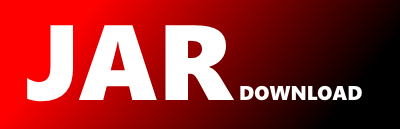
com.google.cloud.hadoop.fs.gcs.AutoValue_SyncableOutputStreamOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gcs-connector Show documentation
Show all versions of gcs-connector Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting Google Cloud Storage
package com.google.cloud.hadoop.fs.gcs;
import java.time.Duration;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_SyncableOutputStreamOptions extends SyncableOutputStreamOptions {
private final boolean appendEnabled;
private final Duration minSyncInterval;
private final boolean syncOnFlushEnabled;
private AutoValue_SyncableOutputStreamOptions(
boolean appendEnabled,
Duration minSyncInterval,
boolean syncOnFlushEnabled) {
this.appendEnabled = appendEnabled;
this.minSyncInterval = minSyncInterval;
this.syncOnFlushEnabled = syncOnFlushEnabled;
}
@Override
public boolean isAppendEnabled() {
return appendEnabled;
}
@Override
public Duration getMinSyncInterval() {
return minSyncInterval;
}
@Override
public boolean isSyncOnFlushEnabled() {
return syncOnFlushEnabled;
}
@Override
public String toString() {
return "SyncableOutputStreamOptions{"
+ "appendEnabled=" + appendEnabled + ", "
+ "minSyncInterval=" + minSyncInterval + ", "
+ "syncOnFlushEnabled=" + syncOnFlushEnabled
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof SyncableOutputStreamOptions) {
SyncableOutputStreamOptions that = (SyncableOutputStreamOptions) o;
return this.appendEnabled == that.isAppendEnabled()
&& this.minSyncInterval.equals(that.getMinSyncInterval())
&& this.syncOnFlushEnabled == that.isSyncOnFlushEnabled();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= appendEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= minSyncInterval.hashCode();
h$ *= 1000003;
h$ ^= syncOnFlushEnabled ? 1231 : 1237;
return h$;
}
@Override
public SyncableOutputStreamOptions.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends SyncableOutputStreamOptions.Builder {
private boolean appendEnabled;
private Duration minSyncInterval;
private boolean syncOnFlushEnabled;
private byte set$0;
Builder() {
}
private Builder(SyncableOutputStreamOptions source) {
this.appendEnabled = source.isAppendEnabled();
this.minSyncInterval = source.getMinSyncInterval();
this.syncOnFlushEnabled = source.isSyncOnFlushEnabled();
set$0 = (byte) 3;
}
@Override
public SyncableOutputStreamOptions.Builder setAppendEnabled(boolean appendEnabled) {
this.appendEnabled = appendEnabled;
set$0 |= (byte) 1;
return this;
}
@Override
public SyncableOutputStreamOptions.Builder setMinSyncInterval(Duration minSyncInterval) {
if (minSyncInterval == null) {
throw new NullPointerException("Null minSyncInterval");
}
this.minSyncInterval = minSyncInterval;
return this;
}
@Override
public SyncableOutputStreamOptions.Builder setSyncOnFlushEnabled(boolean syncOnFlushEnabled) {
this.syncOnFlushEnabled = syncOnFlushEnabled;
set$0 |= (byte) 2;
return this;
}
@Override
public SyncableOutputStreamOptions build() {
if (set$0 != 3
|| this.minSyncInterval == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" appendEnabled");
}
if (this.minSyncInterval == null) {
missing.append(" minSyncInterval");
}
if ((set$0 & 2) == 0) {
missing.append(" syncOnFlushEnabled");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_SyncableOutputStreamOptions(
this.appendEnabled,
this.minSyncInterval,
this.syncOnFlushEnabled);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy