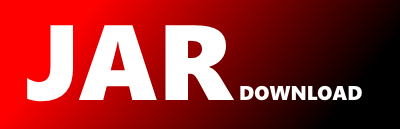
com.google.datastore.v1.QueryOrBuilder Maven / Gradle / Ivy
/*
* Copyright 2023 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/datastore/v1/query.proto
package com.google.datastore.v1;
public interface QueryOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.datastore.v1.Query)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The projection to return. Defaults to returning all properties.
*
*
* repeated .google.datastore.v1.Projection projection = 2;
*/
java.util.List getProjectionList();
/**
*
*
*
* The projection to return. Defaults to returning all properties.
*
*
* repeated .google.datastore.v1.Projection projection = 2;
*/
com.google.datastore.v1.Projection getProjection(int index);
/**
*
*
*
* The projection to return. Defaults to returning all properties.
*
*
* repeated .google.datastore.v1.Projection projection = 2;
*/
int getProjectionCount();
/**
*
*
*
* The projection to return. Defaults to returning all properties.
*
*
* repeated .google.datastore.v1.Projection projection = 2;
*/
java.util.List extends com.google.datastore.v1.ProjectionOrBuilder>
getProjectionOrBuilderList();
/**
*
*
*
* The projection to return. Defaults to returning all properties.
*
*
* repeated .google.datastore.v1.Projection projection = 2;
*/
com.google.datastore.v1.ProjectionOrBuilder getProjectionOrBuilder(int index);
/**
*
*
*
* The kinds to query (if empty, returns entities of all kinds).
* Currently at most 1 kind may be specified.
*
*
* repeated .google.datastore.v1.KindExpression kind = 3;
*/
java.util.List getKindList();
/**
*
*
*
* The kinds to query (if empty, returns entities of all kinds).
* Currently at most 1 kind may be specified.
*
*
* repeated .google.datastore.v1.KindExpression kind = 3;
*/
com.google.datastore.v1.KindExpression getKind(int index);
/**
*
*
*
* The kinds to query (if empty, returns entities of all kinds).
* Currently at most 1 kind may be specified.
*
*
* repeated .google.datastore.v1.KindExpression kind = 3;
*/
int getKindCount();
/**
*
*
*
* The kinds to query (if empty, returns entities of all kinds).
* Currently at most 1 kind may be specified.
*
*
* repeated .google.datastore.v1.KindExpression kind = 3;
*/
java.util.List extends com.google.datastore.v1.KindExpressionOrBuilder> getKindOrBuilderList();
/**
*
*
*
* The kinds to query (if empty, returns entities of all kinds).
* Currently at most 1 kind may be specified.
*
*
* repeated .google.datastore.v1.KindExpression kind = 3;
*/
com.google.datastore.v1.KindExpressionOrBuilder getKindOrBuilder(int index);
/**
*
*
*
* The filter to apply.
*
*
* .google.datastore.v1.Filter filter = 4;
*
* @return Whether the filter field is set.
*/
boolean hasFilter();
/**
*
*
*
* The filter to apply.
*
*
* .google.datastore.v1.Filter filter = 4;
*
* @return The filter.
*/
com.google.datastore.v1.Filter getFilter();
/**
*
*
*
* The filter to apply.
*
*
* .google.datastore.v1.Filter filter = 4;
*/
com.google.datastore.v1.FilterOrBuilder getFilterOrBuilder();
/**
*
*
*
* The order to apply to the query results (if empty, order is unspecified).
*
*
* repeated .google.datastore.v1.PropertyOrder order = 5;
*/
java.util.List getOrderList();
/**
*
*
*
* The order to apply to the query results (if empty, order is unspecified).
*
*
* repeated .google.datastore.v1.PropertyOrder order = 5;
*/
com.google.datastore.v1.PropertyOrder getOrder(int index);
/**
*
*
*
* The order to apply to the query results (if empty, order is unspecified).
*
*
* repeated .google.datastore.v1.PropertyOrder order = 5;
*/
int getOrderCount();
/**
*
*
*
* The order to apply to the query results (if empty, order is unspecified).
*
*
* repeated .google.datastore.v1.PropertyOrder order = 5;
*/
java.util.List extends com.google.datastore.v1.PropertyOrderOrBuilder> getOrderOrBuilderList();
/**
*
*
*
* The order to apply to the query results (if empty, order is unspecified).
*
*
* repeated .google.datastore.v1.PropertyOrder order = 5;
*/
com.google.datastore.v1.PropertyOrderOrBuilder getOrderOrBuilder(int index);
/**
*
*
*
* The properties to make distinct. The query results will contain the first
* result for each distinct combination of values for the given properties
* (if empty, all results are returned).
*
* Requires:
*
* * If `order` is specified, the set of distinct on properties must appear
* before the non-distinct on properties in `order`.
*
*
* repeated .google.datastore.v1.PropertyReference distinct_on = 6;
*/
java.util.List getDistinctOnList();
/**
*
*
*
* The properties to make distinct. The query results will contain the first
* result for each distinct combination of values for the given properties
* (if empty, all results are returned).
*
* Requires:
*
* * If `order` is specified, the set of distinct on properties must appear
* before the non-distinct on properties in `order`.
*
*
* repeated .google.datastore.v1.PropertyReference distinct_on = 6;
*/
com.google.datastore.v1.PropertyReference getDistinctOn(int index);
/**
*
*
*
* The properties to make distinct. The query results will contain the first
* result for each distinct combination of values for the given properties
* (if empty, all results are returned).
*
* Requires:
*
* * If `order` is specified, the set of distinct on properties must appear
* before the non-distinct on properties in `order`.
*
*
* repeated .google.datastore.v1.PropertyReference distinct_on = 6;
*/
int getDistinctOnCount();
/**
*
*
*
* The properties to make distinct. The query results will contain the first
* result for each distinct combination of values for the given properties
* (if empty, all results are returned).
*
* Requires:
*
* * If `order` is specified, the set of distinct on properties must appear
* before the non-distinct on properties in `order`.
*
*
* repeated .google.datastore.v1.PropertyReference distinct_on = 6;
*/
java.util.List extends com.google.datastore.v1.PropertyReferenceOrBuilder>
getDistinctOnOrBuilderList();
/**
*
*
*
* The properties to make distinct. The query results will contain the first
* result for each distinct combination of values for the given properties
* (if empty, all results are returned).
*
* Requires:
*
* * If `order` is specified, the set of distinct on properties must appear
* before the non-distinct on properties in `order`.
*
*
* repeated .google.datastore.v1.PropertyReference distinct_on = 6;
*/
com.google.datastore.v1.PropertyReferenceOrBuilder getDistinctOnOrBuilder(int index);
/**
*
*
*
* A starting point for the query results. Query cursors are
* returned in query result batches and
* [can only be used to continue the same
* query](https://cloud.google.com/datastore/docs/concepts/queries#cursors_limits_and_offsets).
*
*
* bytes start_cursor = 7;
*
* @return The startCursor.
*/
com.google.protobuf.ByteString getStartCursor();
/**
*
*
*
* An ending point for the query results. Query cursors are
* returned in query result batches and
* [can only be used to limit the same
* query](https://cloud.google.com/datastore/docs/concepts/queries#cursors_limits_and_offsets).
*
*
* bytes end_cursor = 8;
*
* @return The endCursor.
*/
com.google.protobuf.ByteString getEndCursor();
/**
*
*
*
* The number of results to skip. Applies before limit, but after all other
* constraints. Optional. Must be >= 0 if specified.
*
*
* int32 offset = 10;
*
* @return The offset.
*/
int getOffset();
/**
*
*
*
* The maximum number of results to return. Applies after all other
* constraints. Optional.
* Unspecified is interpreted as no limit.
* Must be >= 0 if specified.
*
*
* .google.protobuf.Int32Value limit = 12;
*
* @return Whether the limit field is set.
*/
boolean hasLimit();
/**
*
*
*
* The maximum number of results to return. Applies after all other
* constraints. Optional.
* Unspecified is interpreted as no limit.
* Must be >= 0 if specified.
*
*
* .google.protobuf.Int32Value limit = 12;
*
* @return The limit.
*/
com.google.protobuf.Int32Value getLimit();
/**
*
*
*
* The maximum number of results to return. Applies after all other
* constraints. Optional.
* Unspecified is interpreted as no limit.
* Must be >= 0 if specified.
*
*
* .google.protobuf.Int32Value limit = 12;
*/
com.google.protobuf.Int32ValueOrBuilder getLimitOrBuilder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy