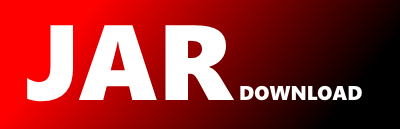
com.google.apphosting.runtime.http.AutoValue_HttpApiHostClient_Config Maven / Gradle / Ivy
package com.google.apphosting.runtime.http;
import java.util.OptionalInt;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_HttpApiHostClient_Config extends HttpApiHostClient.Config {
private final double extraTimeoutSeconds;
private final OptionalInt maxConnectionsPerDestination;
private final boolean ignoreContentLength;
private final boolean treatClosedChannelAsCancellation;
private AutoValue_HttpApiHostClient_Config(
double extraTimeoutSeconds,
OptionalInt maxConnectionsPerDestination,
boolean ignoreContentLength,
boolean treatClosedChannelAsCancellation) {
this.extraTimeoutSeconds = extraTimeoutSeconds;
this.maxConnectionsPerDestination = maxConnectionsPerDestination;
this.ignoreContentLength = ignoreContentLength;
this.treatClosedChannelAsCancellation = treatClosedChannelAsCancellation;
}
@Override
double extraTimeoutSeconds() {
return extraTimeoutSeconds;
}
@Override
OptionalInt maxConnectionsPerDestination() {
return maxConnectionsPerDestination;
}
@Override
boolean ignoreContentLength() {
return ignoreContentLength;
}
@Override
boolean treatClosedChannelAsCancellation() {
return treatClosedChannelAsCancellation;
}
@Override
public String toString() {
return "Config{"
+ "extraTimeoutSeconds=" + extraTimeoutSeconds + ", "
+ "maxConnectionsPerDestination=" + maxConnectionsPerDestination + ", "
+ "ignoreContentLength=" + ignoreContentLength + ", "
+ "treatClosedChannelAsCancellation=" + treatClosedChannelAsCancellation
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof HttpApiHostClient.Config) {
HttpApiHostClient.Config that = (HttpApiHostClient.Config) o;
return Double.doubleToLongBits(this.extraTimeoutSeconds) == Double.doubleToLongBits(that.extraTimeoutSeconds())
&& this.maxConnectionsPerDestination.equals(that.maxConnectionsPerDestination())
&& this.ignoreContentLength == that.ignoreContentLength()
&& this.treatClosedChannelAsCancellation == that.treatClosedChannelAsCancellation();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (int) ((Double.doubleToLongBits(extraTimeoutSeconds) >>> 32) ^ Double.doubleToLongBits(extraTimeoutSeconds));
h$ *= 1000003;
h$ ^= maxConnectionsPerDestination.hashCode();
h$ *= 1000003;
h$ ^= ignoreContentLength ? 1231 : 1237;
h$ *= 1000003;
h$ ^= treatClosedChannelAsCancellation ? 1231 : 1237;
return h$;
}
@Override
HttpApiHostClient.Config.Builder toBuilder() {
return new AutoValue_HttpApiHostClient_Config.Builder(this);
}
static final class Builder extends HttpApiHostClient.Config.Builder {
private double extraTimeoutSeconds;
private OptionalInt maxConnectionsPerDestination = OptionalInt.empty();
private boolean ignoreContentLength;
private boolean treatClosedChannelAsCancellation;
private byte set$0;
Builder() {
}
Builder(HttpApiHostClient.Config source) {
this.extraTimeoutSeconds = source.extraTimeoutSeconds();
this.maxConnectionsPerDestination = source.maxConnectionsPerDestination();
this.ignoreContentLength = source.ignoreContentLength();
this.treatClosedChannelAsCancellation = source.treatClosedChannelAsCancellation();
set$0 = (byte) 7;
}
@Override
HttpApiHostClient.Config.Builder setExtraTimeoutSeconds(double extraTimeoutSeconds) {
this.extraTimeoutSeconds = extraTimeoutSeconds;
set$0 |= (byte) 1;
return this;
}
@Override
HttpApiHostClient.Config.Builder setMaxConnectionsPerDestination(OptionalInt maxConnectionsPerDestination) {
if (maxConnectionsPerDestination == null) {
throw new NullPointerException("Null maxConnectionsPerDestination");
}
this.maxConnectionsPerDestination = maxConnectionsPerDestination;
return this;
}
@Override
HttpApiHostClient.Config.Builder setIgnoreContentLength(boolean ignoreContentLength) {
this.ignoreContentLength = ignoreContentLength;
set$0 |= (byte) 2;
return this;
}
@Override
HttpApiHostClient.Config.Builder setTreatClosedChannelAsCancellation(boolean treatClosedChannelAsCancellation) {
this.treatClosedChannelAsCancellation = treatClosedChannelAsCancellation;
set$0 |= (byte) 4;
return this;
}
@Override
HttpApiHostClient.Config build() {
if (set$0 != 7) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" extraTimeoutSeconds");
}
if ((set$0 & 2) == 0) {
missing.append(" ignoreContentLength");
}
if ((set$0 & 4) == 0) {
missing.append(" treatClosedChannelAsCancellation");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_HttpApiHostClient_Config(
this.extraTimeoutSeconds,
this.maxConnectionsPerDestination,
this.ignoreContentLength,
this.treatClosedChannelAsCancellation);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy