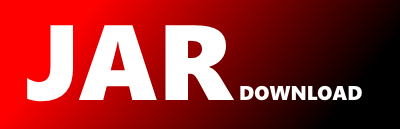
com.google.cloud.pubsub.spi.v1.PublisherSettings Maven / Gradle / Ivy
/*
* Copyright 2016 Google Inc. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.google.cloud.pubsub.spi.v1;
import com.google.api.gax.core.ConnectionSettings;
import com.google.api.gax.core.RetrySettings;
import com.google.api.gax.grpc.ApiCallSettings;
import com.google.api.gax.grpc.BundlingCallSettings;
import com.google.api.gax.grpc.BundlingDescriptor;
import com.google.api.gax.grpc.BundlingSettings;
import com.google.api.gax.grpc.PageStreamingCallSettings;
import com.google.api.gax.grpc.PageStreamingDescriptor;
import com.google.api.gax.grpc.RequestIssuer;
import com.google.api.gax.grpc.ServiceApiSettings;
import com.google.api.gax.grpc.SimpleCallSettings;
import com.google.auth.Credentials;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
import com.google.protobuf.Empty;
import com.google.pubsub.v1.DeleteTopicRequest;
import com.google.pubsub.v1.GetTopicRequest;
import com.google.pubsub.v1.ListTopicSubscriptionsRequest;
import com.google.pubsub.v1.ListTopicSubscriptionsResponse;
import com.google.pubsub.v1.ListTopicsRequest;
import com.google.pubsub.v1.ListTopicsResponse;
import com.google.pubsub.v1.PublishRequest;
import com.google.pubsub.v1.PublishResponse;
import com.google.pubsub.v1.PublisherGrpc;
import com.google.pubsub.v1.PubsubMessage;
import com.google.pubsub.v1.Topic;
import io.grpc.ManagedChannel;
import io.grpc.Status;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.ScheduledExecutorService;
import org.joda.time.Duration;
// AUTO-GENERATED DOCUMENTATION AND CLASS
/**
* Settings class to configure an instance of {@link PublisherApi}.
*
* The default instance has everything set to sensible defaults:
*
*
* - The default service address (pubsub.googleapis.com) and default port (443)
* are used.
*
- Credentials are acquired automatically through Application Default Credentials.
*
- Retries are configured for idempotent methods but not for non-idempotent methods.
*
*
* The builder of this class is recursive, so contained classes are themselves builders.
* When build() is called, the tree of builders is called to create the complete settings
* object. For example, to set the total timeout of createTopic to 30 seconds:
*
*
*
* PublisherSettings.Builder publisherSettingsBuilder =
* PublisherSettings.defaultBuilder();
* publisherSettingsBuilder.createTopicSettings().getRetrySettingsBuilder()
* .setTotalTimeout(Duration.standardSeconds(30));
* PublisherSettings publisherSettings = publisherSettingsBuilder.build();
*
*
*/
@javax.annotation.Generated("by GAPIC")
public class PublisherSettings extends ServiceApiSettings {
/**
* The default address of the service.
*/
private static final String DEFAULT_SERVICE_ADDRESS = "pubsub.googleapis.com";
/**
* The default port of the service.
*/
private static final int DEFAULT_SERVICE_PORT = 443;
/**
* The default scopes of the service.
*/
private static final ImmutableList DEFAULT_SERVICE_SCOPES =
ImmutableList.builder()
.add("https://www.googleapis.com/auth/cloud-platform")
.add("https://www.googleapis.com/auth/pubsub")
.build();
/**
* The default connection settings of the service.
*/
public static final ConnectionSettings DEFAULT_CONNECTION_SETTINGS =
ConnectionSettings.newBuilder()
.setServiceAddress(DEFAULT_SERVICE_ADDRESS)
.setPort(DEFAULT_SERVICE_PORT)
.provideCredentialsWith(DEFAULT_SERVICE_SCOPES)
.build();
private final SimpleCallSettings createTopicSettings;
private final BundlingCallSettings publishSettings;
private final SimpleCallSettings getTopicSettings;
private final PageStreamingCallSettings
listTopicsSettings;
private final PageStreamingCallSettings<
ListTopicSubscriptionsRequest, ListTopicSubscriptionsResponse, String>
listTopicSubscriptionsSettings;
private final SimpleCallSettings deleteTopicSettings;
/**
* Returns the object with the settings used for calls to createTopic.
*/
public SimpleCallSettings createTopicSettings() {
return createTopicSettings;
}
/**
* Returns the object with the settings used for calls to publish.
*/
public BundlingCallSettings publishSettings() {
return publishSettings;
}
/**
* Returns the object with the settings used for calls to getTopic.
*/
public SimpleCallSettings getTopicSettings() {
return getTopicSettings;
}
/**
* Returns the object with the settings used for calls to listTopics.
*/
public PageStreamingCallSettings
listTopicsSettings() {
return listTopicsSettings;
}
/**
* Returns the object with the settings used for calls to listTopicSubscriptions.
*/
public PageStreamingCallSettings<
ListTopicSubscriptionsRequest, ListTopicSubscriptionsResponse, String>
listTopicSubscriptionsSettings() {
return listTopicSubscriptionsSettings;
}
/**
* Returns the object with the settings used for calls to deleteTopic.
*/
public SimpleCallSettings deleteTopicSettings() {
return deleteTopicSettings;
}
/**
* Returns the default service address.
*/
public static String getDefaultServiceAddress() {
return DEFAULT_SERVICE_ADDRESS;
}
/**
* Returns the default service port.
*/
public static int getDefaultServicePort() {
return DEFAULT_SERVICE_PORT;
}
/**
* Returns the default service scopes.
*/
public static ImmutableList getDefaultServiceScopes() {
return DEFAULT_SERVICE_SCOPES;
}
/**
* Returns a builder for this class with recommended defaults.
*/
public static Builder defaultBuilder() {
return Builder.createDefault();
}
/**
* Returns a new builder for this class.
*/
public static Builder newBuilder() {
return new Builder();
}
/**
* Returns a builder containing all the values of this settings class.
*/
public Builder toBuilder() {
return new Builder(this);
}
private PublisherSettings(Builder settingsBuilder) throws IOException {
super(
settingsBuilder.getChannelProvider(),
settingsBuilder.getExecutorProvider(),
settingsBuilder.getGeneratorName(),
settingsBuilder.getGeneratorVersion(),
settingsBuilder.getClientLibName(),
settingsBuilder.getClientLibVersion());
createTopicSettings = settingsBuilder.createTopicSettings().build();
publishSettings = settingsBuilder.publishSettings().build();
getTopicSettings = settingsBuilder.getTopicSettings().build();
listTopicsSettings = settingsBuilder.listTopicsSettings().build();
listTopicSubscriptionsSettings = settingsBuilder.listTopicSubscriptionsSettings().build();
deleteTopicSettings = settingsBuilder.deleteTopicSettings().build();
}
private static PageStreamingDescriptor
LIST_TOPICS_PAGE_STR_DESC =
new PageStreamingDescriptor() {
@Override
public Object emptyToken() {
return "";
}
@Override
public ListTopicsRequest injectToken(ListTopicsRequest payload, Object token) {
return ListTopicsRequest.newBuilder(payload).setPageToken((String) token).build();
}
@Override
public Object extractNextToken(ListTopicsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListTopicsResponse payload) {
return payload.getTopicsList();
}
};
private static PageStreamingDescriptor<
ListTopicSubscriptionsRequest, ListTopicSubscriptionsResponse, String>
LIST_TOPIC_SUBSCRIPTIONS_PAGE_STR_DESC =
new PageStreamingDescriptor<
ListTopicSubscriptionsRequest, ListTopicSubscriptionsResponse, String>() {
@Override
public Object emptyToken() {
return "";
}
@Override
public ListTopicSubscriptionsRequest injectToken(
ListTopicSubscriptionsRequest payload, Object token) {
return ListTopicSubscriptionsRequest.newBuilder(payload)
.setPageToken((String) token)
.build();
}
@Override
public Object extractNextToken(ListTopicSubscriptionsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListTopicSubscriptionsResponse payload) {
return payload.getSubscriptionsList();
}
};
private static BundlingDescriptor PUBLISH_BUNDLING_DESC =
new BundlingDescriptor() {
@Override
public String getBundlePartitionKey(PublishRequest request) {
return request.getTopic() + "|";
}
@Override
public PublishRequest mergeRequests(Collection requests) {
PublishRequest firstRequest = requests.iterator().next();
List elements = new ArrayList<>();
for (PublishRequest request : requests) {
elements.addAll(request.getMessagesList());
}
PublishRequest bundleRequest =
PublishRequest.newBuilder()
.setTopic(firstRequest.getTopic())
.addAllMessages(elements)
.build();
return bundleRequest;
}
@Override
public void splitResponse(
PublishResponse bundleResponse,
Collection extends RequestIssuer> bundle) {
int bundleMessageIndex = 0;
for (RequestIssuer responder : bundle) {
List subresponseElements = new ArrayList<>();
int subresponseCount = responder.getRequest().getMessagesCount();
for (int i = 0; i < subresponseCount; i++) {
subresponseElements.add(bundleResponse.getMessageIds(bundleMessageIndex));
bundleMessageIndex += 1;
}
PublishResponse response =
PublishResponse.newBuilder().addAllMessageIds(subresponseElements).build();
responder.setResponse(response);
}
}
@Override
public void splitException(
Throwable throwable,
Collection extends RequestIssuer> bundle) {
for (RequestIssuer responder : bundle) {
responder.setException(throwable);
}
}
@Override
public long countElements(PublishRequest request) {
return request.getMessagesCount();
}
@Override
public long countBytes(PublishRequest request) {
return request.getSerializedSize();
}
};
/**
* Builder for PublisherSettings.
*/
public static class Builder extends ServiceApiSettings.Builder {
private final ImmutableList methodSettingsBuilders;
private SimpleCallSettings.Builder createTopicSettings;
private BundlingCallSettings.Builder publishSettings;
private SimpleCallSettings.Builder getTopicSettings;
private PageStreamingCallSettings.Builder
listTopicsSettings;
private PageStreamingCallSettings.Builder<
ListTopicSubscriptionsRequest, ListTopicSubscriptionsResponse, String>
listTopicSubscriptionsSettings;
private SimpleCallSettings.Builder deleteTopicSettings;
private static final ImmutableMap> RETRYABLE_CODE_DEFINITIONS;
static {
ImmutableMap.Builder> definitions = ImmutableMap.builder();
definitions.put(
"idempotent",
Sets.immutableEnumSet(
Lists.newArrayList(
Status.Code.DEADLINE_EXCEEDED, Status.Code.UNAVAILABLE)));
definitions.put(
"one_plus_delivery",
Sets.immutableEnumSet(
Lists.newArrayList(
Status.Code.DEADLINE_EXCEEDED, Status.Code.UNAVAILABLE)));
definitions.put("non_idempotent", Sets.immutableEnumSet(Lists.newArrayList()));
RETRYABLE_CODE_DEFINITIONS = definitions.build();
}
private static final ImmutableMap RETRY_PARAM_DEFINITIONS;
static {
ImmutableMap.Builder definitions = ImmutableMap.builder();
RetrySettings.Builder settingsBuilder = null;
settingsBuilder =
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.millis(100L))
.setRetryDelayMultiplier(1.3)
.setMaxRetryDelay(Duration.millis(60000L))
.setInitialRpcTimeout(Duration.millis(60000L))
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.millis(60000L))
.setTotalTimeout(Duration.millis(600000L));
definitions.put("default", settingsBuilder);
settingsBuilder =
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.millis(100L))
.setRetryDelayMultiplier(1.3)
.setMaxRetryDelay(Duration.millis(60000L))
.setInitialRpcTimeout(Duration.millis(12000L))
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.millis(12000L))
.setTotalTimeout(Duration.millis(600000L));
definitions.put("messaging", settingsBuilder);
RETRY_PARAM_DEFINITIONS = definitions.build();
}
private Builder() {
super(DEFAULT_CONNECTION_SETTINGS);
createTopicSettings = SimpleCallSettings.newBuilder(PublisherGrpc.METHOD_CREATE_TOPIC);
publishSettings =
BundlingCallSettings.newBuilder(PublisherGrpc.METHOD_PUBLISH, PUBLISH_BUNDLING_DESC)
.setBundlingSettingsBuilder(BundlingSettings.newBuilder());
getTopicSettings = SimpleCallSettings.newBuilder(PublisherGrpc.METHOD_GET_TOPIC);
listTopicsSettings =
PageStreamingCallSettings.newBuilder(
PublisherGrpc.METHOD_LIST_TOPICS, LIST_TOPICS_PAGE_STR_DESC);
listTopicSubscriptionsSettings =
PageStreamingCallSettings.newBuilder(
PublisherGrpc.METHOD_LIST_TOPIC_SUBSCRIPTIONS,
LIST_TOPIC_SUBSCRIPTIONS_PAGE_STR_DESC);
deleteTopicSettings = SimpleCallSettings.newBuilder(PublisherGrpc.METHOD_DELETE_TOPIC);
methodSettingsBuilders =
ImmutableList.of(
createTopicSettings,
publishSettings,
getTopicSettings,
listTopicsSettings,
listTopicSubscriptionsSettings,
deleteTopicSettings);
}
private static Builder createDefault() {
Builder builder = new Builder();
builder
.createTopicSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("idempotent"))
.setRetrySettingsBuilder(RETRY_PARAM_DEFINITIONS.get("default"));
builder
.publishSettings()
.getBundlingSettingsBuilder()
.setElementCountThreshold(10)
.setElementCountLimit(1000)
.setRequestByteThreshold(1024)
.setRequestByteLimit(10485760)
.setDelayThreshold(Duration.millis(10))
.setBlockingCallCountThreshold(1);
builder
.publishSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("one_plus_delivery"))
.setRetrySettingsBuilder(RETRY_PARAM_DEFINITIONS.get("messaging"));
builder
.getTopicSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("idempotent"))
.setRetrySettingsBuilder(RETRY_PARAM_DEFINITIONS.get("default"));
builder
.listTopicsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("idempotent"))
.setRetrySettingsBuilder(RETRY_PARAM_DEFINITIONS.get("default"));
builder
.listTopicSubscriptionsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("idempotent"))
.setRetrySettingsBuilder(RETRY_PARAM_DEFINITIONS.get("default"));
builder
.deleteTopicSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("idempotent"))
.setRetrySettingsBuilder(RETRY_PARAM_DEFINITIONS.get("default"));
return builder;
}
private Builder(PublisherSettings settings) {
super(settings);
createTopicSettings = settings.createTopicSettings.toBuilder();
publishSettings = settings.publishSettings.toBuilder();
getTopicSettings = settings.getTopicSettings.toBuilder();
listTopicsSettings = settings.listTopicsSettings.toBuilder();
listTopicSubscriptionsSettings = settings.listTopicSubscriptionsSettings.toBuilder();
deleteTopicSettings = settings.deleteTopicSettings.toBuilder();
methodSettingsBuilders =
ImmutableList.of(
createTopicSettings,
publishSettings,
getTopicSettings,
listTopicsSettings,
listTopicSubscriptionsSettings,
deleteTopicSettings);
}
@Override
protected ConnectionSettings getDefaultConnectionSettings() {
return DEFAULT_CONNECTION_SETTINGS;
}
@Override
public Builder provideExecutorWith(ScheduledExecutorService executor, boolean shouldAutoClose) {
super.provideExecutorWith(executor, shouldAutoClose);
return this;
}
@Override
public Builder provideChannelWith(ManagedChannel channel, boolean shouldAutoClose) {
super.provideChannelWith(channel, shouldAutoClose);
return this;
}
@Override
public Builder provideChannelWith(ConnectionSettings settings) {
super.provideChannelWith(settings);
return this;
}
@Override
public Builder provideChannelWith(Credentials credentials) {
super.provideChannelWith(credentials);
return this;
}
@Override
public Builder provideChannelWith(List scopes) {
super.provideChannelWith(scopes);
return this;
}
@Override
public Builder setGeneratorHeader(String name, String version) {
super.setGeneratorHeader(name, version);
return this;
}
@Override
public Builder setClientLibHeader(String name, String version) {
super.setClientLibHeader(name, version);
return this;
}
/**
* Applies the given settings to all of the API methods in this service. Only
* values that are non-null will be applied, so this method is not capable
* of un-setting any values.
*/
public Builder applyToAllApiMethods(ApiCallSettings.Builder apiCallSettings) throws Exception {
super.applyToAllApiMethods(methodSettingsBuilders, apiCallSettings);
return this;
}
/**
* Returns the builder for the settings used for calls to createTopic.
*/
public SimpleCallSettings.Builder createTopicSettings() {
return createTopicSettings;
}
/**
* Returns the builder for the settings used for calls to publish.
*/
public BundlingCallSettings.Builder publishSettings() {
return publishSettings;
}
/**
* Returns the builder for the settings used for calls to getTopic.
*/
public SimpleCallSettings.Builder getTopicSettings() {
return getTopicSettings;
}
/**
* Returns the builder for the settings used for calls to listTopics.
*/
public PageStreamingCallSettings.Builder
listTopicsSettings() {
return listTopicsSettings;
}
/**
* Returns the builder for the settings used for calls to listTopicSubscriptions.
*/
public PageStreamingCallSettings.Builder<
ListTopicSubscriptionsRequest, ListTopicSubscriptionsResponse, String>
listTopicSubscriptionsSettings() {
return listTopicSubscriptionsSettings;
}
/**
* Returns the builder for the settings used for calls to deleteTopic.
*/
public SimpleCallSettings.Builder deleteTopicSettings() {
return deleteTopicSettings;
}
@Override
public PublisherSettings build() throws IOException {
return new PublisherSettings(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy