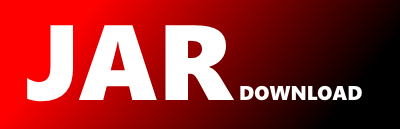
com.google.cloud.hadoop.gcsio.CreateFileOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gcsio Show documentation
Show all versions of gcsio Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting Google Cloud Storage
/**
* Copyright 2014 Google Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.hadoop.gcsio;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableMap;
import java.util.Map;
/**
* Options that can be specified when creating a file in the {@code GoogleCloudFileSystem}.
*/
public class CreateFileOptions {
public static final Map EMPTY_ATTRIBUTES = ImmutableMap.of();
public static final String DEFAULT_CONTENT_TYPE = "application/octet-stream";
public static final CreateFileOptions DEFAULT =
new CreateFileOptions(true, DEFAULT_CONTENT_TYPE, EMPTY_ATTRIBUTES);
private final boolean overwriteExisting;
private final String contentType;
private final Map attributes;
/**
* Create a file with empty attributes and optionally overwriting any existing file.
*
* @param overwriteExisting True to overwrite an existing file with the same name
*/
public CreateFileOptions(boolean overwriteExisting) {
this(overwriteExisting, DEFAULT_CONTENT_TYPE, EMPTY_ATTRIBUTES);
}
/**
* Create a file with empty attributes, and optionally overwriting any existing file with one
* having the given content-type.
*
* @param overwriteExisting True to overwrite an existing file with the same name
* @param contentType content-type for the created file
*/
public CreateFileOptions(boolean overwriteExisting, String contentType) {
this(overwriteExisting, contentType, EMPTY_ATTRIBUTES);
}
/**
* Create a file with specified attributes and optionally overwriting an existing file.
*
* @param overwriteExisting True to overwrite an existing file with the same name
* @param attributes File attributes to apply to the file at creation
*/
public CreateFileOptions(boolean overwriteExisting, Map attributes) {
this(overwriteExisting, DEFAULT_CONTENT_TYPE, attributes);
}
/**
* Create a file with specified attributes, and optionally overwriting an existing file with one
* having the given content-type.
*
* @param overwriteExisting True to overwrite an existing file with the same name
* @param contentType content-type for the created file
* @param attributes File attributes to apply to the file at creation
*/
public CreateFileOptions(boolean overwriteExisting, String contentType,
Map attributes) {
Preconditions.checkArgument(!attributes.containsKey("Content-Type"),
"The Content-Type attribute must be provided explicitly via the 'contentType' parameter");
this.overwriteExisting = overwriteExisting;
this.contentType = contentType;
this.attributes = attributes;
}
/**
* Get the value of overwriteExisting.
*/
public boolean overwriteExisting() {
return overwriteExisting;
}
/**
* Content-type to set when creating a file.
*/
public String getContentType() {
return contentType;
}
/**
* Extended attributes to set when creating a file.
*/
public Map getAttributes() {
return attributes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy