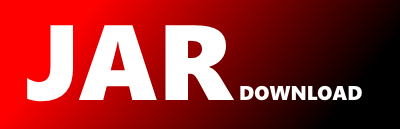
com.google.cloud.dataflow.sdk.transforms.Keys Maven / Gradle / Ivy
Show all versions of google-cloud-dataflow-java-sdk-all Show documentation
/*
* Copyright (C) 2015 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.cloud.dataflow.sdk.transforms;
import com.google.cloud.dataflow.sdk.values.KV;
import com.google.cloud.dataflow.sdk.values.PCollection;
/**
* {@code Keys} takes a {@code PCollection} of {@code KV}s and
* returns a {@code PCollection} of the keys.
*
* Example of use:
*
{@code
* PCollection> wordCounts = ...;
* PCollection words = wordCounts.apply(Keys.create());
* }
*
* Each output element has the same timestamp and is in the same windows
* as its corresponding input element, and the output {@code PCollection}
* has the same
* {@link com.google.cloud.dataflow.sdk.transforms.windowing.WindowFn}
* associated with it as the input.
*
*
See also {@link Values}.
*
* @param the type of the keys in the input {@code PCollection},
* and the type of the elements in the output {@code PCollection}
*/
public class Keys extends PTransform>,
PCollection> {
/**
* Returns a {@code Keys} {@code PTransform}.
*
* @param the type of the keys in the input {@code PCollection},
* and the type of the elements in the output {@code PCollection}
*/
public static Keys create() {
return new Keys<>();
}
private Keys() { }
@Override
public PCollection apply(PCollection extends KV> in) {
return
in.apply(ParDo.named("Keys")
.of(new DoFn, K>() {
@Override
public void processElement(ProcessContext c) {
c.output(c.element().getKey());
}
}));
}
}