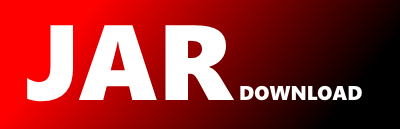
com.google.datastore.v1.CommitRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datastore-v1-protos Show documentation
Show all versions of datastore-v1-protos Show documentation
Protocol buffers for accessing the Google Cloud Datastore API.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/datastore/v1/datastore.proto
package com.google.datastore.v1;
/**
*
* The request for [Datastore.Commit][google.datastore.v1.Datastore.Commit].
*
*
* Protobuf type {@code google.datastore.v1.CommitRequest}
*/
public final class CommitRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.datastore.v1.CommitRequest)
CommitRequestOrBuilder {
// Use CommitRequest.newBuilder() to construct.
private CommitRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CommitRequest() {
projectId_ = "";
mode_ = 0;
mutations_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private CommitRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
transactionSelectorCase_ = 1;
transactionSelector_ = input.readBytes();
break;
}
case 40: {
int rawValue = input.readEnum();
mode_ = rawValue;
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
mutations_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
mutations_.add(
input.readMessage(com.google.datastore.v1.Mutation.parser(), extensionRegistry));
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
projectId_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
mutations_ = java.util.Collections.unmodifiableList(mutations_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.datastore.v1.DatastoreProto.internal_static_google_datastore_v1_CommitRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.datastore.v1.DatastoreProto.internal_static_google_datastore_v1_CommitRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.datastore.v1.CommitRequest.class, com.google.datastore.v1.CommitRequest.Builder.class);
}
/**
*
* The modes available for commits.
*
*
* Protobuf enum {@code google.datastore.v1.CommitRequest.Mode}
*/
public enum Mode
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Unspecified. This value must not be used.
*
*
* MODE_UNSPECIFIED = 0;
*/
MODE_UNSPECIFIED(0),
/**
*
* Transactional: The mutations are either all applied, or none are applied.
* Learn about transactions [here](https://cloud.google.com/datastore/docs/concepts/transactions).
*
*
* TRANSACTIONAL = 1;
*/
TRANSACTIONAL(1),
/**
*
* Non-transactional: The mutations may not apply as all or none.
*
*
* NON_TRANSACTIONAL = 2;
*/
NON_TRANSACTIONAL(2),
UNRECOGNIZED(-1),
;
/**
*
* Unspecified. This value must not be used.
*
*
* MODE_UNSPECIFIED = 0;
*/
public static final int MODE_UNSPECIFIED_VALUE = 0;
/**
*
* Transactional: The mutations are either all applied, or none are applied.
* Learn about transactions [here](https://cloud.google.com/datastore/docs/concepts/transactions).
*
*
* TRANSACTIONAL = 1;
*/
public static final int TRANSACTIONAL_VALUE = 1;
/**
*
* Non-transactional: The mutations may not apply as all or none.
*
*
* NON_TRANSACTIONAL = 2;
*/
public static final int NON_TRANSACTIONAL_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Mode valueOf(int value) {
return forNumber(value);
}
public static Mode forNumber(int value) {
switch (value) {
case 0: return MODE_UNSPECIFIED;
case 1: return TRANSACTIONAL;
case 2: return NON_TRANSACTIONAL;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Mode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Mode findValueByNumber(int number) {
return Mode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.datastore.v1.CommitRequest.getDescriptor().getEnumTypes().get(0);
}
private static final Mode[] VALUES = values();
public static Mode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Mode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.datastore.v1.CommitRequest.Mode)
}
private int bitField0_;
private int transactionSelectorCase_ = 0;
private java.lang.Object transactionSelector_;
public enum TransactionSelectorCase
implements com.google.protobuf.Internal.EnumLite {
TRANSACTION(1),
TRANSACTIONSELECTOR_NOT_SET(0);
private final int value;
private TransactionSelectorCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TransactionSelectorCase valueOf(int value) {
return forNumber(value);
}
public static TransactionSelectorCase forNumber(int value) {
switch (value) {
case 1: return TRANSACTION;
case 0: return TRANSACTIONSELECTOR_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public TransactionSelectorCase
getTransactionSelectorCase() {
return TransactionSelectorCase.forNumber(
transactionSelectorCase_);
}
public static final int PROJECT_ID_FIELD_NUMBER = 8;
private volatile java.lang.Object projectId_;
/**
*
* The ID of the project against which to make the request.
*
*
* optional string project_id = 8;
*/
public java.lang.String getProjectId() {
java.lang.Object ref = projectId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
projectId_ = s;
return s;
}
}
/**
*
* The ID of the project against which to make the request.
*
*
* optional string project_id = 8;
*/
public com.google.protobuf.ByteString
getProjectIdBytes() {
java.lang.Object ref = projectId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
projectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MODE_FIELD_NUMBER = 5;
private int mode_;
/**
*
* The type of commit to perform. Defaults to `TRANSACTIONAL`.
*
*
* optional .google.datastore.v1.CommitRequest.Mode mode = 5;
*/
public int getModeValue() {
return mode_;
}
/**
*
* The type of commit to perform. Defaults to `TRANSACTIONAL`.
*
*
* optional .google.datastore.v1.CommitRequest.Mode mode = 5;
*/
public com.google.datastore.v1.CommitRequest.Mode getMode() {
com.google.datastore.v1.CommitRequest.Mode result = com.google.datastore.v1.CommitRequest.Mode.valueOf(mode_);
return result == null ? com.google.datastore.v1.CommitRequest.Mode.UNRECOGNIZED : result;
}
public static final int TRANSACTION_FIELD_NUMBER = 1;
/**
*
* The identifier of the transaction associated with the commit. A
* transaction identifier is returned by a call to
* [Datastore.BeginTransaction][google.datastore.v1.Datastore.BeginTransaction].
*
*
* optional bytes transaction = 1;
*/
public com.google.protobuf.ByteString getTransaction() {
if (transactionSelectorCase_ == 1) {
return (com.google.protobuf.ByteString) transactionSelector_;
}
return com.google.protobuf.ByteString.EMPTY;
}
public static final int MUTATIONS_FIELD_NUMBER = 6;
private java.util.List mutations_;
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public java.util.List getMutationsList() {
return mutations_;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public java.util.List extends com.google.datastore.v1.MutationOrBuilder>
getMutationsOrBuilderList() {
return mutations_;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public int getMutationsCount() {
return mutations_.size();
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public com.google.datastore.v1.Mutation getMutations(int index) {
return mutations_.get(index);
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public com.google.datastore.v1.MutationOrBuilder getMutationsOrBuilder(
int index) {
return mutations_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (transactionSelectorCase_ == 1) {
output.writeBytes(
1, (com.google.protobuf.ByteString)((com.google.protobuf.ByteString) transactionSelector_));
}
if (mode_ != com.google.datastore.v1.CommitRequest.Mode.MODE_UNSPECIFIED.getNumber()) {
output.writeEnum(5, mode_);
}
for (int i = 0; i < mutations_.size(); i++) {
output.writeMessage(6, mutations_.get(i));
}
if (!getProjectIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, projectId_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (transactionSelectorCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(
1, (com.google.protobuf.ByteString)((com.google.protobuf.ByteString) transactionSelector_));
}
if (mode_ != com.google.datastore.v1.CommitRequest.Mode.MODE_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, mode_);
}
for (int i = 0; i < mutations_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, mutations_.get(i));
}
if (!getProjectIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, projectId_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.datastore.v1.CommitRequest)) {
return super.equals(obj);
}
com.google.datastore.v1.CommitRequest other = (com.google.datastore.v1.CommitRequest) obj;
boolean result = true;
result = result && getProjectId()
.equals(other.getProjectId());
result = result && mode_ == other.mode_;
result = result && getMutationsList()
.equals(other.getMutationsList());
result = result && getTransactionSelectorCase().equals(
other.getTransactionSelectorCase());
if (!result) return false;
switch (transactionSelectorCase_) {
case 1:
result = result && getTransaction()
.equals(other.getTransaction());
break;
case 0:
default:
}
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
hash = (37 * hash) + PROJECT_ID_FIELD_NUMBER;
hash = (53 * hash) + getProjectId().hashCode();
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + mode_;
if (getMutationsCount() > 0) {
hash = (37 * hash) + MUTATIONS_FIELD_NUMBER;
hash = (53 * hash) + getMutationsList().hashCode();
}
switch (transactionSelectorCase_) {
case 1:
hash = (37 * hash) + TRANSACTION_FIELD_NUMBER;
hash = (53 * hash) + getTransaction().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.datastore.v1.CommitRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.datastore.v1.CommitRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.datastore.v1.CommitRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.datastore.v1.CommitRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.datastore.v1.CommitRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.datastore.v1.CommitRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.datastore.v1.CommitRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.datastore.v1.CommitRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.datastore.v1.CommitRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.datastore.v1.CommitRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.datastore.v1.CommitRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The request for [Datastore.Commit][google.datastore.v1.Datastore.Commit].
*
*
* Protobuf type {@code google.datastore.v1.CommitRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.datastore.v1.CommitRequest)
com.google.datastore.v1.CommitRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.datastore.v1.DatastoreProto.internal_static_google_datastore_v1_CommitRequest_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.datastore.v1.DatastoreProto.internal_static_google_datastore_v1_CommitRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.datastore.v1.CommitRequest.class, com.google.datastore.v1.CommitRequest.Builder.class);
}
// Construct using com.google.datastore.v1.CommitRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMutationsFieldBuilder();
}
}
public Builder clear() {
super.clear();
projectId_ = "";
mode_ = 0;
if (mutationsBuilder_ == null) {
mutations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
mutationsBuilder_.clear();
}
transactionSelectorCase_ = 0;
transactionSelector_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.datastore.v1.DatastoreProto.internal_static_google_datastore_v1_CommitRequest_descriptor;
}
public com.google.datastore.v1.CommitRequest getDefaultInstanceForType() {
return com.google.datastore.v1.CommitRequest.getDefaultInstance();
}
public com.google.datastore.v1.CommitRequest build() {
com.google.datastore.v1.CommitRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.datastore.v1.CommitRequest buildPartial() {
com.google.datastore.v1.CommitRequest result = new com.google.datastore.v1.CommitRequest(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.projectId_ = projectId_;
result.mode_ = mode_;
if (transactionSelectorCase_ == 1) {
result.transactionSelector_ = transactionSelector_;
}
if (mutationsBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
mutations_ = java.util.Collections.unmodifiableList(mutations_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.mutations_ = mutations_;
} else {
result.mutations_ = mutationsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
result.transactionSelectorCase_ = transactionSelectorCase_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.datastore.v1.CommitRequest) {
return mergeFrom((com.google.datastore.v1.CommitRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.datastore.v1.CommitRequest other) {
if (other == com.google.datastore.v1.CommitRequest.getDefaultInstance()) return this;
if (!other.getProjectId().isEmpty()) {
projectId_ = other.projectId_;
onChanged();
}
if (other.mode_ != 0) {
setModeValue(other.getModeValue());
}
if (mutationsBuilder_ == null) {
if (!other.mutations_.isEmpty()) {
if (mutations_.isEmpty()) {
mutations_ = other.mutations_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureMutationsIsMutable();
mutations_.addAll(other.mutations_);
}
onChanged();
}
} else {
if (!other.mutations_.isEmpty()) {
if (mutationsBuilder_.isEmpty()) {
mutationsBuilder_.dispose();
mutationsBuilder_ = null;
mutations_ = other.mutations_;
bitField0_ = (bitField0_ & ~0x00000008);
mutationsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMutationsFieldBuilder() : null;
} else {
mutationsBuilder_.addAllMessages(other.mutations_);
}
}
}
switch (other.getTransactionSelectorCase()) {
case TRANSACTION: {
setTransaction(other.getTransaction());
break;
}
case TRANSACTIONSELECTOR_NOT_SET: {
break;
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.datastore.v1.CommitRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.datastore.v1.CommitRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int transactionSelectorCase_ = 0;
private java.lang.Object transactionSelector_;
public TransactionSelectorCase
getTransactionSelectorCase() {
return TransactionSelectorCase.forNumber(
transactionSelectorCase_);
}
public Builder clearTransactionSelector() {
transactionSelectorCase_ = 0;
transactionSelector_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object projectId_ = "";
/**
*
* The ID of the project against which to make the request.
*
*
* optional string project_id = 8;
*/
public java.lang.String getProjectId() {
java.lang.Object ref = projectId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
projectId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The ID of the project against which to make the request.
*
*
* optional string project_id = 8;
*/
public com.google.protobuf.ByteString
getProjectIdBytes() {
java.lang.Object ref = projectId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
projectId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The ID of the project against which to make the request.
*
*
* optional string project_id = 8;
*/
public Builder setProjectId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
projectId_ = value;
onChanged();
return this;
}
/**
*
* The ID of the project against which to make the request.
*
*
* optional string project_id = 8;
*/
public Builder clearProjectId() {
projectId_ = getDefaultInstance().getProjectId();
onChanged();
return this;
}
/**
*
* The ID of the project against which to make the request.
*
*
* optional string project_id = 8;
*/
public Builder setProjectIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
projectId_ = value;
onChanged();
return this;
}
private int mode_ = 0;
/**
*
* The type of commit to perform. Defaults to `TRANSACTIONAL`.
*
*
* optional .google.datastore.v1.CommitRequest.Mode mode = 5;
*/
public int getModeValue() {
return mode_;
}
/**
*
* The type of commit to perform. Defaults to `TRANSACTIONAL`.
*
*
* optional .google.datastore.v1.CommitRequest.Mode mode = 5;
*/
public Builder setModeValue(int value) {
mode_ = value;
onChanged();
return this;
}
/**
*
* The type of commit to perform. Defaults to `TRANSACTIONAL`.
*
*
* optional .google.datastore.v1.CommitRequest.Mode mode = 5;
*/
public com.google.datastore.v1.CommitRequest.Mode getMode() {
com.google.datastore.v1.CommitRequest.Mode result = com.google.datastore.v1.CommitRequest.Mode.valueOf(mode_);
return result == null ? com.google.datastore.v1.CommitRequest.Mode.UNRECOGNIZED : result;
}
/**
*
* The type of commit to perform. Defaults to `TRANSACTIONAL`.
*
*
* optional .google.datastore.v1.CommitRequest.Mode mode = 5;
*/
public Builder setMode(com.google.datastore.v1.CommitRequest.Mode value) {
if (value == null) {
throw new NullPointerException();
}
mode_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The type of commit to perform. Defaults to `TRANSACTIONAL`.
*
*
* optional .google.datastore.v1.CommitRequest.Mode mode = 5;
*/
public Builder clearMode() {
mode_ = 0;
onChanged();
return this;
}
/**
*
* The identifier of the transaction associated with the commit. A
* transaction identifier is returned by a call to
* [Datastore.BeginTransaction][google.datastore.v1.Datastore.BeginTransaction].
*
*
* optional bytes transaction = 1;
*/
public com.google.protobuf.ByteString getTransaction() {
if (transactionSelectorCase_ == 1) {
return (com.google.protobuf.ByteString) transactionSelector_;
}
return com.google.protobuf.ByteString.EMPTY;
}
/**
*
* The identifier of the transaction associated with the commit. A
* transaction identifier is returned by a call to
* [Datastore.BeginTransaction][google.datastore.v1.Datastore.BeginTransaction].
*
*
* optional bytes transaction = 1;
*/
public Builder setTransaction(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
transactionSelectorCase_ = 1;
transactionSelector_ = value;
onChanged();
return this;
}
/**
*
* The identifier of the transaction associated with the commit. A
* transaction identifier is returned by a call to
* [Datastore.BeginTransaction][google.datastore.v1.Datastore.BeginTransaction].
*
*
* optional bytes transaction = 1;
*/
public Builder clearTransaction() {
if (transactionSelectorCase_ == 1) {
transactionSelectorCase_ = 0;
transactionSelector_ = null;
onChanged();
}
return this;
}
private java.util.List mutations_ =
java.util.Collections.emptyList();
private void ensureMutationsIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
mutations_ = new java.util.ArrayList(mutations_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.datastore.v1.Mutation, com.google.datastore.v1.Mutation.Builder, com.google.datastore.v1.MutationOrBuilder> mutationsBuilder_;
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public java.util.List getMutationsList() {
if (mutationsBuilder_ == null) {
return java.util.Collections.unmodifiableList(mutations_);
} else {
return mutationsBuilder_.getMessageList();
}
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public int getMutationsCount() {
if (mutationsBuilder_ == null) {
return mutations_.size();
} else {
return mutationsBuilder_.getCount();
}
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public com.google.datastore.v1.Mutation getMutations(int index) {
if (mutationsBuilder_ == null) {
return mutations_.get(index);
} else {
return mutationsBuilder_.getMessage(index);
}
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder setMutations(
int index, com.google.datastore.v1.Mutation value) {
if (mutationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMutationsIsMutable();
mutations_.set(index, value);
onChanged();
} else {
mutationsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder setMutations(
int index, com.google.datastore.v1.Mutation.Builder builderForValue) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
mutations_.set(index, builderForValue.build());
onChanged();
} else {
mutationsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder addMutations(com.google.datastore.v1.Mutation value) {
if (mutationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMutationsIsMutable();
mutations_.add(value);
onChanged();
} else {
mutationsBuilder_.addMessage(value);
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder addMutations(
int index, com.google.datastore.v1.Mutation value) {
if (mutationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMutationsIsMutable();
mutations_.add(index, value);
onChanged();
} else {
mutationsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder addMutations(
com.google.datastore.v1.Mutation.Builder builderForValue) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
mutations_.add(builderForValue.build());
onChanged();
} else {
mutationsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder addMutations(
int index, com.google.datastore.v1.Mutation.Builder builderForValue) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
mutations_.add(index, builderForValue.build());
onChanged();
} else {
mutationsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder addAllMutations(
java.lang.Iterable extends com.google.datastore.v1.Mutation> values) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, mutations_);
onChanged();
} else {
mutationsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder clearMutations() {
if (mutationsBuilder_ == null) {
mutations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
mutationsBuilder_.clear();
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public Builder removeMutations(int index) {
if (mutationsBuilder_ == null) {
ensureMutationsIsMutable();
mutations_.remove(index);
onChanged();
} else {
mutationsBuilder_.remove(index);
}
return this;
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public com.google.datastore.v1.Mutation.Builder getMutationsBuilder(
int index) {
return getMutationsFieldBuilder().getBuilder(index);
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public com.google.datastore.v1.MutationOrBuilder getMutationsOrBuilder(
int index) {
if (mutationsBuilder_ == null) {
return mutations_.get(index); } else {
return mutationsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public java.util.List extends com.google.datastore.v1.MutationOrBuilder>
getMutationsOrBuilderList() {
if (mutationsBuilder_ != null) {
return mutationsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(mutations_);
}
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public com.google.datastore.v1.Mutation.Builder addMutationsBuilder() {
return getMutationsFieldBuilder().addBuilder(
com.google.datastore.v1.Mutation.getDefaultInstance());
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public com.google.datastore.v1.Mutation.Builder addMutationsBuilder(
int index) {
return getMutationsFieldBuilder().addBuilder(
index, com.google.datastore.v1.Mutation.getDefaultInstance());
}
/**
*
* The mutations to perform.
* When mode is `TRANSACTIONAL`, mutations affecting a single entity are
* applied in order. The following sequences of mutations affecting a single
* entity are not permitted in a single `Commit` request:
* - `insert` followed by `insert`
* - `update` followed by `insert`
* - `upsert` followed by `insert`
* - `delete` followed by `update`
* When mode is `NON_TRANSACTIONAL`, no two mutations may affect a single
* entity.
*
*
* repeated .google.datastore.v1.Mutation mutations = 6;
*/
public java.util.List
getMutationsBuilderList() {
return getMutationsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.datastore.v1.Mutation, com.google.datastore.v1.Mutation.Builder, com.google.datastore.v1.MutationOrBuilder>
getMutationsFieldBuilder() {
if (mutationsBuilder_ == null) {
mutationsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.datastore.v1.Mutation, com.google.datastore.v1.Mutation.Builder, com.google.datastore.v1.MutationOrBuilder>(
mutations_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
mutations_ = null;
}
return mutationsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:google.datastore.v1.CommitRequest)
}
// @@protoc_insertion_point(class_scope:google.datastore.v1.CommitRequest)
private static final com.google.datastore.v1.CommitRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.datastore.v1.CommitRequest();
}
public static com.google.datastore.v1.CommitRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CommitRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CommitRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.google.datastore.v1.CommitRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy