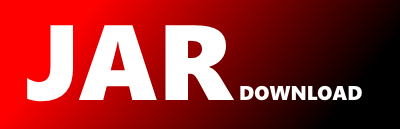
com.google.area120.tables.v1alpha.stub.GrpcTablesServiceStub Maven / Gradle / Ivy
Show all versions of google-area120-tables Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.area120.tables.v1alpha.stub;
import static com.google.area120.tables.v1alpha.TablesServiceClient.ListRowsPagedResponse;
import static com.google.area120.tables.v1alpha.TablesServiceClient.ListTablesPagedResponse;
import static com.google.area120.tables.v1alpha.TablesServiceClient.ListWorkspacesPagedResponse;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.area120.tables.v1alpha1.BatchCreateRowsRequest;
import com.google.area120.tables.v1alpha1.BatchCreateRowsResponse;
import com.google.area120.tables.v1alpha1.BatchDeleteRowsRequest;
import com.google.area120.tables.v1alpha1.BatchUpdateRowsRequest;
import com.google.area120.tables.v1alpha1.BatchUpdateRowsResponse;
import com.google.area120.tables.v1alpha1.CreateRowRequest;
import com.google.area120.tables.v1alpha1.DeleteRowRequest;
import com.google.area120.tables.v1alpha1.GetRowRequest;
import com.google.area120.tables.v1alpha1.GetTableRequest;
import com.google.area120.tables.v1alpha1.GetWorkspaceRequest;
import com.google.area120.tables.v1alpha1.ListRowsRequest;
import com.google.area120.tables.v1alpha1.ListRowsResponse;
import com.google.area120.tables.v1alpha1.ListTablesRequest;
import com.google.area120.tables.v1alpha1.ListTablesResponse;
import com.google.area120.tables.v1alpha1.ListWorkspacesRequest;
import com.google.area120.tables.v1alpha1.ListWorkspacesResponse;
import com.google.area120.tables.v1alpha1.Row;
import com.google.area120.tables.v1alpha1.Table;
import com.google.area120.tables.v1alpha1.UpdateRowRequest;
import com.google.area120.tables.v1alpha1.Workspace;
import com.google.longrunning.stub.GrpcOperationsStub;
import com.google.protobuf.Empty;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the TablesService service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class GrpcTablesServiceStub extends TablesServiceStub {
private static final MethodDescriptor getTableMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/GetTable")
.setRequestMarshaller(ProtoUtils.marshaller(GetTableRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Table.getDefaultInstance()))
.build();
private static final MethodDescriptor
listTablesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/ListTables")
.setRequestMarshaller(ProtoUtils.marshaller(ListTablesRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListTablesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getWorkspaceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/GetWorkspace")
.setRequestMarshaller(ProtoUtils.marshaller(GetWorkspaceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Workspace.getDefaultInstance()))
.build();
private static final MethodDescriptor
listWorkspacesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/ListWorkspaces")
.setRequestMarshaller(
ProtoUtils.marshaller(ListWorkspacesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListWorkspacesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getRowMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/GetRow")
.setRequestMarshaller(ProtoUtils.marshaller(GetRowRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Row.getDefaultInstance()))
.build();
private static final MethodDescriptor
listRowsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/ListRows")
.setRequestMarshaller(ProtoUtils.marshaller(ListRowsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListRowsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor createRowMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/CreateRow")
.setRequestMarshaller(ProtoUtils.marshaller(CreateRowRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Row.getDefaultInstance()))
.build();
private static final MethodDescriptor
batchCreateRowsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/BatchCreateRows")
.setRequestMarshaller(
ProtoUtils.marshaller(BatchCreateRowsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(BatchCreateRowsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor updateRowMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/UpdateRow")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateRowRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Row.getDefaultInstance()))
.build();
private static final MethodDescriptor
batchUpdateRowsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/BatchUpdateRows")
.setRequestMarshaller(
ProtoUtils.marshaller(BatchUpdateRowsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(BatchUpdateRowsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteRowMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/DeleteRow")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteRowRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
batchDeleteRowsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.area120.tables.v1alpha1.TablesService/BatchDeleteRows")
.setRequestMarshaller(
ProtoUtils.marshaller(BatchDeleteRowsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private final UnaryCallable getTableCallable;
private final UnaryCallable listTablesCallable;
private final UnaryCallable listTablesPagedCallable;
private final UnaryCallable getWorkspaceCallable;
private final UnaryCallable listWorkspacesCallable;
private final UnaryCallable
listWorkspacesPagedCallable;
private final UnaryCallable getRowCallable;
private final UnaryCallable listRowsCallable;
private final UnaryCallable listRowsPagedCallable;
private final UnaryCallable createRowCallable;
private final UnaryCallable
batchCreateRowsCallable;
private final UnaryCallable updateRowCallable;
private final UnaryCallable
batchUpdateRowsCallable;
private final UnaryCallable deleteRowCallable;
private final UnaryCallable batchDeleteRowsCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcTablesServiceStub create(TablesServiceStubSettings settings)
throws IOException {
return new GrpcTablesServiceStub(settings, ClientContext.create(settings));
}
public static final GrpcTablesServiceStub create(ClientContext clientContext) throws IOException {
return new GrpcTablesServiceStub(TablesServiceStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcTablesServiceStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcTablesServiceStub(
TablesServiceStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcTablesServiceStub, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcTablesServiceStub(TablesServiceStubSettings settings, ClientContext clientContext)
throws IOException {
this(settings, clientContext, new GrpcTablesServiceCallableFactory());
}
/**
* Constructs an instance of GrpcTablesServiceStub, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcTablesServiceStub(
TablesServiceStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings getTableTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getTableMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listTablesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listTablesMethodDescriptor)
.build();
GrpcCallSettings getWorkspaceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getWorkspaceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listWorkspacesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listWorkspacesMethodDescriptor)
.build();
GrpcCallSettings getRowTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getRowMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listRowsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listRowsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings createRowTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createRowMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
batchCreateRowsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(batchCreateRowsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateRowTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateRowMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("row.name", String.valueOf(request.getRow().getName()));
return builder.build();
})
.build();
GrpcCallSettings
batchUpdateRowsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(batchUpdateRowsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings deleteRowTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteRowMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings batchDeleteRowsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(batchDeleteRowsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
this.getTableCallable =
callableFactory.createUnaryCallable(
getTableTransportSettings, settings.getTableSettings(), clientContext);
this.listTablesCallable =
callableFactory.createUnaryCallable(
listTablesTransportSettings, settings.listTablesSettings(), clientContext);
this.listTablesPagedCallable =
callableFactory.createPagedCallable(
listTablesTransportSettings, settings.listTablesSettings(), clientContext);
this.getWorkspaceCallable =
callableFactory.createUnaryCallable(
getWorkspaceTransportSettings, settings.getWorkspaceSettings(), clientContext);
this.listWorkspacesCallable =
callableFactory.createUnaryCallable(
listWorkspacesTransportSettings, settings.listWorkspacesSettings(), clientContext);
this.listWorkspacesPagedCallable =
callableFactory.createPagedCallable(
listWorkspacesTransportSettings, settings.listWorkspacesSettings(), clientContext);
this.getRowCallable =
callableFactory.createUnaryCallable(
getRowTransportSettings, settings.getRowSettings(), clientContext);
this.listRowsCallable =
callableFactory.createUnaryCallable(
listRowsTransportSettings, settings.listRowsSettings(), clientContext);
this.listRowsPagedCallable =
callableFactory.createPagedCallable(
listRowsTransportSettings, settings.listRowsSettings(), clientContext);
this.createRowCallable =
callableFactory.createUnaryCallable(
createRowTransportSettings, settings.createRowSettings(), clientContext);
this.batchCreateRowsCallable =
callableFactory.createUnaryCallable(
batchCreateRowsTransportSettings, settings.batchCreateRowsSettings(), clientContext);
this.updateRowCallable =
callableFactory.createUnaryCallable(
updateRowTransportSettings, settings.updateRowSettings(), clientContext);
this.batchUpdateRowsCallable =
callableFactory.createUnaryCallable(
batchUpdateRowsTransportSettings, settings.batchUpdateRowsSettings(), clientContext);
this.deleteRowCallable =
callableFactory.createUnaryCallable(
deleteRowTransportSettings, settings.deleteRowSettings(), clientContext);
this.batchDeleteRowsCallable =
callableFactory.createUnaryCallable(
batchDeleteRowsTransportSettings, settings.batchDeleteRowsSettings(), clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable getTableCallable() {
return getTableCallable;
}
@Override
public UnaryCallable listTablesCallable() {
return listTablesCallable;
}
@Override
public UnaryCallable listTablesPagedCallable() {
return listTablesPagedCallable;
}
@Override
public UnaryCallable getWorkspaceCallable() {
return getWorkspaceCallable;
}
@Override
public UnaryCallable listWorkspacesCallable() {
return listWorkspacesCallable;
}
@Override
public UnaryCallable
listWorkspacesPagedCallable() {
return listWorkspacesPagedCallable;
}
@Override
public UnaryCallable getRowCallable() {
return getRowCallable;
}
@Override
public UnaryCallable listRowsCallable() {
return listRowsCallable;
}
@Override
public UnaryCallable listRowsPagedCallable() {
return listRowsPagedCallable;
}
@Override
public UnaryCallable createRowCallable() {
return createRowCallable;
}
@Override
public UnaryCallable batchCreateRowsCallable() {
return batchCreateRowsCallable;
}
@Override
public UnaryCallable updateRowCallable() {
return updateRowCallable;
}
@Override
public UnaryCallable batchUpdateRowsCallable() {
return batchUpdateRowsCallable;
}
@Override
public UnaryCallable deleteRowCallable() {
return deleteRowCallable;
}
@Override
public UnaryCallable batchDeleteRowsCallable() {
return batchDeleteRowsCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}