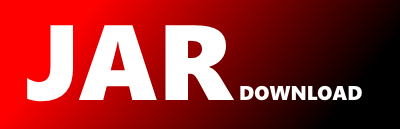
com.google.cloud.hadoop.gcsio.AutoValue_GoogleCloudStorageReadOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gcsio Show documentation
Show all versions of gcsio Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting Google Cloud Storage
package com.google.cloud.hadoop.gcsio;
import com.google.common.collect.ImmutableSet;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_GoogleCloudStorageReadOptions extends GoogleCloudStorageReadOptions {
private final int backoffInitialIntervalMillis;
private final double backoffRandomizationFactor;
private final double backoffMultiplier;
private final int backoffMaxIntervalMillis;
private final int backoffMaxElapsedTimeMillis;
private final boolean fastFailOnNotFound;
private final boolean supportGzipEncoding;
private final long inplaceSeekLimit;
private final GoogleCloudStorageReadOptions.Fadvise fadvise;
private final int minRangeRequestSize;
private final boolean grpcChecksumsEnabled;
private final long grpcReadTimeoutMillis;
private final long grpcReadMetadataTimeoutMillis;
private final boolean grpcReadZeroCopyEnabled;
private final long grpcReadMessageTimeoutMillis;
private final boolean traceLogEnabled;
private final ImmutableSet traceLogExcludeProperties;
private final long traceLogTimeThreshold;
private AutoValue_GoogleCloudStorageReadOptions(
int backoffInitialIntervalMillis,
double backoffRandomizationFactor,
double backoffMultiplier,
int backoffMaxIntervalMillis,
int backoffMaxElapsedTimeMillis,
boolean fastFailOnNotFound,
boolean supportGzipEncoding,
long inplaceSeekLimit,
GoogleCloudStorageReadOptions.Fadvise fadvise,
int minRangeRequestSize,
boolean grpcChecksumsEnabled,
long grpcReadTimeoutMillis,
long grpcReadMetadataTimeoutMillis,
boolean grpcReadZeroCopyEnabled,
long grpcReadMessageTimeoutMillis,
boolean traceLogEnabled,
ImmutableSet traceLogExcludeProperties,
long traceLogTimeThreshold) {
this.backoffInitialIntervalMillis = backoffInitialIntervalMillis;
this.backoffRandomizationFactor = backoffRandomizationFactor;
this.backoffMultiplier = backoffMultiplier;
this.backoffMaxIntervalMillis = backoffMaxIntervalMillis;
this.backoffMaxElapsedTimeMillis = backoffMaxElapsedTimeMillis;
this.fastFailOnNotFound = fastFailOnNotFound;
this.supportGzipEncoding = supportGzipEncoding;
this.inplaceSeekLimit = inplaceSeekLimit;
this.fadvise = fadvise;
this.minRangeRequestSize = minRangeRequestSize;
this.grpcChecksumsEnabled = grpcChecksumsEnabled;
this.grpcReadTimeoutMillis = grpcReadTimeoutMillis;
this.grpcReadMetadataTimeoutMillis = grpcReadMetadataTimeoutMillis;
this.grpcReadZeroCopyEnabled = grpcReadZeroCopyEnabled;
this.grpcReadMessageTimeoutMillis = grpcReadMessageTimeoutMillis;
this.traceLogEnabled = traceLogEnabled;
this.traceLogExcludeProperties = traceLogExcludeProperties;
this.traceLogTimeThreshold = traceLogTimeThreshold;
}
@Override
public int getBackoffInitialIntervalMillis() {
return backoffInitialIntervalMillis;
}
@Override
public double getBackoffRandomizationFactor() {
return backoffRandomizationFactor;
}
@Override
public double getBackoffMultiplier() {
return backoffMultiplier;
}
@Override
public int getBackoffMaxIntervalMillis() {
return backoffMaxIntervalMillis;
}
@Override
public int getBackoffMaxElapsedTimeMillis() {
return backoffMaxElapsedTimeMillis;
}
@Override
public boolean getFastFailOnNotFound() {
return fastFailOnNotFound;
}
@Override
public boolean getSupportGzipEncoding() {
return supportGzipEncoding;
}
@Override
public long getInplaceSeekLimit() {
return inplaceSeekLimit;
}
@Override
public GoogleCloudStorageReadOptions.Fadvise getFadvise() {
return fadvise;
}
@Override
public int getMinRangeRequestSize() {
return minRangeRequestSize;
}
@Override
public boolean isGrpcChecksumsEnabled() {
return grpcChecksumsEnabled;
}
@Override
public long getGrpcReadTimeoutMillis() {
return grpcReadTimeoutMillis;
}
@Override
public long getGrpcReadMetadataTimeoutMillis() {
return grpcReadMetadataTimeoutMillis;
}
@Override
public boolean isGrpcReadZeroCopyEnabled() {
return grpcReadZeroCopyEnabled;
}
@Override
public long getGrpcReadMessageTimeoutMillis() {
return grpcReadMessageTimeoutMillis;
}
@Override
public boolean isTraceLogEnabled() {
return traceLogEnabled;
}
@Override
public ImmutableSet getTraceLogExcludeProperties() {
return traceLogExcludeProperties;
}
@Override
public long getTraceLogTimeThreshold() {
return traceLogTimeThreshold;
}
@Override
public String toString() {
return "GoogleCloudStorageReadOptions{"
+ "backoffInitialIntervalMillis=" + backoffInitialIntervalMillis + ", "
+ "backoffRandomizationFactor=" + backoffRandomizationFactor + ", "
+ "backoffMultiplier=" + backoffMultiplier + ", "
+ "backoffMaxIntervalMillis=" + backoffMaxIntervalMillis + ", "
+ "backoffMaxElapsedTimeMillis=" + backoffMaxElapsedTimeMillis + ", "
+ "fastFailOnNotFound=" + fastFailOnNotFound + ", "
+ "supportGzipEncoding=" + supportGzipEncoding + ", "
+ "inplaceSeekLimit=" + inplaceSeekLimit + ", "
+ "fadvise=" + fadvise + ", "
+ "minRangeRequestSize=" + minRangeRequestSize + ", "
+ "grpcChecksumsEnabled=" + grpcChecksumsEnabled + ", "
+ "grpcReadTimeoutMillis=" + grpcReadTimeoutMillis + ", "
+ "grpcReadMetadataTimeoutMillis=" + grpcReadMetadataTimeoutMillis + ", "
+ "grpcReadZeroCopyEnabled=" + grpcReadZeroCopyEnabled + ", "
+ "grpcReadMessageTimeoutMillis=" + grpcReadMessageTimeoutMillis + ", "
+ "traceLogEnabled=" + traceLogEnabled + ", "
+ "traceLogExcludeProperties=" + traceLogExcludeProperties + ", "
+ "traceLogTimeThreshold=" + traceLogTimeThreshold
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof GoogleCloudStorageReadOptions) {
GoogleCloudStorageReadOptions that = (GoogleCloudStorageReadOptions) o;
return this.backoffInitialIntervalMillis == that.getBackoffInitialIntervalMillis()
&& Double.doubleToLongBits(this.backoffRandomizationFactor) == Double.doubleToLongBits(that.getBackoffRandomizationFactor())
&& Double.doubleToLongBits(this.backoffMultiplier) == Double.doubleToLongBits(that.getBackoffMultiplier())
&& this.backoffMaxIntervalMillis == that.getBackoffMaxIntervalMillis()
&& this.backoffMaxElapsedTimeMillis == that.getBackoffMaxElapsedTimeMillis()
&& this.fastFailOnNotFound == that.getFastFailOnNotFound()
&& this.supportGzipEncoding == that.getSupportGzipEncoding()
&& this.inplaceSeekLimit == that.getInplaceSeekLimit()
&& this.fadvise.equals(that.getFadvise())
&& this.minRangeRequestSize == that.getMinRangeRequestSize()
&& this.grpcChecksumsEnabled == that.isGrpcChecksumsEnabled()
&& this.grpcReadTimeoutMillis == that.getGrpcReadTimeoutMillis()
&& this.grpcReadMetadataTimeoutMillis == that.getGrpcReadMetadataTimeoutMillis()
&& this.grpcReadZeroCopyEnabled == that.isGrpcReadZeroCopyEnabled()
&& this.grpcReadMessageTimeoutMillis == that.getGrpcReadMessageTimeoutMillis()
&& this.traceLogEnabled == that.isTraceLogEnabled()
&& this.traceLogExcludeProperties.equals(that.getTraceLogExcludeProperties())
&& this.traceLogTimeThreshold == that.getTraceLogTimeThreshold();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= backoffInitialIntervalMillis;
h$ *= 1000003;
h$ ^= (int) ((Double.doubleToLongBits(backoffRandomizationFactor) >>> 32) ^ Double.doubleToLongBits(backoffRandomizationFactor));
h$ *= 1000003;
h$ ^= (int) ((Double.doubleToLongBits(backoffMultiplier) >>> 32) ^ Double.doubleToLongBits(backoffMultiplier));
h$ *= 1000003;
h$ ^= backoffMaxIntervalMillis;
h$ *= 1000003;
h$ ^= backoffMaxElapsedTimeMillis;
h$ *= 1000003;
h$ ^= fastFailOnNotFound ? 1231 : 1237;
h$ *= 1000003;
h$ ^= supportGzipEncoding ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (int) ((inplaceSeekLimit >>> 32) ^ inplaceSeekLimit);
h$ *= 1000003;
h$ ^= fadvise.hashCode();
h$ *= 1000003;
h$ ^= minRangeRequestSize;
h$ *= 1000003;
h$ ^= grpcChecksumsEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (int) ((grpcReadTimeoutMillis >>> 32) ^ grpcReadTimeoutMillis);
h$ *= 1000003;
h$ ^= (int) ((grpcReadMetadataTimeoutMillis >>> 32) ^ grpcReadMetadataTimeoutMillis);
h$ *= 1000003;
h$ ^= grpcReadZeroCopyEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (int) ((grpcReadMessageTimeoutMillis >>> 32) ^ grpcReadMessageTimeoutMillis);
h$ *= 1000003;
h$ ^= traceLogEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= traceLogExcludeProperties.hashCode();
h$ *= 1000003;
h$ ^= (int) ((traceLogTimeThreshold >>> 32) ^ traceLogTimeThreshold);
return h$;
}
@Override
public GoogleCloudStorageReadOptions.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends GoogleCloudStorageReadOptions.Builder {
private int backoffInitialIntervalMillis;
private double backoffRandomizationFactor;
private double backoffMultiplier;
private int backoffMaxIntervalMillis;
private int backoffMaxElapsedTimeMillis;
private boolean fastFailOnNotFound;
private boolean supportGzipEncoding;
private long inplaceSeekLimit;
private GoogleCloudStorageReadOptions.Fadvise fadvise;
private int minRangeRequestSize;
private boolean grpcChecksumsEnabled;
private long grpcReadTimeoutMillis;
private long grpcReadMetadataTimeoutMillis;
private boolean grpcReadZeroCopyEnabled;
private long grpcReadMessageTimeoutMillis;
private boolean traceLogEnabled;
private ImmutableSet traceLogExcludeProperties;
private long traceLogTimeThreshold;
private short set$0;
Builder() {
}
private Builder(GoogleCloudStorageReadOptions source) {
this.backoffInitialIntervalMillis = source.getBackoffInitialIntervalMillis();
this.backoffRandomizationFactor = source.getBackoffRandomizationFactor();
this.backoffMultiplier = source.getBackoffMultiplier();
this.backoffMaxIntervalMillis = source.getBackoffMaxIntervalMillis();
this.backoffMaxElapsedTimeMillis = source.getBackoffMaxElapsedTimeMillis();
this.fastFailOnNotFound = source.getFastFailOnNotFound();
this.supportGzipEncoding = source.getSupportGzipEncoding();
this.inplaceSeekLimit = source.getInplaceSeekLimit();
this.fadvise = source.getFadvise();
this.minRangeRequestSize = source.getMinRangeRequestSize();
this.grpcChecksumsEnabled = source.isGrpcChecksumsEnabled();
this.grpcReadTimeoutMillis = source.getGrpcReadTimeoutMillis();
this.grpcReadMetadataTimeoutMillis = source.getGrpcReadMetadataTimeoutMillis();
this.grpcReadZeroCopyEnabled = source.isGrpcReadZeroCopyEnabled();
this.grpcReadMessageTimeoutMillis = source.getGrpcReadMessageTimeoutMillis();
this.traceLogEnabled = source.isTraceLogEnabled();
this.traceLogExcludeProperties = source.getTraceLogExcludeProperties();
this.traceLogTimeThreshold = source.getTraceLogTimeThreshold();
set$0 = (short) 0xffff;
}
@Override
public GoogleCloudStorageReadOptions.Builder setBackoffInitialIntervalMillis(int backoffInitialIntervalMillis) {
this.backoffInitialIntervalMillis = backoffInitialIntervalMillis;
set$0 |= 1;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setBackoffRandomizationFactor(double backoffRandomizationFactor) {
this.backoffRandomizationFactor = backoffRandomizationFactor;
set$0 |= 2;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setBackoffMultiplier(double backoffMultiplier) {
this.backoffMultiplier = backoffMultiplier;
set$0 |= 4;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setBackoffMaxIntervalMillis(int backoffMaxIntervalMillis) {
this.backoffMaxIntervalMillis = backoffMaxIntervalMillis;
set$0 |= 8;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setBackoffMaxElapsedTimeMillis(int backoffMaxElapsedTimeMillis) {
this.backoffMaxElapsedTimeMillis = backoffMaxElapsedTimeMillis;
set$0 |= 0x10;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setFastFailOnNotFound(boolean fastFailOnNotFound) {
this.fastFailOnNotFound = fastFailOnNotFound;
set$0 |= 0x20;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setSupportGzipEncoding(boolean supportGzipEncoding) {
this.supportGzipEncoding = supportGzipEncoding;
set$0 |= 0x40;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setInplaceSeekLimit(long inplaceSeekLimit) {
this.inplaceSeekLimit = inplaceSeekLimit;
set$0 |= 0x80;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setFadvise(GoogleCloudStorageReadOptions.Fadvise fadvise) {
if (fadvise == null) {
throw new NullPointerException("Null fadvise");
}
this.fadvise = fadvise;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setMinRangeRequestSize(int minRangeRequestSize) {
this.minRangeRequestSize = minRangeRequestSize;
set$0 |= 0x100;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setGrpcChecksumsEnabled(boolean grpcChecksumsEnabled) {
this.grpcChecksumsEnabled = grpcChecksumsEnabled;
set$0 |= 0x200;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setGrpcReadTimeoutMillis(long grpcReadTimeoutMillis) {
this.grpcReadTimeoutMillis = grpcReadTimeoutMillis;
set$0 |= 0x400;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setGrpcReadMetadataTimeoutMillis(long grpcReadMetadataTimeoutMillis) {
this.grpcReadMetadataTimeoutMillis = grpcReadMetadataTimeoutMillis;
set$0 |= 0x800;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setGrpcReadZeroCopyEnabled(boolean grpcReadZeroCopyEnabled) {
this.grpcReadZeroCopyEnabled = grpcReadZeroCopyEnabled;
set$0 |= 0x1000;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setGrpcReadMessageTimeoutMillis(long grpcReadMessageTimeoutMillis) {
this.grpcReadMessageTimeoutMillis = grpcReadMessageTimeoutMillis;
set$0 |= 0x2000;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setTraceLogEnabled(boolean traceLogEnabled) {
this.traceLogEnabled = traceLogEnabled;
set$0 |= 0x4000;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setTraceLogExcludeProperties(ImmutableSet traceLogExcludeProperties) {
if (traceLogExcludeProperties == null) {
throw new NullPointerException("Null traceLogExcludeProperties");
}
this.traceLogExcludeProperties = traceLogExcludeProperties;
return this;
}
@Override
public GoogleCloudStorageReadOptions.Builder setTraceLogTimeThreshold(long traceLogTimeThreshold) {
this.traceLogTimeThreshold = traceLogTimeThreshold;
set$0 |= 0x8000;
return this;
}
@Override
GoogleCloudStorageReadOptions autoBuild() {
if (set$0 != -1
|| this.fadvise == null
|| this.traceLogExcludeProperties == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" backoffInitialIntervalMillis");
}
if ((set$0 & 2) == 0) {
missing.append(" backoffRandomizationFactor");
}
if ((set$0 & 4) == 0) {
missing.append(" backoffMultiplier");
}
if ((set$0 & 8) == 0) {
missing.append(" backoffMaxIntervalMillis");
}
if ((set$0 & 0x10) == 0) {
missing.append(" backoffMaxElapsedTimeMillis");
}
if ((set$0 & 0x20) == 0) {
missing.append(" fastFailOnNotFound");
}
if ((set$0 & 0x40) == 0) {
missing.append(" supportGzipEncoding");
}
if ((set$0 & 0x80) == 0) {
missing.append(" inplaceSeekLimit");
}
if (this.fadvise == null) {
missing.append(" fadvise");
}
if ((set$0 & 0x100) == 0) {
missing.append(" minRangeRequestSize");
}
if ((set$0 & 0x200) == 0) {
missing.append(" grpcChecksumsEnabled");
}
if ((set$0 & 0x400) == 0) {
missing.append(" grpcReadTimeoutMillis");
}
if ((set$0 & 0x800) == 0) {
missing.append(" grpcReadMetadataTimeoutMillis");
}
if ((set$0 & 0x1000) == 0) {
missing.append(" grpcReadZeroCopyEnabled");
}
if ((set$0 & 0x2000) == 0) {
missing.append(" grpcReadMessageTimeoutMillis");
}
if ((set$0 & 0x4000) == 0) {
missing.append(" traceLogEnabled");
}
if (this.traceLogExcludeProperties == null) {
missing.append(" traceLogExcludeProperties");
}
if ((set$0 & 0x8000) == 0) {
missing.append(" traceLogTimeThreshold");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_GoogleCloudStorageReadOptions(
this.backoffInitialIntervalMillis,
this.backoffRandomizationFactor,
this.backoffMultiplier,
this.backoffMaxIntervalMillis,
this.backoffMaxElapsedTimeMillis,
this.fastFailOnNotFound,
this.supportGzipEncoding,
this.inplaceSeekLimit,
this.fadvise,
this.minRangeRequestSize,
this.grpcChecksumsEnabled,
this.grpcReadTimeoutMillis,
this.grpcReadMetadataTimeoutMillis,
this.grpcReadZeroCopyEnabled,
this.grpcReadMessageTimeoutMillis,
this.traceLogEnabled,
this.traceLogExcludeProperties,
this.traceLogTimeThreshold);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy