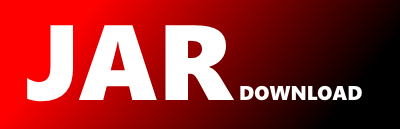
org.eclipse.jetty.client.HttpUpgrader Maven / Gradle / Ivy
//
// ========================================================================
// Copyright (c) 1995 Mort Bay Consulting Pty Ltd and others.
//
// This program and the accompanying materials are made available under the
// terms of the Eclipse Public License v. 2.0 which is available at
// https://www.eclipse.org/legal/epl-2.0, or the Apache License, Version 2.0
// which is available at https://www.apache.org/licenses/LICENSE-2.0.
//
// SPDX-License-Identifier: EPL-2.0 OR Apache-2.0
// ========================================================================
//
package org.eclipse.jetty.client;
import org.eclipse.jetty.http.HttpVersion;
import org.eclipse.jetty.io.EndPoint;
import org.eclipse.jetty.util.Callback;
/**
* HttpUpgrader prepares a HTTP request to upgrade from one protocol to another,
* and implements the upgrade mechanism.
* The upgrade mechanism can be the
* HTTP/1.1 upgrade mechanism
* or the
* HTTP/2 extended CONNECT mechanism.
* Given the differences among mechanism implementations, a request needs to be
* prepared before being sent to comply with the mechanism requirements (for example,
* add required headers, etc.).
*/
public interface HttpUpgrader
{
/**
* The request attribute key for the upgrade protocol,
* used by the HTTP/2 extended CONNECT mechanism.
*/
public static final String PROTOCOL_ATTRIBUTE = HttpUpgrader.class.getName() + ".protocol";
/**
* Prepares the request for the upgrade, for example by setting the HTTP method
* or by setting HTTP headers required for the upgrade.
*
* @param request the request to prepare
*/
public void prepare(Request request);
/**
* Upgrades the given {@code endPoint} to a different protocol.
* The success or failure of the upgrade should be communicated via the given {@code callback}.
* An exception thrown by this method is equivalent to failing the callback.
*
* @param response the response with the information about the upgrade
* @param endPoint the EndPoint to upgrade
* @param callback a callback to notify of the success or failure of the upgrade
*/
public void upgrade(Response response, EndPoint endPoint, Callback callback);
/**
* A factory for {@link HttpUpgrader}s.
* A {@link Request} subclass should implement this interface
* if it wants to create a specific HttpUpgrader.
*/
public interface Factory
{
public HttpUpgrader newHttpUpgrader(HttpVersion version);
}
}