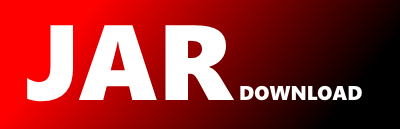
com.google.cloud.hadoop.gcsio.authorization.AuthorizationHandler Maven / Gradle / Ivy
Show all versions of gcsio Show documentation
package com.google.cloud.hadoop.gcsio.authorization;
import java.net.URI;
import java.nio.file.AccessDeniedException;
import java.util.List;
import java.util.Map;
/**
* All custom authorization handler implementation should implement this interface.
*
* The class is created by reflection. All customized AuthorizationHandler should implement this
* interface and read arguments through hadoop's Configurable interface.
*/
public interface AuthorizationHandler {
/**
* This method is called after instantiation and before calling handle. All properties with the
* prefix "fs.gs.authorization.handler.properties." will be set to the properties map.
*
* @param properties Immutable map of properties.
*/
void setProperties(Map properties);
/**
* Handles list object
* request.
*
* @param resource A GCS object URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleListObjects(URI resource) throws AccessDeniedException;
/**
* Handles insert object
* request.
*
* @param resource A GCS object URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleInsertObject(URI resource) throws AccessDeniedException;
/**
* Handles compose
* object request.
*
* @param destinationResource URI of destination GCS object.
* @param sourceResources A list of source GCS objects' URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleComposeObject(URI destinationResource, List sourceResources)
throws AccessDeniedException;
/**
* Handles get object
* request.
*
* @param resource A GCS object URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleGetObject(URI resource) throws AccessDeniedException;
/**
* Handles delete object
* request.
*
* @param resource A GCS object URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleDeleteObject(URI resource) throws AccessDeniedException;
/**
* Handles rewrite
* object request.
*
* @param sourceResource The source GCS object URI.
* @param destinationResource The destination GCS object URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleRewriteObject(URI sourceResource, URI destinationResource)
throws AccessDeniedException;
/**
* Handles copy object
* request.
*
* @param sourceResource The source GCS object URI.
* @param destinationResource The destination GCS object URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleCopyObject(URI sourceResource, URI destinationResource) throws AccessDeniedException;
/**
* Handles patch object
* request.
*
* @param resource A GCS object URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handlePatchObject(URI resource) throws AccessDeniedException;
/**
* Handles list bucket
* request.
*
* @param project A GCP project ID in which buckets are listed.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleListBuckets(String project) throws AccessDeniedException;
/**
* Handles insert bucket
* request.
*
* @param project A GCP project ID where bucket created.
* @param resource A GCS bucket URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleInsertBucket(String project, URI resource) throws AccessDeniedException;
/**
* Handles get bucket
* request.
*
* @param resource A GCS bucket URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleGetBucket(URI resource) throws AccessDeniedException;
/**
* Handles delete bucket
* request.
*
* @param resource A GCS bucket URI.
* @throws AccessDeniedException Thrown when access denied.
*/
void handleDeleteBucket(URI resource) throws AccessDeniedException;
}