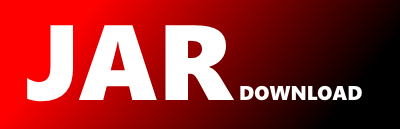
com.google.apphosting.runtime.AutoValue_ServletEngineAdapter_Config Maven / Gradle / Ivy
package com.google.apphosting.runtime;
import com.google.apphosting.runtime.anyrpc.EvaluationRuntimeServerInterface;
import com.google.common.net.HostAndPort;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ServletEngineAdapter_Config extends ServletEngineAdapter.Config {
private final boolean useJettyHttpProxy;
private final String applicationRoot;
@Nullable
private final String fixedApplicationPath;
private final HostAndPort jettyHttpAddress;
private final boolean jettyReusePort;
private final int jettyRequestHeaderSize;
private final int jettyResponseHeaderSize;
@Nullable
private final EvaluationRuntimeServerInterface evaluationRuntimeServerInterface;
private final boolean passThroughPrivateHeaders;
private AutoValue_ServletEngineAdapter_Config(
boolean useJettyHttpProxy,
String applicationRoot,
@Nullable String fixedApplicationPath,
HostAndPort jettyHttpAddress,
boolean jettyReusePort,
int jettyRequestHeaderSize,
int jettyResponseHeaderSize,
@Nullable EvaluationRuntimeServerInterface evaluationRuntimeServerInterface,
boolean passThroughPrivateHeaders) {
this.useJettyHttpProxy = useJettyHttpProxy;
this.applicationRoot = applicationRoot;
this.fixedApplicationPath = fixedApplicationPath;
this.jettyHttpAddress = jettyHttpAddress;
this.jettyReusePort = jettyReusePort;
this.jettyRequestHeaderSize = jettyRequestHeaderSize;
this.jettyResponseHeaderSize = jettyResponseHeaderSize;
this.evaluationRuntimeServerInterface = evaluationRuntimeServerInterface;
this.passThroughPrivateHeaders = passThroughPrivateHeaders;
}
@Override
public boolean useJettyHttpProxy() {
return useJettyHttpProxy;
}
@Override
public String applicationRoot() {
return applicationRoot;
}
@Nullable
@Override
public String fixedApplicationPath() {
return fixedApplicationPath;
}
@Override
public HostAndPort jettyHttpAddress() {
return jettyHttpAddress;
}
@Override
public boolean jettyReusePort() {
return jettyReusePort;
}
@Override
public int jettyRequestHeaderSize() {
return jettyRequestHeaderSize;
}
@Override
public int jettyResponseHeaderSize() {
return jettyResponseHeaderSize;
}
@Nullable
@Override
public EvaluationRuntimeServerInterface evaluationRuntimeServerInterface() {
return evaluationRuntimeServerInterface;
}
@Override
public boolean passThroughPrivateHeaders() {
return passThroughPrivateHeaders;
}
@Override
public String toString() {
return "Config{"
+ "useJettyHttpProxy=" + useJettyHttpProxy + ", "
+ "applicationRoot=" + applicationRoot + ", "
+ "fixedApplicationPath=" + fixedApplicationPath + ", "
+ "jettyHttpAddress=" + jettyHttpAddress + ", "
+ "jettyReusePort=" + jettyReusePort + ", "
+ "jettyRequestHeaderSize=" + jettyRequestHeaderSize + ", "
+ "jettyResponseHeaderSize=" + jettyResponseHeaderSize + ", "
+ "evaluationRuntimeServerInterface=" + evaluationRuntimeServerInterface + ", "
+ "passThroughPrivateHeaders=" + passThroughPrivateHeaders
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ServletEngineAdapter.Config) {
ServletEngineAdapter.Config that = (ServletEngineAdapter.Config) o;
return this.useJettyHttpProxy == that.useJettyHttpProxy()
&& this.applicationRoot.equals(that.applicationRoot())
&& (this.fixedApplicationPath == null ? that.fixedApplicationPath() == null : this.fixedApplicationPath.equals(that.fixedApplicationPath()))
&& this.jettyHttpAddress.equals(that.jettyHttpAddress())
&& this.jettyReusePort == that.jettyReusePort()
&& this.jettyRequestHeaderSize == that.jettyRequestHeaderSize()
&& this.jettyResponseHeaderSize == that.jettyResponseHeaderSize()
&& (this.evaluationRuntimeServerInterface == null ? that.evaluationRuntimeServerInterface() == null : this.evaluationRuntimeServerInterface.equals(that.evaluationRuntimeServerInterface()))
&& this.passThroughPrivateHeaders == that.passThroughPrivateHeaders();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= useJettyHttpProxy ? 1231 : 1237;
h$ *= 1000003;
h$ ^= applicationRoot.hashCode();
h$ *= 1000003;
h$ ^= (fixedApplicationPath == null) ? 0 : fixedApplicationPath.hashCode();
h$ *= 1000003;
h$ ^= jettyHttpAddress.hashCode();
h$ *= 1000003;
h$ ^= jettyReusePort ? 1231 : 1237;
h$ *= 1000003;
h$ ^= jettyRequestHeaderSize;
h$ *= 1000003;
h$ ^= jettyResponseHeaderSize;
h$ *= 1000003;
h$ ^= (evaluationRuntimeServerInterface == null) ? 0 : evaluationRuntimeServerInterface.hashCode();
h$ *= 1000003;
h$ ^= passThroughPrivateHeaders ? 1231 : 1237;
return h$;
}
static final class Builder extends ServletEngineAdapter.Config.Builder {
private boolean useJettyHttpProxy;
private String applicationRoot;
private String fixedApplicationPath;
private HostAndPort jettyHttpAddress;
private boolean jettyReusePort;
private int jettyRequestHeaderSize;
private int jettyResponseHeaderSize;
private EvaluationRuntimeServerInterface evaluationRuntimeServerInterface;
private boolean passThroughPrivateHeaders;
private byte set$0;
Builder() {
}
@Override
public ServletEngineAdapter.Config.Builder setUseJettyHttpProxy(boolean useJettyHttpProxy) {
this.useJettyHttpProxy = useJettyHttpProxy;
set$0 |= (byte) 1;
return this;
}
@Override
public ServletEngineAdapter.Config.Builder setApplicationRoot(String applicationRoot) {
if (applicationRoot == null) {
throw new NullPointerException("Null applicationRoot");
}
this.applicationRoot = applicationRoot;
return this;
}
@Override
public ServletEngineAdapter.Config.Builder setFixedApplicationPath(@Nullable String fixedApplicationPath) {
this.fixedApplicationPath = fixedApplicationPath;
return this;
}
@Override
public ServletEngineAdapter.Config.Builder setJettyHttpAddress(HostAndPort jettyHttpAddress) {
if (jettyHttpAddress == null) {
throw new NullPointerException("Null jettyHttpAddress");
}
this.jettyHttpAddress = jettyHttpAddress;
return this;
}
@Override
public ServletEngineAdapter.Config.Builder setJettyReusePort(boolean jettyReusePort) {
this.jettyReusePort = jettyReusePort;
set$0 |= (byte) 2;
return this;
}
@Override
public ServletEngineAdapter.Config.Builder setJettyRequestHeaderSize(int jettyRequestHeaderSize) {
this.jettyRequestHeaderSize = jettyRequestHeaderSize;
set$0 |= (byte) 4;
return this;
}
@Override
public ServletEngineAdapter.Config.Builder setJettyResponseHeaderSize(int jettyResponseHeaderSize) {
this.jettyResponseHeaderSize = jettyResponseHeaderSize;
set$0 |= (byte) 8;
return this;
}
@Override
public ServletEngineAdapter.Config.Builder setEvaluationRuntimeServerInterface(EvaluationRuntimeServerInterface evaluationRuntimeServerInterface) {
this.evaluationRuntimeServerInterface = evaluationRuntimeServerInterface;
return this;
}
@Override
public ServletEngineAdapter.Config.Builder setPassThroughPrivateHeaders(boolean passThroughPrivateHeaders) {
this.passThroughPrivateHeaders = passThroughPrivateHeaders;
set$0 |= (byte) 0x10;
return this;
}
@Override
public ServletEngineAdapter.Config build() {
if (set$0 != 0x1f
|| this.applicationRoot == null
|| this.jettyHttpAddress == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" useJettyHttpProxy");
}
if (this.applicationRoot == null) {
missing.append(" applicationRoot");
}
if (this.jettyHttpAddress == null) {
missing.append(" jettyHttpAddress");
}
if ((set$0 & 2) == 0) {
missing.append(" jettyReusePort");
}
if ((set$0 & 4) == 0) {
missing.append(" jettyRequestHeaderSize");
}
if ((set$0 & 8) == 0) {
missing.append(" jettyResponseHeaderSize");
}
if ((set$0 & 0x10) == 0) {
missing.append(" passThroughPrivateHeaders");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ServletEngineAdapter_Config(
this.useJettyHttpProxy,
this.applicationRoot,
this.fixedApplicationPath,
this.jettyHttpAddress,
this.jettyReusePort,
this.jettyRequestHeaderSize,
this.jettyResponseHeaderSize,
this.evaluationRuntimeServerInterface,
this.passThroughPrivateHeaders);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy