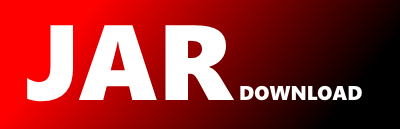
com.google.cloud.tools.maven.run.AbstractRunMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of appengine-maven-plugin Show documentation
Show all versions of appengine-maven-plugin Show documentation
This Maven plugin provides goals to build and deploy Google App Engine applications.
The newest version!
/*
* Copyright 2016 Google LLC. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.tools.maven.run;
import com.google.cloud.tools.maven.cloudsdk.CloudSdkMojo;
import java.io.File;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import org.apache.maven.model.Build;
import org.apache.maven.plugins.annotations.Parameter;
public abstract class AbstractRunMojo extends CloudSdkMojo {
/**
* Path to a yaml file, or a directory containing yaml files, or a directory containing WEB-INF/
* web.xml. Defaults to ${project.build.directory}/${project.build.finalName}
.
*/
@Parameter(alias = "devserver.services", property = "app.devserver.services", required = true)
private List services;
/** Host name to which application modules should bind. (default: localhost) */
@Parameter(alias = "devserver.host", property = "app.devserver.host")
private String host;
/** Lowest port to which application modules should bind. (default: 8080) */
@Parameter(alias = "devserver.port", property = "app.devserver.port")
private Integer port;
/**
* Additional arguments to pass to the java command when launching an instance of the app. May be
* specified more than once. Example: "-Xmx1024m -Xms256m" (default: None)
*/
@Parameter(alias = "devserver.jvmFlags", property = "app.devserver.jvmFlags")
private List jvmFlags;
/**
* Restart instances automatically when files relevant to their module are changed. (default:
* True)
*/
@Parameter(alias = "devserver.automaticRestart", property = "app.devserver.automaticRestart")
private Boolean automaticRestart;
/** Default Google Cloud Storage bucket name. (default: None) */
@Parameter(
alias = "devserver.defaultGcsBucketName",
property = "app.devserver.defaultGcsBucketName")
private String defaultGcsBucketName;
/** Environment variables passed to the devappserver process. */
@Parameter(alias = "devserver.environment", property = "app.devserver.environment")
private Map environment;
/** Environment variables passed to the devappserver process. */
@Parameter(
alias = "devserver.additionalArguments",
property = "app.devserver.additionalArguments")
private List additionalArguments;
/** The Google Cloud Platform project name to use for this invocation of the devserver. */
@Parameter(alias = "devserver.projectId", property = "app.devserver.projectId")
private String projectId;
/**
* Return a list of Paths to services to run. If none are specified by the user, the default
* application directory in the build output is used.
*/
public List getServices() {
if (services == null || services.isEmpty()) {
Build build = getMavenProject().getBuild();
return Collections.singletonList(
Paths.get(build.getDirectory()).resolve(build.getFinalName()));
}
return services.stream().map(File::toPath).collect(Collectors.toList());
}
public String getHost() {
return host;
}
public Integer getPort() {
return port;
}
public List getJvmFlags() {
return jvmFlags;
}
public Boolean getAutomaticRestart() {
return automaticRestart;
}
public String getDefaultGcsBucketName() {
return defaultGcsBucketName;
}
public Map getEnvironment() {
return environment;
}
public List getAdditionalArguments() {
return additionalArguments;
}
public String getProjectId() {
return projectId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy