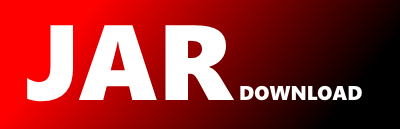
com.google.cloud.dataflow.sdk.values.TimestampedValue Maven / Gradle / Ivy
Show all versions of google-cloud-dataflow-java-sdk-all Show documentation
/*
* Copyright (C) 2015 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.cloud.dataflow.sdk.values;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import com.google.cloud.dataflow.sdk.coders.Coder;
import com.google.cloud.dataflow.sdk.coders.InstantCoder;
import com.google.cloud.dataflow.sdk.coders.StandardCoder;
import com.google.cloud.dataflow.sdk.util.PropertyNames;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.joda.time.Instant;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
/**
* An immutable pair of a value and a timestamp.
*
* The timestamp of a value determines many properties, such as its assignment to
* windows and whether the value is late (with respect to the watermark of a {@link PCollection}).
*
* @param the type of the value
*/
public class TimestampedValue {
/**
* Returns a new {@code TimestampedValue} with the given value and timestamp.
*/
public static TimestampedValue of(V value, Instant timestamp) {
return new TimestampedValue<>(value, timestamp);
}
public V getValue() {
return value;
}
public Instant getTimestamp() {
return timestamp;
}
@Override
public boolean equals(Object other) {
if (!(other instanceof TimestampedValue)) {
return false;
}
TimestampedValue> that = (TimestampedValue>) other;
return Objects.equals(value, that.value) && Objects.equals(timestamp, that.timestamp);
}
@Override
public int hashCode() {
return Objects.hash(value, timestamp);
}
@Override
public String toString() {
return "TimestampedValue(" + value + ", " + timestamp + ")";
}
/////////////////////////////////////////////////////////////////////////////
/**
* A {@link Coder} for {@link TimestampedValue}.
*/
public static class TimestampedValueCoder
extends StandardCoder> {
private final Coder valueCoder;
public static TimestampedValueCoder of(Coder valueCoder) {
return new TimestampedValueCoder<>(valueCoder);
}
@JsonCreator
public static TimestampedValueCoder> of(
@JsonProperty(PropertyNames.COMPONENT_ENCODINGS)
List