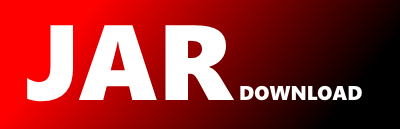
com.google.cloud.hadoop.gcsio.AutoValue_CreateBucketOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gcsio Show documentation
Show all versions of gcsio Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting Google Cloud Storage
package com.google.cloud.hadoop.gcsio;
import java.time.Duration;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_CreateBucketOptions extends CreateBucketOptions {
private final String location;
private final String storageClass;
private final Duration ttl;
private AutoValue_CreateBucketOptions(
@Nullable String location,
@Nullable String storageClass,
@Nullable Duration ttl) {
this.location = location;
this.storageClass = storageClass;
this.ttl = ttl;
}
@Nullable
@Override
public String getLocation() {
return location;
}
@Nullable
@Override
public String getStorageClass() {
return storageClass;
}
@Nullable
@Override
public Duration getTtl() {
return ttl;
}
@Override
public String toString() {
return "CreateBucketOptions{"
+ "location=" + location + ", "
+ "storageClass=" + storageClass + ", "
+ "ttl=" + ttl
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof CreateBucketOptions) {
CreateBucketOptions that = (CreateBucketOptions) o;
return (this.location == null ? that.getLocation() == null : this.location.equals(that.getLocation()))
&& (this.storageClass == null ? that.getStorageClass() == null : this.storageClass.equals(that.getStorageClass()))
&& (this.ttl == null ? that.getTtl() == null : this.ttl.equals(that.getTtl()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (location == null) ? 0 : location.hashCode();
h$ *= 1000003;
h$ ^= (storageClass == null) ? 0 : storageClass.hashCode();
h$ *= 1000003;
h$ ^= (ttl == null) ? 0 : ttl.hashCode();
return h$;
}
@Override
public CreateBucketOptions.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends CreateBucketOptions.Builder {
private String location;
private String storageClass;
private Duration ttl;
Builder() {
}
private Builder(CreateBucketOptions source) {
this.location = source.getLocation();
this.storageClass = source.getStorageClass();
this.ttl = source.getTtl();
}
@Override
public CreateBucketOptions.Builder setLocation(String location) {
this.location = location;
return this;
}
@Override
public CreateBucketOptions.Builder setStorageClass(String storageClass) {
this.storageClass = storageClass;
return this;
}
@Override
public CreateBucketOptions.Builder setTtl(Duration ttl) {
this.ttl = ttl;
return this;
}
@Override
public CreateBucketOptions build() {
return new AutoValue_CreateBucketOptions(
this.location,
this.storageClass,
this.ttl);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy