire HTTP request, as a PB.
*
*
* Protobuf type {@code java.apphosting.HttpRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.HttpRequest)
com.google.apphosting.base.protos.HttpPb.HttpRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.HttpPb.HttpRequest.class, com.google.apphosting.base.protos.HttpPb.HttpRequest.Builder.class);
}
// Construct using com.google.apphosting.base.protos.HttpPb.HttpRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
url_ = "";
postdata_ = com.google.protobuf.ByteString.EMPTY;
if (headersBuilder_ == null) {
headers_ = java.util.Collections.emptyList();
} else {
headers_ = null;
headersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
userIp_ = "";
serverIp_ = "";
trusted_ = false;
protocol_ = "";
originalRequestMethod_ = "";
httpVersion_ = "HTTP/1.1";
isHttps_ = false;
isOffline_ = false;
enablePendingQueue_ = true;
appserverBns_ = "";
warmingRequest_ = false;
warmingRequestId_ = -1L;
shutdownRequestCloneId_ = "";
backgroundRequestCloneId_ = "";
cloneId_ = "";
gzipGfe_ = false;
servingDestination_ = 0;
qfeNodeSelectionRequest_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpRequest_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.HttpRequest getDefaultInstanceForType() {
return com.google.apphosting.base.protos.HttpPb.HttpRequest.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.HttpRequest build() {
com.google.apphosting.base.protos.HttpPb.HttpRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.HttpRequest buildPartial() {
com.google.apphosting.base.protos.HttpPb.HttpRequest result = new com.google.apphosting.base.protos.HttpPb.HttpRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.base.protos.HttpPb.HttpRequest result) {
if (headersBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
headers_ = java.util.Collections.unmodifiableList(headers_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.headers_ = headers_;
} else {
result.headers_ = headersBuilder_.build();
}
}
private void buildPartial0(com.google.apphosting.base.protos.HttpPb.HttpRequest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.url_ = url_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.postdata_ = postdata_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.userIp_ = userIp_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.serverIp_ = serverIp_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.trusted_ = trusted_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.protocol_ = protocol_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.originalRequestMethod_ = originalRequestMethod_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.httpVersion_ = httpVersion_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.isHttps_ = isHttps_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.isOffline_ = isOffline_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.enablePendingQueue_ = enablePendingQueue_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.appserverBns_ = appserverBns_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.warmingRequest_ = warmingRequest_;
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.warmingRequestId_ = warmingRequestId_;
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.shutdownRequestCloneId_ = shutdownRequestCloneId_;
to_bitField0_ |= 0x00004000;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.backgroundRequestCloneId_ = backgroundRequestCloneId_;
to_bitField0_ |= 0x00008000;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.cloneId_ = cloneId_;
to_bitField0_ |= 0x00010000;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.gzipGfe_ = gzipGfe_;
to_bitField0_ |= 0x00020000;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.servingDestination_ = servingDestination_;
to_bitField0_ |= 0x00040000;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.qfeNodeSelectionRequest_ = qfeNodeSelectionRequest_;
to_bitField0_ |= 0x00080000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.HttpPb.HttpRequest) {
return mergeFrom((com.google.apphosting.base.protos.HttpPb.HttpRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.HttpPb.HttpRequest other) {
if (other == com.google.apphosting.base.protos.HttpPb.HttpRequest.getDefaultInstance()) return this;
if (other.hasUrl()) {
url_ = other.url_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasPostdata()) {
setPostdata(other.getPostdata());
}
if (headersBuilder_ == null) {
if (!other.headers_.isEmpty()) {
if (headers_.isEmpty()) {
headers_ = other.headers_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureHeadersIsMutable();
headers_.addAll(other.headers_);
}
onChanged();
}
} else {
if (!other.headers_.isEmpty()) {
if (headersBuilder_.isEmpty()) {
headersBuilder_.dispose();
headersBuilder_ = null;
headers_ = other.headers_;
bitField0_ = (bitField0_ & ~0x00000004);
headersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getHeadersFieldBuilder() : null;
} else {
headersBuilder_.addAllMessages(other.headers_);
}
}
}
if (other.hasUserIp()) {
userIp_ = other.userIp_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasServerIp()) {
serverIp_ = other.serverIp_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasTrusted()) {
setTrusted(other.getTrusted());
}
if (other.hasProtocol()) {
protocol_ = other.protocol_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasOriginalRequestMethod()) {
originalRequestMethod_ = other.originalRequestMethod_;
bitField0_ |= 0x00000080;
onChanged();
}
if (other.hasHttpVersion()) {
httpVersion_ = other.httpVersion_;
bitField0_ |= 0x00000100;
onChanged();
}
if (other.hasIsHttps()) {
setIsHttps(other.getIsHttps());
}
if (other.hasIsOffline()) {
setIsOffline(other.getIsOffline());
}
if (other.hasEnablePendingQueue()) {
setEnablePendingQueue(other.getEnablePendingQueue());
}
if (other.hasAppserverBns()) {
appserverBns_ = other.appserverBns_;
bitField0_ |= 0x00001000;
onChanged();
}
if (other.hasWarmingRequest()) {
setWarmingRequest(other.getWarmingRequest());
}
if (other.hasWarmingRequestId()) {
setWarmingRequestId(other.getWarmingRequestId());
}
if (other.hasShutdownRequestCloneId()) {
shutdownRequestCloneId_ = other.shutdownRequestCloneId_;
bitField0_ |= 0x00008000;
onChanged();
}
if (other.hasBackgroundRequestCloneId()) {
backgroundRequestCloneId_ = other.backgroundRequestCloneId_;
bitField0_ |= 0x00010000;
onChanged();
}
if (other.hasCloneId()) {
cloneId_ = other.cloneId_;
bitField0_ |= 0x00020000;
onChanged();
}
if (other.hasGzipGfe()) {
setGzipGfe(other.getGzipGfe());
}
if (other.hasServingDestination()) {
setServingDestination(other.getServingDestination());
}
if (other.hasQfeNodeSelectionRequest()) {
setQfeNodeSelectionRequest(other.getQfeNodeSelectionRequest());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasUrl()) {
return false;
}
if (!hasUserIp()) {
return false;
}
if (!hasProtocol()) {
return false;
}
for (int i = 0; i < getHeadersCount(); i++) {
if (!getHeaders(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
url_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
postdata_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader m =
input.readMessage(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.PARSER,
extensionRegistry);
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.add(m);
} else {
headersBuilder_.addMessage(m);
}
break;
} // case 26
case 34: {
userIp_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 34
case 40: {
trusted_ = input.readBool();
bitField0_ |= 0x00000020;
break;
} // case 40
case 50: {
protocol_ = input.readBytes();
bitField0_ |= 0x00000040;
break;
} // case 50
case 58: {
httpVersion_ = input.readBytes();
bitField0_ |= 0x00000100;
break;
} // case 58
case 64: {
isHttps_ = input.readBool();
bitField0_ |= 0x00000200;
break;
} // case 64
case 72: {
isOffline_ = input.readBool();
bitField0_ |= 0x00000400;
break;
} // case 72
case 80: {
enablePendingQueue_ = input.readBool();
bitField0_ |= 0x00000800;
break;
} // case 80
case 90: {
appserverBns_ = input.readBytes();
bitField0_ |= 0x00001000;
break;
} // case 90
case 96: {
warmingRequest_ = input.readBool();
bitField0_ |= 0x00002000;
break;
} // case 96
case 120: {
gzipGfe_ = input.readBool();
bitField0_ |= 0x00040000;
break;
} // case 120
case 130: {
serverIp_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 130
case 138: {
shutdownRequestCloneId_ = input.readBytes();
bitField0_ |= 0x00008000;
break;
} // case 138
case 146: {
backgroundRequestCloneId_ = input.readBytes();
bitField0_ |= 0x00010000;
break;
} // case 146
case 152: {
int tmpRaw = input.readEnum();
com.google.apphosting.base.protos.HttpPb.ServingDestination tmpValue =
com.google.apphosting.base.protos.HttpPb.ServingDestination.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(19, tmpRaw);
} else {
servingDestination_ = tmpRaw;
bitField0_ |= 0x00080000;
}
break;
} // case 152
case 170: {
cloneId_ = input.readBytes();
bitField0_ |= 0x00020000;
break;
} // case 170
case 176: {
warmingRequestId_ = input.readInt64();
bitField0_ |= 0x00004000;
break;
} // case 176
case 184: {
qfeNodeSelectionRequest_ = input.readBool();
bitField0_ |= 0x00100000;
break;
} // case 184
case 194: {
originalRequestMethod_ = input.readBytes();
bitField0_ |= 0x00000080;
break;
} // case 194
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object url_ = "";
/**
* required string url = 1;
* @return Whether the url field is set.
*/
public boolean hasUrl() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* required string url = 1;
* @return The url.
*/
public java.lang.String getUrl() {
java.lang.Object ref = url_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
url_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* required string url = 1;
* @return The bytes for url.
*/
public com.google.protobuf.ByteString
getUrlBytes() {
java.lang.Object ref = url_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
url_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* required string url = 1;
* @param value The url to set.
* @return This builder for chaining.
*/
public Builder setUrl(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
url_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* required string url = 1;
* @return This builder for chaining.
*/
public Builder clearUrl() {
url_ = getDefaultInstance().getUrl();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* required string url = 1;
* @param value The bytes for url to set.
* @return This builder for chaining.
*/
public Builder setUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
url_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.ByteString postdata_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* optional bytes postdata = 2 [ctype = CORD];
* @return Whether the postdata field is set.
*/
@java.lang.Override
public boolean hasPostdata() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* optional bytes postdata = 2 [ctype = CORD];
* @return The postdata.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPostdata() {
return postdata_;
}
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* optional bytes postdata = 2 [ctype = CORD];
* @param value The postdata to set.
* @return This builder for chaining.
*/
public Builder setPostdata(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
postdata_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* optional bytes postdata = 2 [ctype = CORD];
* @return This builder for chaining.
*/
public Builder clearPostdata() {
bitField0_ = (bitField0_ & ~0x00000002);
postdata_ = getDefaultInstance().getPostdata();
onChanged();
return this;
}
private java.util.List headers_ =
java.util.Collections.emptyList();
private void ensureHeadersIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
headers_ = new java.util.ArrayList(headers_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder> headersBuilder_;
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public java.util.List getHeadersList() {
if (headersBuilder_ == null) {
return java.util.Collections.unmodifiableList(headers_);
} else {
return headersBuilder_.getMessageList();
}
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public int getHeadersCount() {
if (headersBuilder_ == null) {
return headers_.size();
} else {
return headersBuilder_.getCount();
}
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getHeaders(int index) {
if (headersBuilder_ == null) {
return headers_.get(index);
} else {
return headersBuilder_.getMessage(index);
}
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder setHeaders(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (headersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeadersIsMutable();
headers_.set(index, value);
onChanged();
} else {
headersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder setHeaders(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.set(index, builderForValue.build());
onChanged();
} else {
headersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder addHeaders(com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (headersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeadersIsMutable();
headers_.add(value);
onChanged();
} else {
headersBuilder_.addMessage(value);
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder addHeaders(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (headersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHeadersIsMutable();
headers_.add(index, value);
onChanged();
} else {
headersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder addHeaders(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.add(builderForValue.build());
onChanged();
} else {
headersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder addHeaders(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.add(index, builderForValue.build());
onChanged();
} else {
headersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder addAllHeaders(
java.lang.Iterable extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader> values) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, headers_);
onChanged();
} else {
headersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder clearHeaders() {
if (headersBuilder_ == null) {
headers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
headersBuilder_.clear();
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public Builder removeHeaders(int index) {
if (headersBuilder_ == null) {
ensureHeadersIsMutable();
headers_.remove(index);
onChanged();
} else {
headersBuilder_.remove(index);
}
return this;
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder getHeadersBuilder(
int index) {
return getHeadersFieldBuilder().getBuilder(index);
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getHeadersOrBuilder(
int index) {
if (headersBuilder_ == null) {
return headers_.get(index); } else {
return headersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getHeadersOrBuilderList() {
if (headersBuilder_ != null) {
return headersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(headers_);
}
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder addHeadersBuilder() {
return getHeadersFieldBuilder().addBuilder(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.getDefaultInstance());
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder addHeadersBuilder(
int index) {
return getHeadersFieldBuilder().addBuilder(
index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.getDefaultInstance());
}
/**
*
* The headers included here are only the "safe" headers which may
* be exposed to client code. The headers should not contain
* Google-specific headers which may not be secure for client
* access.
* Note that the "Host" header is required/mandatory for HTTP/1.1
*
*
* repeated .java.apphosting.ParsedHttpHeader headers = 3;
*/
public java.util.List
getHeadersBuilderList() {
return getHeadersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getHeadersFieldBuilder() {
if (headersBuilder_ == null) {
headersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>(
headers_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
headers_ = null;
}
return headersBuilder_;
}
private java.lang.Object userIp_ = "";
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return Whether the userIp field is set.
*/
public boolean hasUserIp() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return The userIp.
*/
public java.lang.String getUserIp() {
java.lang.Object ref = userIp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
userIp_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return The bytes for userIp.
*/
public com.google.protobuf.ByteString
getUserIpBytes() {
java.lang.Object ref = userIp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userIp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @param value The userIp to set.
* @return This builder for chaining.
*/
public Builder setUserIp(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
userIp_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @return This builder for chaining.
*/
public Builder clearUserIp() {
userIp_ = getDefaultInstance().getUserIp();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* filled in from X-User-Ip
*
*
* required string user_ip = 4;
* @param value The bytes for userIp to set.
* @return This builder for chaining.
*/
public Builder setUserIpBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
userIp_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object serverIp_ = "";
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return Whether the serverIp field is set.
*/
public boolean hasServerIp() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return The serverIp.
*/
public java.lang.String getServerIp() {
java.lang.Object ref = serverIp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
serverIp_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return The bytes for serverIp.
*/
public com.google.protobuf.ByteString
getServerIpBytes() {
java.lang.Object ref = serverIp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serverIp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @param value The serverIp to set.
* @return This builder for chaining.
*/
public Builder setServerIp(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
serverIp_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @return This builder for chaining.
*/
public Builder clearServerIp() {
serverIp_ = getDefaultInstance().getServerIp();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* filled from X-Google-GFE-Frontline-Info
*
*
* optional string server_ip = 16;
* @param value The bytes for serverIp to set.
* @return This builder for chaining.
*/
public Builder setServerIpBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
serverIp_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private boolean trusted_ ;
/**
*
* from HTTPServerRequest
*
*
* optional bool trusted = 5 [default = false];
* @return Whether the trusted field is set.
*/
@java.lang.Override
public boolean hasTrusted() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* from HTTPServerRequest
*
*
* optional bool trusted = 5 [default = false];
* @return The trusted.
*/
@java.lang.Override
public boolean getTrusted() {
return trusted_;
}
/**
*
* from HTTPServerRequest
*
*
* optional bool trusted = 5 [default = false];
* @param value The trusted to set.
* @return This builder for chaining.
*/
public Builder setTrusted(boolean value) {
trusted_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* from HTTPServerRequest
*
*
* optional bool trusted = 5 [default = false];
* @return This builder for chaining.
*/
public Builder clearTrusted() {
bitField0_ = (bitField0_ & ~0x00000020);
trusted_ = false;
onChanged();
return this;
}
private java.lang.Object protocol_ = "";
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return Whether the protocol field is set.
*/
public boolean hasProtocol() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return The protocol.
*/
public java.lang.String getProtocol() {
java.lang.Object ref = protocol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
protocol_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return The bytes for protocol.
*/
public com.google.protobuf.ByteString
getProtocolBytes() {
java.lang.Object ref = protocol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Request method.
*
*
* required string protocol = 6;
* @param value The protocol to set.
* @return This builder for chaining.
*/
public Builder setProtocol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
protocol_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Request method.
*
*
* required string protocol = 6;
* @return This builder for chaining.
*/
public Builder clearProtocol() {
protocol_ = getDefaultInstance().getProtocol();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* Request method.
*
*
* required string protocol = 6;
* @param value The bytes for protocol to set.
* @return This builder for chaining.
*/
public Builder setProtocolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
protocol_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object originalRequestMethod_ = "";
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return Whether the originalRequestMethod field is set.
*/
public boolean hasOriginalRequestMethod() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return The originalRequestMethod.
*/
public java.lang.String getOriginalRequestMethod() {
java.lang.Object ref = originalRequestMethod_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
originalRequestMethod_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return The bytes for originalRequestMethod.
*/
public com.google.protobuf.ByteString
getOriginalRequestMethodBytes() {
java.lang.Object ref = originalRequestMethod_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
originalRequestMethod_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @param value The originalRequestMethod to set.
* @return This builder for chaining.
*/
public Builder setOriginalRequestMethod(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
originalRequestMethod_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @return This builder for chaining.
*/
public Builder clearOriginalRequestMethod() {
originalRequestMethod_ = getDefaultInstance().getOriginalRequestMethod();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
* Original request method.
* In some cases, request method could be overridden, for example, for
* a QFE node selection request, protocol is always set to GET. Therefore,
* this field is used to record the original request method.
*
*
* optional string original_request_method = 24;
* @param value The bytes for originalRequestMethod to set.
* @return This builder for chaining.
*/
public Builder setOriginalRequestMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
originalRequestMethod_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private java.lang.Object httpVersion_ = "HTTP/1.1";
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return Whether the httpVersion field is set.
*/
public boolean hasHttpVersion() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return The httpVersion.
*/
public java.lang.String getHttpVersion() {
java.lang.Object ref = httpVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
httpVersion_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return The bytes for httpVersion.
*/
public com.google.protobuf.ByteString
getHttpVersionBytes() {
java.lang.Object ref = httpVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
httpVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @param value The httpVersion to set.
* @return This builder for chaining.
*/
public Builder setHttpVersion(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
httpVersion_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @return This builder for chaining.
*/
public Builder clearHttpVersion() {
httpVersion_ = getDefaultInstance().getHttpVersion();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
* optional string http_version = 7 [default = "HTTP/1.1"];
* @param value The bytes for httpVersion to set.
* @return This builder for chaining.
*/
public Builder setHttpVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
httpVersion_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private boolean isHttps_ ;
/**
*
* Did this request come to Google (GFE) over HTTPS/SSL rather than HTTP?
*
*
* optional bool is_https = 8 [default = false];
* @return Whether the isHttps field is set.
*/
@java.lang.Override
public boolean hasIsHttps() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* Did this request come to Google (GFE) over HTTPS/SSL rather than HTTP?
*
*
* optional bool is_https = 8 [default = false];
* @return The isHttps.
*/
@java.lang.Override
public boolean getIsHttps() {
return isHttps_;
}
/**
*
* Did this request come to Google (GFE) over HTTPS/SSL rather than HTTP?
*
*
* optional bool is_https = 8 [default = false];
* @param value The isHttps to set.
* @return This builder for chaining.
*/
public Builder setIsHttps(boolean value) {
isHttps_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Did this request come to Google (GFE) over HTTPS/SSL rather than HTTP?
*
*
* optional bool is_https = 8 [default = false];
* @return This builder for chaining.
*/
public Builder clearIsHttps() {
bitField0_ = (bitField0_ & ~0x00000200);
isHttps_ = false;
onChanged();
return this;
}
private boolean isOffline_ ;
/**
*
* The request came from Executor. Note: this also includes cron requests
* since they are sent by Executor.
*
*
* optional bool is_offline = 9 [default = false];
* @return Whether the isOffline field is set.
*/
@java.lang.Override
public boolean hasIsOffline() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* The request came from Executor. Note: this also includes cron requests
* since they are sent by Executor.
*
*
* optional bool is_offline = 9 [default = false];
* @return The isOffline.
*/
@java.lang.Override
public boolean getIsOffline() {
return isOffline_;
}
/**
*
* The request came from Executor. Note: this also includes cron requests
* since they are sent by Executor.
*
*
* optional bool is_offline = 9 [default = false];
* @param value The isOffline to set.
* @return This builder for chaining.
*/
public Builder setIsOffline(boolean value) {
isOffline_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* The request came from Executor. Note: this also includes cron requests
* since they are sent by Executor.
*
*
* optional bool is_offline = 9 [default = false];
* @return This builder for chaining.
*/
public Builder clearIsOffline() {
bitField0_ = (bitField0_ & ~0x00000400);
isOffline_ = false;
onChanged();
return this;
}
private boolean enablePendingQueue_ = true;
/**
*
* Whether this request can go on the pending queue.
*
*
* optional bool enable_pending_queue = 10 [default = true];
* @return Whether the enablePendingQueue field is set.
*/
@java.lang.Override
public boolean hasEnablePendingQueue() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* Whether this request can go on the pending queue.
*
*
* optional bool enable_pending_queue = 10 [default = true];
* @return The enablePendingQueue.
*/
@java.lang.Override
public boolean getEnablePendingQueue() {
return enablePendingQueue_;
}
/**
*
* Whether this request can go on the pending queue.
*
*
* optional bool enable_pending_queue = 10 [default = true];
* @param value The enablePendingQueue to set.
* @return This builder for chaining.
*/
public Builder setEnablePendingQueue(boolean value) {
enablePendingQueue_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* Whether this request can go on the pending queue.
*
*
* optional bool enable_pending_queue = 10 [default = true];
* @return This builder for chaining.
*/
public Builder clearEnablePendingQueue() {
bitField0_ = (bitField0_ & ~0x00000800);
enablePendingQueue_ = true;
onChanged();
return this;
}
private java.lang.Object appserverBns_ = "";
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return Whether the appserverBns field is set.
*/
public boolean hasAppserverBns() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return The appserverBns.
*/
public java.lang.String getAppserverBns() {
java.lang.Object ref = appserverBns_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
appserverBns_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return The bytes for appserverBns.
*/
public com.google.protobuf.ByteString
getAppserverBnsBytes() {
java.lang.Object ref = appserverBns_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
appserverBns_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @param value The appserverBns to set.
* @return This builder for chaining.
*/
public Builder setAppserverBns(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
appserverBns_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @return This builder for chaining.
*/
public Builder clearAppserverBns() {
appserverBns_ = getDefaultInstance().getAppserverBns();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
* If set, the appserver which should handle this request.
* NOTE: While the name of this field is 'appserver_bns', it will only
* be a bns task address for appmaster1 apps. For appmaster2 apps, this will
* be the appserver's gns address.
*
*
* optional string appserver_bns = 11;
* @param value The bytes for appserverBns to set.
* @return This builder for chaining.
*/
public Builder setAppserverBnsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
appserverBns_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
private boolean warmingRequest_ ;
/**
*
* If true this request will always load a new clone.
*
*
* optional bool warming_request = 12 [default = false];
* @return Whether the warmingRequest field is set.
*/
@java.lang.Override
public boolean hasWarmingRequest() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* If true this request will always load a new clone.
*
*
* optional bool warming_request = 12 [default = false];
* @return The warmingRequest.
*/
@java.lang.Override
public boolean getWarmingRequest() {
return warmingRequest_;
}
/**
*
* If true this request will always load a new clone.
*
*
* optional bool warming_request = 12 [default = false];
* @param value The warmingRequest to set.
* @return This builder for chaining.
*/
public Builder setWarmingRequest(boolean value) {
warmingRequest_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* If true this request will always load a new clone.
*
*
* optional bool warming_request = 12 [default = false];
* @return This builder for chaining.
*/
public Builder clearWarmingRequest() {
bitField0_ = (bitField0_ & ~0x00002000);
warmingRequest_ = false;
onChanged();
return this;
}
private long warmingRequestId_ = -1L;
/**
*
* If set, this request was issued by an appserver and this field identifies
* the request to the appserver. An id specified by an appserver will be
* non-negative.
*
*
* optional int64 warming_request_id = 22 [default = -1];
* @return Whether the warmingRequestId field is set.
*/
@java.lang.Override
public boolean hasWarmingRequestId() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* If set, this request was issued by an appserver and this field identifies
* the request to the appserver. An id specified by an appserver will be
* non-negative.
*
*
* optional int64 warming_request_id = 22 [default = -1];
* @return The warmingRequestId.
*/
@java.lang.Override
public long getWarmingRequestId() {
return warmingRequestId_;
}
/**
*
* If set, this request was issued by an appserver and this field identifies
* the request to the appserver. An id specified by an appserver will be
* non-negative.
*
*
* optional int64 warming_request_id = 22 [default = -1];
* @param value The warmingRequestId to set.
* @return This builder for chaining.
*/
public Builder setWarmingRequestId(long value) {
warmingRequestId_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* If set, this request was issued by an appserver and this field identifies
* the request to the appserver. An id specified by an appserver will be
* non-negative.
*
*
* optional int64 warming_request_id = 22 [default = -1];
* @return This builder for chaining.
*/
public Builder clearWarmingRequestId() {
bitField0_ = (bitField0_ & ~0x00004000);
warmingRequestId_ = -1L;
onChanged();
return this;
}
private java.lang.Object shutdownRequestCloneId_ = "";
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return Whether the shutdownRequestCloneId field is set.
*/
public boolean hasShutdownRequestCloneId() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return The shutdownRequestCloneId.
*/
public java.lang.String getShutdownRequestCloneId() {
java.lang.Object ref = shutdownRequestCloneId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shutdownRequestCloneId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return The bytes for shutdownRequestCloneId.
*/
public com.google.protobuf.ByteString
getShutdownRequestCloneIdBytes() {
java.lang.Object ref = shutdownRequestCloneId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shutdownRequestCloneId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @param value The shutdownRequestCloneId to set.
* @return This builder for chaining.
*/
public Builder setShutdownRequestCloneId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
shutdownRequestCloneId_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @return This builder for chaining.
*/
public Builder clearShutdownRequestCloneId() {
shutdownRequestCloneId_ = getDefaultInstance().getShutdownRequestCloneId();
bitField0_ = (bitField0_ & ~0x00008000);
onChanged();
return this;
}
/**
*
* If set, the request will shutdown the clone with clone_id set in
* shutdown_clone_id.
*
*
* optional string shutdown_request_clone_id = 17;
* @param value The bytes for shutdownRequestCloneId to set.
* @return This builder for chaining.
*/
public Builder setShutdownRequestCloneIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
shutdownRequestCloneId_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
private java.lang.Object backgroundRequestCloneId_ = "";
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return Whether the backgroundRequestCloneId field is set.
*/
public boolean hasBackgroundRequestCloneId() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return The backgroundRequestCloneId.
*/
public java.lang.String getBackgroundRequestCloneId() {
java.lang.Object ref = backgroundRequestCloneId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
backgroundRequestCloneId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return The bytes for backgroundRequestCloneId.
*/
public com.google.protobuf.ByteString
getBackgroundRequestCloneIdBytes() {
java.lang.Object ref = backgroundRequestCloneId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
backgroundRequestCloneId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @param value The backgroundRequestCloneId to set.
* @return This builder for chaining.
*/
public Builder setBackgroundRequestCloneId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
backgroundRequestCloneId_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @return This builder for chaining.
*/
public Builder clearBackgroundRequestCloneId() {
backgroundRequestCloneId_ = getDefaultInstance().getBackgroundRequestCloneId();
bitField0_ = (bitField0_ & ~0x00010000);
onChanged();
return this;
}
/**
*
* If set, this is a /_ah/background request that will be sent
* to the clone with clone_id set in shutdown_clone_id.
*
*
* optional string background_request_clone_id = 18;
* @param value The bytes for backgroundRequestCloneId to set.
* @return This builder for chaining.
*/
public Builder setBackgroundRequestCloneIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
backgroundRequestCloneId_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
private java.lang.Object cloneId_ = "";
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return Whether the cloneId field is set.
*/
public boolean hasCloneId() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return The cloneId.
*/
public java.lang.String getCloneId() {
java.lang.Object ref = cloneId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
cloneId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return The bytes for cloneId.
*/
public com.google.protobuf.ByteString
getCloneIdBytes() {
java.lang.Object ref = cloneId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cloneId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @param value The cloneId to set.
* @return This builder for chaining.
*/
public Builder setCloneId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
cloneId_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @return This builder for chaining.
*/
public Builder clearCloneId() {
cloneId_ = getDefaultInstance().getCloneId();
bitField0_ = (bitField0_ & ~0x00020000);
onChanged();
return this;
}
/**
*
* If set, this request will be sent to the clone with this clone_id. This
* field is used by Appserver to send /_ah/.* requests to a particular clone.
* The AppHttpRequest.clone_key is also used to route the request to a
* particular clone, but that one is set by PFE for session affinity.
*
*
* optional string clone_id = 21;
* @param value The bytes for cloneId to set.
* @return This builder for chaining.
*/
public Builder setCloneIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
cloneId_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
private boolean gzipGfe_ ;
/**
*
* If true, "gzip(gfe)" was stripped from the User-Agent: value in 'headers'.
*
*
* optional bool gzip_gfe = 15 [default = false];
* @return Whether the gzipGfe field is set.
*/
@java.lang.Override
public boolean hasGzipGfe() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* If true, "gzip(gfe)" was stripped from the User-Agent: value in 'headers'.
*
*
* optional bool gzip_gfe = 15 [default = false];
* @return The gzipGfe.
*/
@java.lang.Override
public boolean getGzipGfe() {
return gzipGfe_;
}
/**
*
* If true, "gzip(gfe)" was stripped from the User-Agent: value in 'headers'.
*
*
* optional bool gzip_gfe = 15 [default = false];
* @param value The gzipGfe to set.
* @return This builder for chaining.
*/
public Builder setGzipGfe(boolean value) {
gzipGfe_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* If true, "gzip(gfe)" was stripped from the User-Agent: value in 'headers'.
*
*
* optional bool gzip_gfe = 15 [default = false];
* @return This builder for chaining.
*/
public Builder clearGzipGfe() {
bitField0_ = (bitField0_ & ~0x00040000);
gzipGfe_ = false;
onChanged();
return this;
}
private int servingDestination_ = 0;
/**
*
* Component within a clone that should serve the request.
*
*
* optional .java.apphosting.ServingDestination serving_destination = 19;
* @return Whether the servingDestination field is set.
*/
@java.lang.Override public boolean hasServingDestination() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
* Component within a clone that should serve the request.
*
*
* optional .java.apphosting.ServingDestination serving_destination = 19;
* @return The servingDestination.
*/
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ServingDestination getServingDestination() {
com.google.apphosting.base.protos.HttpPb.ServingDestination result = com.google.apphosting.base.protos.HttpPb.ServingDestination.forNumber(servingDestination_);
return result == null ? com.google.apphosting.base.protos.HttpPb.ServingDestination.WORKER : result;
}
/**
*
* Component within a clone that should serve the request.
*
*
* optional .java.apphosting.ServingDestination serving_destination = 19;
* @param value The servingDestination to set.
* @return This builder for chaining.
*/
public Builder setServingDestination(com.google.apphosting.base.protos.HttpPb.ServingDestination value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00080000;
servingDestination_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Component within a clone that should serve the request.
*
*
* optional .java.apphosting.ServingDestination serving_destination = 19;
* @return This builder for chaining.
*/
public Builder clearServingDestination() {
bitField0_ = (bitField0_ & ~0x00080000);
servingDestination_ = 0;
onChanged();
return this;
}
private boolean qfeNodeSelectionRequest_ ;
/**
*
* If set, this request is a QFE node selection request.
* It is a "control plane" request for:
* 1) finding an available AppServer and clone to serve the incoming
* request, so that the it can instruct the QFE to route the request
* to this "selected" clone through Node Envoy.
* 2) reserving the clone until the actual request is complete so that
* AppServer would not kill the clone early.
*
*
* optional bool qfe_node_selection_request = 23;
* @return Whether the qfeNodeSelectionRequest field is set.
*/
@java.lang.Override
public boolean hasQfeNodeSelectionRequest() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
* If set, this request is a QFE node selection request.
* It is a "control plane" request for:
* 1) finding an available AppServer and clone to serve the incoming
* request, so that the it can instruct the QFE to route the request
* to this "selected" clone through Node Envoy.
* 2) reserving the clone until the actual request is complete so that
* AppServer would not kill the clone early.
*
*
* optional bool qfe_node_selection_request = 23;
* @return The qfeNodeSelectionRequest.
*/
@java.lang.Override
public boolean getQfeNodeSelectionRequest() {
return qfeNodeSelectionRequest_;
}
/**
*
* If set, this request is a QFE node selection request.
* It is a "control plane" request for:
* 1) finding an available AppServer and clone to serve the incoming
* request, so that the it can instruct the QFE to route the request
* to this "selected" clone through Node Envoy.
* 2) reserving the clone until the actual request is complete so that
* AppServer would not kill the clone early.
*
*
* optional bool qfe_node_selection_request = 23;
* @param value The qfeNodeSelectionRequest to set.
* @return This builder for chaining.
*/
public Builder setQfeNodeSelectionRequest(boolean value) {
qfeNodeSelectionRequest_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* If set, this request is a QFE node selection request.
* It is a "control plane" request for:
* 1) finding an available AppServer and clone to serve the incoming
* request, so that the it can instruct the QFE to route the request
* to this "selected" clone through Node Envoy.
* 2) reserving the clone until the actual request is complete so that
* AppServer would not kill the clone early.
*
*
* optional bool qfe_node_selection_request = 23;
* @return This builder for chaining.
*/
public Builder clearQfeNodeSelectionRequest() {
bitField0_ = (bitField0_ & ~0x00100000);
qfeNodeSelectionRequest_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.HttpRequest)
}
// @@protoc_insertion_point(class_scope:java.apphosting.HttpRequest)
private static final com.google.apphosting.base.protos.HttpPb.HttpRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.HttpPb.HttpRequest();
}
public static com.google.apphosting.base.protos.HttpPb.HttpRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HttpRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.HttpRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HttpResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:java.apphosting.HttpResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* This value will be from
* webutil/http/httpresponse.h:HTTPResponse::ResponseCode.
*
*
* required int32 responsecode = 1;
* @return Whether the responsecode field is set.
*/
boolean hasResponsecode();
/**
*
* This value will be from
* webutil/http/httpresponse.h:HTTPResponse::ResponseCode.
*
*
* required int32 responsecode = 1;
* @return The responsecode.
*/
int getResponsecode();
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
java.util.List
getOutputHeadersList();
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getOutputHeaders(int index);
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
int getOutputHeadersCount();
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getOutputHeadersOrBuilderList();
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getOutputHeadersOrBuilder(
int index);
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* required bytes response = 3;
* @return Whether the response field is set.
*/
boolean hasResponse();
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* required bytes response = 3;
* @return The response.
*/
com.google.protobuf.ByteString getResponse();
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
java.util.List
getOutputTrailersList();
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getOutputTrailers(int index);
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
int getOutputTrailersCount();
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getOutputTrailersOrBuilderList();
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getOutputTrailersOrBuilder(
int index);
/**
*
* If set, indicates two things:
* 1) the response has been compressed by trusted code
* 2) the size of the uncompressed response
*
*
* optional int64 uncompressed_size = 4;
* @return Whether the uncompressedSize field is set.
*/
boolean hasUncompressedSize();
/**
*
* If set, indicates two things:
* 1) the response has been compressed by trusted code
* 2) the size of the uncompressed response
*
*
* optional int64 uncompressed_size = 4;
* @return The uncompressedSize.
*/
long getUncompressedSize();
/**
*
* If true, the response will be uncompressed by the GFE (or another proxy)
* before it is sent to the client. This can happen if a proxy wants all
* responses to be compressed for caching or bandwidth reasons.
*
*
* optional bool uncompress_for_client = 5;
* @return Whether the uncompressForClient field is set.
*/
boolean hasUncompressForClient();
/**
*
* If true, the response will be uncompressed by the GFE (or another proxy)
* before it is sent to the client. This can happen if a proxy wants all
* responses to be compressed for caching or bandwidth reasons.
*
*
* optional bool uncompress_for_client = 5;
* @return The uncompressForClient.
*/
boolean getUncompressForClient();
}
/**
*
* Representation of an entire HTTP response, as a PB.
*
*
* Protobuf type {@code java.apphosting.HttpResponse}
*/
public static final class HttpResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:java.apphosting.HttpResponse)
HttpResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use HttpResponse.newBuilder() to construct.
private HttpResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HttpResponse() {
outputHeaders_ = java.util.Collections.emptyList();
response_ = com.google.protobuf.ByteString.EMPTY;
outputTrailers_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HttpResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.HttpPb.HttpResponse.class, com.google.apphosting.base.protos.HttpPb.HttpResponse.Builder.class);
}
private int bitField0_;
public static final int RESPONSECODE_FIELD_NUMBER = 1;
private int responsecode_ = 0;
/**
*
* This value will be from
* webutil/http/httpresponse.h:HTTPResponse::ResponseCode.
*
*
* required int32 responsecode = 1;
* @return Whether the responsecode field is set.
*/
@java.lang.Override
public boolean hasResponsecode() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* This value will be from
* webutil/http/httpresponse.h:HTTPResponse::ResponseCode.
*
*
* required int32 responsecode = 1;
* @return The responsecode.
*/
@java.lang.Override
public int getResponsecode() {
return responsecode_;
}
public static final int OUTPUT_HEADERS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List outputHeaders_;
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
@java.lang.Override
public java.util.List getOutputHeadersList() {
return outputHeaders_;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getOutputHeadersOrBuilderList() {
return outputHeaders_;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
@java.lang.Override
public int getOutputHeadersCount() {
return outputHeaders_.size();
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getOutputHeaders(int index) {
return outputHeaders_.get(index);
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getOutputHeadersOrBuilder(
int index) {
return outputHeaders_.get(index);
}
public static final int RESPONSE_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString response_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* required bytes response = 3;
* @return Whether the response field is set.
*/
@java.lang.Override
public boolean hasResponse() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* required bytes response = 3;
* @return The response.
*/
@java.lang.Override
public com.google.protobuf.ByteString getResponse() {
return response_;
}
public static final int OUTPUT_TRAILERS_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List outputTrailers_;
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
@java.lang.Override
public java.util.List getOutputTrailersList() {
return outputTrailers_;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
@java.lang.Override
public java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getOutputTrailersOrBuilderList() {
return outputTrailers_;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
@java.lang.Override
public int getOutputTrailersCount() {
return outputTrailers_.size();
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getOutputTrailers(int index) {
return outputTrailers_.get(index);
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getOutputTrailersOrBuilder(
int index) {
return outputTrailers_.get(index);
}
public static final int UNCOMPRESSED_SIZE_FIELD_NUMBER = 4;
private long uncompressedSize_ = 0L;
/**
*
* If set, indicates two things:
* 1) the response has been compressed by trusted code
* 2) the size of the uncompressed response
*
*
* optional int64 uncompressed_size = 4;
* @return Whether the uncompressedSize field is set.
*/
@java.lang.Override
public boolean hasUncompressedSize() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* If set, indicates two things:
* 1) the response has been compressed by trusted code
* 2) the size of the uncompressed response
*
*
* optional int64 uncompressed_size = 4;
* @return The uncompressedSize.
*/
@java.lang.Override
public long getUncompressedSize() {
return uncompressedSize_;
}
public static final int UNCOMPRESS_FOR_CLIENT_FIELD_NUMBER = 5;
private boolean uncompressForClient_ = false;
/**
*
* If true, the response will be uncompressed by the GFE (or another proxy)
* before it is sent to the client. This can happen if a proxy wants all
* responses to be compressed for caching or bandwidth reasons.
*
*
* optional bool uncompress_for_client = 5;
* @return Whether the uncompressForClient field is set.
*/
@java.lang.Override
public boolean hasUncompressForClient() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* If true, the response will be uncompressed by the GFE (or another proxy)
* before it is sent to the client. This can happen if a proxy wants all
* responses to be compressed for caching or bandwidth reasons.
*
*
* optional bool uncompress_for_client = 5;
* @return The uncompressForClient.
*/
@java.lang.Override
public boolean getUncompressForClient() {
return uncompressForClient_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasResponsecode()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasResponse()) {
memoizedIsInitialized = 0;
return false;
}
for (int i = 0; i < getOutputHeadersCount(); i++) {
if (!getOutputHeaders(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
for (int i = 0; i < getOutputTrailersCount(); i++) {
if (!getOutputTrailers(i).isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeInt32(1, responsecode_);
}
for (int i = 0; i < outputHeaders_.size(); i++) {
output.writeMessage(2, outputHeaders_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBytes(3, response_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeInt64(4, uncompressedSize_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeBool(5, uncompressForClient_);
}
for (int i = 0; i < outputTrailers_.size(); i++) {
output.writeMessage(6, outputTrailers_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, responsecode_);
}
for (int i = 0; i < outputHeaders_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, outputHeaders_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, response_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, uncompressedSize_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, uncompressForClient_);
}
for (int i = 0; i < outputTrailers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, outputTrailers_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.apphosting.base.protos.HttpPb.HttpResponse)) {
return super.equals(obj);
}
com.google.apphosting.base.protos.HttpPb.HttpResponse other = (com.google.apphosting.base.protos.HttpPb.HttpResponse) obj;
if (hasResponsecode() != other.hasResponsecode()) return false;
if (hasResponsecode()) {
if (getResponsecode()
!= other.getResponsecode()) return false;
}
if (!getOutputHeadersList()
.equals(other.getOutputHeadersList())) return false;
if (hasResponse() != other.hasResponse()) return false;
if (hasResponse()) {
if (!getResponse()
.equals(other.getResponse())) return false;
}
if (!getOutputTrailersList()
.equals(other.getOutputTrailersList())) return false;
if (hasUncompressedSize() != other.hasUncompressedSize()) return false;
if (hasUncompressedSize()) {
if (getUncompressedSize()
!= other.getUncompressedSize()) return false;
}
if (hasUncompressForClient() != other.hasUncompressForClient()) return false;
if (hasUncompressForClient()) {
if (getUncompressForClient()
!= other.getUncompressForClient()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasResponsecode()) {
hash = (37 * hash) + RESPONSECODE_FIELD_NUMBER;
hash = (53 * hash) + getResponsecode();
}
if (getOutputHeadersCount() > 0) {
hash = (37 * hash) + OUTPUT_HEADERS_FIELD_NUMBER;
hash = (53 * hash) + getOutputHeadersList().hashCode();
}
if (hasResponse()) {
hash = (37 * hash) + RESPONSE_FIELD_NUMBER;
hash = (53 * hash) + getResponse().hashCode();
}
if (getOutputTrailersCount() > 0) {
hash = (37 * hash) + OUTPUT_TRAILERS_FIELD_NUMBER;
hash = (53 * hash) + getOutputTrailersList().hashCode();
}
if (hasUncompressedSize()) {
hash = (37 * hash) + UNCOMPRESSED_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUncompressedSize());
}
if (hasUncompressForClient()) {
hash = (37 * hash) + UNCOMPRESS_FOR_CLIENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUncompressForClient());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.apphosting.base.protos.HttpPb.HttpResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Representation of an entire HTTP response, as a PB.
*
*
* Protobuf type {@code java.apphosting.HttpResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:java.apphosting.HttpResponse)
com.google.apphosting.base.protos.HttpPb.HttpResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.apphosting.base.protos.HttpPb.HttpResponse.class, com.google.apphosting.base.protos.HttpPb.HttpResponse.Builder.class);
}
// Construct using com.google.apphosting.base.protos.HttpPb.HttpResponse.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
responsecode_ = 0;
if (outputHeadersBuilder_ == null) {
outputHeaders_ = java.util.Collections.emptyList();
} else {
outputHeaders_ = null;
outputHeadersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
response_ = com.google.protobuf.ByteString.EMPTY;
if (outputTrailersBuilder_ == null) {
outputTrailers_ = java.util.Collections.emptyList();
} else {
outputTrailers_ = null;
outputTrailersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
uncompressedSize_ = 0L;
uncompressForClient_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.apphosting.base.protos.HttpPb.internal_static_java_apphosting_HttpResponse_descriptor;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.HttpResponse getDefaultInstanceForType() {
return com.google.apphosting.base.protos.HttpPb.HttpResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.HttpResponse build() {
com.google.apphosting.base.protos.HttpPb.HttpResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.HttpResponse buildPartial() {
com.google.apphosting.base.protos.HttpPb.HttpResponse result = new com.google.apphosting.base.protos.HttpPb.HttpResponse(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.apphosting.base.protos.HttpPb.HttpResponse result) {
if (outputHeadersBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
outputHeaders_ = java.util.Collections.unmodifiableList(outputHeaders_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.outputHeaders_ = outputHeaders_;
} else {
result.outputHeaders_ = outputHeadersBuilder_.build();
}
if (outputTrailersBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
outputTrailers_ = java.util.Collections.unmodifiableList(outputTrailers_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.outputTrailers_ = outputTrailers_;
} else {
result.outputTrailers_ = outputTrailersBuilder_.build();
}
}
private void buildPartial0(com.google.apphosting.base.protos.HttpPb.HttpResponse result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.responsecode_ = responsecode_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.response_ = response_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.uncompressedSize_ = uncompressedSize_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.uncompressForClient_ = uncompressForClient_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.apphosting.base.protos.HttpPb.HttpResponse) {
return mergeFrom((com.google.apphosting.base.protos.HttpPb.HttpResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.apphosting.base.protos.HttpPb.HttpResponse other) {
if (other == com.google.apphosting.base.protos.HttpPb.HttpResponse.getDefaultInstance()) return this;
if (other.hasResponsecode()) {
setResponsecode(other.getResponsecode());
}
if (outputHeadersBuilder_ == null) {
if (!other.outputHeaders_.isEmpty()) {
if (outputHeaders_.isEmpty()) {
outputHeaders_ = other.outputHeaders_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureOutputHeadersIsMutable();
outputHeaders_.addAll(other.outputHeaders_);
}
onChanged();
}
} else {
if (!other.outputHeaders_.isEmpty()) {
if (outputHeadersBuilder_.isEmpty()) {
outputHeadersBuilder_.dispose();
outputHeadersBuilder_ = null;
outputHeaders_ = other.outputHeaders_;
bitField0_ = (bitField0_ & ~0x00000002);
outputHeadersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOutputHeadersFieldBuilder() : null;
} else {
outputHeadersBuilder_.addAllMessages(other.outputHeaders_);
}
}
}
if (other.hasResponse()) {
setResponse(other.getResponse());
}
if (outputTrailersBuilder_ == null) {
if (!other.outputTrailers_.isEmpty()) {
if (outputTrailers_.isEmpty()) {
outputTrailers_ = other.outputTrailers_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureOutputTrailersIsMutable();
outputTrailers_.addAll(other.outputTrailers_);
}
onChanged();
}
} else {
if (!other.outputTrailers_.isEmpty()) {
if (outputTrailersBuilder_.isEmpty()) {
outputTrailersBuilder_.dispose();
outputTrailersBuilder_ = null;
outputTrailers_ = other.outputTrailers_;
bitField0_ = (bitField0_ & ~0x00000008);
outputTrailersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getOutputTrailersFieldBuilder() : null;
} else {
outputTrailersBuilder_.addAllMessages(other.outputTrailers_);
}
}
}
if (other.hasUncompressedSize()) {
setUncompressedSize(other.getUncompressedSize());
}
if (other.hasUncompressForClient()) {
setUncompressForClient(other.getUncompressForClient());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasResponsecode()) {
return false;
}
if (!hasResponse()) {
return false;
}
for (int i = 0; i < getOutputHeadersCount(); i++) {
if (!getOutputHeaders(i).isInitialized()) {
return false;
}
}
for (int i = 0; i < getOutputTrailersCount(); i++) {
if (!getOutputTrailers(i).isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
responsecode_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader m =
input.readMessage(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.PARSER,
extensionRegistry);
if (outputHeadersBuilder_ == null) {
ensureOutputHeadersIsMutable();
outputHeaders_.add(m);
} else {
outputHeadersBuilder_.addMessage(m);
}
break;
} // case 18
case 26: {
response_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
case 32: {
uncompressedSize_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 32
case 40: {
uncompressForClient_ = input.readBool();
bitField0_ |= 0x00000020;
break;
} // case 40
case 50: {
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader m =
input.readMessage(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.PARSER,
extensionRegistry);
if (outputTrailersBuilder_ == null) {
ensureOutputTrailersIsMutable();
outputTrailers_.add(m);
} else {
outputTrailersBuilder_.addMessage(m);
}
break;
} // case 50
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int responsecode_ ;
/**
*
* This value will be from
* webutil/http/httpresponse.h:HTTPResponse::ResponseCode.
*
*
* required int32 responsecode = 1;
* @return Whether the responsecode field is set.
*/
@java.lang.Override
public boolean hasResponsecode() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* This value will be from
* webutil/http/httpresponse.h:HTTPResponse::ResponseCode.
*
*
* required int32 responsecode = 1;
* @return The responsecode.
*/
@java.lang.Override
public int getResponsecode() {
return responsecode_;
}
/**
*
* This value will be from
* webutil/http/httpresponse.h:HTTPResponse::ResponseCode.
*
*
* required int32 responsecode = 1;
* @param value The responsecode to set.
* @return This builder for chaining.
*/
public Builder setResponsecode(int value) {
responsecode_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* This value will be from
* webutil/http/httpresponse.h:HTTPResponse::ResponseCode.
*
*
* required int32 responsecode = 1;
* @return This builder for chaining.
*/
public Builder clearResponsecode() {
bitField0_ = (bitField0_ & ~0x00000001);
responsecode_ = 0;
onChanged();
return this;
}
private java.util.List outputHeaders_ =
java.util.Collections.emptyList();
private void ensureOutputHeadersIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
outputHeaders_ = new java.util.ArrayList(outputHeaders_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder> outputHeadersBuilder_;
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public java.util.List getOutputHeadersList() {
if (outputHeadersBuilder_ == null) {
return java.util.Collections.unmodifiableList(outputHeaders_);
} else {
return outputHeadersBuilder_.getMessageList();
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public int getOutputHeadersCount() {
if (outputHeadersBuilder_ == null) {
return outputHeaders_.size();
} else {
return outputHeadersBuilder_.getCount();
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getOutputHeaders(int index) {
if (outputHeadersBuilder_ == null) {
return outputHeaders_.get(index);
} else {
return outputHeadersBuilder_.getMessage(index);
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder setOutputHeaders(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (outputHeadersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputHeadersIsMutable();
outputHeaders_.set(index, value);
onChanged();
} else {
outputHeadersBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder setOutputHeaders(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (outputHeadersBuilder_ == null) {
ensureOutputHeadersIsMutable();
outputHeaders_.set(index, builderForValue.build());
onChanged();
} else {
outputHeadersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder addOutputHeaders(com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (outputHeadersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputHeadersIsMutable();
outputHeaders_.add(value);
onChanged();
} else {
outputHeadersBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder addOutputHeaders(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (outputHeadersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputHeadersIsMutable();
outputHeaders_.add(index, value);
onChanged();
} else {
outputHeadersBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder addOutputHeaders(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (outputHeadersBuilder_ == null) {
ensureOutputHeadersIsMutable();
outputHeaders_.add(builderForValue.build());
onChanged();
} else {
outputHeadersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder addOutputHeaders(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (outputHeadersBuilder_ == null) {
ensureOutputHeadersIsMutable();
outputHeaders_.add(index, builderForValue.build());
onChanged();
} else {
outputHeadersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder addAllOutputHeaders(
java.lang.Iterable extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader> values) {
if (outputHeadersBuilder_ == null) {
ensureOutputHeadersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, outputHeaders_);
onChanged();
} else {
outputHeadersBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder clearOutputHeaders() {
if (outputHeadersBuilder_ == null) {
outputHeaders_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
outputHeadersBuilder_.clear();
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public Builder removeOutputHeaders(int index) {
if (outputHeadersBuilder_ == null) {
ensureOutputHeadersIsMutable();
outputHeaders_.remove(index);
onChanged();
} else {
outputHeadersBuilder_.remove(index);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder getOutputHeadersBuilder(
int index) {
return getOutputHeadersFieldBuilder().getBuilder(index);
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getOutputHeadersOrBuilder(
int index) {
if (outputHeadersBuilder_ == null) {
return outputHeaders_.get(index); } else {
return outputHeadersBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getOutputHeadersOrBuilderList() {
if (outputHeadersBuilder_ != null) {
return outputHeadersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(outputHeaders_);
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder addOutputHeadersBuilder() {
return getOutputHeadersFieldBuilder().addBuilder(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.getDefaultInstance());
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder addOutputHeadersBuilder(
int index) {
return getOutputHeadersFieldBuilder().addBuilder(
index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.getDefaultInstance());
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_headers = 2;
*/
public java.util.List
getOutputHeadersBuilderList() {
return getOutputHeadersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getOutputHeadersFieldBuilder() {
if (outputHeadersBuilder_ == null) {
outputHeadersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>(
outputHeaders_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
outputHeaders_ = null;
}
return outputHeadersBuilder_;
}
private com.google.protobuf.ByteString response_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* required bytes response = 3;
* @return Whether the response field is set.
*/
@java.lang.Override
public boolean hasResponse() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* required bytes response = 3;
* @return The response.
*/
@java.lang.Override
public com.google.protobuf.ByteString getResponse() {
return response_;
}
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* required bytes response = 3;
* @param value The response to set.
* @return This builder for chaining.
*/
public Builder setResponse(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
response_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* This data can be many MB in size. We use Cords to prevent copying.
*
*
* required bytes response = 3;
* @return This builder for chaining.
*/
public Builder clearResponse() {
bitField0_ = (bitField0_ & ~0x00000004);
response_ = getDefaultInstance().getResponse();
onChanged();
return this;
}
private java.util.List outputTrailers_ =
java.util.Collections.emptyList();
private void ensureOutputTrailersIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
outputTrailers_ = new java.util.ArrayList(outputTrailers_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder> outputTrailersBuilder_;
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public java.util.List getOutputTrailersList() {
if (outputTrailersBuilder_ == null) {
return java.util.Collections.unmodifiableList(outputTrailers_);
} else {
return outputTrailersBuilder_.getMessageList();
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public int getOutputTrailersCount() {
if (outputTrailersBuilder_ == null) {
return outputTrailers_.size();
} else {
return outputTrailersBuilder_.getCount();
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader getOutputTrailers(int index) {
if (outputTrailersBuilder_ == null) {
return outputTrailers_.get(index);
} else {
return outputTrailersBuilder_.getMessage(index);
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder setOutputTrailers(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (outputTrailersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputTrailersIsMutable();
outputTrailers_.set(index, value);
onChanged();
} else {
outputTrailersBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder setOutputTrailers(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (outputTrailersBuilder_ == null) {
ensureOutputTrailersIsMutable();
outputTrailers_.set(index, builderForValue.build());
onChanged();
} else {
outputTrailersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder addOutputTrailers(com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (outputTrailersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputTrailersIsMutable();
outputTrailers_.add(value);
onChanged();
} else {
outputTrailersBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder addOutputTrailers(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader value) {
if (outputTrailersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOutputTrailersIsMutable();
outputTrailers_.add(index, value);
onChanged();
} else {
outputTrailersBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder addOutputTrailers(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (outputTrailersBuilder_ == null) {
ensureOutputTrailersIsMutable();
outputTrailers_.add(builderForValue.build());
onChanged();
} else {
outputTrailersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder addOutputTrailers(
int index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder builderForValue) {
if (outputTrailersBuilder_ == null) {
ensureOutputTrailersIsMutable();
outputTrailers_.add(index, builderForValue.build());
onChanged();
} else {
outputTrailersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder addAllOutputTrailers(
java.lang.Iterable extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader> values) {
if (outputTrailersBuilder_ == null) {
ensureOutputTrailersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, outputTrailers_);
onChanged();
} else {
outputTrailersBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder clearOutputTrailers() {
if (outputTrailersBuilder_ == null) {
outputTrailers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
outputTrailersBuilder_.clear();
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public Builder removeOutputTrailers(int index) {
if (outputTrailersBuilder_ == null) {
ensureOutputTrailersIsMutable();
outputTrailers_.remove(index);
onChanged();
} else {
outputTrailersBuilder_.remove(index);
}
return this;
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder getOutputTrailersBuilder(
int index) {
return getOutputTrailersFieldBuilder().getBuilder(index);
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder getOutputTrailersOrBuilder(
int index) {
if (outputTrailersBuilder_ == null) {
return outputTrailers_.get(index); } else {
return outputTrailersBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public java.util.List extends com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getOutputTrailersOrBuilderList() {
if (outputTrailersBuilder_ != null) {
return outputTrailersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(outputTrailers_);
}
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder addOutputTrailersBuilder() {
return getOutputTrailersFieldBuilder().addBuilder(
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.getDefaultInstance());
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder addOutputTrailersBuilder(
int index) {
return getOutputTrailersFieldBuilder().addBuilder(
index, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.getDefaultInstance());
}
/**
* repeated .java.apphosting.ParsedHttpHeader output_trailers = 6;
*/
public java.util.List
getOutputTrailersBuilderList() {
return getOutputTrailersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>
getOutputTrailersFieldBuilder() {
if (outputTrailersBuilder_ == null) {
outputTrailersBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeader.Builder, com.google.apphosting.base.protos.HttpPb.ParsedHttpHeaderOrBuilder>(
outputTrailers_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
outputTrailers_ = null;
}
return outputTrailersBuilder_;
}
private long uncompressedSize_ ;
/**
*
* If set, indicates two things:
* 1) the response has been compressed by trusted code
* 2) the size of the uncompressed response
*
*
* optional int64 uncompressed_size = 4;
* @return Whether the uncompressedSize field is set.
*/
@java.lang.Override
public boolean hasUncompressedSize() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* If set, indicates two things:
* 1) the response has been compressed by trusted code
* 2) the size of the uncompressed response
*
*
* optional int64 uncompressed_size = 4;
* @return The uncompressedSize.
*/
@java.lang.Override
public long getUncompressedSize() {
return uncompressedSize_;
}
/**
*
* If set, indicates two things:
* 1) the response has been compressed by trusted code
* 2) the size of the uncompressed response
*
*
* optional int64 uncompressed_size = 4;
* @param value The uncompressedSize to set.
* @return This builder for chaining.
*/
public Builder setUncompressedSize(long value) {
uncompressedSize_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* If set, indicates two things:
* 1) the response has been compressed by trusted code
* 2) the size of the uncompressed response
*
*
* optional int64 uncompressed_size = 4;
* @return This builder for chaining.
*/
public Builder clearUncompressedSize() {
bitField0_ = (bitField0_ & ~0x00000010);
uncompressedSize_ = 0L;
onChanged();
return this;
}
private boolean uncompressForClient_ ;
/**
*
* If true, the response will be uncompressed by the GFE (or another proxy)
* before it is sent to the client. This can happen if a proxy wants all
* responses to be compressed for caching or bandwidth reasons.
*
*
* optional bool uncompress_for_client = 5;
* @return Whether the uncompressForClient field is set.
*/
@java.lang.Override
public boolean hasUncompressForClient() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* If true, the response will be uncompressed by the GFE (or another proxy)
* before it is sent to the client. This can happen if a proxy wants all
* responses to be compressed for caching or bandwidth reasons.
*
*
* optional bool uncompress_for_client = 5;
* @return The uncompressForClient.
*/
@java.lang.Override
public boolean getUncompressForClient() {
return uncompressForClient_;
}
/**
*
* If true, the response will be uncompressed by the GFE (or another proxy)
* before it is sent to the client. This can happen if a proxy wants all
* responses to be compressed for caching or bandwidth reasons.
*
*
* optional bool uncompress_for_client = 5;
* @param value The uncompressForClient to set.
* @return This builder for chaining.
*/
public Builder setUncompressForClient(boolean value) {
uncompressForClient_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* If true, the response will be uncompressed by the GFE (or another proxy)
* before it is sent to the client. This can happen if a proxy wants all
* responses to be compressed for caching or bandwidth reasons.
*
*
* optional bool uncompress_for_client = 5;
* @return This builder for chaining.
*/
public Builder clearUncompressForClient() {
bitField0_ = (bitField0_ & ~0x00000020);
uncompressForClient_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:java.apphosting.HttpResponse)
}
// @@protoc_insertion_point(class_scope:java.apphosting.HttpResponse)
private static final com.google.apphosting.base.protos.HttpPb.HttpResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.apphosting.base.protos.HttpPb.HttpResponse();
}
public static com.google.apphosting.base.protos.HttpPb.HttpResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HttpResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.apphosting.base.protos.HttpPb.HttpResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_ParsedHttpHeader_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_ParsedHttpHeader_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_HttpRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_HttpRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_java_apphosting_HttpResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_java_apphosting_HttpResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\nhttp.proto\022\017java.apphosting\".\n\020ParsedH" +
"ttpHeader\022\013\n\003key\030\001 \002(\t\022\r\n\005value\030\002 \002(\t\"\215\005" +
"\n\013HttpRequest\022\013\n\003url\030\001 \002(\t\022\024\n\010postdata\030\002" +
" \001(\014B\002\010\001\0222\n\007headers\030\003 \003(\0132!.java.apphost" +
"ing.ParsedHttpHeader\022\017\n\007user_ip\030\004 \002(\t\022\021\n" +
"\tserver_ip\030\020 \001(\t\022\026\n\007trusted\030\005 \001(\010:\005false" +
"\022\020\n\010protocol\030\006 \002(\t\022\037\n\027original_request_m" +
"ethod\030\030 \001(\t\022\036\n\014http_version\030\007 \001(\t:\010HTTP/" +
"1.1\022\027\n\010is_https\030\010 \001(\010:\005false\022\031\n\nis_offli" +
"ne\030\t \001(\010:\005false\022\"\n\024enable_pending_queue\030" +
"\n \001(\010:\004true\022\025\n\rappserver_bns\030\013 \001(\t\022\036\n\017wa" +
"rming_request\030\014 \001(\010:\005false\022\036\n\022warming_re" +
"quest_id\030\026 \001(\003:\002-1\022!\n\031shutdown_request_c" +
"lone_id\030\021 \001(\t\022#\n\033background_request_clon" +
"e_id\030\022 \001(\t\022\020\n\010clone_id\030\025 \001(\t\022\027\n\010gzip_gfe" +
"\030\017 \001(\010:\005false\022@\n\023serving_destination\030\023 \001" +
"(\0162#.java.apphosting.ServingDestination\022" +
"\"\n\032qfe_node_selection_request\030\027 \001(\010J\004\010\r\020" +
"\016J\004\010\016\020\017J\004\010\024\020\025\"\347\001\n\014HttpResponse\022\024\n\014respon" +
"secode\030\001 \002(\005\0229\n\016output_headers\030\002 \003(\0132!.j" +
"ava.apphosting.ParsedHttpHeader\022\020\n\010respo" +
"nse\030\003 \002(\014\022:\n\017output_trailers\030\006 \003(\0132!.jav" +
"a.apphosting.ParsedHttpHeader\022\031\n\021uncompr" +
"essed_size\030\004 \001(\003\022\035\n\025uncompress_for_clien" +
"t\030\005 \001(\010*9\n\022ServingDestination\022\n\n\006WORKER\020" +
"\000\022\027\n\023TITANIUM_SUPERVISOR\020\001B.\n!com.google" +
".apphosting.base.protosB\006HttpPb\370\001\001"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_java_apphosting_ParsedHttpHeader_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_java_apphosting_ParsedHttpHeader_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_ParsedHttpHeader_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_java_apphosting_HttpRequest_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_java_apphosting_HttpRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_HttpRequest_descriptor,
new java.lang.String[] { "Url", "Postdata", "Headers", "UserIp", "ServerIp", "Trusted", "Protocol", "OriginalRequestMethod", "HttpVersion", "IsHttps", "IsOffline", "EnablePendingQueue", "AppserverBns", "WarmingRequest", "WarmingRequestId", "ShutdownRequestCloneId", "BackgroundRequestCloneId", "CloneId", "GzipGfe", "ServingDestination", "QfeNodeSelectionRequest", });
internal_static_java_apphosting_HttpResponse_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_java_apphosting_HttpResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_java_apphosting_HttpResponse_descriptor,
new java.lang.String[] { "Responsecode", "OutputHeaders", "Response", "OutputTrailers", "UncompressedSize", "UncompressForClient", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy