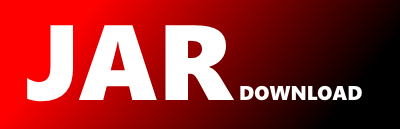
com.google.borg.borgcron.GrocParser Maven / Gradle / Ivy
// $ANTLR 3.5.3 com/google/borg/borgcron/Groc.g 2024-09-17 20:19:06
// Copyright 2008 Google, Inc. All rights reserved.
// Groc (Googley runner of commands) is a microlanguage that provides an
// alternative to traditional cron syntax/semantics for specifying
// recurrent events. Syntactically, it is designed to be more readable
// (more easily "grokked") than crontab language. Groc forfeits certain
// semantics found in crontab, in favor of readability; however,
// certain timespecs which are awkward in crontab are much easier
// to express in Groc (for example, the 3rd tuesday of the month).
// It is these constructs to which Groc is best suited.
//
// Examples of valid Groc include:
// "1st,3rd monday of month 15:30"
// "every wed,fri of jan,jun 13:15"
// "first sunday of quarter 00:00"
//
// FEATURES NOT YET IMPLEMENTED (in approx. order of priority):
// - some way to specify multiple values for minutes/hours (definitely)
// - "am/pm" (probably)
// - other range/interval functionality (maybe)
package com.google.borg.borgcron;
import java.util.Set;
import java.util.TreeSet;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
@SuppressWarnings("all")
public class GrocParser extends Parser {
public static final String[] tokenNames = new String[] {
"", "", "", "", "APRIL", "AUGUST", "COMMA", "DAY",
"DECEMBER", "DIGIT", "DIGITS", "EVERY", "FEBRUARY", "FIFTH", "FIRST",
"FOURTH", "FOURTH_OR_FIFTH", "FRIDAY", "FROM", "HOURS", "JANUARY", "JULY",
"JUNE", "MARCH", "MAY", "MINUTES", "MONDAY", "MONTH", "NOVEMBER", "OCTOBER",
"OF", "QUARTER", "SATURDAY", "SECOND", "SEPTEMBER", "SUNDAY", "SYNCHRONIZED",
"THIRD", "THURSDAY", "TIME", "TO", "TUESDAY", "TWO_DIGIT_HOUR_TIME", "UNKNOWN_TOKEN",
"WEDNESDAY", "WS"
};
public static final int EOF=-1;
public static final int APRIL=4;
public static final int AUGUST=5;
public static final int COMMA=6;
public static final int DAY=7;
public static final int DECEMBER=8;
public static final int DIGIT=9;
public static final int DIGITS=10;
public static final int EVERY=11;
public static final int FEBRUARY=12;
public static final int FIFTH=13;
public static final int FIRST=14;
public static final int FOURTH=15;
public static final int FOURTH_OR_FIFTH=16;
public static final int FRIDAY=17;
public static final int FROM=18;
public static final int HOURS=19;
public static final int JANUARY=20;
public static final int JULY=21;
public static final int JUNE=22;
public static final int MARCH=23;
public static final int MAY=24;
public static final int MINUTES=25;
public static final int MONDAY=26;
public static final int MONTH=27;
public static final int NOVEMBER=28;
public static final int OCTOBER=29;
public static final int OF=30;
public static final int QUARTER=31;
public static final int SATURDAY=32;
public static final int SECOND=33;
public static final int SEPTEMBER=34;
public static final int SUNDAY=35;
public static final int SYNCHRONIZED=36;
public static final int THIRD=37;
public static final int THURSDAY=38;
public static final int TIME=39;
public static final int TO=40;
public static final int TUESDAY=41;
public static final int TWO_DIGIT_HOUR_TIME=42;
public static final int UNKNOWN_TOKEN=43;
public static final int WEDNESDAY=44;
public static final int WS=45;
// delegates
public Parser[] getDelegates() {
return new Parser[] {};
}
// delegators
public GrocParser(TokenStream input) {
this(input, new RecognizerSharedState());
}
public GrocParser(TokenStream input, RecognizerSharedState state) {
super(input, state);
}
@Override public String[] getTokenNames() { return GrocParser.tokenNames; }
@Override public String getGrammarFileName() { return "com/google/borg/borgcron/Groc.g"; }
Set allOrdinals = integerSetOf(1,2,3,4,5);
private Set months;
private Set ordinals;
private Set weekdays;
private Set monthdays;
private String interval;
private String period;
private String time;
private boolean synchronize;
private String startTime;
private String endTime;
/**
* Initialises the parser.
*/
public void init() {
ordinals = new TreeSet();
weekdays = new TreeSet();
months = new TreeSet();
monthdays = new TreeSet();
time = "";
interval = "";
period = "";
synchronize = false;
startTime = "";
endTime = "";
}
public Set getMonths() {
return months;
}
public Set getOrdinals() {
return ordinals;
}
public Set getWeekdays() {
return weekdays;
}
public Set getMonthdays() {
return monthdays;
}
public String getTime() {
return time;
}
public Integer getInterval() {
if (interval == null || interval.equals("")) {
return null;
}
return Integer.parseInt(interval);
}
public String getIntervalPeriod() {
if (period.equals("minutes")) {
return "mins";
}
return period;
}
public boolean getSynchronized() {
return synchronize;
}
public String getStartTime() {
return startTime;
}
public String getEndTime() {
return endTime;
}
// Convert date tokens to int representations of properties.
static int valueOf(int token_type) {
switch(token_type) {
case SUNDAY:
{ return 0; }
case FIRST:
case MONDAY:
case JANUARY:
{ return 1; }
case TUESDAY:
case SECOND:
case FEBRUARY:
{ return 2; }
case WEDNESDAY:
case THIRD:
case MARCH:
{ return 3; }
case THURSDAY:
case FOURTH:
case APRIL:
{ return 4; }
case FRIDAY:
case FIFTH:
case MAY:
{ return 5; }
case SATURDAY:
case JUNE:
{ return 6; }
case JULY:
{ return 7; }
case AUGUST:
{ return 8; }
case SEPTEMBER:
{ return 9; }
case OCTOBER:
{ return 10; }
case NOVEMBER:
{ return 11; }
case DECEMBER:
{ return 12; }
default:
{ return -1; }
}
}
// These three methods make any errors raise an exception immediately
@Override
public boolean mismatchIsUnwantedToken(IntStream input, int ttype) {
return false;
}
@Override
public boolean mismatchIsMissingToken(IntStream input, BitSet follow) {
return false;
}
@Override
public Object recoverFromMismatchedSet(IntStream input,
RecognitionException e, BitSet follow) throws RecognitionException {
throw e;
}
/** Returns a set of the given elements */
private static Set integerSetOf(Integer... elements) {
Set newSet = new TreeSet();
for (Integer element : elements) {
newSet.add(element);
}
return newSet;
}
// $ANTLR start "timespec"
// com/google/borg/borgcron/Groc.g:212:1: timespec : ( specifictime | interval ) EOF ;
public final void timespec() throws RecognitionException {
try {
// com/google/borg/borgcron/Groc.g:213:3: ( ( specifictime | interval ) EOF )
// com/google/borg/borgcron/Groc.g:213:5: ( specifictime | interval ) EOF
{
// com/google/borg/borgcron/Groc.g:213:5: ( specifictime | interval )
int alt1=2;
int LA1_0 = input.LA(1);
if ( (LA1_0==EVERY) ) {
int LA1_1 = input.LA(2);
if ( ((LA1_1 >= DIGIT && LA1_1 <= DIGITS)) ) {
alt1=2;
}
else if ( (LA1_1==DAY||LA1_1==FRIDAY||LA1_1==MONDAY||LA1_1==SATURDAY||LA1_1==SUNDAY||LA1_1==THURSDAY||LA1_1==TUESDAY||LA1_1==WEDNESDAY) ) {
alt1=1;
}
else {
int nvaeMark = input.mark();
try {
input.consume();
NoViableAltException nvae =
new NoViableAltException("", 1, 1, input);
throw nvae;
} finally {
input.rewind(nvaeMark);
}
}
}
else if ( ((LA1_0 >= DIGIT && LA1_0 <= DIGITS)||(LA1_0 >= FIFTH && LA1_0 <= FOURTH)||LA1_0==SECOND||LA1_0==THIRD) ) {
alt1=1;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 1, 0, input);
throw nvae;
}
switch (alt1) {
case 1 :
// com/google/borg/borgcron/Groc.g:213:7: specifictime
{
pushFollow(FOLLOW_specifictime_in_timespec56);
specifictime();
state._fsp--;
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:213:22: interval
{
pushFollow(FOLLOW_interval_in_timespec60);
interval();
state._fsp--;
}
break;
}
match(input,EOF,FOLLOW_EOF_in_timespec64);
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "timespec"
// $ANTLR start "specifictime"
// com/google/borg/borgcron/Groc.g:216:1: specifictime : ( ( ( ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec ) ) | ( ordinals weekdays ) ) TIME ) ;
public final void specifictime() throws RecognitionException {
Token TIME1=null;
try {
// com/google/borg/borgcron/Groc.g:217:3: ( ( ( ( ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec ) ) | ( ordinals weekdays ) ) TIME ) )
// com/google/borg/borgcron/Groc.g:217:5: ( ( ( ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec ) ) | ( ordinals weekdays ) ) TIME )
{
// com/google/borg/borgcron/Groc.g:217:5: ( ( ( ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec ) ) | ( ordinals weekdays ) ) TIME )
// com/google/borg/borgcron/Groc.g:217:6: ( ( ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec ) ) | ( ordinals weekdays ) ) TIME
{
// com/google/borg/borgcron/Groc.g:217:6: ( ( ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec ) ) | ( ordinals weekdays ) )
int alt4=2;
alt4 = dfa4.predict(input);
switch (alt4) {
case 1 :
// com/google/borg/borgcron/Groc.g:217:7: ( ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec ) )
{
// com/google/borg/borgcron/Groc.g:217:7: ( ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec ) )
// com/google/borg/borgcron/Groc.g:217:9: ( ( ordinals weekdays ) | monthdays ) OF ( monthspec | quarterspec )
{
// com/google/borg/borgcron/Groc.g:217:9: ( ( ordinals weekdays ) | monthdays )
int alt2=2;
int LA2_0 = input.LA(1);
if ( (LA2_0==EVERY||(LA2_0 >= FIFTH && LA2_0 <= FOURTH)||LA2_0==SECOND||LA2_0==THIRD) ) {
alt2=1;
}
else if ( ((LA2_0 >= DIGIT && LA2_0 <= DIGITS)) ) {
alt2=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 2, 0, input);
throw nvae;
}
switch (alt2) {
case 1 :
// com/google/borg/borgcron/Groc.g:217:10: ( ordinals weekdays )
{
// com/google/borg/borgcron/Groc.g:217:10: ( ordinals weekdays )
// com/google/borg/borgcron/Groc.g:217:11: ordinals weekdays
{
pushFollow(FOLLOW_ordinals_in_specifictime83);
ordinals();
state._fsp--;
pushFollow(FOLLOW_weekdays_in_specifictime85);
weekdays();
state._fsp--;
}
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:217:30: monthdays
{
pushFollow(FOLLOW_monthdays_in_specifictime88);
monthdays();
state._fsp--;
}
break;
}
match(input,OF,FOLLOW_OF_in_specifictime91);
// com/google/borg/borgcron/Groc.g:217:44: ( monthspec | quarterspec )
int alt3=2;
int LA3_0 = input.LA(1);
if ( ((LA3_0 >= APRIL && LA3_0 <= AUGUST)||LA3_0==DECEMBER||LA3_0==FEBRUARY||(LA3_0 >= JANUARY && LA3_0 <= MAY)||(LA3_0 >= MONTH && LA3_0 <= OCTOBER)||LA3_0==SEPTEMBER) ) {
alt3=1;
}
else if ( (LA3_0==FIRST||LA3_0==QUARTER||LA3_0==SECOND||LA3_0==THIRD) ) {
alt3=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 3, 0, input);
throw nvae;
}
switch (alt3) {
case 1 :
// com/google/borg/borgcron/Groc.g:217:45: monthspec
{
pushFollow(FOLLOW_monthspec_in_specifictime94);
monthspec();
state._fsp--;
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:217:55: quarterspec
{
pushFollow(FOLLOW_quarterspec_in_specifictime96);
quarterspec();
state._fsp--;
}
break;
}
}
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:218:8: ( ordinals weekdays )
{
// com/google/borg/borgcron/Groc.g:218:8: ( ordinals weekdays )
// com/google/borg/borgcron/Groc.g:218:10: ordinals weekdays
{
pushFollow(FOLLOW_ordinals_in_specifictime110);
ordinals();
state._fsp--;
pushFollow(FOLLOW_weekdays_in_specifictime112);
weekdays();
state._fsp--;
Set allMonths = integerSetOf(
valueOf(JANUARY), valueOf(FEBRUARY), valueOf(MARCH), valueOf(APRIL),
valueOf(MAY), valueOf(JUNE), valueOf(JULY), valueOf(AUGUST),
valueOf(SEPTEMBER), valueOf(OCTOBER), valueOf(NOVEMBER),
valueOf(DECEMBER));
months.addAll(allMonths);
}
}
break;
}
TIME1=(Token)match(input,TIME,FOLLOW_TIME_in_specifictime127);
time = (TIME1!=null?TIME1.getText():null);
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "specifictime"
// $ANTLR start "interval"
// com/google/borg/borgcron/Groc.g:228:1: interval : ( EVERY intervalnum= ( DIGIT | DIGITS ) period ( time_range | ( SYNCHRONIZED ) )? ) ;
public final void interval() throws RecognitionException {
Token intervalnum=null;
ParserRuleReturnScope period2 =null;
try {
// com/google/borg/borgcron/Groc.g:229:3: ( ( EVERY intervalnum= ( DIGIT | DIGITS ) period ( time_range | ( SYNCHRONIZED ) )? ) )
// com/google/borg/borgcron/Groc.g:229:5: ( EVERY intervalnum= ( DIGIT | DIGITS ) period ( time_range | ( SYNCHRONIZED ) )? )
{
// com/google/borg/borgcron/Groc.g:229:5: ( EVERY intervalnum= ( DIGIT | DIGITS ) period ( time_range | ( SYNCHRONIZED ) )? )
// com/google/borg/borgcron/Groc.g:229:7: EVERY intervalnum= ( DIGIT | DIGITS ) period ( time_range | ( SYNCHRONIZED ) )?
{
match(input,EVERY,FOLLOW_EVERY_in_interval146);
intervalnum=input.LT(1);
if ( (input.LA(1) >= DIGIT && input.LA(1) <= DIGITS) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
interval = (intervalnum!=null?intervalnum.getText():null);
pushFollow(FOLLOW_period_in_interval172);
period2=period();
state._fsp--;
period = (period2!=null?input.toString(period2.start,period2.stop):null);
// com/google/borg/borgcron/Groc.g:236:7: ( time_range | ( SYNCHRONIZED ) )?
int alt5=3;
int LA5_0 = input.LA(1);
if ( (LA5_0==FROM) ) {
alt5=1;
}
else if ( (LA5_0==SYNCHRONIZED) ) {
alt5=2;
}
switch (alt5) {
case 1 :
// com/google/borg/borgcron/Groc.g:236:9: time_range
{
pushFollow(FOLLOW_time_range_in_interval184);
time_range();
state._fsp--;
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:237:9: ( SYNCHRONIZED )
{
// com/google/borg/borgcron/Groc.g:237:9: ( SYNCHRONIZED )
// com/google/borg/borgcron/Groc.g:237:10: SYNCHRONIZED
{
match(input,SYNCHRONIZED,FOLLOW_SYNCHRONIZED_in_interval197);
synchronize = true;
}
}
break;
}
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "interval"
// $ANTLR start "ordinals"
// com/google/borg/borgcron/Groc.g:241:1: ordinals : ( EVERY | ( ordinal ( COMMA ordinal )* ) ) ;
public final void ordinals() throws RecognitionException {
try {
// com/google/borg/borgcron/Groc.g:242:3: ( ( EVERY | ( ordinal ( COMMA ordinal )* ) ) )
// com/google/borg/borgcron/Groc.g:242:5: ( EVERY | ( ordinal ( COMMA ordinal )* ) )
{
// com/google/borg/borgcron/Groc.g:242:5: ( EVERY | ( ordinal ( COMMA ordinal )* ) )
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0==EVERY) ) {
alt7=1;
}
else if ( ((LA7_0 >= FIFTH && LA7_0 <= FOURTH)||LA7_0==SECOND||LA7_0==THIRD) ) {
alt7=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 7, 0, input);
throw nvae;
}
switch (alt7) {
case 1 :
// com/google/borg/borgcron/Groc.g:242:7: EVERY
{
match(input,EVERY,FOLLOW_EVERY_in_ordinals226);
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:243:5: ( ordinal ( COMMA ordinal )* )
{
// com/google/borg/borgcron/Groc.g:243:5: ( ordinal ( COMMA ordinal )* )
// com/google/borg/borgcron/Groc.g:243:7: ordinal ( COMMA ordinal )*
{
pushFollow(FOLLOW_ordinal_in_ordinals234);
ordinal();
state._fsp--;
// com/google/borg/borgcron/Groc.g:243:15: ( COMMA ordinal )*
loop6:
while (true) {
int alt6=2;
int LA6_0 = input.LA(1);
if ( (LA6_0==COMMA) ) {
alt6=1;
}
switch (alt6) {
case 1 :
// com/google/borg/borgcron/Groc.g:243:16: COMMA ordinal
{
match(input,COMMA,FOLLOW_COMMA_in_ordinals237);
pushFollow(FOLLOW_ordinal_in_ordinals239);
ordinal();
state._fsp--;
}
break;
default :
break loop6;
}
}
}
}
break;
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ordinals"
// $ANTLR start "ordinal"
// com/google/borg/borgcron/Groc.g:246:1: ordinal : ord= ( FIRST | SECOND | THIRD | FOURTH | FIFTH ) ;
public final void ordinal() throws RecognitionException {
Token ord=null;
try {
// com/google/borg/borgcron/Groc.g:247:3: (ord= ( FIRST | SECOND | THIRD | FOURTH | FIFTH ) )
// com/google/borg/borgcron/Groc.g:247:5: ord= ( FIRST | SECOND | THIRD | FOURTH | FIFTH )
{
ord=input.LT(1);
if ( (input.LA(1) >= FIFTH && input.LA(1) <= FOURTH)||input.LA(1)==SECOND||input.LA(1)==THIRD ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
ordinals.add(valueOf((ord!=null?ord.getType():0)));
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ordinal"
public static class period_return extends ParserRuleReturnScope {
};
// $ANTLR start "period"
// com/google/borg/borgcron/Groc.g:252:1: period : ( HOURS | MINUTES ) ;
public final GrocParser.period_return period() throws RecognitionException {
GrocParser.period_return retval = new GrocParser.period_return();
retval.start = input.LT(1);
try {
// com/google/borg/borgcron/Groc.g:253:3: ( ( HOURS | MINUTES ) )
// com/google/borg/borgcron/Groc.g:
{
if ( input.LA(1)==HOURS||input.LA(1)==MINUTES ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
}
retval.stop = input.LT(-1);
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
return retval;
}
// $ANTLR end "period"
// $ANTLR start "monthdays"
// com/google/borg/borgcron/Groc.g:256:1: monthdays : ( monthday ( COMMA monthday )* ) ;
public final void monthdays() throws RecognitionException {
try {
// com/google/borg/borgcron/Groc.g:257:3: ( ( monthday ( COMMA monthday )* ) )
// com/google/borg/borgcron/Groc.g:257:5: ( monthday ( COMMA monthday )* )
{
// com/google/borg/borgcron/Groc.g:257:5: ( monthday ( COMMA monthday )* )
// com/google/borg/borgcron/Groc.g:257:7: monthday ( COMMA monthday )*
{
pushFollow(FOLLOW_monthday_in_monthdays318);
monthday();
state._fsp--;
// com/google/borg/borgcron/Groc.g:257:16: ( COMMA monthday )*
loop8:
while (true) {
int alt8=2;
int LA8_0 = input.LA(1);
if ( (LA8_0==COMMA) ) {
alt8=1;
}
switch (alt8) {
case 1 :
// com/google/borg/borgcron/Groc.g:257:18: COMMA monthday
{
match(input,COMMA,FOLLOW_COMMA_in_monthdays322);
pushFollow(FOLLOW_monthday_in_monthdays324);
monthday();
state._fsp--;
}
break;
default :
break loop8;
}
}
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "monthdays"
// $ANTLR start "monthday"
// com/google/borg/borgcron/Groc.g:260:1: monthday : day= ( DIGIT | DIGITS ) ;
public final void monthday() throws RecognitionException {
Token day=null;
try {
// com/google/borg/borgcron/Groc.g:261:3: (day= ( DIGIT | DIGITS ) )
// com/google/borg/borgcron/Groc.g:261:5: day= ( DIGIT | DIGITS )
{
day=input.LT(1);
if ( (input.LA(1) >= DIGIT && input.LA(1) <= DIGITS) ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
monthdays.add(Integer.parseInt((day!=null?day.getText():null)));
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "monthday"
// $ANTLR start "weekdays"
// com/google/borg/borgcron/Groc.g:265:1: weekdays : ( DAY | ( weekday ( COMMA weekday )* ) ) ;
public final void weekdays() throws RecognitionException {
try {
// com/google/borg/borgcron/Groc.g:266:3: ( ( DAY | ( weekday ( COMMA weekday )* ) ) )
// com/google/borg/borgcron/Groc.g:266:5: ( DAY | ( weekday ( COMMA weekday )* ) )
{
// com/google/borg/borgcron/Groc.g:266:5: ( DAY | ( weekday ( COMMA weekday )* ) )
int alt10=2;
int LA10_0 = input.LA(1);
if ( (LA10_0==DAY) ) {
alt10=1;
}
else if ( (LA10_0==FRIDAY||LA10_0==MONDAY||LA10_0==SATURDAY||LA10_0==SUNDAY||LA10_0==THURSDAY||LA10_0==TUESDAY||LA10_0==WEDNESDAY) ) {
alt10=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 10, 0, input);
throw nvae;
}
switch (alt10) {
case 1 :
// com/google/borg/borgcron/Groc.g:266:7: DAY
{
match(input,DAY,FOLLOW_DAY_in_weekdays369);
Set allDays = integerSetOf(
valueOf(MONDAY), valueOf(TUESDAY), valueOf(WEDNESDAY),
valueOf(THURSDAY), valueOf(FRIDAY), valueOf(SATURDAY),
valueOf(SUNDAY));
if (ordinals.isEmpty()) {
ordinals.addAll(allOrdinals);
weekdays.addAll(allDays);
} else {
// day means day of the month,
// not every day of the week.
monthdays = ordinals;
ordinals = new TreeSet();
}
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:280:11: ( weekday ( COMMA weekday )* )
{
// com/google/borg/borgcron/Groc.g:280:11: ( weekday ( COMMA weekday )* )
// com/google/borg/borgcron/Groc.g:280:13: weekday ( COMMA weekday )*
{
pushFollow(FOLLOW_weekday_in_weekdays377);
weekday();
state._fsp--;
// com/google/borg/borgcron/Groc.g:280:21: ( COMMA weekday )*
loop9:
while (true) {
int alt9=2;
int LA9_0 = input.LA(1);
if ( (LA9_0==COMMA) ) {
alt9=1;
}
switch (alt9) {
case 1 :
// com/google/borg/borgcron/Groc.g:280:22: COMMA weekday
{
match(input,COMMA,FOLLOW_COMMA_in_weekdays380);
pushFollow(FOLLOW_weekday_in_weekdays382);
weekday();
state._fsp--;
}
break;
default :
break loop9;
}
}
if (ordinals.isEmpty())
ordinals.addAll(allOrdinals);
}
}
break;
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "weekdays"
// $ANTLR start "weekday"
// com/google/borg/borgcron/Groc.g:286:1: weekday : dayname= ( MONDAY | TUESDAY | WEDNESDAY | THURSDAY | FRIDAY | SATURDAY | SUNDAY ) ;
public final void weekday() throws RecognitionException {
Token dayname=null;
try {
// com/google/borg/borgcron/Groc.g:287:3: (dayname= ( MONDAY | TUESDAY | WEDNESDAY | THURSDAY | FRIDAY | SATURDAY | SUNDAY ) )
// com/google/borg/borgcron/Groc.g:287:5: dayname= ( MONDAY | TUESDAY | WEDNESDAY | THURSDAY | FRIDAY | SATURDAY | SUNDAY )
{
dayname=input.LT(1);
if ( input.LA(1)==FRIDAY||input.LA(1)==MONDAY||input.LA(1)==SATURDAY||input.LA(1)==SUNDAY||input.LA(1)==THURSDAY||input.LA(1)==TUESDAY||input.LA(1)==WEDNESDAY ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
weekdays.add(valueOf((dayname!=null?dayname.getType():0)));
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "weekday"
// $ANTLR start "monthspec"
// com/google/borg/borgcron/Groc.g:292:1: monthspec : ( MONTH | months ) ;
public final void monthspec() throws RecognitionException {
try {
// com/google/borg/borgcron/Groc.g:293:3: ( ( MONTH | months ) )
// com/google/borg/borgcron/Groc.g:293:5: ( MONTH | months )
{
// com/google/borg/borgcron/Groc.g:293:5: ( MONTH | months )
int alt11=2;
int LA11_0 = input.LA(1);
if ( (LA11_0==MONTH) ) {
alt11=1;
}
else if ( ((LA11_0 >= APRIL && LA11_0 <= AUGUST)||LA11_0==DECEMBER||LA11_0==FEBRUARY||(LA11_0 >= JANUARY && LA11_0 <= MAY)||(LA11_0 >= NOVEMBER && LA11_0 <= OCTOBER)||LA11_0==SEPTEMBER) ) {
alt11=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 11, 0, input);
throw nvae;
}
switch (alt11) {
case 1 :
// com/google/borg/borgcron/Groc.g:293:7: MONTH
{
match(input,MONTH,FOLLOW_MONTH_in_monthspec449);
Set allMonths = integerSetOf(
valueOf(JANUARY), valueOf(FEBRUARY), valueOf(MARCH), valueOf(APRIL),
valueOf(MAY), valueOf(JUNE), valueOf(JULY), valueOf(AUGUST),
valueOf(SEPTEMBER), valueOf(OCTOBER), valueOf(NOVEMBER),
valueOf(DECEMBER));
months.addAll(allMonths);
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:301:7: months
{
pushFollow(FOLLOW_months_in_monthspec459);
months();
state._fsp--;
}
break;
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "monthspec"
// $ANTLR start "months"
// com/google/borg/borgcron/Groc.g:304:1: months : ( month ( COMMA month )* ) ;
public final void months() throws RecognitionException {
try {
// com/google/borg/borgcron/Groc.g:305:3: ( ( month ( COMMA month )* ) )
// com/google/borg/borgcron/Groc.g:305:5: ( month ( COMMA month )* )
{
// com/google/borg/borgcron/Groc.g:305:5: ( month ( COMMA month )* )
// com/google/borg/borgcron/Groc.g:305:7: month ( COMMA month )*
{
pushFollow(FOLLOW_month_in_months476);
month();
state._fsp--;
// com/google/borg/borgcron/Groc.g:305:13: ( COMMA month )*
loop12:
while (true) {
int alt12=2;
int LA12_0 = input.LA(1);
if ( (LA12_0==COMMA) ) {
alt12=1;
}
switch (alt12) {
case 1 :
// com/google/borg/borgcron/Groc.g:305:14: COMMA month
{
match(input,COMMA,FOLLOW_COMMA_in_months479);
pushFollow(FOLLOW_month_in_months481);
month();
state._fsp--;
}
break;
default :
break loop12;
}
}
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "months"
// $ANTLR start "month"
// com/google/borg/borgcron/Groc.g:308:1: month : monthname= ( JANUARY | FEBRUARY | MARCH | APRIL | MAY | JUNE | JULY | AUGUST | SEPTEMBER | OCTOBER | NOVEMBER | DECEMBER ) ;
public final void month() throws RecognitionException {
Token monthname=null;
try {
// com/google/borg/borgcron/Groc.g:309:3: (monthname= ( JANUARY | FEBRUARY | MARCH | APRIL | MAY | JUNE | JULY | AUGUST | SEPTEMBER | OCTOBER | NOVEMBER | DECEMBER ) )
// com/google/borg/borgcron/Groc.g:309:5: monthname= ( JANUARY | FEBRUARY | MARCH | APRIL | MAY | JUNE | JULY | AUGUST | SEPTEMBER | OCTOBER | NOVEMBER | DECEMBER )
{
monthname=input.LT(1);
if ( (input.LA(1) >= APRIL && input.LA(1) <= AUGUST)||input.LA(1)==DECEMBER||input.LA(1)==FEBRUARY||(input.LA(1) >= JANUARY && input.LA(1) <= MAY)||(input.LA(1) >= NOVEMBER && input.LA(1) <= OCTOBER)||input.LA(1)==SEPTEMBER ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
months.add(valueOf((monthname!=null?monthname.getType():0)));
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "month"
// $ANTLR start "quarterspec"
// com/google/borg/borgcron/Groc.g:314:1: quarterspec : ( QUARTER | ( quarter_ordinals MONTH OF QUARTER ) ) ;
public final void quarterspec() throws RecognitionException {
try {
// com/google/borg/borgcron/Groc.g:315:3: ( ( QUARTER | ( quarter_ordinals MONTH OF QUARTER ) ) )
// com/google/borg/borgcron/Groc.g:315:5: ( QUARTER | ( quarter_ordinals MONTH OF QUARTER ) )
{
// com/google/borg/borgcron/Groc.g:315:5: ( QUARTER | ( quarter_ordinals MONTH OF QUARTER ) )
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0==QUARTER) ) {
alt13=1;
}
else if ( (LA13_0==FIRST||LA13_0==SECOND||LA13_0==THIRD) ) {
alt13=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 13, 0, input);
throw nvae;
}
switch (alt13) {
case 1 :
// com/google/borg/borgcron/Groc.g:315:7: QUARTER
{
match(input,QUARTER,FOLLOW_QUARTER_in_quarterspec573);
Set quarterMonths = integerSetOf(
valueOf(JANUARY), valueOf(APRIL), valueOf(JULY), valueOf(OCTOBER));
months.addAll(quarterMonths);
}
break;
case 2 :
// com/google/borg/borgcron/Groc.g:319:7: ( quarter_ordinals MONTH OF QUARTER )
{
// com/google/borg/borgcron/Groc.g:319:7: ( quarter_ordinals MONTH OF QUARTER )
// com/google/borg/borgcron/Groc.g:319:9: quarter_ordinals MONTH OF QUARTER
{
pushFollow(FOLLOW_quarter_ordinals_in_quarterspec585);
quarter_ordinals();
state._fsp--;
match(input,MONTH,FOLLOW_MONTH_in_quarterspec587);
match(input,OF,FOLLOW_OF_in_quarterspec589);
match(input,QUARTER,FOLLOW_QUARTER_in_quarterspec591);
}
}
break;
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "quarterspec"
// $ANTLR start "quarter_ordinals"
// com/google/borg/borgcron/Groc.g:322:1: quarter_ordinals : ( month_of_quarter_ordinal ( COMMA month_of_quarter_ordinal )* ) ;
public final void quarter_ordinals() throws RecognitionException {
try {
// com/google/borg/borgcron/Groc.g:323:3: ( ( month_of_quarter_ordinal ( COMMA month_of_quarter_ordinal )* ) )
// com/google/borg/borgcron/Groc.g:323:5: ( month_of_quarter_ordinal ( COMMA month_of_quarter_ordinal )* )
{
// com/google/borg/borgcron/Groc.g:323:5: ( month_of_quarter_ordinal ( COMMA month_of_quarter_ordinal )* )
// com/google/borg/borgcron/Groc.g:323:7: month_of_quarter_ordinal ( COMMA month_of_quarter_ordinal )*
{
pushFollow(FOLLOW_month_of_quarter_ordinal_in_quarter_ordinals610);
month_of_quarter_ordinal();
state._fsp--;
// com/google/borg/borgcron/Groc.g:323:32: ( COMMA month_of_quarter_ordinal )*
loop14:
while (true) {
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0==COMMA) ) {
alt14=1;
}
switch (alt14) {
case 1 :
// com/google/borg/borgcron/Groc.g:323:33: COMMA month_of_quarter_ordinal
{
match(input,COMMA,FOLLOW_COMMA_in_quarter_ordinals613);
pushFollow(FOLLOW_month_of_quarter_ordinal_in_quarter_ordinals615);
month_of_quarter_ordinal();
state._fsp--;
}
break;
default :
break loop14;
}
}
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "quarter_ordinals"
// $ANTLR start "month_of_quarter_ordinal"
// com/google/borg/borgcron/Groc.g:326:1: month_of_quarter_ordinal : offset= ( FIRST | SECOND | THIRD ) ;
public final void month_of_quarter_ordinal() throws RecognitionException {
Token offset=null;
try {
// com/google/borg/borgcron/Groc.g:327:3: (offset= ( FIRST | SECOND | THIRD ) )
// com/google/borg/borgcron/Groc.g:327:5: offset= ( FIRST | SECOND | THIRD )
{
offset=input.LT(1);
if ( input.LA(1)==FIRST||input.LA(1)==SECOND||input.LA(1)==THIRD ) {
input.consume();
state.errorRecovery=false;
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
throw mse;
}
int jOffset = valueOf((offset!=null?offset.getType():0)) - 1;
Set offsetMonths = integerSetOf(
jOffset + valueOf(JANUARY), jOffset + valueOf(APRIL),
jOffset + valueOf(JULY), jOffset + valueOf(OCTOBER));
months.addAll(offsetMonths);
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "month_of_quarter_ordinal"
// $ANTLR start "time_range"
// com/google/borg/borgcron/Groc.g:335:1: time_range : ( FROM (start_time= TIME ) TO (end_time= TIME ) ) ;
public final void time_range() throws RecognitionException {
Token start_time=null;
Token end_time=null;
try {
// com/google/borg/borgcron/Groc.g:336:3: ( ( FROM (start_time= TIME ) TO (end_time= TIME ) ) )
// com/google/borg/borgcron/Groc.g:336:5: ( FROM (start_time= TIME ) TO (end_time= TIME ) )
{
// com/google/borg/borgcron/Groc.g:336:5: ( FROM (start_time= TIME ) TO (end_time= TIME ) )
// com/google/borg/borgcron/Groc.g:336:7: FROM (start_time= TIME ) TO (end_time= TIME )
{
match(input,FROM,FOLLOW_FROM_in_time_range663);
// com/google/borg/borgcron/Groc.g:336:12: (start_time= TIME )
// com/google/borg/borgcron/Groc.g:336:13: start_time= TIME
{
start_time=(Token)match(input,TIME,FOLLOW_TIME_in_time_range670);
startTime = (start_time!=null?start_time.getText():null);
}
match(input,TO,FOLLOW_TO_in_time_range681);
// com/google/borg/borgcron/Groc.g:337:10: (end_time= TIME )
// com/google/borg/borgcron/Groc.g:337:11: end_time= TIME
{
end_time=(Token)match(input,TIME,FOLLOW_TIME_in_time_range688);
endTime = (end_time!=null?end_time.getText():null);
}
}
}
}
catch (RecognitionException e) {
throw e;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "time_range"
// Delegated rules
protected DFA4 dfa4 = new DFA4(this);
static final String DFA4_eotS =
"\13\uffff";
static final String DFA4_eofS =
"\13\uffff";
static final String DFA4_minS =
"\1\11\1\7\1\6\1\uffff\1\36\1\6\1\15\1\uffff\1\21\2\6";
static final String DFA4_maxS =
"\1\45\2\54\1\uffff\2\47\1\45\1\uffff\2\54\1\47";
static final String DFA4_acceptS =
"\3\uffff\1\1\3\uffff\1\2\3\uffff";
static final String DFA4_specialS =
"\13\uffff}>";
static final String[] DFA4_transitionS = {
"\2\3\1\1\1\uffff\3\2\21\uffff\1\2\3\uffff\1\2",
"\1\4\11\uffff\1\5\10\uffff\1\5\5\uffff\1\5\2\uffff\1\5\2\uffff\1\5\2"+
"\uffff\1\5\2\uffff\1\5",
"\1\6\1\4\11\uffff\1\5\10\uffff\1\5\5\uffff\1\5\2\uffff\1\5\2\uffff\1"+
"\5\2\uffff\1\5\2\uffff\1\5",
"",
"\1\3\10\uffff\1\7",
"\1\10\27\uffff\1\3\10\uffff\1\7",
"\3\11\21\uffff\1\11\3\uffff\1\11",
"",
"\1\12\10\uffff\1\12\5\uffff\1\12\2\uffff\1\12\2\uffff\1\12\2\uffff\1"+
"\12\2\uffff\1\12",
"\1\6\1\4\11\uffff\1\5\10\uffff\1\5\5\uffff\1\5\2\uffff\1\5\2\uffff\1"+
"\5\2\uffff\1\5\2\uffff\1\5",
"\1\10\27\uffff\1\3\10\uffff\1\7"
};
static final short[] DFA4_eot = DFA.unpackEncodedString(DFA4_eotS);
static final short[] DFA4_eof = DFA.unpackEncodedString(DFA4_eofS);
static final char[] DFA4_min = DFA.unpackEncodedStringToUnsignedChars(DFA4_minS);
static final char[] DFA4_max = DFA.unpackEncodedStringToUnsignedChars(DFA4_maxS);
static final short[] DFA4_accept = DFA.unpackEncodedString(DFA4_acceptS);
static final short[] DFA4_special = DFA.unpackEncodedString(DFA4_specialS);
static final short[][] DFA4_transition;
static {
int numStates = DFA4_transitionS.length;
DFA4_transition = new short[numStates][];
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy