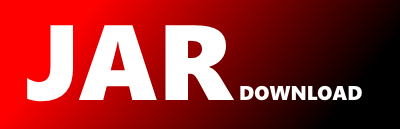
com.google.apphosting.runtime.AutoBuilder_RequestManager_Builder Maven / Gradle / Ivy
package com.google.apphosting.runtime;
import com.google.common.collect.ImmutableMap;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoBuilderProcessor")
class AutoBuilder_RequestManager_Builder extends RequestManager.Builder {
private long softDeadlineDelay;
private long hardDeadlineDelay;
private boolean disableDeadlineTimers;
private Optional runtimeLogSink = Optional.empty();
private ApiProxyImpl apiProxyImpl;
private int maxOutstandingApiRpcs;
private boolean threadStopTerminatesClone;
private boolean interruptFirstOnSoftDeadline;
private long cyclesPerSecond;
private boolean waitForDaemonRequestThreads;
private ImmutableMap environment;
private byte set$0;
AutoBuilder_RequestManager_Builder() {
}
@Override
public RequestManager.Builder setSoftDeadlineDelay(long softDeadlineDelay) {
this.softDeadlineDelay = softDeadlineDelay;
set$0 |= (byte) 1;
return this;
}
@Override
public long softDeadlineDelay() {
if ((set$0 & 1) == 0) {
throw new IllegalStateException("Property \"softDeadlineDelay\" has not been set");
}
return softDeadlineDelay;
}
@Override
public RequestManager.Builder setHardDeadlineDelay(long hardDeadlineDelay) {
this.hardDeadlineDelay = hardDeadlineDelay;
set$0 |= (byte) 2;
return this;
}
@Override
public long hardDeadlineDelay() {
if ((set$0 & 2) == 0) {
throw new IllegalStateException("Property \"hardDeadlineDelay\" has not been set");
}
return hardDeadlineDelay;
}
@Override
public RequestManager.Builder setDisableDeadlineTimers(boolean disableDeadlineTimers) {
this.disableDeadlineTimers = disableDeadlineTimers;
set$0 |= (byte) 4;
return this;
}
@Override
public boolean disableDeadlineTimers() {
if ((set$0 & 4) == 0) {
throw new IllegalStateException("Property \"disableDeadlineTimers\" has not been set");
}
return disableDeadlineTimers;
}
@Override
public RequestManager.Builder setRuntimeLogSink(Optional runtimeLogSink) {
if (runtimeLogSink == null) {
throw new NullPointerException("Null runtimeLogSink");
}
this.runtimeLogSink = runtimeLogSink;
return this;
}
@Override
public RequestManager.Builder setApiProxyImpl(ApiProxyImpl apiProxyImpl) {
if (apiProxyImpl == null) {
throw new NullPointerException("Null apiProxyImpl");
}
this.apiProxyImpl = apiProxyImpl;
return this;
}
@Override
public RequestManager.Builder setMaxOutstandingApiRpcs(int maxOutstandingApiRpcs) {
this.maxOutstandingApiRpcs = maxOutstandingApiRpcs;
set$0 |= (byte) 8;
return this;
}
@Override
public int maxOutstandingApiRpcs() {
if ((set$0 & 8) == 0) {
throw new IllegalStateException("Property \"maxOutstandingApiRpcs\" has not been set");
}
return maxOutstandingApiRpcs;
}
@Override
public RequestManager.Builder setThreadStopTerminatesClone(boolean threadStopTerminatesClone) {
this.threadStopTerminatesClone = threadStopTerminatesClone;
set$0 |= (byte) 0x10;
return this;
}
@Override
public boolean threadStopTerminatesClone() {
if ((set$0 & 0x10) == 0) {
throw new IllegalStateException("Property \"threadStopTerminatesClone\" has not been set");
}
return threadStopTerminatesClone;
}
@Override
public RequestManager.Builder setInterruptFirstOnSoftDeadline(boolean interruptFirstOnSoftDeadline) {
this.interruptFirstOnSoftDeadline = interruptFirstOnSoftDeadline;
set$0 |= (byte) 0x20;
return this;
}
@Override
public boolean interruptFirstOnSoftDeadline() {
if ((set$0 & 0x20) == 0) {
throw new IllegalStateException("Property \"interruptFirstOnSoftDeadline\" has not been set");
}
return interruptFirstOnSoftDeadline;
}
@Override
public RequestManager.Builder setCyclesPerSecond(long cyclesPerSecond) {
this.cyclesPerSecond = cyclesPerSecond;
set$0 |= (byte) 0x40;
return this;
}
@Override
public long cyclesPerSecond() {
if ((set$0 & 0x40) == 0) {
throw new IllegalStateException("Property \"cyclesPerSecond\" has not been set");
}
return cyclesPerSecond;
}
@Override
public RequestManager.Builder setWaitForDaemonRequestThreads(boolean waitForDaemonRequestThreads) {
this.waitForDaemonRequestThreads = waitForDaemonRequestThreads;
set$0 |= (byte) 0x80;
return this;
}
@Override
public boolean waitForDaemonRequestThreads() {
if ((set$0 & 0x80) == 0) {
throw new IllegalStateException("Property \"waitForDaemonRequestThreads\" has not been set");
}
return waitForDaemonRequestThreads;
}
@Override
public RequestManager.Builder setEnvironment(Map environment) {
this.environment = ImmutableMap.copyOf(environment);
return this;
}
@Override
public RequestManager build() {
if (set$0 != -1
|| this.apiProxyImpl == null
|| this.environment == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" softDeadlineDelay");
}
if ((set$0 & 2) == 0) {
missing.append(" hardDeadlineDelay");
}
if ((set$0 & 4) == 0) {
missing.append(" disableDeadlineTimers");
}
if (this.apiProxyImpl == null) {
missing.append(" apiProxyImpl");
}
if ((set$0 & 8) == 0) {
missing.append(" maxOutstandingApiRpcs");
}
if ((set$0 & 0x10) == 0) {
missing.append(" threadStopTerminatesClone");
}
if ((set$0 & 0x20) == 0) {
missing.append(" interruptFirstOnSoftDeadline");
}
if ((set$0 & 0x40) == 0) {
missing.append(" cyclesPerSecond");
}
if ((set$0 & 0x80) == 0) {
missing.append(" waitForDaemonRequestThreads");
}
if (this.environment == null) {
missing.append(" environment");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new RequestManager(
this.softDeadlineDelay,
this.hardDeadlineDelay,
this.disableDeadlineTimers,
this.runtimeLogSink,
this.apiProxyImpl,
this.maxOutstandingApiRpcs,
this.threadStopTerminatesClone,
this.interruptFirstOnSoftDeadline,
this.cyclesPerSecond,
this.waitForDaemonRequestThreads,
this.environment);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy