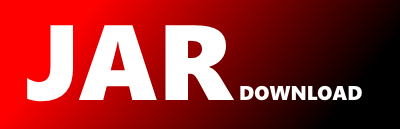
com.google.cloud.dns.spi.DnsRpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gcloud-java-dns Show documentation
Show all versions of gcloud-java-dns Show documentation
Java idiomatic client for Google Cloud DNS.
/*
* Copyright 2016 Google Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.dns.spi;
import com.google.api.services.dns.model.Change;
import com.google.api.services.dns.model.ManagedZone;
import com.google.api.services.dns.model.Project;
import com.google.api.services.dns.model.ResourceRecordSet;
import com.google.cloud.dns.DnsException;
import com.google.common.collect.ImmutableList;
import java.util.Map;
public interface DnsRpc {
enum Option {
FIELDS("fields"),
PAGE_SIZE("maxResults"),
PAGE_TOKEN("pageToken"),
DNS_NAME("dnsName"),
NAME("name"),
DNS_TYPE("type"),
SORTING_ORDER("sortOrder");
private final String value;
Option(String value) {
this.value = value;
}
public String value() {
return value;
}
@SuppressWarnings("unchecked")
T get(Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy