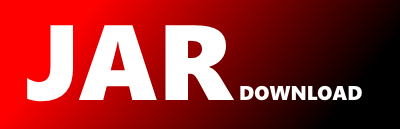
com.google.borg.borgcron.GrocLexer Maven / Gradle / Ivy
// $ANTLR 3.5.3 com/google/borg/borgcron/Groc.g 2024-09-17 20:19:06
// Copyright 2008 Google, Inc. All rights reserved.
package com.google.borg.borgcron;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
@SuppressWarnings("all")
public class GrocLexer extends Lexer {
public static final int EOF=-1;
public static final int APRIL=4;
public static final int AUGUST=5;
public static final int COMMA=6;
public static final int DAY=7;
public static final int DECEMBER=8;
public static final int DIGIT=9;
public static final int DIGITS=10;
public static final int EVERY=11;
public static final int FEBRUARY=12;
public static final int FIFTH=13;
public static final int FIRST=14;
public static final int FOURTH=15;
public static final int FOURTH_OR_FIFTH=16;
public static final int FRIDAY=17;
public static final int FROM=18;
public static final int HOURS=19;
public static final int JANUARY=20;
public static final int JULY=21;
public static final int JUNE=22;
public static final int MARCH=23;
public static final int MAY=24;
public static final int MINUTES=25;
public static final int MONDAY=26;
public static final int MONTH=27;
public static final int NOVEMBER=28;
public static final int OCTOBER=29;
public static final int OF=30;
public static final int QUARTER=31;
public static final int SATURDAY=32;
public static final int SECOND=33;
public static final int SEPTEMBER=34;
public static final int SUNDAY=35;
public static final int SYNCHRONIZED=36;
public static final int THIRD=37;
public static final int THURSDAY=38;
public static final int TIME=39;
public static final int TO=40;
public static final int TUESDAY=41;
public static final int TWO_DIGIT_HOUR_TIME=42;
public static final int UNKNOWN_TOKEN=43;
public static final int WEDNESDAY=44;
public static final int WS=45;
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public GrocLexer() {}
public GrocLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public GrocLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "com/google/borg/borgcron/Groc.g"; }
// $ANTLR start "TIME"
public final void mTIME() throws RecognitionException {
try {
int _type = TIME;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:354:11: ( ( DIGIT ':' '0' .. '5' DIGIT ) )
// com/google/borg/borgcron/Groc.g:354:13: ( DIGIT ':' '0' .. '5' DIGIT )
{
// com/google/borg/borgcron/Groc.g:354:13: ( DIGIT ':' '0' .. '5' DIGIT )
// com/google/borg/borgcron/Groc.g:354:15: DIGIT ':' '0' .. '5' DIGIT
{
mDIGIT(); if (state.failed) return;
match(':'); if (state.failed) return;
matchRange('0','5'); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TIME"
// $ANTLR start "TWO_DIGIT_HOUR_TIME"
public final void mTWO_DIGIT_HOUR_TIME() throws RecognitionException {
try {
int _type = TWO_DIGIT_HOUR_TIME;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:356:11: ( ( ( ( '0' DIGIT ) | ( '1' DIGIT ) | ( '2' '0' .. '3' ) ) ':' ( '0' .. '5' DIGIT ) ) )
// com/google/borg/borgcron/Groc.g:356:13: ( ( ( '0' DIGIT ) | ( '1' DIGIT ) | ( '2' '0' .. '3' ) ) ':' ( '0' .. '5' DIGIT ) )
{
// com/google/borg/borgcron/Groc.g:356:13: ( ( ( '0' DIGIT ) | ( '1' DIGIT ) | ( '2' '0' .. '3' ) ) ':' ( '0' .. '5' DIGIT ) )
// com/google/borg/borgcron/Groc.g:356:15: ( ( '0' DIGIT ) | ( '1' DIGIT ) | ( '2' '0' .. '3' ) ) ':' ( '0' .. '5' DIGIT )
{
// com/google/borg/borgcron/Groc.g:356:15: ( ( '0' DIGIT ) | ( '1' DIGIT ) | ( '2' '0' .. '3' ) )
int alt1=3;
switch ( input.LA(1) ) {
case '0':
{
alt1=1;
}
break;
case '1':
{
alt1=2;
}
break;
case '2':
{
alt1=3;
}
break;
default:
if (state.backtracking>0) {state.failed=true; return;}
NoViableAltException nvae =
new NoViableAltException("", 1, 0, input);
throw nvae;
}
switch (alt1) {
case 1 :
// com/google/borg/borgcron/Groc.g:356:17: ( '0' DIGIT )
{
// com/google/borg/borgcron/Groc.g:356:17: ( '0' DIGIT )
// com/google/borg/borgcron/Groc.g:356:19: '0' DIGIT
{
match('0'); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:356:33: ( '1' DIGIT )
{
// com/google/borg/borgcron/Groc.g:356:33: ( '1' DIGIT )
// com/google/borg/borgcron/Groc.g:356:34: '1' DIGIT
{
match('1'); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
break;
case 3 :
// com/google/borg/borgcron/Groc.g:356:47: ( '2' '0' .. '3' )
{
// com/google/borg/borgcron/Groc.g:356:47: ( '2' '0' .. '3' )
// com/google/borg/borgcron/Groc.g:356:48: '2' '0' .. '3'
{
match('2'); if (state.failed) return;
matchRange('0','3'); if (state.failed) return;
}
}
break;
}
match(':'); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:358:15: ( '0' .. '5' DIGIT )
// com/google/borg/borgcron/Groc.g:358:17: '0' .. '5' DIGIT
{
matchRange('0','5'); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
if ( state.backtracking==0 ) { _type = TIME; }
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TWO_DIGIT_HOUR_TIME"
// $ANTLR start "SYNCHRONIZED"
public final void mSYNCHRONIZED() throws RecognitionException {
try {
int _type = SYNCHRONIZED;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:360:14: ( 'synchronized' )
// com/google/borg/borgcron/Groc.g:360:16: 'synchronized'
{
match("synchronized"); if (state.failed) return;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SYNCHRONIZED"
// $ANTLR start "FIRST"
public final void mFIRST() throws RecognitionException {
try {
int _type = FIRST;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:363:11: ( ( '1st' | 'first' ) )
// com/google/borg/borgcron/Groc.g:363:13: ( '1st' | 'first' )
{
// com/google/borg/borgcron/Groc.g:363:13: ( '1st' | 'first' )
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0=='1') ) {
alt2=1;
}
else if ( (LA2_0=='f') ) {
alt2=2;
}
else {
if (state.backtracking>0) {state.failed=true; return;}
NoViableAltException nvae =
new NoViableAltException("", 2, 0, input);
throw nvae;
}
switch (alt2) {
case 1 :
// com/google/borg/borgcron/Groc.g:363:15: '1st'
{
match("1st"); if (state.failed) return;
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:363:23: 'first'
{
match("first"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FIRST"
// $ANTLR start "SECOND"
public final void mSECOND() throws RecognitionException {
try {
int _type = SECOND;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:364:11: ( ( '2nd' | 'second' ) )
// com/google/borg/borgcron/Groc.g:364:13: ( '2nd' | 'second' )
{
// com/google/borg/borgcron/Groc.g:364:13: ( '2nd' | 'second' )
int alt3=2;
int LA3_0 = input.LA(1);
if ( (LA3_0=='2') ) {
alt3=1;
}
else if ( (LA3_0=='s') ) {
alt3=2;
}
else {
if (state.backtracking>0) {state.failed=true; return;}
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// com/google/borg/borgcron/Groc.g:364:15: '2nd'
{
match("2nd"); if (state.failed) return;
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:364:23: 'second'
{
match("second"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SECOND"
// $ANTLR start "THIRD"
public final void mTHIRD() throws RecognitionException {
try {
int _type = THIRD;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:365:11: ( ( '3rd' | 'third' ) )
// com/google/borg/borgcron/Groc.g:365:13: ( '3rd' | 'third' )
{
// com/google/borg/borgcron/Groc.g:365:13: ( '3rd' | 'third' )
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0=='3') ) {
alt4=1;
}
else if ( (LA4_0=='t') ) {
alt4=2;
}
else {
if (state.backtracking>0) {state.failed=true; return;}
NoViableAltException nvae =
new NoViableAltException("", 4, 0, input);
throw nvae;
}
switch (alt4) {
case 1 :
// com/google/borg/borgcron/Groc.g:365:15: '3rd'
{
match("3rd"); if (state.failed) return;
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:365:23: 'third'
{
match("third"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "THIRD"
// $ANTLR start "FOURTH"
public final void mFOURTH() throws RecognitionException {
try {
int _type = FOURTH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:366:11: ( ( '4th' ) )
// com/google/borg/borgcron/Groc.g:366:13: ( '4th' )
{
// com/google/borg/borgcron/Groc.g:366:13: ( '4th' )
// com/google/borg/borgcron/Groc.g:366:15: '4th'
{
match("4th"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FOURTH"
// $ANTLR start "FIFTH"
public final void mFIFTH() throws RecognitionException {
try {
int _type = FIFTH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:367:11: ( ( '5th' ) )
// com/google/borg/borgcron/Groc.g:367:13: ( '5th' )
{
// com/google/borg/borgcron/Groc.g:367:13: ( '5th' )
// com/google/borg/borgcron/Groc.g:367:15: '5th'
{
match("5th"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FIFTH"
// $ANTLR start "FOURTH_OR_FIFTH"
public final void mFOURTH_OR_FIFTH() throws RecognitionException {
try {
int _type = FOURTH_OR_FIFTH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:374:11: ( ( ( 'fourth' ) | ( 'fifth' ) ) )
// com/google/borg/borgcron/Groc.g:374:13: ( ( 'fourth' ) | ( 'fifth' ) )
{
// com/google/borg/borgcron/Groc.g:374:13: ( ( 'fourth' ) | ( 'fifth' ) )
int alt5=2;
int LA5_0 = input.LA(1);
if ( (LA5_0=='f') ) {
int LA5_1 = input.LA(2);
if ( (LA5_1=='o') ) {
alt5=1;
}
else if ( (LA5_1=='i') ) {
alt5=2;
}
else {
if (state.backtracking>0) {state.failed=true; return;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 5, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
if (state.backtracking>0) {state.failed=true; return;}
NoViableAltException nvae =
new NoViableAltException("", 5, 0, input);
throw nvae;
}
switch (alt5) {
case 1 :
// com/google/borg/borgcron/Groc.g:374:15: ( 'fourth' )
{
// com/google/borg/borgcron/Groc.g:374:15: ( 'fourth' )
// com/google/borg/borgcron/Groc.g:374:16: 'fourth'
{
match("fourth"); if (state.failed) return;
if ( state.backtracking==0 ) { _type = FOURTH; }
}
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:375:15: ( 'fifth' )
{
// com/google/borg/borgcron/Groc.g:375:15: ( 'fifth' )
// com/google/borg/borgcron/Groc.g:375:16: 'fifth'
{
match("fifth"); if (state.failed) return;
if ( state.backtracking==0 ) { _type = FIFTH; }
}
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FOURTH_OR_FIFTH"
// $ANTLR start "DAY"
public final void mDAY() throws RecognitionException {
try {
int _type = DAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:378:11: ( 'day' )
// com/google/borg/borgcron/Groc.g:378:13: 'day'
{
match("day"); if (state.failed) return;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DAY"
// $ANTLR start "MONDAY"
public final void mMONDAY() throws RecognitionException {
try {
int _type = MONDAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:380:11: ( 'mon' ( 'day' )? )
// com/google/borg/borgcron/Groc.g:380:13: 'mon' ( 'day' )?
{
match("mon"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:380:19: ( 'day' )?
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0=='d') ) {
alt6=1;
}
switch (alt6) {
case 1 :
// com/google/borg/borgcron/Groc.g:380:20: 'day'
{
match("day"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MONDAY"
// $ANTLR start "TUESDAY"
public final void mTUESDAY() throws RecognitionException {
try {
int _type = TUESDAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:381:11: ( 'tue' ( 'sday' )? )
// com/google/borg/borgcron/Groc.g:381:13: 'tue' ( 'sday' )?
{
match("tue"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:381:19: ( 'sday' )?
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0=='s') ) {
alt7=1;
}
switch (alt7) {
case 1 :
// com/google/borg/borgcron/Groc.g:381:20: 'sday'
{
match("sday"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TUESDAY"
// $ANTLR start "WEDNESDAY"
public final void mWEDNESDAY() throws RecognitionException {
try {
int _type = WEDNESDAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:382:11: ( 'wed' ( 'nesday' )? )
// com/google/borg/borgcron/Groc.g:382:13: 'wed' ( 'nesday' )?
{
match("wed"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:382:19: ( 'nesday' )?
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0=='n') ) {
alt8=1;
}
switch (alt8) {
case 1 :
// com/google/borg/borgcron/Groc.g:382:20: 'nesday'
{
match("nesday"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WEDNESDAY"
// $ANTLR start "THURSDAY"
public final void mTHURSDAY() throws RecognitionException {
try {
int _type = THURSDAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:383:11: ( 'thu' ( 'rsday' )? )
// com/google/borg/borgcron/Groc.g:383:13: 'thu' ( 'rsday' )?
{
match("thu"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:383:19: ( 'rsday' )?
int alt9=2;
int LA9_0 = input.LA(1);
if ( (LA9_0=='r') ) {
alt9=1;
}
switch (alt9) {
case 1 :
// com/google/borg/borgcron/Groc.g:383:20: 'rsday'
{
match("rsday"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "THURSDAY"
// $ANTLR start "FRIDAY"
public final void mFRIDAY() throws RecognitionException {
try {
int _type = FRIDAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:384:11: ( 'fri' ( 'day' )? )
// com/google/borg/borgcron/Groc.g:384:13: 'fri' ( 'day' )?
{
match("fri"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:384:19: ( 'day' )?
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0=='d') ) {
alt10=1;
}
switch (alt10) {
case 1 :
// com/google/borg/borgcron/Groc.g:384:20: 'day'
{
match("day"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FRIDAY"
// $ANTLR start "SATURDAY"
public final void mSATURDAY() throws RecognitionException {
try {
int _type = SATURDAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:385:11: ( 'sat' ( 'urday' )? )
// com/google/borg/borgcron/Groc.g:385:13: 'sat' ( 'urday' )?
{
match("sat"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:385:19: ( 'urday' )?
int alt11=2;
int LA11_0 = input.LA(1);
if ( (LA11_0=='u') ) {
alt11=1;
}
switch (alt11) {
case 1 :
// com/google/borg/borgcron/Groc.g:385:20: 'urday'
{
match("urday"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SATURDAY"
// $ANTLR start "SUNDAY"
public final void mSUNDAY() throws RecognitionException {
try {
int _type = SUNDAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:386:11: ( 'sun' ( 'day' )? )
// com/google/borg/borgcron/Groc.g:386:13: 'sun' ( 'day' )?
{
match("sun"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:386:19: ( 'day' )?
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0=='d') ) {
alt12=1;
}
switch (alt12) {
case 1 :
// com/google/borg/borgcron/Groc.g:386:20: 'day'
{
match("day"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SUNDAY"
// $ANTLR start "JANUARY"
public final void mJANUARY() throws RecognitionException {
try {
int _type = JANUARY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:389:11: ( 'jan' ( 'uary' )? )
// com/google/borg/borgcron/Groc.g:389:13: 'jan' ( 'uary' )?
{
match("jan"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:389:19: ( 'uary' )?
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0=='u') ) {
alt13=1;
}
switch (alt13) {
case 1 :
// com/google/borg/borgcron/Groc.g:389:20: 'uary'
{
match("uary"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "JANUARY"
// $ANTLR start "FEBRUARY"
public final void mFEBRUARY() throws RecognitionException {
try {
int _type = FEBRUARY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:390:11: ( 'feb' ( 'ruary' )? )
// com/google/borg/borgcron/Groc.g:390:13: 'feb' ( 'ruary' )?
{
match("feb"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:390:19: ( 'ruary' )?
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0=='r') ) {
alt14=1;
}
switch (alt14) {
case 1 :
// com/google/borg/borgcron/Groc.g:390:20: 'ruary'
{
match("ruary"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FEBRUARY"
// $ANTLR start "MARCH"
public final void mMARCH() throws RecognitionException {
try {
int _type = MARCH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:391:11: ( 'mar' ( 'ch' )? )
// com/google/borg/borgcron/Groc.g:391:13: 'mar' ( 'ch' )?
{
match("mar"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:391:19: ( 'ch' )?
int alt15=2;
int LA15_0 = input.LA(1);
if ( (LA15_0=='c') ) {
alt15=1;
}
switch (alt15) {
case 1 :
// com/google/borg/borgcron/Groc.g:391:20: 'ch'
{
match("ch"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MARCH"
// $ANTLR start "APRIL"
public final void mAPRIL() throws RecognitionException {
try {
int _type = APRIL;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:392:11: ( 'apr' ( 'il' )? )
// com/google/borg/borgcron/Groc.g:392:13: 'apr' ( 'il' )?
{
match("apr"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:392:19: ( 'il' )?
int alt16=2;
int LA16_0 = input.LA(1);
if ( (LA16_0=='i') ) {
alt16=1;
}
switch (alt16) {
case 1 :
// com/google/borg/borgcron/Groc.g:392:20: 'il'
{
match("il"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "APRIL"
// $ANTLR start "MAY"
public final void mMAY() throws RecognitionException {
try {
int _type = MAY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:393:11: ( 'may' )
// com/google/borg/borgcron/Groc.g:393:13: 'may'
{
match("may"); if (state.failed) return;
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MAY"
// $ANTLR start "JUNE"
public final void mJUNE() throws RecognitionException {
try {
int _type = JUNE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:394:11: ( 'jun' ( 'e' )? )
// com/google/borg/borgcron/Groc.g:394:13: 'jun' ( 'e' )?
{
match("jun"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:394:19: ( 'e' )?
int alt17=2;
int LA17_0 = input.LA(1);
if ( (LA17_0=='e') ) {
alt17=1;
}
switch (alt17) {
case 1 :
// com/google/borg/borgcron/Groc.g:394:20: 'e'
{
match('e'); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "JUNE"
// $ANTLR start "JULY"
public final void mJULY() throws RecognitionException {
try {
int _type = JULY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:395:11: ( 'jul' ( 'y' )? )
// com/google/borg/borgcron/Groc.g:395:13: 'jul' ( 'y' )?
{
match("jul"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:395:19: ( 'y' )?
int alt18=2;
int LA18_0 = input.LA(1);
if ( (LA18_0=='y') ) {
alt18=1;
}
switch (alt18) {
case 1 :
// com/google/borg/borgcron/Groc.g:395:20: 'y'
{
match('y'); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "JULY"
// $ANTLR start "AUGUST"
public final void mAUGUST() throws RecognitionException {
try {
int _type = AUGUST;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:396:11: ( 'aug' ( 'ust' )? )
// com/google/borg/borgcron/Groc.g:396:13: 'aug' ( 'ust' )?
{
match("aug"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:396:19: ( 'ust' )?
int alt19=2;
int LA19_0 = input.LA(1);
if ( (LA19_0=='u') ) {
alt19=1;
}
switch (alt19) {
case 1 :
// com/google/borg/borgcron/Groc.g:396:20: 'ust'
{
match("ust"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "AUGUST"
// $ANTLR start "SEPTEMBER"
public final void mSEPTEMBER() throws RecognitionException {
try {
int _type = SEPTEMBER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:397:11: ( 'sep' ( 'tember' )? )
// com/google/borg/borgcron/Groc.g:397:13: 'sep' ( 'tember' )?
{
match("sep"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:397:19: ( 'tember' )?
int alt20=2;
int LA20_0 = input.LA(1);
if ( (LA20_0=='t') ) {
alt20=1;
}
switch (alt20) {
case 1 :
// com/google/borg/borgcron/Groc.g:397:20: 'tember'
{
match("tember"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SEPTEMBER"
// $ANTLR start "OCTOBER"
public final void mOCTOBER() throws RecognitionException {
try {
int _type = OCTOBER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:398:11: ( 'oct' ( 'ober' )? )
// com/google/borg/borgcron/Groc.g:398:13: 'oct' ( 'ober' )?
{
match("oct"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:398:19: ( 'ober' )?
int alt21=2;
int LA21_0 = input.LA(1);
if ( (LA21_0=='o') ) {
alt21=1;
}
switch (alt21) {
case 1 :
// com/google/borg/borgcron/Groc.g:398:20: 'ober'
{
match("ober"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OCTOBER"
// $ANTLR start "NOVEMBER"
public final void mNOVEMBER() throws RecognitionException {
try {
int _type = NOVEMBER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:399:11: ( 'nov' ( 'ember' )? )
// com/google/borg/borgcron/Groc.g:399:13: 'nov' ( 'ember' )?
{
match("nov"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:399:19: ( 'ember' )?
int alt22=2;
int LA22_0 = input.LA(1);
if ( (LA22_0=='e') ) {
alt22=1;
}
switch (alt22) {
case 1 :
// com/google/borg/borgcron/Groc.g:399:20: 'ember'
{
match("ember"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NOVEMBER"
// $ANTLR start "DECEMBER"
public final void mDECEMBER() throws RecognitionException {
try {
int _type = DECEMBER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:400:11: ( 'dec' ( 'ember' )? )
// com/google/borg/borgcron/Groc.g:400:13: 'dec' ( 'ember' )?
{
match("dec"); if (state.failed) return;
// com/google/borg/borgcron/Groc.g:400:19: ( 'ember' )?
int alt23=2;
int LA23_0 = input.LA(1);
if ( (LA23_0=='e') ) {
alt23=1;
}
switch (alt23) {
case 1 :
// com/google/borg/borgcron/Groc.g:400:20: 'ember'
{
match("ember"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DECEMBER"
// $ANTLR start "MONTH"
public final void mMONTH() throws RecognitionException {
try {
int _type = MONTH;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:402:11: ( ( 'month' ) )
// com/google/borg/borgcron/Groc.g:402:13: ( 'month' )
{
// com/google/borg/borgcron/Groc.g:402:13: ( 'month' )
// com/google/borg/borgcron/Groc.g:402:15: 'month'
{
match("month"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MONTH"
// $ANTLR start "QUARTER"
public final void mQUARTER() throws RecognitionException {
try {
int _type = QUARTER;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:403:11: ( ( 'quarter' ) )
// com/google/borg/borgcron/Groc.g:403:13: ( 'quarter' )
{
// com/google/borg/borgcron/Groc.g:403:13: ( 'quarter' )
// com/google/borg/borgcron/Groc.g:403:15: 'quarter'
{
match("quarter"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "QUARTER"
// $ANTLR start "EVERY"
public final void mEVERY() throws RecognitionException {
try {
int _type = EVERY;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:404:11: ( ( 'every' ) )
// com/google/borg/borgcron/Groc.g:404:13: ( 'every' )
{
// com/google/borg/borgcron/Groc.g:404:13: ( 'every' )
// com/google/borg/borgcron/Groc.g:404:15: 'every'
{
match("every"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "EVERY"
// $ANTLR start "HOURS"
public final void mHOURS() throws RecognitionException {
try {
int _type = HOURS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:406:11: ( ( 'hours' ) )
// com/google/borg/borgcron/Groc.g:406:13: ( 'hours' )
{
// com/google/borg/borgcron/Groc.g:406:13: ( 'hours' )
// com/google/borg/borgcron/Groc.g:406:15: 'hours'
{
match("hours"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HOURS"
// $ANTLR start "MINUTES"
public final void mMINUTES() throws RecognitionException {
try {
int _type = MINUTES;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:407:11: ( ( 'mins' | 'minutes' ) )
// com/google/borg/borgcron/Groc.g:407:13: ( 'mins' | 'minutes' )
{
// com/google/borg/borgcron/Groc.g:407:13: ( 'mins' | 'minutes' )
int alt24=2;
int LA24_0 = input.LA(1);
if ( (LA24_0=='m') ) {
int LA24_1 = input.LA(2);
if ( (LA24_1=='i') ) {
int LA24_2 = input.LA(3);
if ( (LA24_2=='n') ) {
int LA24_3 = input.LA(4);
if ( (LA24_3=='s') ) {
alt24=1;
}
else if ( (LA24_3=='u') ) {
alt24=2;
}
else {
if (state.backtracking>0) {state.failed=true; return;}
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 4 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 24, 3, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
if (state.backtracking>0) {state.failed=true; return;}
int nvaeMark = input.mark();
try {
for (int nvaeConsume = 0; nvaeConsume < 3 - 1; nvaeConsume++) {
input.consume();
}
NoViableAltException nvae =
new NoViableAltException("", 24, 2, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
if (state.backtracking>0) {state.failed=true; return;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 24, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
if (state.backtracking>0) {state.failed=true; return;}
NoViableAltException nvae =
new NoViableAltException("", 24, 0, input);
throw nvae;
}
switch (alt24) {
case 1 :
// com/google/borg/borgcron/Groc.g:407:15: 'mins'
{
match("mins"); if (state.failed) return;
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:407:24: 'minutes'
{
match("minutes"); if (state.failed) return;
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "MINUTES"
// $ANTLR start "COMMA"
public final void mCOMMA() throws RecognitionException {
try {
int _type = COMMA;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:410:11: ( ( ',' ) )
// com/google/borg/borgcron/Groc.g:410:13: ( ',' )
{
// com/google/borg/borgcron/Groc.g:410:13: ( ',' )
// com/google/borg/borgcron/Groc.g:410:15: ','
{
match(','); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "COMMA"
// $ANTLR start "OF"
public final void mOF() throws RecognitionException {
try {
int _type = OF;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:411:11: ( ( 'of' ) )
// com/google/borg/borgcron/Groc.g:411:13: ( 'of' )
{
// com/google/borg/borgcron/Groc.g:411:13: ( 'of' )
// com/google/borg/borgcron/Groc.g:411:15: 'of'
{
match("of"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "OF"
// $ANTLR start "FROM"
public final void mFROM() throws RecognitionException {
try {
int _type = FROM;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:412:11: ( ( 'from' ) )
// com/google/borg/borgcron/Groc.g:412:13: ( 'from' )
{
// com/google/borg/borgcron/Groc.g:412:13: ( 'from' )
// com/google/borg/borgcron/Groc.g:412:15: 'from'
{
match("from"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FROM"
// $ANTLR start "TO"
public final void mTO() throws RecognitionException {
try {
int _type = TO;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:413:11: ( ( 'to' ) )
// com/google/borg/borgcron/Groc.g:413:13: ( 'to' )
{
// com/google/borg/borgcron/Groc.g:413:13: ( 'to' )
// com/google/borg/borgcron/Groc.g:413:15: 'to'
{
match("to"); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "TO"
// $ANTLR start "WS"
public final void mWS() throws RecognitionException {
try {
int _type = WS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:414:11: ( ( ' ' | '\\t' | '\\n' | '\\r' ) )
// com/google/borg/borgcron/Groc.g:414:13: ( ' ' | '\\t' | '\\n' | '\\r' )
{
if ( (input.LA(1) >= '\t' && input.LA(1) <= '\n')||input.LA(1)=='\r'||input.LA(1)==' ' ) {
input.consume();
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return;}
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
if ( state.backtracking==0 ) { _channel=HIDDEN; }
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WS"
// $ANTLR start "DIGIT"
public final void mDIGIT() throws RecognitionException {
try {
int _type = DIGIT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:416:8: ( ( '0' .. '9' ) )
// com/google/borg/borgcron/Groc.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
state.failed=false;
}
else {
if (state.backtracking>0) {state.failed=true; return;}
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DIGIT"
// $ANTLR start "DIGITS"
public final void mDIGITS() throws RecognitionException {
try {
int _type = DIGITS;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:417:11: ( ( ( DIGIT DIGIT DIGIT DIGIT DIGIT )=> ( DIGIT DIGIT DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT DIGIT DIGIT )=> ( DIGIT DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT ) ) )
// com/google/borg/borgcron/Groc.g:417:13: ( ( DIGIT DIGIT DIGIT DIGIT DIGIT )=> ( DIGIT DIGIT DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT DIGIT DIGIT )=> ( DIGIT DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT ) )
{
// com/google/borg/borgcron/Groc.g:417:13: ( ( DIGIT DIGIT DIGIT DIGIT DIGIT )=> ( DIGIT DIGIT DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT DIGIT DIGIT )=> ( DIGIT DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT DIGIT ) | ( DIGIT DIGIT ) )
int alt25=4;
int LA25_0 = input.LA(1);
if ( ((LA25_0 >= '0' && LA25_0 <= '9')) ) {
int LA25_1 = input.LA(2);
if ( ((LA25_1 >= '0' && LA25_1 <= '9')) ) {
int LA25_2 = input.LA(3);
if ( ((LA25_2 >= '0' && LA25_2 <= '9')) ) {
int LA25_4 = input.LA(4);
if ( ((LA25_4 >= '0' && LA25_4 <= '9')) ) {
int LA25_6 = input.LA(5);
if ( ((LA25_6 >= '0' && LA25_6 <= '9')) && (synpred1_Groc())) {
alt25=1;
}
}
else {
alt25=3;
}
}
else {
alt25=4;
}
}
else {
if (state.backtracking>0) {state.failed=true; return;}
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 25, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else {
if (state.backtracking>0) {state.failed=true; return;}
NoViableAltException nvae =
new NoViableAltException("", 25, 0, input);
throw nvae;
}
switch (alt25) {
case 1 :
// com/google/borg/borgcron/Groc.g:417:15: ( DIGIT DIGIT DIGIT DIGIT DIGIT )=> ( DIGIT DIGIT DIGIT DIGIT DIGIT )
{
// com/google/borg/borgcron/Groc.g:417:52: ( DIGIT DIGIT DIGIT DIGIT DIGIT )
// com/google/borg/borgcron/Groc.g:417:54: DIGIT DIGIT DIGIT DIGIT DIGIT
{
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:418:15: ( DIGIT DIGIT DIGIT DIGIT )=> ( DIGIT DIGIT DIGIT DIGIT )
{
// com/google/borg/borgcron/Groc.g:418:46: ( DIGIT DIGIT DIGIT DIGIT )
// com/google/borg/borgcron/Groc.g:418:48: DIGIT DIGIT DIGIT DIGIT
{
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
break;
case 3 :
// com/google/borg/borgcron/Groc.g:419:15: ( DIGIT DIGIT DIGIT )
{
// com/google/borg/borgcron/Groc.g:419:15: ( DIGIT DIGIT DIGIT )
// com/google/borg/borgcron/Groc.g:419:17: DIGIT DIGIT DIGIT
{
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
break;
case 4 :
// com/google/borg/borgcron/Groc.g:420:15: ( DIGIT DIGIT )
{
// com/google/borg/borgcron/Groc.g:420:15: ( DIGIT DIGIT )
// com/google/borg/borgcron/Groc.g:420:17: DIGIT DIGIT
{
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
break;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DIGITS"
// $ANTLR start "UNKNOWN_TOKEN"
public final void mUNKNOWN_TOKEN() throws RecognitionException {
try {
int _type = UNKNOWN_TOKEN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com/google/borg/borgcron/Groc.g:425:15: ( ( . ) )
// com/google/borg/borgcron/Groc.g:425:17: ( . )
{
// com/google/borg/borgcron/Groc.g:425:17: ( . )
// com/google/borg/borgcron/Groc.g:425:19: .
{
matchAny(); if (state.failed) return;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "UNKNOWN_TOKEN"
@Override
public void mTokens() throws RecognitionException {
// com/google/borg/borgcron/Groc.g:1:8: ( TIME | TWO_DIGIT_HOUR_TIME | SYNCHRONIZED | FIRST | SECOND | THIRD | FOURTH | FIFTH | FOURTH_OR_FIFTH | DAY | MONDAY | TUESDAY | WEDNESDAY | THURSDAY | FRIDAY | SATURDAY | SUNDAY | JANUARY | FEBRUARY | MARCH | APRIL | MAY | JUNE | JULY | AUGUST | SEPTEMBER | OCTOBER | NOVEMBER | DECEMBER | MONTH | QUARTER | EVERY | HOURS | MINUTES | COMMA | OF | FROM | TO | WS | DIGIT | DIGITS | UNKNOWN_TOKEN )
int alt26=42;
alt26 = dfa26.predict(input);
switch (alt26) {
case 1 :
// com/google/borg/borgcron/Groc.g:1:10: TIME
{
mTIME(); if (state.failed) return;
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:1:15: TWO_DIGIT_HOUR_TIME
{
mTWO_DIGIT_HOUR_TIME(); if (state.failed) return;
}
break;
case 3 :
// com/google/borg/borgcron/Groc.g:1:35: SYNCHRONIZED
{
mSYNCHRONIZED(); if (state.failed) return;
}
break;
case 4 :
// com/google/borg/borgcron/Groc.g:1:48: FIRST
{
mFIRST(); if (state.failed) return;
}
break;
case 5 :
// com/google/borg/borgcron/Groc.g:1:54: SECOND
{
mSECOND(); if (state.failed) return;
}
break;
case 6 :
// com/google/borg/borgcron/Groc.g:1:61: THIRD
{
mTHIRD(); if (state.failed) return;
}
break;
case 7 :
// com/google/borg/borgcron/Groc.g:1:67: FOURTH
{
mFOURTH(); if (state.failed) return;
}
break;
case 8 :
// com/google/borg/borgcron/Groc.g:1:74: FIFTH
{
mFIFTH(); if (state.failed) return;
}
break;
case 9 :
// com/google/borg/borgcron/Groc.g:1:80: FOURTH_OR_FIFTH
{
mFOURTH_OR_FIFTH(); if (state.failed) return;
}
break;
case 10 :
// com/google/borg/borgcron/Groc.g:1:96: DAY
{
mDAY(); if (state.failed) return;
}
break;
case 11 :
// com/google/borg/borgcron/Groc.g:1:100: MONDAY
{
mMONDAY(); if (state.failed) return;
}
break;
case 12 :
// com/google/borg/borgcron/Groc.g:1:107: TUESDAY
{
mTUESDAY(); if (state.failed) return;
}
break;
case 13 :
// com/google/borg/borgcron/Groc.g:1:115: WEDNESDAY
{
mWEDNESDAY(); if (state.failed) return;
}
break;
case 14 :
// com/google/borg/borgcron/Groc.g:1:125: THURSDAY
{
mTHURSDAY(); if (state.failed) return;
}
break;
case 15 :
// com/google/borg/borgcron/Groc.g:1:134: FRIDAY
{
mFRIDAY(); if (state.failed) return;
}
break;
case 16 :
// com/google/borg/borgcron/Groc.g:1:141: SATURDAY
{
mSATURDAY(); if (state.failed) return;
}
break;
case 17 :
// com/google/borg/borgcron/Groc.g:1:150: SUNDAY
{
mSUNDAY(); if (state.failed) return;
}
break;
case 18 :
// com/google/borg/borgcron/Groc.g:1:157: JANUARY
{
mJANUARY(); if (state.failed) return;
}
break;
case 19 :
// com/google/borg/borgcron/Groc.g:1:165: FEBRUARY
{
mFEBRUARY(); if (state.failed) return;
}
break;
case 20 :
// com/google/borg/borgcron/Groc.g:1:174: MARCH
{
mMARCH(); if (state.failed) return;
}
break;
case 21 :
// com/google/borg/borgcron/Groc.g:1:180: APRIL
{
mAPRIL(); if (state.failed) return;
}
break;
case 22 :
// com/google/borg/borgcron/Groc.g:1:186: MAY
{
mMAY(); if (state.failed) return;
}
break;
case 23 :
// com/google/borg/borgcron/Groc.g:1:190: JUNE
{
mJUNE(); if (state.failed) return;
}
break;
case 24 :
// com/google/borg/borgcron/Groc.g:1:195: JULY
{
mJULY(); if (state.failed) return;
}
break;
case 25 :
// com/google/borg/borgcron/Groc.g:1:200: AUGUST
{
mAUGUST(); if (state.failed) return;
}
break;
case 26 :
// com/google/borg/borgcron/Groc.g:1:207: SEPTEMBER
{
mSEPTEMBER(); if (state.failed) return;
}
break;
case 27 :
// com/google/borg/borgcron/Groc.g:1:217: OCTOBER
{
mOCTOBER(); if (state.failed) return;
}
break;
case 28 :
// com/google/borg/borgcron/Groc.g:1:225: NOVEMBER
{
mNOVEMBER(); if (state.failed) return;
}
break;
case 29 :
// com/google/borg/borgcron/Groc.g:1:234: DECEMBER
{
mDECEMBER(); if (state.failed) return;
}
break;
case 30 :
// com/google/borg/borgcron/Groc.g:1:243: MONTH
{
mMONTH(); if (state.failed) return;
}
break;
case 31 :
// com/google/borg/borgcron/Groc.g:1:249: QUARTER
{
mQUARTER(); if (state.failed) return;
}
break;
case 32 :
// com/google/borg/borgcron/Groc.g:1:257: EVERY
{
mEVERY(); if (state.failed) return;
}
break;
case 33 :
// com/google/borg/borgcron/Groc.g:1:263: HOURS
{
mHOURS(); if (state.failed) return;
}
break;
case 34 :
// com/google/borg/borgcron/Groc.g:1:269: MINUTES
{
mMINUTES(); if (state.failed) return;
}
break;
case 35 :
// com/google/borg/borgcron/Groc.g:1:277: COMMA
{
mCOMMA(); if (state.failed) return;
}
break;
case 36 :
// com/google/borg/borgcron/Groc.g:1:283: OF
{
mOF(); if (state.failed) return;
}
break;
case 37 :
// com/google/borg/borgcron/Groc.g:1:286: FROM
{
mFROM(); if (state.failed) return;
}
break;
case 38 :
// com/google/borg/borgcron/Groc.g:1:291: TO
{
mTO(); if (state.failed) return;
}
break;
case 39 :
// com/google/borg/borgcron/Groc.g:1:294: WS
{
mWS(); if (state.failed) return;
}
break;
case 40 :
// com/google/borg/borgcron/Groc.g:1:297: DIGIT
{
mDIGIT(); if (state.failed) return;
}
break;
case 41 :
// com/google/borg/borgcron/Groc.g:1:303: DIGITS
{
mDIGITS(); if (state.failed) return;
}
break;
case 42 :
// com/google/borg/borgcron/Groc.g:1:310: UNKNOWN_TOKEN
{
mUNKNOWN_TOKEN(); if (state.failed) return;
}
break;
}
}
// $ANTLR start synpred1_Groc
public final void synpred1_Groc_fragment() throws RecognitionException {
// com/google/borg/borgcron/Groc.g:417:15: ( DIGIT DIGIT DIGIT DIGIT DIGIT )
// com/google/borg/borgcron/Groc.g:417:17: DIGIT DIGIT DIGIT DIGIT DIGIT
{
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
// $ANTLR end synpred1_Groc
// $ANTLR start synpred2_Groc
public final void synpred2_Groc_fragment() throws RecognitionException {
// com/google/borg/borgcron/Groc.g:418:15: ( DIGIT DIGIT DIGIT DIGIT )
// com/google/borg/borgcron/Groc.g:418:17: DIGIT DIGIT DIGIT DIGIT
{
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
mDIGIT(); if (state.failed) return;
}
}
// $ANTLR end synpred2_Groc
public final boolean synpred2_Groc() {
state.backtracking++;
int start = input.mark();
try {
synpred2_Groc_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
public final boolean synpred1_Groc() {
state.backtracking++;
int start = input.mark();
try {
synpred1_Groc_fragment(); // can never throw exception
} catch (RecognitionException re) {
System.err.println("impossible: "+re);
}
boolean success = !state.failed;
input.rewind(start);
state.backtracking--;
state.failed=false;
return success;
}
protected DFA26 dfa26 = new DFA26(this);
static final String DFA26_eotS =
"\1\uffff\4\30\2\27\1\30\1\27\2\30\12\27\5\uffff\1\37\1\uffff\2\37\47\uffff"+
"\1\113\6\uffff";
static final String DFA26_eofS =
"\114\uffff";
static final String DFA26_minS =
"\1\0\4\60\1\141\1\145\1\60\1\150\2\60\2\141\1\145\1\141\1\160\1\143\1"+
"\157\1\165\1\166\1\157\5\uffff\1\72\1\uffff\2\72\4\uffff\1\143\2\uffff"+
"\1\146\1\uffff\1\151\2\uffff\1\151\5\uffff\1\156\1\162\3\uffff\1\154\17"+
"\uffff\1\164\6\uffff";
static final String DFA26_maxS =
"\1\uffff\1\72\1\163\1\156\1\162\1\171\1\162\1\164\1\165\1\164\1\72\1\145"+
"\1\157\1\145\2\165\1\146\1\157\1\165\1\166\1\157\5\uffff\1\72\1\uffff"+
"\2\72\4\uffff\1\160\2\uffff\1\162\1\uffff\1\157\2\uffff\1\165\5\uffff"+
"\1\156\1\171\3\uffff\1\156\17\uffff\1\164\6\uffff";
static final String DFA26_acceptS =
"\25\uffff\1\43\1\47\1\52\1\50\1\1\1\uffff\1\4\2\uffff\1\5\1\51\1\6\1\3"+
"\1\uffff\1\20\1\21\1\uffff\1\11\1\uffff\1\23\1\7\1\uffff\1\14\1\46\1\10"+
"\1\12\1\35\2\uffff\1\42\1\15\1\22\1\uffff\1\25\1\31\1\33\1\44\1\34\1\37"+
"\1\40\1\41\1\43\1\47\1\2\1\32\1\17\1\45\1\16\1\uffff\1\24\1\26\1\27\1"+
"\30\1\36\1\13";
static final String DFA26_specialS =
"\1\0\113\uffff}>";
static final String[] DFA26_transitionS = {
"\11\27\2\26\2\27\1\26\22\27\1\26\13\27\1\25\3\27\1\1\1\2\1\3\1\4\1\7"+
"\1\11\4\12\47\27\1\17\2\27\1\13\1\23\1\6\1\27\1\24\1\27\1\16\2\27\1\14"+
"\1\21\1\20\1\27\1\22\1\27\1\5\1\10\2\27\1\15\uff88\27",
"\12\32\1\31",
"\12\34\1\31\70\uffff\1\33",
"\4\35\6\37\1\31\63\uffff\1\36",
"\12\37\1\31\67\uffff\1\40",
"\1\43\3\uffff\1\42\17\uffff\1\44\3\uffff\1\41",
"\1\50\3\uffff\1\45\5\uffff\1\46\2\uffff\1\47",
"\12\37\1\31\71\uffff\1\51",
"\1\52\6\uffff\1\54\5\uffff\1\53",
"\12\37\1\31\71\uffff\1\55",
"\12\37\1\31",
"\1\56\3\uffff\1\57",
"\1\61\7\uffff\1\62\5\uffff\1\60",
"\1\63",
"\1\64\23\uffff\1\65",
"\1\66\4\uffff\1\67",
"\1\70\2\uffff\1\71",
"\1\72",
"\1\73",
"\1\74",
"\1\75",
"",
"",
"",
"",
"",
"\1\100",
"",
"\1\100",
"\1\100",
"",
"",
"",
"",
"\1\36\14\uffff\1\101",
"",
"",
"\1\46\13\uffff\1\33",
"",
"\1\102\5\uffff\1\103",
"",
"",
"\1\40\13\uffff\1\104",
"",
"",
"",
"",
"",
"\1\105",
"\1\106\6\uffff\1\107",
"",
"",
"",
"\1\111\1\uffff\1\110",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"",
"\1\112",
"",
"",
"",
"",
"",
""
};
static final short[] DFA26_eot = DFA.unpackEncodedString(DFA26_eotS);
static final short[] DFA26_eof = DFA.unpackEncodedString(DFA26_eofS);
static final char[] DFA26_min = DFA.unpackEncodedStringToUnsignedChars(DFA26_minS);
static final char[] DFA26_max = DFA.unpackEncodedStringToUnsignedChars(DFA26_maxS);
static final short[] DFA26_accept = DFA.unpackEncodedString(DFA26_acceptS);
static final short[] DFA26_special = DFA.unpackEncodedString(DFA26_specialS);
static final short[][] DFA26_transition;
static {
int numStates = DFA26_transitionS.length;
DFA26_transition = new short[numStates][];
for (int i=0; i= '6' && LA26_0 <= '9')) ) {s = 10;}
else if ( (LA26_0=='d') ) {s = 11;}
else if ( (LA26_0=='m') ) {s = 12;}
else if ( (LA26_0=='w') ) {s = 13;}
else if ( (LA26_0=='j') ) {s = 14;}
else if ( (LA26_0=='a') ) {s = 15;}
else if ( (LA26_0=='o') ) {s = 16;}
else if ( (LA26_0=='n') ) {s = 17;}
else if ( (LA26_0=='q') ) {s = 18;}
else if ( (LA26_0=='e') ) {s = 19;}
else if ( (LA26_0=='h') ) {s = 20;}
else if ( (LA26_0==',') ) {s = 21;}
else if ( ((LA26_0 >= '\t' && LA26_0 <= '\n')||LA26_0=='\r'||LA26_0==' ') ) {s = 22;}
else if ( ((LA26_0 >= '\u0000' && LA26_0 <= '\b')||(LA26_0 >= '\u000B' && LA26_0 <= '\f')||(LA26_0 >= '\u000E' && LA26_0 <= '\u001F')||(LA26_0 >= '!' && LA26_0 <= '+')||(LA26_0 >= '-' && LA26_0 <= '/')||(LA26_0 >= ':' && LA26_0 <= '`')||(LA26_0 >= 'b' && LA26_0 <= 'c')||LA26_0=='g'||LA26_0=='i'||(LA26_0 >= 'k' && LA26_0 <= 'l')||LA26_0=='p'||LA26_0=='r'||(LA26_0 >= 'u' && LA26_0 <= 'v')||(LA26_0 >= 'x' && LA26_0 <= '\uFFFF')) ) {s = 23;}
if ( s>=0 ) return s;
break;
}
if (state.backtracking>0) {state.failed=true; return -1;}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 26, _s, input);
error(nvae);
throw nvae;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy