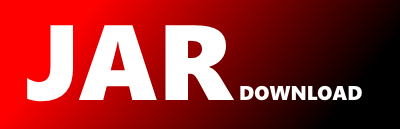
com.google.apphosting.runtime.AutoBuilder_JavaRuntime_Builder Maven / Gradle / Ivy
package com.google.apphosting.runtime;
import com.google.apphosting.runtime.anyrpc.AnyRpcPlugin;
import java.io.File;
import java.nio.file.Path;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoBuilderProcessor")
class AutoBuilder_JavaRuntime_Builder extends JavaRuntime.Builder {
private ServletEngineAdapter servletEngine;
private NullSandboxPlugin sandboxPlugin;
private AnyRpcPlugin rpcPlugin;
private File sharedDirectory;
private RequestManager requestManager;
private String runtimeVersion;
private ApplicationEnvironment.RuntimeConfiguration configuration;
private ApiDeadlineOracle deadlineOracle;
private BackgroundRequestCoordinator coordinator;
private boolean compressResponse;
private boolean enableHotspotPerformanceMetrics;
private boolean pollForNetwork;
private boolean defaultToNativeUrlStreamHandler;
private boolean forceUrlfetchUrlStreamHandler;
private boolean ignoreDaemonThreads;
private boolean useEnvVarsFromAppInfo;
private String fixedApplicationPath;
private boolean redirectStdoutStderr;
private boolean logJsonToFile;
private boolean clearLogHandlers;
private Path jsonLogDir;
private short set$0;
AutoBuilder_JavaRuntime_Builder() {
}
@Override
public JavaRuntime.Builder setServletEngine(ServletEngineAdapter servletEngine) {
if (servletEngine == null) {
throw new NullPointerException("Null servletEngine");
}
this.servletEngine = servletEngine;
return this;
}
@Override
public ServletEngineAdapter servletEngine() {
if (this.servletEngine == null) {
throw new IllegalStateException("Property \"servletEngine\" has not been set");
}
return servletEngine;
}
@Override
public JavaRuntime.Builder setSandboxPlugin(NullSandboxPlugin sandboxPlugin) {
if (sandboxPlugin == null) {
throw new NullPointerException("Null sandboxPlugin");
}
this.sandboxPlugin = sandboxPlugin;
return this;
}
@Override
public JavaRuntime.Builder setRpcPlugin(AnyRpcPlugin rpcPlugin) {
if (rpcPlugin == null) {
throw new NullPointerException("Null rpcPlugin");
}
this.rpcPlugin = rpcPlugin;
return this;
}
@Override
public AnyRpcPlugin rpcPlugin() {
if (this.rpcPlugin == null) {
throw new IllegalStateException("Property \"rpcPlugin\" has not been set");
}
return rpcPlugin;
}
@Override
public JavaRuntime.Builder setSharedDirectory(File sharedDirectory) {
if (sharedDirectory == null) {
throw new NullPointerException("Null sharedDirectory");
}
this.sharedDirectory = sharedDirectory;
return this;
}
@Override
public File sharedDirectory() {
if (this.sharedDirectory == null) {
throw new IllegalStateException("Property \"sharedDirectory\" has not been set");
}
return sharedDirectory;
}
@Override
public JavaRuntime.Builder setRequestManager(RequestManager requestManager) {
if (requestManager == null) {
throw new NullPointerException("Null requestManager");
}
this.requestManager = requestManager;
return this;
}
@Override
public JavaRuntime.Builder setRuntimeVersion(String runtimeVersion) {
if (runtimeVersion == null) {
throw new NullPointerException("Null runtimeVersion");
}
this.runtimeVersion = runtimeVersion;
return this;
}
@Override
public String runtimeVersion() {
if (this.runtimeVersion == null) {
throw new IllegalStateException("Property \"runtimeVersion\" has not been set");
}
return runtimeVersion;
}
@Override
public JavaRuntime.Builder setConfiguration(ApplicationEnvironment.RuntimeConfiguration configuration) {
if (configuration == null) {
throw new NullPointerException("Null configuration");
}
this.configuration = configuration;
return this;
}
@Override
public ApplicationEnvironment.RuntimeConfiguration configuration() {
if (this.configuration == null) {
throw new IllegalStateException("Property \"configuration\" has not been set");
}
return configuration;
}
@Override
public JavaRuntime.Builder setDeadlineOracle(ApiDeadlineOracle deadlineOracle) {
if (deadlineOracle == null) {
throw new NullPointerException("Null deadlineOracle");
}
this.deadlineOracle = deadlineOracle;
return this;
}
@Override
public ApiDeadlineOracle deadlineOracle() {
if (this.deadlineOracle == null) {
throw new IllegalStateException("Property \"deadlineOracle\" has not been set");
}
return deadlineOracle;
}
@Override
public JavaRuntime.Builder setCoordinator(BackgroundRequestCoordinator coordinator) {
if (coordinator == null) {
throw new NullPointerException("Null coordinator");
}
this.coordinator = coordinator;
return this;
}
@Override
public JavaRuntime.Builder setCompressResponse(boolean compressResponse) {
this.compressResponse = compressResponse;
set$0 |= (short) 1;
return this;
}
@Override
public boolean compressResponse() {
if ((set$0 & 1) == 0) {
throw new IllegalStateException("Property \"compressResponse\" has not been set");
}
return compressResponse;
}
@Override
public JavaRuntime.Builder setEnableHotspotPerformanceMetrics(boolean enableHotspotPerformanceMetrics) {
this.enableHotspotPerformanceMetrics = enableHotspotPerformanceMetrics;
set$0 |= (short) 2;
return this;
}
@Override
public boolean enableHotspotPerformanceMetrics() {
if ((set$0 & 2) == 0) {
throw new IllegalStateException("Property \"enableHotspotPerformanceMetrics\" has not been set");
}
return enableHotspotPerformanceMetrics;
}
@Override
public JavaRuntime.Builder setPollForNetwork(boolean pollForNetwork) {
this.pollForNetwork = pollForNetwork;
set$0 |= (short) 4;
return this;
}
@Override
public boolean pollForNetwork() {
if ((set$0 & 4) == 0) {
throw new IllegalStateException("Property \"pollForNetwork\" has not been set");
}
return pollForNetwork;
}
@Override
public JavaRuntime.Builder setDefaultToNativeUrlStreamHandler(boolean defaultToNativeUrlStreamHandler) {
this.defaultToNativeUrlStreamHandler = defaultToNativeUrlStreamHandler;
set$0 |= (short) 8;
return this;
}
@Override
public boolean defaultToNativeUrlStreamHandler() {
if ((set$0 & 8) == 0) {
throw new IllegalStateException("Property \"defaultToNativeUrlStreamHandler\" has not been set");
}
return defaultToNativeUrlStreamHandler;
}
@Override
public JavaRuntime.Builder setForceUrlfetchUrlStreamHandler(boolean forceUrlfetchUrlStreamHandler) {
this.forceUrlfetchUrlStreamHandler = forceUrlfetchUrlStreamHandler;
set$0 |= (short) 0x10;
return this;
}
@Override
public boolean forceUrlfetchUrlStreamHandler() {
if ((set$0 & 0x10) == 0) {
throw new IllegalStateException("Property \"forceUrlfetchUrlStreamHandler\" has not been set");
}
return forceUrlfetchUrlStreamHandler;
}
@Override
public JavaRuntime.Builder setIgnoreDaemonThreads(boolean ignoreDaemonThreads) {
this.ignoreDaemonThreads = ignoreDaemonThreads;
set$0 |= (short) 0x20;
return this;
}
@Override
public boolean ignoreDaemonThreads() {
if ((set$0 & 0x20) == 0) {
throw new IllegalStateException("Property \"ignoreDaemonThreads\" has not been set");
}
return ignoreDaemonThreads;
}
@Override
public JavaRuntime.Builder setUseEnvVarsFromAppInfo(boolean useEnvVarsFromAppInfo) {
this.useEnvVarsFromAppInfo = useEnvVarsFromAppInfo;
set$0 |= (short) 0x40;
return this;
}
@Override
public boolean useEnvVarsFromAppInfo() {
if ((set$0 & 0x40) == 0) {
throw new IllegalStateException("Property \"useEnvVarsFromAppInfo\" has not been set");
}
return useEnvVarsFromAppInfo;
}
@Override
public JavaRuntime.Builder setFixedApplicationPath(String fixedApplicationPath) {
this.fixedApplicationPath = fixedApplicationPath;
return this;
}
@Override
@Nullable public String fixedApplicationPath() {
return fixedApplicationPath;
}
@Override
public JavaRuntime.Builder setRedirectStdoutStderr(boolean redirectStdoutStderr) {
this.redirectStdoutStderr = redirectStdoutStderr;
set$0 |= (short) 0x80;
return this;
}
@Override
public boolean redirectStdoutStderr() {
if ((set$0 & 0x80) == 0) {
throw new IllegalStateException("Property \"redirectStdoutStderr\" has not been set");
}
return redirectStdoutStderr;
}
@Override
public JavaRuntime.Builder setLogJsonToFile(boolean logJsonToFile) {
this.logJsonToFile = logJsonToFile;
set$0 |= (short) 0x100;
return this;
}
@Override
public boolean logJsonToFile() {
if ((set$0 & 0x100) == 0) {
throw new IllegalStateException("Property \"logJsonToFile\" has not been set");
}
return logJsonToFile;
}
@Override
public JavaRuntime.Builder setClearLogHandlers(boolean clearLogHandlers) {
this.clearLogHandlers = clearLogHandlers;
set$0 |= (short) 0x200;
return this;
}
@Override
public JavaRuntime.Builder setJsonLogDir(Path jsonLogDir) {
if (jsonLogDir == null) {
throw new NullPointerException("Null jsonLogDir");
}
this.jsonLogDir = jsonLogDir;
return this;
}
@Override
public Path jsonLogDir() {
if (this.jsonLogDir == null) {
throw new IllegalStateException("Property \"jsonLogDir\" has not been set");
}
return jsonLogDir;
}
@Override
public JavaRuntime build() {
if (set$0 != 0x3ff
|| this.servletEngine == null
|| this.sandboxPlugin == null
|| this.rpcPlugin == null
|| this.sharedDirectory == null
|| this.requestManager == null
|| this.runtimeVersion == null
|| this.configuration == null
|| this.deadlineOracle == null
|| this.coordinator == null
|| this.jsonLogDir == null) {
StringBuilder missing = new StringBuilder();
if (this.servletEngine == null) {
missing.append(" servletEngine");
}
if (this.sandboxPlugin == null) {
missing.append(" sandboxPlugin");
}
if (this.rpcPlugin == null) {
missing.append(" rpcPlugin");
}
if (this.sharedDirectory == null) {
missing.append(" sharedDirectory");
}
if (this.requestManager == null) {
missing.append(" requestManager");
}
if (this.runtimeVersion == null) {
missing.append(" runtimeVersion");
}
if (this.configuration == null) {
missing.append(" configuration");
}
if (this.deadlineOracle == null) {
missing.append(" deadlineOracle");
}
if (this.coordinator == null) {
missing.append(" coordinator");
}
if ((set$0 & 1) == 0) {
missing.append(" compressResponse");
}
if ((set$0 & 2) == 0) {
missing.append(" enableHotspotPerformanceMetrics");
}
if ((set$0 & 4) == 0) {
missing.append(" pollForNetwork");
}
if ((set$0 & 8) == 0) {
missing.append(" defaultToNativeUrlStreamHandler");
}
if ((set$0 & 0x10) == 0) {
missing.append(" forceUrlfetchUrlStreamHandler");
}
if ((set$0 & 0x20) == 0) {
missing.append(" ignoreDaemonThreads");
}
if ((set$0 & 0x40) == 0) {
missing.append(" useEnvVarsFromAppInfo");
}
if ((set$0 & 0x80) == 0) {
missing.append(" redirectStdoutStderr");
}
if ((set$0 & 0x100) == 0) {
missing.append(" logJsonToFile");
}
if ((set$0 & 0x200) == 0) {
missing.append(" clearLogHandlers");
}
if (this.jsonLogDir == null) {
missing.append(" jsonLogDir");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new JavaRuntime(
this.servletEngine,
this.sandboxPlugin,
this.rpcPlugin,
this.sharedDirectory,
this.requestManager,
this.runtimeVersion,
this.configuration,
this.deadlineOracle,
this.coordinator,
this.compressResponse,
this.enableHotspotPerformanceMetrics,
this.pollForNetwork,
this.defaultToNativeUrlStreamHandler,
this.forceUrlfetchUrlStreamHandler,
this.ignoreDaemonThreads,
this.useEnvVarsFromAppInfo,
this.fixedApplicationPath,
this.redirectStdoutStderr,
this.logJsonToFile,
this.clearLogHandlers,
this.jsonLogDir);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy