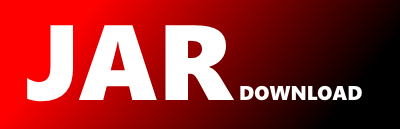
com.google.cloud.hadoop.gcsio.AutoValue_CreateFileOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gcsio Show documentation
Show all versions of gcsio Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting Google Cloud Storage
package com.google.cloud.hadoop.gcsio;
import com.google.common.collect.ImmutableMap;
import java.util.Map;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_CreateFileOptions extends CreateFileOptions {
private final ImmutableMap attributes;
@Nullable
private final String contentType;
private final boolean ensureNoDirectoryConflict;
private final boolean overwriteExisting;
private final long overwriteGenerationId;
private AutoValue_CreateFileOptions(
ImmutableMap attributes,
@Nullable String contentType,
boolean ensureNoDirectoryConflict,
boolean overwriteExisting,
long overwriteGenerationId) {
this.attributes = attributes;
this.contentType = contentType;
this.ensureNoDirectoryConflict = ensureNoDirectoryConflict;
this.overwriteExisting = overwriteExisting;
this.overwriteGenerationId = overwriteGenerationId;
}
@Override
public ImmutableMap getAttributes() {
return attributes;
}
@Nullable
@Override
public String getContentType() {
return contentType;
}
@Override
public boolean isEnsureNoDirectoryConflict() {
return ensureNoDirectoryConflict;
}
@Override
public boolean isOverwriteExisting() {
return overwriteExisting;
}
@Override
public long getOverwriteGenerationId() {
return overwriteGenerationId;
}
@Override
public String toString() {
return "CreateFileOptions{"
+ "attributes=" + attributes + ", "
+ "contentType=" + contentType + ", "
+ "ensureNoDirectoryConflict=" + ensureNoDirectoryConflict + ", "
+ "overwriteExisting=" + overwriteExisting + ", "
+ "overwriteGenerationId=" + overwriteGenerationId
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CreateFileOptions) {
CreateFileOptions that = (CreateFileOptions) o;
return this.attributes.equals(that.getAttributes())
&& (this.contentType == null ? that.getContentType() == null : this.contentType.equals(that.getContentType()))
&& this.ensureNoDirectoryConflict == that.isEnsureNoDirectoryConflict()
&& this.overwriteExisting == that.isOverwriteExisting()
&& this.overwriteGenerationId == that.getOverwriteGenerationId();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= attributes.hashCode();
h$ *= 1000003;
h$ ^= (contentType == null) ? 0 : contentType.hashCode();
h$ *= 1000003;
h$ ^= ensureNoDirectoryConflict ? 1231 : 1237;
h$ *= 1000003;
h$ ^= overwriteExisting ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (int) ((overwriteGenerationId >>> 32) ^ overwriteGenerationId);
return h$;
}
@Override
public CreateFileOptions.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends CreateFileOptions.Builder {
private ImmutableMap attributes;
private String contentType;
private boolean ensureNoDirectoryConflict;
private boolean overwriteExisting;
private long overwriteGenerationId;
private byte set$0;
Builder() {
}
private Builder(CreateFileOptions source) {
this.attributes = source.getAttributes();
this.contentType = source.getContentType();
this.ensureNoDirectoryConflict = source.isEnsureNoDirectoryConflict();
this.overwriteExisting = source.isOverwriteExisting();
this.overwriteGenerationId = source.getOverwriteGenerationId();
set$0 = (byte) 7;
}
@Override
public CreateFileOptions.Builder setAttributes(Map attributes) {
this.attributes = ImmutableMap.copyOf(attributes);
return this;
}
@Override
public CreateFileOptions.Builder setContentType(String contentType) {
this.contentType = contentType;
return this;
}
@Override
public CreateFileOptions.Builder setEnsureNoDirectoryConflict(boolean ensureNoDirectoryConflict) {
this.ensureNoDirectoryConflict = ensureNoDirectoryConflict;
set$0 |= (byte) 1;
return this;
}
@Override
public CreateFileOptions.Builder setOverwriteExisting(boolean overwriteExisting) {
this.overwriteExisting = overwriteExisting;
set$0 |= (byte) 2;
return this;
}
@Override
public CreateFileOptions.Builder setOverwriteGenerationId(long overwriteGenerationId) {
this.overwriteGenerationId = overwriteGenerationId;
set$0 |= (byte) 4;
return this;
}
@Override
CreateFileOptions autoBuild() {
if (set$0 != 7
|| this.attributes == null) {
StringBuilder missing = new StringBuilder();
if (this.attributes == null) {
missing.append(" attributes");
}
if ((set$0 & 1) == 0) {
missing.append(" ensureNoDirectoryConflict");
}
if ((set$0 & 2) == 0) {
missing.append(" overwriteExisting");
}
if ((set$0 & 4) == 0) {
missing.append(" overwriteGenerationId");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_CreateFileOptions(
this.attributes,
this.contentType,
this.ensureNoDirectoryConflict,
this.overwriteExisting,
this.overwriteGenerationId);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy