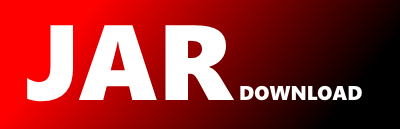
com.google.cloud.dataflow.sdk.transforms.Max Maven / Gradle / Ivy
Show all versions of google-cloud-dataflow-java-sdk-all Show documentation
/*
* Copyright (C) 2015 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.cloud.dataflow.sdk.transforms;
import com.google.cloud.dataflow.sdk.transforms.Combine.BinaryCombineFn;
import com.google.cloud.dataflow.sdk.util.common.Counter;
import com.google.cloud.dataflow.sdk.util.common.Counter.AggregationKind;
import com.google.cloud.dataflow.sdk.util.common.CounterProvider;
import java.io.Serializable;
import java.util.Comparator;
/**
* {@code PTransform}s for computing the maximum of the elements in a {@code PCollection}, or the
* maximum of the values associated with each key in a {@code PCollection} of {@code KV}s.
*
* Example 1: get the maximum of a {@code PCollection} of {@code Double}s.
*
{@code
* PCollection input = ...;
* PCollection max = input.apply(Max.doublesGlobally());
* }
*
* Example 2: calculate the maximum of the {@code Integer}s
* associated with each unique key (which is of type {@code String}).
*
{@code
* PCollection> input = ...;
* PCollection> maxPerKey = input
* .apply(Max.integersPerKey());
* }
*/
public class Max {
private Max() {
// do not instantiate
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection} and returns a
* {@code PCollection} whose contents is the maximum of the input {@code PCollection}'s
* elements, or {@code Integer.MIN_VALUE} if there are no elements.
*/
public static Combine.Globally integersGlobally() {
return Combine.globally(new MaxIntegerFn()).named("Max.Globally");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection>} and
* returns a {@code PCollection>} that contains an output element mapping each
* distinct key in the input {@code PCollection} to the maximum of the values associated with that
* key in the input {@code PCollection}.
*
* See {@link Combine.PerKey} for how this affects timestamps and windowing.
*/
public static Combine.PerKey integersPerKey() {
return Combine.perKey(new MaxIntegerFn()).named("Max.PerKey");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection} and returns a {@code
* PCollection} whose contents is the maximum of the input {@code PCollection}'s elements,
* or {@code Long.MIN_VALUE} if there are no elements.
*/
public static Combine.Globally longsGlobally() {
return Combine.globally(new MaxLongFn()).named("Max.Globally");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection>} and returns a
* {@code PCollection>} that contains an output element mapping each distinct key in
* the input {@code PCollection} to the maximum of the values associated with that key in the
* input {@code PCollection}.
*
* See {@link Combine.PerKey} for how this affects timestamps and windowing.
*/
public static Combine.PerKey longsPerKey() {
return Combine.perKey(new MaxLongFn()).named("Max.PerKey");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection} and returns a
* {@code PCollection} whose contents is the maximum of the input {@code PCollection}'s
* elements, or {@code Double.NEGATIVE_INFINITY} if there are no elements.
*/
public static Combine.Globally doublesGlobally() {
return Combine.globally(new MaxDoubleFn()).named("Max.Globally");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection>} and returns
* a {@code PCollection>} that contains an output element mapping each distinct key
* in the input {@code PCollection} to the maximum of the values associated with that key in the
* input {@code PCollection}.
*
* See {@link Combine.PerKey} for how this affects timestamps and windowing.
*/
public static Combine.PerKey doublesPerKey() {
return Combine.perKey(new MaxDoubleFn()).named("Max.PerKey");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection} and returns a {@code
* PCollection} whose contents is the maximum according to the natural ordering of {@code T}
* of the input {@code PCollection}'s elements, or {@code null} if there are no elements.
*/
public static >
Combine.Globally globally() {
return Combine.globally(MaxFn.naturalOrder()).named("Max.Globally");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection>} and returns a
* {@code PCollection>} that contains an output element mapping each distinct key in the
* input {@code PCollection} to the maximum according to the natural ordering of {@code T} of the
* values associated with that key in the input {@code PCollection}.
*
* See {@link Combine.PerKey} for how this affects timestamps and windowing.
*/
public static >
Combine.PerKey perKey() {
return Combine.perKey(MaxFn.naturalOrder()).named("Max.PerKey");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection} and returns a {@code
* PCollection} whose contents is the maximum of the input {@code PCollection}'s elements, or
* {@code null} if there are no elements.
*/
public static & Serializable>
Combine.Globally globally(ComparatorT comparator) {
return Combine.globally(MaxFn.of(comparator)).named("Max.Globally");
}
/**
* Returns a {@code PTransform} that takes an input {@code PCollection>} and returns a
* {@code PCollection>} that contains one output element per key mapping each
* to the maximum of the values associated with that key in the input {@code PCollection}.
*
* See {@link Combine.PerKey} for how this affects timestamps and windowing.
*/
public static & Serializable>
Combine.PerKey perKey(ComparatorT comparator) {
return Combine.perKey(MaxFn.of(comparator)).named("Max.PerKey");
}
/////////////////////////////////////////////////////////////////////////////
/**
* A {@code CombineFn} that computes the maximum of a collection of elements of type {@code T}
* using an arbitrary {@link Comparator}, useful as an argument to {@link Combine#globally} or
* {@link Combine#perKey}.
*
* @param the type of the values being compared
*/
public static class MaxFn extends BinaryCombineFn {
private final T identity;
private final Comparator super T> comparator;
private & Serializable> MaxFn(
T identity, ComparatorT comparator) {
this.identity = identity;
this.comparator = comparator;
}
public static & Serializable>
MaxFn of(T identity, ComparatorT comparator) {
return new MaxFn(identity, comparator);
}
public static & Serializable>
MaxFn of(ComparatorT comparator) {
return new MaxFn(null, comparator);
}
public static > MaxFn naturalOrder(T identity) {
return new MaxFn(identity, new Top.Largest());
}
public static > MaxFn naturalOrder() {
return new MaxFn(null, new Top.Largest());
}
@Override
public T identity() {
return identity;
}
@Override
public T apply(T left, T right) {
return comparator.compare(left, right) >= 0 ? left : right;
}
}
/**
* A {@code CombineFn} that computes the maximum of a collection of {@code Integer}s, useful as an
* argument to {@link Combine#globally} or {@link Combine#perKey}.
*/
public static class MaxIntegerFn extends MaxFn implements
CounterProvider {
public MaxIntegerFn() {
super(Integer.MIN_VALUE, new Top.Largest());
}
@Override
public Counter getCounter(String name) {
return Counter.ints(name, AggregationKind.MAX);
}
}
/**
* A {@code CombineFn} that computes the maximum of a collection of {@code Long}s, useful as an
* argument to {@link Combine#globally} or {@link Combine#perKey}.
*/
public static class MaxLongFn extends MaxFn implements
CounterProvider {
public MaxLongFn() {
super(Long.MIN_VALUE, new Top.Largest());
}
@Override
public Counter getCounter(String name) {
return Counter.longs(name, AggregationKind.MAX);
}
}
/**
* A {@code CombineFn} that computes the maximum of a collection of {@code Double}s, useful as an
* argument to {@link Combine#globally} or {@link Combine#perKey}.
*/
public static class MaxDoubleFn extends MaxFn implements
CounterProvider {
public MaxDoubleFn() {
super(Double.NEGATIVE_INFINITY, new Top.Largest());
}
@Override
public Counter getCounter(String name) {
return Counter.doubles(name, AggregationKind.MAX);
}
}
}